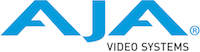 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
22 : mDevCap(driverInterface())
28 : mDevCap(driverInterface())
30 string hostName(inHostName);
97 string dateStr, timeStr;
101 oss << dateStr <<
" at " << timeStr;
103 oss <<
"Unavailable";
112 return ReadRegister (48, status) ? ((status >> 8) & 0xFF) : -1;
120 oss << hex << version;
133 string hostName(
"localhost"), snStr;
140 oss <<
" '" << snStr <<
"'";
141 if (!hostName.empty())
142 oss <<
" on '" << hostName <<
"'";
143 oss <<
" at index " <<
DEC(GetIndexNumber());
149 static const string sDriverBuildTypes [] = {
"",
"b",
"a",
"d"};
150 UWord versions[4] = {0, 0, 0, 0};
157 const string & dabr (sDriverBuildTypes[versBits >> 30]);
159 oss <<
DEC(versions[0]) <<
"." <<
DEC(versions[1]) <<
"." <<
DEC(versions[2]);
161 oss <<
"." <<
DEC(versions[3]);
163 oss << dabr <<
DEC(versions[3]);
171 outMajor = outMinor = outPoint = outBuild = 0;
172 ULWord driverVersionULWord (0);
175 if (!driverVersionULWord)
203 const uint64_t result((hi << 32) | lo);
219 if (outSerialNumberString.empty())
221 outSerialNumberString =
"INVALID?";
227 outSerialNumberString =
"5" + outSerialNumberString;
231 outSerialNumberString =
"6" + outSerialNumberString;
233 outSerialNumberString =
"7" + outSerialNumberString;
237 ULWord serialArray[] = {0,0,0,0};
242 outSerialNumberString.clear();
243 for (
int serialIndex(0); serialIndex < 4; serialIndex++)
244 if (serialArray[serialIndex] != 0xffffffff)
245 for (
int i(0); i < 4; i++)
247 const char tempChar(((serialArray[serialIndex] >> (i*8)) & 0xff));
248 if (tempChar > 0 && tempChar !=
'.')
249 outSerialNumberString.push_back(tempChar);
314 ::memset (&bitFileInfo, 0,
sizeof (bitFileInfo));
322 outDateStr =
reinterpret_cast <char *
> (&bitFileInfo.
dateStr [0]);
323 outTimeStr =
reinterpret_cast <char *
> (&bitFileInfo.
timeStr [0]);
332 oss << inBitFileInfo.
dateStr <<
" " << inBitFileInfo.
timeStr <<
" ";
342 outIsSafeBoot =
false;
351 outCanWarmBoot =
false;
359 outCanWarmBoot =
true;
371 const bool bPhonyKBox (
false);
419 #if !defined(NTV2_DEPRECATE_16_3)
465 return itms.find(
ULWord(inVideoFormat)) != itms.end();
470 return itms.find(
ULWord(inFBFormat)) != itms.end();
476 return wgtIDs.find(inWidgetID) != wgtIDs.end();
481 return itms.find(
ULWord(inConversionMode)) != itms.end();
486 return itms.find(
ULWord(inDSKMode)) != itms.end();
491 return itms.find(
ULWord(inInputSource)) != itms.end();
493 #endif // !defined(NTV2_DEPRECATE_16_3)
514 if (!inDevice.IsOpen())
519 mNumFrames =
UWord(totalBytes / m8MB);
520 if (totalBytes % m8MB)
521 {mNumFrames++; cerr <<
DEC(totalBytes % m8MB) <<
" leftover/spare bytes -- last frame is partial frame" << endl;}
522 for (
UWord frm(0); frm < mNumFrames; frm++)
530 for (FrameTagsConstIter it(mFrameTags.begin()); it != mFrameTags.end(); ++it)
533 oss <<
DEC0N(it->first,3) <<
": " <<
aja::join(tags,
", ") << endl;
541 for (
size_t ndx(0); ndx < inRgn1.size(); ndx++)
542 if (result.find(inRgn1.at(ndx)) == result.end())
543 result.insert(inRgn1.at(ndx));
544 for (
size_t ndx(0); ndx < inRgn2.size(); ndx++)
545 if (result.find(inRgn2.at(ndx)) == result.end())
546 result.insert(inRgn2.at(ndx));
547 for (
size_t ndx(0); ndx < inRgn3.size(); ndx++)
548 if (result.find(inRgn3.at(ndx)) == result.end())
549 result.insert(inRgn3.at(ndx));
561 const ULWord rgnInfo(*it);
562 const UWord startBlk(rgnInfo >> 16), numBlks(
UWord(rgnInfo & 0x0000FFFF));
566 oss <<
"Frms " <<
DEC0N(startBlk,3) <<
"-" <<
DEC0N(startBlk+numBlks-1,3) <<
" : ";
568 oss <<
"Frm " <<
DEC0N(startBlk,3) <<
" : ";
580 outFree.clear(); outUsed.clear(); outBad.clear();
581 FrameTagsConstIter it(mFrameTags.begin());
582 if (it == mFrameTags.end())
584 UWord frmStart(it->first), lastFrm(frmStart);
586 while (++it != mFrameTags.end())
593 if (frmStart != lastFrm)
594 outFree.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
596 outFree.push_back((
ULWord(frmStart) << 16) |
ULWord(1));
598 else if (runTags.size() > 1)
600 if (frmStart != lastFrm)
601 outBad.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
607 if (frmStart != lastFrm)
608 outUsed.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
610 outUsed.push_back((
ULWord(frmStart) << 16) |
ULWord(1));
612 frmStart = lastFrm = it->first;
620 if (frmStart != lastFrm)
621 outFree.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
623 outFree.push_back((
ULWord(frmStart) << 16) |
ULWord(1));
625 else if (runTags.size() > 1)
627 if (frmStart != lastFrm)
628 outBad.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
634 if (frmStart != lastFrm)
635 outUsed.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
637 outUsed.push_back((
ULWord(frmStart) << 16) |
ULWord(1));
645 FrameTagsConstIter it(mFrameTags.find(inIndex));
646 if (it == mFrameTags.end())
648 outTags = it->second;
654 FrameTagsConstIter it(mFrameTags.find(inIndex));
655 if (it == mFrameTags.end())
657 return it->second.size();
663 if (inIsQuad && inIsQuadQuad)
665 if (inSrcRgns.empty())
667 const UWord _8MB_frames_per_dest_frame(
UWord(GetIntrinsicFrameByteCount() / m8MB) * (inIsQuad?4:1) * (inIsQuadQuad?16:1));
668 if (!_8MB_frames_per_dest_frame)
670 if (_8MB_frames_per_dest_frame == 1)
671 {outDestRgns = inSrcRgns;
return true;}
674 for (
size_t ndx(0); ndx < inSrcRgns.size(); ndx++)
675 {
const ULWord val(inSrcRgns.at(ndx));
676 ULWord startBlkOffset(val >> 16), lengthBlks(val & 0x0000FFFF);
677 startBlkOffset = startBlkOffset / _8MB_frames_per_dest_frame + (startBlkOffset % _8MB_frames_per_dest_frame ? 1 : 0);
678 lengthBlks = lengthBlks / _8MB_frames_per_dest_frame;
679 outDestRgns.push_back((startBlkOffset << 16) | lengthBlks);
687 bool isReading(
false), isWriting(
false);
692 tag <<
"Aud" <<
DEC(audSys+1);
697 TagMemoryBlock(addr, m8MB, inMarkStoppedAudioBuffersFree && !isReading && !isWriting ?
string() : tag.str());
709 bool isEnabled(
false), isMultiFormat(
false), isQuad(
false), isQuadQuad(
false), isSquares(
false), isTSI(
false);
711 uint64_t addr(0), len(0);
712 if (skipChannels.find(chan) != skipChannels.end())
719 tag <<
"AC" <<
DEC(chan+1) << (acStatus.
IsInput() ?
" Write" :
" Read");
731 inDevice.
GetDeviceFrameInfo (
UWord(frameNum), chan, mIntrinsicSize, isMultiFormat, isQuad, isQuadQuad, isSquares, isTSI, addr, len);
733 tag <<
"MR" <<
DEC(chan+1);
735 tag <<
"Ch" <<
DEC(chan+1);
743 else if (isQuad && !isQuadQuad && isTSI)
766 if (inStartAddr % m8MB)
768 if (inByteLength % m8MB)
772 const UWord startFrm(
UWord(inStartAddr / m8MB)), frmCnt(
UWord(inByteLength / m8MB));
773 for (
UWord frm(0); frm < frmCnt; frm++)
775 UWord frameNum(startFrm + frm);
777 if (tags.find(inTag) == tags.end())
780 if (frameNum >= mNumFrames)
781 tags.insert(
"Invalid");
virtual ULWord DeviceGetAudioFrameBuffer(void)
@ kDeviceGetNumVideoInputs
The number of SDI video inputs on the device.
virtual bool IsAudioOutputRunning(const NTV2AudioSystem inAudioSystem, bool &outIsRunning)
Answers whether or not the playout side of the given NTV2AudioSystem is currently running.
bool TagMemoryBlock(const ULWord inStartAddr, const ULWord inByteLength, const std::string &inTag)
@ kDeviceCanDoStackedAudio
True if device uses a "stacked" arrangement of its audio buffers.
virtual bool SetFrameBufferSize(const NTV2Framesize inSize)
Sets the device's intrinsic frame buffer size.
virtual ULWord GetSerialNumberHigh(void)
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ kNTV2EnumsID_VideoFormat
Identifies the NTV2VideoFormat enumerated type.
Declares device capability functions.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
bool TagAudioBuffers(CNTV2Card &inDevice, const bool inMarkStoppedAudioBuffersFree)
std::set< std::string > NTV2StringSet
ULWord NTV2DeviceGetNumberFrameBuffers(NTV2DeviceID id, NTV2FrameGeometry fg, NTV2FrameBufferFormat fbf)
std::string & strip(std::string &str, const std::string &ws)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
bool _boardOpened
True if I'm open and connected to the device.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
virtual bool IS_CHANNEL_INVALID(const NTV2Channel inChannel) const
std::string join(const std::vector< std::string > &parts, const std::string &delim)
@ DEVICE_ID_KONA5
See KONA 5.
virtual bool IsBreakoutBoardConnected(void)
ULWord NTV2DeviceGetFrameBufferSize(NTV2DeviceID id, NTV2FrameGeometry fg, NTV2FrameBufferFormat fbf)
virtual Word GetDeviceVersion(void)
Answers with this device's version number.
@ DEVICE_ID_IOX3
See IoX3.
ULWord NTV2DeviceGetActiveMemorySize(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_IOIP_2022
See Io IP.
bool GetTagsForFrameIndex(const UWord inIndex, NTV2StringSet &outTags) const
Answers with the list of tags for the given frame number.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
@ NTV2_BreakoutCableBNC
Identifies the AES/EBU audio breakout cable that has BNC connectors.
virtual bool GetFrameBufferSize(const NTV2Channel inChannel, NTV2Framesize &outValue)
Answers with the frame size currently being used on the device.
@ DEVICE_ID_KONA5_8KMK
See KONA 5.
ULWord _ulNumFrameBuffers
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
#define DEC0N(__x__, __n__)
virtual bool GetInputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current input frame index number for the given FrameStore. This identifies which par...
@ kDeviceGetNumVideoOutputs
The number of SDI video outputs on the device.
virtual bool DeviceCanDoConversionMode(const NTV2ConversionMode inCM)
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
virtual bool GetInstalledBitfileInfo(ULWord &outNumBytes, std::string &outDateStr, std::string &outTimeStr)
Returns the size and time/date stamp of the device's currently-installed firmware.
@ kDeviceCanDo12gRouting
True if device supports 12G routing crosspoints.
virtual bool DeviceCanDoFrameBufferFormat(const NTV2PixelFormat inPF)
ULWord _ulFrameBufferSize
static std::string SerialNum64ToString(const uint64_t inSerialNumber)
Returns a string containing the decoded, human-readable device serial number.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool DeviceCanDoFormat(const NTV2FrameRate inFR, const NTV2FrameGeometry inFG, const NTV2Standard inStd)
@ NTV2_BreakoutNone
No identifiable breakout hardware appears to be attached.
virtual bool DeviceCanDoWidget(const NTV2WidgetID inWgtID)
virtual bool GetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat &outValue)
Returns the current frame buffer format for the given FrameStore on the AJA device.
@ kDeviceCanDoWarmBootFPGA
True if device can warm-boot to load updated firmware.
NTV2DeviceID _boardID
My cached device ID.
NTV2FrameRate
Identifies a particular video frame rate.
ULWord NTV2FramesizeToByteCount(const NTV2Framesize inFrameSize)
Converts the given NTV2Framesize value into an exact byte count.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
@ kDeviceGetNumBufferedAudioSystems
The total number of audio systems on the device that can read/write audio buffer memory....
virtual std::string GetDeviceVersionString(void)
Answers with this device's version number as a human-readable string.
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
ULWordSet::const_iterator ULWordSetConstIter
virtual bool IsSupported(const NTV2BoolParamID inParamID)
#define NTV2DriverVersionDecode_Point(__vers__)
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
virtual std::string GetModelName(void)
Answers with this device's model name.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
static bool isEnabled(CNTV2Card &device, const NTV2Channel inChannel)
virtual bool IS_INPUT_SPIGOT_INVALID(const UWord inInputSpigot)
virtual std::string GetBitfileInfoString(const BITFILE_INFO_STRUCT &inBitFileInfo)
Generates and returns an info string with date, time and name for the given inBifFileInfo.
virtual bool IsAudioInputRunning(const NTV2AudioSystem inAudioSystem, bool &outIsRunning)
Answers whether or not the capture side of the given NTV2AudioSystem is currently running.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
std::ostream & DumpBlocks(std::ostream &oss) const
Dumps all 8MB blocks/frames and their tags, if any, into the given stream.
@ kDeviceGetNumAudioSystems
The number of independent Audio Systems on the device.
virtual bool GetOutputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current output frame number for the given FrameStore (expressed as an NTV2Channel).
virtual bool IS_OUTPUT_SPIGOT_INVALID(const UWord inOutputSpigot)
NTV2Standard
Identifies a particular video standard.
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
virtual std::string GetPCIFPGAVersionString(void)
@ DEVICE_ID_KONA5_8K
See KONA 5.
@ DEVICE_ID_KONA3G
See KONA 3G (UFC Mode).
#define NTV2DriverVersionDecode_Major(__vers__)
static NTV2Buffer NULL_POINTER
Used for default empty NTV2Buffer parameters – do not modify.
@ kDeviceGetNumVideoChannels
The number of video channels supported on the device.
virtual std::string GetDescription(void) const
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
virtual bool DeviceCanDoInputSource(const NTV2InputSource inSrc)
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
virtual ULWord DeviceGetFrameBufferSize(void)
@ DEVICE_ID_KONAX
See KONA X.
virtual bool IS_HDMI_INPUT_SPIGOT_INVALID(const UWord inInputHDMIPort)
@ kNTV2EnumsID_ConversionMode
Identifies the NTV2ConversionMode enumerated type.
@ DEVICE_ID_KONA4UFC
See KONA 4 (UFC Mode).
virtual ULWord DeviceGetNumberFrameBuffers(void)
NTV2Mode
Used to identify the mode of a widget_framestore, or the direction of an AutoCirculate stream: either...
std::ostream & RawDump(std::ostream &oss) const
Dumps a human-readable list of regions into the given stream.
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
Declares the CNTV2Card class.
@ NTV2_BITFILE_IO4KPLUS_MAIN
virtual bool GetDeviceFrameInfo(const UWord inFrameNumber, const NTV2Channel inChannel, uint64_t &outAddress, uint64_t &outLength)
Answers with the address and size of the given frame.
virtual bool IsFailSafeBitfileLoaded(bool &outIsFailSafe)
Answers whether or not the "fail-safe" (aka "safe-boot") bitfile is currently loaded and running in t...
size_t GetTagCount(const UWord inIndex) const
virtual bool GetSerialNumberString(std::string &outSerialNumberString)
Answers with a string that contains my human-readable serial number.
ULWord NTV2DeviceGetAudioFrameBuffer2(NTV2DeviceID boardID, NTV2FrameGeometry frameGeometry, NTV2FrameBufferFormat frameFormat)
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
virtual uint64_t GetSerialNumber(void)
Answers with my serial number.
@ kNTV2EnumsID_InputSource
Identifies the NTV2InputSource enumerated type.
Declares numerous NTV2 utility functions.
NTV2Framesize
Kona2/Xena2 specific enums.
NTV2BreakoutType
Identifies the Breakout Boxes and Cables that may be attached to an AJA NTV2 device.
I interrogate and control an AJA video/audio capture/playout device.
@ DEVICE_ID_KONA5_8K_MV_TX
See KONA 5.
virtual bool CanWarmBootFPGA(bool &outCanWarmBoot)
Answers whether or not the FPGA can be reloaded without powering off.
bool IsStopped(void) const
@ DEVICE_ID_KONALHEPLUS
See KONA LHe Plus.
@ DEVICE_ID_KONA5_OE1
See KONA 5.
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
uint16_t GetEndFrame(void) const
@ kNTV2EnumsID_WidgetID
Identifies the NTV2AudioWidgetID enumerated type.
static NTV2WidgetID WidgetIDFromTypeAndChannel(const NTV2WidgetType inWidgetType, const NTV2Channel inChannel)
virtual ULWord GetNumSupported(const NTV2NumericParamID inParamID)
virtual bool GetMode(const NTV2Channel inChannel, NTV2Mode &outValue)
Answers with the current NTV2Mode of the given FrameStore on the AJA device.
@ NTV2_BreakoutCableXLR
Identifies the AES/EBU audio breakout cable that has XLR connectors.
#define NTV2_IS_VALID_CHANNEL(__x__)
virtual std::string GetDriverVersionString(void)
Answers with this device's driver's version as a human-readable string.
virtual NTV2BreakoutType GetBreakoutHardware(void)
bool AssessDevice(CNTV2Card &inDevice, const bool inIgnoreStoppedAudioBuffers=(0))
Assesses the given device.
@ kDeviceGetNumHDMIVideoInputs
The number of HDMI video inputs on the device.
NTV2InputSource
Identifies a specific video input source.
@ DEVICE_ID_IOIP_2110
See Io IP.
@ DEVICE_ID_KONA5_3DLUT
See KONA 5.
virtual bool DeviceCanDoVideoFormat(const NTV2VideoFormat inVF)
virtual bool GetFrameGeometry(NTV2FrameGeometry &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
bool GetRegions(ULWordSequence &outFree, ULWordSequence &outUsed, ULWordSequence &outBad) const
Answers with the lists of free, in-use and conflicting 8MB memory blocks. Each ULWord represents a re...
bool TranslateRegions(ULWordSequence &outRgns, const ULWordSequence &inRgns, const bool inIsQuad, const bool inIsQuadQuad) const
Translates an 8MB-chunked list of regions into another list of regions with frame indexes and sizes e...
@ kNTV2EnumsID_DSKMode
Identifies the NTV2DSKMode enumerated type.
Private include file for all ajabase sources.
virtual ~CNTV2Card()
My destructor.
#define NTV2DriverVersionDecode_Minor(__vers__)
@ DEVICE_ID_IO4KPLUS
See Io4K Plus.
virtual bool DeviceCanDoDSKMode(const NTV2DSKMode inDSKM)
bool NTV2DeviceCanDoFormat(const NTV2DeviceID inDevID, const NTV2FrameRate inFR, const NTV2FrameGeometry inFG, const NTV2Standard inStd)
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
@ DEVICE_ID_KONA4
See KONA 4 (Quad Mode).
uint16_t GetStartFrame(void) const
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ kDeviceHasMicrophoneInput
True if device has a microphone input connector.
bool TagVideoFrames(CNTV2Card &inDevice)
virtual std::string GetDescription(void) const
NTV2FrameGeometry
Identifies a particular video frame geometry.
virtual bool IsChannelEnabled(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the given FrameStore is enabled.
bool CanDoWidget(const NTV2WidgetID inWgtID)
virtual ULWord GetFrameBufferSize(void) const
CNTV2Card()
My default constructor.
static ULWordSet CoalesceRegions(const ULWordSequence &inRgn1, const ULWordSequence &inRgn2, const ULWordSequence &inRgn3)
virtual NTV2DeviceID GetDeviceID(void)
virtual bool GetDriverVersionComponents(UWord &outMajor, UWord &outMinor, UWord &outPoint, UWord &outBuild)
Answers with the individual version components of this device's driver.
virtual bool IsMultiRasterWidgetChannel(const NTV2Channel inChannel)
virtual bool IsBufferSizeSetBySW(void)
virtual std::string GetFPGAVersionString(const NTV2XilinxFPGA inFPGA=eFPGAVideoProc)
@ kDeviceGetActiveMemorySize
The size, in bytes, of the device's active RAM available for video and audio.
@ NTV2_MODE_INVALID
The invalid mode.
@ DEVICE_ID_KONA3GQUAD
See KONA 3G (Quad Mode).
@ kDeviceCanReportFailSafeLoaded
True if device can report if its "fail-safe" firmware is loaded/running.
#define NTV2DriverVersionDecode_Build(__vers__)
std::string NTV2BitfileTypeToString(const NTV2BitfileType inValue, const bool inCompactDisplay=false)
#define NTV2_IS_INPUT_MODE(__mode__)
virtual ULWord GetSerialNumberLow(void)
@ kVRegDriverVersion
Packed driver version – use NTV2DriverVersionEncode, NTV2DriverVersionDecode* macros to encode/decode...
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
@ DEVICE_ID_IOIP_2110_RGB12
See Io IP.
@ DEVICE_ID_KONA5_2X4K
See KONA 5.
virtual bool HasMultiRasterWidget(void)
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
virtual bool GetAudioMemoryOffset(const ULWord inOffsetBytes, ULWord &outAbsByteOffset, const NTV2AudioSystem inAudioSystem, const bool inCaptureBuffer=(0))
Answers with the byte offset in device SDRAM into the specified Audio System's audio buffer.
virtual bool Open(const UWord inDeviceIndex)
Opens a local/physical AJA device so it can be monitored/controlled.
@ DEVICE_ID_IO4K
See Io4K (Quad Mode).
virtual bool DriverGetBitFileInformation(BITFILE_INFO_STRUCT &outBitFileInfo, const NTV2BitFileType inBitFileType=NTV2_VideoProcBitFile)
Answers with the currently-installed bitfile information.
@ DEVICE_ID_KONALHI
See KONA LHi.
virtual ULWord DeviceGetAudioFrameBuffer2(void)
std::string SerialNum64ToString(const uint64_t &inSerNum)
virtual Word GetPCIFPGAVersion(void)
virtual bool Close(void)
Closes me, releasing host resources that may have been allocated in a previous Open call.
virtual ULWordSet GetSupportedItems(const NTV2EnumsID inEnumsID)
UWord NTV2DeviceGetSPIFlashVersion(const NTV2DeviceID inDeviceID)
@ kNTV2EnumsID_PixelFormat
Identifies the NTV2PixelFormat enumerated type.
ULWord NTV2DeviceGetAudioFrameBuffer(NTV2DeviceID boardID, NTV2FrameGeometry frameGeometry, NTV2FrameBufferFormat frameFormat)