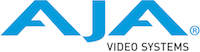 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
18 #if defined(NTV2_DEPRECATE_17_1)
23 uint64_t deviceSerialNumber;
24 string deviceIdentifier;
25 #if defined(VIRTUAL_DEVICES_SUPPORT)
26 bool isVirtualDevice=
false;
27 std::string virtualDeviceName;
28 std::string virtualDeviceID;
29 #endif // defined(VIRTUAL_DEVICES_SUPPORT)
36 {
static const string sHexDigits(
"0123456789ABCDEFabcdef");
37 return sHexDigits.find(inChr) != string::npos;
41 {
static const string sDecDigits(
"0123456789");
42 return sDecDigits.find(inChr) != string::npos;
46 {
static const string sLegalChars(
"0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz");
47 return sLegalChars.find(inChr) != string::npos;
52 if (inStr.length() > inMaxLength)
54 for (
size_t ndx(0); ndx < inStr.size(); ndx++)
62 if (inStr.length() < 3)
65 if (hexStr[0] ==
'0' && hexStr[1] ==
'x')
67 if (hexStr.length() > 16)
69 for (
size_t ndx(0); ndx < hexStr.size(); ndx++)
72 while (hexStr.length() != 16)
73 hexStr =
'0' + hexStr;
74 istringstream iss(hexStr);
82 for (
size_t ndx(0); ndx < inStr.size(); ndx++)
87 #endif // !defined(NTV2_DEPRECATE_17_1)
91 if (inStr.length() != 8 && inStr.length() != 9)
105 #if defined(NTV2_DEPRECATE_17_1)
106 void ScanHardware (
void)
114 if (!tmpDev.IsOpen())
117 info.
deviceID = tmpDev.GetDeviceID();
124 #else // !defined(NTV2_DEPRECATE_17_1)
131 #if !defined(NTV2_DEPRECATE_16_3)
138 #endif // !defined(NTV2_DEPRECATE_16_3)
152 for (
UWord boardNum(0); ; boardNum++)
155 if (!tmpDev.IsOpen())
213 SetAudioAttributes(info, tmpDev);
219 #if defined(VIRTUAL_DEVICES_SUPPORT)
220 NTV2SerialToVirtualDevices vdMap;
221 GetSerialToVirtualDeviceMap(vdMap);
224 for (
auto hwInfo : hwList)
227 auto it = vdMap.find(hwSN);
228 if (it != vdMap.end())
230 std::vector<VirtualDeviceInfo> vdevs = it->second;
231 for (
const auto& vdev : vdevs)
233 hwInfo.deviceIndex = vdIndex++;
234 hwInfo.isVirtualDevice =
true;
235 hwInfo.virtualDeviceID = vdev.vdID;
236 hwInfo.virtualDeviceName = vdev.vdName;
241 #endif // defined(VIRTUAL_DEVICES_SUPPORT)
251 if (iter->deviceID == inDeviceID)
267 return outDeviceInfo.
deviceIndex == inDeviceIndexNumber;
272 #endif // !defined(NTV2_DEPRECATE_17_1)
279 return size_t(inDeviceIndexNumber) <
sDevInfoList.size()
280 ? outDevice.
Open(
UWord(inDeviceIndexNumber))
304 if (inNameSubString.find(
":") != string::npos)
305 return outDevice.
Open(inNameSubString);
311 string nameSubString(inNameSubString);
aja::lower(nameSubString);
315 if (deviceName.find(nameSubString) != string::npos)
318 if (nameSubString ==
"io4kplus")
320 nameSubString =
"avid dnxiv";
324 if (deviceName.find(nameSubString) != string::npos)
338 string searchSerialStr(inSerialStr);
aja::lower(searchSerialStr);
346 if (serNumStr.find(searchSerialStr) != string::npos)
360 if (
sDevInfoList.at(ndx).deviceSerialNumber == inSerialNumber)
369 if (inArgument.empty())
375 string upperArg(inArgument);
aja::upper(upperArg);
376 if (upperArg ==
"LIST" || upperArg ==
"?")
379 cout <<
"No devices detected" << endl;
387 cout <<
" | " << setw(9) << serNum <<
" | " <<
HEX0N(
sDevInfoList.at(ndx).deviceSerialNumber,8);
390 #if defined(VIRTUAL_DEVICES_SUPPORT)
393 cout <<
"*** Virtual Devices ***" << endl;
397 cout <<
DECN(iter->deviceIndex,2) <<
" | " << setw(15) << iter->virtualDeviceName;
398 cout <<
" | " << iter->virtualDeviceID;
401 cout <<
" " << serNum;
406 #endif // defined(VIRTUAL_DEVICES_SUPPORT)
410 #if defined(VIRTUAL_DEVICES_SUPPORT)
413 string cp2ConfigPath;
414 GetCP2ConfigPath(cp2ConfigPath);
415 std::ifstream cfgJsonfile(cp2ConfigPath);
418 if (iter->isVirtualDevice)
421 iter->virtualDeviceName == inArgument ||
422 iter->virtualDeviceID == inArgument)
424 string inVDSpec =
"ntv2virtualdev://localhost/?CP2ConfigPath=" + cp2ConfigPath +
426 "&vdid=" + iter->virtualDeviceID +
428 return outDevice.
Open(inVDSpec);
433 #endif // defined(VIRTUAL_DEVICES_SUPPORT)
434 return outDevice.
Open(inArgument);
441 if (!inDevice.IsOpen())
444 if (!inDevice.GetHostName().empty() && inDevice.IsRemote())
445 return inDevice.GetHostName();
454 if (!str.empty() && str !=
"???")
458 ostringstream oss; oss <<
DEC(inDevice.GetIndexNumber());
463 #if !defined(NTV2_DEPRECATE_17_1)
467 inOutStr <<
" " << *iter;
494 return diffs ?
false :
true;
503 deviceSerialNumber = 0;
506 numAnlgVidInputs = 0;
507 numAnlgVidOutputs = 0;
508 numHDMIVidInputs = 0;
509 numHDMIVidOutputs = 0;
510 numInputConverters = 0;
511 numOutputConverters = 0;
513 numDownConverters = 0;
514 downConverterDelay = 0;
515 isoConvertSupport =
false;
516 rateConvertSupport =
false;
517 dvcproHDSupport =
false;
520 quarterExpandSupport =
false;
521 vidProcSupport =
false;
522 dualLinkSupport =
false;
523 colorCorrectionSupport =
false;
524 programmableCSCSupport =
false;
525 rgbAlphaOutputSupport =
false;
526 breakoutBoxSupport =
false;
527 procAmpSupport =
false;
528 has2KSupport =
false;
529 has4KSupport =
false;
530 has8KSupport =
false;
531 has3GLevelConversion =
false;
532 proResSupport =
false;
533 sdi3GSupport =
false;
534 sdi12GSupport =
false;
536 biDirectionalSDI =
false;
537 ltcInSupport =
false;
538 ltcOutSupport =
false;
539 ltcInOnRefPort =
false;
540 stereoOutSupport =
false;
541 stereoInSupport =
false;
544 numAnalogAudioInputChannels = 0;
545 numAESAudioInputChannels = 0;
546 numEmbeddedAudioInputChannels = 0;
547 numHDMIAudioInputChannels = 0;
548 numAnalogAudioOutputChannels = 0;
549 numAESAudioOutputChannels = 0;
550 numEmbeddedAudioOutputChannels = 0;
551 numHDMIAudioOutputChannels = 0;
555 deviceIdentifier.clear();
556 audioSampleRateList.clear();
557 audioNumChannelsList.clear();
558 audioBitsPerSampleList.clear();
559 audioInSourceList.clear();
560 audioOutSourceList.clear();
578 outBoardsAdded.clear ();
579 outBoardsRemoved.clear ();
583 if (oldIter == inOldList.end () && newIter == inNewList.end ())
586 if (oldIter != inOldList.end () && newIter != inNewList.end ())
590 if (oldInfo != newInfo)
593 outBoardsRemoved.push_back (oldInfo);
597 outBoardsAdded.push_back (newInfo);
606 if (oldIter != inOldList.end () && newIter == inNewList.end ())
608 outBoardsRemoved.push_back (*oldIter);
613 if (oldIter == inOldList.end () && newIter != inNewList.end ())
615 if (newIter->deviceID && newIter->deviceID !=
NTV2DeviceID(0xFFFFFFFF))
616 outBoardsAdded.push_back (*newIter);
626 return !outBoardsAdded.empty () || !outBoardsRemoved.empty ();
634 inOutStr <<
" " << *iter;
643 inOutStr <<
" " << *iter;
661 return inOutStr <<
" ???";
668 inOutStr <<
" " << *iter;
677 <<
" Device Index Number: " << inInfo.
deviceIndex << endl
678 <<
" Device ID: 0x" << hex << inInfo.
deviceID << dec << endl
680 <<
" PCI Slot: 0x" << hex << inInfo.
pciSlot << dec << endl
694 <<
" Qrez: " << (inInfo.
qrezSupport ?
"Y" :
"N") << endl
695 <<
" HDV: " << (inInfo.
hdvSupport ?
"Y" :
"N") << endl
711 <<
" SDI 3G: " << (inInfo.
sdi3GSupport ?
"Y" :
"N") << endl
712 <<
" SDI 12G: " << (inInfo.
sdi12GSupport ?
"Y" :
"N") << endl
713 <<
" IP: " << (inInfo.
ipSupport ?
"Y" :
"N") << endl
715 <<
" LTC In: " << (inInfo.
ltcInSupport ?
"Y" :
"N") << endl
716 <<
" LTC Out: " << (inInfo.
ltcOutSupport ?
"Y" :
"N") << endl
717 <<
" LTC In on Ref Port: " << (inInfo.
ltcInOnRefPort ?
"Y" :
"N") << endl
746 inOutStr <<
"AudioPhysicalFormat:" << endl
747 <<
" boardNumber: " << inFormat.
boardNumber << endl
748 <<
" sampleRate: " << inFormat.
sampleRate << endl
749 <<
" numChannels: " << inFormat.
numChannels << endl
751 #if defined (DEBUG) || defined (AJA_DEBUG)
752 <<
" sourceIn: 0x" << hex << inFormat.
sourceIn << dec << endl
753 <<
" sourceOut: 0x" << hex << inFormat.
sourceOut << dec << endl
754 #endif // DEBUG or AJA_DEBUG
799 if (audioControl &
BIT(21))
830 #if defined(VIRTUAL_DEVICES_SUPPORT)
831 bool CNTV2DeviceScanner::GetSerialToVirtualDeviceMap (NTV2SerialToVirtualDevices & outSerialToVirtualDevMap)
833 string cp2ConfigPath;
834 GetCP2ConfigPath(cp2ConfigPath);
835 std::ifstream cfgJsonfile(cp2ConfigPath);
837 if (cfgJsonfile.is_open())
839 cp2Json = json::parse(cfgJsonfile);
845 for (
const auto& hwdev : cp2Json[
"v2"][
"deviceConfigList"])
847 std::vector<VirtualDeviceInfo> vdevs;
848 json hwdevice = hwdev;
849 for (
const auto& vdev : hwdevice[
"virtualDevices"])
851 VirtualDeviceInfo newVDev;
852 newVDev.vdID = vdev[
"id"];
853 newVDev.vdName = vdev[
"name"];
854 vdevs.push_back(newVDev);
857 outSerialToVirtualDevMap[hwdev[
"serial"]] = vdevs;
863 bool CNTV2DeviceScanner::GetCP2ConfigPath(
string & outCP2ConfigPath)
867 outCP2ConfigPath = outCP2ConfigPath +
"aja/controlpanelConfigPrimary.json";
870 #endif // defined(VIRTUAL_DEVICES_SUPPORT)
871 #endif // !defined(NTV2_DEPRECATE_17_1)
static uint64_t IsLegalHexSerialNumber(const std::string &inStr)
@ kDeviceGetNumVideoInputs
The number of SDI video inputs on the device.
@ kDeviceHasBiDirectionalSDI
True if device SDI connectors are bi-directional.
@ kDeviceCanDoAudio6Channels
True if audio system(s) support 6 or more audio channels.
NTV2AudioSourceList audioOutSourceList
My supported audio output destinations (AES, etc.)
bool quarterExpandSupport
static bool DeviceIDPresent(const NTV2DeviceID inDeviceID, const bool inRescan=(0))
UWord numAnalogAudioInputChannels
Total number of analog audio input channels.
bool biDirectionalSDI
Supports Bi-directional SDI.
NTV2AudioBitsPerSampleList audioBitsPerSampleList
My supported audio bits-per-sample.
@ kDeviceCanDoIP
True if device has SFP connectors.
@ kDeviceCanDoVideoProcessing
True if device can do video processing.
UWord numDownConverters
Total number of down-converters.
UWord numAnlgVidOutputs
Total number of analog video outputs.
Declares device capability functions.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
bool has3GLevelConversion
Supports 3G Level Conversion?
UWord numHDMIVidInputs
Total number of HDMI inputs.
@ kDeviceGetNumDownConverters
The number of down-converters on the device.
@ kDeviceCanDoHDV
True if device can squeeze/stretch between 1920x1080 and 1440x1080.
bool is_hex_digit(const char inChr)
bool sdi12GSupport
Supports 12G?
uint64_t deviceSerialNumber
Unique device serial number.
@ kDeviceGetNumAnalogAudioInputChannels
The number of analog audio input channels on the device.
#define NTV2_ASSERT(_expr_)
bool ltcInSupport
Accepts LTC input?
@ kDeviceGetNumEmbeddedAudioOutputChannels
The number of SDI-embedded output audio channels supported by the device.
NTV2DeviceInfoList GetDeviceInfoList(void)
NTV2AudioSourceList::const_iterator NTV2AudioSourceListConstIter
UWord numHDMIVidOutputs
Total number of HDMI outputs.
static AJALock sDevInfoListLock
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
static bool GetFirstDeviceWithID(const NTV2DeviceID inDeviceID, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device found on the host t...
std::vector< NTV2DeviceInfo > NTV2DeviceInfoList
I am an ordered list of NTV2DeviceInfo structs.
@ kDeviceCanDo4KVideo
True if the device can handle 4K/UHD video.
UWord numSerialPorts
Total number of serial ports.
@ kDeviceCanDoAudio96K
True if Audio System(s) support a 96kHz sample rate.
@ kDeviceCanDo3GLevelConversion
True if device can do 3G level B to 3G level A conversion.
@ kDeviceCanDoQuarterExpand
True if device can handle quarter-sized frames (pixel-halving and line-halving during input,...
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
@ kDeviceGetNumVideoOutputs
The number of SDI video outputs on the device.
CNTV2DeviceScanner(const bool inScanNow=(!(0)))
static std::string SerialNum64ToString(const uint64_t inSerialNumber)
Returns a string containing the decoded, human-readable device serial number.
@ kDeviceGetNumAESAudioOutputChannels
The number of AES/EBU audio output channels on the device.
@ kDeviceCanDoColorCorrection
True if device has any LUTs.
@ kDeviceCanDoDualLink
True if device supports 10-bit RGB input/output over 2-wire SDI.
static size_t GetNumDevices(void)
static bool GetFirstDeviceWithSerial(const std::string &inSerialStr, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device whose serial number...
@ kDeviceGetNumAnalogAudioOutputChannels
The number of analog audio output channels on the device.
@ kDeviceGetNumDMAEngines
The number of DMA engines on the device.
UWord numHDMIAudioOutputChannels
Total number of HDMI audio output channels.
UWord numAnlgVidInputs
Total number of analog video inputs.
NTV2AudioChannelsPerFrameList::const_iterator NTV2AudioChannelsPerFrameListConstIter
virtual bool IsSupported(const NTV2BoolParamID inParamID)
@ kDeviceCanDoDVCProHD
True if device can squeeze/stretch between 1920x1080/1280x1080 and 1280x720/960x720.
std::vector< AudioBitsPerSampleEnum > NTV2AudioBitsPerSampleList
std::vector< AudioSampleRateEnum > NTV2AudioSampleRateList
static bool CompareDeviceInfoLists(const NTV2DeviceInfoList &inOldList, const NTV2DeviceInfoList &inNewList, NTV2DeviceInfoList &outDevicesAdded, NTV2DeviceInfoList &outDevicesRemoved)
@ kDeviceCanDoAnalogAudio
True if device has any analog inputs or outputs.
UWord numVidOutputs
Total number of video outputs – analog, digital, whatever.
std::string & lower(std::string &str)
@ kDeviceGetNumAudioSystems
The number of independent Audio Systems on the device.
std::string deviceIdentifier
Device name as seen in Control Panel, Watcher, Cables, etc.
NTV2AudioSourceList audioInSourceList
My supported audio input sources (AES, ADAT, etc.)
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
Declares the AJALock class.
bool has4KSupport
Supports 4K formats?
virtual AJAStatus GetValue(const AJASystemInfoTag inTag, std::string &outValue) const
Answers with the host system info value string for the given AJASystemInfoTag.
@ kDeviceCanDoProRes
True if device can can accommodate Apple ProRes-compressed video in its frame buffers.
@ kDeviceGetNumAnalogVideoInputs
The number of analog video inputs on the device.
Declares the CNTV2DeviceScanner class.
UWord numOutputConverters
Total number of output converters.
@ kDeviceCanDoLTCInOnRefPort
True if device can read LTC (Linear TimeCode) from its reference input.
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
static void ScanHardware(void)
static bool GetDeviceInfo(const ULWord inDeviceIndexNumber, NTV2DeviceInfo &outDeviceInfo, const bool inRescan=(0))
virtual bool GetSerialNumberString(std::string &outSerialNumberString)
Answers with a string that contains my human-readable serial number.
@ kDeviceGetNumInputConverters
The number of input converter widgets on the device.
std::string & upper(std::string &str)
virtual uint64_t GetSerialNumber(void)
Answers with my serial number.
Declares numerous NTV2 utility functions.
UWord numDMAEngines
Total number of DMA engines.
static bool GetFirstDeviceWithName(const std::string &inNameSubString, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device whose device identi...
bool is_decimal_digit(const char inChr)
static bool GetDeviceWithSerial(const uint64_t inSerialNumber, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device whose serial number...
I interrogate and control an AJA video/audio capture/playout device.
bool rgbAlphaOutputSupport
Supports RGB alpha channel?
@ AJA_SystemInfoTag_Path_PersistenceStoreUser
static NTV2DeviceInfoList sDevInfoList
@ kDeviceCanDoBreakoutBox
True if device supports an AJA breakout box.
UWord numAESAudioOutputChannels
Total number of AES audio output channels.
@ kNTV2EnumsID_WidgetID
Identifies the NTV2AudioWidgetID enumerated type.
static std::string GetDeviceRefName(CNTV2Card &inDevice)
@ kDeviceGetPingLED
The highest bit number of the LED bits in the Global Control Register on the device.
@ kDeviceCanDoStereoIn
True if device supports 3D video input over dual-stream SDI.
virtual ULWord GetNumSupported(const NTV2NumericParamID inParamID)
bool has2KSupport
Supports 2K formats?
@ kDeviceGetDownConverterDelay
The down-converter delay on the device.
bool proResSupport
Supports ProRes?
bool stereoOutSupport
Supports stereo output?
@ kDeviceGetNumAESAudioInputChannels
The number of AES/EBU audio input channels on the device.
UWord numUpConverters
Total number of up-converters.
@ kDeviceGetNumHDMIVideoInputs
The number of HDMI video inputs on the device.
UWord numEmbeddedAudioInputChannels
Total number of embedded (SDI) audio input channels.
static bool IsHexDigit(const char inChr)
bool programmableCSCSupport
Programmable color space converter?
@ kDeviceCanDoRGBPlusAlphaOut
True if device has CSCs capable of splitting the key (alpha) and YCbCr (fill) from RGB frame buffers ...
NTV2DeviceInfoList::const_iterator NTV2DeviceInfoListConstIter
bool multiFormat
Supports multiple video formats?
ULWord deviceIndex
Device index number – this will be phased out someday.
UWord numAnalogAudioOutputChannels
Total number of analog audio output channels.
UWord numHDMIAudioInputChannels
Total number of HDMI audio input channels.
UWord numVidInputs
Total number of video inputs – analog, digital, whatever.
bool has8KSupport
Supports 8K formats?
std::vector< AudioSourceEnum > NTV2AudioSourceList
@ kDeviceGetNumHDMIVideoOutputs
The number of HDMI video outputs on the device.
Private include file for all ajabase sources.
static bool GetDeviceAtIndex(const ULWord inDeviceIndexNumber, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device having the given zero-bas...
@ kDeviceGetNumHDMIAudioOutputChannels
The number of HDMI audio output channels on the device.
@ kDeviceGetNumHDMIAudioInputChannels
The number of HDMI audio input channels on the device.
#define HEX0N(__x__, __n__)
bool is_alpha_numeric(const char inChr)
ostream & operator<<(ostream &inOutStr, const NTV2DeviceInfoList &inList)
NTV2AudioPhysicalFormatList::const_iterator NTV2AudioPhysicalFormatListConstIter
NTV2AudioSampleRateList audioSampleRateList
My supported audio sample rates.
@ kDeviceCanDo8KVideo
True if device supports 8K video formats.
@ kDeviceHasMicrophoneInput
True if device has a microphone input connector.
virtual NTV2DeviceID GetDeviceID(void)
bool operator==(const NTV2DeviceInfo &rhs) const
ULWord pciSlot
PCI slot (if applicable and/or known)
@ kDeviceCanDoAudio2Channels
True if audio system(s) support 2 or more audio channels.
@ kDeviceCanDo12GSDI
True if device has 12G SDI connectors.
NTV2AudioSampleRateList::const_iterator NTV2AudioSampleRateListConstIter
bool ltcOutSupport
Supports LTC output?
UWord numInputConverters
Total number of input converters.
@ kDeviceCanDoRateConvert
True if device can do frame rate conversion.
bool ipSupport
Supports IP IO?
bool colorCorrectionSupport
Supports color correction?
bool sdi3GSupport
Supports 3G?
@ kDeviceCanDoProgrammableCSC
True if device has at least one programmable color space converter widget.
@ kDeviceCanDoAudio8Channels
True if audio system(s) support 8 or more audio channels.
std::string to_string(bool val)
bool breakoutBoxSupport
Can support a breakout box?
@ kDeviceCanDo2KVideo
True if device can handle 2Kx1556 (film) video.
struct NTV2DeviceInfo NTV2DeviceInfo
@ kDeviceGetNumUpConverters
The number of up-converters on the device.
std::vector< NTV2AudioPhysicalFormat > NTV2AudioPhysicalFormatList
I am an ordered list of NTV2AudioPhysicalFormat structs.
bool ltcInOnRefPort
Supports LTC on reference input?
NTV2AudioChannelsPerFrameList audioNumChannelsList
My supported number of audio channels per frame.
std::vector< AudioChannelsPerFrameEnum > NTV2AudioChannelsPerFrameList
@ kDeviceGetNumAnalogVideoOutputs
The number of analog video outputs on the device.
@ kDeviceGetNumOutputConverters
The number of output converter widgets on the device.
virtual bool Open(const UWord inDeviceIndex)
Opens a local/physical AJA device so it can be monitored/controlled.
@ kDeviceGetNumEmbeddedAudioInputChannels
The number of SDI-embedded input audio channels supported by the device.
@ kDeviceGetNumSerialPorts
The number of RS-422 serial ports on the device.
basic_json<> json
default specialization
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
bool stereoInSupport
Supports stereo input?
@ kDeviceCanDoQREZ
True if device can handle QRez.
static bool IsDecimalDigit(const char inChr)
UWord numAESAudioInputChannels
Total number of AES audio input channels.
@ kDeviceCanDoIsoConvert
True if device can do ISO conversion.
@ kDeviceCanDoMultiFormat
True if device can simultaneously handle different video formats on more than one SDI input or output...
NTV2DeviceID deviceID
Device ID/species (e.g., DEVICE_ID_KONA3G, DEVICE_ID_IOXT, etc.)
virtual bool Close(void)
Closes me, releasing host resources that may have been allocated in a previous Open call.
static bool IsAlphaNumeric(const char inStr)
static bool IsLegalSerialNumber(const std::string &inStr)
@ kDeviceGetNumLTCOutputs
The number of analog LTC outputs on the device.
virtual ULWordSet GetSupportedItems(const NTV2EnumsID inEnumsID)
NTV2AudioBitsPerSampleList::const_iterator NTV2AudioBitsPerSampleListConstIter
static bool IsLegalDecimalNumber(const std::string &inStr, const size_t maxLen=2)
UWord numAudioStreams
Maximum number of independent audio streams.
@ kDeviceCanDoStereoOut
True if device supports 3D video output over dual-stream SDI.
bool dualLinkSupport
Supports dual-link?
@ kDeviceGetNumLTCInputs
The number of analog LTC inputs on the device.
#define DECN(__x__, __n__)
UWord numEmbeddedAudioOutputChannels
Total number of embedded (SDI) audio output channels.