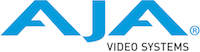 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
9 #if defined(AJA_WINDOWS)
11 #elif defined(AJA_LINUX)
13 #elif defined(AJA_MAC)
15 #elif defined(AJA_BAREMETAL)
94 return mpImpl->
Start();
103 return mpImpl->
Stop(timeout);
111 return mpImpl->
Kill(exitCode);
172 return mpImpl->
Attach(pThreadFunction, pUserContext);
virtual AJAStatus SetRealTime(AJAThreadRealTimePolicy policy, int priority)
virtual bool IsCurrentThread()
virtual AJAStatus ThreadInit()
@ AJA_DebugSeverity_Warning
static uint64_t GetThreadId()
AJAStatus SetThreadName(const char *name)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
Declares the AJATime class.
static uint64_t GetThreadId()
virtual AJAStatus Kill(uint32_t exitCode)
AJAStatus Kill(uint32_t exitCode)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
Declares the AJAThreadImpl class.
#define AJA_SUCCESS(_status_)
#define AJA_REPORT(_index_, _severity_, _format_,...)
Declares the AJAThreadImpl class.
virtual AJAStatus ThreadRun()
virtual AJAStatus Stop(uint32_t timeout=0xffffffff)
Declares the AJAThreadImpl class.
virtual AJAStatus SetThreadName(const char *name)
AJAStatus Stop(uint32_t timeout=0xffffffff)
void AJAThreadFunction(AJAThread *pThread, void *pContext)
virtual bool ThreadLoop()
virtual AJAStatus GetPriority(AJAThreadPriority *pPriority)
virtual AJAStatus SetPriority(AJAThreadPriority priority)
AJAStatus SetPriority(AJAThreadPriority threadPriority)
virtual AJAStatus Start()
AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual AJAStatus ThreadFlush()
AJAStatus GetPriority(AJAThreadPriority *pThreadPriority)
Declares the AJADebug class.
AJAStatus SetRealTime(AJAThreadRealTimePolicy policy, int priority)