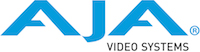 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
8 #ifndef NTV2_DEVICECAPABILITIES_H
9 #define NTV2_DEVICECAPABILITIES_H
24 inline operator bool()
const {
return dev.IsOpen();}
71 inline bool CanDoIP (
void) {
return dev.IsSupported(
kDeviceCanDoIP);}
121 inline bool HasNWL (
void) {
return dev.IsSupported(
kDeviceHasNWL);}
134 inline bool Is64Bit (
void) {
return dev.IsSupported(
kDeviceIs64Bit);}
201 return itms.find(
ULWord(inChannel)) != itms.end();
210 return itms.find(
ULWord(inMode)) != itms.end();
219 return itms.find(
ULWord(inMode)) != itms.end();
228 return itms.find(
ULWord(inPF)) != itms.end();
238 return itms.find(
ULWord(inSrc)) != itms.end();
247 return itms.find(
ULWord(inDest)) != itms.end();
256 return itms.find(
ULWord(inVF)) != itms.end();
265 return itms.find(
ULWord(inWgtID)) != itms.end();
325 return itms.find(
ULWord(inTCNdx)) != itms.end();
334 return itms.find(
ULWord(inTCNdx)) != itms.end();
340 #endif // NTV2_DEVICECAPABILITIES_H
@ kDeviceCanDoMSI
True if device DMA hardware supports MSI (Message Signaled Interrupts).
@ kDeviceGetNumVideoInputs
The number of SDI video inputs on the device.
@ kDeviceHasBiDirectionalSDI
True if device SDI connectors are bi-directional.
@ kDeviceCanDoAudioOutput
True if device has any audio output capability (SDI, HDMI or analog) (New in SDK 17....
@ kDeviceCanDoAudio6Channels
True if audio system(s) support 6 or more audio channels.
@ kDeviceCanDoPIO
True if device supports Programmed I/O.
@ kDeviceCanDoStackedAudio
True if device uses a "stacked" arrangement of its audio buffers.
@ kDeviceGetNumMixers
The number of mixer/keyer widgets on the device.
@ kDeviceGetNumFrameStores
The number of FrameStores on the device.
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
@ kDeviceCanMeasureTemperature
True if device can measure its FPGA die temperature.
@ kDeviceHasSPIv4
Use kDeviceGetSPIVersion instead.
@ kNTV2EnumsID_OutputTCIndex
Identifies the NTV2TCIndex enumerated type for output.
@ kDeviceCanDoIP
True if device has SFP connectors.
NTV2OutputDestination
Identifies a specific video output destination.
@ kDeviceCanDoHDMIMultiView
True if device can rasterize 4 HD signals into a single HDMI output.
@ kDeviceCanDoVideoProcessing
True if device can do video processing.
@ kDeviceGetSPIFlashVersion
The SPI-flash version on the device. (New in SDK 17.1)
NTV2FrameBufferFormatSet NTV2PixelFormats
@ kNTV2EnumsID_VideoFormat
Identifies the NTV2VideoFormat enumerated type.
@ kDeviceGetUFCVersion
The version number of the UFC on the device.
@ kDeviceHasXptConnectROM
True if device has a crosspoint connection ROM (New in SDK 17.0)
@ kDeviceGetNumDownConverters
The number of down-converters on the device.
std::set< NTV2TCIndex > NTV2TCIndexes
@ kDeviceCanDoHDV
True if device can squeeze/stretch between 1920x1080 and 1440x1080.
@ kDeviceCanDoSDIErrorChecks
True if device can perform SDI error checking.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
@ kDeviceGetNumAnalogAudioInputChannels
The number of analog audio input channels on the device.
std::set< NTV2VideoFormat > NTV2VideoFormatSet
A set of distinct NTV2VideoFormat values.
@ kDeviceGetNumEmbeddedAudioOutputChannels
The number of SDI-embedded output audio channels supported by the device.
bool CanDoInputTCIndex(const NTV2TCIndex inTCNdx)
@ kDeviceCanDoAudio192K
True if Audio System(s) support a 192kHz sample rate.
bool CanDoDSKMode(const NTV2DSKMode inMode)
@ kDeviceIsSupported
True if device is supported by this SDK.
@ kDeviceHasGenlockv3
True if device has version 3 genlock hardware and/or firmware.
@ kDeviceCanDoEnhancedCSC
True if device has enhanced CSCs.
@ kDeviceHasHeadphoneJack
True if device has a headphone jack.
@ kDeviceCanDo4KVideo
True if the device can handle 4K/UHD video.
@ kNTV2EnumsID_OutputDest
Identifies the NTV2OutputDest enumerated type.
@ kDeviceGetNumReferenceVideoInputs
The number of external reference video inputs on the device.
@ kDeviceCanReportRunningFirmwareDate
True if device can report its running (and not necessarily installed) firmware date.
@ kDeviceCanDoAudio96K
True if Audio System(s) support a 96kHz sample rate.
@ kDeviceCanDo3GLevelConversion
True if device can do 3G level B to 3G level A conversion.
@ kDeviceHasAudioMonitorRCAJacks
True if device has a pair of unbalanced RCA audio monitor output connectors.
@ kDeviceCanDoQuarterExpand
True if device can handle quarter-sized frames (pixel-halving and line-halving during input,...
NTV2VideoFormatSet VideoFormats(void)
NTV2TCIndexes OutputTCIndexes(void)
@ kDeviceGetNumVideoOutputs
The number of SDI video outputs on the device.
@ kDeviceGetNum4kQuarterSizeConverters
The number of quarter-size 4K/UHD down-converters on the device.
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ kDeviceCanDoFramePulseSelect
True if device supports frame pulse source independent of reference source.
@ kDeviceCanDo12gRouting
True if device supports 12G routing crosspoints.
@ kDeviceHasSPIv2
Use kDeviceGetSPIVersion instead.
@ kDeviceCanThermostat
True if device fan can be thermostatically controlled.
Convenience class/API for inquiring about device capabilities of physical and virtual devices....
NTV2TCIndexes InputTCIndexes(void)
@ kDeviceHasNTV4FrameStores
True if device has NTV4 FrameStores. (New in SDK 17.0)
@ kDeviceGetNumAESAudioOutputChannels
The number of AES/EBU audio output channels on the device.
@ kDeviceCanDoAudioMixer
True if device has a firmware audio mixer.
@ kDeviceCanDoFrameStore1Display
True if device can display/output video from FrameStore 1.
NTV2FrameBufferFormat NTV2PixelFormat
An alias for NTV2FrameBufferFormat.
@ kDeviceCanDoColorCorrection
True if device has any LUTs.
@ kDeviceHasPCIeGen2
True if device supports 2nd-generation PCIe.
@ kDeviceCanDoDualLink
True if device supports 10-bit RGB input/output over 2-wire SDI.
@ kNTV2EnumsID_InputTCIndex
Identifies the NTV2TCIndex enumerated type for input.
@ kDeviceCanDoWarmBootFPGA
True if device can warm-boot to load updated firmware.
@ kDeviceCanChangeFrameBufferSize
True if frame buffer sizes are not fixed.
bool CanDoOutputDestination(const NTV2OutputDestination inDest)
@ kDeviceGetNumAnalogAudioOutputChannels
The number of analog audio output channels on the device.
@ kDeviceROMHasBankSelect
True if device SPIFlash ROM is bank-selected. (New in SDK 17.1)
@ kDeviceGetNumDMAEngines
The number of DMA engines on the device.
NTV2PixelFormats PixelFormats(void)
@ kDeviceGetNumBufferedAudioSystems
The total number of audio systems on the device that can read/write audio buffer memory....
@ kDeviceGetNumLUTs
The number of LUT widgets on the device.
@ kDeviceHasSDIRelays
True if device has bypass relays on its SDI connectors.
@ kDeviceCanDoAudioDelay
True if Audio System(s) support an adjustable delay.
@ kDeviceCanDoBreakoutBoard
True if device supports an AJA breakout board. (New in SDK 17.0)
@ kDeviceGetDACVersion
The version number of the DAC on the device.
ULWordSet::const_iterator ULWordSetConstIter
@ kDeviceCanDoLTC
True if device can read LTC (Linear TimeCode) from one of its inputs.
@ kDeviceCanDoDVCProHD
True if device can squeeze/stretch between 1920x1080/1280x1080 and 1280x720/960x720.
@ kDeviceCanDoHFRRGB
True if device supports 1080p RGB at more than 50Hz frame rates.
@ kDeviceCanDoAnalogAudio
True if device has any analog inputs or outputs.
@ kDeviceCanDoProgrammableRS422
True if device has at least one RS-422 serial port, and it (they) can be programmed (for baud rate,...
@ kDeviceGetNumAudioSystems
The number of independent Audio Systems on the device.
@ kDeviceHasSPIv3
Use kDeviceGetSPIVersion instead.
@ kDeviceSoftwareCanChangeFrameBufferSize
True if device frame buffer size can be changed.
@ kDeviceCanDoThunderbolt
True if device connects to the host using a Thunderbolt cable.
@ kDeviceCanDo2110
True if device supports SMPTE ST2110.
@ kDeviceCanDoRGBLevelAConversion
True if the device can do RGB over 3G Level A.
@ kDeviceGetNum2022ChannelsSFP1
The number of 2022 channels configured on SFP 1 on the device.
@ kDeviceHasRotaryEncoder
True if device has a rotary encoder volume control.
@ kDeviceHasSPIFlashSerial
True if device has serial SPI flash hardware.
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
@ kDeviceCanDoHDMIHDROut
True if device supports HDMI HDR output.
@ kDeviceCanDoProRes
True if device can can accommodate Apple ProRes-compressed video in its frame buffers.
@ kDeviceGetNumVideoChannels
The number of video channels supported on the device.
@ kDeviceGetNumAnalogVideoInputs
The number of analog video inputs on the device.
@ kDeviceCanDoHDMIQuadRasterConversion
True if HDMI in/out supports square-division (quad) raster conversion. (New in SDK 17....
@ kNTV2EnumsID_ConversionMode
Identifies the NTV2ConversionMode enumerated type.
@ kDeviceGetLUTVersion
The version number of the LUT(s) on the device.
@ kDeviceCanDoHDMIOutStereo
True if device supports 3D/stereo HDMI video output.
@ kDeviceCanDoLTCInOnRefPort
True if device can read LTC (Linear TimeCode) from its reference input.
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
@ kDeviceCanDoPCMDetection
True if device can detect which audio channel pairs are not carrying PCM (Pulse Code Modulation) audi...
@ kDeviceIs64Bit
True if device is 64-bit addressable.
@ kDeviceGetHDMIVersion
The version number of the HDMI chipset on the device.
@ kDeviceCanDisableUFC
True if there's at least one UFC, and it can be disabled.
@ kDeviceHasXilinxDMA
True if device has Xilinx DMA hardware.
@ kDeviceGetMaxRegisterNumber
The highest register number for the device.
@ kDeviceCanDoVITC2
True if device can insert or extract RP-188/VITC2.
@ kDeviceHasHEVCM31
True if device has an HEVC M31 encoder.
@ kDeviceGetNumInputConverters
The number of input converter widgets on the device.
@ kNTV2EnumsID_InputSource
Identifies the NTV2InputSource enumerated type.
@ kDeviceHasSPIv5
Use kDeviceGetSPIVersion instead.
@ kDeviceCanDoSDVideo
True if device can handle SD (Standard Definition) video.
@ kDeviceCanDoMultiLinkAudio
True if device supports grouped audio system control.
@ kDeviceCanDoAudioInput
True if device has any audio input capability (SDI, HDMI or analog) (New in SDK 17....
@ kDeviceGetMaxTransferCount
The maximum number of 32-bit words that the DMA engine can move at a time on the device.
@ kDeviceCanDoPCMControl
True if device can mark specific audio channel pairs as not carrying PCM (Pulse Code Modulation) audi...
DeviceCapabilities(CNTV2DriverInterface &inDev)
bool CanDoInputSource(const NTV2InputSource inSrc)
@ kDeviceCanDoAnalogVideoOut
True if device has one or more analog video outputs.
@ kDeviceHasColorSpaceConverterOnChannel2
Calculate based on if NTV2_WgtCSC2 is present.
@ kDeviceCanDoBreakoutBox
True if device supports an AJA breakout box.
@ kNTV2EnumsID_WidgetID
Identifies the NTV2AudioWidgetID enumerated type.
@ kDeviceIsDirectAddressable
True if device is direct addressable.
@ kDeviceGetPingLED
The highest bit number of the LED bits in the Global Control Register on the device.
@ kDeviceCanDoStereoIn
True if device supports 3D video input over dual-stream SDI.
@ kDeviceGetNumMicInputs
The number of microphone inputs on the device.
@ kDeviceGetDownConverterDelay
The down-converter delay on the device.
@ kDeviceGetNumAESAudioInputChannels
The number of AES/EBU audio input channels on the device.
@ kDeviceHasRetailSupport
True if device is supported by AJA "retail" software (AJA ControlPanel & ControlRoom).
@ kDeviceHasGenlockv2
True if device has version 2 genlock hardware and/or firmware.
@ kDeviceGetNumFrameSyncs
The number of frame sync widgets on the device.
@ kDeviceGetNumHDMIVideoInputs
The number of HDMI video inputs on the device.
NTV2InputSource
Identifies a specific video input source.
@ kDeviceCanDoAESAudioIn
True if device has any AES audio inputs or outputs.
@ kDeviceCanDoAnalogVideoIn
True if device has one or more analog video inputs.
@ kDeviceIsLocalPhysical
True if device is local-host-attached, and not remote, software or virtual (new in SDK 17....
@ kDeviceGetNumCSCs
The number of colorspace converter widgets on the device.
@ kDeviceCanDoRGBPlusAlphaOut
True if device has CSCs capable of splitting the key (alpha) and YCbCr (fill) from RGB frame buffers ...
@ kDeviceHasIDSwitch
True if device has a mechanical identification switch. (New in SDK 17.1)
@ kDeviceCanDoCapture
True if device has any SDI, HDMI or analog video inputs.
@ kNTV2EnumsID_DSKMode
Identifies the NTV2DSKMode enumerated type.
@ kDeviceCanReportFrameSize
True if device can report its frame size.
@ kDeviceGetNumHDMIVideoOutputs
The number of HDMI video outputs on the device.
@ kDeviceHasLEDAudioMeters
True if device has LED audio meters.
@ kDeviceCanChangeEmbeddedAudioClock
@ kDeviceGetNumHDMIAudioOutputChannels
The number of HDMI audio output channels on the device.
@ kDeviceHasSPIFlash
True if device has SPI flash hardware.
@ kDeviceGetNumHDMIAudioInputChannels
The number of HDMI audio input channels on the device.
@ kDeviceNeedsRoutingSetup
True if device widget routing can be queried or changed.
@ kDeviceCanDoJ2K
True if device supports JPEG 2000 codec.
@ kDeviceCanDoStreamingDMA
True if device supports streaming DMA. (New in SDK 17.1)
@ kDeviceIsExternalToHost
True if device connects to the host with a cable.
@ kDeviceHasBreakoutBoard
True if device has attached breakout board. (New in SDK 17.0)
@ kDeviceCanDo8KVideo
True if device supports 8K video formats.
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ kDeviceHasMicrophoneInput
True if device has a microphone input connector.
@ kDeviceCanDo425Mux
True if the device supports SMPTE 425 mux control.
@ kDeviceGetNumTSIMuxers
The number of TSI muxers on the device. (New in SDK 17.0)
bool CanDoWidget(const NTV2WidgetID inWgtID)
@ kNTV2EnumsID_Channel
Identifies the NTV2Channel enumerated type.
@ kDeviceGetMaxAudioChannels
The maximum number of audio channels that a single Audio System can support on the device.
@ kDeviceCanDoRP188
True if device can insert and/or extract RP-188/VITC.
@ kDeviceCanDoAudio2Channels
True if audio system(s) support 2 or more audio channels.
bool CanDoConversionMode(const NTV2ConversionMode inMode)
@ kDeviceCanDoAESAudioOut
True if device has any AES audio output channels (New in SDK 17.1)
@ kDeviceCanDo12GSDI
True if device has 12G SDI connectors.
@ kDeviceCanDoHDVideo
True if device can handle HD (High Definition) video.
@ kDeviceCanDoCustomAnc
True if device has SDI ANC inserter/extractor firmware.
@ kDeviceGetActiveMemorySize
The size, in bytes, of the device's active RAM available for video and audio.
@ kDeviceCanDoHDMIAuxPlayback
True if device has HDMI AUX data inserter(s).
@ kDeviceGetTotalNumAudioSystems
The total number of audio systems on the device, including host audio and mixer audio systems,...
@ kDeviceHasNWL
True if device has NorthWest Logic DMA hardware.
@ kDeviceCanReportFailSafeLoaded
True if device can report if its "fail-safe" firmware is loaded/running.
@ kDeviceCanDoRateConvert
True if device can do frame rate conversion.
bool CanDoPixelFormat(const NTV2PixelFormat inPF)
@ kDeviceCanDoAudio8Channels
True if audio system(s) support 8 or more audio channels.
@ kDeviceCanDoCustomHancInsertion
True if device supports custom HANC packet insertion. (New in SDK 17.1)
@ kDeviceHasHEVCM30
True if device has an HEVC M30 encoder/decoder.
@ kDeviceCanDo2KVideo
True if device can handle 2Kx1556 (film) video.
@ kDeviceCanDoHDMIAuxCapture
True if device has HDMI AUX data extractor(s).
@ kDeviceGetNumUpConverters
The number of up-converters on the device.
@ kDeviceGetNumLUTBanks
The number of LUT banks on the device. (New in SDK 17.0)
@ kDeviceHasBiDirectionalAnalogAudio
True if device has a bi-directional analog audio connector.
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
@ kDeviceGetNumAnalogVideoOutputs
The number of analog video outputs on the device.
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
@ kDeviceGetNum2022ChannelsSFP2
The number of 2022 channels configured on SFP 2 on the device.
Declares the CNTV2DriverInterface base class.
@ kDeviceCanDoDSKOpacity
True if device mixer/keyer supports adjustable opacity.
@ kDeviceHasPWMFanControl
True if device has a PWM-controlled cooling fan. (New in SDK 17.1)
@ kDeviceGetNumOutputConverters
The number of output converter widgets on the device.
@ kDeviceGetNumEmbeddedAudioInputChannels
The number of SDI-embedded input audio channels supported by the device.
@ kDeviceGetNumSerialPorts
The number of RS-422 serial ports on the device.
@ kDeviceCanDoQREZ
True if device can handle QRez.
bool CanDoChannel(const NTV2Channel inChannel)
@ kDeviceGetNumCrossConverters
The number of cross-converters on the device.
@ kDeviceCanDoIsoConvert
True if device can do ISO conversion.
@ kDeviceCanDoMultiFormat
True if device can simultaneously handle different video formats on more than one SDI input or output...
@ kDeviceGetNumLTCOutputs
The number of analog LTC outputs on the device.
bool CanDoOutputTCIndex(const NTV2TCIndex inTCNdx)
@ kDeviceCanDoStereoOut
True if device supports 3D video output over dual-stream SDI.
@ kDeviceGetNumLTCInputs
The number of analog LTC inputs on the device.
@ kNTV2EnumsID_PixelFormat
Identifies the NTV2PixelFormat enumerated type.