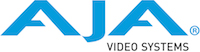 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
33 if (!SubscribeOutputVerticalEvent(*it))
47 if (!SubscribeInputVerticalEvent(*it))
69 if (!UnsubscribeOutputVerticalEvent(*it))
83 if (!UnsubscribeInputVerticalEvent(*it))
144 }
while (--inRepeatCount && result);
159 }
while (--inRepeatCount && result);
168 static ULWord bitShift [] = { 23, 5, 3, 1, 9, 7, 5, 3, 0};
172 outFieldID = ReadRegister (regNum[channel], statusValue)
173 ?
NTV2FieldID((statusValue >> bitShift[channel]) & 0x1)
183 bool bInterruptHappened (WaitForOutputVerticalInterrupt(channel));
187 GetOutputFieldID(channel, currentFieldID);
190 if (currentFieldID != inFieldID)
191 bInterruptHappened = WaitForOutputVerticalInterrupt(channel);
193 return bInterruptHappened;
202 static ULWord bitShift [] = { 21, 19, 21, 19, 17, 15, 13, 3, 0};
206 outFieldID = ReadRegister (regNum[channel], statusValue)
207 ?
NTV2FieldID((statusValue >> bitShift[channel]) & 0x1)
217 bool bInterruptHappened (WaitForInputVerticalInterrupt(channel));
221 GetInputFieldID(channel, currentFieldID);
224 if (currentFieldID != inFieldID)
225 bInterruptHappened = WaitForInputVerticalInterrupt(channel);
227 return bInterruptHappened;
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool GetOutputFieldID(const NTV2Channel channel, NTV2FieldID &outFieldID)
Returns the current field ID of the specified output channel.
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
virtual bool WaitForOutputFieldID(const NTV2FieldID inFieldID, const NTV2Channel inChannel=NTV2_CHANNEL1)
Efficiently sleeps the calling thread/process until the next output VBI for the given field and outpu...
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
NTV2FieldID
These values are used to identify fields for interlaced video. See Field/Frame Interrupts and CNTV2Ca...
NTV2ChannelSet::const_iterator NTV2ChannelSetConstIter
A handy const iterator into an NTV2ChannelSet.
@ NTV2_FIELD0
Identifies the first field in time for an interlaced video frame, or the first and only field in a pr...
#define NTV2_IS_VALID_INTERRUPT_ENUM(__e__)
virtual bool SetOutputVerticalEventCount(const ULWord inCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Resets my output interrupt event tally for the given channel.
virtual bool SubscribeEvent(const INTERRUPT_ENUMS inEventCode)
Causes me to be notified when the given event/interrupt is triggered for the AJA device.
Declares the CNTV2Card class.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
virtual bool GetInputFieldID(const NTV2Channel channel, NTV2FieldID &outFieldID)
Returns the current field ID of the specified input channel.
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
virtual bool GetOutputVerticalEventCount(ULWord &outCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the number of output interrupt events that I successfully waited for on the given channe...
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
#define NTV2_IS_VALID_CHANNEL(__x__)
static INTERRUPT_ENUMS gChannelToInputVerticalInterrupt[]
virtual bool GetInputVerticalEventCount(ULWord &outCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the number of input interrupt events that I successfully waited for on the given channel...
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool SetInputVerticalEventCount(const ULWord inCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Resets my input interrupt event tally for the given channel.
#define NTV2_IS_VALID_FIELD(__x__)
virtual bool UnsubscribeEvent(const INTERRUPT_ENUMS inEventCode)
Unregisters me so I'm no longer notified when the given event/interrupt is triggered on the AJA devic...
static INTERRUPT_ENUMS gChannelToOutputVerticalInterrupt[]
virtual bool GetOutputVerticalInterruptCount(ULWord &outCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the number of output vertical interrupts handled by the driver for the given output chan...
virtual bool WaitForInputFieldID(const NTV2FieldID inFieldID, const NTV2Channel inChannel=NTV2_CHANNEL1)
Efficiently sleeps the calling thread/process until the next input VBI for the given field and input ...
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
virtual bool GetInputVerticalInterruptCount(ULWord &outCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the number of input vertical interrupts handled by the driver for the given input channe...