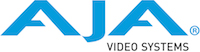 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
125 virtual inline std::string
GetName (
void)
const {
return mFontName;}
250 const ULWord inBytesPerRow,
253 const ULWord inScaledDotWidth = 1,
254 const ULWord inScaledDotHeight = 1)
const;
271 const ULWord inBytesPerRow,
274 const ULWord inScaledDotWidth,
275 const ULWord inScaledDotHeight,
276 const bool inIsHD =
false)
const;
287 const UWord mGlyphCount;
290 const UWord mDotMapWidth;
291 const UWord mLeftMarginDotCount;
292 const UWord mRightMarginDotCount;
293 const UWord mDotMapHeight;
294 const UWord mTopMarginDotCount;
295 const UWord mBottomMarginDotCount;
296 const std::string mFontName;
virtual UWord GetDotMapWidth(void) const
Returns the width of the dot map, in dots.
static std::string Utf8ToCCFontByteArray(const std::string &inUtf8Str)
Converts the given UTF-8 encoded string into a string of CCFont character codes.
Declares several data types used with 608/SD captioning.
virtual UWord GetRightMarginDotCount(void) const
Returns the number of dots of space to appear to the right of each blitted glyph.
virtual NTV2GlyphIndex GetNoUnderlineSpaceGlyphIndex(void) const
Returns the "no underline space" character's zero-based glyph index number.
This is the font used when rendering CEA-608 captions into a frame buffer. I define a "dot" map havin...
static UByte UnicodeCodePointToCharacterCode(const ULWord inCodePoint)
Returns the character code that corresponds to the given unicode codepoint.
virtual UByte GetUnderlineCharacterCode(void) const
Returns the character code of the special underline glyph.
virtual UWord GetDotMapHeight(void) const
Returns the height of the dot map, in dots.
static NTV2GlyphIndex CharacterCodeToGlyphIndex(const UByte inCharacterCode)
Returns the zero-based glyph index number that corresponds to the given character code.
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
static UByte GlyphIndexToCharacterCode(const NTV2GlyphIndex inGlyphIndex)
Returns the character code that corresponds to the given glyph.
virtual UWord GetTotalWidthInDots(void) const
Returns the total width, in dots, a blitted glyph will consume, including any left and/or right margi...
virtual bool RenderGlyph8BitYCbCr(UByte *pDestBuffer, const ULWord inBytesPerRow, const NTV2GlyphIndex inGlyphIndex, const NTV2Line21Attrs &inAttribs, const ULWord inScaledDotWidth=1, const ULWord inScaledDotHeight=1) const
Renders the given glyph with the given display attributes into the specified 8-bit YCbCr '2vuy' desti...
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
virtual UWord GetTotalHeightInDots(void) const
Returns the total height, in dots, a blitted glyph will consume, including any top and/or bottom marg...
virtual UWord GetDotMapRowCount(void) const
Returns the height of the dot map, in dot rows.
virtual NTV2CodePointSet GetCodePointsForCCFontCharCode(const UByte inCharCode) const
Returns the set of codepoints that map to a given CCFont character code.
virtual UByte GetNoUnderlineSpaceCharacterCode(void) const
Returns the character code of the special "underline space" glyph.
virtual UWord GetUnderlineStartingDotRow(void) const
Returns the starting row position of the underline, which is below the dot map. Also takes into accou...
Enumerations for controlling NTV2 devices.
virtual bool HasGlyphFor608CodePoint(const NTV2_CC608_CodePoint in608CodePoint) const
Returns true if there is a glyph available for the given CEA-608 code point.
virtual bool IsValidGlyphIndex(const NTV2GlyphIndex inGlyphIndex) const
Returns true if the given glyph index is valid.
virtual bool RenderGlyph8BitRGB(const NTV2PixelFormat inPixelFormat, UByte *pDestBuffer, const ULWord inBytesPerRow, const NTV2GlyphIndex inGlyphIndex, const NTV2Line21Attrs &inAttribs, const ULWord inScaledDotWidth, const ULWord inScaledDotHeight, const bool inIsHD=false) const
Renders the given glyph with the given display attributes into the specified 8-bit NTV2_FBF_ARGB dest...
virtual UWord GetTopMarginDotCount(void) const
Returns the number of dots of space to appear above each blitted glyph.
void DumpCCFont(const NTV2CCFont &inCCFont=NTV2CCFont::GetInstance())
Dumps all glyphs in the given CC font to stderr in UTF-8. When displayed in a terminal using a monosp...
ULWord NTV2_CC608_CodePoint
Describes a unique CEA-608 caption character code point in 32 bits: 0xSS00XXYY, where....
uint16_t NTV2GlyphIndex
A zero-based index number that uniquely identifies a glyph.
static const NTV2CCFont & GetInstance(void)
Returns a constant reference to the NTV2CCFont singleton.
virtual UWord GetGlyphCount(void) const
Returns the number of glyphs available in this caption font.
virtual std::string GetName(void) const
Returns the name of this caption font.
virtual UWord GetLeftMarginDotCount(void) const
Returns the number of dots of space to appear to the left of each blitted glyph.
CEA-608 Character Attributes.
virtual UWord GetBottomMarginDotCount(void) const
Returns the number of dots of space to appear below each blitted glyph.
virtual std::string GetGlyphRowDotsAsString(const NTV2GlyphIndex inGlyphIndex, const unsigned inRow) const
Returns a UTF-8 encoded string that contains the dot pattern for the given zero-based row number and ...
virtual NTV2GlyphIndex GetUnderlineGlyphIndex(void) const
Returns the underline character's zero-based glyph index number.
virtual UByte GetCCFontCharCode(const NTV2_CC608_CodePoint in608CodePoint) const
Returns the "ASCII-like" character code that best represents the given CEA-608 code point.
virtual UWord GetGlyphRowDots(const NTV2GlyphIndex inGlyphIndex, const unsigned inRow) const
Returns the 16-bit "dot" bitmap of the row of the character glyph having the given offset.
std::set< NTV2_CC608_CodePoint > NTV2CodePointSet
A set of unique CEA-608 caption character code points.
virtual UWord * GetGlyphFor608CodePoint(const NTV2_CC608_CodePoint in608CodePoint) const
Returns a pointer to the "dot" bitmap of the glyph that represents the given CEA-608 code point.
virtual std::ostream & PrintGlyphs(std::ostream &inOutStream, const NTV2GlyphIndex inFirstGlyph, const NTV2GlyphIndex inLastGlyph) const
Renders all glyphs in the given range (inclusive) into the specified output stream as multiple rows o...