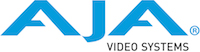 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
23 #define TCFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
24 #define TCWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
25 #define TCNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
26 #define TCINFO(_expr_) AJA_sINFO (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
27 #define TCDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
49 static const double gFrequencies [] = {250.0, 500.0, 1000.0, 2000.0};
63 mToneFrequency (440.0),
89 while (mProducerThread.
Active())
92 while (mConsumerThread.
Active())
95 #if defined(NTV2_BUFFER_LOCKING)
97 #endif // NTV2_BUFFER_LOCKING
118 if (!mDevice.
features().CanDoPlayback())
121 const UWord maxNumChannels (mDevice.
features().GetNumFrameStores());
130 cerr <<
"## ERROR: Cannot use channel '" <<
DEC(mConfig.
fOutputChannel+1) <<
"' -- device only supports channel 1"
131 << (maxNumChannels > 1 ? string(
" thru ") + string(1,
char(maxNumChannels+
'0')) :
"") << endl;
134 if (mDevice.
features().CanDo12gRouting())
137 cerr <<
"## WARNING: '--tsi' option used with device that has 12G FrameStores with integral Tsi muxers" << endl;
150 cerr <<
"## ERROR: Squares requires Ch1 or Ch5, not Ch" <<
DEC(mConfig.
fOutputChannel) << endl;
162 if (mDevice.
features().CanDoMultiFormat())
189 {cerr <<
"## ERROR: RenderTimeCodeFont failed for: " << mFormatDesc << endl;
return AJA_STATUS_UNSUPPORTED;}
194 if (mDevice.IsRemote())
195 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl;
197 #endif // defined(_DEBUG)
209 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
214 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
222 if (mDevice.
features().CanDo12gRouting())
226 if (channels1357.find(mConfig.
fOutputChannel) == channels1357.end())
264 {cerr <<
"## WARNING: HDR Anc requested, but device can't do custom anc" << endl;
282 uint16_t numAudioChannels (mDevice.
features().GetMaxAudioChannels());
287 numAudioChannels = 8;
293 switch (mAudioSystem)
299 for (
UWord chan(0); chan < numChan; chan++)
314 if (!mDevice.
features().CanDo12gRouting())
362 if (mConfig.WithAudio())
365 PLFAIL(
"Failed to allocate " <<
xHEX0N(audioBufferSize,8) <<
"-byte audio buffer");
371 #ifdef NTV2_BUFFER_LOCKING
375 mFrameDataRing.
Add (&frameData);
385 vector<NTV2TestPatternSelect> testPatIDs;
403 mTestPatRasters.clear();
404 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
409 if (mFormatDesc.IsVANC())
413 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
417 {
PLFAIL(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": "
424 if (!testPatternGen.
DrawTestPattern (testPatIDs.at(tpNdx), mFormatDesc, mTestPatRasters.at(tpNdx)))
426 cerr <<
"## ERROR: DrawTestPattern " <<
DEC(tpNdx) <<
" failed: " << mFormatDesc << endl;
430 #ifdef NTV2_BUFFER_LOCKING
433 PLWARN(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": failed to pre-lock");
445 bool useLinkGrouping =
false;
461 if (mDevice.
features().CanDo12GSDI() && !mDevice.
features().CanDo12gRouting())
466 useLinkGrouping =
true;
587 if (mDevice.
features().CanDo12gRouting())
597 return failures == 0;
604 if (mDevice.
features().HasBiDirectionalSDI())
615 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
616 UWord connectFailures (0);
665 return connectFailures == 0;
673 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
674 UWord connectFailures (0);
682 if (mDevice.
features().CanDo12gRouting())
787 return connectFailures == 0;
794 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
795 UWord connectFailures (0);
810 return connectFailures == 0;
816 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
817 UWord connectFailures (0);
852 return connectFailures == 0;
858 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
859 UWord connectFailures (0);
860 if (mDevice.
features().CanDo12gRouting())
884 return connectFailures == 0;
890 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
891 UWord connectFailures (0);
943 return connectFailures == 0;
949 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
950 UWord connectFailures (0);
973 return connectFailures == 0;
979 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
980 UWord connectFailures (0);
995 return connectFailures == 0;
1001 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
1002 UWord connectFailures (0);
1017 return connectFailures == 0;
1023 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
1024 UWord connectFailures (0);
1039 return connectFailures == 0;
1045 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
1046 UWord connectFailures (0);
1068 return connectFailures == 0;
1074 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
1075 UWord connectFailures (0);
1127 return connectFailures == 0;
1133 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
1134 UWord connectFailures (0);
1156 return connectFailures == 0;
1162 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
1163 UWord connectFailures (0);
1185 return connectFailures == 0;
1208 mConsumerThread.
Start();
1231 ULWord goodXfers(0), badXfers(0), prodWaits(0), noRoomWaits(0);
1232 const UWord numACFramesPerChannel(7);
1237 PLNOTE(
"Thread started");
1255 uint32_t hdrPktSize (0);
1260 PLWARN(
"HDR anc insertion disabled -- GenerateTransmitData failed");
1266 #ifdef NTV2_BUFFER_LOCKING
1272 UWord startNum(0), endNum(0);
1273 if (mDevice.
features().CanDo12gRouting())
1287 case NTV2_CHANNEL2: startNum = numACFramesPerChannel * 0;
break;
1292 case NTV2_CHANNEL4: startNum = numACFramesPerChannel * 1;
break;
1295 case NTV2_CHANNEL6: startNum = numACFramesPerChannel * 2;
break;
1298 case NTV2_CHANNEL8: startNum = numACFramesPerChannel * 3;
break;
1300 endNum = startNum + numACFramesPerChannel - 1;
1309 1 , startNum, endNum);
1311 {
PLFAIL(
"AutoCirculateInitForOutput failed"); mGlobalQuit =
true;}
1313 while (!mGlobalQuit)
1324 {prodWaits++;
continue;}
1330 const CRP188 rp188Info (mCurrentFrame++, 0, 0, 10, tcFormat);
1332 string timeCodeString;
1365 PLNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
1366 <<
DEC(prodWaits) <<
" starves, " <<
DEC(noRoomWaits) <<
" VBI waits");
1380 mProducerThread.
Start();
1397 ULWord freqNdx(0), testPatNdx(0), badTally(0);
1398 double timeOfLastSwitch (0.0);
1403 PLNOTE(
"Thread started");
1404 while (!mGlobalQuit)
1417 NTV2Buffer & testPatVidBuffer(mTestPatRasters.at(testPatNdx));
1426 if (currentTime > timeOfLastSwitch + 4.0)
1429 testPatNdx = (testPatNdx + 1) %
ULWord(mTestPatRasters.size());
1431 timeOfLastSwitch = currentTime;
1432 PLINFO(
"F" <<
DEC0N(mCurrentFrame,6) <<
": tone=" << mToneFrequency <<
"Hz, pattern='" << tpNames.at(testPatNdx) <<
"'");
1439 PLNOTE(
"Thread completed: " <<
DEC(mCurrentFrame) <<
" frame(s) produced, " <<
DEC(badTally) <<
" failed");
1461 ULWord bytesWritten(0), startSample(mCurrentSample);
1464 mCurrentSample = startSample;
1475 return bytesWritten;
@ NTV2_XptFrameBuffer6YUV
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
@ NTV2_XptFrameBuffer4YUV
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
@ NTV2_XptDualLinkOut7Input
virtual bool RouteDLOutToSDIOut(void)
Sets up board routing from the Dual Link outputs to the SDI outputs.
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
@ NTV2_XptDuallinkOut8DS2
bool fDoHDMIOutput
If true, enable HDMI output; otherwise, disable HDMI output.
virtual AJAStatus SetUpAudio(void)
Performs all audio setup.
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
virtual bool GetAncRegionOffsetFromBottom(ULWord &outByteOffsetFromBottom, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All)
Answers with the byte offset to the start of an ancillary data region within a device frame buffer,...
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
@ NTV2_TestPatt_CheckField
Declares the AJAAncillaryData_HDR_HLG class.
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
@ NTV2_XptFrameBuffer1_DS2YUV
#define NTV2_IS_VALID_TASK_MODE(__m__)
@ NTV2_XptSDIOut4InputDS2
virtual bool RouteCscTo4xSDIOut(void)
Sets up board routing from the Color Space Converters to the 4xSDI outputs.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
virtual bool SetHDMIOutVideoStandard(const NTV2Standard inNewValue)
UWord firstFrame(void) const
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
@ NTV2_XptFrameBuffer2RGB
virtual bool Set4kSquaresEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 "2K quadrants" mode for the given FrameStore bank on the device....
@ NTV2_TestPatt_MultiBurst
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
@ NTV2_XptSDIOut3InputDS2
#define IS_KNOWN_AJAAncDataType(_x_)
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
bool GetRP188Reg(RP188_STRUCT &outRP188) const
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
@ NTV2_XptSDIOut5InputDS2
ULWord GetByteCount(void) const
@ NTV2_AUDIOSYSTEM_4
This identifies the 4th Audio System.
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
@ NTV2_XptFrameBuffer3_DS2YUV
virtual bool RouteFsToSDIOut(void)
Sets up board routing from the Frame Stores to the SDI outputs.
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
@ NTV2_XptDuallinkOut7DS2
@ NTV2_Wgt4KDownConverter
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
@ NTV2_XptFrameBuffer8YUV
@ NTV2_XptFrameBuffer4_DS2YUV
@ NTV2_XptFrameBuffer3YUV
static const double gFrequencies[]
virtual bool SetHDMIOutVideoFPS(const NTV2FrameRate inNewValue)
bool Deallocate(void)
Deallocates my user-space storage (if I own it – i.e. from a prior call to Allocate).
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
virtual bool SetSDIOut6GEnable(const NTV2Channel inChannel, const bool inEnable)
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
Declares the NTV2TestPatternGen class.
NTV2Buffer acAudioBuffer
The host audio buffer. This field is owned by the client application, and thus is responsible for all...
@ NTV2_TestPatt_ColorBars100
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool RouteTsiMuxTo4xSDIOut(void)
Sets up board routing from the Two Sample Interleave muxes to the 4xSDI outputs.
#define DEC0N(__x__, __n__)
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
@ NTV2_AncRgn_Field2
Identifies the "normal" Field 2 ancillary data region.
virtual void StartProducerThread(void)
Starts my producer thread.
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO3
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 3.
static const uint32_t gAudMaxSizeBytes(256 *1024)
The maximum number of bytes of 48KHz audio that can be transferred for a single frame....
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
@ NTV2_XptFrameBuffer1RGB
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
@ NTV2_XptDuallinkOut6DS2
virtual bool RouteTsiMuxToDLOut(void)
Sets up board routing from the Two Sample Interleave muxes to the Dual Link outputs.
@ NTV2_XptSDIOut2InputDS2
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
virtual AJAStatus SetUpHostBuffers(void)
Sets up my host video & audio buffers.
@ NTV2_XptSDIOut8InputDS2
@ NTV2_XptDualLinkOut5Input
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
@ NTV2_XptFrameBuffer6_DS2RGB
bool SetOutputTimeCode(const NTV2_RP188 &inTimecode, const NTV2TCIndex inTCIndex=NTV2_TCINDEX_SDI1)
Intended for playout, sets one element of my acOutputTimeCodes member.
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
const char * NTV2FrameBufferFormatString(NTV2FrameBufferFormat fmt)
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
bool CanAcceptMoreOutputFrames(void) const
@ NTV2_XptFrameBuffer3RGB
bool fDoLinkGrouping
If true, enables 6/12G output mode on IoX3/Kona5 (4K/8K)
virtual bool RouteCscToDLOut(void)
Sets up board routing from the Color Space Converters to the Dual Link outputs.
NTV2FrameRate
Identifies a particular video frame rate.
This class handles "5251" Frame Status Information packets.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=(0), bool inRDMA=(0))
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
@ NTV2_XptFrameBuffer2_DS2YUV
bool fDoTsiRouting
If true, enable TSI routing; otherwise route for square division (4K/8K)
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO1
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 1.
AJAAncDataType fTransmitHDRType
Specifies the HDR anc data packet to transmit, if any.
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
NTV2AudioSystemSet NTV2MakeAudioSystemSet(const NTV2AudioSystem inFirstAudioSystem, const UWord inCount=1)
virtual class DeviceCapabilities & features(void)
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
virtual bool Enable4KDCRGBMode(bool inEnable)
Sets 4K Down Convert RGB mode.
I am an object that can play out a 4K or UHD test pattern (with timecode) to an output of an AJA devi...
virtual bool DMABufferUnlockAll()
Unlocks all previously-locked buffers used for DMA transfers.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
@ NTV2_XptFrameBuffer4_DS2RGB
@ NTV2_XptFrameBuffer1_DS2RGB
virtual void Quit(void)
Gracefully stops me from running.
@ NTV2_XptFrameBuffer2_DS2RGB
Declares the AJAProcess class.
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
@ NTV2_HDMI_V2_4K_PLAYBACK
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
virtual void ProduceFrames(void)
My producer thread that repeatedly produces video frames.
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the producer thread's static callback function that gets called when the producer thread star...
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual bool GetNumberAudioChannels(ULWord &outNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current number of audio channels being captured or played by a given Audio System on the ...
@ NTV2_TestPatt_FlatField
virtual bool EnableChannels(const NTV2ChannelSet &inChannels, const bool inDisableOthers=(0))
Enables the given FrameStore(s).
virtual std::string GetDescription(void) const
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
@ NTV2_XptFrameBuffer1YUV
@ NTV2_XptDuallinkOut2DS2
@ NTV2_XptDuallinkOut1DS2
static size_t SetDefaultPageSize(void)
@ NTV2_XptDuallinkOut4DS2
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC=false, const bool inIsF2=false)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
std::set< NTV2AudioSystem > NTV2AudioSystemSet
A set of distinct NTV2AudioSystem values. New in SDK 16.2.
virtual bool SetSDIOutRGBLevelAConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables an RGB-over-3G-level-A conversion at the SDI output widget (assuming the AJA devi...
UWord fNumAudioLinks
The number of audio systems to control for multi-link audio (4K/8K)
@ NTV2_TestPatt_ColorQuadrant
virtual bool RouteTsiMuxToCsc(void)
Sets up board routing from the Two Sample Interleave muxes to the color Space Converters.
@ NTV2_TestPatt_ColorQuadrantBorder
virtual bool RouteTsiMuxTo2xSDIOut(void)
Sets up board routing from the Two Sample Interleave muxes to the 2xSDI outputs.
virtual bool RouteOutputSignal(void)
Performs all widget/signal routing for playout.
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
NTV2VideoFormat fVideoFormat
The video format to use.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
#define NTV2_IS_4K_HFR_VIDEO_FORMAT(__f__)
static const bool BUFFER_PAGE_ALIGNED((!(0)))
@ NTV2_XptFrameBuffer6_DS2YUV
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
@ NTV2_XptDualLinkOut4Input
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
NTV2Channel fOutputChannel
The device channel to use.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
virtual void StartConsumerThread(void)
Starts my consumer thread.
This class handles "5251" Frame Status Information packets.
NTV2Buffer & AudioBuffer(void)
@ NTV2_XptFrameBuffer5_DS2RGB
@ NTV2_TestPatt_SlantRamp
@ NTV2_XptSDIOut1InputDS2
@ NTV2_TestPatt_ColorBars75
virtual bool IsDeviceReady(const bool inCheckValid=(0))
@ NTV2_TestPatt_ZonePlate
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
virtual bool SetTsiFrameEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 two-sample interleave (Tsi) frame mode on the device.
virtual AJAStatus Run(void)
Runs me.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual uint32_t AddTone(ULWord *audioBuffer)
Inserts audio tone (based on my current tone frequency) into the given audio buffer.
virtual bool SetHDMIOutAudioRate(const NTV2AudioRate inNewValue)
Sets the HDMI output's audio rate.
This class handles "5251" Frame Status Information packets.
bool AddAudioTone(ULWord &outNumBytesWritten, NTV2Buffer &inAudioBuffer, ULWord &inOutCurrentSample, const ULWord inNumSamples, const double inSampleRate, const double inAmplitude, const double inFrequency, const ULWord inNumBits, const bool inByteSwap, const ULWord inNumChannels)
Fills the given buffer with 32-bit (ULWord) audio tone samples.
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
The NTV2 test pattern generator.
void * GetHostPointer(void) const
virtual AJAStatus SetUpTestPatternBuffers(void)
Creates my test pattern buffers.
NTV2Buffer fAudioBuffer
Host audio buffer.
@ NTV2_Xpt4KDownConverterOut
@ NTV2_XptFrameBuffer8RGB
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=(0))
Connects the given widget signal input (sink) to the given widget signal output (source).
@ NTV2_LHIHDMIColorSpaceYCbCr
bool fDoRGBOnWire
If true, produce RGB on the wire; otherwise output YUV.
static const ULWord gNumFrequencies(sizeof(gFrequencies)/sizeof(double))
@ NTV2_XptFrameBuffer6RGB
@ NTV2_AUDIOSYSTEM_3
This identifies the 3rd Audio System.
@ NTV2_XptFrameBuffer4RGB
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO2
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 2.
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
virtual bool RouteHDMIOutput(void)
Sets up board routing output via the HDMI (if available).
virtual bool SetSDIOut12GEnable(const NTV2Channel inChannel, const bool inEnable)
UWord lastFrame(void) const
Declares the AJAAncillaryData_HDR_HDR10 class.
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
virtual ~NTV2Player4K(void)
virtual bool RouteCscTo2xSDIOut(void)
Sets up board routing from the Color Space Converters to the 2xSDI outputs.
std::string fDeviceSpec
The AJA device to use.
@ NTV2_XptDualLinkOut3Input
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
NTV2ChannelSet NTV2MakeChannelSet(const NTV2Channel inFirstChannel, const UWord inNumChannels=1)
@ NTV2_XptFrameBuffer2YUV
This struct replaces the old RP188_STRUCT.
NTV2Buffer acVideoBuffer
The host video buffer. This field is owned by the client application, and thus is responsible for all...
@ NTV2_XptFrameBuffer5RGB
bool IsRGBFormat(const NTV2FrameBufferFormat format)
@ NTV2_XptFrameBuffer5_DS2YUV
@ NTV2_XptFrameBuffer7YUV
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
static AJA_PixelFormat GetAJAPixelFormat(const NTV2PixelFormat inFormat)
ULWord GetAudioSamplesPerFrame(const NTV2FrameRate inFrameRate, const NTV2AudioRate inAudioRate, ULWord inCadenceFrame=0, bool inIsSMPTE372Enabled=false)
Returns the number of audio samples for a given video frame rate, audio sample rate,...
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
Header file for NTV2Player4K demonstration class.
NTV2Player4K(const PlayerConfig &inConfig)
Constructs me using the given configuration settings.
virtual bool RouteFsToDLOut(void)
Sets up board routing from the Frame Stores to the Dual Link out.
@ NTV2_XptDuallinkOut5DS2
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool RouteFsToTsiMux(void)
Sets up board routing from the Frame Stores to the Two Sample Interleave muxes.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual AJAStatus GenerateTransmitData(uint8_t *pBuffer, const size_t inMaxBytes, uint32_t &outPacketSize)
Generates "raw" ancillary data from my internal ancillary data (playback) – see SDI Anc Buffer Data F...
@ NTV2_XptDualLinkOut6Input
virtual bool RouteFsToCsc(void)
Sets up board routing from the Frame Stores to the Color Space Converters.
@ NTV2_XptDualLinkOut2Input
bool CanDoWidget(const NTV2WidgetID inWgtID)
virtual void ConsumeFrames(void)
My consumer thread that repeatedly plays frames using AutoCirculate (until quit).
@ NTV2_AudioChannel1_8
This selects audio channels 1 thru 8.
virtual NTV2DeviceID GetDeviceID(void)
virtual AJAStatus SetUpVideo(void)
Performs all video setup.
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual void SetupSDITransmitters(const NTV2Channel inFirstSDI, const UWord inNumSDIs)
Sets up bi-directional SDI transmitters.
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
double GetAudioSamplesPerSecond(const NTV2AudioRate inAudioRate)
Returns the audio sample rate as a number of audio samples per second.
virtual bool SetHDMIV2Mode(const NTV2HDMIV2Mode inMode)
Sets HDMI V2 mode for the device.
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
#define NTV2_IS_4K_4096_VIDEO_FORMAT(__f__)
@ NTV2_TestPatt_MultiPattern
Configures an NTV2Player instance.
virtual bool SetHDMIOutAudioSource8Channel(const NTV2Audio8ChannelSelect inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the HDMI output's 8-channel audio source.
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
NTV2PixelFormat fPixelFormat
The pixel format to use.
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
static NTV2TestPatternNames getTestPatternNames(void)
@ NTV2_XptFrameBuffer3_DS2RGB
@ NTV2_XptSDIOut7InputDS2
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
double FramesToSeconds(int64_t frames) const
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
@ NTV2_TestPatt_LineSweep
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
Declares the AJAAncillaryData_HDR_SDR class.
virtual AJAStatus Start()
#define xHEX0N(__x__, __n__)
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
#define AJA_FAILURE(_status_)
@ NTV2_AUDIO_RATE_INVALID
@ NTV2_XptFrameBuffer5YUV
static ULWord gAncMaxSizeBytes(NTV2_ANCSIZE_MAX)
The maximum number of bytes of ancillary data that can be transferred for a single field....
virtual bool SetHDMIV2TxBypass(const bool inBypass)
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
@ NTV2_TestPatt_LinearRamp
virtual bool SetHDMIOutAudioFormat(const NTV2AudioFormat inNewValue)
Sets the HDMI output's audio format.
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
NTV2Buffer acANCBuffer
The host ancillary data buffer. This field is owned by the client application, and thus is responsibl...
@ NTV2_XptFrameBuffer7RGB
virtual bool SetLHIHDMIOutColorSpace(const NTV2LHIHDMIColorSpace inNewValue)
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
virtual void GetACStatus(AUTOCIRCULATE_STATUS &outStatus)
Provides status information about my output (playout) process.
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
bool GetRP188Str(std::string &sRP188) const
virtual bool Route4KDownConverter(void)
Sets up board routing for the 4K DownConverter to SDI Monitor (if available).
@ NTV2_XptSDIOut6InputDS2
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
NTV2StringList NTV2TestPatternNames
An ordered sequence of pattern names.
@ AJAAncDataType_HDR_HDR10
bool Set(const void *pInUserPointer, const size_t inByteCount)
Sets (or resets) me from a client-supplied address and size.
@ NTV2_XptDualLinkOut1Input
@ NTV2_XptDuallinkOut3DS2
@ NTV2_XptDualLinkOut8Input
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread star...
@ NTV2_AUDIOSYSTEM_INVALID
Declares the AJATimeBase class.