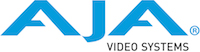 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
24 #if !defined (NTV2_NULL_DEVICE)
27 #include <mach/mach.h>
29 #endif // !defined (NTV2_NULL_DEVICE)
34 static const char *
GetKernErrStr (
const kern_return_t inError);
43 #define HEX2(__x__) xHEX0N(0xFF & uint8_t (__x__),2)
44 #define HEX4(__x__) xHEX0N(0xFFFF & uint16_t(__x__),4)
45 #define HEX8(__x__) xHEX0N(0xFFFFFFFF & uint32_t(__x__),8)
46 #define HEX16(__x__) xHEX0N(uint64_t(__x__),16)
47 #define KR(_kr_) "kernResult=" << HEX8(_kr_) << "(" << GetKernErrStr(_kr_) << ")"
48 #define INSTP(_p_) HEX0N(uint64_t(_p_),16)
50 #define DIFAIL(__x__) AJA_sERROR (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
51 #define DIWARN(__x__) AJA_sWARNING(AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
52 #define DINOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
53 #define DIINFO(__x__) AJA_sINFO (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
54 #define DIDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
56 #define MDIFAIL(__x__) AJA_sERROR (AJA_DebugUnit_DriverInterface, AJAFUNC << ": " << __x__)
57 #define MDIWARN(__x__) AJA_sWARNING(AJA_DebugUnit_DriverInterface, AJAFUNC << ": " << __x__)
58 #define MDINOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_DriverInterface, AJAFUNC << ": " << __x__)
59 #define MDIINFO(__x__) AJA_sINFO (AJA_DebugUnit_DriverInterface, AJAFUNC << ": " << __x__)
60 #define MDIDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_DriverInterface, AJAFUNC << ": " << __x__)
63 #if !defined (NTV2_NULL_DEVICE)
65 #define OS_IOMasterPort(_x_,_y_) ::IOMasterPort ((_x_), (_y_))
66 #define OS_IOServiceOpen(_w_,_x_,_y_,_z_) ::IOServiceOpen((_w_), (_x_), (_y_), (_z_))
67 #define OS_IOServiceClose(_x_) ::IOServiceClose ((_x_))
68 #define OS_IOServiceMatching(_x_) ::IOServiceMatching ((_x_))
69 #define OS_IOServiceNameMatching(_x_) ::IOServiceNameMatching((_x_))
70 #define OS_IOServiceGetMatchingServices(_x_,_y_,_z_) ::IOServiceGetMatchingServices ((_x_), (_y_), (_z_))
71 #define OS_IOIteratorNext(_x_) ::IOIteratorNext ((_x_))
72 #define OS_IOObjectRelease(_x_) ::IOObjectRelease ((_x_))
73 #define OS_IORegistryEntryCreateCFProperty(_w_,_x_,_y_,_z_) ::IORegistryEntryCreateCFProperty ((_w_), (_x_), (_y_), (_z_))
74 #define OS_IOConnectCallMethod(_q_,_r_,_s_,_t_,_u_,_v_,_w_,_x_,_y_,_z_) ::IOConnectCallMethod ((_q_), (_r_), (_s_), (_t_), (_u_), (_v_), (_w_), (_x_), (_y_), (_z_))
75 #define OS_IOConnectCallScalarMethod(_u_,_v_,_w_,_x_,_y_,_z_) ::IOConnectCallScalarMethod ((_u_), (_v_), (_w_), (_x_), (_y_), (_z_))
76 #define OS_IOConnectCallStructMethod(_u_,_v_,_w_,_x_,_y_,_z_) ::IOConnectCallStructMethod ((_u_), (_v_), (_w_), (_x_), (_y_), (_z_))
77 #define OS_IOConnectMapMemory(_u_,_v_,_w_,_x_,_y_,_z_) ::IOConnectMapMemory ((_u_), (_v_), (_w_), (_x_), (_y_), (_z_))
78 #define OS_IOKitGetBusyState(_x_,_y_) ::IOKitGetBusyState ((_x_), (_y_))
79 #define OS_IOKitWaitQuiet(_x_,_y_) ::IOKitWaitQuiet ((_x_), (_y_))
80 #else // NTV2_NULL_DEVICE defined
83 static IOReturn
OS_IOMasterPort (
const mach_port_t inPort, mach_port_t * pOutPort)
85 MDIWARN(
"NTV2_NULL_DEVICE -- will not connect to IOKit");
90 static kern_return_t
OS_IOServiceOpen (
const io_service_t inSvc,
const task_port_t inTask,
const uint32_t inType, io_connect_t * pOutConn)
92 if (pOutConn) *pOutConn = 0;
117 static CFTypeRef
OS_IORegistryEntryCreateCFProperty (
const io_registry_entry_t inEntry,
const CFStringRef inKey,
const CFAllocatorRef inAllocator,
const IOOptionBits inOptions)
121 static IOReturn
OS_IOConnectCallScalarMethod (
const mach_port_t inConnect,
const uint32_t inSelector,
const uint64_t * pInput,
const uint32_t inCount, uint64_t * pOutput, uint32_t * pOutCount)
123 if (pOutput) *pOutput = 0;
124 if (pOutCount) *pOutCount = 0;
127 static kern_return_t
OS_IOConnectCallMethod (
const mach_port_t inConnect,
const uint32_t inSelector,
const uint64_t * pInput, uint32_t inputCnt,
const void * pInStruct,
const size_t inStructCnt, uint64_t * pOutput, uint32_t * pOutputCnt,
void * pOutStruct,
size_t * pOutStructCnt)
137 static IOReturn
OS_IOKitWaitQuiet (
const mach_port_t inPort,
const mach_timespec_t * pInOutWait)
141 static IOReturn
OS_IOConnectCallStructMethod (
const io_connect_t inPort,
const uint32_t inSelector,
const void *inputStruct,
size_t inputStructCnt,
void *pOutStruct,
size_t *pOutStructCnt)
145 static kern_return_t
OS_IOConnectMapMemory (
const io_connect_t inPort,
const uint32_t inMemType,
const task_port_t inTask, mach_vm_address_t * pAddr, mach_vm_size_t * pOutSize,
const IOOptionBits inOpts)
149 #endif // NTV2_NULL_DEVICE defined
172 #if !defined(NTV2_NULL_DEVICE)
179 io_iterator_t ioIterator (0);
180 IOReturn error (kIOReturnSuccess);
181 io_object_t ioObject (0);
184 for (
size_t svcNdx(0); svcNdx < 2 && !mConnection; svcNdx++)
186 const string & svcName (kSvcNames[svcNdx]);
187 const char * pSvcName(svcName.c_str());
188 const bool tryKEXT (svcName.find(
"com_aja_iokit") != string::npos);
194 if (error != kIOReturnSuccess)
195 {
DIWARN(
KR(error) <<
": No '" << svcName <<
"' driver");
continue;}
209 DIWARN(
"No '" << svcName <<
"' devices");
216 if (error != kIOReturnSuccess)
217 {
DIWARN(
KR(error) <<
": IOServiceOpen failed for '" << svcName <<
"' ndx=" << inDeviceIndex);
continue;}
222 _boardOpened = mConnection != 0;
224 DIDBG((mIsDEXT ?
"DEXT" :
"KEXT") <<
" ndx=" << inDeviceIndex <<
" conn=" <<
HEX8(GetIOConnect()) <<
" opened");
226 {
DIFAIL(
INSTP(
this) <<
": No connection: ndx=" << inDeviceIndex);
return false;}
228 _boardNumber = inDeviceIndex;
231 DIFAIL(
"ReadRegister(kRegBoardID) failed: ndx=" << inDeviceIndex <<
" con=" <<
HEX8(GetIOConnect()) <<
" boardID=" <<
HEX8(_boardID));
246 DIDBG(
"Closed " << (mIsDEXT ?
"DEXT" :
"KEXT") <<
" ndx=" << _boardNumber <<
" con=" <<
HEX8(GetIOConnect()) <<
" id=" << ::
NTV2DeviceIDToString(_boardID));
247 _boardOpened =
false;
256 #endif // !defined(NTV2_NULL_DEVICE)
259 #if !defined(NTV2_DEPRECATE_16_0)
283 _pCh1FrameBaseAddress = _pCh2FrameBaseAddress =
AJA_NULL;
286 _pFrameBaseAddress =
reinterpret_cast<ULWord*
>(baseAddr);
293 _pCh1FrameBaseAddress = _pCh2FrameBaseAddress =
AJA_NULL;
308 _pRegisterBaseAddress =
reinterpret_cast<ULWord*
>(baseAddr);
329 _pXena2FlashBaseAddress =
reinterpret_cast<ULWord*
>(baseAddr);
349 mach_vm_size_t size(0);
351 reinterpret_cast<mach_vm_address_t*
>(memPtr),
352 &size, kIOMapDefaultCache | kIOMapAnywhere);
363 kern_return_t kernResult = KERN_FAILURE;
364 uint64_t scalarI_64[2] = {uint64_t(dataPtr), controlCode};
365 uint32_t outputCount = 0;
367 kernResult = KERN_INVALID_ARGUMENT;
368 else if (GetIOConnect())
375 if (kernResult == KERN_SUCCESS)
377 DIFAIL (
KR(kernResult) <<
", con=" <<
HEX8(GetIOConnect()));
380 #endif // !defined(NTV2_DEPRECATE_16_0)
382 #pragma mark - New Driver Calls
393 DIFAIL(
"Shift " <<
DEC(inShift) <<
" > 31, reg=" <<
DEC(inRegNum) <<
" msk=" <<
xHEX0N(inMask,8));
396 #if defined (NTV2_NUB_CLIENT_SUPPORT)
399 #endif // defined (NTV2_NUB_CLIENT_SUPPORT)
400 kern_return_t kernResult(KERN_FAILURE);
401 uint64_t scalarI_64[3] = {inRegNum, inMask, inShift};
402 uint64_t scalarO_64 = outValue;
403 uint32_t outputCount = 1;
415 outValue = uint32_t(scalarO_64);
416 if (kernResult == KERN_SUCCESS)
418 DIFAIL(
KR(kernResult) <<
": ndx=" << _boardNumber <<
", con=" <<
HEX8(GetIOConnect())
419 <<
" -- reg=" <<
DEC(inRegNum) <<
", mask=" <<
HEX8(inMask) <<
", shift=" <<
HEX8(inShift));
434 DIFAIL(
"Shift " <<
DEC(inShift) <<
" > 31, reg=" <<
DEC(inRegNum) <<
" msk=" <<
xHEX0N(inMask,8));
437 #if defined(NTV2_WRITEREG_PROFILING) // Register Write Profiling
438 if (mRecordRegWrites)
441 mRegWrites.push_back(
NTV2RegInfo(inRegNum, inValue, inMask, inShift));
445 #endif // defined(NTV2_WRITEREG_PROFILING) // Register Write Profiling
446 #if defined(NTV2_NUB_CLIENT_SUPPORT)
449 #endif // defined (NTV2_NUB_CLIENT_SUPPORT)
450 kern_return_t kernResult(KERN_FAILURE);
451 uint64_t scalarI_64[4] = {inRegNum, inValue, inMask, inShift};
452 uint32_t outputCount = 0;
464 if (kernResult == KERN_SUCCESS)
466 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()) <<
" -- reg=" << inRegNum
467 <<
", val=" <<
HEX8(inValue) <<
", mask=" <<
HEX8(inMask) <<
", shift=" <<
HEX8(inShift));
483 #if defined(NTV2_NUB_CLIENT_SUPPORT)
486 #endif // defined (NTV2_NUB_CLIENT_SUPPORT)
492 <<
" uninitialized by AJAAgent, requesting app " <<
xHEX0N(appType,8) <<
", pid=" <<
DEC(pid));
494 kern_return_t kernResult = KERN_FAILURE;
495 uint64_t scalarI_64[2] = {uint64_t(appType), uint64_t(pid)};
496 uint32_t outputCount = 0;
506 if (kernResult == KERN_SUCCESS)
508 DIFAIL(
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
518 #if defined(NTV2_NUB_CLIENT_SUPPORT)
521 #endif // defined (NTV2_NUB_CLIENT_SUPPORT)
522 kern_return_t kernResult = KERN_FAILURE;
523 uint64_t scalarI_64[2] = {uint64_t(appType), uint64_t(pid)};
524 uint32_t outputCount = 0;
534 if (kernResult == KERN_SUCCESS)
536 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
553 #if defined(NTV2_NUB_CLIENT_SUPPORT)
556 #endif // defined (NTV2_NUB_CLIENT_SUPPORT)
562 <<
" uninitialized by AJAAgent, requesting app " <<
xHEX0N(appType,8) <<
", pid=" <<
DEC(pid));
564 kern_return_t kernResult = KERN_FAILURE;
565 uint64_t scalarI_64[2] = {uint64_t(appType), uint64_t(pid)};
566 uint32_t outputCount = 0;
576 if (kernResult == KERN_SUCCESS)
578 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
591 #if defined(NTV2_NUB_CLIENT_SUPPORT)
594 #endif // defined (NTV2_NUB_CLIENT_SUPPORT)
595 kern_return_t kernResult = KERN_FAILURE;
596 uint64_t scalarI_64[2] = {uint64_t(appType), uint64_t(pid)};
597 uint32_t outputCount = 0;
607 if (kernResult == KERN_SUCCESS)
609 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
625 #if defined(NTV2_NUB_CLIENT_SUPPORT)
628 #endif // defined (NTV2_NUB_CLIENT_SUPPORT)
629 kern_return_t kernResult = KERN_FAILURE;
630 uint64_t scalarI_64[2] = {uint64_t(appType), uint64_t(pid)};
631 uint32_t outputCount = 0;
641 if (kernResult == KERN_SUCCESS)
643 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
653 #if defined(NTV2_NUB_CLIENT_SUPPORT)
656 #endif // defined (NTV2_NUB_CLIENT_SUPPORT)
657 kern_return_t kernResult = KERN_FAILURE;
658 uint64_t scalarO_64[2] = {0, 0};
659 uint32_t outputCount(2);
669 outAppType =
ULWord(scalarO_64[0]);
670 outProcessID = int32_t(scalarO_64[1]);
671 if (kernResult == KERN_SUCCESS)
673 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
683 kern_return_t kernResult = KERN_FAILURE;
684 uint64_t scalarI_64[2] = {uint64_t(dataPtr), dataSize};
685 uint32_t outputCount = 0;
693 if (kernResult == KERN_SUCCESS)
695 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
704 AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
AJA_DebugStat_WaitForInterruptOthers,
731 return WaitForChangeEvent(timeout);
733 kern_return_t kernResult = KERN_FAILURE;
734 uint64_t scalarI_64[2] = {type, timeout};
735 uint64_t scalarO_64 = 0;
736 uint32_t outputCount = 1;
739 kernResult = KERN_INVALID_VALUE;
740 else if (GetIOConnect())
751 UInt32 interruptOccurred = uint32_t(scalarO_64);
752 if (kernResult != KERN_SUCCESS)
753 {
DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
return false;}
754 if (interruptOccurred)
755 BumpEventCount(type);
756 return interruptOccurred;
766 kern_return_t kernResult = KERN_FAILURE;
767 uint64_t scalarI_64[1] = {eInterrupt};
768 uint64_t scalarO_64 = 0;
769 uint32_t outputCount = 1;
781 outCount =
ULWord(scalarO_64);
782 if (kernResult == KERN_SUCCESS)
784 DIFAIL(
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
797 kern_return_t kernResult = KERN_FAILURE;
798 uint64_t scalarI_64[1] = {timeout};
799 uint64_t scalarO_64 = 0;
800 uint32_t outputCount = 1;
808 if (kernResult != KERN_SUCCESS)
809 DIFAIL(
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
810 return bool(scalarO_64);
826 const ULWord inFrameNumber,
828 const ULWord inOffsetBytes,
830 const bool inSynchronous)
834 inOffsetBytes, inByteCount, inSynchronous);
837 kern_return_t kernResult = KERN_FAILURE;
838 uint64_t scalarI_64[6] = {inDMAEngine, uint64_t(pFrameBuffer), inFrameNumber, inOffsetBytes, inByteCount, !inIsRead};
839 uint32_t outputCount = 0;
851 if (kernResult == KERN_SUCCESS)
853 DIFAIL(
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()) <<
", eng=" << inDMAEngine <<
", frm=" << inFrameNumber
854 <<
", off=" <<
HEX8(inOffsetBytes) <<
", len=" <<
HEX8(inByteCount) <<
", " << (inIsRead ?
"R" :
"W"));
871 const ULWord inFrameNumber,
873 const ULWord inCardOffsetBytes,
875 const ULWord inNumSegments,
876 const ULWord inSegmentHostPitch,
877 const ULWord inSegmentCardPitch,
878 const bool inSynchronous)
882 inNumSegments, inSegmentHostPitch, inSegmentCardPitch, inSynchronous);
885 kern_return_t kernResult = KERN_FAILURE;
886 size_t outputStructSize = 0;
887 const ULWord numSegments = inNumSegments ? inNumSegments : 1;
893 dmaTransfer64.
dmaSize = inByteCount;
913 if (kernResult == KERN_SUCCESS)
915 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
922 const bool inIsTarget,
923 const ULWord inFrameNumber,
924 const ULWord inCardOffsetBytes,
926 const ULWord inNumSegments,
927 const ULWord inSegmentHostPitch,
928 const ULWord inSegmentCardPitch,
933 inNumSegments, inSegmentHostPitch, inSegmentCardPitch, inP2PData);
941 bool CNTV2MacDriverInterface::RestoreHardwareProcampRegisters (
void)
943 kern_return_t kernResult = KERN_FAILURE;
944 uint32_t outputCount = 0;
952 if (kernResult == KERN_SUCCESS)
954 DIFAIL (
KR(kernResult) <<
": con=" <<
HEX8(GetIOConnect()));
964 bool CNTV2MacDriverInterface::SystemStatus (
void* dataPtr,
SystemStatusCode statusCode)
966 kern_return_t kernResult = KERN_FAILURE;
967 uint64_t scalarI_64[2] = {uint64_t(dataPtr), statusCode};
968 uint32_t outputCount = 0;
978 if (kernResult == KERN_SUCCESS)
995 kern_return_t kernResult = KERN_FAILURE;
996 io_connect_t conn(GetIOConnect());
1014 size_t outputStructSize = 0;
1016 CopyTo_AUTOCIRCULATE_DATA_64 (&autoCircData, &autoCircData64);
1031 uint64_t scalarI_64[1];
1032 uint32_t outputCount = 0;
1061 CopyTo_AUTOCIRCULATE_DATA_64 (&autoCircData, &autoCircData64);
1095 CopyTo_AUTOCIRCULATE_DATA_64 (&autoCircData, &autoCircData64);
1123 kernResult = KERN_INVALID_ARGUMENT;
1127 success = (kernResult == KERN_SUCCESS);
1128 if (kernResult != KERN_SUCCESS && kernResult != kIOReturnOffline)
1138 if (!pInOutMessage->
IsValid())
1140 if (!pInOutMessage->GetSizeInBytes())
1146 ULWord* pU32 =
reinterpret_cast<ULWord*
>(pInOutMessage); pU32[6] = 0;
1148 kern_return_t kernResult (KERN_FAILURE);
1149 io_connect_t connection (GetIOConnect ());
1150 uint64_t scalarI_64 [2] = {uint64_t(pInOutMessage), pInOutMessage->GetSizeInBytes()};
1151 uint32_t numScalarOutputs(0);
1163 if (kernResult != KERN_SUCCESS && kernResult != kIOReturnOffline)
1164 MDIFAIL (
KR(kernResult) <<
INSTP(
this) <<
", con=" <<
HEX8(connection) << endl << *pInOutMessage);
1165 return kernResult == KERN_SUCCESS;
1171 #pragma mark Old Driver Calls
1299 printf(
"----------------------\n");
1302 uint8_t * ptr = (uint8_t *)p64;
1307 printf(
"%x ", *ptr++);
1309 printf(
"\n\n", *ptr++);
1314 printf(
"----------------------\n");
1328 printf(
"rp188.DBB %x\n",p64->
rp188.
DBB);
1329 printf(
"rp188.Low %x\n",p64->
rp188.
Low);
1330 printf(
"rp188.High %x\n",p64->
rp188.
High);
1333 printf(
"hUser %x\n",p64->
hUser);
1438 case kIOReturnError:
return "general error";
1439 case kIOReturnNoMemory:
return "can't allocate memory";
1440 case kIOReturnNoResources:
return "resource shortage";
1441 case kIOReturnIPCError:
return "error during IPC";
1442 case kIOReturnNoDevice:
return "no such device";
1443 case kIOReturnNotPrivileged:
return "privilege violation";
1444 case kIOReturnBadArgument:
return "invalid argument";
1445 case kIOReturnLockedRead:
return "device read locked";
1446 case kIOReturnLockedWrite:
return "device write locked";
1447 case kIOReturnExclusiveAccess:
return "exclusive access and device already open";
1448 case kIOReturnBadMessageID:
return "sent/received messages had different msg_id";
1449 case kIOReturnUnsupported:
return "unsupported function";
1450 case kIOReturnVMError:
return "misc. VM failure";
1451 case kIOReturnInternalError:
return "internal error";
1452 case kIOReturnIOError:
return "General I/O error";
1453 case kIOReturnCannotLock:
return "can't acquire lock";
1454 case kIOReturnNotOpen:
return "device not open";
1455 case kIOReturnNotReadable:
return "read not supported";
1456 case kIOReturnNotWritable:
return "write not supported";
1457 case kIOReturnNotAligned:
return "alignment error";
1458 case kIOReturnBadMedia:
return "Media Error";
1459 case kIOReturnStillOpen:
return "device(s) still open";
1460 case kIOReturnRLDError:
return "rld failure";
1461 case kIOReturnDMAError:
return "DMA failure";
1462 case kIOReturnBusy:
return "Device Busy";
1463 case kIOReturnTimeout:
return "I/O Timeout";
1464 case kIOReturnOffline:
return "device offline";
1465 case kIOReturnNotReady:
return "not ready";
1466 case kIOReturnNotAttached:
return "device not attached";
1467 case kIOReturnNoChannels:
return "no DMA channels left";
1468 case kIOReturnNoSpace:
return "no space for data";
1469 case kIOReturnPortExists:
return "port already exists";
1470 case kIOReturnCannotWire:
return "can't wire down physical memory";
1471 case kIOReturnNoInterrupt:
return "no interrupt attached";
1472 case kIOReturnNoFrames:
return "no DMA frames enqueued";
1473 case kIOReturnMessageTooLarge:
return "oversized msg received on interrupt port";
1474 case kIOReturnNotPermitted:
return "not permitted";
1475 case kIOReturnNoPower:
return "no power to device";
1476 case kIOReturnNoMedia:
return "media not present";
1477 case kIOReturnUnformattedMedia:
return "media not formatted";
1478 case kIOReturnUnsupportedMode:
return "no such mode";
1479 case kIOReturnUnderrun:
return "data underrun";
1480 case kIOReturnOverrun:
return "data overrun";
1481 case kIOReturnDeviceError:
return "the device is not working properly!";
1482 case kIOReturnNoCompletion:
return "a completion routine is required";
1483 case kIOReturnAborted:
return "operation aborted";
1484 case kIOReturnNoBandwidth:
return "bus bandwidth would be exceeded";
1485 case kIOReturnNotResponding:
return "device not responding";
1486 case kIOReturnIsoTooOld:
return "isochronous I/O request for distant past!";
1487 case kIOReturnIsoTooNew:
return "isochronous I/O request for distant future";
1488 case kIOReturnNotFound:
return "data was not found";
1489 case MACH_SEND_INVALID_DEST:
return "MACH_SEND_INVALID_DEST";
const IOReturn kNTV2DriverNotReadyErr
virtual bool MapRegisters(void)
@ kDriverReleaseStreamForApplicationWithReference
const IOReturn kNTV2DriverBadDMA
const IOReturn kNTV2DriverUnmapperErr
@ AJA_DebugStat_GetInterruptCount
ULWord videoSegmentHostPitch
@ AJA_DebugStat_DMATransferEx
virtual bool SetStreamingApplication(const ULWord inAppType, const int32_t inProcessID)
Sets the four-CC type and process ID of the application that should "own" the AJA device (i....
ULWord videoSegmentHostPitch
static AJAStatus StatTimerStop(const uint32_t inKey)
NTV2Crosspoint channelSpec
const IOReturn kNTV2HeaderVersionErr
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
AUTO_CIRC_COMMAND eCommand
@ kDriverWaitForInterrupt
virtual bool GetAudioOutputMode(NTV2_GlobalAudioPlaybackMode *mode)
virtual bool KernelLog(void *dataPtr, UInt32 dataSize)
static const char * GetKernErrStr(const kern_return_t inError)
Declares device capability functions.
virtual ~CNTV2MacDriverInterface()
My destructor.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
virtual bool AcquireStreamForApplication(const ULWord inAppType, const int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
@ kDriverAutoCirculateTransfer
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
@ AJA_DebugStat_ReadRegister
NTV2QuarterSizeExpandMode videoQuarterSizeExpand
@ AJA_DebugStat_NTV2Message
static AJAStatus StatTimerStart(const uint32_t inKey)
#define NTV2_ASSERT(_expr_)
@ AJA_DebugStat_AutoCirculate
@ AJA_DebugStat_WaitForInterruptOthers
Declares the AJATime class.
virtual bool WaitForInterrupt(const INTERRUPT_ENUMS eInterrupt, const ULWord timeOutMs=68)
const IOReturn kNTV2DriverParamErr
NTV2VideoFrameBufferOrientation frameBufferOrientation
@ AJA_DebugStat_WaitForInterruptIn2
static const string sNTV2PCIDEXTName("AJANTV2")
Implements the MacOS-specific flavor of CNTV2DriverInterface.
Pointer64 ccLookupTables
only used in 3way color correction mode.
virtual bool AutoCirculate(AUTOCIRCULATE_DATA &autoCircData)
Sends an AutoCirculate command to the NTV2 driver.
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
BOOL_ bDisableExtraAudioInfo
NTV2ColorCorrectionInfo_64 colorCorrectionInfo
const IOReturn kNTV2DriverDMABusy
virtual bool OpenLocalPhysical(const UWord inDeviceIndex)
Opens the local/physical device connection.
virtual bool ReleaseStreamForApplication(const ULWord inAppType, const int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
#define OS_IOKitGetBusyState(_x_, _y_)
virtual bool WaitForInterrupt(const INTERRUPT_ENUMS type, const ULWord timeout=50)
@ kVRegGlobalAudioPlaybackMode
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
virtual bool GetInterruptCount(const INTERRUPT_ENUMS eInterrupt, ULWord &outCount)
Answers with the number of interrupts of the given type processed by the driver.
#define OS_IOServiceClose(_x_)
@ AJA_DebugStat_WaitForInterruptIn8
const IOReturn kNTV2DriverBadHeaderTag
#define NTV2_IS_VALID_INTERRUPT_ENUM(__e__)
NTV2ColorCorrectionInfo colorCorrectionInfo
struct AUTOCIRCULATE_STATUS_STRUCT AUTOCIRCULATE_STATUS_STRUCT
#define OS_IORegistryEntryCreateCFProperty(_w_, _x_, _y_, _z_)
@ kDriverAutoCirculateStatus
virtual bool AcquireStreamForApplicationWithReference(const ULWord inAppType, const int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::AcquireStreamForApplication useful for process g...
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
@ kDriverReleaseStreamForApplication
NTV2FrameBufferFormat frameBufferFormat
virtual bool ReleaseStreamForApplicationWithReference(const ULWord inAppType, const int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::ReleaseStreamForApplication useful for process g...
NTV2_GlobalAudioPlaybackMode
const IOReturn kNTV2DriverMapperErr
NTV2Crosspoint foregroundKeyCrosspoint
AUTO_CIRC_COMMAND eCommand
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
Declares the AJALock class.
const uint32_t kAgentAppFcc(((((uint32_t)( 'A'))<< 24)|(((uint32_t)( 'j'))<< 16)|(((uint32_t)( 'a'))<< 8)|(((uint32_t)( 'A'))<< 0)))
#define OS_IOConnectCallStructMethod(_u_, _v_, _w_, _x_, _y_, _z_)
NTV2Crosspoint channelSpec
#define OS_IOServiceNameMatching(_x_)
virtual bool DmaTransfer(const NTV2DMAEngine inDMAEngine, const bool inIsRead, const ULWord inFrameNumber, ULWord *pFrameBuffer, const ULWord inCardOffsetBytes, const ULWord inTotalByteCount, const bool inSynchronous=(!(0)))
Transfers data between the AJA device and the host. This function will block and not return to the ca...
@ AJA_DebugStat_WaitForInterruptUartTx1
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
@ AJA_DebugStat_AutoCirculateXfer
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
virtual bool MapXena2Flash(void)
ULWord dmaCardFrameNumber
@ AJA_DebugStat_WaitForInterruptUartTx2
ULWord dmaSegmentCardPitch
AutoCircGenericTask * taskArray
#define OS_IOKitWaitQuiet(_x_, _y_)
All new NTV2 structs start with this common header.
CUSTOM_ANC_STRUCT customAncInfo
This field is obsolete. Do not use.
const IOReturn kNTV2DriverBadTrailerTag
Declares numerous NTV2 utility functions.
AutoCircVidProcInfo vidProcInfo
#define OS_IOMasterPort(_x_, _y_)
virtual bool WaitForChangeEvent(UInt32 timeout=0)
@ eTransferAutoCirculateEx
NTV2Crosspoint channelSpec
ULWord dmaCardFrameOffset
AutoCircVidProcInfo vidProcInfo
virtual bool MapMemory(const MemoryType memType, void **memPtr)
@ kDriverRestoreProcAmpRegisters
static const string sNTV2PCIKEXTClassName("com_aja_iokit_ntv2")
struct NTV2RegInfo NTV2RegInfo
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
#define OS_IOObjectRelease(_x_)
ULWord videoSegmentCardPitch
@ AJA_DebugStat_WaitForInterruptUartRx2
const IOReturn kNTV2DriverPgmXilinxErr
NTV2Crosspoint backgroundVideoCrosspoint
virtual bool SystemControl(void *dataPtr, SystemControlCode systemControlCode)
ULWord * ccLookupTables
only used in 3way color correction mode.
virtual bool UnmapXena2Flash(void)
@ AJA_DebugStat_WaitForInterruptIn7
NTV2Crosspoint foregroundVideoCrosspoint
Fixed_ transitionCoefficient
@ AJA_DebugStat_WaitForInterruptIn6
@ AJA_DebugStat_WaitForInterruptUartRx1
CUSTOM_ANC_STRUCT customAncInfo
This field is obsolete. Do not use.
virtual bool MapFrameBuffers(void)
#define OS_IOServiceOpen(_w_, _x_, _y_, _z_)
virtual bool SetStreamingApplication(const ULWord appType, const int32_t pid)
Sets the four-CC type and process ID of the application that should "own" the AJA device (i....
virtual bool NTV2Message(NTV2_HEADER *pInMessage)
Sends a message to the NTV2 driver (the new, improved, preferred way).
ULWord videoSegmentCardPitch
@ kDriverAutoCirculateControl
@ AJA_DebugStat_WaitForInterruptOut1
NTV2VideoFrameBufferOrientation frameBufferOrientation
virtual bool AcquireStreamForApplicationWithReference(ULWord inApplicationType, int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::AcquireStreamForApplication useful for process g...
virtual bool UnmapRegisters(void)
ULWord dmaNumberOfSegments
NTV2ColorCorrectionMode mode
Declares the AJAAtomic class.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
#define OS_IOConnectMapMemory(_u_, _v_, _w_, _x_, _y_, _z_)
std::set< NTV2DeviceID > NTV2DeviceIDSet
A set of NTV2DeviceIDs.
CNTV2MacDriverInterface()
My default constructor.
virtual bool DmaTransfer(const NTV2DMAEngine inDMAEngine, const bool inIsRead, const ULWord inFrameNumber, ULWord *pFrameBuffer, const ULWord inCardOffsetBytes, const ULWord inByteCount, const bool inSynchronous=(!(0)))
Transfers data between the AJA device and the host. This function will block and not return to the ca...
virtual bool UnmapFrameBuffers(void)
@ AJA_DebugStat_WriteRegister
virtual bool SetAudioOutputMode(NTV2_GlobalAudioPlaybackMode mode)
NTV2Crosspoint backgroundKeyCrosspoint
Fixed_ transitionSoftness
@ kVRegServicesInitialized
@ AJA_DebugStat_DMATransfer
BOOL_ bDisableExtraAudioInfo
#define OS_IOServiceMatching(_x_)
@ kDriverWaitForChangeEvent
@ SSC_GetFirmwareProgress
virtual bool AutoCirculate(AUTOCIRCULATE_DATA &pAutoCircData)
Sends an AutoCirculate command to the NTV2 driver.
@ kDriverSetStreamForApplication
@ eTransferAutoCirculateEx2
@ kDriverGetInterruptCount
ULWord dmaSegmentHostPitch
virtual bool ReleaseStreamForApplicationWithReference(ULWord inApplicationType, int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::ReleaseStreamForApplication useful for process g...
#define xHEX0N(__x__, __n__)
NTV2Crosspoint channelSpec
@ kDriverGetStreamForApplication
@ AJA_DebugStat_WaitForInterruptIn1
#define OS_IOIteratorNext(_x_)
#define OS_IOConnectCallScalarMethod(_u_, _v_, _w_, _x_, _y_, _z_)
#define OS_IOConnectCallMethod(_q_, _r_, _s_, _t_, _u_, _v_, _w_, _x_, _y_, _z_)
@ AJA_DebugStat_WaitForInterruptIn3
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
virtual bool NTV2Message(NTV2_HEADER *pInMessage)
Sends a message to the NTV2 driver (the new, improved, preferred way).
@ kDriverAutoCirculateFramestamp
@ AJA_DebugStat_WaitForInterruptIn4
#define OS_IOServiceGetMatchingServices(_x_, _y_, _z_)
NTV2QuarterSizeExpandMode videoQuarterSizeExpand
static NTV2DeviceIDSet gLegalDeviceIDs
static const uint32_t sIntEnumToStatKeys[]
NTV2FrameBufferFormat frameBufferFormat
Declares NTV2 "nub" client functions.
const IOReturn kNTV2DriverPrepMemErr
NTV2ColorCorrectionMode mode
static AJALock gLegalDevIDsLock
const IOReturn kNTV2DriverDMATooLarge
Declares the AJADebug class.
virtual ULWord GetPCISlotNumber(void) const
@ AJA_DebugStat_WaitForInterruptIn5
@ kDriverAcquireStreamForApplicationWithReference
const IOReturn kNTV2UnknownStructType
virtual bool CloseLocalPhysical(void)
Releases host resources associated with the local/physical device connection.
@ kDriverAcquireStreamForApplication