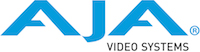 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
7 #ifndef __NTV2_CAPTIONRENDERER__
8 #define __NTV2_CAPTIONRENDERER__
43 const bool inAutoOpen =
true);
48 static bool FlushGlyphCaches (
void);
61 static bool BurnChar (
const UByte inCharCode,
88 static bool BurnString (
const std::string & inString,
92 const UWord inRowNum = 15,
93 const UWord inColumnNum = 1);
110 static bool BurnStringAtXY (
const std::string & inString,
123 virtual bool Open (
void);
130 virtual bool Close (
void);
136 virtual inline bool IsOpen (
void)
const {
return mGlyphHeightInLines && mGlyphWidthInBytes && mGlyphWidthInPixels;}
183 virtual bool GetCharacterRasterOrigin (
const UWord in608CaptionRow,
const UWord in608CaptionCol,
184 UWord & outVertLineOffset,
UWord & outHorzPixelOffset)
const;
226 virtual inline ULWord GetTotalBytes (
void)
const {
return mBytesPerAttribute * GetNumActivePreloadedGlyphsRasters();}
236 virtual ULWordSequence GetActivePreloadedGlyphsRastersAttributes (
void)
const;
243 virtual std::ostream & Print (std::ostream & inOutStream,
const bool inIncludeActives =
false)
const;
269 virtual bool CreatePreloadedGlyphsRasterForAttribute (
const NTV2Line21Attributes & inAttribs)
const;
270 virtual bool HasPreloadedGlyphsRasterForAttribute (
const NTV2Line21Attributes & inAttribs)
const;
274 #if !defined(NTV2_DEPRECATE_16_0) // Old APIs
279 #endif // !defined(NTV2_DEPRECATE_16_0)
299 typedef std::map <ULWord, UByte *> AttribToBufferMap;
300 typedef AttribToBufferMap::iterator AttribToBufferMapIter;
301 typedef AttribToBufferMap::const_iterator AttribToBufferMapConstIter;
305 mutable AttribToBufferMap mpRasters;
306 UWord mHRasterPixelsPerGlyphDot;
307 UWord mVRasterLinesPerGlyphDot;
308 UWord mVCaptionRasterOrigin;
309 UWord mHCaptionRasterOrigin;
310 UWord mGlyphWidthInPixels;
311 UWord mGlyphWidthInBytes;
312 UWord mGlyphHeightInLines;
313 ULWord mBytesPerAttribute;
322 #endif // __NTV2_CAPTIONRENDERER__
virtual UWord GlyphDotHeightToRasterLines(const UWord inVHeightInGlyphDots) const
Returns the equivalent number of horizontal raster pixels that correspond to the given number of glyp...
Declares several data types used with 608/SD captioning.
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
This is the font used when rendering CEA-608 captions into a frame buffer. I define a "dot" map havin...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
I am a reference-counted pointer template class. I am intended to be a proxy for an underlying object...
virtual ULWord GetPreloadedGlyphsRasterRowBytes(void) const
virtual UWord GetGlyphWidthInBytes(void) const
virtual UWord GetPreloadedGlyphsRasterWidthInPixels(void) const
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
Declaration of NTV2CCFont.
virtual NTV2PixelFormat GetPixelFormat(void) const
Returns the frame buffer format that I'm currently "open" for.
AJARefPtr< CNTV2CaptionRenderer > CNTV2CaptionRendererPtr
Enumerations for controlling NTV2 devices.
virtual bool IsOpen(void) const
Answers true if I'm currently open.
virtual UWord GetPreloadedGlyphsRasterHeightInLines(void) const
virtual UWord GlyphDotWidthToRasterPixels(const UWord inHWidthInGlyphDots) const
Returns the equivalent number of horizontal raster pixels that correspond to the given number of glyp...
virtual UWord GetGlyphHeightInLines(void) const
#define NTV2_DEPRECATED_f(__f__)
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
virtual ULWord GetNumActivePreloadedGlyphsRasters(void) const
virtual ULWord GetTotalBytes(void) const
CEA-608 Character Attributes.
Defines the AJARefPtr template class.
std::ostream & operator<<(std::ostream &inOutStream, const CNTV2CaptionRendererPtr &inObjPtr)
virtual UWord GetGlyphWidthInPixels(void) const