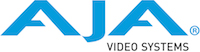 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
11 #if defined (AJALinux)
13 #elif defined (MSWindows)
14 #pragma warning(disable: 4800)
47 if (!GetHDMIInputStatusRegNum(regNum, inChannel, in12BitDetection))
49 return ReadRegister (regNum, outValue);
142 if (!GetHDMIInDynamicRange(registerValues, inChannel))
159 outIsDolbyVision =
false;
208 if (numInputs <=
UWord(inChannel))
219 ULWord status(0), maskVal, shiftVal;
223 if (!GetHDMIInputStatus(status, inChannel, bV2))
233 if (!GetHDMIInputStatus(status, inChannel))
243 if (!GetHDMIInputStatus(status, inChannel))
246 outIsLocked = (status & (
BIT(0) |
BIT(1))) == (
BIT(0) |
BIT(1));
254 const ULWord tempVal (!value);
267 outEnabled = !(
static_cast <bool> (tempVal));
288 outIsSwapped =
false;
402 ULWord newCorrectValue (0);
407 default:
return false;
429 default:
return false;
624 GetEveryFrameServices(taskMode);
644 GetEveryFrameServices(taskMode);
664 GetEveryFrameServices(taskMode);
684 GetEveryFrameServices(taskMode);
704 GetEveryFrameServices(taskMode);
724 GetEveryFrameServices(taskMode);
744 GetEveryFrameServices(taskMode);
764 GetEveryFrameServices(taskMode);
784 GetEveryFrameServices(taskMode);
804 GetEveryFrameServices(taskMode);
824 GetEveryFrameServices(taskMode);
844 GetEveryFrameServices(taskMode);
864 GetEveryFrameServices(taskMode);
878 uint32_t regValue = 0;
880 return regValue ?
true :
false;
887 GetEveryFrameServices(taskMode);
907 GetEveryFrameServices(taskMode);
937 uint32_t regValue = 0;
939 return regValue ?
true :
false;
956 uint32_t regValue = 0;
958 return regValue ?
true :
false;
965 SetHDRData(registerValues);
975 SetHDMIHDRRedPrimaryX(inRegisterValues.
redPrimaryX);
976 SetHDMIHDRRedPrimaryY(inRegisterValues.
redPrimaryY);
977 SetHDMIHDRWhitePointX(inRegisterValues.
whitePointX);
978 SetHDMIHDRWhitePointY(inRegisterValues.
whitePointY);
991 GetHDRData(registerValues);
1000 GetHDMIHDRBluePrimaryY(outRegisterValues.
bluePrimaryY);
1001 GetHDMIHDRRedPrimaryX(outRegisterValues.
redPrimaryX);
1002 GetHDMIHDRRedPrimaryY(outRegisterValues.
redPrimaryY);
1003 GetHDMIHDRWhitePointX(outRegisterValues.
whitePointX);
1004 GetHDMIHDRWhitePointY(outRegisterValues.
whitePointY);
1018 EnableHDMIHDR(
false);
1019 SetHDRData(registerValues);
1020 EnableHDMIHDR(
true);
1028 EnableHDMIHDR(
false);
1029 SetHDRData(registerValues);
1030 EnableHDMIHDR(
true);
1036 outIsSwapped =
false;
1080 #pragma warning(default: 4800)
@ kVRegShiftHDMIInColorimetry
void setHDRDefaultsForBT2020(HDRRegValues &outRegisterValues)
@ DEVICE_ID_KONALHIDVI
See KONA LHi.
uint16_t maxContentLightLevel
virtual bool SetHDMIInAudioChannel34Swap(const bool inIsSwapped, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the given HDMI input's audio channel 3/4 swap state.
virtual bool GetHDMIHDREnabled(void)
virtual bool EnableHDMIHDR(const bool inEnableHDMIHDR)
Enables or disables HDMI HDR.
virtual bool GetHDMIOutDownstreamBitDepth(NTV2HDMIBitDepth &outValue)
@ kVRegHdrMasterLumMaxCh1
@ kRegShiftHDRStaticMetadataDescriptorID
@ kVRegHDMIInDrmBluePrimary1
virtual bool GetHDMIOutTsiIO(bool &tsiEnabled)
@ kRegShiftHDMIHDRWhitePointY
virtual bool GetHDMIOutPrefer420(bool &outValue)
virtual bool SetHDMIHDRStaticMetadataDescriptorID(const uint8_t inSMDId)
UWord NTV2DeviceGetNumHDMIVideoInputs(const NTV2DeviceID inDeviceID)
virtual bool GetHDMIHDRWhitePointX(uint16_t &outWhitePointX)
Answers with the Display Mastering data for White Point X as defined in SMPTE ST 2086....
virtual bool EnableHDMIOutCenterCrop(const bool inEnable)
Controls the 4k/2k -> UHD/HD HDMI center cropping feature.
@ DEVICE_ID_KONAHDMI
See KONA HDMI.
virtual bool GetHDMIOutStatus(NTV2HDMIOutputStatus &outStatus)
Answers with the current HDMI output status.
virtual bool GetHDMIInAudioSampleRateConverterEnable(bool &outIsEnabled, const NTV2Channel inChannel=NTV2_CHANNEL1)
NTV2HDMIBitDepth
Indicates or specifies the HDMI video bit depth.
@ NTV2_CHANNEL2
Specifies channel or FrameStore 2 (or the 2nd item).
virtual bool SetHDMIOutVideoStandard(const NTV2Standard inNewValue)
@ NTV2_HDMIAudio2Channels
2 audio channels
virtual bool GetHDMIOutColorSpace(NTV2HDMIColorSpace &outValue)
@ kRegShiftHDMIHDRGreenPrimaryX
Declares device capability functions.
@ kRegMaskHDMIOutForceConfig
@ kRegMaskHDMIHDRBluePrimaryY
virtual bool GetHDMIHDRDolbyVisionEnabled(void)
virtual bool GetHDMIOutSampleStructure(NTV2HDMISampleStructure &outValue)
@ NTV2_HDMIColorimetryNoData
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
NTV2HDMIColorSpace
Indicates or specifies HDMI Color Space.
@ kRegShiftHDMIOutVideoStd
@ kLHIRegMaskHDMIInputProtocol
@ kRegMaskHDMIHDRRedPrimaryX
@ kVRegHDMIInDrmRedPrimary1
@ kRegShiftHDMIOutForceConfig
virtual bool GetHDMIInBitDepth(NTV2HDMIBitDepth &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current bit depth setting.
virtual bool SetHDMIOutRange(const NTV2HDMIRange inNewValue)
virtual bool GetHDMIOutVideoFPS(NTV2FrameRate &outValue)
static const ULWord gHDMIChannelToControlRegNum[]
@ kRegMaskHDMIHDRGreenPrimaryX
@ kRegMaskHDMIHDRMaxMasteringLuminance
virtual bool GetHDMIOutAudioChannel34Swap(bool &outIsSwapped, const NTV2Channel inWhichHDMIOut=NTV2_CHANNEL1)
Answers with the HDMI output's current audio channel 3/4 swap setting.
@ kRegShiftHDMIHDRMaxContentLightLevel
virtual bool EnableHDMIOutUserOverride(const bool inEnable)
Enables or disables override of HDMI parameters.
@ kRegShiftHDMIColorSpace
virtual bool SetHDMIOutVideoFPS(const NTV2FrameRate inNewValue)
@ kRegHDMIHDRGreenPrimary
virtual bool GetHDMIOutDecimateMode(bool &outIsEnabled)
virtual bool GetHDMIOut3DMode(NTV2HDMIOut3DMode &outValue)
NTV2HDMIAudioChannels
Indicates or specifies the HDMI audio channel count.
@ kRegShiftHDMIV2TxBypass
@ kRegMaskHDMIOut3DPresent
virtual bool SetHDMIHDRBT2020(void)
virtual bool SetHDMIInAudioSampleRateConverterEnable(const bool inNewValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool GetHDMIHDRBluePrimaryY(uint16_t &outBluePrimaryY)
Answers with the Display Mastering data for Blue Primary Y as defined in SMPTE ST 2086....
@ kLHIRegShiftHDMIInputProtocol
@ kRegMaskElectroOpticalTransferFunction
@ kRegShiftHDMISampleRateConverterEnable
virtual bool GetHDMIHDRGreenPrimaryY(uint16_t &outGreenPrimaryY)
Answers with the Display Mastering data for Green Primary Y as defined in SMPTE ST 2086....
virtual bool SetHDMIOutAudioChannel34Swap(const bool inIsSwapped, const NTV2Channel inWhichHDMIOut=NTV2_CHANNEL1)
Sets the HDMI output's audio channel 3/4 swap state.
virtual bool SetHDMIHDRConstantLuminance(const bool inEnableConstantLuminance)
Enables or disables BT.2020 Y'cC'bcC'rc versus BT.2020 Y'C'bC'r or R'G'B'.
bool convertHDRRegisterToFloatValues(const HDRRegValues &inRegisterValues, HDRFloatValues &outFloatValues)
@ NTV2_LHIHDMIColorSpaceRGB
@ NTV2_INVALID_HDMI_AUDIO_CHANNELS
virtual bool GetEnableHDMIOutCenterCrop(bool &outIsEnabled)
Answers if the HDMI 4k/2k -> UHD/HD center cropping is enabled or not.
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
@ kRegShiftHDMIHDRGreenPrimaryY
virtual bool SetHDMIOutSampleStructure(const NTV2HDMISampleStructure inNewValue)
void Clear(void)
Resets me to an invalid state.
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
@ kVRegHDMIInDrmLightLevel2
@ NTV2_FIELD0
Identifies the first field in time for an interlaced video frame, or the first and only field in a pr...
virtual bool SetHDMIHDRGreenPrimaryX(const uint16_t inGreenPrimaryX)
Sets the Display Mastering data for Green Primary X as defined in SMPTE ST 2086. This is Byte 3 and 4...
void setHDRDefaultsForDCIP3(HDRRegValues &outRegisterValues)
virtual bool GetHDMIInAudioChannel34Swap(bool &outIsSwapped, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current audio channel 3/4 swap setting.
NTV2HDMIRange
Indicates or specifies the HDMI RGB range.
@ kVRegHDMIInDrmBluePrimary2
@ kRegShiftHDMIInputRange
Reports HDMI output status information.
@ NTV2_HDMIAudio8Channels
8 audio channels
@ kVRegHDMIInDrmMasteringLuminence1
NTV2FrameRate
Identifies a particular video frame rate.
virtual bool GetHDMIOutDownstreamColorSpace(NTV2LHIHDMIColorSpace &outValue)
uint16_t maxFrameAverageLightLevel
@ kRegShiftHDMIHDRWhitePointX
@ kRegMaskHDMIHDRNonContantLuminance
@ kVRegHDMIInDrmGreenPrimary1
bool convertHDRFloatToRegisterValues(const HDRFloatValues &inFloatValues, HDRRegValues &outRegisterValues)
#define NTV2_IS_VALID_HDR_MASTERING_LUMINENCE(__val__)
virtual bool GetEnableHDMIOutUserOverride(bool &outIsEnabled)
Answers if override of HDMI parameters is enabled or not.
@ kVRegHDMIInDrmGreenPrimary2
virtual bool GetHDMIHDRBluePrimaryX(uint16_t &outBluePrimaryX)
Answers with the Display Mastering data for Blue Primary X as defined in SMPTE ST 2086....
uint16_t minMasteringLuminance
@ kLHIRegMaskHDMIOutColorSpace
virtual bool SetHDMIHDRMaxContentLightLevel(const uint16_t inMaxContentLightLevel)
Sets the Display Mastering data for the Max Content Light Level(Max CLL) value. This is Byte 23 and 2...
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
@ kRegMaskHDMISwapOutputAudCh34
ULWord NTV2DeviceGetHDMIVersion(const NTV2DeviceID inDeviceID)
@ kRegMaskHDMIHDRDolbyVisionEnable
static bool isEnabled(CNTV2Card &device, const NTV2Channel inChannel)
virtual bool SetHDMIOutLevelBMode(const bool inEnable)
Enables or disables level-B mode on the device's HDMI rasterizer.
@ kRegMaskHDMIOutVideoStd
@ kRegShiftHDMIOut3DPresent
@ kLHIRegShiftHDMIInputBitDepth
@ kRegHDMIHDRMasteringLuminence
@ kRegShiftHDMISwapInputAudCh34
virtual bool GetHDMIHDRMaxFrameAverageLightLevel(uint16_t &outMaxFrameAverageLightLevel)
Answers with the Display Mastering data for the Max Frame Average Light Level(Max FALL) value....
@ kRegShiftHDMIInColorDepth
@ kRegMaskHDRStaticMetadataDescriptorID
virtual bool GetHDMIOutProtocol(NTV2HDMIProtocol &outValue)
virtual bool GetHDMIOutLevelBMode(bool &outIsEnabled)
virtual bool GetHDMIOutRange(NTV2HDMIRange &outValue)
NTV2Standard
Identifies a particular video standard.
virtual bool SetHDMIHDRDCIP3(void)
virtual bool SetHDMIOut3DPresent(const bool inIs3DPresent)
@ kDeviceCanDoHDMIHDROut
True if device supports HDMI HDR output.
@ kRegMaskHDMIHDRBluePrimaryX
#define NTV2_IS_VALID_HDMI_SAMPLE_STRUCT(_x_)
@ kLHIRegMaskHDMIOutBitDepth
virtual bool GetHDMIInputStatus(ULWord &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1, const bool in12BitDetection=(0))
Answers with the contents of the HDMI Input status register for the given HDMI input.
@ kLHIRegMaskHDMIOutputEDID10Bit
@ kRegMaskHDMIHDRMinMasteringLuminance
@ kVRegHDMIInDrmWhitePoint2
#define NTV2_IS_VALID_HDMI_RANGE(__x__)
NTV2HDMIProtocol
Indicates or specifies the HDMI protocol.
virtual bool SetHDMIOutForceConfig(const bool inNewValue)
#define NTV2_IS_VALID_HDMI_PROTOCOL(__x__)
Declares the CNTV2Card class.
virtual bool GetHDMIOutVideoStandard(NTV2Standard &outValue)
@ kRegMaskHDMIHDRWhitePointX
@ kRegMaskHDMIHDRRedPrimaryY
virtual bool GetHDMIHDRMinMasteringLuminance(uint16_t &outMinMasteringLuminance)
Answers with the Display Mastering data for the Min Mastering Luminance value as defined in SMPTE ST ...
@ kDeviceGetHDMIVersion
The version number of the HDMI chipset on the device.
@ kRegMaskHDMIHDRGreenPrimaryY
virtual bool SetHDMIHDRElectroOpticalTransferFunction(const uint8_t inEOTFByte)
@ kRegShiftRasterDecimate
virtual bool GetHDRData(HDRFloatValues &outFloatValues)
@ NTV2_HDMIColorSpaceYCbCr
YCbCr color space.
UWord NTV2DeviceGetNumHDMIVideoOutputs(const NTV2DeviceID inDeviceID)
virtual bool GetHDMIHDRElectroOpticalTransferFunction(uint8_t &outEOTFByte)
virtual bool GetLHIHDMIOutColorSpace(NTV2LHIHDMIColorSpace &outValue)
virtual bool SetHDMIOutBitDepth(const NTV2HDMIBitDepth inNewValue)
Declares numerous NTV2 utility functions.
virtual bool GetHDMIInDolbyVision(bool &outIsDolbyVision, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's Dolby Vision flag is set.
@ kVRegMaskHDMIInColorimetry
virtual bool SetHDMIOutPrefer420(const bool inNewValue)
virtual bool GetHDMIInputAudioChannels(NTV2HDMIAudioChannels &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current number of audio channels being received on the given HDMI input.
@ kRegShiftHDMIHDRMaxMasteringLuminance
virtual bool GetHDMIInProtocol(NTV2HDMIProtocol &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's protocol.
virtual bool EnableHDMIHDRDolbyVision(const bool inEnable)
Enables or disables HDMI HDR Dolby Vision.
#define NTV2_IS_VALID_HDR_PRIMARY(__val__)
@ kRegMaskHDMIHDRMaxContentLightLevel
virtual bool SetHDMIHDRMaxMasteringLuminance(const uint16_t inMaxMasteringLuminance)
Sets the Display Mastering data for the Max Mastering Luminance value as defined in SMPTE ST 2086....
virtual bool SetHDMIInColorSpace(const NTV2HDMIColorSpace inNewValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the given HDMI input's color space.
@ kLHIRegMaskHDMIOutputEDIDRGB
virtual bool SetHDMIHDRMinMasteringLuminance(const uint16_t inMinMasteringLuminance)
Sets the Display Mastering data for the Min Mastering Luminance value as defined in SMPTE ST 2086....
@ kRegShiftHDMIHDRBluePrimaryY
@ kRegMaskHDMISampleRateConverterEnable
virtual bool SetHDMIInBitDepth(const NTV2HDMIBitDepth inNewValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the given HDMI input's bit depth.
uint16_t maxMasteringLuminance
@ NTV2_INVALID_HDMI_PROTOCOL
virtual bool GetHDMIV2Mode(NTV2HDMIV2Mode &outMode)
Answers with the current HDMI V2 mode of the device.
virtual bool GetHDMIOut3DPresent(bool &outIs3DPresent)
virtual bool SetHDMIHDRRedPrimaryX(const uint16_t inRedPrimaryX)
Sets the Display Mastering data for Red Primary X as defined in SMPTE ST 2086. This is Byte 11 and 12...
@ kVRegHDMIInDrmLightLevel1
@ kVRegMaskHDMIInDolbyVision
@ NTV2_INVALID_HDMIBitDepth
NTV2HDMIColorimetry
Indicates or specifies the HDMI colorimetry.
virtual bool GetHDMIInVideoRange(NTV2HDMIRange &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's video black/white range.
virtual bool SetHDMIHDRWhitePointX(const uint16_t inWhitePointX)
Sets the Display Mastering data for White Point X as defined in SMPTE ST 2086. This is Byte 15 and 16...
virtual bool GetHDMIInColorSpace(NTV2HDMIColorSpace &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current color space setting.
@ kVRegShiftHDMIInDolbyVision
@ kRegMaskInputStatusLock
virtual bool GetHDMIHDRMaxContentLightLevel(uint16_t &outMaxContentLightLevel)
Answers with the Display Mastering data for the Max Content Light Level(Max CLL) value....
virtual bool GetHDMIHDRGreenPrimaryX(uint16_t &outGreenPrimaryX)
Answers with the Display Mastering data for Green Primary X as defined in SMPTE ST 2086....
virtual bool GetHDMIHDRWhitePointY(uint16_t &outWhitePointY)
Answers with the Display Mastering data for White Point Y as defined in SMPTE ST 2086....
@ NTV2_LHIHDMIColorSpaceYCbCr
virtual bool SetHDMIHDRBluePrimaryX(const uint16_t inBluePrimaryX)
Sets the Display Mastering data for Blue Primary X as defined in SMPTE ST 2086. This is Byte 7 and 8 ...
#define NTV2_IS_VALID_HDR_LIGHT_LEVEL(__val__)
static const ULWord gHDMIChannelToInputStatusRegNum[]
@ kLHIRegShiftHDMIOutputEDIDRGB
@ kDeviceGetNumHDMIVideoOutputs
The number of HDMI video outputs on the device.
virtual bool GetHDMIHDRRedPrimaryX(uint16_t &outRedPrimaryX)
Answers with the Display Mastering data for Red Primary X as defined in SMPTE ST 2086....
@ kRegMaskHDMISwapInputAudCh34
virtual bool GetHDMIOutForceConfig(bool &outValue)
@ kLHIRegMaskHDMIInputColorSpace
@ kLHIRegShiftHDMIOutputEDID10Bit
virtual bool GetHDMIInIsLocked(bool &outIsLocked, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers if the given HDMI input is genlocked or not.
@ kRegShiftHDMIHDRRedPrimaryY
virtual bool GetHDMIHDRConstantLuminance(void)
@ kVRegHDMIInDrmMasteringLuminence2
virtual bool GetHDMIHDRStaticMetadataDescriptorID(uint8_t &outSMDId)
virtual bool GetHDMIInColorimetry(NTV2HDMIColorimetry &outColorimetry, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current colorimetry.
@ kLHIRegMaskHDMIInputBitDepth
virtual bool SetHDMIOutProtocol(const NTV2HDMIProtocol inNewValue)
@ kLHIRegShiftHDMIInputColorSpace
virtual bool SetHDMIHDRRedPrimaryY(const uint16_t inRedPrimaryY)
Sets the Display Mastering data for Red Primary Y as defined in SMPTE ST 2086. This is Byte 13 and 14...
@ kVRegMaskHDMIInMetadataID
virtual bool SetHDMIOutTsiIO(const bool inTsiEnable)
Enables or disables two sample interleave I/O mode on the device's HDMI rasterizer.
@ kVRegHDMIInDrmWhitePoint1
virtual bool SetHDMIHDRWhitePointY(const uint16_t inWhitePointY)
Sets the Display Mastering data for White Point Y as defined in SMPTE ST 2086. This is Byte 17 and 18...
@ kVRegShiftHDMIInMetadataID
virtual bool GetHDMIInputColor(NTV2LHIHDMIColorSpace &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current colorspace for the given HDMI input.
@ kRegMaskHDMIHDRWhitePointY
virtual bool GetHDMIOutBitDepth(NTV2HDMIBitDepth &outValue)
virtual bool GetHDMIHDRRedPrimaryY(uint16_t &outRedPrimaryY)
Answers with the Display Mastering data for Red Primary Y as defined in SMPTE ST 2086....
static const ULWord gKonaHDMICtrlRegs[]
virtual bool SetHDMIOutColorSpace(const NTV2HDMIColorSpace inNewValue)
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
@ kRegShiftHDMIOutCropEnable
@ kRegMaskHDMIOutPrefer420
@ kRegShiftHDMISwapOutputAudCh34
@ kRegMaskHDMIOutCropEnable
#define NTV2_IS_VALID_NTV2FrameRate(__r__)
@ kRegShiftHDMIHDRMinMasteringLuminance
@ kLHIRegMaskHDMIInput2ChAudio
@ kRegMaskHDMIHDRMaxFrameAverageLightLevel
virtual bool SetHDMIInputRange(const NTV2HDMIRange inNewValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the given HDMI input's input range.
@ kRegShiftHDMIOutPrefer420
@ kRegMaskHDMIOutV2VideoStd
virtual bool SetHDMIV2Mode(const NTV2HDMIV2Mode inMode)
Sets HDMI V2 mode for the device.
#define NTV2_IS_VALID_HDMI_BITDEPTH(__x__)
@ kVRegHDMIInDrmRedPrimary2
bool SetFromRegValue(const ULWord inData)
Sets my fields from the given status register value.
virtual bool SetHDMIOutDecimateMode(const bool inEnable)
Enables or disables decimate mode on the device's HDMI rasterizer, which halves the output frame rate...
@ NTV2_HDMIColorSpaceRGB
RGB color space.
@ kRegShiftHDMIHDRMaxFrameAverageLightLevel
@ kRegShiftHDMIHDRDolbyVisionEnable
virtual bool SetHDMIHDRGreenPrimaryY(const uint16_t inGreenPrimaryY)
Sets the Display Mastering data for Green Primary Y as defined in SMPTE ST 2086. This is Byte 5 and 6...
virtual bool SetHDMIHDRBluePrimaryY(const uint16_t inBluePrimaryY)
Sets the Display Mastering data for Blue Primary Y as defined in SMPTE ST 2086. This is Byte 9 and 10...
@ kRegShiftElectroOpticalTransferFunction
@ kRegShiftHDMIHDRNonContantLuminance
virtual bool SetHDMIHDRMaxFrameAverageLightLevel(const uint16_t inMaxFrameAverageLightLevel)
Sets the Display Mastering data for the Max Frame Average Light Level(Max FALL) value....
virtual bool GetHDMIInputRange(NTV2HDMIRange &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current input range setting.
virtual bool GetHDMIInputStatusRegNum(ULWord &outRegNum, const NTV2Channel inChannel=NTV2_CHANNEL1, const bool in12BitDetection=(0))
Answers with the HDMI Input status register number for the given HDMI input.
@ kRegShiftHDMIHDRRedPrimaryX
virtual bool SetHDRData(const HDRFloatValues &inFloatValues)
@ kRegMaskHDMIInColorDepth
@ kRegShiftHDMIHDRBluePrimaryX
NTV2HDMIOut3DMode
This specifies the HDMI Out Stereo 3D Mode.
@ kLHIRegShiftHDMIOutBitDepth
virtual bool SetHDMIV2TxBypass(const bool inBypass)
@ kVRegHdrMasterLumMinCh1
@ kLHIRegShiftHDMIOutColorSpace
virtual bool SetLHIHDMIOutColorSpace(const NTV2LHIHDMIColorSpace inNewValue)
uint8_t staticMetadataDescriptorID
virtual bool SetHDMIOut3DMode(const NTV2HDMIOut3DMode inValue)
uint8_t electroOpticalTransferFunction
virtual bool GetHDMIInDynamicRange(HDRRegValues &outRegValues, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's video dynamic range and mastering information.
virtual bool GetHDMIHDRMaxMasteringLuminance(uint16_t &outMaxMasteringLuminance)
Answers with the Display Mastering data for the Max Mastering Luminance value as defined in SMPTE ST ...
@ NTV2_OEM_TASKS
2: OEM (recommended): device configured by client application(s) with some driver involvement.
#define NTV2_IS_DRIVER_ACTIVE_TASKS(__m__)