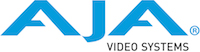 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
34 #define INSTP(_p_) HEX0N(uint64_t(_p_),16)
35 #define DIFAIL(__x__) AJA_sERROR (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
36 #define DIWARN(__x__) AJA_sWARNING(AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
37 #define DINOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
38 #define DIINFO(__x__) AJA_sINFO (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
39 #define DIDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
47 #if defined(_DEBUGSTATS_)
48 #define DIDBGX(__x__) AJA_sDEBUG (AJA_DebugUnit_DriverInterface, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
58 result.push_back(
"ntv2nub"); result.push_back(
"ntv2"); result.push_back(
"ntv2local");
77 mRecordRegWrites (
false),
78 mSkipRegWrites (
false),
82 mInterruptEventHandles (),
88 #if !defined(NTV2_DEPRECATE_16_0)
91 _pRegisterBaseAddressLength (0),
96 _ulNumFrameBuffers (0),
97 _ulFrameBufferSize (0)
98 #if !defined(NTV2_DEPRECATE_16_0)
136 if (inDeviceIndex >= MaxNumDevices())
137 {
DIFAIL(
"Requested device index '" <<
DEC(inDeviceIndex) <<
"' at/past limit of '" <<
DEC(MaxNumDevices()) <<
"'");
return false;}
141 #if !defined(NTV2_ALLOW_OPEN_UNSUPPORTED)
144 if (legalDeviceIDs.find(
_boardID) == legalDeviceIDs.end())
146 DIFAIL(
"Device ID " <<
xHEX0N(
_boardID,8) <<
" (at device index " << inDeviceIndex <<
") is not in list of supported devices");
150 #endif // NTV2_ALLOW_OPEN_UNSUPPORTED
153 uint16_t drvrVersComps[4] = {0, 0, 0, 0};
154 ULWord driverVersionRaw (0);
156 {
DIFAIL(
"ReadRegister(kVRegDriverVersion) failed");
Close();
return false;}
165 DIWARN (
"Driver version v" <<
DEC(drvrVersComps[0]) <<
"." <<
DEC(drvrVersComps[1]) <<
"." <<
DEC(drvrVersComps[2]) <<
"."
166 <<
DEC(drvrVersComps[3]) <<
" ignored for client SDK v0.0.0.0 (dev mode), driverVersionRaw=" <<
xHEX0N(driverVersionRaw,8));
168 DIDBG (
"Driver v" <<
DEC(drvrVersComps[0]) <<
"." <<
DEC(drvrVersComps[1])
169 <<
"." <<
DEC(drvrVersComps[2]) <<
"." <<
DEC(drvrVersComps[3]) <<
" == client SDK v"
173 DIWARN (
"Driver v" <<
DEC(drvrVersComps[0]) <<
"." <<
DEC(drvrVersComps[1])
174 <<
"." <<
DEC(drvrVersComps[2]) <<
"." <<
DEC(drvrVersComps[3]) <<
" != client SDK v"
177 <<
", driverVersionRaw=" <<
xHEX0N(driverVersionRaw,8));
191 if (specParser.HasErrors())
192 {
DIFAIL(
"Bad device specification '" << inURLSpec <<
"': " << specParser.Error());
return false;}
195 if (specParser.IsLocalDevice())
200 Open(card.GetIndexNumber());
204 Open(card.GetIndexNumber());
208 Open(card.GetIndexNumber());
211 Open(specParser.DeviceIndex());
213 {
DIFAIL(
"Failed to open " << specParser.InfoString());
return false;}
249 #if defined(NTV2_NULL_DEVICE)
250 DIFAIL(
"SDK built with 'NTV2_NULL_DEVICE' defined -- cannot OpenLocalPhysical '" <<
DEC(inDeviceIndex) <<
"'");
251 #else // else defined(NTV2_NULL_DEVICE)
252 (
void) inDeviceIndex;
253 NTV2_ASSERT(
false &&
"Requires platform-specific implementation");
254 #endif // else NTV2_NULL_DEVICE defined
260 NTV2_ASSERT(
false &&
"Requires platform-specific implementation");
264 #if defined(AJA_WINDOWS)
265 static bool winsock_inited =
false;
266 static WSADATA wsaData;
268 static void initWinsock(
void)
272 wret = WSAStartup(MAKEWORD(2,2), &wsaData);
273 winsock_inited =
true;
275 #endif // AJA_WINDOWS
280 #if defined(AJA_WINDOWS)
282 #endif // defined(AJA_WINDOWS)
286 #if defined(NTV2_NUB_CLIENT_SUPPORT)
287 if (inParser.Failed())
288 {ostringstream errs; inParser.
PrintErrors(errs);
DIFAIL(
"Bad parser: " << errs.str());
return false;}
289 if (inParser.IsLocalDevice())
290 {
DIFAIL(
"Parser infers local device: " << inParser.
InfoString());
return false;}
309 if (!pClient->IsConnected())
311 {
DIFAIL(
"Failed to connect/open '" << inParser.DeviceSpec() <<
"'");
323 if (!IsRemote() || !IsOpen())
324 DIFAIL(
"Failed to open '" << inParser.DeviceSpec() <<
"'");
325 return IsRemote() && IsOpen();
326 #else // NTV2_NUB_CLIENT_SUPPORT
327 DIFAIL(
"SDK built without 'NTV2_NUB_CLIENT_SUPPORT' -- cannot OpenRemote '" << inParser.DeviceSpec() <<
"'");
329 #endif // NTV2_NUB_CLIENT_SUPPORT
373 NTV2_ASSERT(
false &&
"Needs subclass implementation");
385 { (
void) bEnable; (
void) eInterruptType;
386 NTV2_ASSERT(
false &&
"Needs subclass implementation");
399 <<
"), event counter reset");
404 <<
mEventCounts[eInterruptType] <<
" event(s) received");
435 #if defined(NTV2_NUB_CLIENT_SUPPORT)
448 if (inOutValues.empty())
460 if (!
ReadRegister (iter->registerNumber, iter->registerValue))
465 #if !defined(NTV2_DEPRECATE_16_0)
469 if (!pOutWhichRegFailed)
471 *pOutWhichRegFailed = 0xFFFFFFFF;
477 regReads.reserve(inNumRegs); result.reserve(inNumRegs);
478 for (
size_t ndx(0); ndx < size_t(inNumRegs); ndx++)
479 regReads.push_back(pOutRegInfos[ndx]);
483 if (result.size() < regReads.size())
484 *pOutWhichRegFailed = result.empty() ? regReads.front().registerNumber : result.back().registerNumber;
493 #endif // !defined(NTV2_DEPRECATE_16_0)
499 #if defined(NTV2_WRITEREG_PROFILING)
501 #endif // NTV2_WRITEREG_PROFILING
502 #if defined(NTV2_NUB_CLIENT_SUPPORT)
514 const ULWord inFrameNumber,
516 const ULWord inCardOffsetBytes,
517 const ULWord inTotalByteCount,
518 const bool inSynchronous)
520 #if defined(NTV2_NUB_CLIENT_SUPPORT)
522 NTV2Buffer buffer(pFrameBuffer, inTotalByteCount);
524 0, 0, 0, inSynchronous);
526 (
void) inDMAEngine; (
void) inIsRead; (
void) inFrameNumber; (
void) pFrameBuffer; (
void) inCardOffsetBytes;
527 (
void) inTotalByteCount; (
void) inSynchronous;
534 const ULWord inFrameNumber,
536 const ULWord inCardOffsetBytes,
537 const ULWord inTotalByteCount,
538 const ULWord inNumSegments,
539 const ULWord inHostPitchPerSeg,
540 const ULWord inCardPitchPerSeg,
541 const bool inSynchronous)
543 #if defined(NTV2_NUB_CLIENT_SUPPORT)
545 NTV2Buffer buffer(pFrameBuffer, inTotalByteCount);
547 inNumSegments, inHostPitchPerSeg, inCardPitchPerSeg, inSynchronous);
549 (
void) inDMAEngine; (
void) inIsRead; (
void) inFrameNumber; (
void) pFrameBuffer; (
void) inCardOffsetBytes;
550 (
void) inTotalByteCount; (
void) inNumSegments; (
void) inHostPitchPerSeg; (
void) inCardPitchPerSeg; (
void) inSynchronous;
557 const bool inIsTarget,
558 const ULWord inFrameNumber,
559 const ULWord inCardOffsetBytes,
561 const ULWord inNumSegments,
562 const ULWord inSegmentHostPitch,
563 const ULWord inSegmentCardPitch,
565 { (
void) inDMAEngine; (
void) inDMAChannel; (
void) inIsTarget; (
void) inFrameNumber; (
void) inCardOffsetBytes;
566 (
void) inByteCount; (
void) inNumSegments; (
void) inSegmentHostPitch; (
void) inSegmentCardPitch; (
void) inP2PData;
567 #if defined(NTV2_NUB_CLIENT_SUPPORT)
578 #if defined(NTV2_NUB_CLIENT_SUPPORT)
591 #if defined(NTV2_NUB_CLIENT_SUPPORT)
616 #if defined(NTV2_NUB_CLIENT_SUPPORT)
629 ::memset(&bitFileInfo, 0,
sizeof(bitFileInfo));
710 #if !defined (_DEBUG)
719 const string bitFileDesignNameString = string(bitFileInfo.
designNameStr) +
".bit";
738 vector<uint8_t> mcsInfoData;
739 if (spiFlash.
Read(offset, mcsInfoData, 256))
741 packInfo.assign(mcsInfoData.begin(), mcsInfoData.end());
744 size_t found = packInfo.find(
'\0');
745 if (found != string::npos)
747 packInfo.resize(found);
755 ULWord baseAddress = (16 * 1024 * 1024) - (3 * 256 * 1024);
756 const ULWord dwordSizeCount = 256/4;
761 ULWord timeoutCount = 1000;
766 if (regValue &
BIT(8))
773 }
while (busy ==
true && timeoutCount > 0);
774 if (timeoutCount == 0)
778 for (
ULWord count = 0; count < dwordSizeCount; count++, baseAddress += 4 )
788 if ( regValue &
BIT(8))
795 }
while(busy ==
true && timeoutCount > 0);
796 if (timeoutCount == 0)
798 delete [] bitFilePtr;
804 packInfo =
reinterpret_cast<char*
>(bitFilePtr);
805 delete [] bitFilePtr;
808 istringstream iss(packInfo);
809 vector<string> results;
811 while (getline(iss,token,
' '))
812 results.push_back(token);
814 if (results.size() < 8)
817 packageInfo.
date = results[1];
819 token.erase(remove(token.begin(), token.end(),
'\n'), token.end());
820 packageInfo.
time = token;
830 ::memset(&buildInfo, 0,
sizeof(buildInfo));
842 ULWord attempts(counts >> 16), successes(counts & 0x0000FFFF);
847 counts = (attempts << 16) | successes;
864 outRegValues.clear();
872 outRegValues.push_back(bsMsg.
mRegisters[ndx]);
924 ULWord returnVal1 =
false;
925 ULWord returnVal2 =
false;
931 #if !defined(NTV2_DEPRECATE_16_0)
938 #endif // !defined(NTV2_DEPRECATE_16_0)
961 ULWord timeoutCount = 1000;
966 if (regValue &
BIT(8))
973 }
while (busy && timeoutCount);
979 static const ULWord dwordCount(256/4);
981 if (!bitFileHdrBuffer)
984 ULWord* pULWord(bitFileHdrBuffer), baseAddress(0);
985 for (
ULWord count(0); count < dwordCount; count++, baseAddress += 4)
990 std::string headerError;
993 { fileInfo.
Open(
"/Users/demo/dev-svn/firmware/T3_Tap/t_tap_pro.bit");
998 if (headerError.empty())
1006 return headerError.empty();
1016 ULWord timeoutCount(inRetryCount);
1021 if (regValue &
BIT(8))
1028 }
while (busy && timeoutCount);
1043 ULWord svcInitialized(0);
1045 if (!svcInitialized)
1048 <<
" uninitialized by AJAAgent, requesting app " <<
xHEX0N(inAppCode,8) <<
", pid=" <<
DEC(inProcessID));
1050 ULWord currentCode(0), currentPID(0);
1064 for (
int count(0); count < 20; count++)
1076 else if (currentCode == inAppCode && currentPID ==
ULWord(inProcessID))
1086 ULWord currentCode(0), currentPID(0), currentCount(0);
1092 if (currentCode == inAppCode && currentPID ==
ULWord(inProcessID))
1094 if (currentCount > 1)
1096 if (currentCount == 1)
1105 ULWord svcInitialized(0);
1107 if (!svcInitialized)
1110 <<
" uninitialized by AJAAgent, requesting app " <<
xHEX0N(inAppCode,8) <<
", pid=" <<
DEC(inProcessID));
1113 for (
int count(0); count < 20; count++)
1121 ULWord currentCode(0), currentPID(0);
1129 for (
int count(0); count < 20; count++)
1170 if (desc.empty() && !parms.empty())
1206 ULWord dbbReg(0), msReg(0), lsReg(0);
1243 uint32_t tcReceived = 0;
1256 if (pRP188Data->
DBB == 0x01 || pRP188Data->
DBB == 0x02)
1263 uint32_t ltcPresent = 0;
1265 if (ltcPresent != 1)
1275 if (pRP188Data->
DBB != 0x00)
1290 *pRP188Data = rp188;
1299 if (rp188.
DBB == pRP188Data->
DBB &&
1300 rp188.
Low == pRP188Data->
Low &&
1361 return (val < 2) ?
false :
true;
1377 #if defined(NTV2_WRITEREG_PROFILING) // Register Write Profiling
1432 #endif // NTV2_WRITEREG_PROFILING
1448 result.insert(
ULWord(*it));
1455 result.insert(
ULWord(*it));
1462 result.insert(
ULWord(*it));
1469 result.insert(
ULWord(*it));
1476 result.insert(
ULWord(*it));
1487 result.insert(
ULWord(*it));
1499 result.insert(
ULWord(*it));
1506 result.insert(
ULWord(*it));
1522 if (result.find(
ULWord(refSrc)) == result.end())
1523 result.insert(
ULWord(refSrc));
1544 if (result.find(
ULWord(audSrc)) == result.end())
1545 result.insert(
ULWord(audSrc));
1553 result.insert(
ULWord(*it));
1559 result.insert(
ULWord(cm));
1565 result.insert(
ULWord(ndx));
1571 result.insert(
ULWord(ndx));
1590 outValue = value ? 1 : 0;
1745 default:
return false;
1822 for (
size_t ndx(0); ndx <
sizeof(s425MuxerIDs)/
sizeof(
NTV2WidgetID); ndx++)
1823 if (wgtIDs.find(s425MuxerIDs[ndx]) != wgtIDs.end())
1827 default:
return false;
@ kRegShiftCanDoValidXptROM
std::set< NTV2InputSource > NTV2InputSourceSet
A set of distinct NTV2InputSource values.
virtual bool NTV2WriteRegisterRemote(const ULWord regNum, const ULWord regValue, const ULWord regMask, const ULWord regShift)
UWord NTV2DeviceGetNumLTCInputs(const NTV2DeviceID inDeviceID)
@ kRP188SourceEmbeddedLTC
virtual bool NTV2GetNumericParamRemote(const ULWord inParamID, ULWord &outValue)
@ kDeviceCanDoMSI
True if device DMA hardware supports MSI (Message Signaled Interrupts).
UWord NTV2DeviceGetNumEmbeddedAudioOutputChannels(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoCustomAux(const NTV2DeviceID inDeviceID)
@ kDeviceGetNumVideoInputs
The number of SDI video inputs on the device.
ULWord mRegisters[16]
Register data.
virtual bool ReadFlashULWord(const ULWord inAddress, ULWord &outValue, const ULWord inRetryCount=1000)
bool NTV2DeviceCanDo12GSDI(const NTV2DeviceID inDeviceID)
@ kDeviceHasBiDirectionalSDI
True if device SDI connectors are bi-directional.
@ kDeviceCanDoAudioOutput
True if device has any audio output capability (SDI, HDMI or analog) (New in SDK 17....
@ DEVICE_ID_KONALHIDVI
See KONA LHi.
@ NTV2_BITFILE_KONA5_OE3_MAIN
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
@ kNTV2EnumsID_Mode
Identifies the NTV2Mode enumerated type.
@ kDeviceCanDoAudio6Channels
True if audio system(s) support 6 or more audio channels.
@ kDeviceCanDoPIO
True if device supports Programmed I/O.
bool NTV2DeviceCanDoAnalogVideoIn(const NTV2DeviceID inDeviceID)
virtual bool ParseFlashHeader(BITFILE_INFO_STRUCT &outBitfileInfo)
UWord NTV2DeviceGetNumDownConverters(const NTV2DeviceID inDeviceID)
virtual bool SetStreamingApplication(const ULWord inAppType, const int32_t inProcessID)
Sets the four-CC type and process ID of the application that should "own" the AJA device (i....
UWord NTV2DeviceGetNumAnalogAudioOutputChannels(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_KONAIP_2110
@ kDeviceCanDoStackedAudio
True if device uses a "stacked" arrangement of its audio buffers.
@ kDeviceGetNumMixers
The number of mixer/keyer widgets on the device.
@ NTV2_BITFILE_KONA5_3DLUT_MAIN
@ kDeviceGetNumFrameStores
The number of FrameStores on the device.
virtual bool GetRegInfoForBoolParam(const NTV2BoolParamID inParamID, NTV2RegInfo &outRegInfo)
Answers with the NTV2RegInfo of the register associated with the given boolean (i....
bool mSkipRegWrites
True if actual register writes are skipped while recording.
bool NTV2DeviceCanDoConversionMode(const NTV2DeviceID inDeviceID, const NTV2ConversionMode inConversionMode)
@ DEVICE_ID_KONAIP_2110
See KONA IP.
@ kDeviceCanMeasureTemperature
True if device can measure its FPGA die temperature.
@ NTV2_BITFILE_KONAIP_4CH_2SFP
static void SetShareMode(const bool inSharedMode)
Specifies if subsequent Open calls should open the device in shared mode or not.
UWord NTV2DeviceGetNumHDMIVideoInputs(const NTV2DeviceID inDeviceID)
virtual bool ReadRegisters(NTV2RegisterReads &inOutValues)
Reads the register(s) specified by the given NTV2RegInfo sequence.
NTV2FrameBufferFormatSet::const_iterator NTV2FrameBufferFormatSetConstIter
A handy const iterator for iterating over an NTV2FrameBufferFormatSet.
@ DEVICE_ID_KONAHDMI
See KONA HDMI.
ULWord64 mBufferCookie
Buffer User cookie.
virtual bool StreamBufferOps(const NTV2Channel inChannel, NTV2Buffer &inBuffer, ULWord64 bufferCookie, ULWord flags, NTV2StreamBuffer &status)
@ NTV2_BITFILE_SOJI_OE7_MAIN
UWord NTV2DeviceGetNumReferenceVideoInputs(const NTV2DeviceID inDeviceID)
bool NTV2DeviceGetSupportedStandards(const NTV2DeviceID inDeviceID, NTV2StandardSet &outStandards)
Returns a set of distinct NTV2Standard values supported on the given device.
@ kDeviceHasSPIv4
Use kDeviceGetSPIVersion instead.
std::set< NTV2FrameBufferFormat > NTV2FrameBufferFormatSet
A set of distinct NTV2FrameBufferFormat values.
@ kNTV2EnumsID_DeviceID
Identifies the NTV2DeviceID enumerated type.
@ kNTV2EnumsID_OutputTCIndex
Identifies the NTV2TCIndex enumerated type for output.
@ kDeviceCanDoIP
True if device has SFP connectors.
#define BITSTREAM_RESUME
Used in peta to resume board after bitstream load.
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
@ DEVICE_ID_CORVID44_2X4K
See Corvid 44 12G.
#define kNTV2PluginRegInfoKey_Vendor
Plugin vendor (manufacturer) name.
@ NTV2_CHANNEL2
Specifies channel or FrameStore 2 (or the 2nd item).
@ kDeviceCanDoHDMIMultiView
True if device can rasterize 4 HD signals into a single HDMI output.
virtual bool GetRecordedRegisterWrites(NTV2RegisterWrites &outRegWrites) const
Answers with the recorded register writes.
@ kDeviceCanDoVideoProcessing
True if device can do video processing.
@ DEVICE_ID_KONA5_OE9
See KONA 5.
@ kRegRP188InOut2Bits0_31
bool NTV2DeviceCanDoAnalogAudio(const NTV2DeviceID inDeviceID)
bool NTV2DeviceHasNTV4FrameStores(const NTV2DeviceID inDeviceID)
@ kDeviceGetSPIFlashVersion
The SPI-flash version on the device. (New in SDK 17.1)
virtual bool BitstreamReset(const bool inConfiguration, const bool inInterface)
#define kRegSarekIfVersion
#define BITSTREAM_FRAGMENT
Used in NTV2Bitstream to indicate bitstream is a fragment.
@ kRegRP188InOut1Bits0_31
@ kNTV2EnumsID_VideoFormat
Identifies the NTV2VideoFormat enumerated type.
std::set< NTV2OutputDestination > NTV2OutputDestinations
A set of distinct NTV2OutputDestination values.
#define NEW_SELECT_RP188_RCVD
NTV2_DriverDebugMessageSet
Declares device capability functions.
UWord NTV2DeviceGetGenlockVersion(const NTV2DeviceID inDeviceID)
ULWord * _pXena2FlashBaseAddress
#define kNTV2PluginRegInfoKey_Copyright
Plugin copyright notice.
virtual bool NTV2Disconnect(void)
Disconnects me from the remote/fake host, closing the connection.
@ kDeviceGetUFCVersion
The version number of the UFC on the device.
@ kNTV2EnumsID_AudioRate
Identifies the NTV2AudioRate enumerated type.
@ kDeviceHasXptConnectROM
True if device has a crosspoint connection ROM (New in SDK 17.0)
virtual bool AcquireStreamForApplication(const ULWord inAppType, const int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
ULWord NTV2DeviceGetNumberFrameBuffers(NTV2DeviceID id, NTV2FrameGeometry fg, NTV2FrameBufferFormat fbf)
static uint32_t gOpenCount(0)
#define AJA_NTV2_SDK_VERSION_POINT
The SDK "point" release version, an unsigned decimal integer.
bool NTV2DeviceCanDoOutputTCIndex(const NTV2DeviceID inDeviceID, const NTV2TCIndex inTCIndex)
virtual uint32_t Offset(SpiFlashSection sectionID=SPI_FLASH_SECTION_TOTAL)
@ kDeviceGetNumDownConverters
The number of down-converters on the device.
@ NTV2_BITFILE_SOJI_OE4_MAIN
@ kDeviceCanDoHDV
True if device can squeeze/stretch between 1920x1080 and 1440x1080.
bool NTV2DeviceCanDoRP188(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumCrossConverters(const NTV2DeviceID inDeviceID)
static uint32_t gConstructCount(0)
@ kDeviceCanDoSDIErrorChecks
True if device can perform SDI error checking.
NTV2DeviceIDSet NTV2GetSupportedDevices(const NTV2DeviceKinds inKinds=NTV2_DEVICEKIND_ALL)
Returns an NTV2DeviceIDSet of devices supported by the SDK.
bool NTV2DeviceCanDoEnhancedCSC(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_KONA5_OE6_MAIN
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
bool _boardOpened
True if I'm open and connected to the device.
Describes a user-space buffer on the host computer. I have an address and a length,...
static bool DeviceSupported(NTV2DeviceID deviceId)
bool NTV2DeviceCanDo25GIP(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_KONA5_OE11_MAIN
std::string join(const std::vector< std::string > &parts, const std::string &delim)
const char * NTV2InterruptEnumString(const unsigned inInterruptEnum)
@ NTV2_BITFILE_KONA5_8K_MAIN
@ DEVICE_ID_KONA5
See KONA 5.
@ kDeviceGetNumAnalogAudioInputChannels
The number of analog audio input channels on the device.
ULWord GetByteCount(void) const
@ kRegLTC2EmbeddedBits0_31
#define NTV2_ASSERT(_expr_)
std::set< NTV2VideoFormat > NTV2VideoFormatSet
A set of distinct NTV2VideoFormat values.
#define BITSTREAM_SUSPEND
Used in peta to suspend board before bitstream load.
ULWord NTV2DeviceGetFrameBufferSize(NTV2DeviceID id, NTV2FrameGeometry fg, NTV2FrameBufferFormat fbf)
@ NTV2_BITFILE_NUMBITFILETYPES
virtual ~CNTV2DriverInterface()
My destructor.
@ kDeviceGetNumEmbeddedAudioOutputChannels
The number of SDI-embedded output audio channels supported by the device.
bool NTV2DeviceIs64Bit(const NTV2DeviceID inDeviceID)
std::set< NTV2Standard > NTV2StandardSet
A set of distinct NTV2Standard values.
static NTV2StringList GetLegalSchemeNames(void)
Instances of me can parse a bitfile.
@ kDeviceCanDoAudio192K
True if Audio System(s) support a 192kHz sample rate.
@ NTV2_BITFILE_CORVID44_8K_MAIN
@ DEVICE_ID_IOX3
See Io X3.
@ kVRegAcquireLinuxReferenceCount
#define kConnectParamDevID
First device having this ID (e.g. '0x10518400')
@ kDeviceIsSupported
True if device is supported by this SDK.
Declares the AJATime class.
UWord NTV2DeviceGetNumFrameSyncs(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_KONA5_MAIN
@ kDeviceHasMultiRasterWidget
True if device can rasterize 4 HD signals into a single HDMI output.
virtual bool WaitForInterrupt(const INTERRUPT_ENUMS eInterrupt, const ULWord timeOutMs=68)
ULWord NTV2DeviceGetActiveMemorySize(const NTV2DeviceID inDeviceID)
virtual bool GetPackageInformation(PACKAGE_INFO_STRUCT &outPkgInfo)
Answers with the IP device's package information.
NTV2StandardSet::const_iterator NTV2StandardSetConstIter
A handy const iterator for iterating over an NTV2StandardSet.
bool NTV2DeviceCanReportFailSafeLoaded(const NTV2DeviceID inDeviceID)
NTV2GeometrySet::const_iterator NTV2GeometrySetConstIter
A handy const iterator for iterating over an NTV2GeometrySet.
@ kDeviceHasGenlockv3
True if device has version 3 genlock hardware and/or firmware.
@ kDeviceCanDoEnhancedCSC
True if device has enhanced CSCs.
@ kDeviceHasHeadphoneJack
True if device has a headphone jack.
virtual bool StreamChannelOps(const NTV2Channel inChannel, ULWord flags, NTV2StreamChannel &status)
bool NTV2DeviceCanDoMultiLinkAudio(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoHDMIOutStereo(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_CORVID44_8KMK
See Corvid 44 12G.
@ DEVICE_ID_KONAIP_2110_RGB12
See KONA IP.
@ NTV2_BITFILE_IOIP_2110_RGB12
@ DEVICE_ID_CORVID22
See Corvid 22.
@ DEVICE_ID_IOIP_2022
See Io IP.
static bool GetFirstDeviceWithID(const NTV2DeviceID inDeviceID, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device found on the host t...
char designNameStr[(100)]
ULWord NTV2DeviceGetLUTVersion(const NTV2DeviceID inDeviceID)
@ kRegMaskAudioMixerPresent
@ NTV2_BITFILE_KONA5_8KMK_MAIN
NTV2OutputDestinations::const_iterator NTV2OutputDestinationsConstIter
A handy const iterator for iterating over an NTV2OutputDestinations.
virtual bool NTV2DMATransferRemote(const NTV2DMAEngine inDMAEngine, const bool inIsRead, const ULWord inFrameNumber, NTV2Buffer &inOutBuffer, const ULWord inCardOffsetBytes, const ULWord inNumSegments, const ULWord inSegmentHostPitch, const ULWord inSegmentCardPitch, const bool inSynchronous)
UWord NTV2DeviceGetNumAnalogVideoInputs(const NTV2DeviceID inDeviceID)
@ kDeviceCanDo4KVideo
True if the device can handle 4K/UHD video.
@ DEVICE_ID_CORVIDHEVC
See Corvid HEVC.
@ NTV2_BITFILE_SOJI_OE1_MAIN
@ kNTV2EnumsID_OutputDest
Identifies the NTV2OutputDest enumerated type.
@ kDeviceGetNumReferenceVideoInputs
The number of external reference video inputs on the device.
UWord NTV2DeviceGetNumInputConverters(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
@ kDeviceCanReportRunningFirmwareDate
True if device can report its running (and not necessarily installed) firmware date.
@ NTV2_BITFILE_CORVID44_8KMK_MAIN
@ NTV2_NUM_CONVERSIONMODES
bool NTV2DeviceHasSPIFlash(const NTV2DeviceID inDeviceID)
virtual bool ControlDriverDebugMessages(NTV2_DriverDebugMessageSet msgSet, bool enable)
bool NTV2DeviceCanDoDualLink(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoMSI(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoAudio96K
True if Audio System(s) support a 96kHz sample rate.
@ kDeviceCanDo3GLevelConversion
True if device can do 3G level B to 3G level A conversion.
@ kDeviceHasAudioMonitorRCAJacks
True if device has a pair of unbalanced RCA audio monitor output connectors.
@ kDeviceCanDoQuarterExpand
True if device can handle quarter-sized frames (pixel-halving and line-halving during input,...
virtual bool BitstreamWrite(const NTV2Buffer &inBuffer, const bool inFragment, const bool inSwap)
@ DEVICE_ID_KONA5_8KMK
See KONA 5.
@ NTV2_BITFILE_IOEXPRESS_MAIN
ULWord _ulNumFrameBuffers
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
@ NTV2_BITFILE_CORVID44_2X4K_MAIN
const uint32_t kAgentAppFcc(((((uint32_t)( 'A'))<< 24)|(((uint32_t)( 'j'))<< 16)|(((uint32_t)( 'a'))<< 8)|(((uint32_t)( 'A'))<< 0)))
@ kDeviceGetNumVideoOutputs
The number of SDI video outputs on the device.
virtual bool IsRecordingRegisterWrites(void) const
CNTV2DriverInterface()
My default constructor.
ULWord * _pCh2FrameBaseAddress
@ kDeviceGetNum4kQuarterSizeConverters
The number of quarter-size 4K/UHD down-converters on the device.
bool NTV2DeviceCanThermostat(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNum2022ChannelsSFP1(const NTV2DeviceID inDeviceID)
virtual bool ResumeRecordRegisterWrites(void)
Resumes recording WriteRegister calls (after a prior call to PauseRecordRegisterWrites).
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ kDeviceCanDoFramePulseSelect
True if device supports frame pulse source independent of reference source.
bool NTV2DeviceCanDoHDV(const NTV2DeviceID inDeviceID)
@ kDeviceCanDo12gRouting
True if device supports 12G routing crosspoints.
static bool GetDeviceWithSerial(const std::string &inSerialNumber, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device whose serial number...
virtual bool GetInterruptCount(const INTERRUPT_ENUMS eInterrupt, ULWord &outCount)
Answers with the number of interrupts of the given type processed by the driver.
virtual const std::string & GetLastError(void) const
@ kDeviceHasSPIv2
Use kDeviceGetSPIVersion instead.
@ kDeviceCanThermostat
True if device fan can be thermostatically controlled.
bool NTV2DeviceCanDoAudio96K(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumCSCs(const NTV2DeviceID inDeviceID)
virtual bool GetRegInfoForNumericParam(const NTV2NumericParamID inParamID, NTV2RegInfo &outRegInfo)
Answers with the NTV2RegInfo of the register associated with the given numeric (i....
ULWord _ulFrameBufferSize
@ kDeviceCanDoVersalSysMon
True if device supports Versal Adaptive SoC System Monitor.
NTV2DeviceIDSet::const_iterator NTV2DeviceIDSetConstIter
A convenient const iterator for NTV2DeviceIDSet.
std::string packageNumber
bool NTV2DeviceCanDoFrameStore1Display(const NTV2DeviceID inDeviceID)
@ kRegShiftCanDoAudioWaitForVBI
virtual const std::string & GetTime(void) const
@ kDeviceHasNTV4FrameStores
True if device has NTV4 FrameStores. (New in SDK 17.0)
UWord _boardNumber
My device index number.
@ kDeviceGetNumAESAudioOutputChannels
The number of AES/EBU audio output channels on the device.
virtual bool ReleaseStreamForApplication(const ULWord inAppType, const int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
@ kDeviceCanDoAudioMixer
True if device has a firmware audio mixer.
@ kDeviceCanDoFrameStore1Display
True if device can display/output video from FrameStore 1.
NTV2FrameBufferFormat NTV2PixelFormat
An alias for NTV2FrameBufferFormat.
@ kDeviceCanDoColorCorrection
True if device has any LUTs.
@ kDeviceHasPCIeGen2
True if device supports 2nd-generation PCIe.
@ kDeviceCanDoDualLink
True if device supports 10-bit RGB input/output over 2-wire SDI.
@ kNTV2EnumsID_InputTCIndex
Identifies the NTV2TCIndex enumerated type for input.
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
@ DEVICE_ID_KONAIP_1RX_1TX_1SFP_J2K
See KONA IP.
bool NTV2DeviceHasBiDirectionalAnalogAudio(const NTV2DeviceID inDeviceID)
#define BITSTREAM_RESET_CONFIG
Used in NTV2Bitstream to reset config.
@ kDeviceCanDoWarmBootFPGA
True if device can warm-boot to load updated firmware.
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
@ kRegMaskCanDoValidXptROM
@ NTV2_BITFILE_KONAIP_2TX_1SFP_J2K
@ kDeviceCanChangeFrameBufferSize
True if frame buffer sizes are not fixed.
NTV2DeviceID _boardID
My cached device ID.
@ NTV2_BITFILE_KONA5_OE2_MAIN
@ DEVICE_ID_IO4KUFC
See Io 4K (UFC Mode).
@ kDeviceGetNumAnalogAudioOutputChannels
The number of analog audio output channels on the device.
bool NTV2DeviceCanDo425Mux(const NTV2DeviceID inDeviceID)
_EventCounts mEventCounts
My event tallies, one for each interrupt type. Note that these.
ULWord NTV2DeviceGetMaxTransferCount(const NTV2DeviceID inDeviceID)
@ kRegMaskCanDoAudioWaitForVBI
@ kDeviceROMHasBankSelect
True if device SPIFlash ROM is bank-selected. (New in SDK 17.1)
@ kRegShiftFrameFormatHiBit
@ DEVICE_ID_CORVID1
See Corvid, Corvid 3G.
@ kNTV2EnumsID_RefSource
Identifies the NTV2RefSource enumerated type.
@ kDeviceGetNumDMAEngines
The number of DMA engines on the device.
#define NTV2_IS_VALID_INTERRUPT_ENUM(__e__)
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
Defines the KonaIP/IoIP registers.
@ kDeviceGetNumBufferedAudioSystems
The total number of audio systems on the device that can read/write audio buffer memory....
@ kDeviceGetNumLUTs
The number of LUT widgets on the device.
#define NTV2_WRITEREG_PROFILING
@ kDeviceHasSDIRelays
True if device has bypass relays on its SDI connectors.
bool NTV2DeviceCanDoStereoOut(const NTV2DeviceID inDeviceID)
static bool gSharedMode((0))
@ kDeviceCanDoAudioDelay
True if Audio System(s) support an adjustable delay.
UWord NTV2DeviceGetNumSerialPorts(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoBreakoutBoard
True if device supports an AJA breakout board. (New in SDK 17.0)
bool NTV2DeviceHasLEDAudioMeters(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_CORVID44_8K
See Corvid 44 12G.
@ DEVICE_ID_KONAIP_2TX_1SFP_J2K
See KONA IP.
@ kDeviceGetDACVersion
The version number of the DAC on the device.
bool NTV2DeviceCanDoThunderbolt(const NTV2DeviceID inDeviceID)
@ NTV2_MODE_INPUT
Input (capture) mode, which writes into device SDRAM.
virtual bool ReadRP188Registers(const NTV2Channel inChannel, RP188_STRUCT *pRP188Data)
@ kVRegReleaseApplication
#define kConnectParamDevModel
First device of this model (e.g. 'kona4')
virtual bool IsSupported(const NTV2BoolParamID inParamID)
#define NTV2DriverVersionDecode_Point(__vers__)
@ kDeviceCanDoLTC
True if device can read LTC (Linear TimeCode) from one of its inputs.
bool NTV2DeviceNeedsRoutingSetup(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoProRes(const NTV2DeviceID inDeviceID)
virtual bool AcquireStreamForApplicationWithReference(const ULWord inAppType, const int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::AcquireStreamForApplication useful for process g...
@ kDeviceCanDoDVCProHD
True if device can squeeze/stretch between 1920x1080/1280x1080 and 1280x720/960x720.
bool NTV2DeviceCanDoStereoIn(const NTV2DeviceID inDeviceID)
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
@ NTV2_BITFILE_CORVID3G_MAIN
ULWord NTV2DeviceGetHDMIVersion(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_TYPE_INVALID
@ kDeviceCanDoHFRRGB
True if device supports 1080p RGB at more than 50Hz frame rates.
NTV2RegisterWrites mRegWrites
Stores WriteRegister data.
@ DEVICE_ID_KONAIP_4CH_2SFP
See KONA IP.
@ NTV2_BITFILE_KONA5_OE1_MAIN
ULWord NTV2DeviceGetUFCVersion(const NTV2DeviceID inDeviceID)
virtual bool ReleaseStreamForApplicationWithReference(const ULWord inAppType, const int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::ReleaseStreamForApplication useful for process g...
virtual bool ConfigureInterrupt(const bool bEnable, const INTERRUPT_ENUMS eInterruptType)
ULWord NTV2DeviceGetPingLED(const NTV2DeviceID inDeviceID)
NTV2WidgetIDSet::const_iterator NTV2WidgetIDSetConstIter
An iterator for iterating over a read-only NTV2WidgetIDSet.
virtual bool Open(const std::string &inBitfilePath)
Opens the bitfile at the given path, then parses its header.
@ NTV2_BITFILE_KONA4UFC_MAIN
virtual size_t GetProgramStreamLength(void) const
static bool GetOverlappedMode(void)
@ kDeviceCanDoAnalogAudio
True if device has any analog inputs or outputs.
@ kVRegForceApplicationPID
bool NTV2DeviceHasSPIFlashSerial(const NTV2DeviceID inDeviceID)
virtual NTV2ConnectParams ConnectParams(void) const
Declares the AJAProcess class.
@ kDeviceCanDoProgrammableRS422
True if device has at least one RS-422 serial port, and it (they) can be programmed (for baud rate,...
@ kDeviceGetNumAudioSystems
The number of independent Audio Systems on the device.
@ kDeviceHasSPIv3
Use kDeviceGetSPIVersion instead.
@ kDeviceSoftwareCanChangeFrameBufferSize
True if device frame buffer size can be changed.
@ kDeviceCanDoThunderbolt
True if device connects to the host using a Thunderbolt cable.
This is used by the CNTV2Card::ReadRegisters function.
@ kDeviceCanDo2110
True if device supports SMPTE ST2110.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
enum _NTV2NumericParamID NTV2NumericParamID
Used with CNTV2DriverInterface::GetNumericParam to determine device capabilities.
@ kDeviceCanDoRGBLevelAConversion
True if the device can do RGB over 3G Level A.
@ kDeviceGetNum2022ChannelsSFP1
The number of 2022 channels configured on SFP 1 on the device.
AUTO_CIRC_COMMAND eCommand
@ NTV2_BITFILE_KONA5_OE4_MAIN
virtual bool SetInterruptEventCount(const INTERRUPT_ENUMS inEventCode, const ULWord inCount)
Resets my interrupt event tally for the given interrupt type. (This is my count of the number of succ...
@ kNTV2EnumsID_FrameGeometry
Identifies the NTV2FrameGeometry enumerated type.
bool NTV2DeviceIsSupported(const NTV2DeviceID inDeviceID)
@ kDeviceHasRotaryEncoder
True if device has a rotary encoder volume control.
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
@ kDeviceHasSPIFlashSerial
True if device has serial SPI flash hardware.
@ NTV2_BITFILE_KONAIP_25G
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
bool NTV2DeviceCanDoIsoConvert(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoHDMIHDROut
True if device supports HDMI HDR output.
static uint32_t gDestructCount(0)
@ kRegMaskFrameFormatHiBit
@ DEVICE_ID_KONA5_8K
See KONA 5.
@ DEVICE_ID_KONA3G
See KONA 3G (UFC Mode).
#define NTV2DriverVersionDecode_Major(__vers__)
bool NTV2DeviceCanDoRateConvert(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoProRes
True if device can can accommodate Apple ProRes-compressed video in its frame buffers.
bool NTV2DeviceCanDoWidget(const NTV2DeviceID inDeviceID, const NTV2WidgetID inWidgetID)
@ kDeviceGetNumVideoChannels
The number of video channels supported on the device.
bool NTV2DeviceCanReportRunningFirmwareDate(const NTV2DeviceID inDeviceID)
@ kRegXenaxFlashControlStatus
virtual bool NTV2GetBoolParamRemote(const ULWord inParamID, ULWord &outValue)
@ kDeviceGetNumAnalogVideoInputs
The number of analog video inputs on the device.
Declares the CNTV2DeviceScanner class.
@ DEVICE_ID_KONAXM
See KONA XM™.
virtual bool DmaTransfer(const NTV2DMAEngine inDMAEngine, const bool inIsRead, const ULWord inFrameNumber, ULWord *pFrameBuffer, const ULWord inCardOffsetBytes, const ULWord inTotalByteCount, const bool inSynchronous=(!(0)))
Transfers data between the AJA device and the host. This function will block and not return to the ca...
@ kDeviceCanDoHDMIQuadRasterConversion
True if HDMI in/out supports square-division (quad) raster conversion. (New in SDK 17....
@ DEVICE_ID_CORVID3G
See Corvid, Corvid 3G.
@ DEVICE_ID_KONAX
See KONA X™.
@ NTV2_1080i_5994to525_5994
bool NTV2DeviceHasAudioMonitorRCAJacks(const NTV2DeviceID inDeviceID)
@ kNTV2EnumsID_ConversionMode
Identifies the NTV2ConversionMode enumerated type.
@ DEVICE_ID_KONA5_OE5
See KONA 5.
enum _NTV2EnumsID NTV2EnumsID
Identifies NTV2 enumerated types, used in CNTV2DriverInterface::GetSupportedItems.
NTV2ReferenceSource
These enum values identify a specific source for the device's (output) reference clock.
@ kDeviceGetLUTVersion
The version number of the LUT(s) on the device.
@ DEVICE_ID_KONA4UFC
See KONA 4 (UFC Mode).
bool NTV2DeviceHasBiDirectionalSDI(const NTV2DeviceID inDeviceID)
#define BITSTREAM_SWAP
Used in NTV2Bitstream to byte swap bitstream data.
NTV2FrameRateSet::const_iterator NTV2FrameRateSetConstIter
A handy const iterator for iterating over an NTV2FrameRateSet.
@ kDeviceCanDoHDMIOutStereo
True if device supports 3D/stereo HDMI video output.
@ DEVICE_ID_KONAIP_1RX_1TX_2110
See KONA IP.
std::set< NTV2FrameGeometry > NTV2GeometrySet
A set of distinct NTV2FrameGeometry values.
bool NTV2DeviceCanDoIDSwitch(const NTV2DeviceID inDeviceID)
@ kRegRP188InOut1Bits32_63
static void SetOverlappedMode(const bool inOverlapMode)
Specifies if the next Open call should try to open the device in overlapped mode or not.
bool NTV2DeviceCanDoSDIErrorChecks(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoLTCInOnRefPort
True if device can read LTC (Linear TimeCode) from its reference input.
bool NTV2DeviceSoftwareCanChangeFrameBufferSize(const NTV2DeviceID inDeviceID)
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
@ kNTV2EnumsID_ScanGeometry
Identifies the NTV2ScanGeometry enumerated type.
bool NTV2DeviceGetSupportedVideoFormats(const NTV2DeviceID inDeviceID, NTV2VideoFormatSet &outFormats)
Returns a set of distinct NTV2VideoFormat values supported on the given device.
@ NTV2_BITFILE_IO4KPLUS_MAIN
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
UWord NTV2DeviceGetDownConverterDelay(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoPCMDetection
True if device can detect which audio channel pairs are not carrying PCM (Pulse Code Modulation) audi...
@ kDeviceIs64Bit
True if device is 64-bit addressable.
bool NTV2DeviceCanReportFrameSize(const NTV2DeviceID inDeviceID)
@ kDeviceGetHDMIVersion
The version number of the HDMI chipset on the device.
@ kDeviceCanDisableUFC
True if there's at least one UFC, and it can be disabled.
@ kDeviceAudioCanWaitForVBI
True if device audio systems can wait for VBI before starting. (New in SDK 17.0)
virtual HANDLE GetInterruptEvent(const INTERRUPT_ENUMS eInterruptType)
@ kDeviceHasXilinxDMA
True if device has Xilinx DMA hardware.
virtual std::string Description(void) const
@ kDeviceGetMaxRegisterNumber
The highest register number for the device.
virtual bool CloseRemote(void)
Releases host resources associated with the remote/special device connection.
@ NTV2_BITFILE_SOJI_DIAGS_MAIN
UWord NTV2DeviceGetNumVideoInputs(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumHDMIVideoOutputs(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoVITC2
True if device can insert or extract RP-188/VITC2.
UWord NTV2DeviceGetNumLUTs(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_TTAP_PRO
See T-TAP Pro.
All new NTV2 structs start with this common header.
@ kDeviceHasHEVCM31
True if device has an HEVC M31 encoder.
@ kDeviceGetNumInputConverters
The number of input converter widgets on the device.
virtual std::string GetDesignName(void) const
@ NTV2_BITFILE_KONA5_OE10_MAIN
bool NTV2DeviceGetSupportedFrameRates(const NTV2DeviceID inDeviceID, NTV2FrameRateSet &outRates)
Returns a set of distinct NTV2FrameRate values supported on the given device.
Enumerations for controlling NTV2 devices.
NTV2RegWrites NTV2RegisterReads
bool NTV2DeviceCanDo4KVideo(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_KONAIP_25G
See konaip25g.
#define BITSTREAM_MCAP_DATA
MCAP data register.
bool NTV2DeviceHasRetailSupport(const NTV2DeviceID inDeviceID)
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
@ kNTV2EnumsID_InputSource
Identifies the NTV2InputSource enumerated type.
Declares numerous NTV2 utility functions.
@ kDeviceHasSPIv5
Use kDeviceGetSPIVersion instead.
virtual bool OpenRemote(const NTV2DeviceSpecParser &inSpec)
Peforms the housekeeping details of opening the remote/virtual device using the given specParser.
@ kDeviceCanDoSDVideo
True if device can handle SD (Standard Definition) video.
bool NTV2DeviceCanDo3GLevelConversion(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoMultiLinkAudio
True if device supports grouped audio system control.
@ kRegLTC2EmbeddedBits32_63
NTV2VideoFormatSet::const_iterator NTV2VideoFormatSetConstIter
A handy const iterator for iterating over an NTV2VideoFormatSet.
@ NTV2_BITFILE_KONAIP_1RX_1TX_1SFP_J2K
@ DEVICE_ID_KONAIP_2022
See KONA IP.
virtual Word SleepMs(const LWord msec)
@ kDeviceCanDoAudioInput
True if device has any audio input capability (SDI, HDMI or analog) (New in SDK 17....
@ NTV2_BITFILE_KONA5_2X4K_MAIN
static bool GetFirstDeviceWithName(const std::string &inNameSubString, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device whose device identi...
@ NTV2_MAX_NUM_TIMECODE_INDEXES
@ kDeviceGetMaxTransferCount
The maximum number of 32-bit words that the DMA engine can move at a time on the device.
bool NTV2DeviceCanDoAudioDelay(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_IOXT
See Io XT.
virtual bool BitstreamLoad(const bool inSuspend, const bool inResume)
virtual bool GetNumericParam(const ULWord inParamID, ULWord &outValue)
UWord NTV2DeviceGetNumHDMIAudioInputChannels(const NTV2DeviceID inDeviceID)
I interrogate and control an AJA video/audio capture/playout device.
@ DEVICE_ID_KONA5_8K_MV_TX
See KONA 5.
UWord NTV2DeviceGetNumFrameStores(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumAnalogVideoOutputs(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_KONA5_OE8
See KONA 5.
@ NTV2_BITFILE_KONA3G_MAIN
UWord NTV2DeviceGetNumEmbeddedAudioInputChannels(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoPCMControl
True if device can mark specific audio channel pairs as not carrying PCM (Pulse Code Modulation) audi...
std::vector< ULWord > NTV2ULWordVector
An ordered sequence of ULWords.
@ DEVICE_ID_KONALHEPLUS
See KONA LHe Plus.
bool NTV2DeviceCanDoQuarterExpand(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumLTCOutputs(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoHFRRGB(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_KONA5_OE1
See KONA 5.
virtual bool IsDeviceReady(const bool inCheckValid=(0))
bool NTV2DeviceIsDirectAddressable(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_CORVID88
See Corvid 88.
virtual bool StopRecordRegisterWrites(void)
Stops recording all WriteRegister calls.
@ kDeviceCanDoAnalogVideoOut
True if device has one or more analog video outputs.
virtual bool IsMBSystemValid(void)
Defines for the NTV2 SDK version number, used by ajantv2/includes/ntv2enums.h. See the ajantv2/includ...
bool NTV2DeviceHasPWMFanControl(const NTV2DeviceID inDeviceID)
bool NTV2DeviceHasHEVCM31(const NTV2DeviceID inDeviceID)
bool NTV2DeviceIsExternalToHost(const NTV2DeviceID inDeviceID)
virtual bool BitstreamStatus(NTV2ULWordVector &outRegValues)
@ kDeviceHasColorSpaceConverterOnChannel2
Calculate based on if NTV2_WgtCSC2 is present.
bool NTV2DeviceCanDo2KVideo(const NTV2DeviceID inDeviceID)
virtual bool IsLPSystemReady(void)
bool NTV2DeviceCanDoRGBLevelAConversion(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_KONALHE_PLUS
@ kDeviceCanDoBreakoutBox
True if device supports an AJA breakout box.
@ SPI_FLASH_SECTION_MCSINFO
@ kNTV2EnumsID_WidgetID
Identifies the NTV2AudioWidgetID enumerated type.
#define AJA_NTV2_SDK_VERSION_MAJOR
The SDK major version number, an unsigned decimal integer.
@ kDeviceIsDirectAddressable
True if device is direct addressable.
@ kDeviceGetPingLED
The highest bit number of the LED bits in the Global Control Register on the device.
@ kDeviceCanDoStereoIn
True if device supports 3D video input over dual-stream SDI.
std::vector< std::string > NTV2StringList
virtual ULWord GetNumSupported(const NTV2NumericParamID inParamID)
@ kRegShiftAudioMixerPresent
bool NTV2DeviceCanDisableUFC(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoAudio192K(const NTV2DeviceID inDeviceID)
@ kDeviceGetNumMicInputs
The number of microphone inputs on the device.
@ kDeviceGetDownConverterDelay
The down-converter delay on the device.
enum _NTV2BoolParamID NTV2BoolParamID
Used with CNTV2DriverInterface::GetBoolParam to determine device capabilities.
#define kNTV2PluginRegInfoKey_Description
Brief plugin description.
virtual bool NTV2AutoCirculateRemote(AUTOCIRCULATE_DATA &autoCircData)
virtual bool NTV2WaitForInterruptRemote(const INTERRUPT_ENUMS eInterrupt, const ULWord timeOutMs)
bool NTV2DeviceCanDoHDVideo(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNum2022ChannelsSFP2(const NTV2DeviceID inDeviceID)
#define NTV2_IS_VALID_AUDIO_SOURCE(_x_)
@ kDeviceGetNumAESAudioInputChannels
The number of AES/EBU audio input channels on the device.
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
One-stop shop for parsing device specifications. (New in SDK 16.3) I do very little in the way of val...
static bool GetWidgetIDs(const NTV2DeviceID inDeviceID, NTV2WidgetIDSet &outWidgets)
Returns the widget IDs supported by the given device.
@ kDeviceHasRetailSupport
True if device is supported by AJA "retail" software (AJA ControlPanel & ControlRoom).
@ NTV2_BITFILE_SOJI_OE2_MAIN
@ kDeviceHasGenlockv2
True if device has version 2 genlock hardware and/or firmware.
UWord NTV2DeviceGetNumMixers(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoDVCProHD(const NTV2DeviceID inDeviceID)
static uint32_t gCloseCount(0)
void * GetHostPointer(void) const
@ kDeviceGetNumFrameSyncs
The number of frame sync widgets on the device.
@ kDeviceGetNumHDMIVideoInputs
The number of HDMI video inputs on the device.
@ DEVICE_ID_IOIP_2110
See Io IP.
virtual void FinishOpen(void)
Initializes my member variables after a successful Open.
@ DEVICE_ID_KONA5_3DLUT
See KONA 5.
@ kDeviceCanDoAESAudioIn
True if device has any AES audio inputs or outputs.
@ NTV2_BITFILE_KONA5_OE5_MAIN
virtual ULWord GetNumRecordedRegisterWrites(void) const
@ kDeviceCanDoAnalogVideoIn
True if device has one or more analog video inputs.
@ kDeviceIsLocalPhysical
True if device is local-host-attached, and not remote, software or virtual (new in SDK 17....
bool NTV2DeviceCanDoVideoProcessing(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoPCMDetection(const NTV2DeviceID inDeviceID)
Declares the CNTV2Bitfile class.
@ kDeviceGetNumCSCs
The number of colorspace converter widgets on the device.
@ kDeviceCanDoRGBPlusAlphaOut
True if device has CSCs capable of splitting the key (alpha) and YCbCr (fill) from RGB frame buffers ...
bool NTV2DeviceCanChangeEmbeddedAudioClock(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDo12gRouting(const NTV2DeviceID inDeviceID)
@ kDeviceHasIDSwitch
True if device has a mechanical identification switch. (New in SDK 17.1)
@ NTV2_BITFILE_KONAIP_2022
@ NTV2_BITFILE_IO4KUFC_MAIN
#define AJA_NTV2_SDK_BUILD_NUMBER
The SDK build number, an unsigned decimal integer.
static int32_t Increment(int32_t volatile *pTarget)
virtual bool GetBoolParam(const ULWord inParamID, ULWord &outValue)
#define BITSTREAM_WRITE
Used in NTV2Bitstream to write a bitstream.
bool NTV2DeviceHasPCIeGen2(const NTV2DeviceID inDeviceID)
static bool gOverlappedMode((0))
#define kConnectParamDevIndex
Device having this index number.
NTV2Channel mChannel
Stream channel.
@ kDeviceCanDoCapture
True if device has any SDI, HDMI or analog video inputs.
@ kRegRP188InOut2Bits32_63
bool NTV2DeviceHasSDIRelays(const NTV2DeviceID inDeviceID)
NTV2Channel mChannel
Stream channel.
bool NTV2DeviceCanDoProgrammableRS422(const NTV2DeviceID inDeviceID)
@ kDeviceCanReportFrameSize
True if device can report its frame size.
@ kDeviceGetNumHDMIVideoOutputs
The number of HDMI video outputs on the device.
@ kDeviceHasLEDAudioMeters
True if device has LED audio meters.
bool NTV2DeviceHasXilinxDMA(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoIP(const NTV2DeviceID inDeviceID)
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
@ kDeviceCanChangeEmbeddedAudioClock
Private include file for all ajabase sources.
@ DEVICE_ID_CORVID24
See Corvid 24.
bool NTV2DeviceCanDoSDVideo(const NTV2DeviceID inDeviceID)
std::vector< NTV2RegInfo > NTV2RegisterWrites
#define NTV2DriverVersionDecode_Minor(__vers__)
@ kDeviceGetNumHDMIAudioOutputChannels
The number of HDMI audio output channels on the device.
This is used for bitstream maintainance. (New in SDK 16.0)
ULWord NTV2DeviceGetNumDMAEngines(const NTV2DeviceID inDeviceID)
ULWord _pRegisterBaseAddressLength
@ DEVICE_ID_KONA1
See KONA 1.
std::ostream & PrintErrors(std::ostream &oss) const
@ kDeviceHasSPIFlash
True if device has SPI flash hardware.
@ kDeviceGetNumHDMIAudioInputChannels
The number of HDMI audio input channels on the device.
ULWord registerNumber
My register number to use in a ReadRegister or WriteRegister call.
@ NTV2_BITFILE_KONA5_OE12_MAIN
bool NTV2DeviceHasHEVCM30(const NTV2DeviceID inDeviceID)
static NTV2RPCClientAPI * CreateClient(NTV2ConnectParams &inParams)
Instantiates a new NTV2RPCClientAPI instance using the given NTV2ConnectParams.
@ kDeviceNeedsRoutingSetup
True if device widget routing can be queried or changed.
bool NTV2DeviceGetSupportedGeometries(const NTV2DeviceID inDeviceID, NTV2GeometrySet &outGeometries)
Returns a set of distinct NTV2FrameGeometry values supported on the given device.
@ NTV2_BITFILE_CORVID24_MAIN
@ DEVICE_ID_IO4KPLUS
See Io 4K Plus.
@ kDeviceCanDoJ2K
True if device supports JPEG 2000 codec.
@ NTV2_BITFILE_CORVIDHEVC
bool NTV2DeviceGetSupportedOutputDests(const NTV2DeviceID inDeviceID, NTV2OutputDestinations &outOutputDests, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL)
Returns a set of distinct NTV2OutputDest values supported on the given device.
void Set(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Sets me from the given parameters.
virtual bool ConfigureSubscription(const bool bSubscribe, const INTERRUPT_ENUMS inInterruptType, PULWord &outSubcriptionHdl)
@ kDeviceCanDoStreamingDMA
True if device supports streaming DMA. (New in SDK 17.1)
virtual const std::string & GetDate(void) const
@ NTV2_BITFILE_KONA5_OE9_MAIN
bool NTV2DeviceCanDoHDMIHDROut(const NTV2DeviceID inDeviceID)
NTV2InputSourceSet::const_iterator NTV2InputSourceSetConstIter
A handy const iterator for iterating over an NTV2InputSourceSet.
@ kDeviceIsExternalToHost
True if device connects to the host with a cable.
bool NTV2DeviceGetSupportedPixelFormats(const NTV2DeviceID inDeviceID, NTV2PixelFormats &outFormats)
Returns a set of distinct NTV2FrameBufferFormat values supported on the given device.
@ DEVICE_ID_CORVID44
See Corvid 44.
@ NTV2_BITFILE_KONA5_OE8_MAIN
bool NTV2DeviceCanDoBreakoutBoard(const NTV2DeviceID inDeviceID)
virtual bool NTV2Connect(void)
@ NTV2_MODE_OUTPUT
Output (playout, display) mode, which reads from device SDRAM.
#define kRegSarekMBUptime
Declares the CNTV2SpiFlash and CNTV2AxiSpiFlash classes.
ULWord registerShift
My register shift value to use in a ReadRegister or WriteRegister call.
bool GetRegisterValues(NTV2RegisterValueMap &outValues) const
Returns an NTV2RegisterValueMap built from my mOutGoodRegisters and mOutValues fields.
ULWord * _pCh1FrameBaseAddress
bool NTV2DeviceCanDoAnalogVideoOut(const NTV2DeviceID inDeviceID)
#define NTV2_BITFILE_PARTNAME_STRINGLENGTH
@ kDeviceHasBreakoutBoard
True if device has attached breakout board. (New in SDK 17.0)
@ DEVICE_ID_KONA4
See KONA 4 (Quad Mode).
bool NTV2DeviceCanDoLTCInOnRefPort(const NTV2DeviceID inDeviceID)
@ kDeviceCanDo8KVideo
True if device supports 8K video formats.
@ kVRegReleaseLinuxReferenceCount
@ kDeviceHasMicrophoneInput
True if device has a microphone input connector.
UWord NTV2DeviceGetDACVersion(const NTV2DeviceID inDeviceID)
@ NTV2_TCINDEX_DEFAULT
The "default" timecode (mostly used by the AJA "Retail" service and Control Panel)
virtual bool Read(const uint32_t address, std::vector< uint8_t > &data, uint32_t maxBytes=1)
ULWord NTV2DeviceGetNumVideoChannels(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_SOJI_OE5_MAIN
@ NTV2_BITFILE_CORVID1_MAIN
@ NTV2_BITFILE_KONA4_MAIN
virtual std::string GetDescription(void) const
Declares the AJAAtomic class.
bool NTV2DeviceCanDoStackedAudio(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_TTAP
See T-TAP.
bool NTV2DeviceGetSupportedInputSources(const NTV2DeviceID inDeviceID, NTV2InputSourceSet &outInputSources, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL)
Returns a set of distinct NTV2InputSource values supported on the given device.
NTV2FrameGeometry
Identifies a particular video frame geometry.
@ kDeviceCanDo425Mux
True if the device supports SMPTE 425 mux control.
@ kDeviceGetNumTSIMuxers
The number of TSI muxers on the device. (New in SDK 17.0)
@ kVRegForceApplicationCode
@ DEVICE_ID_KONA5_OE3
See KONA 5.
virtual bool NTV2GetSupportedRemote(const ULWord inEnumsID, ULWordSet &outSupported)
std::set< NTV2DeviceID > NTV2DeviceIDSet
A set of NTV2DeviceIDs.
@ kNTV2EnumsID_Channel
Identifies the NTV2Channel enumerated type.
UWord NTV2DeviceGetNumOutputConverters(const NTV2DeviceID inDeviceID)
_EventHandles mInterruptEventHandles
For subscribing to each possible event, one for each interrupt type.
virtual NTV2DeviceID GetDeviceID(void)
bool NTV2DeviceCanDoPIO(const NTV2DeviceID inDeviceID)
@ kDeviceGetMaxAudioChannels
The maximum number of audio channels that a single Audio System can support on the device.
@ DEVICE_ID_KONA5_OE7
See KONA 5.
@ kDeviceCanDoRP188
True if device can insert and/or extract RP-188/VITC.
UWord NTV2DeviceGetNumHDMIAudioOutputChannels(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoAudio2Channels
True if audio system(s) support 2 or more audio channels.
virtual NTV2Dictionary ConnectParams(void) const
bool mRecordRegWrites
True if recording; otherwise false when not recording.
bool NTV2DeviceCanDoWarmBootFPGA(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoRGBPlusAlphaOut(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoAESAudioOut
True if device has any AES audio output channels (New in SDK 17.1)
@ NTV2_BITFILE_CORVID22_MAIN
bool NTV2DeviceCanDoInputTCIndex(const NTV2DeviceID inDeviceID, const NTV2TCIndex inTCIndex)
@ DEVICE_ID_KONA5_OE10
See KONA 5.
@ kDeviceCanDo12GSDI
True if device has 12G SDI connectors.
@ kDeviceCanDoHDVideo
True if device can handle HD (High Definition) video.
@ kDeviceCanDoCustomAnc
True if device has SDI ANC inserter/extractor firmware.
bool NTV2DeviceHasRotaryEncoder(const NTV2DeviceID inDeviceID)
ULWord registerMask
My register mask value to use in a ReadRegister or WriteRegister call.
@ kDeviceGetActiveMemorySize
The size, in bytes, of the device's active RAM available for video and audio.
bool NTV2DeviceCanDoVersalSysMon(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_TTAP_PRO_MAIN
@ kDeviceCanDoHDMIAuxPlayback
True if device has HDMI AUX data inserter(s).
#define AJA_NTV2_SDK_VERSION_MINOR
The SDK minor version number, an unsigned decimal integer.
ULWord NTV2DeviceGetMaxRegisterNumber(const NTV2DeviceID inDeviceID)
@ kNTV2EnumsID_AudioSource
Identifies the NTV2AudioSource enumerated type.
@ NTV2_BITFILE_KONA3G_QUAD
@ kVRegServicesInitialized
static bool IsValid(uint64_t pid)
virtual bool NTV2MessageRemote(NTV2_HEADER *pInMessage)
bool NTV2DeviceCanDoHDMIMultiView(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumUpConverters(const NTV2DeviceID inDeviceID)
@ kDeviceGetTotalNumAudioSystems
The total number of audio systems on the device, including host audio and mixer audio systems,...
bool NTV2DeviceCanChangeFrameBufferSize(const NTV2DeviceID inDeviceID)
ULWord mFlags
Action flags.
@ DEVICE_ID_KONA3GQUAD
See KONA 3G (Quad Mode).
@ DEVICE_ID_CORVIDHBR
See Corvid HB-R.
NTV2Buffer mBuffer
Virtual address of a stream buffer and its length.
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
@ kDeviceHasNWL
True if device has NorthWest Logic DMA hardware.
NTV2RegWrites NTV2RegReads
An ordered sequence of zero or more NTV2RegInfo structs intended for ReadRegister.
@ kVRegDynFirmwareUpdateCounts
@ kDeviceCanReportFailSafeLoaded
True if device can report if its "fail-safe" firmware is loaded/running.
virtual bool StartRecordRegisterWrites(const bool inSkipActualWrites=(0))
Starts recording all WriteRegister calls.
@ NTV2_BITFILE_KONAIP_1RX_1TX_2110
bool NTV2DeviceCanDoCustomAnc(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_SOJI_3DLUT_MAIN
bool NTV2DeviceCanMeasureTemperature(const NTV2DeviceID inDeviceID)
#define NTV2DriverVersionDecode_Build(__vers__)
#define NTV2_IS_VALID_NTV2ReferenceSource(__x__)
@ kDeviceCanDoRateConvert
True if device can do frame rate conversion.
#define BITSTREAM_READ_REGISTERS
Used in NTV2Bitstream to get status registers.
virtual std::string ParseHeaderFromBuffer(const uint8_t *inBitfileBuffer, const size_t inBufferSize)
Parse a bitfile header that's stored in a buffer.
bool NTV2DeviceCanDo8KVideo(const NTV2DeviceID inDeviceID)
virtual bool AutoCirculate(AUTOCIRCULATE_DATA &pAutoCircData)
Sends an AutoCirculate command to the NTV2 driver.
virtual bool PauseRecordRegisterWrites(void)
Pauses recording WriteRegister calls.
UWord NTV2DeviceGetNumAnalogAudioInputChannels(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumAESAudioOutputChannels(const NTV2DeviceID inDeviceID)
virtual bool GetInterruptEventCount(const INTERRUPT_ENUMS inEventCode, ULWord &outCount)
Answers with the number of interrupt events that I successfully waited for.
@ kDeviceCanDoProgrammableCSC
True if device has at least one programmable color space converter widget.
@ DEVICE_ID_KONA5_OE11
See KONA 5.
static bool GetShareMode(void)
ULWord * _pRegisterBaseAddress
void MakeInvalid(void)
Invalidates me, setting my register number, value, mask and shift values to 0xFFFFFFFF.
@ NTV2_BITFILE_KONA5_8K_MV_TX_MAIN
@ NTV2_REFERENCE_EXTERNAL
Specifies the External Reference connector.
NTV2AudioSource NTV2InputSourceToAudioSource(const NTV2InputSource inInputSource)
@ kDeviceCanDoAudio8Channels
True if audio system(s) support 8 or more audio channels.
@ kDeviceCanDoCustomHancInsertion
True if device supports custom HANC packet insertion. (New in SDK 17.1)
@ kRegLTCEmbeddedBits0_31
AJALock mRegWritesLock
Guard mutex for mRegWrites.
bool NTV2DeviceHasNWL(const NTV2DeviceID inDeviceID)
@ kDeviceHasHEVCM30
True if device has an HEVC M30 encoder/decoder.
bool NTV2DeviceCanDoJ2K(const NTV2DeviceID inDeviceID)
@ kVRegDriverVersion
Packed driver version – use NTV2DriverVersionEncode, NTV2DriverVersionDecode* macros to encode/decode...
@ kDeviceCanDo2KVideo
True if device can handle 2Kx1556 (film) video.
#define xHEX0N(__x__, __n__)
virtual bool ReadRegisterMulti(const ULWord numRegs, ULWord *pOutWhichRegFailed, NTV2RegInfo aRegs[])
@ kDeviceCanDoHDMIAuxCapture
True if device has HDMI AUX data extractor(s).
@ DEVICE_ID_IOIP_2110_RGB12
See Io IP.
virtual void BumpEventCount(const INTERRUPT_ENUMS eInterruptType)
Atomically increments the event count tally for the given interrupt type.
UWord NTV2DeviceGetNum4kQuarterSizeConverters(const NTV2DeviceID inDeviceID)
@ kDeviceGetNumUpConverters
The number of up-converters on the device.
@ DEVICE_ID_KONA5_2X4K
See KONA 5.
@ kRegLTCEmbeddedBits32_63
#define kConnectParamDevSerial
Device with this serial number.
@ kDeviceHasBiDirectionalAnalogAudio
True if device has a bi-directional analog audio connector.
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
An object that can connect to, and operate remote or fake devices. I have three general API groups:
std::string valueForKey(const std::string &inKey) const
@ NTV2_BITFILE_KONA5_OE7_MAIN
NTV2RegWritesIter NTV2RegisterReadsIter
@ kDeviceGetNumAnalogVideoOutputs
The number of analog video outputs on the device.
@ DEVICE_ID_KONA5_OE2
See KONA 5.
#define NTV2_BITFILE_DATETIME_STRINGLENGTH
NTV2AudioSource
This enum value determines/states where an audio system will obtain its audio samples.
@ kDeviceGetNum2022ChannelsSFP2
The number of 2022 channels configured on SFP 2 on the device.
bool NTV2DeviceCanDo2110(const NTV2DeviceID inDeviceID)
Declares the CNTV2DriverInterface base class.
virtual bool CloseLocalPhysical(void)
Releases host resources associated with the local/physical device connection.
@ kDeviceCanDoDSKOpacity
True if device mixer/keyer supports adjustable opacity.
@ DEVICE_ID_SOFTWARE
Software device that doesn't emulate one of the above devices.
A simple (not thread-safe) set of key/value pairs. (New in SDK 16.3)
@ kDeviceHasPWMFanControl
True if device has a PWM-controlled cooling fan. (New in SDK 17.1)
std::set< NTV2FrameRate > NTV2FrameRateSet
A set of distinct NTV2FrameRate values. New in SDK 17.0.
@ kDeviceGetNumOutputConverters
The number of output converter widgets on the device.
std::string InfoString(void) const
@ NTV2_BITFILE_KONAIP_2110_RGB12
virtual bool Open(const UWord inDeviceIndex)
Opens a local/physical AJA device so it can be monitored/controlled.
@ DEVICE_ID_IO4K
See Io 4K (Quad Mode).
@ kDeviceGetNumEmbeddedAudioInputChannels
The number of SDI-embedded input audio channels supported by the device.
@ kDeviceGetNumSerialPorts
The number of RS-422 serial ports on the device.
virtual bool NTV2Message(NTV2_HEADER *pInMessage)
Sends a message to the NTV2 driver (the new, improved, preferred way).
@ kNTV2EnumsID_Standard
Identifies the NTV2Standard enumerated type.
bool NTV2DeviceHasHeadphoneJack(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_SOJI_OE3_MAIN
ULWord * _pFrameBaseAddress
UWord NTV2DeviceGetNumAESAudioInputChannels(const NTV2DeviceID inDeviceID)
virtual const std::string & GetPartName(void) const
virtual bool DriverGetBitFileInformation(BITFILE_INFO_STRUCT &outBitFileInfo, const NTV2BitFileType inBitFileType=NTV2_VideoProcBitFile)
Answers with the currently-installed bitfile information.
bool NTV2DeviceCanDoDSKOpacity(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_KONALHI
See KONA LHi.
bool NTV2DeviceCanDoPCMControl(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
virtual bool IsMBSystemReady(void)
@ kDeviceCanDoQREZ
True if device can handle QRez.
virtual bool NTV2ReadRegisterRemote(const ULWord regNum, ULWord &outRegValue, const ULWord regMask, const ULWord regShift)
Declares NTV2 "nub" client functions.
@ DEVICE_ID_KONA5_OE4
See KONA 5.
std::set< NTV2WidgetID > NTV2WidgetIDSet
A collection of distinct NTV2WidgetID values.
#define NTV2_BITFILE_DESIGNNAME_STRINGLENGTH
@ kDeviceGetNumCrossConverters
The number of cross-converters on the device.
@ kDeviceCanDoIsoConvert
True if device can do ISO conversion.
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
@ kDeviceCanDoMultiFormat
True if device can simultaneously handle different video formats on more than one SDI input or output...
bool NTV2DeviceCanDoLTC(const NTV2DeviceID inDeviceID)
@ kNTV2EnumsID_FrameRate
Identifies the NTV2FrameRate enumerated type.
virtual bool Close(void)
Closes me, releasing host resources that may have been allocated in a previous Open call.
Declares the AJADebug class.
@ kDeviceGetNumLTCOutputs
The number of analog LTC outputs on the device.
#define BITSTREAM_RESET_MODULE
Used in NTV2Bitstream to reset module.
NTV2RPCAPI * _pRPCAPI
Points to remote or software device interface; otherwise NULL for local physical device.
virtual ULWordSet GetSupportedItems(const NTV2EnumsID inEnumsID)
@ NTV2_BITFILE_SOJI_OE6_MAIN
@ NTV2_BITFILE_CORVID44_PLNR_MAIN
bool Set(const void *pInUserPointer, const size_t inByteCount)
Sets (or resets) me from a client-supplied address and size.
@ DEVICE_ID_KONA5_OE6
See KONA 5.
@ kDeviceCanDoStereoOut
True if device supports 3D video output over dual-stream SDI.
@ DEVICE_ID_KONA5_OE12
See KONA 5.
bool NTV2DeviceCanDoFramePulseSelect(const NTV2DeviceID inDeviceID)
ULWord mFlags
Action flags.
@ kDeviceGetNumLTCInputs
The number of analog LTC inputs on the device.
UWord NTV2DeviceGetSPIFlashVersion(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoVITC2(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoQREZ(const NTV2DeviceID inDeviceID)
virtual bool OpenLocalPhysical(const UWord inDeviceIndex)
Opens the local/physical device connection.
@ DEVICE_ID_CORVID44_PLNR
See Corvid 44 12G.
virtual bool DriverGetBuildInformation(BUILD_INFO_STRUCT &outBuildInfo)
Answers with the driver's build information.
@ kNTV2EnumsID_PixelFormat
Identifies the NTV2PixelFormat enumerated type.
#define kNTV2PluginRegInfoKey_LongName
Plugin long name.
bool NTV2DeviceCanDoBreakoutBox(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_IOEXPRESS
See Io Express.