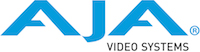 |
AJA NTV2 SDK
17.6.0.1688
NTV2 SDK 17.6.0.1688
|
Go to the documentation of this file.
8 #ifndef NTV2FORMATDESC_H
9 #define NTV2FORMATDESC_H
18 #if defined (AJALinux)
66 const ULWord in1stActiveLine = 0,
67 const UByte inNumLumaBits = 0,
68 const UByte inNumChromaBits = 0,
69 const UByte inNumAlphaBits = 0);
112 inline bool IsValid (
void)
const {
return numLines && numPixels && mNumPlanes && mLinePitch[0]
113 && (mNumBitsLuma || mNumBitsChroma);}
114 inline bool IsVANC (
void)
const {
return GetFirstActiveLine() > 0;}
115 inline bool IsPlanar (
void)
const {
return GetNumPlanes() > 1 ||
NTV2_IS_FBF_PLANAR (mPixelFormat);}
124 ULWord GetVerticalSampleRatio (
const UWord inPlaneIndex0 = 0)
const;
133 {
const ULWord vSamp(GetVerticalSampleRatio(inPlaneIndex0));
134 return vSamp ? (GetFullRasterHeight() * GetBytesPerRow(inPlaneIndex0) / vSamp) : 0;
140 ULWord GetTotalBytes (
void)
const;
160 inline ULWord GetRasterWidth (
void)
const {
return numPixels;}
161 inline UWord GetNumPlanes (
void)
const {
return mNumPlanes;}
162 std::string PlaneToString (
const UWord inPlaneIndex0)
const;
169 UWord ByteOffsetToPlane (
const ULWord inByteOffset)
const;
176 UWord ByteOffsetToRasterLine (
const ULWord inByteOffset)
const;
182 bool IsAtLineStart (
ULWord inByteOffset)
const;
188 inline ULWord GetRasterHeight (
const bool inVisibleOnly =
false)
const {
return inVisibleOnly ? GetVisibleRasterHeight() : GetFullRasterHeight();}
223 const void * GetRowAddress (
const void * pInStartAddress,
const ULWord inRowIndex0,
const UWord inPlaneIndex0 = 0)
const;
232 void * GetWriteableRowAddress (
void * pInStartAddress,
const ULWord inRowIndex0,
const UWord inPlaneIndex0 = 0)
const;
241 ULWord RasterLineToByteOffset (
const ULWord inRowIndex0,
const UWord inPlaneIndex0 = 0)
const;
258 bool GetFirstChangedRow (
const void * pInStartAddress1,
const void * pInStartAddress2,
ULWord & outFirstChangedRowNum)
const;
271 bool GetChangedLines (
NTV2RasterLineOffsets & outDiffs,
const void * pInBuffer1,
const void * pInBuffer2,
const ULWord inMaxLines = 0)
const;
290 bool GetSMPTELineNumber (
const ULWord inLineOffset,
ULWord & outSMPTELine,
bool & outIsField2)
const;
298 bool GetLineOffsetFromSMPTELine (
const ULWord inSMPTELine,
ULWord & outLineOffset)
const;
321 std::ostream & Print (std::ostream & inOutStream,
const bool inDetailed =
true)
const;
330 std::ostream & PrintSMPTELineNumber (std::ostream & inOutStream,
const ULWord inLineOffset,
const bool inForTextMode =
false)
const;
332 inline NTV2Standard GetVideoStandard (
void)
const {
return mStandard;}
333 inline NTV2VideoFormat GetVideoFormat (
void)
const {
return mVideoFormat;}
335 inline NTV2VANCMode GetVANCMode (
void)
const {
return mVancMode;}
346 inline UByte GetNumBitsLuma (
void)
const {
return mNumBitsLuma;}
347 inline UByte GetNumBitsChroma (
void)
const {
return mNumBitsChroma;}
348 inline UByte GetNumBitsAlpha (
void)
const {
return mNumBitsAlpha;}
349 inline bool HasAlpha (
void)
const {
return IsValid() && GetNumBitsAlpha();}
350 inline bool IsRGB (
void)
const {
return GetNumBitsLuma() ?
false :
true;}
352 #if !defined(NTV2_DEPRECATE_16_3)
356 void MakeInvalid (
void);
360 inline void SetPixelFormat (
const NTV2PixelFormat inPixFmt) {mPixelFormat = inPixFmt;}
361 inline void SetBitsPerComponent (
const UByte inLuma,
const UByte inChroma,
const UByte inAlpha) {mNumBitsLuma = inLuma; mNumBitsChroma = inChroma; mNumBitsAlpha = inAlpha;}
362 void FinalizePlanar (
void);
379 UByte mNumBitsChroma;
407 #endif // NTV2FORMATDESC_H
#define NTV2_IS_UHD2_STANDARD(__s__)
#define NTV2_IS_UHD_STANDARD(__s__)
#define NTV2_IS_4K_STANDARD(__s__)
#define NTV2_IS_SD_VIDEO_FORMAT(__f__)
Defines the import/export macros for producing DLLs or LIBs.
#define NTV2_IS_FBF_PLANAR(__s__)
Describes a user-space buffer on the host computer. I have an address and a length,...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
bool operator==(const json_pointer< RefStringTypeLhs > &lhs, const json_pointer< RefStringTypeRhs > &rhs) noexcept
#define NTV2_IS_8K_VIDEO_FORMAT(__f__)
#define NTV2_IS_QUAD_STANDARD(__s__)
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
#define NTV2_IS_4K_VIDEO_FORMAT(__f__)
Describes a segmented data transfer (copy or move) from a source memory location to a destination loc...
#define NTV2_IS_UHD_VIDEO_FORMAT(__f__)
@ NTV2_VANCMODE_TALL
This identifies the "tall" mode in which there are some VANC lines in the frame buffer.
NTV2Standard
Identifies a particular video standard.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
std::vector< uint16_t > UWordSequence
An ordered sequence of UWord (uint16_t) values.
Enumerations for controlling NTV2 devices.
#define NTV2_IS_HD_VIDEO_FORMAT(__f__)
ULWord GetVideoWriteSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
Identical to the GetVideoActiveSize function, except rounds the result up to the nearest 4K page size...
NTV2VANCMode
These enum values identify the available VANC modes.
#define NTV2_IS_8K_STANDARD(__s__)
#define NTV2_IS_HD_STANDARD(__s__)
@ NTV2_VANCMODE_TALLER
This identifies the mode in which there are some + extra VANC lines in the frame buffer.
#define NTV2_IS_SD_STANDARD(__s__)
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
NTV2FrameGeometry
Identifies a particular video frame geometry.
Declares enums and structs used by all platform drivers and the SDK.
#define NTV2_IS_UHD2_VIDEO_FORMAT(__f__)
bool Is2KFormat(const NTV2VideoFormat format)