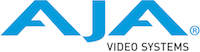 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
57 if (GetLocationLineNumber() == 0)
92 uint8_t char1(0), char2(0);
93 bool bGotClock (
false);
152 if (pInAncData->GetLocationLineNumber() == 21 || pInAncData->GetLocationLineNumber() == 284)
208 static const uint8_t cc_clock[27] = { 16, 17, 22, 29, 38, 49, 61, 74, 86, 98, 108, 116, 122, 125,
209 125, 122, 116, 108, 98, 86, 74, 61, 49, 38, 29, 22, 17 };
219 const uint8_t kSMPTE_Y_Black (0x10);
227 for (j = 0; j < 7; j++)
248 while (pos < GetDC())
253 dataStartOffset = lineStartOffset + (10 *
CC_BIT_WIDTH);
294 for (uint8_t j = 0; j < 8; j++)
303 uint8_t nextMask = mask << 1;
323 const uint8_t * pTrans (
NULL);
325 if (inStartLevel == 0 && inEndLevel == 0)
326 pTrans = cc_trans_lo_lo;
327 else if (inStartLevel == 0 && inEndLevel != 0)
328 pTrans = cc_trans_lo_hi;
329 else if (inStartLevel != 0 && inEndLevel == 0)
330 pTrans = cc_trans_hi_lo;
332 pTrans = cc_trans_hi_hi;
353 outChar1 = outChar2 = 0xFF;
380 const uint8_t *pFirstYSample = pInLine;
381 const uint8_t *pFirstClockEdge =
NULL;
382 const uint8_t *pLastClockEdge =
NULL;
383 const uint8_t *pFirstDataBit = pFirstYSample;
392 uint32_t startSearch = 10;
393 uint32_t stopSearch = 30;
394 for (pos = startSearch; pos < stopSearch; pos++)
399 if (pos < stopSearch)
401 pFirstClockEdge = &pFirstYSample[pos];
407 for (pos = 0; pos < 7; pos++)
429 for (pos = startSearch; pos < stopSearch; pos++)
436 pLastClockEdge = &pFirstClockEdge[pos+1];
462 return pFirstDataBit;
474 for (uint8_t i = 0; i < 8; i++)
477 outChar1 += (bit << i);
485 for (uint8_t i = 0; i < 8; i++)
488 outChar2 += (bit << i);
492 if (char1 == 0x80 && char2 == 0x80)
493 cerr <<
"--- AJAAncillaryData_Cea608_Line21::DecodeCharacters: returned NULL" << endl;
494 else if (((outChar1 & 0x7f) >= 0x20) && ((outChar2 & 0x7f) >= 0x20))
495 cerr <<
"--- AJAAncillaryData_Cea608_Line21::DecodeCharacters: returned '" << (outChar1 & 0x7f) <<
"' '" << (outChar2 & 0x7f) << endl;
497 cerr <<
"--- AJAAncillaryData_Cea608_Line21::DecodeCharacters: returned " <<
xHEX0N(outChar1,2) <<
" " <<
xHEX0N(outChar2) << endl;
const uint32_t CLOCK_RUN_IN_OFFSET
static const uint8_t * CheckDecodeClock(const uint8_t *pInLine, bool &outGotClock)
Checks for the existence of a CEA-608 "analog" waveform and, if found, returns a pointer to the start...
const uint32_t AJAAncillaryData_Cea608_Line21_PayloadSize
virtual AJAStatus EncodeLine(const uint8_t inChar1, const uint8_t inChar2, const uint32_t inDataStartOffset)
Encode and insert the given 8-bit characters into the (already initialized) payload buffer.
uint8_t m_DID
Official SMPTE ancillary packet ID (w/o parity)
Declares the AJAAncillaryData_Cea608_line21 class.
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
virtual void Clear(void)
Frees my allocated memory, if any, and resets my members to their default values.
ByteVector::size_type ByteVectorIndex
virtual void Clear(void)
Frees my allocated memory, if any, and resets my members to their default values.
This class handles "analog" (Line 21) based CEA-608 caption data packets.
const uint8_t CC_LEVEL_HI
const uint32_t TRANSITION_POST
const uint8_t AJAAncillaryData_Cea608_Line21_SID
AJAAncDataType
Identifies the ancillary data types that are known to this module.
AJAStatus AllocDataMemory(const uint32_t inNumBytes)
uint32_t m_dataStartOffset
Offset into the encode buffer where data starts.
virtual ~AJAAncillaryData_Cea608_Line21()
My destructor.
@ AJAAncDataCoding_Raw
The ancillary data is in the form of a digitized waveform (e.g. CEA-608 captions, VITC,...
static AJAStatus DecodeCharacters(const uint8_t *ptr, uint8_t &outChar1, uint8_t &outChar2)
Decodes the two CEA-608 data characters for this line.
virtual uint8_t * EncodeTransition(uint8_t *ptr, const uint8_t inStartLevel, const uint8_t inEndLevel)
Encodes a single bit transition from the "from" level to the "to" level.
static AJAAncDataType RecognizeThisAncillaryData(const AJAAncillaryData *pInAncData)
This is the base class for handling CEA-608 caption data packets.
bool m_bEncodeBufferInitialized
Set 'true' after successfully allocating and initializing the m_payload buffer for encoding.
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
virtual AJAStatus AllocEncodeBuffer(void)
virtual const uint8_t * GetPayloadData(void) const
AJAAncillaryData_Cea608_Line21()
My default constructor.
virtual AJAStatus ParsePayloadData(void)
Parses out (interprets) the "local" ancillary data from my payload data.
const uint8_t CC_BIT_WIDTH
const uint8_t CC_LEVEL_LO
AJAAncDataCoding m_coding
Analog or digital data.
virtual AJAStatus DecodeLine(uint8_t &outChar1, uint8_t &outChar2, bool &outGotClock) const
Decodes the payload to extract the two captioning characters. The caller must check outGotClock to de...
const uint8_t CC_LEVEL_TRANS
#define AJA_SUCCESS(_status_)
const uint32_t TRANSITION_PRE
bool m_rcvDataValid
This is set true (or not) by ParsePayloadData()
const uint32_t CC_DEFAULT_ENCODE_OFFSET
uint8_t m_SID
Official SMPTE secondary ID (or DBN - w/o parity)
virtual AJAStatus SetLocationLineNumber(const uint16_t inLineNum)
Sets my ancillary data "location" frame line number.
ByteVector m_payload
My payload data (DC = size)
virtual AJAStatus InitEncodeBuffer(const uint32_t inLineStartOffset, uint32_t &outDataStartOffset)
Initializes the payload buffer with all of the "static" pixels, e.g. run-in clock,...
virtual uint8_t * EncodeCharacter(uint8_t *ptr, const uint8_t inChar)
Encodes a single 8-bit character from just after the transition to the first bit until just before th...
const uint8_t CC_LEVEL_MID
virtual AJAAncillaryData_Cea608_Line21 & operator=(const AJAAncillaryData_Cea608_Line21 &inRHS)
Assignment operator – replaces my contents with the right-hand-side value.
AJAAncDataType m_ancType
One of a known set of ancillary data types (or "Custom" if not identified)
virtual AJAStatus GeneratePayloadData(void)
Generate the payload data from the "local" ancillary data.
#define xHEX0N(__x__, __n__)
@ AJAAncDataType_Cea608_Line21
CEA608 SD Closed Captioning ("Line 21" waveform)
const uint8_t AJAAncillaryData_Cea608_Line21_DID
const uint32_t TRANSITION_WIDTH
AJAAncillaryData_Cea608 & operator=(const AJAAncillaryData_Cea608 &inRHS)
Assignment operator – replaces my contents with the right-hand-side value.