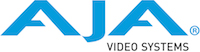 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
17 #if !defined(NTV2_PREVENT_PLUGIN_LOAD)
19 #include "mbedtls/x509.h"
20 #include "mbedtls/error.h"
21 #include "mbedtls/md.h"
22 #include "mbedtls/ssl.h"
23 #endif // defined(NTV2_PREVENT_PLUGIN_LOAD)
25 #include <CoreFoundation/CoreFoundation.h>
27 #define DLL_EXTENSION ".dylib"
28 #define PATH_DELIMITER "/"
29 #define FIRMWARE_FOLDER "Firmware"
30 #elif defined(AJALinux)
32 #define DLL_EXTENSION ".so"
33 #define PATH_DELIMITER "/"
34 #define FIRMWARE_FOLDER "firmware"
35 #elif defined(MSWindows)
36 #define DLL_EXTENSION ".dll"
37 #define PATH_DELIMITER "\\"
38 #define FIRMWARE_FOLDER "Firmware"
39 #elif defined(AJABareMetal)
40 #define DLL_EXTENSION ".so"
41 #define PATH_DELIMITER "/"
42 #define FIRMWARE_FOLDER "firmware"
44 #define SIG_EXTENSION ".sig"
48 #define INSTP(_p_) xHEX0N(uint64_t(_p_),16)
49 #define NBFAIL(__x__) AJA_sERROR (AJA_DebugUnit_RPCClient, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
50 #define NBWARN(__x__) AJA_sWARNING(AJA_DebugUnit_RPCClient, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
51 #define NBNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_RPCClient, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
52 #define NBINFO(__x__) AJA_sINFO (AJA_DebugUnit_RPCClient, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
53 #define NBDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_RPCClient, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
54 #define NBCFAIL(__x__) AJA_sERROR (AJA_DebugUnit_RPCClient, AJAFUNC << ": " << __x__)
55 #define NBCWARN(__x__) AJA_sWARNING(AJA_DebugUnit_RPCClient, AJAFUNC << ": " << __x__)
56 #define NBCNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_RPCClient, AJAFUNC << ": " << __x__)
57 #define NBCINFO(__x__) AJA_sINFO (AJA_DebugUnit_RPCClient, AJAFUNC << ": " << __x__)
58 #define NBCDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_RPCClient, AJAFUNC << ": " << __x__)
59 #define NBSFAIL(__x__) AJA_sERROR (AJA_DebugUnit_RPCServer, AJAFUNC << ": " << __x__)
60 #define NBSWARN(__x__) AJA_sWARNING(AJA_DebugUnit_RPCServer, AJAFUNC << ": " << __x__)
61 #define NBSNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_RPCServer, AJAFUNC << ": " << __x__)
62 #define NBSINFO(__x__) AJA_sINFO (AJA_DebugUnit_RPCServer, AJAFUNC << ": " << __x__)
63 #define NBSDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_RPCServer, AJAFUNC << ": " << __x__)
65 #define PLGFAIL(__x__) AJA_sERROR (AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
66 #define PLGWARN(__x__) AJA_sWARNING(AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
67 #define PLGNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
68 #define PLGINFO(__x__) AJA_sINFO (AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
69 #define PLGDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
71 #define P_FAIL(__x__) do \
74 _os_ << AJAFUNC << ": " << __x__; \
76 cout << "## ERROR: " << _os_.str() << endl; \
77 AJA_sERROR (AJA_DebugUnit_Plugins, _os_.str()); \
78 errMsg = _os_.str(); \
80 #define P_WARN(__x__) if (useStdout()) cout << "## WARNING: " << AJAFUNC << ": " << __x__ << endl; \
81 AJA_sWARNING(AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
82 #define P_NOTE(__x__) if (useStdout()) cout << "## NOTE: " << AJAFUNC << ": " << __x__ << endl; \
83 AJA_sNOTICE (AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
84 #define P_INFO(__x__) if (useStdout()) cout << "## INFO: " << AJAFUNC << ": " << __x__ << endl; \
85 AJA_sINFO (AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
86 #define P_DBG(__x__) if (useStdout()) cout << "## DEBUG: " << AJAFUNC << ": " << __x__ << endl; \
87 AJA_sDEBUG (AJA_DebugUnit_Plugins, AJAFUNC << ": " << __x__)
88 #define _DEBUGSTATS_ // Define this to log above construct/destruct & open/close tallies
89 #if defined(_DEBUGSTATS_)
90 #define PDBGX(__x__) AJA_sDEBUG (AJA_DebugUnit_Plugins, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
112 if (it == mDict.end())
119 string str(valueForKey(inKey));
122 if (str.find(
"0x") == 0 || str.find(
"0X") == 0)
129 if (str.find(
"x") == 0 || str.find(
"X") == 0)
136 if (str.find(
"o") == 0 || str.find(
"O") == 0)
143 if (str.find(
"b") == 0 || str.find(
"B") == 0)
158 const string & key(it->first), val(it->second), quote(val.find(
' ') != string::npos ?
"'" :
"");
159 oss << key <<
"=" << quote << val << quote;
160 if (++it != mDict.end())
167 const int kyWdth(
int(largestKeySize()+0)), valWdth(
int(largestValueSize()+0));
168 oss << string(
size_t(kyWdth),
'-') <<
" " << string(
size_t(valWdth),
'-') << endl;
171 const string & key(it->first), val(it->second);
172 oss << std::setw(kyWdth) << key <<
" : " << val;
173 if (++it != mDict.end())
182 size_t badKVPairs(0), insertFailures(0);
188 if (keyValPair.size() != 2)
189 {badKVPairs++;
continue;}
190 const string k(keyValPair.at(0)), v(keyValPair.at(1));
194 return !empty() && !badKVPairs && !insertFailures;
203 oss << it->first <<
"\t" << it->second;
204 if (++it != mDict.end())
208 return !outStr.empty();
214 for (
DictConstIter it(mDict.begin()); it != mDict.end(); ++it)
215 result.insert(it->first);
222 for (
DictConstIter it(mDict.begin()); it != mDict.end(); ++it)
223 if (it->first.length() > result)
224 result = it->first.length();
231 for (
DictConstIter it(mDict.begin()); it != mDict.end(); ++it)
232 if (it->second.length() > result)
233 result = it->second.length();
241 if (inKey.find(
"\t") != string::npos)
243 if (inKey.find(
"\n") != string::npos)
245 if (inValue.find(
"\t") != string::npos)
247 if (inValue.find(
"\n") != string::npos)
249 mDict[inKey] = inValue;
255 size_t numUpdated(0);
256 for (
DictConstIter it(inDict.mDict.begin()); it != inDict.mDict.end(); ++it)
257 if (hasKey(it->first))
258 {mDict[it->first] = it->second; numUpdated++;}
265 for (
DictConstIter it(inDict.mDict.begin()); it != inDict.mDict.end(); ++it)
266 if (!hasKey(it->first))
267 {mDict[it->first] = it->second; numAdded++;}
281 mQueryParams.clear();
293 if (!inStripLeadSlash)
295 if (rsrc.at(0) ==
'/')
300 void NTV2DeviceSpecParser::Parse (
void)
309 string tokDevID, tokIndexNum, tokScheme, tokSerial, tokModelName;
310 size_t posDevID(0), posIndexNum(0), posScheme(0), posSerial(0), posModelName(0);
311 bool isSerial(ParseSerialNum(posSerial, tokSerial)), isScheme(ParseScheme(posScheme, tokScheme));
312 bool isIndexNum(ParseDecNumber(posIndexNum, tokIndexNum)), isDeviceID(ParseDeviceID(posDevID, tokDevID));
313 bool isModelName(ParseModelName(posModelName, tokModelName));
316 posDevID = posIndexNum = posSerial = posModelName = posScheme;
317 isSerial = ParseSerialNum(posSerial, tokSerial);
318 isIndexNum = ParseDecNumber(posIndexNum, tokIndexNum);
319 isDeviceID = ParseDeviceID(posDevID, tokDevID);
320 isModelName = ParseModelName(posModelName, tokModelName);
333 bool converted(
false);
335 if (tokSerial.length() == 18)
338 const bool hasLeading0X (tokSerial.find(
"0X") == 0 || tokSerial.find(
"0x") == 0);
339 const string hex64(tokSerial.substr(hasLeading0X ? 2 : 0, 16));
342 for (
size_t ndx(0); ndx < 8; ndx++)
343 serTxt +=
char(serNum64 >> ((7-ndx)*8));
349 for (
size_t ndx(0); ndx < tokSerial.length(); ndx++)
350 {
char ch(tokSerial.at(ndx));
351 if ( ! ( ( (ch >=
'0') && (ch <=
'9') ) ||
352 ( (ch >=
'A') && (ch <=
'Z') ) ||
353 ( (ch >=
'a') && (ch <=
'z') ) ||
354 (ch ==
' ') || (ch ==
'-') ) )
356 err <<
"Illegal serial number character '" << (ch ? ch :
'?') <<
"' (" <<
xHEX0N(
UWord(ch),2) <<
")";
358 mPos -= converted ? 16 : 8; mPos += ndx * (converted ? 2 : 1) + (converted ? 1 : 0);
382 err <<
"Invalid local device specification";
384 mPos += isScheme ? 12 : 0;
395 size_t posURL(posScheme), posRsrc(0);
396 string host, port, rsrcPath;
397 if (!ParseHostAddressAndPortNumber(posURL, host, port))
398 {mPos = posURL; AddError(
"Bad host address or port number");
break;}
407 if (ParseResourcePath(posRsrc, rsrcPath))
410 size_t posQuery(mPos);
412 if (ParseQuery(posQuery, params))
415 mQueryParams = params;
418 if (mPos < SpecLength())
419 {err <<
"Extra character(s) at " <<
DEC(mPos); AddError(err.str());
break;}
428 #endif // defined(_DEBUG)
434 oss <<
"local device";
435 else if (HasScheme())
436 oss <<
"device '" << Scheme() <<
"'";
440 oss <<
" serial '" << DeviceSerial() <<
"'";
442 oss <<
" model '" << DeviceModel() <<
"'";
444 oss <<
" ID '" << DeviceID() <<
"'";
446 oss <<
" " << DeviceIndex();
456 {oss << endl; Results().Print(oss,
false);}
470 if (devIDStr.find(
"0X") != string::npos)
485 oss <<
DEC(ErrorCount()) << (ErrorCount() == 1 ?
" error" :
" errors") << (HasErrors() ?
":" :
"");
489 << DeviceSpec() << endl
490 << string(mPos ? mPos : 0,
' ') <<
"^" << endl;
491 for (
size_t num(0); num < ErrorCount(); )
494 if (++num < ErrorCount())
501 bool NTV2DeviceSpecParser::ParseHexNumber (
size_t & pos,
string & outToken)
505 while (pos < SpecLength())
507 const char ch(CharAt(pos));
508 if (tokHexNum.length() == 0)
512 ++pos; tokHexNum = ch;
514 else if (tokHexNum.length() == 1)
516 if (ch !=
'x' && ch !=
'X')
518 ++pos; tokHexNum += ch;
524 ++pos; tokHexNum += ch;
527 if (tokHexNum.length() > 2)
528 {
aja::upper(tokHexNum); outToken = tokHexNum;}
529 return !outToken.empty();
532 bool NTV2DeviceSpecParser::ParseDecNumber (
size_t & pos,
string & outToken)
536 while (pos < SpecLength())
538 const char ch(CharAt(pos));
539 if (!IsDecimalDigit(ch))
542 if (ch !=
'0' || tokDecNum !=
"0")
545 if (tokDecNum.length() > 0)
546 outToken = tokDecNum;
547 return !outToken.empty();
550 bool NTV2DeviceSpecParser::ParseAlphaNumeric (
size_t & pos,
string & outToken,
const std::string & inOtherChars)
554 while (pos < SpecLength())
556 const char ch(CharAt(pos));
557 if (!IsLetter(ch) && !IsDecimalDigit(ch) && inOtherChars.find(ch) == string::npos)
559 ++pos; tokAlphaNum += ch;
561 if (tokAlphaNum.length() > 1)
562 outToken = tokAlphaNum;
563 return !outToken.empty();
566 bool NTV2DeviceSpecParser::ParseScheme (
size_t & pos,
string & outToken)
569 string rawScheme, tokScheme;
570 while (ParseAlphaNumeric(pos, rawScheme))
572 tokScheme = rawScheme;
573 char ch(CharAt(pos));
576 ++pos; tokScheme += ch;
581 ++pos; tokScheme += ch;
586 ++pos; tokScheme += ch;
589 if (tokScheme.find(
"://") != string::npos)
590 {
aja::lower(rawScheme); outToken = rawScheme;}
591 return !outToken.empty();
594 bool NTV2DeviceSpecParser::ParseSerialNum (
size_t & pos,
string & outToken)
597 string tokAlphaNum, tokHexNum;
598 size_t posAlphaNum(pos), posHexNum(pos);
601 while (posAlphaNum < SpecLength())
603 const char ch(CharAt(posAlphaNum));
604 if (!IsUpperLetter(ch) && !IsDecimalDigit(ch) && ch !=
'-' && ch !=
' ')
606 ++posAlphaNum; tokAlphaNum += ch;
608 if (tokAlphaNum.length() < 2)
610 else if (tokAlphaNum.length() == 8 || tokAlphaNum.length() == 9)
611 {pos = posAlphaNum; outToken = tokAlphaNum;
break;}
613 if (ParseHexNumber(posHexNum, tokHexNum))
614 if (tokHexNum.length() == 18)
615 {pos = posHexNum; outToken = tokHexNum;}
617 return !outToken.empty();
620 bool NTV2DeviceSpecParser::ParseDeviceID (
size_t & pos,
string & outToken)
624 if (!ParseHexNumber(pos, tokHexNum))
626 if (tokHexNum.length() != 10)
635 ostringstream devID; devID <<
xHEX0N(*it,8);
636 string devIDStr(devID.str());
638 devIDStrs.insert(devIDStr);
640 if (devIDStrs.find(tokHexNum) != devIDStrs.end())
641 outToken = tokHexNum;
642 return !outToken.empty();
645 bool NTV2DeviceSpecParser::ParseModelName (
size_t & pos,
string & outToken)
649 if (!ParseAlphaNumeric(pos, tokName))
660 modelNames.insert(modelName);
662 if (modelNames.find(tokName) != modelNames.end())
664 return !outToken.empty();
667 bool NTV2DeviceSpecParser::ParseDNSName (
size_t & pos,
string & outDNSName)
670 string dnsName, name;
673 while (ParseAlphaNumeric(dnsPos, name,
"_-"))
675 if (!dnsName.empty())
683 if (!dnsName.empty())
685 outDNSName = dnsName;
686 return !outDNSName.empty();
689 bool NTV2DeviceSpecParser::ParseIPv4Address (
size_t & pos,
string & outIPv4)
692 string ipv4Name, num;
695 while (ParseDecNumber(ipv4Pos, num))
697 if (!ipv4Name.empty())
700 ch = CharAt(ipv4Pos);
705 if (!ipv4Name.empty())
708 return !outIPv4.empty();
711 bool NTV2DeviceSpecParser::ParseHostAddressAndPortNumber (
size_t & pos,
string & outAddr,
string & outPort)
713 outAddr.clear(); outPort.clear();
715 string dnsName, ipv4, port;
716 size_t dnsPos(pos), ipv4Pos(pos), portPos(0);
717 bool isDNS(ParseDNSName(dnsPos, dnsName)), isIPv4(ParseIPv4Address(ipv4Pos, ipv4));
718 if (!isDNS && !isIPv4)
719 {pos = dnsPos < ipv4Pos ? ipv4Pos : dnsPos;
return false;}
722 {outAddr = ipv4; pos = portPos = ipv4Pos;}
724 {outAddr = dnsName; pos = portPos = dnsPos;}
727 char ch (CharAt(portPos));
731 if (!ParseDecNumber(portPos, port))
732 {pos = portPos;
return false;}
738 bool NTV2DeviceSpecParser::ParseResourcePath (
size_t & pos,
string & outRsrc)
743 char ch(CharAt(rsrcPos));
748 if (!ParseAlphaNumeric(rsrcPos, name))
751 ch = CharAt(rsrcPos);
756 return !outRsrc.empty();
759 bool NTV2DeviceSpecParser::ParseParamAssignment (
size_t & pos,
string & outKey,
string & outValue)
761 outKey.clear(); outValue.clear();
763 size_t paramPos(pos);
764 char ch(CharAt(paramPos));
766 ch = CharAt(++paramPos);
769 if (!ParseAlphaNumeric(paramPos, key))
771 ch = CharAt(paramPos);
774 ch = CharAt(++paramPos);
775 while (ch != 0 && ch !=
'&')
778 ch = CharAt(++paramPos);
782 {pos = paramPos; outKey =
key; outValue =
value;}
786 bool NTV2DeviceSpecParser::ParseQuery (
size_t & pos,
NTV2Dictionary & outParams)
790 size_t queryPos(pos);
791 char ch(CharAt(queryPos));
796 while (ParseParamAssignment(queryPos, key, value))
798 outParams.
insert(key, value);
799 ch = CharAt(queryPos);
803 if (!outParams.empty())
805 return !outParams.empty();
808 bool NTV2DeviceSpecParser::IsUpperLetter (
const char inChar)
809 {
static const string sHexDigits(
"_ABCDEFGHIJKLMNOPQRSTUVWXYZ");
810 return sHexDigits.find(inChar) != string::npos;
813 bool NTV2DeviceSpecParser::IsLowerLetter (
const char inChar)
814 {
static const string sHexDigits(
"abcdefghijklmnopqrstuvwxyz");
815 return sHexDigits.find(inChar) != string::npos;
818 bool NTV2DeviceSpecParser::IsLetter (
const char inChar,
const bool inIncludeUnderscore)
819 {
return (inIncludeUnderscore && inChar ==
'_') || IsUpperLetter(inChar) || IsLowerLetter(inChar);
822 bool NTV2DeviceSpecParser::IsDecimalDigit (
const char inChar)
823 {
static const string sDecDigits(
"0123456789");
824 return sDecDigits.find(inChar) != string::npos;
827 bool NTV2DeviceSpecParser::IsHexDigit (
const char inChar)
828 {
static const string sHexDigits(
"0123456789ABCDEFabcdef");
829 return sHexDigits.find(inChar) != string::npos;
832 bool NTV2DeviceSpecParser::IsLegalSerialNumChar (
const char inChar)
833 {
return IsLetter(inChar) || IsDecimalDigit(inChar);
837 void NTV2DeviceSpecParser::test (
void)
840 specParser.
Reset(
"1");
841 specParser.
Reset(
"00000000000000000000000000000000000000000000000000000000000000000000000000000000000001");
842 specParser.
Reset(
"corvid24");
843 specParser.
Reset(
"corvid88");
844 specParser.
Reset(
"konalhi");
845 specParser.
Reset(
"alpha");
846 specParser.
Reset(
"00T64450");
847 specParser.
Reset(
"00t6-450");
848 specParser.
Reset(
"BLATZBE0");
849 specParser.
Reset(
"0x424C41545A424530");
850 specParser.
Reset(
"0x424C415425424530");
852 specParser.
Reset(
"badscheme://1");
854 specParser.
Reset(
"ntv2local://1");
855 specParser.
Reset(
"NtV2lOcAl://00000000000000000000000000000000000000000000000000000000000000000000000000000000000001");
856 specParser.
Reset(
"NTV2Local://corvid24");
857 specParser.
Reset(
"ntv2local://corvid88");
858 specParser.
Reset(
"ntv2local://konalhi");
859 specParser.
Reset(
"ntv2local://alpha");
860 specParser.
Reset(
"ntv2local://00T64450");
861 specParser.
Reset(
"ntv2local://00t6-450");
862 specParser.
Reset(
"ntv2local://BLATZBE0");
864 specParser.
Reset(
"ntv2nub://1.2.3.4");
865 specParser.
Reset(
"ntv2nub://1.2.3.4/doc");
866 specParser.
Reset(
"ntv2nub://1.2.3.4/doc/");
867 specParser.
Reset(
"ntv2nub://1.2.3.4/doc/alpha?one&two=2&three=&four=4");
868 specParser.
Reset(
"ntv2nub://1.2.3.4/doc/?one&two=2&three=&four=4");
869 specParser.
Reset(
"ntv2nub://1.2.3.4:badport/doc?one&two=2&three=&four=4");
870 specParser.
Reset(
"ntv2nub://1.2.3.4:200/doc?one&two=2&three=&four=4");
871 specParser.
Reset(
"ntv2nub://1.2.3.4:200/doc/?one&two=2&three=&four=4");
872 specParser.
Reset(
"ntv2nub://1.2.3.4:12345");
873 specParser.
Reset(
"ntv2nub://1.2.3.4:65000/doc");
874 specParser.
Reset(
"ntv2nub://1.2.3.4:32767/doc/");
875 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc/");
876 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc/?");
877 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc?");
878 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc/?one");
879 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc?one");
880 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc/?one=");
881 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc?one=");
882 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc/?one=1");
883 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc?one=1");
884 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc/?one=1&two");
885 specParser.
Reset(
"ntv2nub://1.2.3.4/path/to/doc?one=1&two");
886 specParser.
Reset(
"ntv2nub://50.200.250.300");
887 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.com/path/to/doc/?one=1&two");
888 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.edu:badport/path/to/doc/?one=1&two");
889 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.info:5544/path/to/doc/?one=1&two");
890 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.org/path/to/doc/?one=1&two");
891 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.nz:badport/path/to/doc/?one=1&two");
892 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.au:000004/path/to/doc/?one=1&two");
893 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.ch:4/corvid88");
894 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.cn:4/00T64450");
895 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.ru:4/2");
896 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.co.uk:4/00000000000000000000000000000001");
897 specParser.
Reset(
"ntv2nub://fully.qualified.domain.name.com:4/0000000000000000000000000000000001");
898 specParser.
Reset(
"ntv2://swdevice/?"
900 "&supportlog=file%3A%2F%2F%2FUsers%2Fdemo%2FDesktop%2FAJAWatcherSupport.log"
901 "&sdram=file%3A%2F%2F%2FUsers%2Fdemo%2FDesktop%2FSDRAMsnapshot.dat");
903 #endif // defined(_DEBUG)
905 #if defined(MSWindows)
906 static string WinErrStr (
const DWORD inErr)
908 string result(
"foo");
910 const DWORD res(FormatMessage ( FORMAT_MESSAGE_ALLOCATE_BUFFER
911 | FORMAT_MESSAGE_FROM_SYSTEM
912 | FORMAT_MESSAGE_IGNORE_INSERTS,
915 MAKELANGID(LANG_NEUTRAL, SUBLANG_DEFAULT),
921 result =
reinterpret_cast<const char *
>(lpMsgBuf);
929 #if !defined(NTV2_PREVENT_PLUGIN_LOAD)
942 static bool LoadPlugin (
const string & path,
const string & folderPath,
NTV2PluginPtr & outPtr,
string & outErrMsg,
const bool inUseStdout);
945 #if defined(MSWindows)
946 NTV2Plugin (HMODULE handle,
const string & path,
const bool useStdout);
947 inline operator HMODULE()
const {
return mHandle;}
949 NTV2Plugin (
void * handle,
const string & path,
const bool useStdout);
950 inline operator void*()
const {
return mHandle;}
953 inline bool isLoaded (
void)
const {
return mHandle && !mPath.empty() ?
true :
false;}
954 void * addressForSymbol (
const string & inSymbol,
string & outErrorMsg);
960 inline bool useStdout(
void) {
return mUseStdout;}
962 #if defined(MSWindows)
974 ostringstream loadErr;
975 #if defined(AJABareMetal)
977 #elif defined(MSWindows)
979 std::wstring dllsFolderW;
981 if (!AddDllDirectory(dllsFolderW.c_str()))
983 loadErr <<
"AddDllDirectory '" << path <<
"' failed: " << WinErrStr(::GetLastError());
986 HMODULE h = ::LoadLibraryExA(LPCSTR(path.c_str()),
AJA_NULL, LOAD_LIBRARY_SEARCH_USER_DIRS);
988 loadErr <<
"Unable to open '" << path <<
"': " << WinErrStr(::GetLastError());
989 #else // MacOS or Linux
991 void * h = ::dlopen(path.c_str(), RTLD_LAZY);
994 const char * pErrorStr(::dlerror());
995 const string errStr (pErrorStr ? pErrorStr :
"");
996 loadErr <<
"Unable to open '" << path <<
"': " << errStr;
998 #endif // MacOS or Linux
999 if (!loadErr.str().empty())
1000 outErrMsg = loadErr.str();
1003 outPtr =
new NTV2Plugin(h, path, inUseStdout);
1007 NTV2Plugin::NTV2Plugin()
1025 #if defined(MSWindows)
1036 P_NOTE(
"Dynamic/shared library '" << mPath <<
"' (" <<
INSTP(mHandle) <<
") loaded, "
1043 #if defined(AJABareMetal)
1045 #elif !defined(MSWindows)
1047 #else // macOS or Linux
1048 ::FreeLibrary(mHandle);
1051 P_NOTE(
"Dynamic/shared library '" << mPath <<
"' (" <<
INSTP(mHandle) <<
") unloaded, "
1059 outErrorMsg.clear();
1062 if (inSymbolName.empty())
1066 #if defined(AJABareMetal)
1068 #elif defined(MSWindows)
1069 result =
reinterpret_cast<void*
>(::GetProcAddress(
reinterpret_cast<HMODULE
>(mHandle), inSymbolName.c_str()));
1071 err <<
"'GetProcAddress' failed for '" << inSymbolName <<
"': " << WinErrStr(::GetLastError());
1072 #else // MacOS or Linux
1073 result = ::dlsym(mHandle, inSymbolName.c_str());
1075 {
const char * pErrorStr(::dlerror());
1076 const string errStr (pErrorStr ? pErrorStr :
"");
1077 err <<
"'dlsym' failed for '" << inSymbolName <<
"': " << errStr;
1079 #endif // MacOS or Linux
1080 outErrorMsg = err.str();
1099 static void Terminate (
void);
1100 static inline void EnableDebugging (
const bool inEnable =
true) {sDebugRegistry = inEnable;}
1106 bool loadPlugin (
const string & path,
const string & folderPath,
NTV2PluginPtr & outPtr,
string & errMsg,
const bool useStdout);
1107 bool unloadPlugin (
const string & path,
string & errMsg);
1108 bool pluginIsLoaded (
const string & path);
1109 bool pluginForPath (
const string & path,
NTV2PluginPtr & outHandle);
1112 bool hasPath (
const string & path);
1113 bool indexForPath (
const string & path,
size_t & outIndex);
1114 ULWord countForPath (
const string & path);
1115 uint32_t * refConForPath (
const string & path);
1116 inline bool useStdout (
void)
const {
return DebuggingEnabled();}
1119 typedef map<string, NTV2PluginPtr> NTV2PluginMap;
1123 NTV2PluginMap mPluginMap;
1128 static void Monitor (
AJAThread * pThread,
void * pContext);
1129 void monitor (
void);
1132 static bool sDebugRegistry;
1136 AJALock PluginRegistry::sMutex;
1137 bool PluginRegistry::sDebugRegistry(
false);
1154 void PluginRegistry::Monitor (
AJAThread * pThread,
void * pContext)
1162 : mQuitMonitor(
false)
1164 P_NOTE (
"PluginRegistry " <<
INSTP(
this) <<
" constructed");
1165 mPluginCounts.reserve(256);
1166 for (
size_t num(0); num < 256; num++)
1167 mPluginCounts.push_back(0);
1168 mCompareCounts = mPluginCounts;
1169 mMonitor.
Attach(Monitor,
this);
1176 mQuitMonitor =
true;
1177 while (mMonitor.
Active())
1187 {
P_FAIL(
"empty path");
return false;}
1194 {
P_WARN(
INSTP(
this) <<
": '" << path <<
"': 'pluginForPath' returned false, but 'hasPath' returned true, count=" <<
countForPath(path));}
1197 {
P_FAIL(msg);
return false;}
1198 P_NOTE(
INSTP(
this) <<
": Dynamic/shared library '" << path <<
"' loaded");
1199 mPluginMap[path] = outPtr;
1200 mPluginPaths.push_back(path);
1201 mPluginCounts.at(mPluginPaths.size()-1) = 0;
1212 {
P_FAIL(
INSTP(
this) <<
": '" << path <<
"' requested to unload, but not loaded");
return false;}
1213 mPluginMap.erase(path);
1214 P_NOTE(
INSTP(
this) <<
": '" << path <<
"' unloaded");
1221 return mPluginMap.find(path) != mPluginMap.end();
1227 NTV2PluginMap::const_iterator it(mPluginMap.find(path));
1228 if (it == mPluginMap.end())
1231 outHandle = it->second;
1239 for (NTV2PluginMap::const_iterator it(mPluginMap.begin()); it != mPluginMap.end(); ++it)
1240 result.push_back(it->first);
1248 for (
size_t ndx(0); ndx < mPluginPaths.size(); ndx++)
1250 const string path (mPluginPaths.at(ndx));
1254 oss <<
"\t" << (p->
isLoaded() ?
"loaded" :
"unloaded");
1256 oss <<
"\t" <<
"---";
1257 result.push_back(oss.str());
1271 for (outIndex = 0; outIndex < mPluginPaths.size(); outIndex++)
1272 if (path == mPluginPaths.at(outIndex))
1282 return &mPluginCounts[ndx];
1290 return mPluginCounts.at(ndx);
1294 void PluginRegistry::monitor (
void)
1296 P_NOTE(
"PluginRegistry " <<
INSTP(
this) <<
" monitor started");
1297 while (!mQuitMonitor)
1301 for (
size_t ndx(0); ndx < mPluginPaths.size(); ndx++)
1303 const uint32_t oldCount(mCompareCounts.at(ndx)), newCount(mPluginCounts.at(ndx));
1304 if (newCount != oldCount)
1306 string errMsg, path(mPluginPaths.at(ndx));
1307 if (newCount > oldCount)
1308 {
P_NOTE(
"PluginRegistry " <<
INSTP(
this) <<
": Plugin '" << path <<
"' utilization "
1309 <<
"increased from " <<
DEC(oldCount) <<
" to " <<
DEC(newCount));}
1312 P_NOTE(
"PluginRegistry " <<
INSTP(
this) <<
": Plugin '" << path <<
"' utilization "
1313 <<
"decreased from " <<
DEC(oldCount) <<
" to " <<
DEC(newCount));
1317 mCompareCounts.at(ndx) = newCount;
1323 P_NOTE(
"PluginRegistry " <<
INSTP(
this) <<
" monitor stopped");
1341 void *
refCon (
void)
const;
1345 void *
getSymbolAddress (
const string & inSymbolName,
string & outErrorMsg);
1348 inline bool isOpen (
void) {
return mpPlugin ? mpPlugin->
isLoaded() :
false;}
1359 mutable string errMsg;
1365 static string mbedErrStr (
const int mbedtlsReturnCode);
1393 { cout <<
"## NOTE: Original params for '" <<
pluginPath() <<
"':" << endl;
1394 originalParams.
Print(cout,
false) << endl;
1399 { cout <<
"## NOTE: Final params for '" <<
pluginPath() <<
"':" << endl;
1400 mDict.
Print(cout,
false) << endl;
1416 mbedtls_strerror (mbedtlsReturnCode, errBuff, errBuff);
1428 for (
size_t lineNdx(0); lineNdx < lines.size(); lineNdx++)
1430 string line (lines.at(lineNdx));
1431 const bool indented (line.empty() ?
false : line.at(0) ==
' ');
1436 if (keyValPair.size() != 2)
1438 if (keyValPair.size() == 1)
1440 keyPrefix = keyValPair.at(0);
1445 PLGFAIL(
"cert info line " <<
DEC(lineNdx+1) <<
" '" << line <<
"' has "
1446 <<
DEC(keyValPair.size()) <<
" column(s) -- expected 2");
1449 string key(keyValPair.at(0)), val(keyValPair.at(1));
1451 {
PLGFAIL(
"cert info line " <<
DEC(lineNdx+1) <<
" '" << line <<
"' empty key for value '" << val <<
"'");
continue;}
1452 if (indented && !keyPrefix.empty())
1453 {
aja::strip(key); key = keyPrefix +
": " + key;}
1456 if (outInfo.hasKey(key))
1471 for (
size_t ndx(0); ndx < pairs.size(); ndx++)
1473 string assignment (pairs.at(ndx));
1474 if (assignment.find(
'=') == string::npos)
1476 if (!lastKey.empty())
1482 if (pieces.size() != 2)
1483 {
PLGFAIL(
"'" << inParentKey <<
"' assignment '" << assignment <<
"' has " << pieces.size() <<
" component(s) -- expected 2");
continue;}
1484 lastKey = pieces.at(0);
1485 string val(pieces.at(1));
1497 if (!queryStr.empty())
1498 if (queryStr[0] ==
'?')
1499 queryStr.erase(0,1);
1503 string str(*it), key, value;
1504 if (str.find(
"=") == string::npos)
1507 outQueryParams.
insert(key, value);
1508 PLGDBG(
"'" << key <<
"' = ''");
1515 if (pieces.size() > 1)
1516 value = pieces.at(1);
1518 {
PLGWARN(
"Empty key '" << key <<
"'");
continue;}
1519 if (outQueryParams.hasKey(key))
1520 PLGDBG(
"Param '" << key <<
"' value '" << outQueryParams.
valueForKey(key) <<
"' to be replaced with '" << value <<
"'");
1529 outErrorMsg.clear();
1547 if (outPath.empty())
1549 PLGDBG(
"AJA firmware path is '" << outPath <<
"'");
1551 {
P_FAIL(
"'" << outPath <<
"' doesn't end with '" <<
FIRMWARE_FOLDER <<
"'"); outPath.clear();
return false;}
1555 outPath.erase(outPath.length() - 1, 1);
1556 return !outPath.empty();
1566 {
P_FAIL(
"Missing scheme -- params: " << mDict);
return false;}
1570 return !outName.empty();
1591 const size_t maxBufSize = 512*1024*1024;
1594 dllF.open(
pluginPath(), std::ios::in | std::ios::binary);
1599 ifstream::pos_type curOffset(dllF.tellg());
1600 if (
int(curOffset) == -1)
1602 size_t size = size_t(curOffset);
1605 if (size > maxBufSize)
1606 {
P_FAIL(
"EOF not reached in plugin file '" <<
pluginPath() <<
"' -- over 500MB in size?");
return fail();}
1610 if (!dllF.seekg(0, ios_base::beg))
1611 {
P_FAIL(
"Could not seek back to start of plugin file '" <<
pluginPath() <<
"'");
return fail();}
1613 {
P_FAIL(
"EOF not reached in plugin file '" <<
pluginPath() <<
"' -- over 500MB in size?");
return fail();}
1614 tmp.
Truncate(
size_t(dllF.gcount()));
1618 ifstream sigF(
pluginSigPath().c_str(), std::ios::in | std::ios::binary);
1622 {
P_FAIL(
"EOF not reached in signature file '" <<
pluginSigPath() <<
"' -- over 500MB in size?");
return fail();}
1623 tmp.
Truncate(
size_t(sigF.gcount()));
1634 NTV2Buffer checksumFromSigFile, x509CertFromSigFile, signature;
1645 mbedtls_x509_crt crt;
1646 mbedtls_x509_crt_init(&crt);
1647 int ret = mbedtls_x509_crt_parse(&crt, x509CertFromSigFile, x509CertFromSigFile);
1650 mbedtls_x509_crt_free(&crt);
1658 int msgLength (mbedtls_x509_crt_info (msgBuff, msgBuff,
"", &crt));
1659 string msg (msgBuff,
size_t(msgLength));
1661 {
P_FAIL(
"'mbedtls_x509_crt_info' returned no info for X509 cert found in '" <<
pluginSigPath() <<
"'");
1665 cout <<
"## DEBUG: Raw X509 certificate info extracted from signature file '" <<
pluginSigPath() <<
"':" << endl
1666 <<
" " << msg << endl;
1670 { cout <<
"## NOTE: X509 certificate info extracted from signature file '" <<
pluginSigPath() <<
"':" << endl;
1671 certInfo.
Print(cout,
false) << endl;
1673 if (certInfo.hasKey(
"issuer name"))
1676 if (certInfo.hasKey(
"subject name"))
1684 { cout <<
"## NOTE: 'issuer name' info:" << endl;
1685 issuerInfo.
Print(cout,
false) << endl;
1687 if (
isVerbose() && !subjectInfo.empty())
1688 { cout <<
"## NOTE: 'subject name' info:" << endl;
1689 subjectInfo.
Print(cout,
false) << endl;
1708 ret = mbedtls_md_file (mbedtls_md_info_from_type(MBEDTLS_MD_SHA256),
pluginPath().c_str(), checksumFromDLL);
1713 if (
isVerbose()) {
string str;
if (checksumFromDLL.
toHexString(str)) cout <<
"## DEBUG: Digest: " << str << endl;}
1716 ret = mbedtls_pk_verify (&crt.pk, MBEDTLS_MD_SHA256,
1718 signature, signature);
1723 mbedtls_x509_crt_free(&crt);
1724 P_DBG(
"'mbedtls_pk_verify' succeeded for '" <<
pluginPath() <<
"' -- signature valid");
1744 if (regInfo.empty())
1753 for (
size_t ndx(0); ndx < reqKeys.size(); ndx++)
1754 if (!regInfo.hasKey(reqKeys.at(ndx)))
1755 missingRegInfoKeys.push_back(reqKeys.at(ndx));
1756 if (!missingRegInfoKeys.empty())
1757 {
P_FAIL(
"'" <<
pluginPath() <<
"': missing key(s) in registration info: '"
1758 <<
aja::join(missingRegInfoKeys,
"','") <<
"'");
1775 if (onReg != onCert)
1778 <<
"') \"" << onCert <<
"\" from X509 certificate 'Issuer' in '" <<
pluginSigPath() <<
"'");
1781 if (cnReg != cnCert)
1784 <<
"') \"" << cnCert <<
"\" from X509 certificate 'Issuer' in '" <<
pluginSigPath() <<
"'");
1787 if (ouReg != ouCert)
1790 <<
"') \"" << ouCert <<
"\" from X509 certificate 'Subject' in '" <<
pluginSigPath() <<
"'");
1793 if (myVers != plVers)
1795 <<
"\" doesn't match client SDK version '" << myVers <<
"'");
1798 if (fingerprint != ajaFingerprint)
1800 <<
"Issuer serial: " << fingerprint <<
"|AJA serial: " << ajaFingerprint);
1829 return mpPlugin && mValidated;
1836 cout <<
"0 plugins" << endl;
1837 else if (paths.size() == 1)
1838 cout <<
"1 plugin: " << paths.at(0) << endl;
1839 else cout <<
DEC(paths.size()) <<
" plugins:" << endl <<
aja::join(paths,
"\n") << endl;
1841 #endif // !defined(NTV2_PREVENT_PLUGIN_LOAD)
1859 {cout << __FILE__ <<
"(" << __LINE__ <<
"):" <<
AJAFUNC <<
":" << endl;
mParams.
Print(cout,
false) << endl;}
1874 size_t oldCount(
mParams.size()), updated(0), added(0);
1884 NBSDBG(
DEC(oldCount) <<
" param(s) removed, replaced with " << inNewParams);
1898 while (nums.size() > 3)
1906 static const string sAJAFingerprint (
"70:1A:37:93:FA:4F:34:30:58:55:51:0C:01:4E:45:7C:BE:5B:41:65");
1907 string result(sAJAFingerprint);
1945 return mParams.hasKey(inParam);
1961 oss << (IsConnected() ?
"Connected" :
"Disconnected");
1962 if (IsConnected() && !Name().empty())
1963 oss <<
" to '" << Name() <<
"'";
1995 { (
void) autoCircData;
2000 { (
void) eInterrupt; (
void) timeOutMs;
2004 #if !defined(NTV2_DEPRECATE_16_3)
2006 { (
void) bitFileType;
2007 ::memset(&bitFileInfo, 0,
sizeof(bitFileInfo));
2013 ::memset(&buildInfo, 0,
sizeof(buildInfo));
2018 const UWord signalMask,
const bool testPatDMAEnb,
const ULWord testPatNum)
2019 { (
void) channel; (
void) testPatternFBF; (
void) signalMask; (
void) testPatDMAEnb; (
void) testPatNum;
2024 { (
void) numRegs; (
void) outFailedRegNum; (
void) outRegs;
2030 outDriverVersion = 0xFFFFFFFF;
2033 #endif // !defined(NTV2_DEPRECATE_16_3)
2037 const ULWord inNumSegments,
const ULWord inSegmentHostPitch,
2038 const ULWord inSegmentCardPitch,
const bool inSynchronous)
2039 { (
void) inDMAEngine; (
void) inIsRead; (
void) inFrameNumber; (
void) inOutFrameBuffer;
2040 (
void) inCardOffsetBytes; (
void) inNumSegments; (
void) inSegmentHostPitch;
2041 (
void) inSegmentCardPitch; (
void) inSynchronous;
2046 { (
void) pInMessage;
2064 outSupported.clear();
2082 #if defined(NTV2_PREVENT_PLUGIN_LOAD)
2110 #if defined(NTV2_PREVENT_PLUGIN_LOAD)
2134 if (parser.HasErrors())
2182 return mParams.hasKey(inParam);
virtual bool NTV2WriteRegisterRemote(const ULWord regNum, const ULWord regValue, const ULWord regMask, const ULWord regShift)
virtual bool NTV2GetNumericParamRemote(const ULWord inParamID, ULWord &outValue)
#define kNTV2PluginRegInfoKey_ShortName
Plugin short name.
unsigned long stoul(const std::string &str, std::size_t *idx, int base)
@ value
the parser finished reading a JSON value
static void Terminate(void)
static NTV2RPCServerAPI * CreateServer(NTV2ConfigParams &inParams)
Factory method that instantiates a new NTV2RPCServerAPI instance using a plugin based on the specifie...
virtual std::string ConfigParam(const std::string &inParam) const
static bool DebuggingEnabled(void)
#define kLegalSchemeNTV2Local
void Reset(const std::string inSpec="")
Resets me, then parses the given device specification.
static string mbedErrStr(const int mbedtlsReturnCode)
virtual bool NTV2OpenRemote(void)
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
#define kNTV2PluginRegInfoKey_Vendor
Plugin vendor (manufacturer) name.
bool unloadPlugin(const string &path, string &errMsg)
#define kQParamShowX509Cert
Query parameter option that dumps X509 certificate info into message log.
size_t addFrom(const NTV2Dictionary &inDict)
Adds all values from inDict with non-matching keys, ignoring all matching keys.
#define kNTV2PluginRegInfoKey_Copyright
Plugin copyright notice.
virtual bool NTV2Disconnect(void)
Disconnects me from the remote/fake host, closing the connection.
static std::string AJAFingerprint(const bool inLowerCase=false, const bool inStripColons=false)
std::set< std::string > NTV2StringSet
virtual bool NTV2DriverGetBitFileInformationRemote(BITFILE_INFO_STRUCT &bitFileInfo, const NTV2BitFileType bitFileType)
std::string & strip(std::string &str, const std::string &ws)
ULWord countForPath(const string &path)
#define kQParamDebugRegistry
Query parameter option that enables debugging of PluginRegistry.
NTV2DeviceIDSet NTV2GetSupportedDevices(const NTV2DeviceKinds inKinds=NTV2_DEVICEKIND_ALL)
Returns an NTV2DeviceIDSet of devices supported by the SDK.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
Describes a user-space buffer on the host computer. I have an address and a length,...
NTV2Plugin(void *handle, const string &path, const bool useStdout)
std::string join(const std::vector< std::string > &parts, const std::string &delim)
void split(const std::string &str, const char delim, std::vector< std::string > &elems)
ULWord GetByteCount(void) const
#define NTV2_ASSERT(_expr_)
static uint32_t gBaseDestructCount(0)
NTV2RPCClientAPI *(* fpCreateClient)(void *, const NTV2ConnectParams &, const uint32_t)
Instantiates a new client instance to talk to a remote server.
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
static bool ExtractIssuerInfo(NTV2Dictionary &outInfo, const string &inStr, const string &inParentKey)
NTV2RPCServerAPI *(* fpCreateServer)(void *, const NTV2ConfigParams &, const uint32_t)
Instantiates a new server instance for talking to clients.
#define kConnectParamDevID
First device having this ID (e.g. '0x10518400')
#define kNTV2PluginInfoKey_PluginSigPath
Local host full path to plugin signature file.
Declares the AJATime class.
Base class of objects that can serve device operation RPCs with NTV2RPCClientAPI instances.
Common base class for NTV2RPCClientAPI and NTV2RPCServerAPI.
#define kNTV2PluginX500AttrKey_OrganizationName
virtual ~NTV2RPCClientAPI()
My destructor, automatically calls NTV2Disconnect.
virtual bool NTV2DMATransferRemote(const NTV2DMAEngine inDMAEngine, const bool inIsRead, const ULWord inFrameNumber, NTV2Buffer &inOutBuffer, const ULWord inCardOffsetBytes, const ULWord inNumSegments, const ULWord inSegmentHostPitch, const ULWord inSegmentCardPitch, const bool inSynchronous)
bool SetParams(const NTV2ConfigParams &inNewParams, const bool inAugment=false)
bool showParams(void) const
std::string NTV2GetFirmwareFolderPath(void)
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
NTV2DeviceID DeviceID(void) const
static uint32_t gPluginConstructCount(0)
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
std::ostream & Print(std::ostream &oss, const bool inCompact=true) const
Prints human-readable representation to ostream.
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
#define kNTV2PluginInfoKey_Errors
Plugin load or validation error(s), if any.
virtual bool ConnectHasScheme(void) const
NTV2DeviceIDSet::const_iterator NTV2DeviceIDSetConstIter
A convenient const iterator for NTV2DeviceIDSet.
bool insert(const std::string &inKey, const std::string &inValue)
Stores the given value using the given key; overwrites existing value if already present.
bool toHexString(std::string &outStr, const size_t inLineBreakInterval=0) const
Converts my contents into a hex-encoded string.
#define kConnectParamPort
Port number (optional)
NTV2RPCServerAPI(NTV2ConnectParams inParams, void *pRefCon)
My constructor.
virtual bool HasConnectParam(const std::string &inParam) const
virtual bool NTV2GetDriverVersionRemote(ULWord &outDriverVersion)
AJARefPtr< NTV2Plugin > NTV2PluginPtr
void * addressForSymbol(const string &inSymbol, string &outErrorMsg)
#define kFuncNameCreateServer
Create an NTV2RPCServerAPI instance.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
std::ostream & Print(std::ostream &oss, const bool inDumpResults=false) const
virtual bool NTV2CloseRemote(void)
std::string Resource(const bool inStripLeadSlash=true) const
@ key
the parser read a key of a value in an object
#define kConnectParamDevModel
First device of this model (e.g. 'kona4')
static uint32_t gServerDestructCount(0)
size_t updateFrom(const NTV2Dictionary &inDict)
Updates all values from inDict with matching keys, ignoring all non-matching keys.
uint32_t mSpare[1024]
Reserved.
NTV2Dictionary mParams
Copy of config params passed to my constructor.
#define kNTV2PluginSigFileKey_X509Certificate
X509 certificate (encoded as hex string)
string pluginPath(void) const
virtual NTV2ConnectParams ConnectParams(void) const
std::string & lower(std::string &str)
#define kNTV2PluginX500AttrKey_OrganizationalUnitName
#define kQParamLogToStdout
Query parameter option that logs messages to standard output.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
#define kNTV2PluginRegInfoKey_CommonName
Plugin vendor domain name.
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
std::string & replace(std::string &str, const std::string &from, const std::string &to)
virtual bool NTV2GetBoolParamRemote(const ULWord inParamID, ULWord &outValue)
virtual std::string ConnectParam(const std::string &inParam) const
#define kNTV2PluginInfoKey_Fingerprint
Issuer cert fingerprint.
NTV2PluginLoader(NTV2Dictionary ¶ms)
#define kConnectParamHost
DNS name, IPv4 or sw device DLL name.
virtual NTV2ConfigParams ConfigParams(void) const
virtual std::ostream & Print(std::ostream &oss) const
virtual bool HasConfigParam(const std::string &inParam) const
static PluginRegistry & Get(void)
static bool ParseQueryParams(const NTV2Dictionary &inParams, NTV2Dictionary &outQueryParams)
static std::string ShortSDKVersion(void)
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
virtual bool NTV2DriverGetBuildInformationRemote(BUILD_INFO_STRUCT &buildInfo)
bool mTerminate
Set true to stop server.
NTV2StringList loadedPlugins(void)
#define kNTV2PluginInfoKey_PluginPath
Local host full path to plugin file.
static bool ExtractCertInfo(NTV2Dictionary &outInfo, const string &inStr)
bool deserialize(const std::string &inStr)
Resets me from the given string.
virtual std::string Description(void) const
bool showCertificate(void) const
static uint32_t gBaseConstructCount(0)
#define kNTV2PluginRegInfoKey_OrgUnit
Plugin organization unit (to match certificate subject OU)
void DumpLoadedPlugins(void)
All new NTV2 structs start with this common header.
std::string & upper(std::string &str)
virtual ~NTV2RPCServerAPI()
My destructor, automatically calls NTV2Disconnect.
NTV2DeviceSpecParser(const std::string inSpec="")
My constructor. If given device specification is non-empty, proceeds to Parse it.
bool string_to_wstring(const std::string &str, std::wstring &wstr)
#define kNTV2PluginInfoKey_PluginBaseName
Plugin base name (i.e. without extension)
Declares numerous NTV2 utility functions.
string pluginsPath(void) const
std::string NTV2Version(const bool inDetailed=false)
NTV2StringList pluginStats(void)
bool getBaseNameFromScheme(string &outName) const
virtual bool NTV2DownloadTestPatternRemote(const NTV2Channel channel, const NTV2PixelFormat testPatternFBF, const UWord signalMask, const bool testPatDMAEnb, const ULWord testPatNum)
UWord DeviceIndex(void) const
#define AJA_NTV2_SDK_VERSION
bool useStdout(void) const
NTV2RPCBase(NTV2Dictionary params, uint32_t *pRefCon)
bool GetString(std::string &outString, const size_t inU8Offset=0, const size_t inMaxSize=128) const
Answers with my contents as a character string.
AJALock mParamLock
Mutex to protect mParams.
unsigned long long stoull(const std::string &str, std::size_t *idx, int base)
static bool LoadPlugin(const string &path, const string &folderPath, NTV2PluginPtr &outPtr, string &outErrMsg, const bool inUseStdout)
Defines for the NTV2 SDK version number, used by ajantv2/includes/ntv2enums.h. See the ajantv2/includ...
Dict::const_iterator DictConstIter
#define kNTV2PluginX500AttrKey_CommonName
std::string PercentDecode(const std::string &inStr)
uint16_t u16ValueForKey(const std::string &inKey, const uint16_t inDefault=0) const
bool loadPlugin(const string &path, const string &folderPath, NTV2PluginPtr &outPtr, string &errMsg, const bool useStdout)
std::vector< std::string > NTV2StringList
#define kConnectParamScheme
URL scheme.
static AJAStatus Open(bool incrementRefCount=false)
#define kNTV2PluginRegInfoKey_Description
Brief plugin description.
bool pluginIsLoaded(const string &path)
virtual bool NTV2AutoCirculateRemote(AUTOCIRCULATE_DATA &autoCircData)
bool isLoaded(void) const
virtual bool NTV2WaitForInterruptRemote(const INTERRUPT_ENUMS eInterrupt, const ULWord timeOutMs)
One-stop shop for parsing device specifications. (New in SDK 16.3) I do very little in the way of val...
static uint32_t gClientConstructCount(0)
virtual void RunServer(void)
Principal server thread function, subclsses should override.
void * GetHostPointer(void) const
#define kNTV2PluginRegInfoKey_NTV2SDKVersion
NTV2 SDK version that plugin was compiled with.
static int32_t Increment(int32_t volatile *pTarget)
#define kConnectParamDevIndex
Device having this index number.
string pluginBaseName(void) const
#define kFuncNameCreateClient
Create an NTV2RPCClientAPI instance.
bool serialize(std::string &outStr) const
Serializes my contents into the given string.
bool pluginForPath(const string &path, NTV2PluginPtr &outHandle)
#define kQParamShowParams
Query parameter option that dumps parameters into message log.
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
Private include file for all ajabase sources.
std::ostream & PrintErrors(std::ostream &oss) const
bool SetFromHexString(const std::string &inStr)
Replaces my contents from the given hex-encoded string, resizing me if necessary.
bool hasPath(const string &path)
static NTV2RPCClientAPI * CreateClient(NTV2ConnectParams &inParams)
Instantiates a new NTV2RPCClientAPI instance using the given NTV2ConnectParams.
AJARefPtr< PluginRegistry > PluginRegistryPtr
static uint32_t gClientDestructCount(0)
virtual bool NTV2Connect(void)
#define kFuncNameGetRegInfo
Answers with plugin registration info.
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
#define kNTV2PluginSigFileKey_Signature
X509 digital signature (encoded as hex string)
#define AJA_sERROR(_index_, _expr_)
@ AJA_DebugUnit_Application
static uint32_t gLoaderConstructCount(0)
#define kNTV2PluginInfoKey_PluginsPath
Local host full path to folder containing plugins.
virtual bool NTV2GetSupportedRemote(const ULWord inEnumsID, ULWordSet &outSupported)
#define kConnectParamResource
Resource path – everything past URL [scheme://host[:port]/], excluding [?query].
std::set< NTV2DeviceID > NTV2DeviceIDSet
A set of NTV2DeviceIDs.
static void EnableDebugging(const bool inEnable=true)
NTV2StringList::const_iterator NTV2StringListConstIter
bool Truncate(const size_t inByteCount)
Truncates me to the given length. No reallocation takes place.
void * refCon(void) const
virtual bool NTV2MessageRemote(NTV2_HEADER *pInMessage)
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool NTV2ReadRegisterMultiRemote(const ULWord numRegs, ULWord &outFailedRegNum, NTV2RegInfo outRegs[])
static uint32_t gPluginDestructCount(0)
Declares enums and structs used by all platform drivers and the SDK.
bool indexForPath(const string &path, size_t &outIndex)
static uint32_t gServerConstructCount(0)
#define kConnectParamQuery
Query – everything past '?' in URL.
size_t largestKeySize(void) const
virtual AJAStatus Start()
virtual std::ostream & Print(std::ostream &oss) const
#define kNTV2PluginRegInfoKey_Version
Plugin version (string)
static uint32_t gLoaderDestructCount(0)
#define xHEX0N(__x__, __n__)
bool isValidated(void) const
#define kConnectParamDevSerial
Device with this serial number.
bool(* fpGetRegistrationInfo)(const uint32_t, NTV2Dictionary &)
Obtains a plugin's registration information. Starting in SDK 17.1, all plugins must implement this fu...
bool getPluginsFolder(string &outPath) const
string pluginSigPath(void) const
An object that can connect to, and operate remote or fake devices. I have three general API groups:
bool isVerbose(void) const
std::string valueForKey(const std::string &inKey) const
Declares the AJAThread class.
uint32_t * mpRefCon
Reserved for internal use.
size_t largestValueSize(void) const
NTV2StringSet keys(void) const
A simple (not thread-safe) set of key/value pairs. (New in SDK 16.3)
void * getFunctionAddress(const string &inFuncName)
std::string InfoString(void) const
#define AJA_sDEBUG(_index_, _expr_)
virtual bool NTV2ReadRegisterRemote(const ULWord regNum, ULWord &outRegValue, const ULWord regMask, const ULWord regShift)
Declares NTV2 "nub" client functions.
bool useStdout(void) const
uint32_t * refConForPath(const string &path)
Declares the AJADebug class.
static int32_t Decrement(int32_t volatile *pTarget)
NTV2RPCClientAPI(NTV2ConnectParams inParams, void *pRefCon)
My constructor.
void * getSymbolAddress(const string &inSymbolName, string &outErrorMsg)
#define kQParamVerboseLogging
Query parameter option that enables verbose message logging.
#define kNTV2PluginRegInfoKey_LongName
Plugin long name.