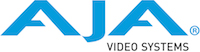 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
18 #if defined (AJAMac) || defined (AJALinux)
95 typedef pair <string, NTV2VideoFormat> String2VideoFormatPair;
96 typedef pair <string, NTV2FrameBufferFormat> String2PixelFormatPair;
97 typedef pair <string, NTV2AudioSystem> String2AudioSystemPair;
98 typedef pair <string, NTV2VANCMode> String2VANCModePair;
99 typedef pair <string, NTV2InputSource> String2InputSourcePair;
100 typedef pair <string, NTV2OutputDestination> String2OutputDestPair;
123 if (str.at (str.length() - 1) ==
'a')
124 str.erase (str.length() - 1);
126 if (str.find(
".00") != string::npos)
127 str.erase (str.find(
".00"), 3);
129 while (str.find(
" ") != string::npos)
130 str.erase (str.find(
" "), 1);
132 if (str.find(
".") != string::npos)
133 str.erase (str.find(
"."), 1);
228 while (str.find (
" ") != string::npos)
229 str.erase (str.find (
" "), 1);
231 while (str.find (
"-") != string::npos)
232 str.erase (str.find (
"-"), 1);
234 if (str.find (
"compatible") != string::npos)
235 str.erase (str.find (
"compatible"), 10);
237 if (str.find (
"ittle") != string::npos)
238 str.erase (str.find (
"ittle"), 5);
240 if (str.find (
"ndian") != string::npos)
241 str.erase (str.find (
"ndian"), 5);
248 if (str.find (
"NTV2_FBF_") == 0)
251 while (str.find (
" ") != string::npos)
252 str.erase (str.find (
" "), 1);
254 while (str.find (
"_") != string::npos)
255 str.erase (str.find (
"_"), 1);
300 for (uint8_t ndx (0); ndx < 8; ndx++)
378 string tpName(tpNames.at(tp));
381 ostringstream oss; oss <<
DEC(tp);
386 string colorName(*it);
393 string ULWordToString (
const ULWord inNum)
407 if (it != fTimecodes.end())
414 size_t errorCount(0);
435 size_t errorCount(0);
458 const string deviceSpec (inDeviceSpec.empty() ?
"0" : inDeviceSpec);
461 if (deviceSpec !=
"LIST" && deviceSpec !=
"list" && deviceSpec !=
"?")
462 cerr <<
"## ERROR: Failed to open device spec '" << deviceSpec <<
"'" << endl;
490 if (!inDevSpec.empty())
493 oss << setw(25) << left <<
"Supported Video Format" <<
"\t" << setw(16) << left <<
"Legal -v Values" << endl
494 << setw(25) << left <<
"------------------------" <<
"\t" << setw(16) << left <<
"----------------" << endl;
498 if (vfName ==
"Unknown")
502 if (vf == it->second)
506 vfNames.push_back(it->first);
508 if (!vfNames.empty())
509 oss << setw(25) << left << vfName <<
"\t" <<
aja::join(vfNames,
", ") << endl;
522 if (!inDevSpec.empty())
531 if (inStr != iter->first)
573 if (!inDevSpec.empty())
576 oss << setw(34) << left <<
"Frame Buffer Format" <<
"\t" << setw(32) << left <<
"Legal -p Values" << endl
577 << setw(34) << left <<
"----------------------------------" <<
"\t" << setw(32) << left <<
"--------------------------------" << endl;
586 if (pf == it->second)
590 pfNames.push_back(it->first);
592 if (!pfNames.empty())
593 oss << setw(35) << left << pfName <<
"\t" <<
aja::join(pfNames,
", ") << endl;
606 if (!inDevSpec.empty())
615 if (inStr != iter->first)
657 if (!inDevSpec.empty())
660 oss << setw(25) << left <<
"Input Source" <<
"\t" << setw(16) << left <<
"Legal -i Values" << endl
661 << setw(25) << left <<
"------------------------" <<
"\t" << setw(16) << left <<
"----------------" << endl;
670 if (src == it->second)
674 srcNames.push_back(it->first);
676 if (!srcNames.empty())
677 oss << setw(25) << left << srcName <<
"\t" <<
aja::join(srcNames,
", ") << endl;
690 if (!inDevSpec.empty())
699 if (inStr != iter->first)
737 if (!inDevSpec.empty())
740 oss << setw(25) << left <<
"Output Destination" <<
"\t" << setw(16) << left <<
"Legal -o Values" << endl
741 << setw(25) << left <<
"------------------------" <<
"\t" << setw(16) << left <<
"----------------" << endl;
746 if (destName.empty())
750 if (*iter == it->second)
754 destNames.push_back(it->first);
756 if (!destNames.empty())
757 oss << setw(25) << left << destName <<
"\t" <<
aja::join(destNames,
", ") << endl;
770 if (!inDevSpec.empty())
779 if (inStr != iter->first)
815 const string inDevSpec,
816 const bool inIsInputOnly)
818 const NTV2TCIndexes & tcIndexes (GetSupportedTCIndexes(inKinds));
822 if (!inDevSpec.empty())
825 oss << setw(25) << left <<
"Timecode Index" <<
"\t" << setw(16) << left <<
"Legal Values" << endl
826 << setw(25) << left <<
"------------------------" <<
"\t" << setw(16) << left <<
"----------------" << endl;
831 if (tcNdxName.empty())
835 if (tcNdx == it->second)
837 if (!inDevSpec.empty() && dev.IsOpen())
840 tcNdxNames.push_back(it->first);
842 if (!tcNdxNames.empty())
843 oss << setw(25) << left << tcNdxName <<
"\t" <<
aja::join(tcNdxNames,
", ") << endl;
856 if (!inDevSpec.empty())
866 if (inStr != iter->first)
868 tcNdx = iter->second;
893 if (!inDeviceSpecifier.empty())
900 const UWord numAudioSystems (device.
features().GetNumAudioSystems());
901 oss << setw(12) << left <<
"Audio System" << endl
902 << setw(12) << left <<
"------------" << endl;
903 for (
UWord ndx(0); ndx < 8; ndx++)
905 oss << setw(12) << left << (ndx+1);
906 if (!displayName.empty() && ndx >= numAudioSystems)
907 oss <<
"\t## Incompatible with " << displayName;
922 typedef map<string,string> NTV2StringMap;
927 if (keys.find(val) == keys.end())
931 NTV2StringMap legals;
932 for (NTV2StringSet::const_iterator kit(keys.begin()); kit != keys.end(); ++kit)
937 if (it->second == officialVM)
938 legalValues.push_back(it->first);
943 oss << setw(12) << left <<
"VANC Mode" <<
"\t" << setw(32) << left <<
"Legal --vanc Values " << endl
944 << setw(12) << left <<
"---------" <<
"\t" << setw(32) << left <<
"--------------------------------" << endl;
945 for (NTV2StringMap::const_iterator it(legals.begin()); it != legals.end(); ++it)
946 oss << setw(12) << left << it->first <<
"\t" << setw(32) << left << it->second << endl;
960 typedef map<string,string> NTV2StringMap;
963 if (keys.find(it->second) == keys.end())
964 keys.insert(it->second);
966 NTV2StringMap legals;
967 for (NTV2StringSet::const_iterator kit(keys.begin()); kit != keys.end(); ++kit)
969 const string & officialPatName(*kit);
972 if (it->second == officialPatName)
973 legalValues.push_back(it->first);
974 legals[officialPatName] =
aja::join(legalValues,
", ");
978 oss << setw(25) << left <<
"Test Pattern or Color " <<
"\t" << setw(22) << left <<
"Legal --pattern Values" << endl
979 << setw(25) << left <<
"------------------------" <<
"\t" << setw(22) << left <<
"----------------------" << endl;
980 for (NTV2StringMap::const_iterator it(legals.begin()); it != legals.end(); ++it)
981 oss << setw(25) << left << it->first <<
"\t" << setw(22) << left << it->second << endl;
988 string tpName(inStr);
997 string result(inStr);
1004 string result (inStr);
1005 while (result.find (
" ") != string::npos)
1006 result.erase (result.find (
" "), 1);
1007 while (result.find (
"00") != string::npos)
1008 result.erase (result.find (
"00"), 2);
1009 while (result.find (
".") != string::npos)
1010 result.erase (result.find (
"."), 1);
1018 #if defined (AJAMac) || defined (AJALinux)
1019 struct termios terminalStatus;
1020 ::memset (&terminalStatus, 0,
sizeof (terminalStatus));
1021 if (::tcgetattr (0, &terminalStatus) < 0)
1022 cerr <<
"tcsetattr()";
1023 terminalStatus.c_lflag &= ~uint32_t(ICANON);
1024 terminalStatus.c_lflag &= ~uint32_t(ECHO);
1025 terminalStatus.c_cc[VMIN] = 1;
1026 terminalStatus.c_cc[VTIME] = 0;
1027 if (::tcsetattr (0, TCSANOW, &terminalStatus) < 0)
1028 cerr <<
"tcsetattr ICANON";
1029 if (::read (0, &result, 1) < 0)
1030 cerr <<
"read()" << endl;
1031 terminalStatus.c_lflag |= ICANON;
1032 terminalStatus.c_lflag |= ECHO;
1033 if (::tcsetattr (0, TCSADRAIN, &terminalStatus) < 0)
1034 cerr <<
"tcsetattr ~ICANON" << endl;
1035 #elif defined (MSWindows) || defined (AJAWindows)
1036 HANDLE hdl (GetStdHandle (STD_INPUT_HANDLE));
1038 INPUT_RECORD buffer;
1039 PeekConsoleInput (hdl, &buffer, 1, &nEvents);
1042 ReadConsoleInput (hdl, &buffer, 1, &nEvents);
1043 result = char (buffer.Event.KeyEvent.wVirtualKeyCode);
1052 cout <<
"## Press Enter/Return key to exit: ";
1061 switch (inFrameRate)
1082 switch (inFrameRate)
1086 #if !defined(NTV2_DEPRECATE_16_0)
1092 case NTV2_FRAMERATE_5000: return AJA_FrameRate_5000;
1159 static struct VideoFormatPair
1163 } VideoFormatPairs[] = {
1191 for (
size_t formatNdx(0); formatNdx <
sizeof(VideoFormatPairs) /
sizeof(VideoFormatPair); formatNdx++)
1192 if (VideoFormatPairs[formatNdx].vIn == inOutVideoFormat)
1194 inOutVideoFormat = VideoFormatPairs[formatNdx].vOut;
1203 static struct VideoFormatPair
1207 } VideoFormatPairs[] = {
1235 for (
size_t formatNdx(0); formatNdx <
sizeof(VideoFormatPairs) /
sizeof(VideoFormatPair); formatNdx++)
1236 if (VideoFormatPairs[formatNdx].vIn == inOutVideoFormat)
1238 inOutVideoFormat = VideoFormatPairs[formatNdx].vOut;
1253 UWord totFrameStores(inDevice.
features().GetNumFrameStores());
1256 UWord tsiMux(firstFramestoreIndex);
1258 if (totFrameStores > totTSIMuxers)
1259 tsiMux = firstFramestoreIndex/2;
1260 else if (totFrameStores < totTSIMuxers)
1261 tsiMux = firstFramestoreIndex*2;
1262 for (
UWord num(0); num < inCount; num++)
1270 const bool isInputRGB)
1279 if (isInputRGB && !isFrameRGB)
1284 else if (!isInputRGB && isFrameRGB)
1292 return !conns.empty();
1300 const bool isInputRGB)
1302 UWord sdi(0), mux(0), csc(0), fb(0), path(0);
1311 if (isInputRGB == isFrameRGB)
1313 for (path = 0; path < 4; path++)
1324 else if (isInputRGB && !isFrameRGB)
1326 for (path = 0; path < 4; path++)
1344 for (path = 0; path < 4; path++)
1363 cerr <<
"## ERROR: Ch5678 must be for Corvid88, but no HDMI on that device" << endl;
1377 {fb = 4; sdi = fb; mux = fb / 2; csc = fb;}
1382 for (path = 0; path < 4; path++)
1400 for (path = 0; path < 4; path++)
1417 for (path = 0; path < 4; path++)
1431 for (path = 0; path < 4; path++)
1441 return !conns.empty();
1449 const bool isInputRGB)
1451 UWord fb(0), path(0);
1470 for (path = 0; path < 4; path++)
1486 else if (isQuadQuadHFR)
1505 for (path = 0; path < 4; path++)
1528 return !conns.empty();
1535 UWord numAudChannels(inDevice.
features().GetMaxAudioChannels());
1557 if (hwPageSizeBytes != sdkPageSizeBytes)
1560 cerr <<
"## NOTE: Page size changed from " <<
DEC(sdkPageSizeBytes/1024) <<
"K to " <<
DEC(hwPageSizeBytes/1024) <<
"K" << endl;
1562 cerr <<
"## WARNING: Failed to change page size from " <<
DEC(sdkPageSizeBytes/1024) <<
"K to " <<
DEC(hwPageSizeBytes/1024) <<
"K" << endl;
1564 return hwPageSizeBytes;
1573 { ostringstream oss;
1582 mOtherArgs.push_back(
string(pStr));
1596 typedef struct {
string fName;
NTV2VideoFormat fFormat;} FormatNameDictionary;
1597 static const FormatNameDictionary sVFmtDict[] = {
1715 cout << endl << endl;
1716 for (
unsigned ndx(0); !sVFmtDict[ndx].fName.empty(); ndx++)
1718 const string & str (sVFmtDict[ndx].fName);
1722 if (vFormat != vFormat2)
1745 AJASystemInfo::append (result,
"Anc Capture File", fAncDataFilePath.empty() ?
"---" : fAncDataFilePath);
1776 AJASystemInfo::append (result,
"Anc Playback File", fAncDataFilePath.empty() ?
"---" : fAncDataFilePath);
1804 if (fDeviceSpec2.empty())
std::set< NTV2InputSource > NTV2InputSourceSet
A set of distinct NTV2InputSource values.
static NTV2StringList getColorNames(void)
@ NTV2_FORMAT_3840x2160p_6000
@ NTV2_FBF_10BIT_YCBCR_420PL3_LE
See 3-Plane 10-Bit YCbCr 4:2:0 ('I420_10LE' a.k.a. 'YUV-P420-L10').
static NTV2VideoFormat GetVideoFormatFromString(const std::string &inStr, const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD, const std::string &inDevSpec=std::string())
Returns the NTV2VideoFormat that matches the given string.
unsigned long stoul(const std::string &str, std::size_t *idx, int base)
static NTV2VideoFormatSet GetSupportedVideoFormats(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD)
@ NTV2_FORMAT_4x1920x1080p_6000
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
@ NTV2_FORMAT_1080psf_2398
virtual bool DMABufferUnlock(const NTV2Buffer &inBuffer)
Unlocks the given host buffer that was previously locked using CNTV2Card::DMABufferLock.
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
static bool SetDefaultPageSize(const size_t inNewSize)
Changes the default page size for use in future page-aligned allocations.
@ NTV2_FORMAT_4096x2160p_2400
static const string gGlobalMutexName("com.aja.ntv2.mutex.demo")
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
@ AJA_PixelFormat_YCBCR10_422PL
@ NTV2_FBF_ARGB
See 8-Bit ARGB, RGBA, ABGR Formats.
NTV2OutputXptID GetSDIInputOutputXptFromChannel(const NTV2Channel inSDIInput, const bool inIsDS2=false)
@ NTV2_IOKINDS_HDMI
Specifies HDMI input/output kinds.
@ NTV2_FBF_10BIT_YCBCR_420PL2
10-Bit 4:2:0 2-Plane YCbCr
String2PixelFormatMap::const_iterator String2PixelFormatMapConstIter
static NTV2TCIndexSet gTCIndexesVITC1
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
static String2OutputDestMap gString2OutputDestMap
@ NTV2_FORMAT_4096x2160p_12000
NTV2FrameBufferFormatSet::const_iterator NTV2FrameBufferFormatSetConstIter
A handy const iterator for iterating over an NTV2FrameBufferFormatSet.
#define NTV2_IS_ATC_VITC1_TIMECODE_INDEX(__x__)
@ NTV2_OUTPUTDESTINATION_HDMI1
@ NTV2_FORMAT_4x4096x2160p_4800
std::set< NTV2FrameBufferFormat > NTV2FrameBufferFormatSet
A set of distinct NTV2FrameBufferFormat values.
NTV2OutputDestination
Identifies a specific video output destination.
static NTV2InputSourceSet gInputSources
@ NTV2_FORMAT_4x4096x2160p_2398
@ AJA_PixelFormat_YCBCR8_422PL3
@ NTV2_FBF_12BIT_RGB_PACKED
See 12-Bit Packed RGB.
static NTV2PixelFormats GetSupportedPixelFormats(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL)
map< string, NTV2FrameBufferFormat > String2PixelFormatMap
NTV2InputXptID GetDLInInputXptFromChannel(const NTV2Channel inChannel, const bool inLinkB=false)
std::set< NTV2OutputDestination > NTV2OutputDestinations
A set of distinct NTV2OutputDestination values.
static NTV2PixelFormat GetPixelFormatFromString(const std::string &inStr, const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2PixelFormat that matches the given string.
Declares device capability functions.
@ NTV2_FORMAT_4096x2160p_11988
static NTV2InputSourceSet gInputSourcesHDMI
static NTV2VANCMode GetVANCModeFromString(const std::string &inStr)
std::set< std::string > NTV2StringSet
#define NTV2_IS_FBF_PLANAR(__s__)
std::string & strip(std::string &str, const std::string &ws)
NTV2TestPatternSelect
Identifies a predefined NTV2 test pattern.
@ NTV2_FBF_PRORES_HDV
Apple ProRes HDV.
@ NTV2_FORMAT_4096x2160p_2500
#define NTV2_FBF_IS_RAW(__fbf__)
@ NTV2_FRAMERATE_1500
15 frames per second
std::set< NTV2TCIndex > NTV2TCIndexes
@ NTV2_FRAMERATE_6000
60 frames per second
static NTV2ChannelList GetTSIMuxesForFrameStore(CNTV2Card &inDevice, const NTV2Channel in1stFrameStore, const UWord inCount)
#define NTV2_IS_FBF_PRORES(__fbf__)
static char ReadCharacterPress(void)
Returns the character that represents the last key that was pressed on the keyboard without waiting f...
@ NTV2_FORMAT_525psf_2997
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
static NTV2FrameBufferFormatSet gPixelFormats
@ NTV2_FORMAT_4x2048x1080p_4795
static NTV2VideoFormatSet g4KFormats
@ NTV2_FBF_10BIT_DPX_LE
10-Bit DPX Little-Endian
std::string join(const std::vector< std::string > &parts, const std::string &delim)
@ NTV2_FORMAT_3840x2160p_5994_B
#define NTV2_ASSERT(_expr_)
std::set< NTV2VideoFormat > NTV2VideoFormatSet
A set of distinct NTV2VideoFormat values.
@ NTV2_FRAMERATE_2997
Fractional rate of 30,000 frames per 1,001 seconds.
@ NTV2_FORMAT_1080p_2K_6000_B
@ NTV2_FORMAT_4096x2160p_5000_B
const char * poptGetArg(poptContext con)
@ NTV2_FBF_RGBA
See 8-Bit ARGB, RGBA, ABGR Formats.
@ AJA_PixelFormat_BGR8_PACK
@ NTV2_FORMAT_1080psf_2K_2398
bool CanDoInputTCIndex(const NTV2TCIndex inTCNdx)
#define NTV2_IS_QUAD_QUAD_HFR_VIDEO_FORMAT(__f__)
static bool GetInputRouting(NTV2XptConnections &outConnections, const CaptureConfig &inConfig, const bool isInputRGB=(0))
Answers with the crosspoint connections needed to implement the given capture configuration.
static std::string GetAudioSystemStrings(const std::string inDeviceSpecifier=std::string())
@ NTV2_FORMAT_1080p_2K_4800_A
pair< string, NTV2TCIndex > String2TCIndexPair
@ NTV2_FORMAT_4x2048x1080p_11988
@ NTV2_FRAMERATE_12000
120 frames per second
@ NTV2_FBF_48BIT_RGB
See 48-Bit RGB.
map< string, NTV2InputSource > String2InputSourceMap
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
static String2TPNamesMap gString2TPNamesMap
const char * poptStrerror(const int error)
NTV2OutputDestinations::const_iterator NTV2OutputDestinationsConstIter
A handy const iterator for iterating over an NTV2OutputDestinations.
@ AJA_PixelFormat_PRORES_DVPRO
@ NTV2_FORMAT_4x1920x1080psf_3000
String2VANCModeMap::const_iterator String2VANCModeMapConstIter
NTV2PixelFormat fPixelFormat
Pixel format to use.
NTV2Channel NTV2OutputDestinationToChannel(const NTV2OutputDestination inOutputDest)
Converts a given NTV2OutputDestination to its equivalent NTV2Channel value.
String2VideoFormatMMap::const_iterator String2VideoFormatMMapCI
NTV2EmbeddedAudioInput NTV2InputSourceToEmbeddedAudioInput(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2EmbeddedAudioInput value.
@ NTV2_FBF_8BIT_HDV
See 8-Bit HDV.
@ AJA_PixelFormat_YCBCR8_422PL
Declares the NTV2TestPatternGen class.
@ NTV2_FORMAT_1080p_2K_3000
map< string, NTV2TCIndex > String2TCIndexMap
@ NTV2_FORMAT_4x2048x1080p_2997
@ NTV2_TestPatt_ColorBars100
@ NTV2_FBF_10BIT_ARGB
10-Bit ARGB
@ NTV2_FBF_10BIT_YCBCRA
10-Bit YCbCrA
const std::string & AJAAncDataTypeToString(const AJAAncDataType inValue, const bool inCompact=true)
@ NTV2_FORMAT_4x4096x2160p_2500
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
ULWord NTV2VideoFormatKinds
@ NTV2_FORMAT_4x4096x2160p_4795
@ NTV2_FORMAT_4x1920x1080p_2997
@ NTV2_FORMAT_4x1920x1080p_2500
@ NTV2_FORMAT_4x2048x1080p_4800
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ NTV2_FORMAT_4x3840x2160p_2500
multimap< string, NTV2VideoFormat > String2VideoFormatMMap
#define NTV2_IS_ATC_VITC2_TIMECODE_INDEX(__x__)
#define NTV2_IS_ATC_LTC_TIMECODE_INDEX(__x__)
static NTV2FrameBufferFormatSet gFBFsProRes
@ NTV2_FBF_10BIT_DPX
See 10-Bit RGB - DPX Format.
@ NTV2_FORMAT_4x3840x2160p_5000_B
enum NTV2InputCrosspointID NTV2InputXptID
@ NTV2_OUTPUT_CROSSPOINT_INVALID
AJALabelValuePairs Get(const bool inCompact=(0)) const
NTV2TimeCodes::const_iterator NTV2TimeCodesConstIter
A handy const interator for iterating over NTV2TCIndex/NTV2TimeCodeList pairs.
std::ostream & operator<<(std::ostream &ioStrm, const CaptureConfig &inObj)
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
static NTV2OutputDestinations gOutputDestsHDMI
@ NTV2_FORMAT_4x3840x2160p_2398
@ NTV2_FBF_24BIT_RGB
See 24-Bit RGB.
@ NTV2_FORMAT_4x4096x2160p_6000_B
@ AJA_PixelFormat_YCBCR8_420PL3
bool CanDoOutputDestination(const NTV2OutputDestination inDest)
ULWord GetIndexForNTV2Channel(const NTV2Channel inChannel)
@ NTV2_IOKINDS_ANALOG
Specifies analog input/output kinds.
@ NTV2_FRAMERATE_2500
25 frames per second
@ NTV2_FORMAT_1080p_2K_4795_A
static bool Get4KInputFormat(NTV2VideoFormat &inOutVideoFormat)
Given a video format, if all 4 inputs are the same and promotable to 4K, this function does the promo...
@ NTV2_FORMAT_3840x2160p_2500
NTV2FrameRate
Identifies a particular video frame rate.
map< string, string > String2TPNamesMap
@ NTV2_FORMAT_4096x2160p_6000_B
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
enum NTV2OutputDestination NTV2OutputDest
@ NTV2_FORMAT_4x4096x2160p_2997
@ NTV2_FRAMERATE_4800
48 frames per second
#define NTV2_IS_4K_VIDEO_FORMAT(__f__)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=(0), bool inRDMA=(0))
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
static std::string GetOutputDestinationStrings(const NTV2IOKinds inKinds, const std::string inDevSpec=std::string())
@ NTV2_FRAMERATE_2400
24 frames per second
static NTV2TCIndexSet gTCIndexesATCLTC
virtual class DeviceCapabilities & features(void)
@ NTV2_FORMAT_4x2048x1080psf_2398
@ NTV2_FBF_10BIT_RGB_PACKED
10-Bit Packed RGB
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
pair< string, string > String2TPNamePair
@ NTV2_FORMAT_4x2048x1080p_2398
@ AJA_PixelFormat_RGB_DPX_LE
@ AJA_PixelFormat_YCbCr_DPX
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
#define NTV2_IS_ANALOG_TIMECODE_INDEX(__x__)
virtual std::string GetDisplayName(void)
Answers with this device's display name.
@ NTV2_IOKINDS_SDI
Specifies SDI input/output kinds.
static bool IsValidDevice(const std::string &inDeviceSpec)
static NTV2VideoFormatSet gAllFormats
@ AJA_PixelFormat_RGB8_PACK
static size_t DefaultPageSize(void)
static NTV2AudioSystem GetAudioSystemFromString(const std::string &inStr)
Returns the NTV2AudioSystem that matches the given string.
@ NTV2_FORMAT_1080psf_2K_2500
ULWord NTV2PixelFormatKinds
String2TPNamesMap::const_iterator String2TPNamesMapConstIter
static std::string GetTestPatternStrings(void)
#define NTV2_IS_FBF_RGB(__fbf__)
@ NTV2_FORMAT_4x2048x1080psf_2400
std::string & lower(std::string &str)
@ NTV2_FBF_10BIT_RAW_RGB
10-Bit Raw RGB
std::string NTV2InputSourceToString(const NTV2InputSource inValue, const bool inForRetailDisplay=false)
Popt(const int inArgc, const char **pArgs, const PoptOpts *pInOptionsTable)
static NTV2TCIndexSet gTCIndexesVITC2
std::string NTV2TCIndexToString(const NTV2TCIndex inValue, const bool inCompactDisplay=false)
@ NTV2_FORMAT_1080p_5994_B
static NTV2FrameBufferFormatSet gFBFsRaw
@ NTV2_FORMAT_1080psf_3000_2
@ NTV2_FORMAT_1080p_2K_2400
@ NTV2_FORMAT_4x2048x1080p_3000
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
@ NTV2_FORMAT_4096x2160p_4795
std::string & replace(std::string &str, const std::string &from, const std::string &to)
@ NTV2_FRAMERATE_2398
Fractional rate of 24,000 frames per 1,001 seconds.
static bool ConfigureAudioSystems(CNTV2Card &inDevice, const CaptureConfig &inConfig, const NTV2AudioSystemSet inAudioSystems)
Configures capture audio systems.
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
@ NTV2_FORMAT_4x4096x2160p_3000
static AJALabelValuePairs & append(AJALabelValuePairs &inOutTable, const std::string &inLabel, const std::string &inValue=std::string())
A convenience function that appends the given label and value strings to the provided AJALabelValuePa...
#define NTV2_OUTPUT_DEST_IS_HDMI(_dest_)
Declares the CNTV2DeviceScanner class.
This class is used to configure an NTV2Capture instance.
static NTV2FrameBufferFormatSet gFBFsPacked
@ NTV2_FORMAT_4x2048x1080psf_3000
@ NTV2_FORMAT_1080p_2K_4795_B
@ AJA_PixelFormat_YCBCR10_420PL
@ NTV2_FRAMERATE_1498
Fractional rate of 15,000 frames per 1,001 seconds.
static String2VideoFormatMMap gString2VideoFormatMMap
static size_t SetDefaultPageSize(void)
NTV2_RP188 Timecode(const NTV2TCIndex inTCNdx) const
@ NTV2_FORMAT_4x3840x2160p_5994
@ NTV2_FORMAT_4096x2160p_2398
@ NTV2_INPUTSOURCE_INVALID
The invalid video input.
std::set< NTV2AudioSystem > NTV2AudioSystemSet
A set of distinct NTV2AudioSystem values. New in SDK 16.2.
@ NTV2_MAX_NUM_VIDEO_FORMATS
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
map< string, NTV2AudioSystem > String2AudioSystemMap
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
#define GetFrameBufferInputXptFromChannel
@ NTV2_FORMAT_4x2048x1080p_5000
@ NTV2_FBF_24BIT_BGR
See 24-Bit BGR.
@ AJA_PixelFormat_YCBCR10_422PL3LE
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
@ NTV2_FORMAT_4096x2160p_6000
#define NTV2_OUTPUT_DEST_IS_ANALOG(_dest_)
std::string NTV2VANCModeToString(const NTV2VANCMode inValue, const bool inCompactDisplay=false)
@ AJA_PixelFormat_YCBCR10_420PL3LE
static std::string GetVANCModeStrings(void)
static const NTV2TCIndexes GetSupportedTCIndexes(const NTV2TCIndexKinds inKinds)
@ NTV2_FORMAT_4x1920x1080p_3000
@ NTV2_FORMAT_4x3840x2160p_2997
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
static NTV2OutputDestination GetOutputDestinationFromString(const std::string &inStr, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2OutputDestination that matches the given string.
Declares numerous NTV2 utility functions.
@ AJA_PixelFormat_RGB10_PACK
AJALabelValuePairs Get(const bool inCompact=(0)) const
Renders a human-readable representation of me.
@ NTV2_FORMAT_1080p_5000_B
@ AJA_PixelFormat_RGB_DPX
poptContext poptFreeContext(poptContext con)
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
NTV2VideoFormatSet::const_iterator NTV2VideoFormatSetConstIter
A handy const iterator for iterating over an NTV2VideoFormatSet.
static std::string GetVideoFormatStrings(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD, const std::string inDevSpec=std::string())
@ NTV2_FORMAT_4x3840x2160p_5000
@ NTV2_FORMAT_1080p_2K_5994_A
#define NTV2_INPUT_SOURCE_IS_HDMI(_inpSrc_)
@ NTV2_MAX_NUM_TIMECODE_INDEXES
static const DemoCommonInitializer gInitializer
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
I interrogate and control an AJA video/audio capture/playout device.
@ NTV2_FORMAT_4x2048x1080p_12000
static std::string GetTCIndexStrings(const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDeviceSpecifier=std::string(), const bool inIsInputOnly=(!(0)))
@ NTV2_FBF_10BIT_YCBCR_422PL2
10-Bit 4:2:2 2-Plane YCbCr
std::pair< NTV2InputXptID, NTV2OutputXptID > NTV2Connection
This links an NTV2InputXptID and an NTV2OutputXptID.
@ NTV2_FORMAT_625psf_2500
static void WaitForEnterKeyPress(void)
Prompts the user (via stdout) to press the Return or Enter key, then waits for it to happen.
@ NTV2_AUDIO_BUFFER_SIZE_4MB
@ NTV2_FORMAT_1080p_2K_2500
@ NTV2_FORMAT_4x4096x2160p_4800_B
@ NTV2_FORMAT_4x1920x1080psf_2997
bool CanDoInputSource(const NTV2InputSource inSrc)
static std::string GetTestPatternNameFromString(const std::string &inStr)
@ NTV2_FORMAT_1080p_6000_A
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
NTV2OutputXptID GetDLInOutputXptFromChannel(const NTV2Channel inDLInput)
@ NTV2_FRAMERATE_11988
Fractional rate of 120,000 frames per 1,001 seconds.
@ NTV2_FORMAT_1080p_2K_2398
@ NTV2_FORMAT_3840x2160p_2997
@ NTV2_FORMAT_4096x2160p_4800
std::vector< std::string > NTV2StringList
static String2VANCModeMap gString2VANCModeMap
NTV2VANCMode
These enum values identify the available VANC modes.
@ NTV2_INPUT_CROSSPOINT_INVALID
@ NTV2_FORMAT_1080psf_2K_2400
@ AJA_PixelFormat_YCbCrA10
#define NTV2_OUTPUT_DEST_IS_SDI(_dest_)
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
@ NTV2_FORMAT_3840x2160p_5994
@ NTV2_FORMAT_4x4096x2160p_2400
@ NTV2_FBF_8BIT_YCBCR_YUY2
See Alternate 8-Bit YCbCr ('YUY2').
NTV2Channel fInputChannel
The device channel to use.
static NTV2VideoFormatSet gSDHDFormats
@ NTV2_FORMAT_4x4096x2160p_6000
std::vector< NTV2Channel > NTV2ChannelList
An ordered sequence of NTV2Channel values.
@ NTV2_FORMAT_3840x2160p_2398
@ AJA_PixelFormat_PRORES_HDV
NTV2InputSource
Identifies a specific video input source.
static NTV2OutputDestinations gOutputDestinations
#define NTV2_IS_QUAD_QUAD_FORMAT(__f__)
@ NTV2_FORMAT_4x1920x1080psf_2398
bool NTV2DeviceCanDo12gRouting(const NTV2DeviceID inDeviceID)
poptContext poptGetContext(const char *name, int argc, const char **argv, const struct poptOption *options, unsigned int flags)
static std::string GetInputSourceStrings(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
static bool GetInputRouting8K(NTV2XptConnections &outConnections, const CaptureConfig &inConfig, const NTV2VideoFormat inVidFormat, const NTV2DeviceID inDevID=DEVICE_ID_INVALID, const bool isInputRGB=(0))
Answers with the crosspoint connections needed to implement the given 8K/UHD2 capture configuration.
@ NTV2_FORMAT_1080p_2K_6000_A
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
String2InputSourceMap::const_iterator String2InputSourceMapConstIter
@ NTV2_FBF_PRORES_DVCPRO
Apple ProRes DVC Pro.
static NTV2VideoFormatSet g8KFormats
@ NTV2_IOKINDS_ALL
Specifies any/all input/output kinds.
@ NTV2_FORMAT_3840x2160p_5000_B
@ NTV2_FORMAT_4096x2160p_3000
@ NTV2_FORMAT_4x1920x1080psf_2500
@ NTV2_FRAMERATE_5994
Fractional rate of 60,000 frames per 1,001 seconds.
int poptGetNextOpt(poptContext con)
This file contains some structures, constants, classes and functions that are used in some of the dem...
Private include file for all ajabase sources.
@ NTV2_FORMAT_4x3840x2160p_2400
NTV2AudioSystemSet::const_iterator NTV2AudioSystemSetConstIter
A handy const iterator into an NTV2AudioSystemSet. New in SDK 16.2.
@ NTV2_FORMAT_4x4096x2160p_5000_B
@ AJA_PixelFormat_Unknown
NTV2TCIndexes::const_iterator NTV2TCIndexesConstIter
@ NTV2_FBF_10BIT_RAW_YCBCR
See 10-Bit Raw YCbCr (CION).
@ NTV2_OUTPUTDESTINATION_INVALID
@ NTV2_FORMAT_4x2048x1080p_2500
@ NTV2_FORMAT_4x1920x1080p_5994
@ NTV2_FORMAT_4x4096x2160p_5000
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
@ NTV2_FORMAT_4x3840x2160p_6000
bool fDoTSIRouting
If true, do TSI routing; otherwise squares.
bool NTV2DeviceGetSupportedOutputDests(const NTV2DeviceID inDeviceID, NTV2OutputDestinations &outOutputDests, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL)
Returns a set of distinct NTV2OutputDest values supported on the given device.
@ AJA_PixelFormat_YCbCr10
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
NTV2OutputXptID GetTSIMuxOutputXptFromChannel(const NTV2Channel inTSIMuxer, const bool inLinkB=false, const bool inIsRGB=false)
@ NTV2_FBF_10BIT_YCBCR_DPX
See 10-Bit YCbCr - DPX Format.
NTV2InputSourceSet::const_iterator NTV2InputSourceSetConstIter
A handy const iterator for iterating over an NTV2InputSourceSet.
static NTV2OutputDestinations gOutputDestsAnalog
This struct replaces the old RP188_STRUCT.
@ NTV2_FBF_10BIT_YCBCR_422PL3_LE
See 3-Plane 10-Bit YCbCr 4:2:2 ('I422_10LE' a.k.a. 'YUV-P-L10').
AJALabelValuePairs Get(const bool inCompact=(0)) const
Renders a human-readable representation of me.
bool IsRGBFormat(const NTV2FrameBufferFormat format)
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
@ NTV2_FRAMERATE_UNKNOWN
Represents an unknown or invalid frame rate.
@ NTV2_FORMAT_1080p_5000_A
static AJA_PixelFormat GetAJAPixelFormat(const NTV2PixelFormat inFormat)
@ NTV2_FORMAT_4x2048x1080p_6000
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
static bool GetInputRouting4K(NTV2XptConnections &outConnections, const CaptureConfig &inConfig, const NTV2DeviceID inDevID=DEVICE_ID_INVALID, const bool isInputRGB=(0))
Answers with the crosspoint connections needed to implement the given 4K/UHD capture configuration.
@ NTV2_FORMAT_4096x2160p_5000
@ NTV2_TCINDEX_DEFAULT
The "default" timecode (mostly used by the AJA "Retail" service and Control Panel)
static NTV2TCIndexSet gTCIndexesAnalog
@ NTV2_FORMAT_4x1920x1080p_5000
@ NTV2_FBF_8BIT_YCBCR_420PL3
See 3-Plane 8-Bit YCbCr 4:2:0 ('I420' a.k.a. 'YUV-P420').
static NTV2OutputDestinations gOutputDestsSDI
@ NTV2_FORMAT_4x1920x1080p_2398
bool NTV2DeviceGetSupportedInputSources(const NTV2DeviceID inDeviceID, NTV2InputSourceSet &outInputSources, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL)
Returns a set of distinct NTV2InputSource values supported on the given device.
static NTV2InputSourceSet gInputSourcesSDI
@ NTV2_FORMAT_1080p_6000_B
@ NTV2_FORMAT_4x4096x2160p_5994_B
static String2AudioSystemMap gString2AudioSystemMap
@ NTV2_FORMAT_4096x2160p_2997
@ NTV2_FBF_8BIT_DVCPRO
See 8-Bit DVCPro.
static NTV2StringList gTestPatternNames
@ NTV2_FORMAT_4096x2160p_5994_B
@ NTV2_FORMAT_4x3840x2160p_5994_B
@ NTV2_FORMAT_1080p_5994_A
@ NTV2_FORMAT_4x3840x2160p_6000_B
@ NTV2_FBF_8BIT_YCBCR_422PL2
8-Bit 4:2:2 2-Plane YCbCr
NTV2OutputXptID GetInputSourceOutputXpt(const NTV2InputSource inInputSource, const bool inIsSDI_DS2=false, const bool inIsHDMI_RGB=false, const UWord inHDMI_Quadrant=0)
@ NTV2_FORMAT_4x2048x1080p_5994
static NTV2FrameBufferFormatSet gFBFsRGB
NTV2StringList::const_iterator NTV2StringListConstIter
bool UnlockAll(CNTV2Card &inDevice)
static NTV2TCIndexSet gTCIndexesSDI
NTV2InputSource NTV2TimecodeIndexToInputSource(const NTV2TCIndex inTCIndex)
Converts the given NTV2TCIndex value into the appropriate NTV2InputSource value.
@ NTV2_FORMAT_4x3840x2160p_3000
@ NTV2_FORMAT_1080p_2K_5994_B
static String2InputSourceMap gString2InputSourceMap
@ NTV2_FBF_16BIT_ARGB
16-Bit ARGB
Configures an NTV2Player instance.
std::string NTV2ChannelToString(const NTV2Channel inValue, const bool inForRetailDisplay=false)
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
enum NTV2OutputCrosspointID NTV2OutputXptID
@ NTV2_FBF_8BIT_YCBCR_422PL3
See 3-Plane 8-Bit YCbCr 4:2:2 (Weitek 'Y42B' a.k.a. 'YUV-P8').
static NTV2TestPatternNames getTestPatternNames(void)
@ NTV2_FORMAT_3840x2160p_5000
static const NTV2InputSourceSet GetSupportedInputSources(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL)
bool LockAll(CNTV2Card &inDevice)
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
@ NTV2_FORMAT_1080p_2K_5000_A
@ NTV2_FRAMERATE_5000
50 frames per second
@ NTV2_FORMAT_4x4096x2160p_4795_B
static size_t HostPageSize(void)
static NTV2TCIndex GetTCIndexFromString(const std::string &inStr, const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDevSpec=std::string())
Returns the NTV2TCIndex that matches the given string.
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
@ NTV2_FORMAT_4x2048x1080psf_2500
static NTV2InputSource GetInputSourceFromString(const std::string &inStr, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2InputSource that matches the given string.
@ NTV2_FBF_10BIT_RGB
See 10-Bit RGB Format.
bool CanDoPixelFormat(const NTV2PixelFormat inPF)
static NTV2FrameBufferFormatSet gFBFsAlpha
static std::string StripFormatString(const std::string &inStr)
static const char * GetGlobalMutexName(void)
const char * poptBadOption(poptContext con, unsigned int flags)
@ NTV2_FRAMERATE_4795
Fractional rate of 48,000 frames per 1,001 seconds.
std::string to_string(bool val)
@ NTV2_FRAMERATE_3000
30 frames per second
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
@ NTV2_FORMAT_1080p_2K_5000_B
static String2PixelFormatMap gString2PixelFormatMap
static bool Get8KInputFormat(NTV2VideoFormat &inOutVideoFormat)
Given a video format, if all 4 inputs are the same and promotable to 8K, this function does the promo...
@ NTV2_FORMAT_1080psf_2997_2
map< string, NTV2OutputDestination > String2OutputDestMap
String2AudioSystemMap::const_iterator String2AudioSystemMapConstIter
#define NTV2_INPUT_SOURCE_IS_ANALOG(_inpSrc_)
@ NTV2_FORMAT_4x4096x2160p_5994
@ NTV2_FORMAT_4x2048x1080p_2400
static NTV2FrameBufferFormatSet gFBFsPlanar
@ NTV2_FORMAT_1080p_2K_4800_B
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
@ NTV2_FORMAT_4x1920x1080p_2400
@ NTV2_FORMAT_4x1920x1080psf_2400
static NTV2TCIndexSet gTCIndexesHDMI
@ NTV2_FORMAT_3840x2160p_6000_B
@ NTV2_FORMAT_1080psf_2500_2
virtual bool Open(const UWord inDeviceIndex)
Opens a local/physical AJA device so it can be monitored/controlled.
enum _NTV2TCIndexKinds NTV2TCIndexKinds
@ NTV2_FORMAT_4096x2160p_4795_B
NTV2InputXptID GetTSIMuxInputXptFromChannel(const NTV2Channel inTSIMuxer, const bool inLinkB=false)
String2OutputDestMap::const_iterator String2OutputDestMapConstIter
static NTV2InputSourceSet gInputSourcesAnalog
@ NTV2_FORMAT_4096x2160p_4800_B
@ NTV2_FORMAT_3840x2160p_2400
map< string, NTV2VANCMode > String2VANCModeMap
static const NTV2OutputDestinations GetSupportedOutputDestinations(const NTV2IOKinds inKinds)
@ NTV2_FBF_ABGR
See 8-Bit ARGB, RGBA, ABGR Formats.
NTV2InputSource fInputSource
The device input connector to use.
#define NTV2_IS_VALID_VIDEO_FORMAT(__f__)
static String2TCIndexMap gString2TCIndexMap
@ NTV2_FORMAT_3840x2160p_3000
@ NTV2_FORMAT_1080psf_2400
@ AJA_PixelFormat_YCBCR8_420PL
std::set< NTV2TCIndex > NTV2TCIndexSet
A set of distinct NTV2TCIndex values.
bool CanDoOutputTCIndex(const NTV2TCIndex inTCNdx)
String2TCIndexMap::const_iterator String2TCIndexMapConstIter
@ NTV2_FORMAT_4096x2160p_5994
#define NTV2_FBF_HAS_ALPHA(__fbf__)
static NTV2TCIndexSet gTCIndexes
const char * NTV2VideoFormatString(NTV2VideoFormat fmt)
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_FORMAT_4x2048x1080psf_2997
std::string NTV2OutputDestinationToString(const NTV2OutputDestination inValue, const bool inForRetailDisplay=false)
@ NTV2_AUDIOSYSTEM_INVALID
@ NTV2_FORMAT_1080p_2K_2997