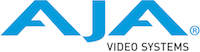 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
26 using namespace std::rel_ops;
28 #define AsConstUBytePtr(__p__) reinterpret_cast<const UByte*>(__p__)
29 #define AsConstULWordPtr(__p__) reinterpret_cast<const ULWord*>(__p__)
30 #define AsULWordPtr(__p__) reinterpret_cast<ULWord*>(__p__)
31 #define AsUBytePtr(__p__) reinterpret_cast<UByte*>(__p__)
42 : mConfig (inConfigData),
53 mCaptionDataTally (0),
54 m608Channel (mConfig.fCaptionChannel),
66 mHeadUpDisplayOn (
true),
75 NTV2_ASSERT (m608Decoder && m708DecoderAnc && m708DecoderVanc);
107 while (mCaptureThread.
Active())
110 while (mPlayoutThread.
Active())
135 if (mDevice.
features().GetNumFrameStores() < 2)
137 cerr <<
"## ERROR: Device '" << deviceStr <<
"' can't burn-in captions because at least 2 frame stores are required" << endl;
140 if (!mDevice.
features().CanDoPlayback())
142 cerr <<
"## ERROR: Device '" << deviceStr <<
"' can't burn-in captions because device can only capture/ingest" << endl;
145 if (mDevice.
features().GetNumVideoInputs() < 1 || mDevice.
features().GetNumVideoOutputs() < 1)
147 cerr <<
"## ERROR: Device '" << deviceStr <<
"' can't be used -- at least 1 SDI input and 1 SDI output required" << endl;
150 if (!mDevice.
features().GetNumMixers())
152 cerr <<
"## ERROR: Device '" << deviceStr <<
"' can't burn-in captions because a mixer/keyer widget is required" << endl;
157 cerr <<
"## ERROR: Device '" << deviceStr <<
"' can't burn-in captions because it can't do 8-bit RGB (with alpha)" << endl;
165 {cerr <<
"## ERROR: Cannot acquire -- device busy" << endl;
return AJA_STATUS_BUSY;}
171 if (mDevice.
features().CanDoMultiFormat())
176 cerr <<
"## WARNING: Enabling VANC because no Anc extractors" << endl;
191 {cerr <<
"## WARNING: SubscribeChangeNotification failed" << endl;
return AJA_STATUS_FAIL;}
193 cerr << mConfig << endl;
194 #endif // defined(_DEBUG)
222 if (mDevice.
features().CanDoCustomAnc())
229 #if defined(NTV2_BUFFER_LOCK)
232 mCircularBuffer.
Add(&frameData);
243 #if defined(NTV2_BUFFER_LOCK)
244 for (
size_t ndx(0); ndx < mHostBuffers.size(); ndx++)
249 #endif // NTV2_BUFFER_LOCK
250 mHostBuffers.clear();
251 mCircularBuffer.
Clear();
259 bool isTransmit (
false);
260 const UWord numFrameStores (mDevice.
features().GetNumFrameStores());
268 {cerr <<
"## ERROR: Must specify at least input source or input channel" << endl;
return AJA_STATUS_FAIL;}
277 {cerr <<
"## ERROR: '" << deviceName <<
"' cannot grab captions from '" << inputName <<
"'" << endl;
return AJA_STATUS_FAIL;}
279 {cerr <<
"## ERROR: Input '" << inputName <<
"' not SDI" << endl;
return AJA_STATUS_FAIL;}
283 {cerr <<
"## ERROR: " << deviceName <<
" has no " << channelName <<
" input" << endl;
return AJA_STATUS_FAIL;}
286 mActiveSDIInputs.insert(sdiInput);
287 if (mDevice.
features().HasBiDirectionalSDI())
328 cerr <<
"## NOTE: Using " << deviceName <<
" " << (mConfig.
fUseVanc ?
"VANC" :
"AncExt") <<
" with spigot=" << inputName
342 const UWord numAudioSystems (mDevice.
features().GetNumAudioSystems());
357 UWord sdiOutput(mOutputChannel);
358 if (sdiOutput >= mDevice.
features().GetNumVideoOutputs())
359 sdiOutput = mDevice.
features().GetNumVideoOutputs() - 1;
363 for (
unsigned sdiOutput(0); sdiOutput < mDevice.
features().GetNumVideoOutputs(); sdiOutput++)
364 if (!mDevice.
features().HasBiDirectionalSDI()
396 static const bool ShowRoutingProgress(
false);
398 mInputConnections.clear();
402 if (isRGBFBF && isRGBWire)
406 for (
size_t ndx(0); ndx < sdiInputs.size(); ndx++)
407 {
NTV2Channel frmSt(frameStores.at(ndx)), sdiIn(sdiInputs.at(ndx));
422 UWord(frameStores.size()*2));
424 for (
size_t ndx(0); ndx < sdiInputs.size(); ndx++)
425 {
NTV2Channel frmSt(frameStores.at(ndx/2)), tsiMux(tsiMuxes.at(ndx/2)), sdiIn(sdiInputs.at(ndx));
440 else if (isRGBFBF && !isRGBWire)
443 for (
size_t ndx(0); ndx < sdiInputs.size(); ndx++)
444 {
NTV2Channel frmSt(frameStores.at(ndx)), sdiIn(sdiInputs.at(ndx));
455 UWord(2*sdiInputs.size()));
457 UWord(frameStores.size()));
462 for (
size_t ndx(0); ndx < cscs.size(); ndx++)
463 {
NTV2Channel frmSt(frameStores.at(ndx/2)), sdiIn(sdiInputs.at(ndx/2)), tsiMux(tsiMuxes.at(ndx/2)),
475 else if (!isRGBFBF && isRGBWire)
479 for (
size_t ndx(0); ndx < sdiInputs.size(); ndx++)
480 {
NTV2Channel frmSt(frameStores.at(ndx)), csc(activeCSCs.at(ndx)), sdi(sdiInputs.at(ndx));
501 for (
size_t ndx(0); ndx < sdiInputs.size(); ndx++)
502 {
NTV2Channel frmSt(frameStores.at(ndx)), sdiIn(sdiInputs.at(ndx));
513 UWord(frameStores.size()));
514 cerr << (is4KHFR ?
"4KHFR" :
"") << endl <<
"FrameStores: " << ::
NTV2ChannelListToStr(frameStores) << endl
516 for (
size_t ndx(0); ndx < sdiInputs.size(); ndx++)
517 {
NTV2Channel frmSt(frameStores.at(is4KHFR ? ndx/2 : ndx)),
518 tsiMux(tsiMuxes.at(is4KHFR ? ndx/2 : ndx)),
519 sdiIn(sdiInputs.at(ndx));
542 if (mDevice.
features().HasBiDirectionalSDI())
546 set_difference (allSDIs.begin(), allSDIs.end(),
547 mActiveSDIInputs.begin(), mActiveSDIInputs.end(),
548 inserter(sdiOuts, sdiOuts.begin()));
555 for (
size_t sdiNdx(0); sdiNdx < sdiOutputs.size(); sdiNdx++)
557 const NTV2Channel sdiIn(sdiInputs.at(sdiNdx < sdiInputs.size() ? sdiNdx : sdiInputs.size()-1));
558 if (mDevice.
features().HasBiDirectionalSDI() && mActiveSDIInputs.find(sdiOut) == mActiveSDIInputs.end())
560 cerr <<
"Switching SDI " << (sdiOut+1) <<
" to output" << endl;
597 if (chan != sdiInputAsChan)
629 result = mCaptureThread.
Start();
650 ULWord xferTally(0), xferFails(0), noVideoTally(0), waitTally(0);
680 if (mDevice.
features().CanDo12gRouting())
688 CAPWARN(
"VANC mode incompatible with 4K/UHD format");
775 ULWord vpidDS1(0), vpidDS2(0);
780 const bool vfChanged(newVF != currentVF);
781 const bool vpidChgd(mVPIDInfoDS1.GetVPID() != vpidDS1 || mVPIDInfoDS2.GetVPID() != vpidDS2);
782 if (vfChanged || vpidChgd)
789 CAPWARN(
"Input VPID changed: DS1 (" <<
xHEX0N(mVPIDInfoDS1.GetVPID(),8) <<
" to " <<
xHEX0N(vpidDS1,8)
790 <<
"), DS2 (" <<
xHEX0N(mVPIDInfoDS2.GetVPID(),8) <<
" to " <<
xHEX0N(vpidDS2,8) <<
")");
802 CAPWARN(
"FrameStore pixel format changed from '"
819 CAPNOTE(
"Thread completed, " <<
DEC(xferTally) <<
" of " <<
DEC(xferTally+xferFails) <<
" frms xferred, "
820 <<
DEC(waitTally) <<
" waits, " <<
DEC(noVideoTally) <<
" sig chgs");
827 ULWord numConsecutiveFrames(0), MIN_NUM_CONSECUTIVE_FRAMES(6);
829 NTV2Channel sdiConnector(*(mActiveSDIInputs.begin()));
847 if (++loopCount % 500 == 0)
848 CAPDBG(
"Waiting for valid video signal to appear at "
851 else if (numConsecutiveFrames == 0)
854 numConsecutiveFrames++;
856 else if (numConsecutiveFrames == MIN_NUM_CONSECUTIVE_FRAMES)
858 numConsecutiveFrames = 0;
862 numConsecutiveFrames = (lastVF == currVF) ? numConsecutiveFrames + 1 : 0;
869 {
ULWord vpidDS1(0), vpidDS2(0);
874 if (mVPIDInfoDS1.IsValid())
878 if (mVPIDInfoDS2.IsValid())
880 if (vfVPID != result && !mSquares)
887 ostringstream osserr;
891 osserr << mDevice.
GetModelName() <<
" can't handle TSI";
892 if (!osserr.str().empty())
910 mInputFrameStores.clear(); mInputFrameStores.insert(mConfig.
fInputChannel);
911 mActiveSDIInputs.clear(); mActiveSDIInputs.insert(sdiConnector);
918 mActiveCSCs = mActiveSDIInputs;
936 cerr << endl <<
"## NOTE: VANC frame geometry " << (mConfig.
fUseVanc ?
"enabled" :
"disabled") << endl;
979 CaptionData captionData708Anc, captionData708Vanc, captionData608Anc, captionData608Vanc, captionDataL21Anc, captionDataL21;
992 if (mDevice.
features().CanDoCustomAnc())
1006 CAPDBG(
DEC(diffs.size()) <<
" VANC/AncExt diff(s): " << lines.at(0));
1007 for (
size_t n(1); n < lines.size(); n++)
1016 ULWord line21RowOffset(0);
1028 line21RowOffset+1));
1069 bool hasParityErrors (
false);
1074 vector<UByte> svcBlk;
1075 size_t blkSize(0), byteCount(0);
1077 bool isExtendedSvc(
false);
1078 if (!hasParityErrors)
1088 bool hasParityErrors (
false);
1093 vector<UByte> svcBlk;
1094 size_t blkSize(0), byteCount(0);
1096 bool isExtendedSvc(
false);
1097 if (!hasParityErrors)
1118 ostringstream ossCompare;
1124 if (captionDataL21 != captionDataL21Anc)
1125 ossCompare <<
"L21Vanc != L21Anlg: " << captionDataL21 <<
" " << captionDataL21Anc << endl;
1127 pL21CaptionData = &captionDataL21;
1128 else if (captionDataL21Anc.
HasData())
1129 pL21CaptionData = &captionDataL21Anc;
1130 if (captionData608Anc.
HasData() && pL21CaptionData)
1131 if (captionData608Anc != *pL21CaptionData)
1132 ossCompare <<
"608 != L21: " << captionData608Anc <<
" " << *pL21CaptionData << endl;
1133 if (captionData608Anc.
HasData())
1134 p608CaptionData = &captionData608Anc;
1135 else if (pL21CaptionData)
1136 p608CaptionData = pL21CaptionData;
1141 if (captionData608Anc != captionData708Anc)
1142 ossCompare <<
"608anc != 708anc: " << captionData608Anc <<
" " << captionData708Anc << endl;
1143 if (captionData608Vanc.
HasData() && captionData708Vanc.
HasData())
1144 if (captionData608Vanc != captionData708Vanc)
1145 ossCompare <<
"608vanc != 708vanc: " << captionData608Vanc <<
" " << captionData708Vanc << endl;
1146 if (captionData708Anc.
HasData())
1147 p608CaptionData = &captionData708Anc;
1148 else if (captionData708Vanc.
HasData())
1149 p608CaptionData = &captionData708Vanc;
1150 else if (captionData608Anc.
HasData())
1151 p608CaptionData = &captionData608Anc;
1152 else if (captionData608Vanc.
HasData())
1153 p608CaptionData = &captionData608Vanc;
1155 if (!ossCompare.str().empty())
1156 CAPDBG(
"CaptionData mis-compare(s): " << ossCompare.str());
1169 if (!p608CaptionData)
1177 DoCCOutput(inFrameNum, *p608CaptionData, inVideoFormat);
1185 const bool isFirstTime (mLastOutFrame == 0 && inFrameNum > mLastOutFrame);
1186 char char1(0), char2(0);
1197 if (char1 >=
' ' && char1 <=
'~')
1199 if (mLastOutStr !=
" " || char1 !=
' ')
1200 {cout << char1 << flush; mLastOutStr = string(1,char1);}
1201 if (char2 >=
' ' && char2 <=
'~')
1203 if (mLastOutStr !=
" " || char2 !=
' ')
1204 {cout << char2 << flush; mLastOutStr = string(1,char2);}
1207 else if (mLastOutStr !=
" ")
1208 {cout <<
" " << flush; mLastOutStr =
" ";}
1213 if (inFrameNum == (mLastOutFrame+1))
1215 mLastOutFrame = inFrameNum;
1220 if (currentScreen != mLastOutStr)
1221 cout << currentScreen << endl;
1222 mLastOutStr = currentScreen;
1223 mFirstOutFrame = mLastOutFrame = inFrameNum;
1230 oss <<
Hex0N(u16,4);
1231 mLastOutStr.append(mLastOutStr.empty() ?
"\t" :
" ");
1232 mLastOutStr.append(oss.str());
1233 if (inFrameNum == (mLastOutFrame+1))
1235 mLastOutFrame = inFrameNum;
1240 cout <<
"Scenarist_SCC V1.0" << endl;
1246 cout << endl << tcStr <<
":" << mLastOutStr << endl;
1247 mFirstOutFrame = mLastOutFrame = inFrameNum;
1248 mLastOutStr.clear();
1252 if (inFrameNum % 60 == 0)
1257 const vector<uint32_t> stats (chDecoder->GetStats());
1260 for (
size_t num(0); num < stats.size(); num++)
1267 ostringstream oss; oss <<
DEC(stats[num]);
1293 mDevice.
SetVideoFormat (inVideoFormat,
false,
false, mOutputChannel);
1302 cerr <<
"## NOTE: Caption burn-in using mixer/keyer " << (mixerNumber+1) <<
" on " << ::
NTV2ChannelToString(mOutputChannel)
1320 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
1321 UWord connectFailures (0);
1326 if (mDevice.
features().HasBiDirectionalSDI())
1337 const ULWord numVideoOutputs (mDevice.
features().GetNumVideoOutputs());
1344 if (
ULWord(chan) >= numVideoOutputs)
1346 if (mDevice.
features().HasBiDirectionalSDI())
1348 if (chan == sdiInputAsChan)
1381 return connectFailures == 0;
1395 result = mPlayoutThread.
Start();
1418 const string indicators [] = {
"/",
"-",
"\\",
"|",
""};
1430 const NTV2FormatDesc formatDesc (videoFormat, mPlayoutFBF, vancMode);
1434 UWord consecSyncErrs (0);
1436 static uint64_t frameTally (0);
1438 ULWord lastErrorTally (0);
1442 if (!hostBuffer.
Allocate(bufferSizeBytes,
true))
1443 {cerr <<
"## NOTE: Caption burn-in failed -- unable to allocate " << bufferSizeBytes <<
"-byte caption video buffer" << endl;
return;}
1451 pingPong = pingPong ? 0 : 1;
1454 while (!mGlobalQuit)
1464 if (mHeadUpDisplayOn)
1470 oss << indicators [mCaptionDataTally % 4] <<
" " << strCaptionChan <<
" " << frameTally++ <<
" "
1473 const ULWord newErrorTally (mErrorTally);
1476 lastErrorTally = newErrorTally;
1492 pingPong = pingPong ? 0 : 1;
1501 cerr <<
"## NOTE: Caption burn-in stopped due to video format change -- was " << strVideoFormat
1503 m608Decoder->
Reset();
1504 m708DecoderAnc->
Reset();
1505 m708DecoderVanc->
Reset();
1510 bool mixerSyncOK (
false);
1514 else if (++consecSyncErrs > 60)
1515 {cerr <<
"#MXSYNC#"; consecSyncErrs = 0;}
1521 CAPNOTE(
"Thread completed, will exit");
1528 if (m608Channel != inNewChannel)
1530 m608Channel = inNewChannel;
1535 if (m708DecoderVanc)
1537 cerr << endl <<
"## NOTE: Caption display channel changed to '" <<
::NTV2Line21ChannelToStr(m608Channel) <<
"'" << endl;
1543 { (
void) inChangeInfo;
1544 mCaptionDataTally++;
1563 result += inDelimiterStr;
1600 static const string gModeStrs[] = {
"stream",
"screen",
"scc",
"mcc",
"stats",
""};
1602 return gModeStrs[inMode];
1604 for (
unsigned ndx(0); ndx < 5; )
1606 result += gModeStrs[ndx];
1607 if (!(gModeStrs[++ndx].empty()))
1615 typedef pair<string,OutputMode> StringToOutputModePair;
1616 typedef map<string,OutputMode> StringToOutputModeMap;
1617 typedef StringToOutputModeMap::const_iterator StringToOutputModeConstIter;
1618 static StringToOutputModeMap sStringToOutputModeMap;
1619 static AJALock sStringToOutputModeMapLock;
1620 if (sStringToOutputModeMap.empty())
1622 AJAAutoLock autoLock(&sStringToOutputModeMapLock);
1627 sStringToOutputModeMap.insert(StringToOutputModePair(outputModeStr,om));
1630 string modeStr(inModeStr);
1632 StringToOutputModeConstIter iter(sStringToOutputModeMap.find(modeStr));
1651 static const string gSrcStrs[] = {
"default",
"line21",
"608vanc",
"708vanc",
"608anc",
"708anc",
""};
1653 return gSrcStrs[inDataSrc];
1655 for (
unsigned ndx(0); ndx < 6; )
1657 result += gSrcStrs[ndx];
1658 if (!gSrcStrs[++ndx].empty())
1666 typedef pair<string,CaptionDataSrc> StringToCaptionDataSrcPair;
1667 typedef map<string,CaptionDataSrc> StringToCaptionDataSrcMap;
1668 typedef StringToCaptionDataSrcMap::const_iterator StringToCaptionDataSrcConstIter;
1669 static StringToCaptionDataSrcMap sStringToCaptionDataSrcMap;
1670 static AJALock sStringToCaptionDataSrcMapLock;
1671 if (sStringToCaptionDataSrcMap.empty())
1673 AJAAutoLock autoLock(&sStringToCaptionDataSrcMapLock);
1678 sStringToCaptionDataSrcMap.insert(StringToCaptionDataSrcPair(captionDataSrcStr,cds));
1681 string cdsStr(inDataSrcStr);
1683 StringToCaptionDataSrcConstIter iter(sStringToCaptionDataSrcMap.find(cdsStr));
NTV2FrameBufferFormat acFrameBufferFormat
Specifies the frame buffer format to change to. Ignored if AUTOCIRCULATE_WITH_FBFCHANGE option is not...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
bool fDoMultiFormat
If true, use multi-format/multi-channel mode, if device supports it; otherwise normal mode.
#define AsULWordPtr(__p__)
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
std::string NTV2ChannelSetToStr(const NTV2ChannelSet &inObj, const bool inCompact=true)
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2TCIndex fTimecodeSrc
Timecode source to use (if any)
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
static OutputMode StringToOutputMode(const std::string &inModeStr)
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
virtual AJAStatus SetupAudio(void)
Sets up audio for capture (and playout, if burning captions).
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
#define NTV2_IS_SD_VIDEO_FORMAT(__f__)
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
#define NTV2_IS_VANCMODE_TALLER(__v__)
@ NTV2_FBF_ARGB
See 8-Bit ARGB, RGBA, ABGR Formats.
#define AsUBytePtr(__p__)
NTV2OutputXptID GetSDIInputOutputXptFromChannel(const NTV2Channel inSDIInput, const bool inIsDS2=false)
const AUTOCIRCULATE_TRANSFER_STATUS & GetTransferStatus(void) const
Returns a constant reference to my AUTOCIRCULATE_TRANSFER_STATUS.
void QueryString(std::string &str, const AJATimeBase &timeBase, bool bDropFrame)
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
NTV2InputXptID GetMixerFGInputXpt(const NTV2Channel inChannel, const bool inIsKey=false)
std::string NTV2ChannelListToStr(const NTV2ChannelList &inObj, const bool inCompact=true)
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
@ NTV2MIXERINPUTCONTROL_UNSHAPED
@ AJAAncDataType_Cea608_Vanc
CEA608 SD Closed Captioning (SMPTE 334 VANC packet)
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
UWord firstFrame(void) const
static bool Create(CNTV2CaptionDecoder708Ptr &outDecoder)
Creates a new CNTV2CaptionEncoder708 instance.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
ULWord GetProcessedFrameCount(void) const
Declares the AJAAncillaryData_Cea608_line21 class.
virtual bool Set4kSquaresEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 "2K quadrants" mode for the given FrameStore bank on the device....
NTV2InputXptID GetDLInInputXptFromChannel(const NTV2Channel inChannel, const bool inLinkB=false)
Declares a number of pixel format transcoder functions.
OutputMode fOutputMode
Desired output (captionStream, Screen etc)
Header file for NTV2CCGrabber demonstration class.
UByte f2_char1
Caption Byte 1 of Field 2.
Declares the CNTV2Line21Captioner class.
virtual bool GetVPIDValidA(const NTV2Channel inChannel)
virtual AJAStatus GetCEA608Bytes(uint8_t &outByte1, uint8_t &outByte2, bool &outIsValid) const
Answers with the CEA608 payload bytes.
NTV2Line21Channel fCaptionChannel
Caption channel to monitor (defaults to CC1)
#define NTV2_IS_FBF_PLANAR(__s__)
std::string & strip(std::string &str, const std::string &ws)
@ kCaptionDataSrc_608FBVanc
Declares common types used in the ajabase library.
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
virtual void SetOutputStandards(const NTV2VideoFormat inVideoFormat)
Sets the device output standard based on the given video format.
bool Is8BitFrameBufferFormat(const NTV2FrameBufferFormat fbFormat)
virtual bool GetMixerSyncStatus(const UWord inWhichMixer, bool &outIsSyncOK)
Returns the current sync state of the given mixer/keyer.
static NTV2ChannelList GetTSIMuxesForFrameStore(CNTV2Card &inDevice, const NTV2Channel in1stFrameStore, const UWord inCount)
virtual void ExtractClosedCaptionData(const uint32_t inFrameCount, const NTV2VideoFormat inVideoFormat)
Extracts closed-caption data, if present, and emits it on a character-by-character basis to the stand...
static AJAStatus SetFromDeviceAncBuffers(const NTV2Buffer &inF1AncBuffer, const NTV2Buffer &inF2AncBuffer, AJAAncillaryList &outPackets, const uint32_t inFrameNum=0)
Returns all ancillary data packets found in the given F1 and F2 ancillary data buffers.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
#define IsField2Line21CaptionChannel(_chan_)
LWord acStartFrame
First frame to circulate. FIXFIXFIX Why is this signed? CHANGE TO ULWord??
I am a reference-counted pointer template class. I am intended to be a proxy for an underlying object...
void split(const std::string &str, const char delim, std::vector< std::string > &elems)
static const uint32_t MAX_ACCUM_FRAME_COUNT(30)
#define NTV2_ASSERT(_expr_)
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual CNTV2VPID & MakeInvalid(void)
This class handles "analog" (Line 21) based CEA-608 caption data packets.
virtual bool SetMixerBGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the background input control value for the given mixer/keyer.
virtual bool ReadSDIInVPID(const NTV2Channel inSDIInput, ULWord &outValueA, ULWord &outValueB)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
NTV2Buffer & AncBuffer2(void)
virtual bool IsCaptureThreadRunning(void) const
Returns true if my capture thread is currently running.
static const NTV2WidgetID g3GSDIOutputs[]
virtual bool SetDisplayChannel(const NTV2Line21Channel inChan)
Setsthe caption channel I'm currently focused on (i.e. that I'm currently "burning" into video).
virtual void SwitchPixelFormat(void)
Switches/rotates –pixelFormat.
Declares the AJAAncillaryList class.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
NTV2ChannelList NTV2MakeChannelList(const NTV2Channel inFirstChannel, const UWord inNumChannels=1)
AJALabelValuePairs Get(const bool inCompact=(0)) const
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
NTV2PixelFormat fPixelFormat
Pixel format to use.
#define NTV2_IS_HFR_STANDARD(__s__)
static bool BurnString(const std::string &inString, const NTV2Line21Attrs &inAttribs, NTV2Buffer &inFB, const NTV2FormatDesc &inFBDescriptor, const UWord inRowNum=15, const UWord inColumnNum=1)
Blits the contents of the given UTF-8 encoded string into the given host buffer using the given displ...
NTV2EmbeddedAudioInput NTV2InputSourceToEmbeddedAudioInput(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2EmbeddedAudioInput value.
virtual uint32_t CountAncillaryDataWithType(const AJAAncDataType inMatchType) const
Answers with the number of AJAAncillaryData objects having the given type.
NTV2InputXptID GetFrameBufferInputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsBInput=false)
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual void PlayFrames(void)
Repeatedly updates captions (until global quit flag set).
std::pair< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnection
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
virtual AJAStatus ParseAllAncillaryData(void)
Sends a "ParsePayloadData" command to all of my AJAAncillaryData objects.
virtual void CaptioningChanged(const NTV2Caption608ChangeInfo &inChangeInfo)
This function gets called whenever caption changes occur.
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
virtual bool SetOutputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the output frame index number for the given FrameStore. This identifies which frame in device SD...
NTV2Buffer fAncBuffer2
Additional "F2" host anc buffer.
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
virtual AJAStatus ParsePayloadData(void)
Parses out (interprets) the "local" ancillary data from my payload data.
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
@ kCaptionDataSrc_INVALID
virtual bool GetVANCMode(NTV2VANCMode &outVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Retrieves the current VANC mode for the given FrameStore.
virtual void Reset(void)
Flushes me, clearing all in-progress data.
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=(0))
Applies the given routing table to the AJA device.
virtual bool BurnCaptions(NTV2Buffer &inFB, const NTV2FormatDesc &inFD)
Blits all of my current caption channel's "on-air" captions into the given host buffer with the corre...
NTV2FrameBufferFormat NTV2PixelFormat
An alias for NTV2FrameBufferFormat.
AJALabelValuePairs Get(const bool inCompact=(0)) const
NTV2Buffer fAncBuffer
Host ancillary data buffer.
virtual CaptionData GetCC608CaptionData(void)
Pops the next CC608 data.
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
UByte f1_char2
Caption Byte 2 of Field 1.
virtual bool GetSDITransmitEnable(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the specified SDI connector is currently acting as a transmitter (i....
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
virtual AJAAncillaryData * GetAncillaryDataWithType(const AJAAncDataType inMatchType, const uint32_t inIndex=0) const
Answers with the AJAAncillaryData object having the given type and index.
virtual AJAStatus Run(void)
Runs me.
I am an ordered collection of AJAAncillaryData instances which represent one or more SMPTE 291 data p...
CaptionDataSrc fCaptionSrc
Caption data source (Line21? 608 VANC? 608 Anc? etc)
virtual AJAStatus StartCaptureThread(void)
Starts my capture thread.
enum _CaptionDataSrc CaptionDataSrc
virtual void Quit(void)
Gracefully stops me from running.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
NTV2OutputXptID GetMixerOutputXptFromChannel(const NTV2Channel inChannel, const bool inIsKey=false)
virtual std::string GetOnAirCharacters(const UWord inRowNumber=0) const
Retrieves all "on-air" characters either for all rows, or a specific row.
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
virtual void DoCCOutput(const uint32_t inFrameNum, const CaptionData &inCCData, const NTV2VideoFormat inVideoFormat)
Outputs CC data.
NTV2Buffer & VideoBuffer(void)
#define NTV2_IS_4K_VIDEO_FORMAT(__f__)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
virtual std::string GetModelName(void)
Answers with this device's model name.
enum _CaptionDecode608Stats CaptionDecode608Stats
The currently supported caption decoder stats.
virtual class DeviceCapabilities & features(void)
virtual void SwitchOutput(void)
Switches/rotates –output mode.
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
This class is used to respond to dynamic events that occur during CEA-608 caption decoding.
NTV2InputXptID GetMixerBGInputXpt(const NTV2Channel inChannel, const bool inIsKey=false)
std::string & lower(std::string &str)
std::string NTV2InputSourceToString(const NTV2InputSource inValue, const bool inForRetailDisplay=false)
static const ULWord kAppSignature((((uint32_t)( 'C'))<< 24)|(((uint32_t)( 'C'))<< 16)|(((uint32_t)( 'G'))<< 8)|(((uint32_t)( 'R'))<< 0))
std::string NTV2TCIndexToString(const NTV2TCIndex inValue, const bool inCompactDisplay=false)
virtual AJAStatus SetupOutputVideo(const NTV2VideoFormat inVideoFormat)
Sets up everything I need for capturing video.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
static std::string GetStatTitle(const CaptionDecode608Stats inStat)
@ NTV2_VANCMODE_TALL
This identifies the "tall" mode in which there are some VANC lines in the frame buffer.
NTV2Standard
Identifies a particular video standard.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual CNTV2CaptionDecodeChannel608Ptr Get608ChannelDecoder(const NTV2Line21Channel inChannel) const
virtual bool EnableChannels(const NTV2ChannelSet &inChannels, const bool inDisableOthers=(0))
Enables the given FrameStore(s).
@ NTV2_VANCDATA_8BITSHIFT_ENABLE
static AJALabelValuePairs & append(AJALabelValuePairs &inOutTable, const std::string &inLabel, const std::string &inValue=std::string())
A convenience function that appends the given label and value strings to the provided AJALabelValuePa...
#define AsConstUBytePtr(__p__)
NTV2Buffer fVideoBuffer
Host video buffer.
@ NTV2_VANCDATA_8BITSHIFT_DISABLE
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
ULWord VideoBufferSize(void) const
@ NTV2_CC608_CapModeUnknown
Unknown or invalid caption mode.
static std::string GetLine21ChannelNames(std::string inDelimiter=", ")
Returns a string containing a concatenation of human-readable names of every available NTV2Line21Chan...
NTV2ReferenceSource
These enum values identify a specific source for the device's (output) reference clock.
virtual const uint8_t * GetPayloadData(void) const
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=(!(0)))
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
@ kOutputMode_CaptionScreen
static size_t SetDefaultPageSize(void)
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
virtual AJAStatus ParsePayloadData(void)
Parses out (interprets) the "local" ancillary data from my payload data.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
virtual bool GetNextServiceBlockInfoFromQueue(const size_t svcIndex, size_t &outBlockSize, size_t &outDataSize, int &outServiceNum, bool &outIsExtended) const
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
ULWord AncBufferSize(void) const
enum _OutputMode OutputMode
virtual bool SubscribeChangeNotification(NTV2Caption608Changed *pInCallback, void *pInUserData=0)
Subscribes to change notifications.
virtual bool ParseSMPTE334AncPacket(bool &outHasParityErrors)
Parses the current frame's SMPTE 334 Ancillary packet and extracts the 608 and 708 caption data from ...
virtual AJAStatus SetupHostBuffers(const NTV2VideoFormat inVideoFormat)
Sets up my circular buffers.
This class is used to configure an NTV2CCGrabber instance.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
ULWord GetCapturedAncByteCount(const bool inField2=false) const
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord AudioBufferSize(void) const
ULWord AncBuffer2Size(void) const
#define NTV2_IS_VANCMODE_ON(__v__)
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
std::string fDeviceSpec
The AJA device to use.
virtual AJAStatus StartPlayThread(void)
Starts my playout thread.
static std::string CaptionDataSrcToString(const CaptionDataSrc inDataSrc)
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
NTV2Buffer & AudioBuffer(void)
#define AJA_SUCCESS(_status_)
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
@ NTV2MIXERMODE_FOREGROUND_ON
Passes only foreground video + key to the Mixer output.
static const NTV2Line21Attributes kRedOnTransparentBG(NTV2_CC608_Red, NTV2_CC608_Blue, NTV2_CC608_Transparent)
#define NTV2_IS_HD_VIDEO_FORMAT(__f__)
static std::string GetLegalCaptionDataSources(void)
bool IsStopped(void) const
static bool Create(CNTV2CaptionDecoder608Ptr &outEncoder)
Creates a new CNTV2CaptionEncoder608 instance.
std::ostream & operator<<(std::ostream &ioStrm, const CCGrabberConfig &inObj)
virtual bool IsDeviceReady(const bool inCheckValid=(0))
bool bGotField2Data
True if Field 2 bytes have been set; otherwise false.
ULWord GetVideoWriteSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
Identical to the GetVideoActiveSize function, except rounds the result up to the nearest 4K page size...
static std::string GetLegalOutputModes(void)
bool CanDoInputSource(const NTV2InputSource inSrc)
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
NTV2Buffer acANCField2Buffer
The host "Field 2" ancillary data buffer. This field is owned by the client application,...
virtual bool SetTsiFrameEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 two-sample interleave (Tsi) frame mode on the device.
#define IS_VALID_OutputMode(_x_)
virtual bool GetReference(NTV2ReferenceSource &outRefSource)
Answers with the device's current clock reference source.
@ kOutputMode_CaptionFileSCC
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
uint16_t GetEndFrame(void) const
NTV2OutputXptID GetDLInOutputXptFromChannel(const NTV2Channel inDLInput)
#define NTV2_AUDIOSIZE_MAX
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual void IdleFrame(void)
Call this once per video frame when I'm doing display "burn-in", whether there is new caption data to...
std::vector< std::string > NTV2StringList
#define AsConstULWordPtr(__p__)
NTV2VANCMode
These enum values identify the available VANC modes.
#define IS_VALID_CaptionDataSrc(_x_)
#define NTV2_IS_VALID_CHANNEL(__x__)
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
@ NTV2_DISABLE_TASKS
0: Disabled: Device is completely configured by controlling application(s) – no driver involvement.
static void PlayThreadStatic(AJAThread *pThread, void *pContext)
This is the playout thread's static callback function that gets called when the playout thread runs....
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
virtual bool SetMixerFGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the foreground input control value for the given mixer/keyer.
virtual std::string CompareWithInfo(const AJAAncillaryList &inCompareList, const bool inIgnoreLocation=true, const bool inIgnoreChecksum=true) const
Compares me with another list and returns a std::string that contains a human-readable explanation of...
virtual AJAStatus SetupInputVideo(void)
Sets up everything I need for capturing video.
NTV2Channel fInputChannel
The device channel to use.
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
NTV2OutputCrosspointID
Identifies a widget output, a signal source, that potentially can drive another widget's input (ident...
std::vector< NTV2Channel > NTV2ChannelList
An ordered sequence of NTV2Channel values.
void * GetHostPointer(void) const
virtual bool IsStandardTwoSampleInterleave(void) const
virtual void ReleaseHostBuffers(void)
Releases my circular buffers.
static const NTV2WidgetID gSDIOutputs[]
virtual void CaptureFrames(void)
Repeatedly captures frames using AutoCirculate (until global quit flag set).
NTV2Buffer fAudioBuffer
Host audio buffer.
virtual size_t GetNextServiceBlockFromQueue(const size_t svcIndex, std::vector< UByte > &outData)
Declares the CNTV2CaptionRenderer class.
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=(0))
Connects the given widget signal input (sink) to the given widget signal output (source).
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
static void Caption608ChangedStatic(void *pInstance, const NTV2Caption608ChangeInfo &inChangeInfo)
This static function gets called whenever 608 captioning changes.
virtual NTV2VideoFormat WaitForStableInputSignal(void)
Wait for a stable input signal, and return it.
virtual bool SetDisplayChannel(const NTV2Line21Channel inChannel)
Changes the caption channel that I'm focused on (or that I'm currently "burning" into video).
const std::string & NTV2Line21ChannelToStr(const NTV2Line21Channel inLine21Channel, const bool inCompact=true)
Converts the given NTV2Line21Channel value into a human-readable string.
virtual bool SetSDIOutputStandard(const UWord inOutputSpigot, const NTV2Standard inValue)
Sets the SDI output spigot's video standard.
@ kOutputMode_CaptionStream
virtual bool DisableChannels(const NTV2ChannelSet &inChannels)
Disables the given FrameStore(s).
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
virtual bool SetMixerVancOutputFromForeground(const UWord inWhichMixer, const bool inFromForegroundSource=(!(0)))
Sets the VANC source for the given mixer/keyer to the foreground video (or not). See the SDI Ancillar...
UWord lastFrame(void) const
bool ConvertLine_v210_to_2vuy(const ULWord *pInSrcLine_v210, UByte *pOutDstLine_2vuy, const ULWord inNumPixels)
Converts a single 10-bit YCbCr 'v210' raster line to 8-bit YCbCr '2vuy'.
bool IsRunning(void) const
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
const char * GetRP188CString() const
virtual void SetCaptionDisplayChannel(const NTV2Line21Channel inNewChannel)
Changes the caption channel I'm displaying.
@ NTV2_AUDIO_LOOPBACK_ON
Embeds SDI input source audio into the data stream.
bool IsValid(void) const
Answers true if I'm valid, or false if I'm not valid.
Declares the AJAAncillaryData_Cea608_Vanc class.
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
virtual AJAStatus ParsePayloadData(void)
Parses (interprets) the "local" ancillary data from my payload data.
@ NTV2MIXERINPUTCONTROL_FULLRASTER
I can decode captions from frames captured from an AJA device in real time. I can optionally play the...
void Clear(void)
Clears my frame collection, their locks, everything.
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
NTV2OutputXptID GetTSIMuxOutputXptFromChannel(const NTV2Channel inTSIMuxer, const bool inLinkB=false, const bool inIsRGB=false)
virtual NTV2VideoFormat GetVideoFormat(void) const
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual void Switch608Source(void)
Switches/rotates –608src.
NTV2ChannelSet NTV2MakeChannelSet(const NTV2Channel inFirstChannel, const UWord inNumChannels=1)
This struct replaces the old RP188_STRUCT.
NTV2Buffer acVideoBuffer
The host video buffer. This field is owned by the client application, and thus is responsible for all...
virtual bool SetMixerMode(const UWord inWhichMixer, const NTV2MixerKeyerMode inMode)
Sets the mode for the given mixer/keyer.
bool IsRGBFormat(const NTV2FrameBufferFormat format)
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
@ AJAAncDataType_Cea708
CEA708 (SMPTE 334) HD Closed Captioning.
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
#define Hex0N(__x__, __n__)
virtual bool ProcessNew608FrameData(const CaptionData &inCC608Data)
Notifies me that new frame data has arrived. Clients should call this method once per video frame wit...
@ kCaptionDataSrc_Default
virtual bool IsRGBSampling(void) const
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
bool CanDoWidget(const NTV2WidgetID inWgtID)
virtual bool RouteInputSignal(const NTV2VideoFormat inVideoFormat)
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
static std::string OutputModeToString(const OutputMode inMode)
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
static const NTV2Line21Attributes kGreenOnTransparentBG(NTV2_CC608_Green, NTV2_CC608_Blue, NTV2_CC608_Transparent)
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
bool UnlockAll(CNTV2Card &inDevice)
virtual bool SetEnableVANCData(const bool inVANCenabled, const bool inTallerVANC, const NTV2Standard inStandard, const NTV2FrameGeometry inGeometry, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual CNTV2VPID & SetVPID(const ULWord inData)
const int NTV2_CC708PrimaryCaptionServiceNum
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
This class handles VANC-based CEA-608 caption data packets (not "analog" Line 21).
std::string NTV2ChannelToString(const NTV2Channel inValue, const bool inForRetailDisplay=false)
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
#define NTV2_IS_VANCMODE_TALL(__v__)
@ kCaptionDataSrc_708FBVanc
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
Declares the AJAAncillaryData_Cea708 class.
static bool DecodeLine(const UByte *pLineData, UByte &outChar1, UByte &outChar2)
Decodes the supplied line of 8-bit uncompressed ('2vuy') data and, if successful, returns the two 8-b...
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
bool bGotField1Data
True if Field 1 bytes have been set; otherwise false.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
NTV2InputXptID GetDLOutInputXptFromChannel(const NTV2Channel inDLOutWidget)
CEA-608 Character Attributes.
bool fUseVanc
If true, use Vanc, even if the device supports Anc insertion.
This structure encapsulates all possible CEA-608 caption data bytes that may be associated with a giv...
bool LockAll(CNTV2Card &inDevice)
static const UWord gMixerNums[]
bool GetInputTimeCodes(NTV2TimeCodeList &outValues) const
Intended for capture, answers with the timecodes captured in my acTransferStatus member's acFrameStam...
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
virtual AJAStatus Start()
static std::string StripFormatString(const std::string &inStr)
std::string NTV2ReferenceSourceToString(const NTV2ReferenceSource inValue, const bool inForRetailDisplay=false)
UByte f2_char2
Caption Byte 2 of Field 2.
static CaptionDataSrc StringToCaptionDataSrc(const std::string &inDataSrcStr)
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
#define xHEX0N(__x__, __n__)
#define AJA_FAILURE(_status_)
#define NTV2_IS_VALID_AUDIO_SYSTEM(__x__)
static void CaptureThreadStatic(AJAThread *pThread, void *pContext)
This is the capture thread's static callback function that gets called when the capture thread runs....
@ AJAAncDataType_Cea608_Line21
CEA608 SD Closed Captioning ("Line 21" waveform)
virtual bool SetSMPTE334AncData(const AJAAncillaryData_Cea708 &inPacket)
Copies the given SMPTE 334 CDP data from the given ancillary data packet into my private CDP buffer.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
NTV2Buffer & AncBuffer(void)
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
NTV2Buffer acANCBuffer
The host ancillary data buffer. This field is owned by the client application, and thus is responsibl...
NTV2CCGrabber(const CCGrabberConfig &inConfigData)
Constructs me using the given configuration settings.
virtual bool RouteOutputSignal(const NTV2VideoFormat inVideoFormat)
bool fBurnCaptions
If true, burn-in captions on 2nd channel.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
NTV2InputXptID GetTSIMuxInputXptFromChannel(const NTV2Channel inTSIMuxer, const bool inLinkB=false)
Declares the AJAMemory class.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
NTV2InputSource fInputSource
The device input connector to use.
UByte f1_char1
Caption Byte 1 of Field 1.
bool SetBuffers(ULWord *pInVideoBuffer, const ULWord inVideoByteCount, ULWord *pInAudioBuffer, const ULWord inAudioByteCount, ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
virtual NTV2Line21Channel GetDisplayChannel(void) const
Answers with the caption channel that I'm currently focused on (or that I'm currently "burning" into ...
virtual NTV2Line21Channel GetDisplayChannel(void) const
Answers with the caption channel that I'm currently focused on (or that I'm currently "burning" into ...
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
NTV2OutputXptID GetDLOutOutputXptFromChannel(const NTV2Channel inDLOutput, const bool inIsLinkB=false)
virtual void ToggleVANC(void)
Toggles the use of VANC. (Debug, experimental)
Utility class for timecodes.
static AJAStatus SetFromVANCData(const NTV2Buffer &inFrameBuffer, const NTV2FormatDescriptor &inFormatDesc, AJAAncillaryList &outPackets, const uint32_t inFrameNum=0)
Returns all packets found in the VANC lines of the given NTV2 frame buffer.
NTV2TimeCodes fTimecodes
Map of TC indexes to NTV2_RP188 values.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID
virtual bool UnsubscribeChangeNotification(NTV2Caption608Changed *pInCallback, void *pInUserData=0)
Unsubscribes a prior change notification subscription.