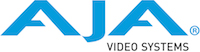 |
AJA NTV2 SDK
17.6.0.1664
NTV2 SDK 17.6.0.1664
|
Go to the documentation of this file.
15 #include "ajabase/persistence/persistence.h"
22 #if defined(MSWindows)
23 #define PATH_DELIMITER "\\"
25 #define PATH_DELIMITER "/"
42 static string makeHeader(ostringstream & oss,
const string & inName)
44 oss << setfill(
'=') << setw(96) <<
" " << inName <<
":" << setfill(
' ') << endl << endl;
64 const string sigStr(NTV2_4CC_AS_STRING(inAppSignature));
65 if (isprint(sigStr.at(0)) && isprint(sigStr.at(1)) && isprint(sigStr.at(2)) && isprint(sigStr.at(3)))
66 oss <<
"'" << sigStr <<
"'";
67 else if (inAppSignature)
68 oss <<
"0x" << hex << setw (8) << setfill (
'0') << inAppSignature << dec <<
" (" << inAppSignature <<
")";
77 #if defined (MSWindows)
87 #elif defined (AJALinux)
89 #elif defined (AJAMac)
108 static uint32_t gMaxSampleCount16 [] = { 16320, 65280, 32640, 130560, 0 };
109 static uint32_t gMaxSampleCount8 [] = { 32640, 130560, 65280, 261120, 0 };
110 static uint32_t gMaxSampleCount6 [] = { 87040, 174080, 87040, 348160, 0 };
112 switch (inChannelCount)
114 case 16:
return gMaxSampleCount16 [inBufferSize];
115 case 8:
return gMaxSampleCount8 [inBufferSize];
116 case 6:
return gMaxSampleCount6 [inBufferSize];
139 device.
GetMode(inChannel, result);
225 outChannelPairsPresent.clear();
226 switch (inAudioSource)
228 default:
return false;
250 outChannelPairsPresent.insert (chPair);
263 bool bBitFileInfoAvailable =
false;
265 if( bBitFileInfoAvailable )
268 if (outDateString.find(
".ncd") != string::npos)
270 outDateString = outDateString.substr(0, outDateString.find(
".ncd"));
271 outDateString +=
".bit ";
272 outDateString += bitFileInfo.
dateStr;
273 outDateString +=
" ";
274 outDateString += bitFileInfo.
timeStr;
276 else if (outDateString.find(
";") != string::npos)
278 outDateString = outDateString.substr(0, outDateString.find(
";"));
279 outDateString +=
".bit ";
280 outDateString += bitFileInfo.
dateStr;
281 outDateString +=
" ";
282 outDateString += bitFileInfo.
timeStr;
284 else if (outDateString.find(
".bit") != string::npos && outDateString !=
".bit")
287 outDateString +=
" ";
288 outDateString += bitFileInfo.
dateStr;
289 outDateString +=
" ";
290 outDateString += bitFileInfo.
timeStr;
294 outDateString =
"bad bitfile date string";
337 if (mPrependMap.find(section) != mPrependMap.end())
339 mPrependMap.at(section).insert(0,
"\n");
340 mPrependMap.at(section).insert(0, sectionData);
344 mPrependMap[section] = sectionData;
345 mPrependMap.at(section).append(
"\n");
351 if (mAppendMap.find(section) != mAppendMap.end())
353 mAppendMap.at(section).append(
"\n");
354 mAppendMap.at(section).append(sectionData);
358 mAppendMap[section] =
"\n";
359 mAppendMap.at(section).append(sectionData);
367 oss << sectionData <<
"\n";
368 mHeaderStr.append(oss.str());
375 oss << sectionData <<
"\n";
376 mFooterStr.append(oss.str());
384 #define LoggerSectionToFunctionMacro(_SectionEnum_, _SectionString_, _SectionMethod_) \
385 if (mSections & _SectionEnum_) \
387 makeHeader(oss, _SectionString_); \
388 if (mPrependMap.find(_SectionEnum_) != mPrependMap.end()) \
389 oss << mPrependMap.at(_SectionEnum_); \
391 _SectionMethod_(oss); \
393 if (mAppendMap.find(_SectionEnum_) != mAppendMap.end()) \
394 oss << mAppendMap.at(_SectionEnum_); \
400 vector<char> dateBufferLocal(128, 0);
401 vector<char> dateBufferUTC(128, 0);
405 struct tm *localTimeinfo;
406 localTimeinfo = localtime(
reinterpret_cast<const time_t*
>(&now));
407 strcpy(&dateBufferLocal[0],
"");
409 ::strftime(&dateBufferLocal[0], dateBufferLocal.size(),
"%B %d, %Y %I:%M:%S %p %Z (local)", localTimeinfo);
411 struct tm *utcTimeinfo;
412 utcTimeinfo = gmtime(
reinterpret_cast<const time_t*
>(&now));
413 strcpy(&dateBufferUTC[0],
"");
415 ::strftime(&dateBufferUTC[0], dateBufferUTC.size(),
"%Y-%m-%dT%H:%M:%SZ UTC", utcTimeinfo);
417 oss <<
"Begin NTV2 Support Log" <<
"\n" <<
419 "Generated: " << &dateBufferLocal[0] <<
420 " " << &dateBufferUTC[0] <<
"\n\n" << flush;
422 if (!mHeaderStr.empty())
428 if (mDevice.IsOpen())
436 if (!mFooterStr.empty())
438 oss << endl <<
"End NTV2 Support Log";
447 static inline string HEX0NStr (
const uint32_t inNum,
const uint16_t inWidth) {ostringstream oss; oss <<
HEX0N(inNum,inWidth);
return oss.str();}
448 static inline string xHEX0NStr(
const uint32_t inNum,
const uint16_t inWidth) {ostringstream oss; oss <<
xHEX0N(inNum,inWidth);
return oss.str();}
449 template <
typename T>
string DECStr (
const T inT) {ostringstream oss; oss <<
DEC(inT);
return oss.str();}
451 void CNTV2SupportLogger::FetchInfoLog (ostringstream & oss)
const
458 if (mDevice.IsOpen())
462 ULWord drvrType(0), dextType(0x44455854);
465 str =
"Kernel Extension ('KEXT')";
466 else if (drvrType == dextType)
467 str =
"DriverKit ('DEXT')";
470 oss <<
"(Unknown/Invalid " <<
xHEX0N(drvrType,8) <<
")";
474 #endif // defined(AJAMac)
478 if (mDevice.IsOpen())
488 string dateStr, timeStr, connType;
497 std::string urlString;
504 if (mDevice.IsIPDevice())
517 if (ntv2Card.ReadMACAddresses(mac1, mac2))
527 ULWord dnaLo(0), dnaHi(0);
534 ntv2Card.ReadLicenseInfo(licenseInfo);
546 if (mDevice.IsRemote())
548 if (!mDevice.GetHostName().empty())
555 if (!connType.empty())
567 std::vector<std::pair<std::string, bool> > persistenceChecks;
568 persistenceChecks.push_back(std::pair<std::string, bool>(
"User Persistence Health",
false));
569 persistenceChecks.push_back(std::pair<std::string, bool>(
"System Persistence Health",
true));
570 std::vector<std::pair<std::string, bool> >::const_iterator it(persistenceChecks.begin());
572 std::string errMessage;
573 for (; it != persistenceChecks.end(); ++it)
575 std::string label(it->first);
576 bool shared(it->second);
577 AJAPersistence p(
"com.aja.devicesettings",
"Unknown",
"00000000", shared);
578 if (p.StorageHealthCheck(errCode, errMessage))
584 if (shared && errCode == -1)
595 void CNTV2SupportLogger::FetchRegisterLog (ostringstream & oss)
const
601 static const string sDashes (96,
'-');
606 deviceRegs.insert(regNum);
608 oss << endl << deviceRegs.size() <<
" Device Registers " << sDashes << endl << endl;
611 oss <<
"## NOTE: Driver failed to return one or more registers (those will be zero)" << endl;
615 const uint32_t regNum (regInfo.registerNumber);
617 const uint32_t
value (regInfo.registerValue);
620 <<
"Register Number: " << regNum << endl
621 <<
"Register Value: " <<
value <<
" : " <<
xHEX0N(value,8) << endl
627 oss << endl << virtualRegs.size() <<
" Virtual Registers " << sDashes << endl << endl;
629 oss <<
"## NOTE: Driver failed to return one or more virtual registers (those will be zero)" << endl;
633 const uint32_t regNum (regInfo.registerNumber);
635 const uint32_t
value (regInfo.registerValue);
638 <<
"VReg Number: " << setw(10) << regNum << endl
639 <<
"VReg Value: " <<
value <<
" : " <<
xHEX0N(value,8) << endl
645 void CNTV2SupportLogger::FetchAutoCirculateLog (ostringstream & oss)
const
654 static const string dashes (25,
'-');
686 bool multiFormatMode(
false);
689 if (!multiFormatMode)
692 oss <<
"MultiFormat Mode" << endl;
699 <<
"Chan/FrameStore State Start End Act FrmProc FrmDrop BufLvl Audio RP188 LTC FBFch FBOch Color VidPr Anc HDMIx Field VidFmt PixFmt" << endl
700 <<
"-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------" << endl;
708 << setw(12) << status[0]
709 << setw( 7) << status[1]
710 << setw( 6) << status[2]
712 << setw(10) << status[9]
713 << setw(10) << status[10]
714 << setw( 7) << status[11]
715 << setw( 9) << status[12]
716 << setw( 8) << status[13]
717 << setw( 8) << status[14]
718 << setw( 8) << status[15]
719 << setw( 8) << status[16]
720 << setw( 8) << status[17]
721 << setw( 8) << status[18]
722 << setw( 8) << status[19]
723 << setw( 8) << status[20]
724 << setw( 8) << status[21];
725 if (!status.IsStopped() ||
isEnabled(mDevice,chan))
730 oss << setw(12) <<
"---"
733 if (!status.IsStopped() && status.WithAudio())
735 ULWord audChlsPerSample(0);
744 const double bytesPerChannel (4.0);
745 const double channelsPerSample (
double(audChlsPerSample+0));
746 const double bytesPerFrame (samplesPerSecond * bytesPerChannel * channelsPerSample / framesPerSecond);
747 const ULWord maxVideoFrames (4UL * 1024UL * 1024UL /
ULWord(bytesPerFrame));
748 if (status.GetFrameCount() > maxVideoFrames)
749 oss <<
"## WARNING: " <<
DEC(status.GetFrameCount()) <<
" frames (" <<
DEC(status.GetStartFrame())
750 <<
" thru " <<
DEC(status.GetEndFrame()) <<
") exceeds " <<
DEC(maxVideoFrames)
751 <<
"-frame max audio buffer capacity" << endl;
757 oss << endl <<
"Device SDRAM Map (8MB frms):" << endl;
767 if (status.IsStopped())
771 if (it == perChannelTCs.end())
775 oss << endl << dashes <<
" " << (
NTV2_IS_INPUT_CROSSPOINT(status.acCrosspoint) ?
"Input " :
"Output ") <<
DEC(chan+1) <<
" Per-Frame Valid Timecodes:" << endl;
778 const uint16_t frameNum(i->first);
780 oss <<
"Frame " << frameNum <<
":" << endl;
781 for (uint16_t tcNdx(0); tcNdx < timecodes.size(); tcNdx++)
784 if (!tcVal.IsValid())
788 << setw(12) << tcStr << setw(0) <<
"\t" << tcVal << endl;
795 void CNTV2SupportLogger::FetchAudioLog (ostringstream & oss)
const
828 bool isEmbedderEnabled =
false;
830 UWord inChannelCount = isEmbedderEnabled ? maxNumChannels : 0;
833 for (
UWord audioChannel (0); audioChannel < inChannelCount; audioChannel++)
835 if (audioChannel & 1)
846 bool isNonPCM (
true);
857 oss <<
" (max)" << endl;
859 oss <<
" (" << maxNumChannels <<
" (max))" << endl;
860 oss <<
" Total Samples:\t[" <<
DEC0N(maxSamples,6) <<
"]" << endl
866 oss <<
"Write Head Position:\t[" <<
DEC0N(currentPosSampleNdx,6) <<
"]" << endl
871 <<
" Channels Present:\t" << channelPairsPresent << endl
872 <<
" Non-PCM Channels:\t" << nonPCMChannelPairs << endl;
876 oss <<
" Read Head Position:\t[" <<
DEC0N(currentPosSampleNdx,6) <<
"]" << endl;
878 oss <<
" Non-PCM Channels:\t" << nonPCMChannelPairs << endl;
880 oss <<
" Non-PCM Channels:\t" << (isNonPCM ?
"All Channel Pairs" :
"Normal") << endl;
886 void CNTV2SupportLogger::FetchRoutingLog (ostringstream & oss)
const
891 oss <<
"(NTV2InputCrosspointID <== NTV2OutputCrosspointID)" << endl;
892 router.
Print (oss,
false);
1017 fileInput.open(inLogFilePath.c_str());
1018 string lineContents;
1019 int i = 0, numLines = 0;
1021 string searchString;
1022 bool isCompatible =
false;
1024 while(getline(fileInput, lineContents))
1029 fileInput.seekg(0, ios::beg);
1030 while(getline(fileInput, lineContents) && i < size && !bForceLoad)
1032 searchString =
"Device: ";
1034 if (lineContents.find(searchString, 0) != string::npos)
1037 isCompatible =
true;
1051 getline(fileInput, lineContents);
1056 fileInput.seekg(0, ios::beg);
1060 searchString =
"Register Name: ";
1062 if (lineContents.find(searchString, 0) != string::npos)
1064 getline(fileInput, lineContents);
1065 getline(fileInput, lineContents);
1066 searchString =
"Register Value: ";
1067 size_t start = lineContents.find(searchString);
1068 if(start != string::npos)
1070 size_t end = lineContents.find(
" : ");
1071 stringstream registerValueString(lineContents.substr(start + searchString.length(),
end));
1072 uint32_t registerValue = 0;
1073 registerValueString >> registerValue;
1079 cout <<
"The format of the log file is not compatible with this option." << endl;
1102 if (!homePath.empty())
1104 oss << inPrefix <<
"_" << deviceName <<
"_" << ::time(&rawtime) <<
"." << inExtension;
1110 if (!inDevice.IsOpen())
1112 if (inFilePath.empty())
1120 ofstream ofs(inFilePath.c_str(), ofstream::out | ofstream::binary);
1122 {msgStrm <<
"## ERROR: Unable to open '" << inFilePath <<
"' for writing" << endl;
return false;}
1124 for (
ULWord frameNdx(0); frameNdx < numFrames; frameNdx++)
1127 {badDMAs.push_back(frameNdx);
continue;}
1128 if (!ofs.write(buffer, streamsize(buffer.
GetByteCount())).good())
1129 {badWrites.push_back(frameNdx);
continue;}
1130 goodFrames.push_back(frameNdx);
1132 if (!badDMAs.empty())
1134 msgStrm <<
"## ERROR: DMARead failed for " <<
DEC(badDMAs.size()) <<
" " <<
DEC(megs) <<
"MB frame(s): ";
1137 if (!badWrites.empty())
1139 msgStrm <<
"## ERROR: Write failures for " <<
DEC(badWrites.size()) <<
" " <<
DEC(megs) <<
"MB frame(s): ";
1142 msgStrm <<
"## NOTE: " <<
DEC(goodFrames.size()) <<
" x " <<
DEC(megs) <<
"MB frames from device '"
@ value
the parser finished reading a JSON value
ULWord GetScaleFromFrameRate(const NTV2FrameRate inFrameRate)
std::ostream & NTV2PrintULWordVector(const NTV2ULWordVector &inObj, std::ostream &inOutStream=std::cout)
Streams a human-readable dump of the given NTV2ULWordVector into the specified output stream.
static bool DumpDeviceSDRAM(CNTV2Card &inDevice, const std::string &inFilePath, std::ostream &msgStream)
#define kRegSarekLicenseStatus
@ NTV2_SupportLoggerSectionRouting
#define kRegClass_Virtual
static ULWord readCurrentAudioPosition(CNTV2Card &device, NTV2AudioSystem audioSystem, NTV2Mode mode)
static string xHEX0NStr(const uint32_t inNum, const uint16_t inWidth)
virtual bool GetInputAudioChannelPairsWithPCM(const NTV2Channel inSDIInputConnector, NTV2AudioChannelPairs &outChannelPairs)
For the given SDI input (specified as a channel number), returns the set of audio channel pairs that ...
Declares the AJASystemInfo class.
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
@ kRegOutputTimingControlch6
virtual bool ReadRegisters(NTV2RegisterReads &inOutValues)
Reads the register(s) specified by the given NTV2RegInfo sequence.
static NTV2RegNumSet GetRegistersForDevice(const NTV2DeviceID inDeviceID, const int inOtherRegsToInclude=0)
Declares the CNTV2SupportLogger class.
#define NTV2_AUDIO_SOURCE_IS_EMBEDDED(_x_)
NTV2Crosspoint acCrosspoint
The crosspoint (channel number with direction)
static string appSignatureToString(const ULWord inAppSignature)
FrameToTCList::const_iterator FrameToTCListConstIter
static string makeHeader(ostringstream &oss, const string &inName)
@ kRegInvalidValidXptROMRegister
virtual bool GetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, NTV2AudioSource &outAudioSource, NTV2EmbeddedAudioInput &outEmbeddedSource)
Answers with the device's current NTV2AudioSource (and also possibly its NTV2EmbeddedAudioInput) for ...
Declares device capability functions.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
@ kDeviceHasXptConnectROM
True if device has a crosspoint connection ROM (New in SDK 17.0)
std::set< NTV2AudioChannelPair > NTV2AudioChannelPairs
A set of distinct NTV2AudioChannelPair values.
@ kRegOutputTimingControlch7
static string timecodeToString(const NTV2_RP188 &inRP188)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
Describes a user-space buffer on the host computer. I have an address and a length,...
ULWord GetByteCount(void) const
#define SAREK_LICENSE_PRESENT
std::string NTV2AudioBufferSizeToString(const NTV2AudioBufferSize inValue, const bool inForRetailDisplay=false)
enum NTV2TCIndex NTV2TimecodeIndex
virtual bool GetAudioBufferSize(NTV2AudioBufferSize &outSize, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Retrieves the size of the input or output audio buffer being used for a given Audio System on the AJA...
static string HEX0NStr(const uint32_t inNum, const uint16_t inWidth)
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_1
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
Generates a standard support log (register log) for any NTV2 device attached to the host....
ULWord NTV2DeviceGetActiveMemorySize(const NTV2DeviceID inDeviceID)
virtual bool GetPackageInformation(PACKAGE_INFO_STRUCT &outPkgInfo)
Answers with the IP device's package information.
pair< NTV2Channel, AUTOCIRCULATE_STATUS > ChannelToACStatusPair
@ kRegOutputTimingControlch3
virtual void PrependToSection(uint32_t section, const std::string §ionData)
Prepends arbitrary string data to my support log, ahead of a given section.
virtual NTV2VideoFormat GetAnalogInputVideoFormat(void)
Returns the video format of the signal that is present on the device's analog video input.
char designNameStr[(100)]
NTV2HDMIAudioChannels
Indicates or specifies the HDMI audio channel count.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
virtual bool GetLPExternalPortURLString(std::string &outURLString)
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
virtual bool GetFrameBufferSize(const NTV2Channel inChannel, NTV2Framesize &outValue)
Answers with the frame size currently being used on the device.
#define NTV2_IS_VALID_AUDIO_BUFFER_SIZE(_x_)
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
#define DEC0N(__x__, __n__)
virtual bool GetInputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current input frame index number for the given FrameStore. This identifies which par...
static ULWord getNumAudioChannels(CNTV2Card &device, NTV2AudioSystem audioSystem)
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
virtual void AddHeader(const std::string §ionName, const std::string §ionData)
Adds header text to my log.
virtual bool GetInstalledBitfileInfo(ULWord &outNumBytes, std::string &outDateStr, std::string &outTimeStr)
Returns the bitfile size and time/date stamp from the header of the bitfile that's currently installe...
#define SAREK_LICENSE_VALID
std::string packageNumber
static ULWord getCurrentPositionSamples(CNTV2Card &device, NTV2AudioSystem audioSystem, NTV2Mode mode)
virtual bool GetMultiFormatMode(bool &outIsEnabled)
Answers if the device is operating in multiple-format per channel (independent channel) mode or not....
@ NTV2_INVALID_HDMI_AUDIO_CHANNELS
std::string AsString(void) const
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
virtual bool GetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat &outValue)
Returns the current frame buffer format for the given FrameStore on the AJA device.
Audits an NTV2 device's SDRAM utilization, and can report contiguous regions of SDRAM,...
#define NTV2_IS_VALID_AUDIO_RATE(_x_)
@ NTV2_HDMIAudio8Channels
8 audio channels
map< NTV2Channel, AUTOCIRCULATE_STATUS > ChannelToACStatus
NTV2FrameRate
Identifies a particular video frame rate.
ULWord NTV2FramesizeToByteCount(const NTV2Framesize inFrameSize)
Converts the given NTV2Framesize value into an exact byte count.
This class/object reports information about the current and/or requested AutoCirculate frame.
Defines the KonaIP/IoIP registers.
static string pidToString(const uint32_t inPID)
NTV2RegisterNumberSet NTV2RegNumSet
A set of distinct NTV2RegisterNumbers.
virtual ~CNTV2SupportLogger()
My default destructor.
static NTV2VideoFormat getVideoFormat(CNTV2Card &device, const NTV2Channel inChannel)
pair< NTV2Channel, FrameToTCList > ChannelToPerFrameTCListPair
virtual bool GetDetectedAudioChannelPairs(const NTV2AudioSystem inAudioSystem, NTV2AudioChannelPairs &outDetectedChannelPairs)
Answers which audio channel pairs are present in the given Audio System's input stream.
virtual bool IsSupported(const NTV2BoolParamID inParamID)
@ kRegOutputTimingControlch5
static bool isEnabled(CNTV2Card &device, const NTV2Channel inChannel)
@ NTV2_AudioChannel9_10
This selects audio channels 9 and 10 (Group 3 channels 1 and 2)
virtual std::string GetDisplayName(void)
Answers with this device's display name.
std::ostream & DumpBlocks(std::ostream &oss) const
Dumps all 8MB blocks/frames and their tags, if any, into the given stream.
std::string NTV2TCIndexToString(const NTV2TCIndex inValue, const bool inCompactDisplay=false)
virtual bool GetOutputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current output frame number for the given FrameStore (expressed as an NTV2Channel).
virtual bool AutoCirculateGetFrameStamp(const NTV2Channel inChannel, const ULWord inFrameNumber, FRAME_STAMP &outFrameInfo)
Returns precise timing information for the given frame and channel that's currently AutoCirculating.
ChannelToPerFrameTCList::const_iterator ChannelToPerFrameTCListConstIter
virtual bool GetNumberAudioChannels(ULWord &outNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current number of audio channels being captured or played by a given Audio System on the ...
virtual std::string GetPCIFPGAVersionString(void)
virtual bool GetVideoFormat(NTV2VideoFormat &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
@ NTV2_SupportLoggerSectionRegisters
virtual AJAStatus GetValue(const AJASystemInfoTag inTag, std::string &outValue) const
Answers with the host system info value string for the given AJASystemInfoTag.
static AJALabelValuePairs & append(AJALabelValuePairs &inOutTable, const std::string &inLabel, const std::string &inValue=std::string())
A convenience function that appends the given label and value strings to the provided AJALabelValuePa...
#define LoggerSectionToFunctionMacro(_SectionEnum_, _SectionString_, _SectionMethod_)
virtual std::string GetDescription(void) const
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
static std::string GetDisplayName(const uint32_t inRegNum)
#define NTV2_IS_INPUT_CROSSPOINT(__x__)
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
static NTV2Channel findActiveACChannel(CNTV2Card &device, NTV2AudioSystem audioSystem, AUTOCIRCULATE_STATUS &outStatus)
bool GetInputTimeCodes(NTV2TimeCodeList &outValues) const
Returns all RP188 timecodes associated with the frame in NTV2TCIndex order.
NTV2Mode
Used to identify the mode of a widget_framestore, or the direction of an AutoCirculate stream: either...
virtual bool GetInputAudioChannelPairsWithoutPCM(const NTV2Channel inSDIInputConnector, NTV2AudioChannelPairs &outChannelPairs)
For the given SDI input (specified as a channel number), returns the set of audio channel pairs that ...
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
static ULWord bytesToSamples(CNTV2Card &device, NTV2AudioSystem audioSystem, const ULWord inBytes)
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
NTV2RegisterNumber registerNum
NTV2RegWritesConstIter NTV2RegisterReadsConstIter
virtual std::string GetConnectionType(void) const
#define NTV2_IS_VALID_EMBEDDED_AUDIO_INPUT(_x_)
@ NTV2_AudioChannel3_4
This selects audio channels 3 and 4 (Group 1 channels 3 and 4)
virtual bool ReadAudioLastIn(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset to the last byte of the latest chunk of 4-by...
virtual bool GetSerialNumberString(std::string &outSerialNumberString)
Answers with a string that contains my human-readable serial number.
virtual AJAStatus GetLabelValuePairs(AJALabelValuePairs &outTable, bool clearTable=false) const
Generates a "table" of label/value pairs that contains the complete host system info table.
virtual bool ReadAudioLastOut(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset of the tail end of the last chunk of audio s...
NTV2AudioChannelPair
Identifies a pair of audio channels.
NTV2RegWrites NTV2RegisterReads
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
@ kRegOutputTimingControlch8
NTV2Framesize
Kona2/Xena2 specific enums.
virtual bool GetLPTunnelPortURLString(std::string &outURLString)
std::string NTV2TaskModeToString(const NTV2EveryFrameTaskMode inValue, const bool inCompactDisplay=false)
virtual NTV2VideoFormat GetAnalogCompositeInputVideoFormat(void)
Returns the video format of the signal that is present on the device's composite video input.
virtual bool GetHDMIInputAudioChannels(NTV2HDMIAudioChannels &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current number of audio channels being received on the given HDMI input.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
virtual bool DMAReadFrame(const ULWord inFrameNumber, ULWord *pOutFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the AJA device to the host.
I interrogate and control an AJA video/audio capture/playout device.
ostream & operator<<(ostream &outStream, const CNTV2SupportLogger &inData)
bool IsStopped(void) const
std::vector< ULWord > NTV2ULWordVector
An ordered sequence of ULWords.
NTV2AudioLoopBack
This enum value determines/states if an audio output embedder will embed silence (zeroes) or de-embed...
std::string NTV2GetVersionString(const bool inDetailed=false)
@ kRegOutputTimingControlch4
This class is a collection of widget input-to-output connections that can be applied all-at-once to a...
uint16_t GetEndFrame(void) const
@ NTV2_SupportLoggerSectionAutoCirculate
static std::string GetDeviceRefName(CNTV2Card &inDevice)
std::string NTV2AudioSourceToString(const NTV2AudioSource inValue, const bool inCompactDisplay=false)
virtual bool GetMode(const NTV2Channel inChannel, NTV2Mode &outValue)
Answers with the current NTV2Mode of the given FrameStore on the AJA device.
@ NTV2_DISABLE_TASKS
0: Disabled (never recommended): device configured exclusively by client application(s).
const registerToLoadString registerToLoadStrings[]
static uint32_t maxSampleCountForNTV2AudioBufferSize(const NTV2AudioBufferSize inBufferSize, const uint16_t inChannelCount)
virtual std::string GetDriverVersionString(void)
Answers with this device's driver's version as a human-readable string.
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
bool AssessDevice(CNTV2Card &inDevice, const bool inIgnoreStoppedAudioBuffers=(0))
Assesses the given device.
static ULWord getMaxNumSamples(CNTV2Card &device, NTV2AudioSystem audioSystem)
virtual bool GetRouting(CNTV2SignalRouter &outRouting)
Answers with the current signal routing between any and all widgets on the AJA device.
@ kRegOutputTimingControlch2
bool NTV2DeviceCanDoPCMDetection(const NTV2DeviceID inDeviceID)
@ NTV2_AUDIO_AES
Obtain audio samples from the device AES inputs, if available.
virtual NTV2VideoFormat GetHDMIInputVideoFormat(NTV2Channel inHDMIInput=NTV2_CHANNEL1)
@ NTV2_AUDIO_HDMI
Obtain audio samples from the device HDMI input, if available.
static NTV2RegNumSet GetRegistersForClass(const std::string &inClassName)
static bool detectInputChannelPairs(CNTV2Card &device, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedSource, NTV2AudioChannelPairs &outChannelPairsPresent)
string DECStr(const T inT)
static std::string InventLogFilePathAndName(CNTV2Card &inDevice, std::string inPrefix="aja_supportlog", std::string inExtension="log")
const char * NTV2DeviceIDString(const NTV2DeviceID id)
virtual bool GetAudioLoopBack(NTV2AudioLoopBack &outMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Answers if NTV2AudioLoopBack mode is currently on or off for the given NTV2AudioSystem.
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
Private include file for all ajabase sources.
virtual bool GetDetectedAESChannelPairs(NTV2AudioChannelPairs &outDetectedChannelPairs)
Answers which AES/EBU audio channel pairs are present on the device.
#define HEX0N(__x__, __n__)
Declares the CNTV2KonaFlashProgram class.
bool IsValid(void) const
Answers true if I'm valid, or false if I'm not valid.
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
virtual std::ostream & Print(std::ostream &inOutStream, const bool inForRetailDisplay=false) const
Prints me in a human-readable format to the given output stream.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
This struct replaces the old RP188_STRUCT.
@ kRegFirstValidXptROMRegister
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
pair< uint16_t, NTV2TimeCodeList > FrameToTCListPair
uint16_t GetStartFrame(void) const
@ AJA_SystemInfoTag_Path_UserHome
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
NTV2RegisterReads FromRegNumSet(const NTV2RegNumSet &inRegNumSet)
ULWord NTV2DeviceGetNumVideoChannels(const NTV2DeviceID inDeviceID)
static string getActiveFrameStr(CNTV2Card &device, const NTV2Channel inChannel)
virtual bool GetSDIOutputAudioEnabled(const NTV2Channel inSDIOutput, bool &outIsEnabled)
Answers with the current state of the audio output embedder for the given SDI output connector (speci...
ChannelToACStatus::const_iterator ChannelToACStatusConstIter
std::vector< NTV2_RP188 > NTV2TimeCodeList
An ordered sequence of zero or more NTV2_RP188 structures. An NTV2TCIndex enum value can be used as a...
virtual bool IsChannelEnabled(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the given FrameStore is enabled.
Declares the CRP188 class. See SMPTE RP188 standard for details.
std::string NTV2AudioLoopBackToString(const NTV2AudioLoopBack inValue, const bool inForRetailDisplay=false)
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
static ULWord getActiveFrame(CNTV2Card &device, const NTV2Channel inChannel)
virtual NTV2DeviceID GetDeviceID(void)
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
CNTV2SupportLogger(CNTV2Card &card, NTV2SupportLoggerSections sections=NTV2_SupportLoggerSectionsAll)
Construct from CNTV2Card instance.
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
double GetAudioSamplesPerSecond(const NTV2AudioRate inAudioRate)
Returns the audio sample rate as a number of audio samples per second.
@ NTV2_MODE_INVALID
The invalid mode.
std::string NTV2ChannelToString(const NTV2Channel inValue, const bool inForRetailDisplay=false)
std::string NTV2AudioRateToString(const NTV2AudioRate inValue, const bool inForRetailDisplay=false)
std::string NTV2EmbeddedAudioInputToString(const NTV2EmbeddedAudioInput inValue, const bool inCompactDisplay=false)
@ NTV2_AUDIO_ANALOG
Obtain audio samples from the device analog input(s), if available.
virtual bool LoadFromLog(const std::string &inLogFilePath, const bool bForceLoad)
#define NTV2_IS_INPUT_MODE(__mode__)
std::string to_string(bool val)
NTV2EmbeddedAudioInput
This enum value determines/states which SDI video input will be used to supply audio samples to an au...
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
#define xHEX0N(__x__, __n__)
@ kRegOutputTimingControl
virtual void AddFooter(const std::string §ionName, const std::string §ionData)
Adds footer text to my log.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
@ NTV2_AUDIO_RATE_INVALID
#define NTV2_IS_SUPPORTED_NTV2FrameRate(__r__)
@ NTV2_AudioChannel1_2
This selects audio channels 1 and 2 (Group 1 channels 1 and 2)
@ NTV2_SupportLoggerSectionInfo
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
std::string NTV2ModeToString(const NTV2Mode inValue, const bool inCompactDisplay=false)
map< NTV2Channel, FrameToTCList > ChannelToPerFrameTCList
NTV2AudioSource
This enum value determines/states where an audio system will obtain its audio samples.
Declares the CNTV2RegisterExpert class.
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
static NTV2Mode getMode(CNTV2Card &device, const NTV2Channel inChannel)
@ NTV2_SupportLoggerSectionAudio
map< uint16_t, NTV2TimeCodeList > FrameToTCList
#define NTV2_IS_OUTPUT_MODE(__mode__)
virtual bool DriverGetBitFileInformation(BITFILE_INFO_STRUCT &outBitFileInfo, const NTV2BitFileType inBitFileType=NTV2_VideoProcBitFile)
Answers with the currently-installed bitfile information.
bool NTV2DeviceCanDoPCMControl(const NTV2DeviceID inDeviceID)
NTV2AudioBufferSize
Represents the size of the audio buffer used by a device audio system for storing captured samples or...
static std::string GetDisplayValue(const uint32_t inRegNum, const uint32_t inRegValue, const NTV2DeviceID inDeviceID=DEVICE_ID_NOTFOUND)
static bool getBitfileDate(CNTV2Card &device, string &outDateString, NTV2XilinxFPGA whichFPGA)
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
#define NTV2_IS_VALID_VIDEO_FORMAT(__f__)
virtual void AppendToSection(uint32_t section, const std::string §ionData)
Appends arbitrary string data to my support log, after a given section.
NTV2SupportLoggerSections
NTV2AudioSystem GetAudioSystem(void) const
bool IsOutput(void) const
static NTV2PixelFormat getPixelFormat(CNTV2Card &device, const NTV2Channel inChannel)
std::string NTV2AudioSystemToString(const NTV2AudioSystem inValue, const bool inCompactDisplay=false)
bool NTV2DeviceHasLPProductCode(const NTV2DeviceID inDeviceID)
virtual std::string ToString(void) const