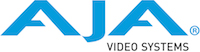 |
AJA NTV2 SDK
17.6.0.1688
NTV2 SDK 17.6.0.1688
|
Go to the documentation of this file.
25 #if defined(MSWindows)
31 #define AJA_NTV2_CLEAR_DEVICE_ANC_BUFFER_AFTER_CAPTURE_XFER // Requires non-zero kVRegZeroDeviceAncPostCapture
32 #define AJA_NTV2_CLEAR_HOST_ANC_BUFFER_TAIL_AFTER_CAPTURE_XFER // Requires non-zero kVRegZeroHostAncPostCapture
36 #define ACINSTP(_p_) " " << HEX0N(uint64_t(_p_),8)
37 #define ACTHIS ACINSTP(this)
39 #define ACFAIL(__x__) AJA_sERROR (AJA_DebugUnit_AutoCirculate, ACTHIS << "::" << AJAFUNC << ": " << __x__)
40 #define ACWARN(__x__) AJA_sWARNING(AJA_DebugUnit_AutoCirculate, ACTHIS << "::" << AJAFUNC << ": " << __x__)
41 #define ACNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_AutoCirculate, ACTHIS << "::" << AJAFUNC << ": " << __x__)
42 #define ACINFO(__x__) AJA_sINFO (AJA_DebugUnit_AutoCirculate, ACTHIS << "::" << AJAFUNC << ": " << __x__)
43 #define ACDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_AutoCirculate, ACTHIS << "::" << AJAFUNC << ": " << __x__)
45 #define RCVFAIL(__x__) AJA_sERROR (AJA_DebugUnit_Anc2110Rcv, ACTHIS << "::" << AJAFUNC << ": " << __x__)
46 #define RCVWARN(__x__) AJA_sWARNING(AJA_DebugUnit_Anc2110Rcv, ACTHIS << "::" << AJAFUNC << ": " << __x__)
47 #define RCVNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_Anc2110Rcv, ACTHIS << "::" << AJAFUNC << ": " << __x__)
48 #define RCVINFO(__x__) AJA_sINFO (AJA_DebugUnit_Anc2110Rcv, ACTHIS << "::" << AJAFUNC << ": " << __x__)
49 #define RCVDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_Anc2110Rcv, ACTHIS << "::" << AJAFUNC << ": " << __x__)
51 #define XMTFAIL(__x__) AJA_sERROR (AJA_DebugUnit_Anc2110Xmit, ACTHIS << "::" << AJAFUNC << ": " << __x__)
52 #define XMTWARN(__x__) AJA_sWARNING(AJA_DebugUnit_Anc2110Xmit, ACTHIS << "::" << AJAFUNC << ": " << __x__)
53 #define XMTNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_Anc2110Xmit, ACTHIS << "::" << AJAFUNC << ": " << __x__)
54 #define XMTINFO(__x__) AJA_sINFO (AJA_DebugUnit_Anc2110Xmit, ACTHIS << "::" << AJAFUNC << ": " << __x__)
55 #define XMTDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_Anc2110Xmit, ACTHIS << "::" << AJAFUNC << ": " << __x__)
71 if (! _boardOpened )
return false;
81 pFrameStamp->
frame = frameNum;
84 return AutoCirculate (autoCircData);
99 if (!_boardOpened)
return false;
102 autoCirculateStatus -> channelSpec = channelSpec;
109 return AutoCirculate (autoCircData);
115 outStartFrame = outEndFrame = -1;
117 {
ACFAIL(
"Not open");
return false;}
119 {
ACFAIL(
"Must request at least one frame");
return false;}
125 bool isQuad(
false), isQuadQuad(
false), wasEnabled(
false);
129 while (IsChannelEnabled(ch, wasEnabled) && wasEnabled)
131 enabledFrameStores.insert(ch);
132 if (enabledFrameStores.size() == 1)
134 GetVideoFormat(vFmt, inFrameStore);
137 if (!isQuad && !isQuadQuad)
144 DisableChannels(enabledFrameStores);
151 if (!enabledFrameStores.empty())
152 EnableChannels(enabledFrameStores);
154 if (freeRgns8MB.empty())
155 {
ACFAIL(
"No free regions");
return false;}
157 {
ACFAIL(
"TranslateRegions failed");
return false;}
158 if (freeRgns.empty())
159 {
ACFAIL(
"No free regions after translation");
return false;}
162 for (
size_t ndx(0); ndx < freeRgns.size(); ndx++)
163 {
const ULWord val(freeRgns.at(ndx));
164 UWord startFrame(val >> 16), lengthFrames(
UWord(val & 0x0000FFFF));
165 if (inFrameCount > lengthFrames)
168 outStartFrame =
LWord(startFrame);
169 outEndFrame =
LWord(startFrame + inFrameCount - 1);
172 const string qstr (isQuad ?
" quad" : (isQuadQuad ?
" quad-quad" :
""));
173 if (outStartFrame < 0 || outEndFrame < 0)
178 ostringstream dump; dump <<
DEC(freeRgns.size()) <<
" free region(s):" << endl;
179 if (!freeRgns.empty())
180 dump <<
" Tgt Frms 8MB Frms" << endl;
181 for (
size_t ndx(0); ndx < freeRgns.size(); ndx++)
182 {
ULWord rgn(freeRgns.at(ndx)), rgn8(freeRgns8MB.at(ndx));
183 UWord startBlk(rgn >> 16), numBlks(
UWord(rgn & 0x0000FFFF));
184 UWord startBlk8(rgn8 >> 16), numBlks8(
UWord(rgn8 & 0x0000FFFF));
186 dump <<
"Frms " <<
DEC0N(startBlk,3) <<
"-" <<
DEC0N(startBlk+numBlks-1,3) <<
" ";
188 dump <<
"Frm " <<
DEC0N(startBlk,3) <<
" ";
190 dump <<
DEC0N(startBlk8,3) <<
"-" <<
DEC0N(startBlk8+numBlks8-1,3) << endl;
192 dump <<
DEC0N(startBlk8,3) << endl;
194 ACFAIL(
"Cannot find " <<
DEC(inFrameCount) <<
" contiguous" << qstr <<
" frames in these " << dump.str());
197 ACINFO(
"Found requested " <<
DEC(inFrameCount) <<
" contiguous" << qstr <<
" frames (" <<
DEC(outStartFrame) <<
"-" <<
DEC(outEndFrame) <<
")");
209 if (!inDevice.IsOpen ())
214 if (!inDevice.
GetMode (inChannel, mode))
222 const UWord inFrameCount,
224 const ULWord inOptionFlags,
225 const UByte inNumChannels,
226 const UWord inStartFrameNumber,
227 const UWord inEndFrameNumber)
230 {
ACFAIL(
"Ch" <<
DEC(inChannel+1) <<
" is illegal channel value");
return false;}
231 if (!inNumChannels || inNumChannels > 8)
232 {
ACFAIL(
"Input Ch" <<
DEC(inChannel+1) <<
": illegal 'inNumChannels' value '" <<
DEC(inNumChannels) <<
"' -- must be 1-8");
return false;}
234 {
ACFAIL(
"Input Ch" <<
DEC(inChannel+1) <<
": FBAllocLock mutex not ready");
return false;}
237 LWord startFrameNumber(
LWord(inStartFrameNumber+0));
238 LWord endFrameNumber (
LWord(inEndFrameNumber+0));
239 if (!endFrameNumber && !startFrameNumber)
242 {
ACFAIL(
"Input Ch" <<
DEC(inChannel+1) <<
": Zero frames requested");
return false;}
243 if (!FindUnallocatedFrames (inFrameCount, startFrameNumber, endFrameNumber, inChannel))
246 else if (inFrameCount)
247 ACWARN (
"Input Ch" <<
DEC(inChannel+1) <<
": FrameCount " <<
DEC(inFrameCount) <<
" ignored -- using start/end " <<
DEC(inStartFrameNumber)
248 <<
"/" <<
DEC(inEndFrameNumber) <<
" frame numbers");
249 if (endFrameNumber < startFrameNumber)
250 {
ACFAIL(
"Input Ch" <<
DEC(inChannel+1) <<
": EndFrame(" <<
DEC(endFrameNumber) <<
") precedes StartFrame(" <<
DEC(startFrameNumber) <<
")");
return false;}
251 if ((endFrameNumber - startFrameNumber + 1) < 2)
252 {
ACFAIL(
"Input Ch" <<
DEC(inChannel+1) <<
": Frames " <<
DEC(startFrameNumber) <<
"-" <<
DEC(endFrameNumber) <<
" < 2 frames");
return false;}
258 ACWARN(
"Input Ch" <<
DEC(inChannel+1) <<
": MultiLink Audio requested, but device doesn't support it");
262 if (numAudSystems &&
UWord(inAudioSystem) >= numAudSystems)
263 {
ACFAIL(
"Invalid audio system specified: AudSys" <<
DEC(inAudioSystem+1) <<
" -- exceeds max legal AudSys" <<
DEC(numAudSystems));
return false;}
268 autoCircData.
lVal1 = startFrameNumber;
269 autoCircData.
lVal2 = endFrameNumber;
270 autoCircData.
lVal3 = inAudioSystem;
277 autoCircData.
lVal4 = inNumChannels;
283 autoCircData.
bVal1 =
false;
294 const bool result (AutoCirculate(autoCircData));
302 for (
size_t ndx(0); ndx < badRgns.size(); ndx++)
303 {
const ULWord rgnInfo(badRgns.at(ndx));
304 const UWord startBlk(rgnInfo >> 16), numBlks(
UWord(rgnInfo & 0x0000FFFF));
307 const string infoStr (
aja::join(tags,
", "));
308 ostringstream acLabel; acLabel <<
"AC" <<
DEC(inChannel+1);
309 if (infoStr.find(acLabel.str()) != string::npos)
310 { ostringstream warning;
312 warning <<
"Frms " <<
DEC0N(startBlk,3) <<
"-" <<
DEC0N(startBlk+numBlks-1,3);
314 warning <<
"Frm " <<
DEC0N(startBlk,3);
315 ACWARN(
"Input Ch" <<
DEC(inChannel+1) <<
": memory overlap/interference: " << warning.str() <<
": " << infoStr);
321 if (AutoCirculateGetStatus (inChannel, stat) && !stat.
IsStopped() && stat.
WithAudio())
323 ULWord audChlsPerSample(0);
332 const double bytesPerChannel (4.0);
333 const double channelsPerSample (
double(audChlsPerSample+0));
334 const double bytesPerFrame (samplesPerSecond * bytesPerChannel * channelsPerSample / framesPerSecond);
335 const ULWord maxVideoFrames (4UL * 1024UL * 1024UL /
ULWord(bytesPerFrame));
339 <<
DEC(maxVideoFrames) <<
"-frame max buffer capacity of AudSys" <<
DEC(stat.
GetAudioSystem()+1));
344 ACINFO(
"Input Ch" <<
DEC(inChannel+1) <<
" initialized using frames " <<
DEC(startFrameNumber) <<
"-" <<
DEC(endFrameNumber));
347 ACFAIL(
"Input Ch" <<
DEC(inChannel+1) <<
" initialization failed");
354 const UWord inFrameCount,
356 const ULWord inOptionFlags,
357 const UByte inNumChannels,
358 const UWord inStartFrameNumber,
359 const UWord inEndFrameNumber)
362 {
ACFAIL(
"Ch" <<
DEC(inChannel+1) <<
" is illegal channel value");
return false;}
363 if (!inNumChannels || inNumChannels > 8)
364 {
ACFAIL(
"Output Ch" <<
DEC(inChannel+1) <<
": illegal 'inNumChannels' value '" <<
DEC(inNumChannels) <<
"' -- must be 1-8");
return false;}
366 {
ACFAIL(
"Output Ch" <<
DEC(inChannel+1) <<
": FBAllocLock mutex not ready");
return false;}
369 LWord startFrameNumber(
LWord(inStartFrameNumber+0));
370 LWord endFrameNumber (
LWord(inEndFrameNumber+0));
371 if (!endFrameNumber && !startFrameNumber)
374 {
ACFAIL(
"Output Ch" <<
DEC(inChannel+1) <<
": Zero frames requested");
return false;}
375 if (!FindUnallocatedFrames (inFrameCount, startFrameNumber, endFrameNumber, inChannel))
378 else if (inFrameCount)
379 ACWARN (
"Output Ch" <<
DEC(inChannel+1) <<
": FrameCount " <<
DEC(inFrameCount) <<
" ignored -- using start/end "
380 <<
DEC(inStartFrameNumber) <<
"/" <<
DEC(inEndFrameNumber) <<
" frame numbers");
381 if (endFrameNumber < startFrameNumber)
382 {
ACFAIL(
"Output Ch" <<
DEC(inChannel+1) <<
": EndFrame(" <<
DEC(endFrameNumber) <<
") precedes StartFrame("
383 <<
DEC(startFrameNumber) <<
")");
return false;}
384 if ((endFrameNumber - startFrameNumber + 1) < 2)
385 {
ACFAIL(
"Output Ch" <<
DEC(inChannel+1) <<
": Frames " <<
DEC(startFrameNumber) <<
"-" <<
DEC(endFrameNumber) <<
" < 2 frames");
return false;}
391 ACWARN(
"Output Ch" <<
DEC(inChannel+1) <<
": MultiLink Audio requested, but device doesn't support it");
395 if (numAudSystems &&
UWord(inAudioSystem) >= numAudSystems)
396 {
ACFAIL(
"Invalid audio system specified: AudSys" <<
DEC(inAudioSystem+1) <<
" -- exceeds max legal AudSys" <<
DEC(numAudSystems));
return false;}
404 ACWARN(
"Output Ch" <<
DEC(inChannel+1) <<
"AUTOCIRCULATE_WITH_ANC set, but also has "
410 autoCircData.
lVal1 = startFrameNumber;
411 autoCircData.
lVal2 = endFrameNumber;
412 autoCircData.
lVal3 = inAudioSystem;
419 autoCircData.
lVal4 = inNumChannels;
425 autoCircData.
bVal1 =
false;
439 autoCircData.
bVal7 =
true;
441 <<
": AUTOCIRCULATE_WITH_RP188 requested without AUTOCIRCULATE_WITH_ANC -- enabled AUTOCIRCULATE_WITH_ANC anyway");
444 const bool result (AutoCirculate(autoCircData));
452 for (
size_t ndx(0); ndx < badRgns.size(); ndx++)
453 {
const ULWord rgnInfo(badRgns.at(ndx));
454 const UWord startBlk(rgnInfo >> 16), numBlks(
UWord(rgnInfo & 0x0000FFFF));
457 const string infoStr (
aja::join(tags,
", "));
458 ostringstream acLabel; acLabel <<
"AC" <<
DEC(inChannel+1);
459 if (infoStr.find(acLabel.str()) != string::npos)
460 { ostringstream warning;
462 warning <<
"Frms " <<
DEC0N(startBlk,3) <<
"-" <<
DEC0N(startBlk+numBlks-1,3);
464 warning <<
"Frm " <<
DEC0N(startBlk,3);
465 ACWARN(
"Output Ch" <<
DEC(inChannel+1) <<
": memory overlap/interference: " << warning.str() <<
": " << infoStr);
471 if (AutoCirculateGetStatus (inChannel, stat) && !stat.
IsStopped() && stat.
WithAudio())
473 ULWord audChlsPerSample(0);
482 const double bytesPerChannel (4.0);
483 const double channelsPerSample (
double(audChlsPerSample+0));
484 const double bytesPerFrame (samplesPerSecond * bytesPerChannel * channelsPerSample / framesPerSecond);
485 const ULWord maxVideoFrames (4UL * 1024UL * 1024UL /
ULWord(bytesPerFrame));
489 <<
DEC(maxVideoFrames) <<
"-frame max buffer capacity of AudSys" <<
DEC(stat.
GetAudioSystem()+1));
494 ACINFO(
"Output Ch" <<
DEC(inChannel+1) <<
" initialized using frames " <<
DEC(startFrameNumber) <<
"-" <<
DEC(endFrameNumber));
497 ACFAIL(
"Output Ch" <<
DEC(inChannel+1) <<
" initialization failed");
506 autoCircData.
lVal1 =
LWord(inStartTime >> 32);
507 autoCircData.
lVal2 =
LWord(inStartTime & 0xFFFFFFFF);
510 const bool result (AutoCirculate(autoCircData));
512 ACINFO(
"Started Ch" <<
DEC(inChannel+1));
514 ACFAIL(
"Failed to start Ch" <<
DEC(inChannel+1));
531 const bool stopInputFailed (!AutoCirculate (stopInput));
532 const bool stopOutputFailed (!AutoCirculate (stopOutput));
533 if (stopInputFailed && stopOutputFailed)
535 ACFAIL(
"Failed to stop Ch" <<
DEC(inChannel+1));
540 ACINFO(
"Aborted Ch" <<
DEC(inChannel+1));
545 bool result (GetMode(inChannel, mode));
552 ACWARN(
"Failed to stop Ch" <<
DEC(inChannel+1) <<
" -- retrying with ABORT");
553 return AutoCirculateStop(inChannel,
true);
555 ACINFO(
"Stopped Ch" <<
DEC(inChannel+1));
564 if (!AutoCirculateStop(*it, inAbort))
571 { (
void) inAtFrameNum;
574 autoCircData.
bVal1 =
false;
581 const bool result(AutoCirculate(autoCircData));
585 ACFAIL(
"Failed to pause Ch" <<
DEC(inChannel+1));
595 autoCircData.
bVal1 =
true;
596 autoCircData.
bVal2 = inClearDropCount;
600 const bool result(AutoCirculate(autoCircData));
602 ACINFO(
"Resumed Ch" <<
DEC(inChannel+1));
604 ACFAIL(
"Failed to resume Ch" <<
DEC(inChannel+1));
614 autoCircData.
bVal1 = inClearDropCount;
618 const bool result(AutoCirculate(autoCircData));
620 ACINFO(
"Flushed Ch" <<
DEC(inChannel+1) <<
", " << (inClearDropCount?
"cleared":
"retained") <<
" drop count");
622 ACFAIL(
"Failed to flush Ch" <<
DEC(inChannel+1));
636 const bool result(AutoCirculate(autoCircData));
638 ACINFO(
"Prerolled " <<
DEC(inPreRollFrames) <<
" frame(s) on Ch" <<
DEC(inChannel+1));
640 ACFAIL(
"Failed to preroll " <<
DEC(inPreRollFrames) <<
" frame(s) on Ch" <<
DEC(inChannel+1));
655 outStatus = notRunningStatus;
659 const bool result(NTV2Message(outStatus));
661 ACFAIL(
"Failed to get status on Ch" <<
DEC(inChannel+1));
672 return NTV2Message(outFrameStamp);
685 const bool result(AutoCirculate(autoCircData));
687 ACINFO(
"Set active frame to " <<
DEC(inNewActiveFrame) <<
" on Ch" <<
DEC(inChannel+1));
689 ACFAIL(
"Failed to set active frame to " <<
DEC(inNewActiveFrame) <<
" on Ch" <<
DEC(inChannel+1));
700 NTV2_ASSERT (inOutXferInfo.NTV2_IS_STRUCT_VALID ());
709 GetEveryFrameServices(taskMode);
715 bool isProgressive (
false);
716 IsProgressiveStandard(isProgressive, inChannel);
725 bool tmpLocalF1AncBuffer(
false), tmpLocalF2AncBuffer(
false);
734 ULWord F1OffsetFromBottom(0), F2OffsetFromBottom(0);
735 size_t F1SizeInBytes(0), F2SizeInBytes(0);
739 F2SizeInBytes = size_t(F2OffsetFromBottom);
740 if (F2OffsetFromBottom < F1OffsetFromBottom)
741 F1SizeInBytes = size_t(F1OffsetFromBottom - F2OffsetFromBottom);
743 F1SizeInBytes = size_t(F2OffsetFromBottom - F1OffsetFromBottom);
747 ULWord F1MonOffsetFromBottom(0), F2MonOffsetFromBottom(0);
751 && F2MonOffsetFromBottom < F2OffsetFromBottom
752 && F2OffsetFromBottom < F1MonOffsetFromBottom
753 && F1MonOffsetFromBottom < F1OffsetFromBottom)
755 F1SizeInBytes = size_t(F1OffsetFromBottom - F2OffsetFromBottom);
756 F2SizeInBytes = size_t(F2OffsetFromBottom);
760 XMTWARN(
"IoIP 2110 playout anc rgns disordered (offsets from bottom): F2Mon=" <<
HEX0N(F2MonOffsetFromBottom,8)
761 <<
" F2=" <<
HEX0N(F2OffsetFromBottom,8) <<
" F1Mon=" <<
HEX0N(F1MonOffsetFromBottom,8)
762 <<
" F1=" <<
HEX0N(F1OffsetFromBottom,8));
763 F1SizeInBytes = F2SizeInBytes = 0;
791 S2110DeviceAncToXferBuffers(inChannel, inOutXferInfo);
804 bool result = NTV2Message(inOutXferInfo);
811 S2110DeviceAncFromXferBuffers(inChannel, inOutXferInfo);
831 switch (TimecodeSource)
847 if (tcValue.
fLo && tcValue.
fHi && tcValue.
fLo != 0xFFFFFFFF && tcValue.
fHi != 0xFFFFFFFF)
848 tcValue.
fDBB |= 0x00020000;
865 if (tmpLocalF1AncBuffer)
867 if (tmpLocalF2AncBuffer)
870 #if defined (AJA_NTV2_CLEAR_DEVICE_ANC_BUFFER_AFTER_CAPTURE_XFER)
883 GetFrameBufferSize(inChannel, fbSize);
885 const ULWord ancOffset (ancOffsetF2 > ancOffsetF1 ? ancOffsetF2 : ancOffsetF1);
887 if (gClearDeviceAncBuffer.
IsNULL() || (gClearDeviceAncBuffer.
GetByteCount() != ancOffset))
889 gClearDeviceAncBuffer.
Allocate(ancOffset);
892 if (xferFrame != -1 && fbByteCount && !gClearDeviceAncBuffer.
IsNULL())
893 DMAWriteSegments (
ULWord(xferFrame),
895 fbByteCount - ancOffset,
902 #endif // AJA_NTV2_CLEAR_DEVICE_ANC_BUFFER_AFTER_CAPTURE_XFER
904 #if defined (AJA_NTV2_CLEAR_HOST_ANC_BUFFER_TAIL_AFTER_CAPTURE_XFER)
914 void * pF1TailEnd (clientAncBufferF1.
GetHostAddress(ancF1ByteCount));
915 void * pF2TailEnd (clientAncBufferF2.
GetHostAddress(ancF2ByteCount));
916 if (pF1TailEnd && clientAncBufferF1.
GetByteCount() > ancF1ByteCount)
917 ::memset (pF1TailEnd, 0, clientAncBufferF1.
GetByteCount() - ancF1ByteCount);
918 if (pF2TailEnd && clientAncBufferF2.
GetByteCount() > ancF2ByteCount)
919 ::memset (pF2TailEnd, 0, clientAncBufferF2.
GetByteCount() - ancF2ByteCount);
922 #endif // AJA_NTV2_CLEAR_HOST_ANC_BUFFER_TAIL_AFTER_CAPTURE_XFER
925 ACDBG(
"Transfer successful for Ch" <<
DEC(inChannel+1));
927 ACFAIL(
"Transfer failed on Ch" <<
DEC(inChannel+1));
948 #if !defined(NTV2_DEPRECATE_16_0)
953 #endif // !defined(NTV2_DEPRECATE_16_0)
971 #if !defined(NTV2_DEPRECATE_16_0)
976 #endif // !defined(NTV2_DEPRECATE_16_0)
980 static const uint16_t
sVPIDLineNumsF1[] = { 10, 10, 13, 9, 10, 10, 10, 10, 10, 10, 10, 10 };
981 static const uint16_t
sVPIDLineNumsF2[] = { 572, 0, 276, 322, 0, 0, 0, 572, 0, 0, 0, 0 };
992 bool result (GetFrameRate(ntv2Rate, inChannel));
993 bool isProgressive (
false);
999 uint32_t vpidA(0), vpidB(0);
1006 if (!GetStandard(standard, inChannel))
1016 const uint32_t F2StartLine (isProgressive ? 0 : smpteLineNumInfo.
GetLastLine());
1022 if (pPkt->GetDID() == 0x41 && pPkt->GetSID() == 0x01)
1024 if (pPkt->GetDC() != 4)
1026 const uint32_t* pULWord (
reinterpret_cast<const uint32_t*
>(pPkt->
GetPayloadData()));
1027 uint32_t vpidValue (pULWord ? *pULWord : 0);
1028 if (!pPkt->GetDataLocation().IsHanc())
1099 if (!timecodes.empty())
RCVDBG(
"Channel" <<
DEC(inChannel+1) <<
" timecodes: " << timecodes);
1104 RCVDBG(
"WriteSDIInVPID chan=" <<
DEC(inChannel+1) <<
" VPIDa=" <<
xHEX0N(vpidA,4) <<
" VPIDb=" <<
xHEX0N(vpidB,4));
1105 WriteSDIInVPID(inChannel, vpidA, vpidB);
1121 if (!S2110DeviceAncFromXferBuffers (inChannel, tmpXfer))
1122 {
RCVFAIL(
"S2110DeviceAncFromXferBuffers failed");
return false;}
1126 {
RCVFAIL(
"GetInputTimeCodes failed");
return false;}
1132 SetRP188Data (inChannel, ntv2rp188);
1148 bool result (GetFrameRate(ntv2Rate, inChannel));
1149 bool isProgressive (
false);
1150 bool generateRTP (
false);
1157 ULWord vpidA(0), vpidB(0);
1160 ULWord F1OffsetFromBottom(0), F2OffsetFromBottom(0), F1MonOffsetFromBottom(0), F2MonOffsetFromBottom(0);
1165 if (!GetStandard(standard, inChannel))
1171 const uint32_t F2StartLine (smpteLineNumInfo.
GetLastLine());
1180 F1MonOffsetFromBottom - F2OffsetFromBottom);
1182 F2MonOffsetFromBottom);
1195 if (isIoIP2110 && isF1RTP && isF2RTP)
1197 packetList.GetSDITransmitData(gumpF1, gumpF2, isProgressive, F2StartLine);
1208 packetList.GetSDITransmitData(gumpF1, skipF2Data, isProgressive, F2StartLine);
1216 const ULWord gumpLength (std::min(F1MonOffsetFromBottom - F2OffsetFromBottom, gumpF1.
GetByteCount()));
1217 gumpF1.
CopyFrom(ancF1, 0, 0, gumpLength);
1228 packetList.GetSDITransmitData(skipF1Data, gumpF2, isProgressive, F2StartLine);
1237 gumpF2.
CopyFrom(ancF2, 0, 0, gumpLength);
1245 if (isMonitoring)
XMTDBG(
"ORIG: " << packetList);
1250 if (GetSDIOutVPID(vpidA, vpidB,
UWord(SDISpigotChannel)))
1308 if (
size_t(tcNdx) >= maxNumTCs)
1321 atc.
SetDBB (uint8_t(regTC.
fDBB & 0x000000FF), uint8_t(regTC.
fDBB & 0x0000FF00 >> 8));
1340 else if (isMonitoring) {
XMTWARN(
"Cannot insert ATC/VITC -- Xfer struct has no acOutputTimeCodes array!");}
1342 else if (isMonitoring) {
XMTDBG(
"ATC and/or VITC packet(s) already provided, won't insert any here");}
1355 DMAWriteAnc(31, rtpF1, rtpF2, NTV2_CHANNEL_INVALID); // DEBUG: DMA RTP into frame 31
1362 string compRTP (compareRTP.
CompareWithInfo(packetList,
false,
false));
1363 if (!compRTP.empty())
1364 XMTWARN(
"MISCOMPARE: " << compRTP);
1375 string compGUMP (compareGUMP.
CompareWithInfo(packetList,
false,
false));
1376 if (!compGUMP.empty())
1377 XMTWARN(
"MISCOMPARE: " << compGUMP);
1391 bool result (GetFrameRate(ntv2Rate, inChannel));
1392 bool isProgressive (
false);
1393 bool changed (
false);
1397 ULWord vpidA(0), vpidB(0);
1404 if (!GetStandard(standard, inChannel))
1414 const uint32_t F2StartLine (smpteLineNumInfo.
GetLastLine());
1422 if (GetSDIOutVPID(vpidA, vpidB,
UWord(SDISpigotChannel)))
1476 GetRP188Data (inChannel, regTC);
1494 atc.AJAAncillaryData_Timecode_ATC::SetDBB (uint8_t(regTC.
fDBB & 0x000000FF), uint8_t(regTC.
fDBB & 0x0000FF00 >> 8));
1517 else if (isMonitoring) {
XMTDBG(
"ATC and/or VITC packet(s) already provided, won't insert any here");}
1531 if (!compareResult.empty())
1532 XMTWARN(
"MISCOMPARE: " << compareResult);
@ kRP188SourceEmbeddedLTC
virtual AJAStatus SetLocationHorizOffset(const uint16_t inOffset)
Sets my ancillary data "location" horizontal offset.
LWord GetTransferFrameNumber(void) const
@ kDeviceHasBiDirectionalSDI
True if device SDI connectors are bi-directional.
ULWord GetScaleFromFrameRate(const NTV2FrameRate inFrameRate)
enum NTV2EveryFrameTaskMode NTV2TaskMode
@ kDeviceCanDoStackedAudio
True if device uses a "stacked" arrangement of its audio buffers.
@ AJAAncillaryData_Timecode_ATC_DBB1PayloadType_VITC1
NTV2Crosspoint channelSpec
NTV2Crosspoint channelSpec
virtual void SetAllowMultiRTPTransmit(const bool inAllow)
Determines if multiple RTP packets will be encoded for playout (via GetIPTransmitData)....
#define NTV2_IS_SDI_TIMECODE_INDEX(__x__)
#define NTV2_IS_ATC_VITC1_TIMECODE_INDEX(__x__)
virtual AJAStatus SetSID(const uint8_t inSID)
Sets my Secondary Data ID (SID) - (aka the Data Block Number (DBN) for "Type 1" SMPTE-291 packets).
NTV2Crosspoint acCrosspoint
The crosspoint (channel number with direction)
#define AUTOCIRCULATE_WITH_FIELDS
Use this to AutoCirculate with fields as frames for interlaced formats.
virtual bool AutoCirculateSetActiveFrame(const NTV2Channel inChannel, const ULWord inNewActiveFrame)
Immediately changes the Active Frame for the given channel.
AJAAncillaryData_Timecode_ATC_DBB1PayloadType
@ kRP188SourceEmbeddedVITC1
std::set< std::string > NTV2StringSet
virtual bool S2110DeviceAncFromXferBuffers(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &inOutXferInfo)
static bool QueryIsRP188DropFrame(const uint32_t inDBB, const uint32_t inLo, const uint32_t inHi)
#define NTV2ChannelToOutputChannelSpec
@ NTV2_TCINDEX_SDI2_2
SDI 2 embedded VITC 2.
std::set< NTV2TCIndex > NTV2TCIndexes
virtual bool FindUnallocatedFrames(const UWord inFrameCount, LWord &outStartFrame, LWord &outEndFrame, const NTV2Channel inFrameStore=NTV2_CHANNEL_INVALID)
Returns the device frame buffer numbers of the first unallocated contiguous band of frame buffers hav...
bool GetRP188Reg(RP188_STRUCT &outRP188) const
static AJAStatus SetFromDeviceAncBuffers(const NTV2Buffer &inF1AncBuffer, const NTV2Buffer &inF2AncBuffer, AJAAncillaryList &outPackets, const uint32_t inFrameNum=0)
Returns all ancillary data packets found in the given F1 and F2 ancillary data buffers.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
Describes a user-space buffer on the host computer. I have an address and a length,...
ULWord GetLastLine(const NTV2FieldID inRasterFieldID=NTV2_FIELD0) const
std::string join(const std::vector< std::string > &parts, const std::string &delim)
NTV2TCIndexes GetTCIndexesForSDIConnector(const NTV2Channel inSDIConnector)
static const TimecodeFormat sNTV2Rate2TCFormat[]
ULWord GetByteCount(void) const
#define NTV2_ASSERT(_expr_)
virtual bool IsValid(void) const
virtual AJAStatus GetIPTransmitData(NTV2Buffer &F1Buffer, NTV2Buffer &F2Buffer, const bool inIsProgressive=true, const uint32_t inF2StartLine=0)
Explicitly encodes my AJAAncillaryData packets into the given buffers in RTP Anc Buffer Data Format ....
virtual AJAStatus SetLocationDataStream(const AJAAncDataStream inStream)
Sets my ancillary data "location" data stream value (DS1,DS2...).
virtual bool AutoCirculatePreRoll(const NTV2Channel inChannel, const ULWord inPreRollFrames)
Tells AutoCirculate how many frames to skip before playout starts for the given channel.
virtual bool S2110DeviceAncFromBuffers(const NTV2Channel inChannel, NTV2Buffer &ancF1, NTV2Buffer &ancF2)
bool Deallocate(void)
Deallocates my user-space storage (if I own it – i.e. from a prior call to Allocate).
Declares the AJAAncillaryList class.
@ AJAAncDataLink_B
The ancillary data is associated with Link B of the video stream.
Declares the AJAAncillaryData_Timecode_ATC class.
#define NTV2_IS_VALID_TIMECODE_INDEX(__x__)
Defines a number of handy byte-swapping macros.
bool GetTagsForFrameIndex(const UWord inIndex, NTV2StringSet &outTags) const
Answers with the list of tags for the given frame number.
AJAAncDataType
Identifies the ancillary data types that are known to this module.
virtual uint32_t CountAncillaryDataWithType(const AJAAncDataType inMatchType) const
Answers with the number of AJAAncillaryData objects having the given type.
virtual AJAStatus GeneratePayloadData(void)
Generates the payload data from the "local" ancillary data.
@ AJAAncDataType_Timecode_VITC
SMPTE 12-M Vertical Interval Timecode (aka "VITC")
bool GetFreeRegions(ULWordSequence &outBlks) const
Answers with the list of free memory regions.
static uint32_t EndianSwap32NtoH(const uint32_t inValue)
bool GetInputTimeCode(NTV2_RP188 &outTimeCode, const NTV2TCIndex inTCIndex=NTV2_TCINDEX_SDI1) const
Intended for capture, answers with a specific timecode captured in my acTransferStatus member's acFra...
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
#define DEC0N(__x__, __n__)
@ NTV2_AncRgn_MonField1
Identifies the "monitor" or "auxiliary" Field 1 ancillary data region.
@ NTV2_AncRgn_Field2
Identifies the "normal" Field 2 ancillary data region.
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO3
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 3.
Declares the AJATimeCode class.
#define NTV2_IS_ATC_VITC2_TIMECODE_INDEX(__x__)
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
#define NTV2_IS_ATC_LTC_TIMECODE_INDEX(__x__)
virtual AJAStatus SetDID(const uint8_t inDataID)
Sets my Data ID (DID).
NTV2ChannelSet::const_iterator NTV2ChannelSetConstIter
A handy const iterator into an NTV2ChannelSet.
NTV2TimeCodes::const_iterator NTV2TimeCodesConstIter
A handy const interator for iterating over NTV2TCIndex/NTV2TimeCodeList pairs.
virtual AJAStatus GetDBB1PayloadType(AJAAncillaryData_Timecode_ATC_DBB1PayloadType &outType) const
Answers with my current payload type.
static AJAAncillaryData_Timecode_Format GetTimecodeFormatFromTimeBase(const AJATimeBase &inTimeBase)
Get the timecode format that matches the input timebase.
bool WithAudio(void) const
@ NTV2_FIELD0
Identifies the first field in time for an interlaced video frame, or the first and only field in a pr...
Audits an NTV2 device's SDRAM utilization, and can report contiguous regions of SDRAM,...
#define NTV2_IS_VALID_AUDIO_RATE(_x_)
virtual AJAStatus GetDropFrameFlag(bool &bFlag, AJAAncillaryData_Timecode_Format tcFmt=AJAAncillaryData_Timecode_Format_Unknown) const
virtual bool GetFrameStamp(NTV2Crosspoint channelSpec, ULWord frameNum, FRAME_STAMP_STRUCT *pFrameStamp)
static bool GetCurrentACChannelCrosspoint(CNTV2Card &inDevice, const NTV2Channel inChannel, NTV2Crosspoint &outCrosspoint)
@ AJAAncillaryData_Timecode_ATC_DBB1PayloadType_LTC
I am an ordered collection of AJAAncillaryData instances which represent one or more SMPTE 291 data p...
NTV2FrameRate
Identifies a particular video frame rate.
ULWord NTV2FramesizeToByteCount(const NTV2Framesize inFrameSize)
Converts the given NTV2Framesize value into an exact byte count.
This class/object reports information about the current and/or requested AutoCirculate frame.
static const uint32_t gSDIInRxStatusRegs[]
@ kVRegAncField2Offset
Anc Field2 byte offset from end of frame buffer (GUMP on all boards except RTP for SMPTE2022/IP)
NTV2AutoCirculateState acState
Current AutoCirculate state.
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO1
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 1.
virtual bool IsEmpty(void) const
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
enum _AutoCircCommand_ AUTO_CIRC_COMMAND
#define AUTOCIRCULATE_WITH_FBFCHANGE
Use this to AutoCirculate with the possibility of frame buffer format changes.
@ NTV2_AUTOCIRCULATE_INVALID
NTV2Buffer acTimeCodes
Intended for capture, this is a sequence of NTV2_RP188 values received from the device (in NTV2TCInde...
@ NTV2_TCINDEX_SDI2_LTC
SDI 2 embedded ATC LTC.
std::ostream & DumpBlocks(std::ostream &oss) const
Dumps all 8MB blocks/frames and their tags, if any, into the given stream.
ULWord fLo
| BG 4 | Secs10 | BG 3 | Secs 1 | BG 2 | Frms10 | BG 1 | Frms 1 |
@ NTV2_TCINDEX_SDI1_LTC
SDI 1 embedded ATC LTC.
#define NTV2_IS_STANDARD_TASKS(__m__)
@ kDeviceGetNumAudioSystems
The number of independent Audio Systems on the device.
ULWord fHi
| BG 8 | Hrs 10 | BG 7 | Hrs 1 | BG 6 | Mins10 | BG 5 | Mins 1 |
@ AJA_DebugUnit_Anc2110Xmit
@ kDeviceCanDo2110
True if device supports SMPTE ST2110.
@ NTV2_TCINDEX_SDI1
SDI 1 embedded VITC.
@ NTV2_TCINDEX_SDI2
SDI 2 embedded VITC.
NTV2Standard
Identifies a particular video standard.
virtual bool AutoCirculateGetFrameStamp(const NTV2Channel inChannel, const ULWord inFrameNumber, FRAME_STAMP &outFrameInfo)
Returns precise timing information for the given frame and channel that's currently AutoCirculating.
virtual AJAStatus SetLocationVideoLink(const AJAAncDataLink inLink)
Sets my ancillary data "location" within the video stream.
Declares the AJALock class.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
@ kVRegAncField1Offset
Anc Field1 byte offset from end of frame buffer (GUMP on all boards except RTP for SMPTE2022/IP)
@ NTV2_AncRgn_Field1
Identifies the "normal" Field 1 ancillary data region.
@ AJAAncDataChannel_Y
The ancillary data is associated with the luminance (Y) channel of the video stream.
#define NTV2_IS_INPUT_CROSSPOINT(__x__)
virtual const uint8_t * GetPayloadData(void) const
static bool IsActive(int32_t index)
virtual bool AutoCirculateResume(const NTV2Channel inChannel, const bool inClearDropCount=(0))
Resumes AutoCirculate for the given channel, picking up at the next frame without loss of audio synch...
static const uint16_t sVPIDLineNumsF2[]
bool GetInputTimeCodes(NTV2TimeCodeList &outValues) const
Returns all RP188 timecodes associated with the frame in NTV2TCIndex order.
NTV2Mode
Used to identify the mode of a widget_framestore, or the direction of an AutoCirculate stream: either...
#define NTV2_IS_VALID_STANDARD(__s__)
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC=false, const bool inIsF2=false)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
void SetRP188(const uint32_t inDBB, const uint32_t inLo, const uint32_t inHi, const AJATimeBase &inTimeBase)
Declares the CNTV2Card class.
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
NTV2Crosspoint acCrosspoint
Will be deprecated – used internally by the SDK. Will be removed when the driver changes to use NTV2C...
static const AJA_FrameRate sNTV2Rate2AJARate[]
ULWord GetCapturedAncByteCount(const bool inField2=false) const
@ NTV2_CHANNEL3
Specifies channel or FrameStore 3 (or the 3rd item).
bool CopyFrom(const void *pInSrcBuffer, const ULWord inByteCount)
Replaces my contents from the given memory buffer, resizing me to the new byte count.
std::string NTV2VANCModeToString(const NTV2VANCMode inValue, const bool inCompactDisplay=false)
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
#define NTV2_IS_VANCMODE_ON(__v__)
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
Declares numerous NTV2 utility functions.
NTV2Framesize
Kona2/Xena2 specific enums.
virtual bool S2110DeviceAncToBuffers(const NTV2Channel inChannel, NTV2Buffer &ancF1, NTV2Buffer &ancF2)
@ kDeviceCanDoMultiLinkAudio
True if device supports grouped audio system control.
virtual bool AutoCirculatePause(const NTV2Channel inChannel, const UWord inAtFrameNum=0xFFFF)
Pauses AutoCirculate for the given channel. Once paused, AutoCirculate can be resumed later by callin...
#define AUTOCIRCULATE_WITH_AUDIO_CONTROL
Use this to AutoCirculate with no audio but with audio control.
#define AJA_SUCCESS(_status_)
I interrogate and control an AJA video/audio capture/playout device.
virtual AJAAncillaryData * GetAncillaryDataAtIndex(const uint32_t inIndex) const
Answers with the AJAAncillaryData object at the given index.
virtual std::string AsString(const uint16_t inDumpMaxBytes=0) const
NTV2Crosspoint NTV2ChannelToInputCrosspoint(const NTV2Channel inChannel)
virtual AJAStatus GetTimecode(AJATimeCode &outTimecode, const AJATimeBase &inTimeBase) const
Answers with my timecode "time" as an AJATimeCode.
bool IsStopped(void) const
virtual AJAStatus AddAncillaryData(const AJAAncillaryList &inPackets)
Appends a copy of the given list's packets to me.
NTV2Crosspoint NTV2ChannelToOutputCrosspoint(const NTV2Channel inChannel)
virtual AJAStatus SetPayloadData(const uint8_t *pInData, const uint32_t inByteCount)
Copy data from external memory into my local payload memory.
virtual AJAStatus SetTimecode(const AJATimeCode &inTimecode, const AJATimeBase &inTimeBase, const bool inIsDropFrame)
Sets my timecode "time" from an AJATimeCode.
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
FRAME_STAMP acFrameStamp
Frame stamp for the transferred frame.
NTV2Buffer acANCField2Buffer
The host "Field 2" ancillary data buffer. This field is owned by the client application,...
virtual uint32_t CountAncillaryData(void) const
Answers with the number of AJAAncillaryData objects I contain (any/all types).
virtual AJAStatus SetDBB1PayloadType(const AJAAncillaryData_Timecode_ATC_DBB1PayloadType inType)
Sets my payload type.
virtual bool S2110DeviceAncToXferBuffers(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &inOutXferInfo)
@ NTV2_STANDARD_TASKS
1: Standard/Retail: device configured by AJA ControlPanel, service/daemon, and driver.
uint16_t GetEndFrame(void) const
@ AJAAncillaryData_Timecode_ATC_DBB1PayloadType_VITC2
static const char gFBAllocLockName[]
@ NTV2_AncRgn_MonField2
Identifies the "monitor" or "auxiliary" Field 2 ancillary data region.
NTV2VANCMode
These enum values identify the available VANC modes.
virtual bool GetMode(const NTV2Channel inChannel, NTV2Mode &outValue)
Answers with the current NTV2Mode of the given FrameStore on the AJA device.
NTV2SmpteLineNumber GetSmpteLineNumber(const NTV2Standard inStandard)
For the given video standard, returns the SMPTE-designated line numbers for Field 1 and Field 2 that ...
AUTOCIRCULATE_TRANSFER_STATUS acTransferStatus
Contains status information that's valid after CNTV2Card::AutoCirculateTransfer returns,...
#define NTV2_IS_VALID_CHANNEL(__x__)
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
#define NTV2ChannelToInputChannelSpec
virtual std::string CompareWithInfo(const AJAAncillaryList &inCompareList, const bool inIgnoreLocation=true, const bool inIgnoreChecksum=true) const
Compares me with another list and returns a std::string that contains a human-readable explanation of...
#define AJAAncDataHorizOffset_AnyHanc
HANC – Packet placed/found in any legal area of raster line after EAV.
I am the ATC-specific (analog) subclass of the AJAAncillaryData_Timecode class.
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
void * GetHostPointer(void) const
@ DEVICE_ID_IOIP_2110
See Io IP.
#define AUTOCIRCULATE_WITH_VIDPROC
Use this to AutoCirculate with video processing.
virtual uint32_t CountAncillaryDataWithID(const uint8_t inDID, const uint8_t inSID) const
Answers with the number of AncillaryData objects having the given DataID and SecondaryID.
#define NTV2_IS_QUAD_QUAD_FORMAT(__f__)
#define AUTOCIRCULATE_WITH_FBOCHANGE
Use this to AutoCirculate with the possibility of frame buffer orientation changes.
bool TranslateRegions(ULWordSequence &outRgns, const ULWordSequence &inRgns, const bool inIsQuad, const bool inIsQuadQuad) const
Translates an 8MB-chunked list of regions into another list of regions with frame indexes and sizes e...
virtual AJAStatus SetDBB(uint8_t dbb1, uint8_t dbb2)
@ NTV2_TCINDEX_SDI1_2
SDI 1 embedded VITC 2.
Used to describe Start of Active Video (SAV) location and field dominance for a given NTV2Standard....
virtual AJAStatus SetLocationLineNumber(const uint16_t inLineNum)
Sets my ancillary data "location" frame line number.
@ AJAAncDataStream_1
The ancillary data is associated with DS1 of the video stream (Link A).
Private include file for all ajabase sources.
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO2
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 2.
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
@ kVRegZeroHostAncPostCapture
bool SetInputTimecode(const NTV2TCIndex inTCNdx, const NTV2_RP188 &inTimecode)
Sets one of my input timecodes.
NTV2TCIndexes::const_iterator NTV2TCIndexesConstIter
#define HEX0N(__x__, __n__)
NTV2AutoCirculateState acState
Current AutoCirculate state after the transfer.
@ AJAAncillaryData_Timecode_ATC_DBB1PayloadType_Unknown
bool IsValid(void) const
Answers true if I'm valid, or false if I'm not valid.
void Clear(void)
Clears my data.
#define NTV2_IS_VALID_NTV2CROSSPOINT(__x__)
std::map< NTV2TCIndex, NTV2_RP188 > NTV2TimeCodes
A mapping of NTV2TCIndex enum values to NTV2_RP188 structures.
virtual AJAStatus GetTransmitData(NTV2Buffer &F1Buffer, NTV2Buffer &F2Buffer, const bool inIsProgressive=true, const uint32_t inF2StartLine=0)
Encodes my AJAAncillaryData packets into the given buffers in the default SDI Anc Buffer Data Format ...
This struct replaces the old RP188_STRUCT.
AJAAncillaryData_Timecode_Format
@ kRP188SourceEmbeddedVITC2
#define AUTOCIRCULATE_WITH_HDMIAUX
Use this to AutoCirculate with HDMI auxiliary data.
@ AJA_DebugUnit_Anc2110Rcv
@ NTV2_FRAMERATE_UNKNOWN
Represents an unknown or invalid frame rate.
#define NTV2EndianSwap32BtoH(__val__)
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
bool SetAllOutputTimeCodes(const NTV2_RP188 &inTimecode, const bool inIncludeF2=true)
Intended for playout, replaces all elements of my acOutputTimeCodes member with the given timecode va...
static AJALock gFBAllocLock(gFBAllocLockName)
uint16_t GetStartFrame(void) const
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ NTV2_TCINDEX_DEFAULT
The "default" timecode (mostly used by the AJA "Retail" service and Control Panel)
static const uint16_t sVPIDLineNumsF1[]
#define AUTOCIRCULATE_WITH_LTC
Use this to AutoCirculate with analog LTC.
Declares the CRP188 class. See SMPTE RP188 standard for details.
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
@ AJAAncDataStream_2
The ancillary data is associated with DS2 of the video stream (Link A).
void SetDropFrame(bool bDropFrameFlag)
#define NTV2_IS_QUAD_FRAME_FORMAT(__f__)
#define NTV2_IS_VALID_NTV2FrameRate(__r__)
virtual AJAStatus GeneratePayloadData(void)
Generate the payload data from the "local" ancillary data.
double GetAudioSamplesPerSecond(const NTV2AudioRate inAudioRate)
Returns the audio sample rate as a number of audio samples per second.
NTV2Buffer acOutputTimeCodes
Intended for playout, this is an ordered sequence of NTV2_RP188 values to send to the device....
@ NTV2_MODE_INVALID
The invalid mode.
std::string NTV2ChannelToString(const NTV2Channel inValue, const bool inForRetailDisplay=false)
#define IS_LINKB_AJAAncDataStream(_x_)
void QueryString(std::string &str, const AJATimeBase &timeBase, bool bDropFrame, bool bStdTcForHfr, AJATimecodeNotation notation=AJA_TIMECODE_LEGACY)
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
#define NTV2_IS_OUTPUT_CROSSPOINT(__x__)
ULWord GetFrameCount(void) const
@ kVRegZeroDeviceAncPostCapture
#define AUTOCIRCULATE_WITH_COLORCORRECT
Use this to AutoCirculate with color correction.
LWord64 acFrameTime
(input/ingest/capture only) The absolute timestamp at the VBI when the frame started recording into d...
#define NTV2_IS_INPUT_MODE(__mode__)
ULWord frame
The frame requested or -1 if not available.
bool GetBadRegions(ULWordSequence &outBlks) const
Answers with the list of colliding and illegal memory regions.
ULWord acRequestedFrame
On entry for NTV2_TYPE_ACFRAMESTAMP message, the requested frame. Upon exit, 0xFFFFFFFF means "not ...
#define xHEX0N(__x__, __n__)
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
#define AJA_FAILURE(_status_)
@ NTV2_AUDIO_RATE_INVALID
#define NTV2_IS_SUPPORTED_NTV2FrameRate(__r__)
@ DEVICE_ID_IOIP_2110_RGB12
See Io IP.
#define NTV2_IS_VALID_AUDIO_SYSTEM(__x__)
@ NTV2_TCINDEX_LTC1
Analog LTC 1.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
NTV2Buffer acANCBuffer
The host ancillary data buffer. This field is owned by the client application, and thus is responsibl...
NTV2Crosspoint
Logically, these are an NTV2Channel combined with an NTV2Mode.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
const FRAME_STAMP & GetFrameStamp(void) const
#define NTV2_IS_OUTPUT_MODE(__mode__)
virtual bool AutoCirculateFlush(const NTV2Channel inChannel, const bool inClearDropCount=(0))
Flushes AutoCirculate for the given channel.
@ AJAAncDataLink_A
The ancillary data is associated with Link A of the video stream.
@ AJAAncDataType_Timecode_ATC
SMPTE 12-M Ancillary Timecode (formerly known as "RP-188")
Declares the AJADebug class.
#define NTV2_IS_PROGRESSIVE_STANDARD(__s__)
@ NTV2_OEM_TASKS
2: OEM (recommended): device configured by client application(s) with some driver involvement.
virtual bool GetAutoCirculate(NTV2Crosspoint channelSpec, AUTOCIRCULATE_STATUS_STRUCT *autoCirculateStatus)
virtual AJAStatus SetLocationDataChannel(const AJAAncDataChannel inChannel)
Sets my ancillary data "location" data channel value (Y or C).
NTV2AudioSystem GetAudioSystem(void) const
NTV2_RP188 acRP188
Will be deprecated – use AUTOCIRCULATE_TRANSFER::SetOutputTimeCode instead.
@ NTV2_AUTOCIRCULATE_DISABLED
The AutoCirculate channel is stopped.
Utility class for timecodes.
@ NTV2_AUDIOSYSTEM_INVALID