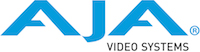 |
AJA NTV2 SDK
17.6.0.1688
NTV2 SDK 17.6.0.1688
|
Go to the documentation of this file.
51 while (mConsumerThread.
Active())
54 while (mProducerThread.
Active())
81 if (!mDevice.
features().CanDoCapture())
83 if (!mDevice.
features().CanDo12gRouting())
87 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support '"
101 cerr <<
"## ERROR: Unable to acquire '" << mDevice.
GetDisplayName() <<
"' because another app (pid " << appPID <<
") owns it" << endl;
108 if (mDevice.
features().CanDoMultiFormat())
149 if (mDevice.IsRemote())
150 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl << endl;
151 #endif // defined(_DEBUG)
178 {cerr <<
"## ERROR: No input signal or unknown format" << endl;
return AJA_STATUS_NOINPUT;}
208 if (mDevice.
features().GetNumAudioSystems() > 1)
224 ULWord F1AncSize(0), F2AncSize(0);
227 ULWord F1OffsetFromEnd(0), F2OffsetFromEnd(0);
231 F1AncSize = F2OffsetFromEnd > F1OffsetFromEnd ? 0 : F1OffsetFromEnd - F2OffsetFromEnd;
232 F2AncSize = F2OffsetFromEnd > F1OffsetFromEnd ? F2OffsetFromEnd - F1OffsetFromEnd : F2OffsetFromEnd;
238 cout <<
"## NOTE: Buffer size: vid=" << mFormatDesc.
GetVideoWriteSize() <<
" aud=" << audioBufferSize <<
" anc=" << F1AncSize << endl;
247 mAVCircularBuffer.
Add(&frameData);
288 mConsumerThread.
Start();
324 CAPNOTE(
"Thread completed, will exit");
337 mProducerThread.
Start();
420 CAPNOTE(
"Thread completed, will exit");
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
bool fDoMultiFormat
If true, use multi-format/multi-channel mode, if device supports it; otherwise normal mode.
std::string NTV2ChannelSetToStr(const NTV2ChannelSet &inObj, const bool inCompact=true)
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
virtual bool RouteInputSignal(void)
Sets up device routing for capture.
ULWord GetCapturedAudioByteCount(void) const
virtual void GetACStatus(ULWord &outGoodFrames, ULWord &outDroppedFrames, ULWord &outBufferLevel)
Provides status information about my input (capture) process.
@ AJA_ThreadPriority_High
UWord firstFrame(void) const
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
Declares device capability functions.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
#define NTV2_IS_QUAD_QUAD_HFR_VIDEO_FORMAT(__f__)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
NTV2Buffer & AncBuffer2(void)
virtual void Quit(void)
Gracefully stops me from running.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
ULWord GetProcessedFrameCount(void) const
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
NTV2PixelFormat fPixelFormat
Pixel format to use.
NTV2Capture8K(const CaptureConfig &inConfig)
Constructs me using the given settings.
ULWord GetBufferLevel(void) const
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
NTV2Buffer fAncBuffer2
Additional "F2" host anc buffer.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=(0))
Applies the given routing table to the AJA device.
bool SetAncBuffers(ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my ancillary data buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2Buffer fAncBuffer
Host ancillary data buffer.
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
bool WithAudio(void) const
bool fWithAnc
If true, also capture Anc.
NTV2Buffer & VideoBuffer(void)
@ kVRegAncField2Offset
Anc Field2 byte offset from end of frame buffer (GUMP on all boards except RTP for SMPTE2022/IP)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=(0), bool inRDMA=(0))
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
NTV2AudioSystemSet NTV2MakeAudioSystemSet(const NTV2AudioSystem inFirstAudioSystem, const UWord inCount=1)
virtual class DeviceCapabilities & features(void)
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
Declares the NTV2Capture class.
virtual bool DMABufferUnlockAll()
Unlocks all previously-locked buffers used for DMA transfers.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
Declares the AJAProcess class.
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread runs...
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
static bool ConfigureAudioSystems(CNTV2Card &inDevice, const CaptureConfig &inConfig, const NTV2AudioSystemSet inAudioSystems)
Configures capture audio systems.
virtual bool EnableChannels(const NTV2ChannelSet &inChannels, const bool inDisableOthers=(0))
Enables the given FrameStore(s).
virtual std::string GetDescription(void) const
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
This class is used to configure an NTV2Capture instance.
ULWord VideoBufferSize(void) const
@ kVRegAncField1Offset
Anc Field1 byte offset from end of frame buffer (GUMP on all boards except RTP for SMPTE2022/IP)
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
std::set< NTV2AudioSystem > NTV2AudioSystemSet
A set of distinct NTV2AudioSystem values. New in SDK 16.2.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
ULWord AncBufferSize(void) const
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the capture thread's static callback function that gets called when the capture thread runs....
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord AudioBufferSize(void) const
ULWord AncBuffer2Size(void) const
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
std::string fDeviceSpec
The AJA device to use.
Declares numerous NTV2 utility functions.
NTV2Buffer & AudioBuffer(void)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
virtual AJAStatus Run(void)
Runs me.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
virtual bool IsDeviceReady(const bool inCheckValid=(0))
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
#define NTV2_AUDIOSIZE_MAX
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
virtual void CaptureFrames(void)
Repeatedly captures frames using AutoCirculate (until global quit flag set).
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
@ NTV2_DISABLE_TASKS
0: Disabled (never recommended): device configured exclusively by client application(s).
NTV2Channel fInputChannel
The device channel to use.
NTV2Buffer fAudioBuffer
Host audio buffer.
static bool GetInputRouting8K(NTV2XptConnections &outConnections, const CaptureConfig &inConfig, const NTV2VideoFormat inVidFormat, const NTV2DeviceID inDevID=DEVICE_ID_INVALID, const bool isInputRGB=(0))
Answers with the crosspoint connections needed to implement the given 8K/UHD2 capture configuration.
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
UWord lastFrame(void) const
bool IsRunning(void) const
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
bool fDoTSIRouting
If true, do TSI routing; otherwise squares.
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
NTV2ChannelSet NTV2MakeChannelSet(const NTV2Channel inFirstChannel, const UWord inNumChannels=1)
bool IsRGBFormat(const NTV2FrameBufferFormat format)
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
ULWord GetDroppedFrameCount(void) const
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
bool setExactRange(const UWord inFirstFrame, const UWord inLastFrame)
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual void StartConsumerThread(void)
Starts my frame consumer thread.
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
bool WithCustomAnc(void) const
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
virtual void StartProducerThread(void)
Starts my capture thread.
virtual bool SetQuadQuadFrameEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables "quad-quad" 8K frame mode on the device.
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
virtual bool SetQuadQuadSquaresEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables quad-quad-frame (8K) squares mode on the device.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
bool GetInputTimeCodes(NTV2TimeCodeList &outValues) const
Intended for capture, answers with the timecodes captured in my acTransferStatus member's acFrameStam...
virtual AJAStatus Start()
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
#define AJA_FAILURE(_status_)
#define NTV2_IS_VALID_AUDIO_SYSTEM(__x__)
static bool Get8KInputFormat(NTV2VideoFormat &inOutVideoFormat)
Given a video format, if all 4 inputs are the same and promotable to 8K, this function does the promo...
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
NTV2Buffer & AncBuffer(void)
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
NTV2InputSource fInputSource
The device input connector to use.
@ NTV2_OEM_TASKS
2: OEM (recommended): device configured by client application(s) with some driver involvement.
virtual void ConsumeFrames(void)
Repeatedly consumes frames from the circular buffer (until global quit flag set).
NTV2TimeCodes fTimecodes
Map of TC indexes to NTV2_RP188 values.
bool fWithAudio
If true, also capture Audio.
@ NTV2_AUDIOSYSTEM_INVALID