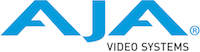 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
20 #if defined (MSWindows)
21 #pragma warning(disable: 4800)
24 #define HEX16(__x__) "0x" << hex << setw(16) << setfill('0') << uint64_t(__x__) << dec
25 #define INSTP(_p_) HEX16(uint64_t(_p_))
26 #define CVIDFAIL(__x__) AJA_sERROR (AJA_DebugUnit_VideoGeneric, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
27 #define CVIDWARN(__x__) AJA_sWARNING(AJA_DebugUnit_VideoGeneric, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
28 #define CVIDNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_VideoGeneric, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
29 #define CVIDINFO(__x__) AJA_sINFO (AJA_DebugUnit_VideoGeneric, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
30 #define CVIDDBG(__x__) AJA_sDEBUG (AJA_DebugUnit_VideoGeneric, INSTP(this) << "::" << AJAFUNC << ": " << __x__)
44 #define K2_NOMINAL_H 0x1000
45 #define K2_MIN_H (K2_NOMINAL_H-0x800)
46 #define K2_MAX_H (K2_NOMINAL_H+0x800)
47 #define K2_NOMINAL_V 0x0800
48 #define K2_MIN_V (K2_NOMINAL_V-0x400)
49 #define K2_MAX_V (K2_NOMINAL_V+0x400)
51 #define KLS_NOMINAL_525_H 0x0640
52 #define KLS_MIN_525_H 0x0000
53 #define KLS_MAX_525_H 0x06B3
54 #define KLS_NOMINAL_525_V 0x010A
55 #define KLS_MIN_525_V 0x0001
56 #define KLS_MAX_525_V 0x020D
58 #define KLS_NOMINAL_625_H 0x0638
59 #define KLS_MIN_625_H 0x0000
60 #define KLS_MAX_625_H 0x06BF
61 #define KLS_NOMINAL_625_V 0x0139
62 #define KLS_MIN_625_V 0x0001
63 #define KLS_MAX_625_V 0x0271
82 #if !defined(NTV2_DEPRECATE_16_2)
85 #endif // !defined(NTV2_DEPRECATE_16_2)
104 #if defined (NTV2_ALLOW_2MB_FRAMES)
107 #endif // defined (NTV2_ALLOW_2MB_FRAMES)
189 #if !defined(NTV2_DEPRECATE_16_3)
199 #endif // !defined(NTV2_DEPRECATE_16_3)
206 bool ajaRetail(inIsRetail);
209 GetEveryFrameServices(mode);
214 int hOffset(0), vOffset(0);
218 GetVideoHOffset(hOffset);
219 GetVideoVOffset(vOffset);
224 if (itms.find(
ULWord(value)) == itms.end())
246 SetStandard(inStandard, channel);
249 SetFrameGeometry(inFrameGeometry, ajaRetail, channel);
252 SetFrameRate(inFrameRate, channel);
263 SetQuadQuadFrameEnable(
false, channel);
264 Get4kSquaresEnable(squares, channel);
267 Set4kSquaresEnable(
true, channel);
271 SetQuadFrameEnable(
true, channel);
276 GetQuadQuadSquaresEnable(squares, channel);
279 SetQuadQuadSquaresEnable(
true, channel);
283 SetQuadQuadFrameEnable(
true, channel);
289 SetQuadFrameEnable(
false, channel);
290 SetQuadQuadFrameEnable(
false, channel);
291 if (!IsMultiFormatActive())
303 SetVideoHOffset(hOffset);
304 SetVideoVOffset(vOffset);
316 GetRegisterWriteMode(writeMode);
317 SetRegisterWriteMode(writeMode);
327 if (!SetVideoFormat(inVideoFormat, inIsAJARetail,
false, *it))
337 if (!IsMultiFormatActive () && !IsMultiRasterWidgetChannel(inChannel))
341 GetStandard (standard, inChannel);
344 GetFrameGeometry (frameGeometry, inChannel);
347 GetFrameRate (frameRate, inChannel);
350 GetSmpte372 (smpte372Enabled, inChannel);
352 ULWord progressivePicture;
353 GetProgressivePicture (progressivePicture);
355 bool isSquares =
false;
361 Get4kSquaresEnable(isSquares, inChannel);
374 return GetNTV2VideoFormat(frameRate, standard, isThreeG, inputGeometry, progressivePicture);
414 if (isThreeG && progressivePicture)
420 if (isThreeG && progressivePicture)
426 if (isThreeG && progressivePicture)
428 if (inputGeometry == 8)
433 else if (inputGeometry == 8)
601 if (GetVideoFormat(videoFormat))
618 int nominalH(0), minH(0), maxH(0), nominalV(0), minV(0), maxV(0), count(0);
619 ULWord timingValue(0), lineCount(0), lineCount2(0);
623 if (!GetNominalMinMaxHV(nominalH, minH, maxH, nominalV, minV, maxV))
628 nominalH = nominalH + hOffset;
630 nominalH = nominalH - hOffset;
635 else if (nominalH < minH)
638 if (!ReadOutputTimingControl(timingValue, inOutputSpigot))
647 if (
LWord((timingValue & 0x0000FFFF)) == nominalH)
649 if (((
LWord((timingValue & 0x0000FFFF)) + 1) == nominalH) )
653 timingValue &= 0xFFFF0000;
654 timingValue |=
ULWord(nominalH + 2);
655 WriteOutputTimingControl(timingValue, inOutputSpigot);
659 ReadLineCount (lineCount);
662 ReadLineCount (lineCount2);
663 if (count > 1000000)
return false;
665 }
while (lineCount == lineCount2);
670 else if ( ((
LWord((timingValue & 0x0000FFFF)) -1) == nominalH ) )
674 timingValue &= 0xFFFF0000;
675 timingValue |=
ULWord(nominalH - 2);
676 WriteOutputTimingControl(timingValue, inOutputSpigot);
680 ReadLineCount (lineCount);
683 ReadLineCount (lineCount2);
684 if (count > 1000000)
return false;
686 }
while (lineCount == lineCount2);
695 timingValue &= 0xFFFF0000;
696 timingValue |=
ULWord(nominalH);
698 return WriteOutputTimingControl(timingValue, inOutputSpigot);
704 int nominalH(0), minH(0), maxH(0), nominalV(0), minV(0), maxV(0);
707 if (!GetNominalMinMaxHV(nominalH, minH, maxH, nominalV, minV, maxV))
711 if (!ReadOutputTimingControl(timingValue, inOutputSpigot))
713 timingValue &= 0xFFFF;
717 outHOffset = int(timingValue) - nominalH;
719 outHOffset = nominalH - int(timingValue);
725 int nominalH(0), minH(0), maxH(0), nominalV(0), minV(0), maxV(0);
728 if (!GetNominalMinMaxHV(nominalH, minH, maxH, nominalV, minV, maxV))
733 nominalV = nominalV + vOffset;
735 nominalV = nominalV - vOffset;
740 else if (nominalV < minV)
744 if (!ReadOutputTimingControl(timingValue, inOutputSpigot))
746 timingValue &= 0x0000FFFF;
747 return WriteOutputTimingControl(timingValue |
ULWord(nominalV << 16), inOutputSpigot);
752 int nominalH(0), minH(0), maxH(0), nominalV(0), minV(0), maxV(0);
755 if (!GetNominalMinMaxHV(nominalH, minH, maxH, nominalV, minV, maxV))
759 if (!ReadOutputTimingControl(timingValue, inOutputSpigot))
761 timingValue = (timingValue >> 16);
765 outVOffset =
int(timingValue) - nominalV;
767 outVOffset = nominalV - int(timingValue);
771 #if !defined(NTV2_DEPRECATE_16_2)
774 outNumActiveLines = 0;
776 if (!GetStandard(st) || !GetVANCMode(vm))
785 outFrameDimensions.
Reset();
786 if (IsXilinxProgrammed() && GetStandard(st, inChannel) && GetVANCMode(vm, inChannel))
790 return outFrameDimensions.IsValid();
797 if (!IsXilinxProgrammed() || !GetStandard(st, inChannel) || !GetVANCMode(vm, inChannel))
802 #endif // defined(NTV2_DEPRECATE_16_2)
809 if (IsMultiRasterWidgetChannel(inChannel))
811 if (!IsMultiFormatActive())
830 if (IsMultiRasterWidgetChannel(inChannel))
832 if (!IsMultiFormatActive())
837 bool quadFrameEnabled(
false);
838 status = GetQuadFrameEnable(quadFrameEnabled, inChannel);
839 if (status && quadFrameEnabled)
843 bool quadQuadFrameEnabled(
false);
844 status = GetQuadQuadFrameEnable(quadQuadFrameEnabled);
845 if(status && quadQuadFrameEnabled)
857 ULWord smpte372Enabled (0);
859 outIsProgressive =
false;
861 if (!IsMultiFormatActive())
864 if (GetStandard (standard, inChannel) && GetSmpte372 (smpte372Enabled, inChannel))
867 outIsProgressive =
true;
879 outIsStandardDef =
false;
881 if (!IsMultiFormatActive())
884 if (GetStandard (standard, inChannel))
887 outIsStandardDef =
true;
901 GetEveryFrameServices(mode);
907 if (IsMultiRasterWidgetChannel(channel))
909 if (!IsMultiFormatActive())
911 else if (IS_CHANNEL_INVALID(channel))
921 bool status = GetFrameGeometry(oldGeometry, channel);
925 status = GetLargestFrameBufferFormatInUse(format);
932 newFrameStoreGeometry = newGeometry;
950 if ( GetFBSizeAndCountFromHW(_ulFrameBufferSize, _ulNumFrameBuffers) )
952 changeBufferSize =
false;
958 if ( changeBufferSize )
960 _ulFrameBufferSize = newFrameBufferSize;
973 if (IsMultiRasterWidgetChannel(inChannel))
978 if (!IsMultiFormatActive())
980 else if (IS_CHANNEL_INVALID(inChannel))
987 bool quadFrameEnabled(
false);
988 status = GetQuadFrameEnable(quadFrameEnabled, inChannel);
989 if (status && quadFrameEnabled)
993 bool quadQuadFrameEnabled(
false);
994 status = GetQuadQuadFrameEnable(quadQuadFrameEnabled);
995 if (status && quadQuadFrameEnabled)
1007 const ULWord loValue (value & 0x7);
1008 const ULWord hiValue ((value & 0x8) >> 3);
1010 if (IsMultiRasterWidgetChannel(inChannel))
1012 if (!IsMultiFormatActive ())
1024 ULWord returnVal1 (0), returnVal2 (0);
1026 if (IsMultiRasterWidgetChannel(inChannel))
1028 if (!IsMultiFormatActive())
1030 else if (IS_CHANNEL_INVALID(inChannel))
1036 outValue =
NTV2FrameRate((returnVal1 & 0x7) | ((returnVal2 & 0x1) << 3));
1047 if (IsMultiRasterWidgetChannel(inChannel))
1048 return inValue == 0;
1049 if (!IsMultiFormatActive())
1060 if (IsMultiRasterWidgetChannel(inChannel))
1061 {outValue = 0;
return true;}
1062 if (!IsMultiFormatActive())
1085 outValue = result1 ? returnVal : 0;
1096 if (IsMultiRasterWidgetChannel(inChannel))
1097 return inEnable ==
true;
1106 if (ok) ok = SetTsiFrameEnable(
true, inChannel);
1110 if(ok) ok = SetTsiFrameEnable(
true, inChannel);
1114 if(ok) ok = Set4kSquaresEnable(
true, inChannel);
1119 SetTsiFrameEnable(
false, inChannel);
1120 Set4kSquaresEnable(
false, inChannel);
1129 if (IsMultiRasterWidgetChannel(inChannel))
1134 if (!IsMultiFormatActive())
1154 if(ok) ok = SetQuadQuadSquaresEnable(
false, inChannel);
1157 if (!IsMultiFormatActive())
1213 bool quadEnabled (0);
1214 bool status2 (
true);
1215 bool s425Enabled (
false);
1216 bool status1 = Get4kSquaresEnable (quadEnabled, inChannel);
1218 status2 = GetTsiFrameEnable (s425Enabled, inChannel);
1220 outValue = (status1 & status2) ? ((quadEnabled | s425Enabled) ?
true :
false) :
false;
1244 if (IsMultiRasterWidgetChannel(inChannel))
1245 return inEnable ==
true;
1252 if (!IsMultiFormatActive())
1277 if (!IsMultiFormatActive())
1291 outIsEnabled =
false;
1292 if (IsMultiRasterWidgetChannel(inChannel))
1293 {outIsEnabled =
true;
return true;}
1296 ULWord squaresEnabled (0);
1304 outIsEnabled = (squaresEnabled ?
true :
false);
1317 if (IsMultiRasterWidgetChannel(inChannel))
1318 return enable ==
true;
1335 if (!IsMultiFormatActive())
1345 else if (!IsMultiFormatActive())
1374 if (!IsMultiFormatActive())
1383 else if (!IsMultiFormatActive())
1406 outIsEnabled =
false;
1409 if (IsMultiRasterWidgetChannel(inChannel))
1410 {outIsEnabled =
true;
return true;}
1415 bool returnVal(
false), readOkay(
false);
1419 readOkay = GetQuadQuadFrameEnable(returnVal, inChannel);
1435 outIsEnabled = readOkay ? returnVal : 0;
1442 outSyncFailed =
false;
1449 if (value & (1<<inWhichTsiMux))
1450 outSyncFailed =
true;
1456 ULWord standard(0), rate1(0), rate2(0), s372(0), geometry(0), format(0);
1465 if (!status)
return false;
1467 for (
int channel = inFirst; channel <= inLast; channel++)
1475 if (!status)
return false;
1487 SetLTCInputEnable(
false);
1490 EnableFramePulseReference(
false);
1494 ULWord refControl1 =
ULWord(inRefSource), refControl2 = 0, ptpControl = 0;
1495 switch (inRefSource)
1525 ULWord refControl2(0), ptpControl(0);
1539 case 4:
if (IsIPDevice())
1544 case 5:
if (IsIPDevice())
1582 outValue = returnValue == 0 ?
false :
true;
1608 { (
void) inIsRetail;
1609 if (IsMultiRasterWidgetChannel(inChannel))
1611 if (IS_CHANNEL_INVALID(inChannel))
1620 if (!SetMode(*it, inMode))
1627 if (IsMultiRasterWidgetChannel(inChannel))
1629 if (IS_CHANNEL_INVALID(inChannel))
1636 bool status = GetFrameGeometry(outGeometry);
1640 status = GetFrameBufferFormat(inChannel, outFBF);
1656 if (IsBufferSizeSetBySW())
1657 changeBufferSize =
false;
1659 return changeBufferSize;
1672 if (IsBufferSizeSetBySW())
1673 changeBufferSize =
false;
1675 return changeBufferSize;
1687 return swControl != 0;
1690 bool CNTV2Card::GetFBSizeAndCountFromHW (
ULWord & outSize,
ULWord & outCount)
1692 if (!IsBufferSizeSetBySW())
1695 ULWord ch1Control(0), multiplier(0), sizeMultiplier(0);
1703 case 0: multiplier = 4;
1707 case BIT_20: multiplier = 2;
1711 case BIT_21: multiplier = 1;
1716 sizeMultiplier = 16;
1720 outSize = sizeMultiplier * 1024 * 1024;
1721 outCount = multiplier ? multiplier * DeviceGetNumberFrameBuffers() : DeviceGetNumberFrameBuffers() / 2;
1724 GetFrameGeometry(geometry);
1755 return GetFBSizeAndCountFromHW(_ulFrameBufferSize, _ulNumFrameBuffers);
1771 if (!GetFrameGeometry(geometry))
1776 if ( ch1FrameBufferSize >= ch2FrameBufferSize )
1783 bool CNTV2Card::IsMultiFormatActive (
void)
1797 outConnections.clear();
1803 && ReadRegisters(ROMregs)
1814 { (
void) inIsRetailMode;
1816 if (IsMultiRasterWidgetChannel(inChannel))
1818 if (IS_CHANNEL_INVALID(inChannel))
1822 const ULWord loValue (inNewFormat & 0x0f);
1823 const ULWord hiValue ((inNewFormat & 0x10) >> 4);
1826 bool status = GetFrameInfo(inChannel, currentGeometry, currentFormat);
1835 if ( !GetFBSizeAndCountFromHW(_ulFrameBufferSize, _ulNumFrameBuffers) &&
1836 IsBufferSizeChangeRequired(inChannel, currentGeometry, currentFormat, inNewFormat) )
1843 {
if (inNewFormat != currentFormat)
1844 CVIDINFO(
"'" << GetDisplayName() <<
"': Channel " <<
DEC(
UWord(inChannel)+1) <<
" FBF changed from "
1847 <<
" numFBs=" <<
DEC(_ulNumFrameBuffers) <<
")");}
1849 CVIDFAIL(
"'" << GetDisplayName() <<
"': Failed to change channel " <<
DEC(
UWord(inChannel)+1) <<
" FBF from "
1852 SetVPIDTransferCharacteristics(inXferChars, inChannel);
1853 SetVPIDColorimetry(inColorimetry, inChannel);
1854 SetVPIDLuminance(inLuminance, inChannel);
1860 const bool inIsAJARetail,
1867 if (!SetFrameBufferFormat (*it, inNewFormat, inIsAJARetail, inXferChars, inColorimetry, inLuminance))
1869 return failures == 0;
1877 if (IsMultiRasterWidgetChannel(inChannel))
1879 if (IS_CHANNEL_INVALID (inChannel))
1882 ULWord returnVal1, returnVal2;
1887 return result1 && result2;
1895 if (IS_CHANNEL_INVALID (channel))
1905 if (IS_CHANNEL_INVALID (inChannel))
1915 if (IS_CHANNEL_INVALID (channel))
1918 ULWord loValue = quality & 0x1;
1919 ULWord hiValue = (quality >> 1) & 0x3;
1929 if (IS_CHANNEL_INVALID (inChannel))
1933 ULWord loValue(0), hiValue(0);
1949 if (IS_CHANNEL_INVALID (channel))
1959 if (IS_CHANNEL_INVALID (inChannel))
1969 if (IsMultiRasterWidgetChannel(inChannel))
1971 if (IS_CHANNEL_INVALID(inChannel))
1981 if (IsMultiRasterWidgetChannel(inChannel))
1983 if (IS_CHANNEL_INVALID (inChannel))
1994 if (IS_CHANNEL_INVALID(inChannel))
1996 #if defined (NTV2_ALLOW_2MB_FRAMES)
2020 default:
return false;
2025 #endif // defined (NTV2_ALLOW_2MB_FRAMES)
2039 #if defined (NTV2_ALLOW_2MB_FRAMES)
2066 default:
return false;
2068 NTV2_ASSERT (NTV2_IS_8MB_OR_16MB_FRAMESIZE(outValue));
2072 #endif // defined (NTV2_ALLOW_2MB_FRAMES)
2079 if (IsMultiRasterWidgetChannel(inChannel))
2080 return SetMultiRasterBypassEnable(
false);
2088 {
UWord failures(0);
2090 if (!DisableChannel(*it))
2099 if (IsMultiRasterWidgetChannel(inChannel))
2100 return SetMultiRasterBypassEnable(
true);
2108 {
UWord failures(0);
2110 if (inChannels.find(chan) != inChannels.end())
2112 if (!EnableChannel(chan))
2115 else if (inDisableOthers)
2116 DisableChannel(chan);
2124 bool disabled (
false);
2125 if (IsMultiRasterWidgetChannel(inChannel))
2126 return GetMultiRasterBypassEnable(outEnabled);
2127 if (IS_CHANNEL_INVALID(inChannel))
2131 outEnabled = disabled ?
false :
true;
2139 bool enabled(
false);
2140 outChannels.clear();
2142 if (!IsChannelEnabled (ch, enabled))
2145 outChannels.insert(ch);
2153 bool enabled(
false);
2154 outChannels.clear();
2156 if (!IsChannelEnabled (ch, enabled))
2159 outChannels.insert(ch);
2163 #if !defined(NTV2_DEPRECATE_16_2)
2166 if (IS_CHANNEL_INVALID(inChannel))
2170 WaitForOutputVerticalInterrupt (inChannel);
2176 return !IS_CHANNEL_INVALID(inChannel)
2182 ULWord nextFrm(0), outFrm(0);
2183 return !IS_CHANNEL_INVALID(inCh)
2185 && GetOutputFrame(inCh, outFrm)
2186 && SetOutputFrame(inCh, nextFrm)
2189 #endif // !defined (NTV2_DEPRECATE_16_2)
2193 if (IsMultiRasterWidgetChannel(inChannel))
2195 if (IS_CHANNEL_INVALID(inChannel))
2202 if (IsMultiRasterWidgetChannel(inChannel))
2203 {outValue = 0;
return false;}
2204 if (IS_CHANNEL_INVALID(inChannel))
2211 if (IsMultiRasterWidgetChannel(inChannel))
2213 if (IS_CHANNEL_INVALID(inChannel))
2220 if (IsMultiRasterWidgetChannel(inChannel))
2222 if (IS_CHANNEL_INVALID(inChannel))
2246 ULWord programFlashValue;
2247 if(ReadFlashProgramControl(programFlashValue))
2249 if ((programFlashValue &
BIT(9)) ==
BIT(9))
2260 ULWord totalProgress = 0;
2282 progResults = devFlasher.
Program(
false);
2285 if (!progResults.empty())
2287 return progResults.empty();
2306 uint32_t regValue (0);
2310 outRevision = uint16_t((regValue & 0x0000FF00) >> 8);
2316 outYear = outMonth = outDay = 0;
2320 uint32_t regValue (0);
2324 const UWord yearBCD ((regValue & 0xFFFF0000) >> 16);
2325 const UWord monthBCD ((regValue & 0x0000FF00) >> 8);
2326 const UWord dayBCD (regValue & 0x000000FF);
2328 outYear = ((yearBCD & 0xF000) >> 12) * 1000
2329 + ((yearBCD & 0x0F00) >> 8) * 100
2330 + ((yearBCD & 0x00F0) >> 4) * 10
2331 + (yearBCD & 0x000F);
2333 outMonth = ((monthBCD & 0x00F0) >> 4) * 10 + (monthBCD & 0x000F);
2335 outDay = ((dayBCD & 0x00F0) >> 4) * 10 + (dayBCD & 0x000F);
2337 return outYear > 2010
2338 && outMonth > 0 && outMonth < 13
2339 && outDay > 0 && outDay < 32;
2345 outHours = outMinutes = outSeconds = 0;
2349 uint32_t regValue (0);
2353 const UWord hoursBCD ((regValue & 0x00FF0000) >> 16);
2354 const UWord minutesBCD ((regValue & 0x0000FF00) >> 8);
2355 const UWord secondsBCD (regValue & 0x000000FF);
2357 outHours = ((hoursBCD & 0x00F0) >> 4) * 10 + (hoursBCD & 0x000F);
2359 outMinutes = ((minutesBCD & 0x00F0) >> 4) * 10 + (minutesBCD & 0x000F);
2361 outSeconds = ((secondsBCD & 0x00F0) >> 4) * 10 + (secondsBCD & 0x000F);
2363 return outHours < 24 && outMinutes < 60 && outSeconds < 60;
2369 outDate = outTime = string();
2370 UWord yr(0), mo(0), dy(0), hr(0), mn(0), sec(0);
2371 if (!GetRunningFirmwareDate (yr, mo, dy))
2373 if (!GetRunningFirmwareTime (hr, mn, sec))
2376 ostringstream date, time;
2380 outDate = date.str();
2381 outTime = time.str();
2396 outUserID = regValue;
2409 if (GetPCIDeviceID (pciID))
2431 if (GetPCIDeviceID (pciID))
2452 if (IS_CHANNEL_INVALID(inFrameStore))
2454 if (IsMultiFormatActive())
2472 if (IS_CHANNEL_INVALID (inFrameStore))
2493 if (IS_CHANNEL_INVALID (inChannel))
2501 if (IS_CHANNEL_INVALID (inChannel))
2513 if (IS_CHANNEL_INVALID (inChannel))
2523 if (IS_CHANNEL_INVALID (inChannel))
2535 if (IS_CHANNEL_INVALID (inChannel))
2543 if (IS_CHANNEL_INVALID (inChannel))
2551 if (IS_CHANNEL_INVALID (inChannel))
2557 outIsBypassEnabled = regValue &
BIT(23);
2564 if (IS_CHANNEL_INVALID (inChannel))
2573 if (IS_CHANNEL_INVALID (inChannel))
2583 if (IS_CHANNEL_INVALID(inSDIOutput))
2592 if (IS_CHANNEL_INVALID(inSDIOutput))
2599 case 0: outSDIInput = inSDIOutput <
NTV2_CHANNEL5 ? 0 : 4;
break;
2600 case 2: outSDIInput = inSDIOutput <
NTV2_CHANNEL5 ? 1 : 5;
break;
2601 case 1: outSDIInput = inSDIOutput <
NTV2_CHANNEL5 ? 2 : 6;
break;
2602 case 3: outSDIInput = inSDIOutput <
NTV2_CHANNEL5 ? 3 : 7;
break;
2603 default:
return false;
2634 if (!SetVANCMode (inVancMode, *it))
2643 if (IsMultiRasterWidgetChannel(ch))
2645 if (IS_CHANNEL_INVALID(ch))
2652 GetStandard(st, ch);
2653 GetFrameGeometry(fg, ch);
2669 CVIDWARN(
"'taller' mode requested for 720p -- using 'tall' geometry instead");
2705 default:
return false;
2708 SetFrameGeometry (fg,
false, ch);
2721 { (
void) inStandard; (
void) inFrameGeometry;
2722 if (inTallerVANC && !inVANCenabled)
2730 bool isTall (
false);
2731 bool isTaller (
false);
2736 if (IsMultiRasterWidgetChannel(channel))
2740 if (IS_CHANNEL_INVALID (channel))
2743 GetStandard (standard, channel);
2744 GetFrameGeometry (frameGeometry, channel);
2789 #if defined (_DEBUG)
2792 default:
return false;
2802 if (IsMultiRasterWidgetChannel(inChannel))
2804 if (IS_CHANNEL_INVALID (inChannel))
2806 CVIDINFO(
"'" << GetDisplayName() <<
"' Ch" <<
DEC(inChannel+1) <<
": Vanc data shift " << (inValue ?
"enabled" :
"disabled"));
2815 if (!SetVANCShiftMode(*it, inMode))
2823 if (IsMultiRasterWidgetChannel(inChannel))
2825 if (IS_CHANNEL_INVALID (inChannel))
2833 if (IS_CHANNEL_INVALID (inChannel))
2842 if (IS_CHANNEL_INVALID (inChannel))
2846 outValue = value ?
true :
false;
2857 CVIDINFO(
"'" << GetDisplayName() <<
"' Mixer" <<
DEC(inWhichMixer+1) <<
": Vanc from " << (inFromForegroundSource ?
"FG" :
"BG"));
2870 outIsFromForegroundSource = value ?
true :
false;
2948 CVIDINFO(
"'" << GetDisplayName() <<
"' Mixer" <<
DEC(inWhichMixer+1) <<
": mixCoeff=" <<
xHEX0N(inMixCoefficient,8));
2955 outMixCoefficient = 0;
2967 bool syncFail (
false);
2970 outIsSyncOK = syncFail ?
false :
true;
2976 outIsEnabled =
false;
2991 outIsEnabled =
false;
3009 outYCbCrValue.
cb = outYCbCrValue.
y = outYCbCrValue.
cr = 0;
3015 outYCbCrValue.
cb = packedValue & 0x03FF;
3016 outYCbCrValue.
y = ((packedValue >> 10) & 0x03FF) + 0x0040;
3017 outYCbCrValue.
cr = (packedValue >> 20) & 0x03FF;
3027 if (ycbcrPixel.
y < 0x40)
3030 ycbcrPixel.
y -= 0x40;
3031 ycbcrPixel.
y &= 0x3FF;
3032 ycbcrPixel.
cb &= 0x3FF;
3033 ycbcrPixel.
cr &= 0x3FF;
3037 CVIDINFO(
"'" << GetDisplayName() <<
"' Mixer" <<
DEC(inWhichMixer+1) <<
": set to YCbCr=" <<
DEC(ycbcrPixel.
y)
3038 <<
"|" <<
DEC(ycbcrPixel.
cb) <<
"|" <<
DEC(ycbcrPixel.
cr) <<
":" <<
HEXN(ycbcrPixel.
y,3) <<
"|"
3039 <<
HEXN(ycbcrPixel.
cb,3) <<
"|" <<
HEXN(ycbcrPixel.
cr,3) <<
", write " <<
xHEX0N(packedValue,8)
3048 outIsSupported =
false;
3073 #if !defined(NTV2_DEPRECATE_16_0)
3079 if (IS_CHANNEL_INVALID(channel))
3084 if (ulFrame > GetNumFrameBuffers())
3089 if (!_pFrameBaseAddress)
3090 if (!MapFrameBuffers())
3092 *pBaseAddress = _pFrameBaseAddress + ((ulFrame * _ulFrameBufferSize) /
sizeof(
ULWord));
3096 if (!_pCh1FrameBaseAddress)
3097 if (!MapFrameBuffers())
3099 *pBaseAddress = (channel ==
NTV2_CHANNEL1) ? _pCh1FrameBaseAddress : _pCh2FrameBaseAddress;
3109 if (!_pFrameBaseAddress)
3110 if (!MapFrameBuffers())
3112 *pBaseAddress = _pFrameBaseAddress;
3121 if (!_pRegisterBaseAddress)
3122 if (!MapRegisters())
3125 if ((regNumber*4) >= _pRegisterBaseAddressLength)
3128 *pBaseAddress = _pRegisterBaseAddress + regNumber;
3136 if (!_pXena2FlashBaseAddress)
3137 if (!MapXena2Flash())
3139 *pXena2FlashAddress = _pXena2FlashBaseAddress;
3142 #endif // !defined(NTV2_DEPRECATE_16_0)
3151 outIsEnabled =
false;
3164 outIsEnabled =
false;
3173 if (IS_CHANNEL_INVALID(inChannel))
3180 if (IS_CHANNEL_INVALID(inChannel))
3202 if (IS_OUTPUT_SPIGOT_INVALID(inOutputSpigot))
3206 bool is2Kx1080(
false);
3246 && SetSDIOut2Kx1080Enable(
NTV2Channel(inOutputSpigot), is2Kx1080);
3253 if (!SetSDIOutputStandard(*it, inValue))
3260 if (IS_OUTPUT_SPIGOT_INVALID(inOutputSpigot))
3262 bool is2kx1080(
false);
3267 && GetSDIOut2Kx1080Enable(
NTV2Channel(inOutputSpigot), is2kx1080)
3268 && GetSDIOut6GEnable(
NTV2Channel(inOutputSpigot), is6G)
3269 && GetSDIOut12GEnable(
NTV2Channel(inOutputSpigot), is12G));
3293 if (IS_OUTPUT_SPIGOT_INVALID(inOutputSpigot))
3295 if (IsMultiFormatActive())
3326 if (IS_OUTPUT_SPIGOT_INVALID (inOutputSpigot))
3350 #if !defined(R2_DEPRECATE)
3362 #endif // R2_DEPRECATE
3388 ULWord vpidDS1(0), vpidDS2(0);
3390 if (IS_CHANNEL_INVALID(inChannel))
3393 bool isValidVPID (GetVPIDValidA(inChannel));
3396 ReadSDIInVPID(inChannel, vpidDS1, vpidDS2);
3398 isValidVPID = inputVPID.IsValid();
3403 bool isProgressiveTrans (isValidVPID ? inputVPID.
GetProgressiveTransport() : GetSDIInputIsProgressive(inChannel));
3405 bool isInput3G (
false);
3415 if (canDo3GIn || canDo12GIn)
3417 GetSDIInput3GPresent(isInput3G, inChannel);
3418 NTV2VideoFormat format = isValidVPID ? inputVPID.
GetVideoFormat() : GetNTV2VideoFormat(inputRate, inputGeometry, isProgressiveTrans, isInput3G, isProgressivePic);
3422 isProgressiveTrans = GetSDIInputIsProgressive(inChannel);
3423 isProgressivePic = inIsProgressivePicture;
3424 format = GetNTV2VideoFormat(inputRate, inputGeometry, isProgressiveTrans, isInput3G, isProgressivePic);
3428 bool is6G(
false), is12G(
false);
3429 GetSDIInput6GPresent(is6G, inChannel);
3430 GetSDIInput12GPresent(is12G, inChannel);
3449 return GetNTV2VideoFormat(inputRate, inputGeometry, isProgressiveTrans, isInput3G, isProgressivePic);
3460 if (GetHDMIInputStatus(status, inChannel))
3465 if(hdmiVersion == 1)
3482 else if(hdmiVersion > 1)
3484 bool squareDivision = hdmiVersion == 5 ?
false :
true;
3487 UByte inputGeometry = 0;
3490 format = GetNTV2VideoFormat (hdmiRate, hdmiStandard,
false, inputGeometry,
false, squareDivision);
3551 return GetNTV2VideoFormat (
NTV2FrameRate((status >> 16) & 0xF),
3552 ((status >> 20) & 0x7),
3553 (status &
BIT_23) ?
true :
false,
3560 if (IS_CHANNEL_INVALID (channel))
3563 ULWord rateLow (0), rateHigh (0);
3577 if (IS_CHANNEL_INVALID (channel))
3580 ULWord geometryLow (0), geometryHigh (0);
3594 if (IS_CHANNEL_INVALID (channel))
3597 ULWord isProgressive = 0;
3599 return isProgressive ?
true :
false;
3604 if (IS_CHANNEL_INVALID (channel))
3609 outValue =
static_cast <bool> (value);
3616 if (IS_CHANNEL_INVALID (channel))
3621 outValue =
static_cast <bool> (value);
3628 if (IS_CHANNEL_INVALID (channel))
3633 outValue =
static_cast <bool> (value);
3640 if (IS_CHANNEL_INVALID (channel))
3645 outValue =
static_cast <bool> (value);
3654 inEnable = !inEnable;
3664 outIsEnabled = !outIsEnabled;
3683 #if !defined(NTV2_DEPRECATE_16_3)
3693 #endif // !defined(NTV2_DEPRECATE_16_3)
3698 if (!ReadAnalogLTCInput(inLTCInput, result))
3700 outRP188Data = result;
3713 outRP188Data.
fDBB = 0;
3714 return ReadRegister(regLo, outRP188Data.
fLo) && ReadRegister(regHi, outRP188Data.
fHi);
3735 if (IS_CHANNEL_INVALID(inChannel))
3744 return WriteAnalogLTCOutput (inLTCOutput, rp188data);
3764 bool isMultiFormat(
false);
3765 if (!GetMultiFormatMode(isMultiFormat))
3780 if (IS_CHANNEL_INVALID (inChannel))
3782 bool isMultiFormat(
false);
3783 if (!GetMultiFormatMode(isMultiFormat))
3798 if (IS_CHANNEL_INVALID(inChannel))
3812 if (!SetSDITransmitEnable(*it, inEnable))
3819 if (IS_CHANNEL_INVALID(inChannel))
3824 {outIsEnabled =
true;
return true;}
3831 outXmitSDIs.clear();
3836 if (!biDirectionalSDI || (GetSDITransmitEnable(ch, isXmit) && isXmit))
3837 outXmitSDIs.insert(ch);
3844 if (IS_CHANNEL_INVALID (inChannel))
3851 if (IS_CHANNEL_INVALID (inChannel))
3858 if (IS_CHANNEL_INVALID(inChannel))
3865 if (IS_CHANNEL_INVALID(inChannel))
3873 if (IS_CHANNEL_INVALID(inChannel))
3880 if (IS_CHANNEL_INVALID(inChannel))
3887 if (IS_CHANNEL_INVALID(inChannel))
3897 if (IS_CHANNEL_INVALID(inChannel))
3899 bool is6G(
false), is12G(
false);
3904 outIsEnabled =
true;
3906 outIsEnabled =
false;
3912 if (IS_CHANNEL_INVALID(inChannel))
3922 if (IS_CHANNEL_INVALID(inChannel))
3935 ULWord b2(0), b1(0), b0(0);
3937 if (!ReadRegister (regNum, b2,
BIT(18), 18))
3939 if (!ReadRegister (regNum, b1,
BIT(28), 28))
3941 if (!ReadRegister (regNum, b0,
BIT(30), 30))
3956 ULWord value (inAudioSystem);
3978 size_t numFailures(0);
3979 for (NTV2ChannelSet::const_iterator it(inSDIOutputs.begin()); it != inSDIOutputs.end(); ++it)
3980 if (!(inDS2 ? SetSDIOutputDS2AudioSystem(*it, inAudioSystem) : SetSDIOutputAudioSystem(*it, inAudioSystem)))
3982 return numFailures == 0;
3992 ULWord b2(0), b1(0), b0(0);
3994 if (!ReadRegister (regNum, b2,
BIT(19), 19))
3996 if (!ReadRegister (regNum, b1,
BIT(29), 29))
3998 if (!ReadRegister (regNum, b0,
BIT(31), 31))
4013 ULWord value (inAudioSystem);
4123 outValue = statusBit ?
true :
false;
4161 outIsEnabled =
static_cast <bool> (tempVal);
4174 outIsEnabled =
static_cast <bool> (tempVal);
4187 outIsEnabled =
static_cast <bool> (tempVal);
4200 outIsEnabled =
static_cast <bool> (tempVal);
4209 if (IS_CHANNEL_INVALID(inChannel))
4213 return value ?
true :
false;
4220 if (IS_CHANNEL_INVALID(inChannel))
4224 return value ?
true :
false;
4231 if (IS_CHANNEL_INVALID(inChannel))
4242 if (IS_CHANNEL_INVALID(inChannel))
4253 if (IS_CHANNEL_INVALID(inChannel))
4264 if (IS_INPUT_SPIGOT_INVALID (inInputSpigot))
4267 ULWord regNum, mask, shift;
4268 switch (inInputSpigot)
4278 default:
return false;
4280 return WriteRegister(regNum, inEnable, mask, shift);
4287 if (!SetSDIInLevelBtoLevelAConversion(*it, inEnable))
4296 if (IS_INPUT_SPIGOT_INVALID (inInputSpigot))
4299 ULWord regNum, mask, shift;
4300 switch (inInputSpigot)
4310 default:
return false;
4319 if (IS_OUTPUT_SPIGOT_INVALID(inOutputSpigot))
4329 if (!SetSDIOutLevelAtoLevelBConversion(*it, inEnable))
4338 if (IS_OUTPUT_SPIGOT_INVALID (inOutputSpigot))
4343 outEnable =
static_cast <bool> (tempVal);
4351 if (IS_OUTPUT_SPIGOT_INVALID (inOutputSpigot))
4361 if (!SetSDIOutRGBLevelAConversion(*it, inEnable))
4370 if (IS_OUTPUT_SPIGOT_INVALID (inOutputSpigot))
4375 outEnable =
static_cast <bool> (tempVal);
4417 default:
return false;
4459 #if !defined(_DEBUG)
4483 default:
return false;
4518 const UWord dieTempRaw ((rawRegValue & 0x0000FFFF) >> 6);
4519 const double celsius (
double (dieTempRaw) * 503.975 / 1024.0 - 273.15);
4520 switch (inTempScale)
4526 default:
return false;
4540 const UWord coreVoltageRaw ((rawRegValue>>22) & 0x00003FF);
4541 const double coreVoltageFloat (
double(coreVoltageRaw)/ 1024.0 * 3.0);
4542 outVoltage = coreVoltageFloat;
4548 bool canReboot =
false;
4549 CanWarmBootFPGA(canReboot);
4555 #if defined(READREGMULTICHANGE)
4561 if (inRegisters.empty())
4565 if (NTV2Message(getRegsParams))
4567 if (!getRegsParams.GetRegisterValues(outValues))
4575 if (ReadRegister (*iter, tempVal))
4576 outValues[*iter] = tempVal;
4578 return outValues.size() == inRegisters.size();
4580 #endif // !defined(READREGMULTICHANGE)
4587 if (inRegWrites.empty())
4593 result = NTV2Message(setRegsParams);
4600 if (!WriteRegister(pRegInfos[ndx].registerNumber, pRegInfos[ndx].registerValue, pRegInfos[ndx].registerMask, pRegInfos[ndx].registerShift))
4606 if (!result)
CVIDFAIL(
"Failed: setRegsParams: " << setRegsParams);
4614 if (!NTV2Message(bankSelGetSetMsg))
4625 if (!NTV2Message(bankSelGetSetMsg))
4630 inOutRegInfo = bankSelGetSetMsg.
GetRegInfo();
4636 NTV2VirtualData virtualDataMsg (inTag, inVirtualData, inVirtualDataSize,
true);
4638 return NTV2Message(virtualDataMsg);
4643 NTV2VirtualData virtualDataMsg (inTag, outVirtualData, inVirtualDataSize,
false);
4645 return NTV2Message(virtualDataMsg);
4655 if (!NTV2Message(
reinterpret_cast<NTV2_HEADER*
>(&outStats)))
4659 for (
size_t sdi(0); sdi < numSDIInputs; sdi++)
4660 for (
ULWord reg(0); reg < 6; reg++)
4665 if (!ReadRegisters(sdiStatRegInfos))
4668 for (
size_t sdi(0); sdi < numSDIInputs; sdi++)
4671 size_t ndx(sdi*6 + 0);
4680 regInfo = sdiStatRegInfos.at(ndx+1);
4696 bool hasMultiRasterWidget(
false);
4699 && hasMultiRasterWidget;
4709 if (!HasMultiRasterWidget())
4711 NTV2ULWordVector regs; regs.push_back(0); regs.push_back(0); regs.push_back(0); regs.push_back(0);
4733 bool BOBConnected(
false);
4736 && (BOBConnected == 0);
4743 #pragma warning(default: 4800)
@ kRegMaskSDIIn43GbpsMode
@ kRegShiftInput1Geometry
@ kRegMaskSDIIn33GbpsSMPTELevelBMode
@ kRegShiftSDIIn612GbpsMode
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
@ kRegShiftSDIIn1LevelBtoLevelA
@ NTV2_REFERENCE_HDMI_INPUT2
Specifies the HDMI In 2 connector.
static const ULWord gSDIOutToRP188Input[]
@ kDeviceGetNumVideoInputs
The number of SDI video inputs on the device.
virtual bool SetSDIInLevelBtoLevelAConversion(const NTV2ChannelSet &inSDIInputs, const bool inEnable)
Enables or disables 3G level B to 3G level A conversion at the SDI input(s).
@ NTV2_FORMAT_3840x2160p_6000
@ kVRegProgressivePicture
@ NTV2_FORMAT_4096x2160psf_2500
virtual bool SetMixerFGMatteEnabled(const UWord inWhichMixer, const bool inIsEnabled)
Answers if the given mixer/keyer's foreground matte is enabled or not.
@ kRegMaskVidProcLimiting
@ kDeviceHasBiDirectionalSDI
True if device SDI connectors are bi-directional.
@ DEVICE_ID_KONALHIDVI
See KONA LHi.
@ kRegMaskLTCOnRefInSelect
virtual bool GetDieTemperature(double &outTemp, const NTV2DieTempScale inTempScale=NTV2DieTempScale_Celsius)
Reads the current die temperature of the device.
@ NTV2_FORMAT_4x1920x1080p_6000
static const ULWord gChannelToSDIInputProgressiveMask[]
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
@ NTV2_INPUTSOURCE_SDI4
Identifies the 4th SDI video input.
@ kK2RegMaskXena2BgVidProcInputControl
virtual bool GetSDIInput3GPresent(bool &outValue, const NTV2Channel channel)
@ NTV2_FG_4x2048x1080
4096x2160, for 4K, NTV2_VANCMODE_OFF
#define NTV2VANCModeFromBools(_tall_, _taller_)
@ kRegRXSDI3CRCErrorCount
@ kRegShiftInputStatusFPS
@ NTV2_FORMAT_3840x2160psf_2500
@ NTV2_FORMAT_1080psf_2398
virtual bool SetPCIAccessFrame(const NTV2Channel inChannel, const ULWord inValue, const bool inWaitForVBI=(!(0)))
virtual bool GetSDIRelayPosition(NTV2RelayState &outValue, const UWord inIndex0)
Answers if the bypass relays between connectors 1/2 or 3/4 are currently in bypass or routing the sig...
@ kDeviceGetNumMixers
The number of mixer/keyer widgets on the device.
virtual bool SetFrameBufferSize(const NTV2Framesize inSize)
Sets the device's intrinsic frame buffer size.
bool NTV2DeviceGetVideoFormatFromState_Ex2(NTV2VideoFormat *pOutValue, const NTV2FrameRate inFrameRate, const NTV2FrameGeometry inFrameGeometry, const NTV2Standard inStandard, const ULWord inIsSMPTE372Enabled, const bool inIsProgressivePicture, const bool inIsSquareDivision)
virtual bool GetSDIOut12GEnable(const NTV2Channel inChannel, bool &outIsEnabled)
@ kRegShiftSDIOutLevelAtoLevelB
@ kDeviceGetNumFrameStores
The number of FrameStores on the device.
@ NTV2_FORMAT_4096x2160p_2400
@ kRegShift4KDCPSFOutMode
@ kK2RegShiftSDI1Out_2Kx1080Mode
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
void Clear(void)
Resets the struct to its initialized state.
#define NTV2_IS_VANCMODE_TALLER(__v__)
ULWordSetConstIter NTV2RegNumSetConstIter
A const iterator that iterates over a set of distinct NTV2RegisterNumbers.
virtual bool GetRS422Parity(const NTV2Channel inSerialPort, NTV2_RS422_PARITY &outParity)
Answers with the current parity control for the specified RS422 serial port.
std::map< ULWord, ULWord > NTV2RegisterValueMap
A mapping of distinct NTV2RegisterNumbers to their corresponding ULWord values.
@ NTV2_RELAY_STATE_INVALID
@ kRegOutputTimingControlch6
@ kRegShiftRS422ParityDisable
@ kRegShiftInputStatusStd
virtual bool ReadRegisters(NTV2RegisterReads &inOutValues)
Reads the register(s) specified by the given NTV2RegInfo sequence.
virtual bool GetLTCInputPresent(bool &outIsPresent, const UWord inLTCInputNdx=0)
Answers whether or not a valid analog LTC signal is being applied to the device's analog LTC input co...
std::string NTV2FrameGeometryToString(const NTV2FrameGeometry inValue, const bool inForRetailDisplay=false)
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
@ DEVICE_ID_KONAHDMI
See KONA HDMI.
virtual bool SetStandard(NTV2Standard inValue, NTV2Channel inChannel=NTV2_CHANNEL1)
@ NTV2_INPUTSOURCE_SDI6
Identifies the 6th SDI video input.
#define NTV2_IS_VALID_NTV2FrameGeometry(__s__)
@ kRegShiftAnalogCompositeLocked
@ kRegShiftPCRReferenceEnable
@ NTV2_STANDARD_2Kx1080p
Identifies SMPTE HD 2K1080p.
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
@ NTV2_FORMAT_4x4096x2160p_4800
virtual bool GetRegisterWriteMode(NTV2RegisterWriteMode &outValue, const NTV2Channel inFrameStore=NTV2_CHANNEL1)
Answers with the FrameStore's current NTV2RegisterWriteMode setting, which determines when CNTV2Card:...
@ kRegMaskHDMIInV2VideoStd
static const ULWord gChannelToOutputTimingCtrlRegNum[]
virtual bool SetWarmBootFirmwareReload(bool enable)
virtual bool SetSDIOut2Kx1080Enable(const NTV2Channel inChannel, const bool inIsEnabled)
@ NTV2MIXERMODE_INVALID
Invalid/uninitialized.
virtual bool SetQuadFrameEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables quad-frame mode on the device.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ NTV2_FORMAT_4x4096x2160p_2398
virtual bool GetForce64(ULWord *force64)
static const ULWord gChannelToSDIOutControlRegNum[]
@ kRegRP188InOut2Bits0_31
virtual bool SetSDIOut3GEnable(const NTV2Channel inChannel, const bool inEnable)
@ kRegMaskSDIIn23GbpsMode
virtual bool KickSDIWatchdog(void)
Restarts the countdown timer to prevent the watchdog timer from timing out.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
@ kRegMaskInput2FrameRate
@ NTV2WidgetType_SDIIn12G
virtual bool Set4kSquaresEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 "2K quadrants" mode for the given FrameStore bank on the device....
@ kRegRP188InOut1Bits0_31
@ kNTV2EnumsID_VideoFormat
Identifies the NTV2VideoFormat enumerated type.
@ NTV2_FG_1920x1114
1920x1080, NTV2_VANCMODE_TALLER
@ kRegRP188InOut7Bits32_63
virtual bool SetSDIWatchdogEnable(const bool inEnable, const UWord inIndex0)
Sets the connector pair relays to be under watchdog timer control or manual control.
virtual bool SetFrameBufferQuarterSizeMode(NTV2Channel inChannel, NTV2QuarterSizeExpandMode inValue)
static const ULWord gChannelToSDIInputRateShift[]
virtual bool GetSDIOut3GEnable(const NTV2Channel inChannel, bool &outIsEnabled)
virtual bool GetRegisterBaseAddress(ULWord regNumber, ULWord **pRegAddress)
Declares device capability functions.
@ NTV2_FG_720x576
720x576, for PAL 625i, NTV2_VANCMODE_OFF
virtual bool GetVideoHOffset(int &outHOffset, const UWord inOutputSpigot=0)
Answers with the current horizontal timing offset, in pixels, for the given SDI output connector.
@ NTV2_FG_720x508
720x486, for NTSC 525i, NTV2_VANCMODE_TALL
virtual bool AcquireMailBoxLock(void)
virtual bool SetRP188Mode(const NTV2Channel inChannel, const NTV2_RP188Mode inMode)
Sets the current RP188 mode – NTV2_RP188_INPUT or NTV2_RP188_OUTPUT – for the given channel.
@ kK2RegShiftXena2VidProcMode
@ kDeviceHasXptConnectROM
True if device has a crosspoint connection ROM (New in SDK 17.0)
ULWord NTV2DeviceGetNumberFrameBuffers(NTV2DeviceID id, NTV2FrameGeometry fg, NTV2FrameBufferFormat fbf)
ULWord registerValue
My register value to use in a ReadRegister or WriteRegister call.
@ kK2RegMaskXena2VidProcMode
@ NTV2_FORMAT_4096x2160p_2500
@ kRegShiftSDIIn16GbpsMode
virtual bool GetPCIAccessFrame(const NTV2Channel inChannel, ULWord &outValue)
@ NTV2_FRAMERATE_1500
15 frames per second
@ kRegShiftSDIRelayPosition34
@ NTV2_FRAMERATE_6000
60 frames per second
@ kRegMaskSDIIn86GbpsMode
virtual bool GetMixerSyncStatus(const UWord inWhichMixer, bool &outIsSyncOK)
Returns the current sync state of the given mixer/keyer.
@ kRegOutputTimingControlch7
@ NTV2_INPUTSOURCE_SDI7
Identifies the 7th SDI video input.
@ kDeviceCanDoSDIErrorChecks
True if device can perform SDI error checking.
@ NTV2DieTempScale_Kelvin
NTV2FrameGeometry GetNTV2FrameGeometryFromVideoFormat(const NTV2VideoFormat inVideoFormat)
@ NTV2_REFERENCE_SFP2_PCR
Specifies the PCR source on SFP 2.
virtual bool GetMixerBGInputControl(const UWord inWhichMixer, NTV2MixerKeyerInputControl &outInputControl)
Returns the current background input control value for the given mixer/keyer.
@ NTV2_FORMAT_525psf_2997
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
@ NTV2_FG_4x4096x2160
8192x4320, for 8K, NTV2_VANCMODE_OFF
@ kRegShiftSDIOut12GbpsMode
NTV2FrameGeometry GetQuarterSizedGeometry(const NTV2FrameGeometry inGeometry)
virtual bool IsProgressiveStandard(bool &outIsProgressive, NTV2Channel inChannel=NTV2_CHANNEL1)
@ NTV2_FORMAT_4x2048x1080p_4795
virtual bool GetDieVoltage(double &outVoltage)
Reads the current "Vcc" voltage of the device.
@ kRegMaskSDIIn612GbpsMode
@ NTV2_REFERENCE_INPUT7
Specifies the SDI In 7 connector.
virtual bool GetStandard(NTV2Standard &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
#define NTV2_IS_2K1080_STANDARD(__s__)
virtual bool GetSDIOutputStandard(const UWord inOutputSpigot, NTV2Standard &outValue)
Answers with the current video standard of the given SDI output spigot.
@ kRegShiftInput2FrameRate
@ NTV2_FORMAT_4096x2160psf_2398
virtual bool SetFrameBufferOrientation(const NTV2Channel inChannel, const NTV2FBOrientation inValue)
Sets the frame buffer orientation for the given NTV2Channel.
@ kRegShiftLTCOnRefInSelect
virtual bool IsBreakoutBoardConnected(void)
#define NTV2_ASSERT(_expr_)
std::string Program(bool fullVerify=(0))
@ kRegMaskSDIIn43GbpsSMPTELevelBMode
@ kFS1RefMaskLTCOnRefInSelect
@ NTV2_STANDARD_1080
Identifies SMPTE HD 1080i or 1080psf.
@ NTV2_STANDARD_3840x2160p
Identifies Ultra-High-Definition (UHD)
@ kRegMaskSDIIn53GbpsSMPTELevelBMode
@ kRegShiftSDIIn312GbpsMode
virtual bool SetSmpte372(ULWord inValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables the device's SMPTE-372 (dual-link) mode (used for older 3G-levelB-capable devices...
@ NTV2_FRAMERATE_2997
Fractional rate of 30,000 frames per 1,001 seconds.
ULWord NTV2DeviceGetFrameBufferSize(NTV2DeviceID id, NTV2FrameGeometry fg, NTV2FrameBufferFormat fbf)
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
@ NTV2_VIDEOLIMITING_LEGALSDI
Identifies the "Legal SDI" mode (Ymax=0x3AC, Cmax=0x3C0)
virtual bool SetMixerCoefficient(const UWord inWhichMixer, const ULWord inMixCoefficient)
Sets the current mix coefficient of the given mixer/keyer.
virtual bool GetEnable4KPSFOutMode(bool &outIsEnabled)
virtual bool GetVideoVOffset(int &outVOffset, const UWord inOutputSpigot=0)
Answers with the current vertical timing offset, in lines, for the given SDI output connector.
@ kRegRP188InOut6Bits0_31
@ NTV2_STANDARD_625
Identifies SMPTE SD 625i.
virtual bool SetMixerBGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the background input control value for the given mixer/keyer.
virtual bool GetSDI1OutHTiming(ULWord *value)
@ NTV2_FORMAT_1080psf_2K_2398
@ kRegShiftSDIOut6GbpsMode
virtual bool IsXilinxProgrammed()
virtual bool SetRP188Data(const NTV2Channel inSDIOutput, const NTV2_RP188 &inRP188Data)
Writes the raw RP188 data into the DBB/Low/Hi registers for the given SDI output. These values are la...
virtual bool SetFrameRate(NTV2FrameRate inNewValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the AJA device's frame rate.
static const ULWord sSDIXmitEnableShifts[]
virtual bool GetQuadFrameEnable(bool &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the device's current quad-frame mode, whether it's enabled or not.
virtual bool GetMixerMode(const UWord inWhichMixer, NTV2MixerKeyerMode &outMode)
Returns the current operating mode of the given mixer/keyer.
virtual bool ReadAnalogLTCInput(const UWord inLTCInput, RP188_STRUCT &outRP188Data)
Reads the current contents of the device's analog LTC input registers.
@ NTV2_FG_2048x1080
2048x1080, for 2Kx1080p, NTV2_VANCMODE_OFF
@ NTV2_STANDARD_4096HFR
Identifies high frame-rate 4K.
static const ULWord gChannelToSmpte372RegisterNum[]
@ NTV2_STANDARD_4096x2160p
Identifies 4K.
virtual bool GetInputVideoSelect(NTV2InputVideoSelect &outInputSelect)
@ kRegMaskInput2GeometryHigh
@ NTV2_FORMAT_1080p_2K_4800_A
@ NTV2_FORMAT_4x2048x1080p_11988
static const ULWord gChlToRP188DBBRegNum[]
NTV2Buffer mOutBadRegIndexes
Array of UWords containing index numbers of the register writes that failed. The SDK owns this memory...
@ NTV2_FRAMERATE_12000
120 frames per second
@ kRegMaskSDIIn16GbpsMode
virtual bool GetVideoLimiting(NTV2VideoLimiting &outValue)
Answers with the current video range limiting mode for the device.
static const ULWord gChannelToSDIIn6GModeShift[]
@ kRegMaskAlphaFromInput2
@ kRegOutputTimingControlch3
virtual bool GetRP188BypassSource(const NTV2Channel inSDIOutput, UWord &outSDIInput)
For the given SDI output that's in RP188 bypass mode (E-E), answers with the SDI input that's current...
@ kRegShiftQuadQuadSquaresMode
virtual bool SetSDIOut6GEnable(const NTV2Channel inChannel, const bool inEnable)
virtual NTV2VideoFormat GetAnalogInputVideoFormat(void)
Returns the video format of the signal that is present on the device's analog video input.
@ kRegMaskRP188SourceSelect
Defines a number of handy byte-swapping macros.
virtual bool GetEnable4KDCYCC444Mode(bool &outIsEnabled)
@ kRegShiftSDIIn23GbpsMode
@ kRegMaskVidProcBGMatteEnable
@ NTV2_FORMAT_4x1920x1080psf_3000
@ NTV2_FRAMEBUFFER_ORIENTATION_NORMAL
@ kRegShiftSDIIn73GbpsSMPTELevelBMode
@ kDeviceCanDo4KVideo
True if the device can handle 4K/UHD video.
@ kRegRP188InOut4Bits0_31
@ kDeviceCanReportRunningFirmwareDate
True if device can report its running (and not necessarily installed) firmware date.
virtual bool GetRunningFirmwareDate(UWord &outYear, UWord &outMonth, UWord &outDay)
Reports the (local Pacific) build date of the currently-running firmware.
@ NTV2_FORMAT_1080p_2K_3000
@ NTV2_FORMAT_4096x2160psf_2997
@ kRegShiftSDIIn53GbpsMode
virtual bool SetProgressivePicture(ULWord value)
@ NTV2_FORMAT_4x2048x1080p_2997
virtual bool GetSDIWatchdogEnable(bool &outIsEnabled, const UWord inIndex0)
Answers true if the given connector pair relays are under watchdog timer control, or false if they're...
virtual bool DisableChannel(const NTV2Channel inChannel)
Disables the given FrameStore.
static const ULWord gChannelToSmpte372Masks[]
void Set(const ULWord inDBB=0xFFFFFFFF, const ULWord inLow=0xFFFFFFFF, const ULWord inHigh=0xFFFFFFFF)
Sets my fields from the given DBB, low and high ULWord components.
#define NTV2_IS_QUAD_QUAD_FRAME_GEOMETRY(geom)
@ kRegMaskSDIOut6GbpsMode
static const ULWord gChannelToSDIInput3GStatusRegNum[]
@ kDeviceCanDo3GLevelConversion
True if device can do 3G level B to 3G level A conversion.
@ kK2RegShiftPulldownMode
virtual bool GetSDIInput12GPresent(bool &outValue, const NTV2Channel channel)
virtual bool GetSDIOut6GEnable(const NTV2Channel inChannel, bool &outIsEnabled)
@ kRegShiftInput1FrameRateHigh
virtual bool GetFramePulseReference(NTV2ReferenceSource &outRefSource)
Answers with the device's current frame pulse reference source.
@ NTV2_FORMAT_4x4096x2160p_2500
@ kRegMaskInput2FrameRateHigh
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
@ kRegMaskSDIIn312GbpsMode
@ kRegMaskSDIIn63GbpsSMPTELevelBMode
#define DEC0N(__x__, __n__)
@ kRegShiftInput2FrameRateHigh
@ kRegRXSDI7CRCErrorCount
virtual bool GetInputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current input frame index number for the given FrameStore. This identifies which par...
virtual bool SetDualLinkInputEnable(const bool inIsEnabled)
@ kDeviceGetNumVideoOutputs
The number of SDI video outputs on the device.
@ NTV2_FORMAT_4x4096x2160p_4795
NTV2Standard GetQuarterSizedStandard(const NTV2Standard inGeometry)
@ NTV2_FORMAT_4x1920x1080p_2997
@ NTV2_FORMAT_4x1920x1080p_2500
virtual bool EnableRP188Bypass(const NTV2Channel inSDIOutput)
Configures the SDI output's embedder to embed SMPTE 12M timecode obtained from an SDI input,...
virtual bool SetOutputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the output frame index number for the given FrameStore. This identifies which frame in device SD...
@ NTV2_FORMAT_4x2048x1080p_4800
@ kRegRP188InOut3Bits0_31
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ NTV2_FORMAT_4x3840x2160p_2500
static bool WriteWatchdogControlBit(CNTV2Card &card, const ULWord inValue, const ULWord inMask, const ULWord inShift)
@ kDeviceCanDoFramePulseSelect
True if device supports frame pulse source independent of reference source.
@ kRegRXSDI8CRCErrorCount
#define NTV2_IS_QUAD_QUAD_STANDARD(__s__)
@ kDeviceCanDo12gRouting
True if device supports 12G routing crosspoints.
@ NTV2_REFERENCE_SFP2_PTP
Specifies the PTP source on SFP 2.
@ kRegShiftSDIRelayControl34
@ kRegShiftSDIIn3GbpsMode
@ NTV2_FG_720x486
720x486, for NTSC 525i and 525p60, NTV2_VANCMODE_OFF
virtual bool SetSDI1OutHTiming(ULWord value)
@ kRegShiftSDIInCRCErrorCountB
@ kRegShiftSDIIn53GbpsSMPTELevelBMode
virtual bool GetVANCMode(NTV2VANCMode &outVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Retrieves the current VANC mode for the given FrameStore.
@ kRegShiftVidProcRGBRange
virtual bool GetMultiFormatMode(bool &outIsEnabled)
Answers if the device is operating in multiple-format per channel (independent channel) mode or not....
@ kRegMaskQuadQuadSquaresMode
virtual bool GetSDIRelayManualControl(NTV2RelayState &outValue, const UWord inIndex0)
Answers if the bypass relays between connectors 1 and 2 would be in bypass or would route signals thr...
virtual bool SupportsP2PTransfer(void)
virtual bool GetLTCEmbeddedOutEnable(bool &outValue)
virtual bool GetProgressivePicture(void) const
#define NTV2_IS_QUAD_STANDARD(__s__)
virtual bool FlipFlopPage(const NTV2Channel inChannel)
NTV2ChannelSet::const_iterator NTV2ChannelSetConstIter
A handy const iterator into an NTV2ChannelSet.
virtual bool MixerHasRGBModeSupport(const UWord inWhichMixer, bool &outIsSupported)
Answers if the given mixer/keyer's has RGB mode support.
virtual bool SetFrameGeometry(NTV2FrameGeometry inGeometry, bool inIsRetail=(!(0)), NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the frame geometry of the given channel.
virtual bool WriteOutputTimingControl(const ULWord inValue, const UWord inOutputSpigot=0)
Adjusts the output timing for the given SDI output connector.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool ReadFlashProgramControl(ULWord &outValue)
NTV2RelayState
This enumerated data type identifies the two possible states of the bypass relays....
#define NTV2_IS_2K_1080_FRAME_GEOMETRY(geom)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
@ kRegMaskFramePulseEnable
@ kRegLTC2AnalogBits32_63
@ NTV2_FORMAT_4x3840x2160p_2398
@ kRegMaskSDIOut12GbpsMode
@ kRegShiftVidProcBGMatteEnable
@ kRegMaskSDIIn76GbpsMode
virtual bool GetSDITransmitEnable(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the specified SDI connector is currently acting as a transmitter (i....
@ kRegMaskDualLinkOutEnable
@ kRegShiftInput2GeometryHigh
@ kRegRP188InOut4Bits32_63
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
@ kRegShiftSDIIn66GbpsMode
virtual bool GetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat &outValue)
Returns the current frame buffer format for the given FrameStore on the AJA device.
@ kRegMaskSDIIn63GbpsMode
virtual bool GetEnable4KDCPSFInMode(bool &outIsEnabled)
@ kRegMaskInput1FrameRate
@ kRegShiftInput1FrameRate
@ kVRegSecondaryFormatSelect
@ NTV2_STANDARD_UNDEFINED
virtual bool GetXena2FlashBaseAddress(ULWord **pXena2FlashAddress)
@ kDeviceCanChangeFrameBufferSize
True if frame buffer sizes are not fixed.
@ kLHIRegShiftSDIOutSMPTELevelBMode
virtual bool GetSDIOut2Kx1080Enable(const NTV2Channel inChannel, bool &outIsEnabled)
@ kK2RegShiftSDIOutStandard
@ kFS1RefShiftLTCEmbeddedOutEnable
@ NTV2_FRAMERATE_2500
25 frames per second
@ kRegSysmonVccIntDieTemp
@ kVRegDefaultVideoOutMode
virtual bool GetQuadQuadSquaresEnable(bool &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the device's current "quad-quad-squares" frame mode, whether it's enabled or not.
@ NTV2_FORMAT_1080p_2K_4795_A
virtual bool Enable4KDCYCC444Mode(bool inEnable)
Sets 4K Down Convert YCC 444 mode.
@ NTV2_FORMAT_3840x2160p_2500
@ kRegShiftFrameRateHiBit
@ kRegShiftSDIIn46GbpsMode
@ kRegShiftFrameFormatHiBit
@ kRegMaskSDIIn112GbpsMode
NTV2FrameRate
Identifies a particular video frame rate.
@ NTV2_INPUTSOURCE_HDMI3
Identifies the 3rd HDMI video input.
@ kRegMaskVidProcRGBRange
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
NTV2FrameDimensions & Set(const ULWord inWidth, const ULWord inHeight)
Sets my dimension values.
Defines the KonaIP/IoIP registers.
@ kRegMaskVidProcVancSource
virtual bool WriteRegisters(const NTV2RegisterWrites &inRegWrites)
Writes the given sequence of NTV2RegInfo's.
virtual bool SetFrameBufferQuality(NTV2Channel inChannel, NTV2FrameBufferQuality inValue)
virtual bool GetMixerBGMatteEnabled(const UWord inWhichMixer, bool &outIsEnabled)
Answers if the given mixer/keyer's background matte is enabled or not.
static const ULWord gChannelToSDIInputProgressiveShift[]
@ NTV2_FORMAT_4x4096x2160p_2997
@ kDeviceHasSDIRelays
True if device has bypass relays on its SDI connectors.
NTV2RegisterNumberSet NTV2RegNumSet
A set of distinct NTV2RegisterNumbers.
@ NTV2_FRAMERATE_4800
48 frames per second
virtual bool GetRunningFirmwareUserID(ULWord &outUserID)
Reports the UserID number of the currently-running firmware.
@ kRegShiftAlphaFromInput2
NTV2MixerKeyerInputControl
These enum values identify the Mixer/Keyer foreground and background input control values.
@ NTV2_FORMAT_4096x2160psf_2400
bool IsProgressivePicture(const NTV2VideoFormat format)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
@ kRegRP188InOut6Bits32_63
This is used to atomically perform bank-selected register reads or writes.
@ kRegMaskSDIOutLevelAtoLevelB
@ kRegShiftSDIIn63GbpsSMPTELevelBMode
enum NTV2VPIDTransferCharacteristics NTV2HDRXferChars
NTV2_RS422_PARITY
These enum values identify RS-422 serial port parity configuration.
virtual bool GetSDIOutputDS2AudioSystem(const NTV2Channel inSDIOutputConnector, NTV2AudioSystem &outAudioSystem)
Answers with the device's Audio System that is currently providing audio for the given SDI output's a...
@ NTV2_MODE_INPUT
Input (capture) mode, which writes into device SDRAM.
@ kRegMaskSDIRelayPosition34
virtual bool GetSDILock(const NTV2Channel inChannel)
@ kRegShiftSDIIn5LevelBtoLevelA
bool NTV2DeviceNeedsRoutingSetup(const NTV2DeviceID inDeviceID)
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
@ kRegMaskSDIWatchdogEnable12
@ NTV2_FRAMERATE_2400
24 frames per second
@ kRegOutputTimingControlch5
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
virtual bool GetSDIInput6GPresent(bool &outValue, const NTV2Channel channel)
@ NTV2_FORMAT_4x2048x1080psf_2398
@ NTV2_INPUTSOURCE_ANALOG1
Identifies the first analog video input.
#define NTV2_IS_3Gb_FORMAT(__f__)
@ kRegMaskRS422ParityDisable
@ NTV2_FORMAT_4x2048x1080p_2398
virtual bool Enable4KDCPSFInMode(bool inEnable)
Sets 4K Down Convert PSF in mode.
static bool isEnabled(CNTV2Card &device, const NTV2Channel inChannel)
virtual bool Enable4KDCRGBMode(bool inEnable)
Sets 4K Down Convert RGB mode.
virtual bool GetPulldownMode(NTV2Channel inChannel, bool &outValue)
@ kRegShiftInput2Geometry
@ kRegMaskAnalogInputIntegerRate
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
@ kRegRXSDIFreeRunningClockHigh
@ NTV2_REFERENCE_HDMI_INPUT4
Specifies the HDMI In 4 connector.
virtual NTV2VideoFormat GetReferenceVideoFormat(void)
Returns the video format of the signal that is present on the device's reference input.
virtual bool GetAnalogLTCInClockChannel(const UWord inLTCInput, NTV2Channel &outChannel)
Answers with the (SDI) input channel that's providing the clock reference being used by the given dev...
virtual bool SetMixerBGMatteEnabled(const UWord inWhichMixer, const bool inIsEnabled)
Answers if the given mixer/keyer's background matte is enabled or not.
@ kRegMaskSDIRelayControl34
@ kRegShiftSDIInUnlockCount
ULWord fLo
| BG 4 | Secs10 | BG 3 | Secs 1 | BG 2 | Frms10 | BG 1 | Frms 1 |
#define HEXN(__x__, __n__)
NTV2Standard GetNTV2StandardFromScanGeometry(const UByte inScanGeometry, const bool inIsProgressiveTransport)
virtual bool GetMixerMatteColor(const UWord inWhichMixer, YCbCr10BitPixel &outYCbCrValue)
Answers with the given mixer/keyer's current matte color value being used.
virtual bool GetMixerFGInputControl(const UWord inWhichMixer, NTV2MixerKeyerInputControl &outInputControl)
Returns the current foreground input control value for the given mixer/keyer.
static const ULWord gChannelToSDIIn6GModeMask[]
virtual bool GetPossibleConnections(NTV2PossibleConnections &outConnections)
Answers with the implemented crosspoint connections (if known).
virtual bool GetVideoDACMode(NTV2VideoDACMode &outValue)
@ NTV2_FORMAT_1080psf_2K_2500
@ NTV2_REFERENCE_INPUT5
Specifies the SDI In 5 connector.
@ NTV2_FORMAT_4x2048x1080psf_2400
virtual bool SetVideoLimiting(const NTV2VideoLimiting inValue)
Sets the device's video range limiting mode.
@ kRegShiftSDIIn36GbpsMode
@ kVRegEveryFrameTaskFilter
virtual bool GetRP188Data(const NTV2Channel inSDIInput, NTV2_RP188 &outRP188Data)
Reads the raw RP188 data from the DBB/Low/Hi registers for the given SDI input. On newer devices with...
virtual bool SetRP188SourceFilter(const NTV2Channel inSDIInput, const UWord inFilterValue)
Sets the RP188 DBB filter for the given SDI input.
@ kDeviceCanDoProgrammableRS422
True if device has at least one RS-422 serial port, and it (they) can be programmed (for baud rate,...
ULWord registerNumber
My register number to use in a ReadRegister or WriteRegister call.
@ NTV2_FORMAT_1080p_5994_B
ULWord fHi
| BG 8 | Hrs 10 | BG 7 | Hrs 1 | BG 6 | Mins10 | BG 5 | Mins 1 |
virtual bool GetOutputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current output frame number for the given FrameStore (expressed as an NTV2Channel).
This is used by the CNTV2Card::ReadRegisters function.
@ kRegShiftSDIIn712GbpsMode
virtual bool GetActiveFrameDimensions(NTV2FrameDimensions &outFrameDimensions, const NTV2Channel inChannel=NTV2_CHANNEL1)
@ NTV2_FORMAT_1080psf_3000_2
@ NTV2_FORMAT_1080p_2K_2400
virtual bool GetTsiMuxSyncFail(bool &outSyncFailed, const NTV2Channel inWhichTsiMux)
Answers if the SMPTE 425 two-sample-interleave mux/demux input sync has failed or not.
@ kDeviceCanDoRGBLevelAConversion
True if the device can do RGB over 3G Level A.
@ kRegRP188InOut8Bits32_63
@ NTV2_FG_2048x1588
2048x1556, for 2Kx1556psf film format, NTV2_VANCMODE_TALL
@ NTV2_RS422_BAUD_RATE_9600
9600 baud
@ NTV2_FORMAT_4x2048x1080p_3000
NTV2Standard
Identifies a particular video standard.
@ kRegShiftSDIIn3LevelBtoLevelA
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
static const ULWord gIndexToVidProcControlRegNum[]
@ kRegRXSDI4CRCErrorCount
ULWord registerMask
My register mask value to use in a ReadRegister or WriteRegister call.
@ NTV2_FORMAT_4096x2160p_4795
virtual bool SetSecondaryVideoFormat(NTV2VideoFormat inFormat)
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual bool SupportsP2PTarget(void)
virtual bool GetRP188SourceFilter(const NTV2Channel inSDIInput, UWord &outFilterValue)
Returns the current RP188 filter setting for the given SDI input.
@ NTV2DieTempScale_Rankine
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
@ kRegMaskFrameFormatHiBit
virtual bool GetVideoFormat(NTV2VideoFormat &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
@ NTV2_FRAMERATE_2398
Fractional rate of 24,000 frames per 1,001 seconds.
NTV2FrameDimensions & Reset(void)
Sets both my width and height to zero (an invalid state).
@ kRegMaskSDIInVpidValidB
@ NTV2_FORMAT_4x4096x2160p_3000
virtual bool EnableChannels(const NTV2ChannelSet &inChannels, const bool inDisableOthers=(0))
Enables the given FrameStore(s).
@ kK2RegShiftXena2BgVidProcInputControl
@ NTV2_RS422_BAUD_RATE_19200
19200 baud
@ kDeviceGetNumVideoChannels
The number of video channels supported on the device.
@ kRegRP188InOut5Bits32_63
ULWord mInNumRegisters
The number of NTV2RegInfo's to be set.
virtual bool SetAnalogLTCOutClockChannel(const UWord inLTCOutput, const NTV2Channel inChannel)
Sets the (SDI) output channel that is to provide the clock reference to be used by the given analog L...
virtual bool GetSDIWatchdogTimeout(ULWord &outValue)
Answers with the amount of time that must elapse before the watchdog timer times out.
static const ULWord gChannelToSDIIn3GbModeMask[]
virtual bool GetQuadQuadFrameEnable(bool &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the device's current "quad-quad" frame mode, whether it's enabled or not.
@ kRegMaskFrameSizeSetBySW
@ kLHIRegMaskSDIOut3GbpsMode
virtual bool GetSDI2OutHTiming(ULWord *value)
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
@ kRegShiftVidProcRGBModeSupported
virtual bool ReleaseMailBoxLock(void)
@ kRegMaskSDIInTsiMuxSyncFail
@ NTV2_FORMAT_4x2048x1080psf_3000
virtual bool WriteVirtualData(const ULWord inTag, const void *inVirtualData, const ULWord inVirtualDataSize)
Writes the block of virtual data.
@ kRegMaskSDIIn73GbpsSMPTELevelBMode
virtual bool GetRunningFirmwareTime(UWord &outHours, UWord &outMinutes, UWord &outSeconds)
Reports the (local Pacific) build time of the currently-running firmware.
@ kRegShift4KDCYCC444Mode
NTV2ReferenceSource
These enum values identify a specific source for the device's (output) reference clock.
@ NTV2_FORMAT_1080p_2K_4795_B
virtual bool SetLEDState(ULWord inValue)
The four on-board LEDs can be set by writing 0-15.
@ kRegMaskSDIIn46GbpsMode
@ kRegShiftInput1GeometryHigh
A convenience class that simplifies encoding or decoding the 4-byte VPID payload that can be read or ...
@ NTV2_FG_720x612
720x576, for PAL 625i, NTV2_VANCMODE_TALLER
@ NTV2_FRAMERATE_1498
Fractional rate of 15,000 frames per 1,001 seconds.
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=(!(0)))
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
@ NTV2_FORMAT_4x3840x2160p_5994
virtual bool GetSDIOut3GbEnable(const NTV2Channel inChannel, bool &outIsEnabled)
@ NTV2_FORMAT_4096x2160p_2398
NTV2Mode
Used to identify the mode of a widget_framestore, or the direction of an AutoCirculate stream: either...
@ kRegRP188InOut1Bits32_63
@ kRegShiftSDIIn3GbpsSMPTELevelBMode
@ kDeviceCanDoLTCInOnRefPort
True if device can read LTC (Linear TimeCode) from its reference input.
virtual bool GetRunningFirmwarePackageRevision(ULWord &outRevision)
Reports the revision number of the currently-running firmware package. KonaIP style boards have a pac...
bool NTV2DeviceSoftwareCanChangeFrameBufferSize(const NTV2DeviceID inDeviceID)
static const ULWord gChannelToSmpte372Shifts[]
@ kRegShiftSDIIn7LevelBtoLevelA
Declares the CNTV2Card class.
virtual bool SetForce64(ULWord force64)
virtual bool SetSDIOutRGBLevelAConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables an RGB-over-3G-level-A conversion at the SDI output widget (assuming the AJA devi...
static const ULWord gChannelToSDIInputRateHighMask[]
@ kRegShiftSDIWatchdogStatus
std::string NTV2MixerInputControlToString(const NTV2MixerKeyerInputControl inValue, const bool inCompactDisplay=false)
@ NTV2_FORMAT_3840x2160psf_3000
virtual bool GetEncodeAsPSF(NTV2Channel inChannel, NTV2EncodeAsPSF &outValue)
static const ULWord gChannelToRXSDICRCErrorCountRegs[]
@ kRegShiftVidProcVANCShift
@ kDeviceGetHDMIVersion
The version number of the HDMI chipset on the device.
virtual bool SetAnalogLTCInClockChannel(const UWord inLTCInput, const NTV2Channel inChannel)
Sets the (SDI) input channel that is to provide the clock reference to be used by the given analog LT...
@ kRegMaskDualLinkInEnable
@ kRegShiftSDIRelayPosition12
@ NTV2_INPUTSOURCE_SDI5
Identifies the 5th SDI video input.
@ kRegShiftSDIIn412GbpsMode
virtual bool GetEnable4KDCRGBMode(bool &outIsEnabled)
virtual bool SetMixerMatteColor(const UWord inWhichMixer, const YCbCr10BitPixel inYCbCrValue)
Sets the matte color to use for the given mixer/keyer.
virtual bool GetAnalogLTCOutClockChannel(const UWord inLTCOutput, NTV2Channel &outChannel)
Answers with the (SDI) output channel that's providing the clock reference being used by the given de...
virtual bool SetRP188BypassSource(const NTV2Channel inSDIOutput, const UWord inSDIInput)
For the given SDI output that's in RP188 bypass mode (E-E), specifies the SDI input to be used as a t...
@ kRegMaskVidProcVANCShift
@ NTV2_FORMAT_4x2048x1080p_5000
std::string NTV2StandardToString(const NTV2Standard inValue, const bool inForRetailDisplay=false)
virtual bool GetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, NTV2AudioSystem &outAudioSystem)
Answers with the device's NTV2AudioSystem that is currently providing audio for the given SDI output'...
static NTV2VideoFormat GetNTV2VideoFormat(NTV2FrameRate frameRate, UByte inputGeometry, bool progressiveTransport, bool isThreeG, bool progressivePicture=(0))
@ kRegShiftSDIIn73GbpsMode
static const ULWord gChannelToSDIInputGeometryHighMask[]
virtual bool SetFramePulseReference(const NTV2ReferenceSource inRefSource)
Sets the device's frame pulse reference source. See Video Output Clocking & Synchronization for more ...
@ kRegMaskInput1FrameRateHigh
@ NTV2_FORMAT_4096x2160p_6000
@ NTV2_REFERENCE_INPUT6
Specifies the SDI In 6 connector.
@ NTV2_FG_720x514
720x486, for NTSC 525i and 525p60, NTV2_VANCMODE_TALLER
@ kRegMaskSDIIn23GbpsSMPTELevelBMode
static const ULWord gChannelToSDIInputRateHighShift[]
@ kRegShiftMRFrameLocation
virtual bool SetVideoHOffset(const int inHOffset, const UWord inOutputSpigot=0)
Adjusts the horizontal timing offset, in pixels, for the given SDI output connector.
@ kRegRXSDI1CRCErrorCount
virtual bool SetLTCInputEnable(const bool inEnable)
Enables or disables the ability for the device to read analog LTC on the reference input connector.
@ NTV2_THROUGH_DEVICE
Input & output routed through device.
All new NTV2 structs start with this common header.
@ kRegShiftVidProcLimiting
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
@ kRegShiftSDIIn76GbpsMode
virtual bool GetLEDState(ULWord &outValue)
Answers with the current state of the four on-board LEDs.
@ kRegMaskSmpte372Enable4
@ NTV2_STANDARD_1080p
Identifies SMPTE HD 1080p.
@ kRegRP188InOut7Bits0_31
@ kK2RegMaskXena2FgVidProcInputControl
NTV2RegWrites NTV2RegisterReads
std::string NTV2VANCModeToString(const NTV2VANCMode inValue, const bool inCompactDisplay=false)
@ kRegShiftChannelDisable
@ NTV2_FORMAT_4x1920x1080p_3000
#define NTV2_IS_VANCMODE_ON(__v__)
@ NTV2_FORMAT_4x3840x2160p_2997
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
@ kRegShiftInput2Progressive
@ NTV2MIXERINPUTCONTROL_INVALID
Declares numerous NTV2 utility functions.
static const ULWord gChannelToRP188ModeMasks[]
virtual bool SetSDI2OutHTiming(ULWord value)
@ kRegOutputTimingControlch8
@ kRegShiftSDIIn86GbpsMode
@ NTV2_FORMAT_1080p_5000_B
@ kRegMaskSDIIn812GbpsMode
NTV2Framesize
Kona2/Xena2 specific enums.
virtual NTV2VideoFormat GetAnalogCompositeInputVideoFormat(void)
Returns the video format of the signal that is present on the device's composite video input.
@ kRegMaskSDIIn3LevelBtoLevelA
NTV2FrameGeometry Get4xSizedGeometry(const NTV2FrameGeometry inGeometry)
@ NTV2_RS422_ODD_PARITY
Odd parity – this is the power-up default.
virtual bool GetDefaultVideoOutMode(ULWord &outMode)
virtual ULWord GetSDIUnlockCount(const NTV2Channel inChannel)
@ NTV2_FORMAT_4x3840x2160p_5000
@ kK2RegShiftXena2FgVidProcInputControl
@ NTV2_FORMAT_1080p_2K_5994_A
@ kRegMaskFramePulseRefSelect
@ kRegMaskSDIIn1LevelBtoLevelA
@ kRegShiftSDIIn812GbpsMode
virtual bool GetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, bool &outIsEnabled)
Answers with the device's current 3G level A to 3G level B conversion setting for the given SDI outpu...
@ kRegShiftSDIIn56GbpsMode
@ kRegMaskSDIWatchdogEnable34
@ kRegMaskSDIIn36GbpsMode
static const ULWord gChannelToPCIAccessFrameRegNum[]
I interrogate and control an AJA video/audio capture/playout device.
@ NTV2_FORMAT_4x2048x1080p_12000
NTV2RegInfo GetRegInfo(const UWord inIndex0=0) const
ProgramState programState
static const ULWord gChannelToSDIInputGeometryShift[]
@ kRegMaskSDIIn512GbpsMode
static const ULWord gIndexToVidProcMixCoeffRegNum[]
static const ULWord gChannelToRP188ModeShifts[]
virtual bool GetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode &outValue)
Retrieves the current "VANC Shift Mode" feature for the given channel.
@ NTV2DieTempScale_Celsius
@ NTV2_REFERENCE_HDMI_INPUT1
Specifies the HDMI In 1 connector.
std::vector< ULWord > NTV2ULWordVector
An ordered sequence of ULWords.
@ NTV2_FORMAT_625psf_2500
@ kRegMaskSDIRelayControl12
@ kRegShiftSDIIn23GbpsSMPTELevelBMode
@ NTV2_INPUTSOURCE_HDMI4
Identifies the 4th HDMI video input.
bool NTV2DeviceIsDirectAddressable(const NTV2DeviceID inDeviceID)
@ kRegMaskSDIIn5LevelBtoLevelA
@ kRegRXSDI6CRCErrorCount
@ kRegShiftSDIIn8LevelBtoLevelA
@ NTV2_FG_1280x720
1280x720, for 720p, NTV2_VANCMODE_OFF
@ kRegShiftSDIIn33GbpsSMPTELevelBMode
@ kK2RegMaskSDI1Out_2Kx1080Mode
@ NTV2_FORMAT_1080p_2K_2500
@ NTV2_FORMAT_4x1920x1080psf_2997
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
virtual bool GetSecondaryVideoFormat(NTV2VideoFormat &outFormat)
enum NTV2VPIDColorimetry NTV2HDRColorimetry
virtual bool SetTsiFrameEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 two-sample interleave (Tsi) frame mode on the device.
@ kRegOutputTimingControlch4
@ NTV2_FORMAT_1080p_6000_A
@ NTV2_FORMAT_3840x2160psf_2398
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
virtual bool GetReference(NTV2ReferenceSource &outRefSource)
Answers with the device's current clock reference source.
@ kRegSDIWatchdogControlStatus
@ kRegMaskSDIInCRCErrorCountA
@ kRegMaskSDIInVpidValidA
virtual NTV2FrameRate GetSDIInputRate(const NTV2Channel channel)
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
virtual bool SetDitherFor8BitInputs(const NTV2Channel inChannel, const ULWord inDither)
@ NTV2_STANDARD_TASKS
1: Standard/Retail: Device is completely controlled by AJA ControlPanel, service/daemon,...
@ kRegShiftAnalogInputIntegerRate
@ NTV2_FRAMERATE_11988
Fractional rate of 120,000 frames per 1,001 seconds.
static const ULWord gChannelToRXSDIStatusRegs[]
@ kNTV2EnumsID_WidgetID
Identifies the NTV2AudioWidgetID enumerated type.
NTV2Buffer mInRegInfos
Read-only array of NTV2RegInfo structs to be set. The SDK owns this memory.
@ kRegMaskSDIIn2LevelBtoLevelA
NTV2QuarterSizeExpandMode
@ NTV2_FORMAT_1080p_2K_2398
static const ULWord gChannelToSDIIn3GbModeShift[]
@ kRegMaskSDIIn33GbpsMode
@ NTV2_FORMAT_3840x2160p_2997
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
@ NTV2_FORMAT_4096x2160p_4800
static NTV2WidgetID WidgetIDFromTypeAndChannel(const NTV2WidgetType inWidgetType, const NTV2Channel inChannel)
@ kRegShiftFrameSizeSetBySW
NTV2VANCMode
These enum values identify the available VANC modes.
@ AJA_DebugUnit_UserGeneric
virtual bool SetSDIWatchdogTimeout(const ULWord inValue)
Specifies the amount of time that must elapse before the watchdog timer times out.
@ NTV2_STANDARD_4096i
Identifies 4K psf.
struct NTV2RegInfo NTV2RegInfo
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
virtual bool GetMode(const NTV2Channel inChannel, NTV2Mode &outValue)
Answers with the current NTV2Mode of the given FrameStore on the AJA device.
@ NTV2_FORMAT_3840x2160psf_2400
@ NTV2_FORMAT_1080psf_2K_2400
#define NTV2_IS_PSF_VIDEO_FORMAT(__f__)
#define NTV2_IS_VALID_CHANNEL(__x__)
virtual ULWord GetCRCErrorCountB(const NTV2Channel inChannel)
@ kRegShiftSDIIn512GbpsMode
std::string NTV2MixerKeyerModeToString(const NTV2MixerKeyerMode inValue, const bool inCompactDisplay=false)
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
@ NTV2_FORMAT_3840x2160p_5994
@ kRegShiftSDIIn26GbpsMode
virtual bool SetMixerFGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the foreground input control value for the given mixer/keyer.
@ NTV2_FORMAT_4x4096x2160p_2400
NTV2Buffer mInRegInfos
NTV2RegInfo array of registers be read/written. The SDK owns this memory.
@ kRegShiftVidProcVancSource
@ NTV2DieTempScale_Fahrenheit
virtual bool Get64BitAutodetect(ULWord *autodetect64)
@ NTV2_INPUTSOURCE_HDMI2
Identifies the 2nd HDMI video input.
@ NTV2_FORMAT_4x4096x2160p_6000
@ NTV2_FORMAT_3840x2160p_2398
@ NTV2_FG_1920x1112
1920x1080, for 1080i and 1080p, NTV2_VANCMODE_TALL
NTV2InputSource
Identifies a specific video input source.
@ kRegMaskSDIIn56GbpsMode
virtual bool GetEnableFramePulseReference(bool &outEnabled)
Answers whether or not the device's current frame pulse reference source is enabled....
virtual bool GetMultiRasterBypassEnable(bool &outEnabled)
virtual bool GetMixerVancOutputFromForeground(const UWord inWhichMixer, bool &outIsFromForegroundSource)
Answers whether or not the VANC source for the given mixer/keyer is currently the foreground video....
@ kRegMaskSDIInCRCErrorCountB
@ kFS1RefMaskLTCEmbeddedOutEnable
virtual bool GetFrameGeometry(NTV2FrameGeometry &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
@ kRegMaskSmpte372Enable6
@ kRegSDI5678Input3GStatus
static const ULWord gChannelToOutputFrameRegNum[]
virtual bool ReadOutputTimingControl(ULWord &outValue, const UWord inOutputSpigot=0)
Returns the current output timing control value for the given SDI output connector.
@ kRegOutputTimingControlch2
#define NTV2_IS_QUAD_QUAD_FORMAT(__f__)
@ NTV2_FORMAT_4x1920x1080psf_2398
@ kRegMaskInputStatusLock
virtual NTV2VideoFormat GetHDMIInputVideoFormat(NTV2Channel inHDMIInput=NTV2_CHANNEL1)
static const ULWord gChannelToSDIInputRateMask[]
@ NTV2_FORMAT_1080p_2K_6000_A
@ kK2RegShiftVideoDACMode
virtual bool SetRS422Parity(const NTV2Channel inSerialPort, const NTV2_RS422_PARITY inParity)
Sets the parity control on the specified RS422 serial port.
@ NTV2_FG_720x598
720x576, for PAL 625i, NTV2_VANCMODE_TALL
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
static const ULWord gChannelToGlobalControlRegNum[]
@ kRegShiftSmpte372Enable6
virtual bool SetVideoDACMode(NTV2VideoDACMode inValue)
Declares the CNTV2VPID class. See SMPTE 352 standard for details.
@ kRegRXSDI5CRCErrorCount
virtual bool EnableFramePulseReference(const bool inEnable)
Enables the device's frame pulse reference select.
@ kRegShiftIndependentMode
virtual bool GetSDITRSError(const NTV2Channel inChannel)
@ kRegShiftSDIRelayControl12
@ kRegRXSDIFreeRunningClockLow
virtual bool GetDualLinkInputEnable(bool &outIsEnabled)
@ kRegRP188InOut2Bits32_63
virtual bool GetDualLinkOutputEnable(bool &outIsEnabled)
virtual bool SetMixerRGBRange(const UWord inWhichMixer, const NTV2MixerRGBRange inRGBRange)
Sets the RGB range for the given mixer/keyer.
@ kRegMaskSDIIn8LevelBtoLevelA
#define NTV2_IS_QUAD_FRAME_GEOMETRY(geom)
@ NTV2_RS422_EVEN_PARITY
Even parity.
@ NTV2_FORMAT_4096x2160p_3000
@ kK2RegMaskSDIOutStandard
virtual bool SetSDIOutputStandard(const UWord inOutputSpigot, const NTV2Standard inValue)
Sets the SDI output spigot's video standard.
NTV2VideoLimiting
These enum values identify the available SDI video output limiting modes.
static const ULWord gChannelToSDIInputGeometryMask[]
@ NTV2_FORMAT_4x1920x1080psf_2500
This is used by the CNTV2Card::ReadSDIStatistics function.
@ NTV2_FRAMERATE_5994
Fractional rate of 60,000 frames per 1,001 seconds.
@ kRegShiftFramePulseRefSelect
virtual bool DisableChannels(const NTV2ChannelSet &inChannels)
Disables the given FrameStore(s).
@ kRegMaskIndependentMode
@ DEVICE_ID_CORVID24
See Corvid 24.
@ NTV2_FORMAT_4x3840x2160p_2400
virtual bool Enable4KPSFOutMode(bool inEnable)
Sets 4K Down Convert PSF out Mode.
std::vector< NTV2RegInfo > NTV2RegisterWrites
@ NTV2_STANDARD_525
Identifies SMPTE SD 525i.
static const ULWord gChannelToSDIInputGeometryHighShift[]
@ kRegShiftHDMIInV2VideoStd
static const ULWord gChannelToInputFrameRegNum[]
virtual bool SetSDIOut12GEnable(const NTV2Channel inChannel, const bool inEnable)
virtual bool BankSelectReadRegister(const NTV2RegInfo &inBankSelect, NTV2RegInfo &inOutRegInfo)
Reads the given set of registers from the bank specified in position 0.
virtual bool SetMixerVancOutputFromForeground(const UWord inWhichMixer, const bool inFromForegroundSource=(!(0)))
Sets the VANC source for the given mixer/keyer to the foreground video (or not). See the SDI Ancillar...
@ kRegMaskInput1Progressive
virtual ULWord GetCRCErrorCountA(const NTV2Channel inChannel)
virtual bool Get4kSquaresEnable(bool &outIsEnabled, const NTV2Channel inChannel)
Answers whether the FrameStore bank's current SMPTE 425 "4K squares" (i.e., "2K quadrants") mode is e...
virtual bool GetFrameBufferQuarterSizeMode(NTV2Channel inChannel, NTV2QuarterSizeExpandMode &outValue)
Declares the CNTV2KonaFlashProgram class.
@ NTV2_FORMAT_4x2048x1080p_2500
@ kLHIRegMaskSDIOutSMPTELevelBMode
bool IsValid(void) const
Answers true if I'm valid, or false if I'm not valid.
@ NTV2_FORMAT_4x1920x1080p_5994
@ NTV2_FORMAT_4x4096x2160p_5000
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
virtual bool GetProgressivePicture(ULWord &outValue)
NTV2RegisterWriteMode
These values are used to determine when certain register writes actually take effect....
virtual bool GetBaseAddress(NTV2Channel channel, ULWord **pBaseAddress)
virtual bool GetAnalogOutHTiming(ULWord &outValue)
@ kRegShiftAnalogCompositeFormat625
@ NTV2_INPUTSOURCE_SDI8
Identifies the 8th SDI video input.
@ NTV2_FORMAT_4x3840x2160p_6000
#define NTV2_IS_VALID_VIDEOLIMITING(__v__)
@ NTV2_STANDARD_3840HFR
Identifies high frame-rate UHD.
static const ULWord gChannelToControlRegNum[]
virtual NTV2VideoFormat GetVideoFormat(void) const
virtual bool GetProgramStatus(SSC_GET_FIRMWARE_PROGRESS_STRUCT *statusStruct)
virtual bool GetSDIInLevelBtoLevelAConversion(const UWord inInputSpigot, bool &outIsEnabled)
Answers with the device's current 3G level B to 3G level A conversion setting for the given SDI input...
@ NTV2_INPUTSOURCE_SDI2
Identifies the 2nd SDI video input.
static const ULWord gChannelToSDIIn3GModeMask[]
@ kRegMaskSDIIn53GbpsMode
This struct replaces the old RP188_STRUCT.
bool NTV2DeviceCanDoBreakoutBoard(const NTV2DeviceID inDeviceID)
virtual bool SetLTCEmbeddedOutEnable(const bool inNewValue)
@ kRegRXSDI2CRCErrorCount
virtual bool SetMixerMode(const UWord inWhichMixer, const NTV2MixerKeyerMode inMode)
Sets the mode for the given mixer/keyer.
@ NTV2_STANDARD_2Kx1080i
Identifies SMPTE HD 2K1080psf.
@ NTV2_FRAMERATE_UNKNOWN
Represents an unknown or invalid frame rate.
virtual bool GetDisabledChannels(NTV2ChannelSet &outChannels)
Answers with the set of channels that are currently disabled.
@ NTV2_RS422_BAUD_RATE_38400
38400 baud – this is the power-up default
@ kRegMaskSDIWatchdogStatus
@ NTV2_FORMAT_1080p_5000_A
@ NTV2_FORMAT_4x2048x1080p_6000
static const ULWord gChannelToSDIIn12GModeShift[]
@ kRegMaskDitherOn8BitInput
@ kDeviceCanDo8KVideo
True if device supports 8K video formats.
@ NTV2_RS422_BAUD_RATE_INVALID
@ NTV2_FORMAT_3840x2160psf_2997
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ kRegShiftSDIIn33GbpsMode
@ NTV2_STANDARD_8192
Identifies 8K.
static const ULWord gMatteColorRegs[]
virtual bool SetSDIRelayManualControl(const NTV2RelayState inValue, const UWord inIndex0)
Sets the state of the given connector pair relays to NTV2_DEVICE_BYPASSED (or NTV2_THROUGH_DEVICE if ...
#define AJA_sERROR(_index_, _expr_)
@ NTV2_FORMAT_4096x2160p_5000
virtual bool SetRS422BaudRate(const NTV2Channel inSerialPort, const NTV2_RS422_BAUD_RATE inBaudRate)
Sets the baud rate of the specified RS422 serial port.
@ kRegShiftSDIIn2LevelBtoLevelA
virtual bool GetProgressiveTransport(void) const
virtual bool SetSDIOut3GbEnable(const NTV2Channel inChannel, const bool inEnable)
virtual bool SetMultiRasterBypassEnable(const bool inEnable)
@ NTV2_FORMAT_4x1920x1080p_5000
std::multimap< NTV2InputXptID, NTV2OutputXptID > NTV2PossibleConnections
A map of zero or more one-to-many possible NTV2InputXptID to NTV2OutputXptID connections.
@ NTV2_DEVICE_BYPASSED
Input & output directly connected.
@ kRegMaskFrameOrientation
@ NTV2_STANDARD_720
Identifies SMPTE HD 720p.
@ NTV2_RS422_NO_PARITY
No parity.
@ NTV2_FORMAT_4x1920x1080p_2398
@ NTV2_FG_2048x1112
2048x1080, for 2Kx1080p, NTV2_VANCMODE_TALL
@ kRegMaskSDIIn73GbpsMode
virtual bool AbortMailBoxLock(void)
virtual bool ReadVirtualData(const ULWord inTag, void *outVirtualData, const ULWord inVirtualDataSize)
Reads the block of virtual data for a specific tag.
virtual bool SetSDIOutputDS2AudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the Audio System that will supply audio for the given SDI output's audio embedder for Data Strea...
NTV2FrameGeometry
Identifies a particular video frame geometry.
virtual bool IsChannelEnabled(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the given FrameStore is enabled.
@ kRegShiftVidProcSyncFail
@ kDeviceCanDo425Mux
True if the device supports SMPTE 425 mux control.
@ NTV2_FORMAT_1080p_6000_B
virtual bool WriteAnalogLTCOutput(const UWord inLTCOutput, const RP188_STRUCT &inRP188Data)
Writes the given timecode to the specified analog LTC output register.
virtual ULWord GetFrameBufferSize(void) const
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
NTV2VideoFormat GetQuadSizedVideoFormat(const NTV2VideoFormat inVideoFormat, const bool isSquareDivision=true)
@ NTV2_FORMAT_4096x2160p_2997
virtual bool GetMixerRGBRange(const UWord inWhichMixer, NTV2MixerRGBRange &outRGBRange)
Answers with the given mixer/keyer's current RGB Range.
@ kFS1RefShiftLTCOnRefInSelect
static const ULWord gChannelToSDIIn3GModeShift[]
@ kRegMaskPCRReferenceEnable
virtual bool SetInputVideoSelect(NTV2InputVideoSelect inInputSelect)
This is used by the CNTV2Card::WriteRegisters function.
@ kRegShiftSDIIn4LevelBtoLevelA
#define NTV2_IS_QUAD_FRAME_FORMAT(__f__)
virtual bool IsMultiRasterWidgetChannel(const NTV2Channel inChannel)
@ NTV2_FORMAT_1080p_5994_A
@ NTV2_FG_2048x1114
2048x1080, NTV2_VANCMODE_TALLER
std::string NTV2VideoLimitingToString(const NTV2VideoLimiting inValue, const bool inCompactDisplay=false)
virtual bool SetDefaultVideoOutMode(ULWord mode)
virtual bool GetMixerFGMatteEnabled(const UWord inWhichMixer, bool &outIsEnabled)
Answers if the given mixer/keyer's foreground matte is enabled or not.
ULWord mOutNumFailures
The number of registers unsuccessfully written.
#define NTV2_IS_VALID_NTV2FrameRate(__r__)
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
@ kRegShiftSDIIn43GbpsMode
virtual bool IsBufferSizeSetBySW(void)
NTV2Standard Get4xSizedStandard(const NTV2Standard inGeometry, const bool bIs4k=false)
virtual bool GetPCIDeviceID(ULWord &outPCIDeviceID)
Answers with my PCI device ID.
static const ULWord gChlToRP188Bits031RegNum[]
@ NTV2_FORMAT_4x2048x1080p_5994
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
virtual bool SetInputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the input frame index number for the given FrameStore. This identifies which frame in device SDR...
virtual bool SetEnableVANCData(const bool inVANCenabled, const bool inTallerVANC, const NTV2Standard inStandard, const NTV2FrameGeometry inGeometry, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual CNTV2VPID & SetVPID(const ULWord inData)
@ kRegShiftSDIWatchdogEnable12
@ NTV2_FG_4x1920x1080
3840x2160, for UHD, NTV2_VANCMODE_OFF
virtual bool IsRP188BypassEnabled(const NTV2Channel inSDIOutput, bool &outIsBypassEnabled)
Answers if the SDI output's RP-188 bypass mode is enabled or not.
virtual bool DisableRP188Bypass(const NTV2Channel inSDIOutput)
Configures the SDI output's embedder to embed SMPTE 12M timecode specified in calls to CNTV2Card::Set...
enum NTV2VPIDLuminance NTV2HDRLuminance
@ NTV2_FORMAT_4x3840x2160p_3000
This is used to perform virtual data reads or writes.
@ kRegShiftSmpte372Enable4
bool NTV2DeviceCanDoHDMIMultiView(const NTV2DeviceID inDeviceID)
virtual bool SetQuadQuadFrameEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables "quad-quad" 8K frame mode on the device.
@ kRegMaskAnalogCompositeLocked
@ kRegMaskSDIIn26GbpsMode
@ kRegMaskSmpte372Enable8
@ kRegMaskInput1GeometryHigh
#define NTV2_IS_VANCMODE_TALL(__v__)
@ kDeviceGetTotalNumAudioSystems
The total number of audio systems on the device, including host audio and mixer audio systems,...
@ kRegRP188InOut5Bits0_31
@ kRegMaskInput2Progressive
@ kRegShiftSDIInTsiMuxSyncFail
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual bool SetQuadQuadSquaresEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables quad-quad-frame (8K) squares mode on the device.
@ kRegMaskVidProcSyncFail
virtual bool ProgramMainFlash(const std::string &inFileName, const bool bInForceUpdate=(0), const bool bInQuiet=(0))
ULWord registerShift
My register shift value to use in a ReadRegister or WriteRegister call.
NTV2RegWrites NTV2RegReads
An ordered sequence of zero or more NTV2RegInfo structs intended for ReadRegister.
@ kRegShift2MFrameSupport
@ NTV2_FORMAT_3840x2160p_5000
virtual bool SetAnalogOutHTiming(ULWord inValue)
@ NTV2_FORMAT_4096x2160psf_3000
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
@ kRegMaskSDIIn712GbpsMode
@ kRegMaskSDIIn7LevelBtoLevelA
#define kRegSarekPackageVersion
virtual bool IsSDStandard(bool &outIsStandardDef, NTV2Channel inChannel=NTV2_CHANNEL1)
@ NTV2_FG_1280x740
1280x720, for 720p, NTV2_VANCMODE_TALL
virtual bool GetDitherFor8BitInputs(const NTV2Channel inChannel, ULWord &outDither)
static bool MakeRouteROMRegisters(NTV2RegReads &outROMRegisters)
Prepares an initialized, zeroed NTV2RegReads that's prepared to read all ROM registers from a device.
@ NTV2_FORMAT_1080p_2K_5000_A
@ NTV2_FRAMERATE_5000
50 frames per second
virtual bool GetNumberActiveLines(ULWord &outNumActiveLines)
virtual bool GetSDIInputIsProgressive(const NTV2Channel channel)
@ kRegShiftSDIWatchdogEnable34
@ kRegMaskSDIIn212GbpsMode
@ kRegMaskSDIIn4LevelBtoLevelA
virtual bool GetLTCInputEnable(bool &outIsEnabled)
Answers true if the device is currently configured to read analog LTC from the reference input connec...
@ NTV2_FORMAT_4x2048x1080psf_2500
@ NTV2_INPUTSOURCE_SDI3
Identifies the 3rd SDI video input.
virtual bool GetRS422BaudRate(const NTV2Channel inSerialPort, NTV2_RS422_BAUD_RATE &outBaudRate)
Answers with the current baud rate of the specified RS422 serial port.
@ NTV2_FG_2048x1556
2048x1556, for 2Kx1556psf film format, NTV2_VANCMODE_OFF
@ kRegRP188InOut3Bits32_63
virtual bool GetRP188Mode(const NTV2Channel inChannel, NTV2_RP188Mode &outMode)
Returns the current RP188 mode – NTV2_RP188_INPUT or NTV2_RP188_OUTPUT – for the given channel.
@ NTV2_REFERENCE_EXTERNAL
Specifies the External Reference connector.
@ NTV2_FRAMERATE_4795
Fractional rate of 48,000 frames per 1,001 seconds.
#define NTV2_IS_TSI_FORMAT(__f__)
virtual bool SetPulldownMode(NTV2Channel inChannel, bool inValue)
@ kRegMaskMRFrameLocation
@ NTV2_FRAMERATE_3000
30 frames per second
virtual bool GetSDIWatchdogStatus(NTV2RelayState &outValue)
Answers if the watchdog timer would put the SDI relays into NTV2_DEVICE_BYPASSED or NTV2_THROUGH_DEVI...
@ kLHIRegShiftSDIOut3GbpsMode
@ kRegMaskSDIRelayPosition12
@ kRegShiftSDIIn43GbpsSMPTELevelBMode
virtual NTV2VideoFormat GetSDIInputVideoFormat(NTV2Channel inChannel, bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given SDI input source.
static const ULWord sSDIXmitEnableMasks[]
#define xHEX0N(__x__, __n__)
virtual bool GetSDIOutRGBLevelAConversion(const UWord inOutputSpigot, bool &outIsEnabled)
Answers with the device's current RGB-over-3G-level-A conversion at the given SDI output spigot (assu...
@ kRegOutputTimingControl
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
@ kRegShiftDitherOn8BitInput
@ kRegShiftSDIIn83GbpsSMPTELevelBMode
virtual bool GetNominalMinMaxHV(int &outNominalH, int &outMinH, int &outMaxH, int &outNominalV, int &outMinV, int &outMaxV)
virtual bool SetDualLinkOutputEnable(const bool inIsEnabled)
virtual bool GetRunningFirmwareRevision(UWord &outRevision)
Reports the revision number of the currently-running firmware.
@ NTV2_FG_4x3840x2160
7680x4320, for UHD2, NTV2_VANCMODE_OFF
virtual bool SetAlphaFromInput2Bit(ULWord inValue)
@ kRegShiftSDIIn63GbpsMode
@ NTV2_FORMAT_1080psf_2997_2
@ NTV2_RS422_PARITY_INVALID
bool SetBitFile(const std::string &inBitfileName, std::ostream &outMsgs, const FlashBlockID blockNumber=AUTO_FLASHBLOCK)
virtual bool HasMultiRasterWidget(void)
@ NTV2_REFERENCE_INPUT8
Specifies the SDI In 8 connector.
@ kRegMaskSDIIn83GbpsMode
virtual bool GetEnabledChannels(NTV2ChannelSet &outChannels)
Answers with the set of channels that are currently enabled.
#define NTV2_IS_VALID_VANCMODE(__v__)
@ NTV2_FORMAT_4x4096x2160p_5994
@ NTV2_FORMAT_4x2048x1080p_2400
@ kRegMaskVidProcRGBModeSupported
virtual bool SetRegisterWriteMode(const NTV2RegisterWriteMode inValue, const NTV2Channel inFrameStore=NTV2_CHANNEL1)
Sets the FrameStore's NTV2RegisterWriteMode, which determines when CNTV2Card::SetInputFrame or CNTV2C...
NTV2MixerKeyerMode
These enum values identify the mixer mode.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
virtual NTV2FrameGeometry GetSDIInputGeometry(const NTV2Channel channel)
@ NTV2_FORMAT_1080p_2K_4800_B
@ kRegShiftSDIIn112GbpsMode
static const ULWord gChlToRP188Bits3263RegNum[]
@ NTV2_REFERENCE_HDMI_INPUT3
Specifies the HDMI In 3 connector.
virtual bool IsStandardMultiLink4320(void) const
@ NTV2_STANDARD_7680
Identifies UHD2.
virtual bool GetSmpte372(ULWord &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the device's current SMPTE-372 (dual-link) mode, whether it's enabled or not.
@ NTV2_FORMAT_4x1920x1080p_2400
Declares the CNTV2RegisterExpert class.
@ kRegMaskSDIInUnlockCount
@ NTV2_FORMAT_4x1920x1080psf_2400
virtual bool GetTsiFrameEnable(bool &outIsEnabled, const NTV2Channel inChannel)
Returns the current SMPTE 425 two-sample-interleave frame mode on the device, whether it's enabled or...
@ kRegMaskSDIIn3GbpsSMPTELevelBMode
@ NTV2_FG_1920x1080
1920x1080, for 1080i and 1080p, NTV2_VANCMODE_OFF
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
static const ULWord gChannelToRS422ControlRegNum[]
NTV2_RS422_BAUD_RATE
These enum values identify RS-422 serial port baud rate configuration.
@ kRegShiftSmpte372Enable8
@ NTV2_FORMAT_1080psf_2500_2
@ kDeviceGetNumSerialPorts
The number of RS-422 serial ports on the device.
static const ULWord gChannelToSDIInputStatusRegNum[]
@ kRegShiftQuarterSizeMode
static bool GetPossibleConnections(const NTV2RegReads &inROMRegisters, NTV2PossibleConnections &outConnections)
Answers with the implemented crosspoint connections as obtained from the given ROM registers.
virtual bool SetEncodeAsPSF(NTV2Channel inChannel, NTV2EncodeAsPSF inValue)
@ DEVICE_ID_KONALHI
See KONA LHi.
virtual bool ReadSDIStatistics(NTV2SDIInStatistics &outStats)
For devices that support it (see the NTV2DeviceCanDoSDIErrorChecks function in "ntv2devicefeatures....
@ NTV2_FORMAT_3840x2160p_2400
virtual bool GetSDIInput3GbPresent(bool &outValue, const NTV2Channel channel)
@ kRegShiftSDIIn6LevelBtoLevelA
@ kRegRP188InOut8Bits0_31
virtual bool GetMixerCoefficient(const UWord inWhichMixer, ULWord &outMixCoefficient)
Returns the current mix coefficient the given mixer/keyer.
@ kRegShiftSDIIn83GbpsMode
virtual bool GetTransmitSDIs(NTV2ChannelSet &outXmitSDIs)
Answers with the transmitting/output SDI connectors.
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
@ kRegShiftFramePulseEnable
@ kRegMaskSDIIn412GbpsMode
enum NTV2VideoFrameBufferOrientation NTV2FBOrientation
@ kDeviceCanDoMultiFormat
True if device can simultaneously handle different video formats on more than one SDI input or output...
@ kRegMaskAnalogCompositeFormat625
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
@ NTV2_STANDARD_3840i
Identifies Ultra-High-Definition (UHD) psf.
@ kRegShiftSDIIn212GbpsMode
virtual bool BankSelectWriteRegister(const NTV2RegInfo &inBankSelect, const NTV2RegInfo &inRegInfo)
Writes the given set of registers to the bank specified at position 0.
Declares the AJADebug class.
@ NTV2_FORMAT_3840x2160p_3000
@ kRegMaskSDIIn66GbpsMode
@ kRegShiftSDIInCRCErrorCountA
@ NTV2_FORMAT_1080psf_2400
@ kDeviceGetNumLTCOutputs
The number of analog LTC outputs on the device.
@ kRegShiftInput1Progressive
virtual bool SetVideoVOffset(const int inVOffset, const UWord inOutputSpigot=0)
Adjusts the vertical timing offset, in lines, for the given SDI output connector.
#define NTV2_IS_PROGRESSIVE_STANDARD(__s__)
@ kRegMaskSDIIn6LevelBtoLevelA
virtual bool GetAlphaFromInput2Bit(ULWord &outValue)
@ NTV2_FORMAT_4096x2160p_5994
@ kRegShiftVidProcFGMatteEnable
virtual bool ReadLineCount(ULWord &outValue)
Answers with the line offset into the frame currently being read (NTV2_MODE_DISPLAY) or written (NTV2...
@ kDeviceGetNumLTCInputs
The number of analog LTC inputs on the device.
@ NTV2_REFERENCE_SFP1_PCR
Specifies the PCR source on SFP 1.
@ kRegMaskQuarterSizeMode
@ kRegShiftDualLinKOutput
virtual bool GetFrameBufferOrientation(const NTV2Channel inChannel, NTV2FBOrientation &outValue)
Answers with the current frame buffer orientation for the given NTV2Channel.
@ kRegMaskRS422ParitySense
@ NTV2_FORMAT_4x2048x1080psf_2997
@ kRegMaskVidProcFGMatteEnable
static const ULWord gChannelToSDIIn12GModeMask[]
@ NTV2_VIDEOLIMITING_OFF
Disables normal FrameBuffer Y/C value read limiting (NOT RECOMMENDED).
@ kRegMaskSDIIn83GbpsSMPTELevelBMode
@ NTV2_AUDIOSYSTEM_INVALID
@ NTV2_FORMAT_1080p_2K_2997
@ kRegShiftFrameOrientation
virtual bool GetFrameBufferQuality(NTV2Channel inChannel, NTV2FrameBufferQuality &outValue)
static const ULWord gChannelToRP188ModeGCRegisterNum[]