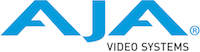 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
9 #ifndef _NTV2DEMOCOMMON_H
10 #define _NTV2DEMOCOMMON_H
28 #define CAPFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_DemoCapture, AJAFUNC << ": " << _expr_)
29 #define CAPWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_DemoCapture, AJAFUNC << ": " << _expr_)
30 #define CAPDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_DemoCapture, AJAFUNC << ": " << _expr_)
31 #define CAPNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_DemoCapture, AJAFUNC << ": " << _expr_)
32 #define CAPINFO(_expr_) AJA_sINFO (AJA_DebugUnit_DemoCapture, AJAFUNC << ": " << _expr_)
34 #define PLFAIL(_xpr_) AJA_sERROR (AJA_DebugUnit_DemoPlayout, AJAFUNC << ": " << _xpr_)
35 #define PLWARN(_xpr_) AJA_sWARNING(AJA_DebugUnit_DemoPlayout, AJAFUNC << ": " << _xpr_)
36 #define PLNOTE(_xpr_) AJA_sNOTICE (AJA_DebugUnit_DemoPlayout, AJAFUNC << ": " << _xpr_)
37 #define PLINFO(_xpr_) AJA_sINFO (AJA_DebugUnit_DemoPlayout, AJAFUNC << ": " << _xpr_)
38 #define PLDBG(_xpr_) AJA_sDEBUG (AJA_DebugUnit_DemoPlayout, AJAFUNC << ": " << _xpr_)
40 #define BURNFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
41 #define BURNWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
42 #define BURNDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
43 #define BURNNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
44 #define BURNINFO(_expr_) AJA_sINFO (AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
46 #define NTV2_AUDIOSIZE_MAX (401 * 1024)
47 #define NTV2_ANCSIZE_MAX (0x2000) // 8K
142 fNumAudioBytes = fNumAncBytes = fNumAnc2Bytes = 0;
170 inline Bouncer (
const T inUpperLimit,
const T inLowerLimit = T(0),
const T inStartValue = T(0),
const bool inStartAscend =
true)
171 : mMin (inLowerLimit),
173 mValue (inStartValue),
175 mAscend (inStartAscend)
179 else if (mMin == mMax)
180 mMax = mMin + mIncrement;
198 mValue += mIncrement;
205 mValue -= mIncrement;
213 inline T
Value (
void)
const {
return mValue;}
216 T mMin, mMax, mValue, mIncrement;
285 : fDeviceSpec (inDeviceSpec),
292 fDoABConversion (
false),
293 fDoMultiFormat (
false),
336 inline explicit PlayerConfig (
const std::string & inDeviceSpecifier =
"0")
398 inline explicit BurnConfig (
const std::string & inDeviceSpecifier =
"0")
419 inline bool WithAnc(
void)
const {
return fWithAnc;}
420 inline bool WithHanc(
void)
const {
return fWithHanc;}
422 inline bool FieldMode(
void)
const {
return fIsFieldMode;}
459 static bool IsValidDevice (
const std::string & inDeviceSpec);
489 const std::string inDeviceSpecifier = std::string ());
534 const std::string inDeviceSpecifier = std::string ());
569 const std::string inDeviceSpecifier = std::string ());
576 static NTV2InputSource GetInputSourceFromString (
const std::string & inStr);
590 static std::string GetOutputDestinationStrings (
const std::string inDeviceSpecifier = std::string ());
623 const std::string inDeviceSpecifier = std::string(),
624 const bool inIsInputOnly =
true);
631 static NTV2TCIndex GetTCIndexFromString (
const std::string & inStr);
645 static std::string GetAudioSystemStrings (
const std::string inDeviceSpecifier = std::string ());
652 static NTV2AudioSystem GetAudioSystemFromString (
const std::string & inStr);
664 static std::string GetTestPatternStrings (
void);
670 static std::string GetTestPatternNameFromString (
const std::string & inStr);
682 static std::string GetVANCModeStrings (
void);
688 static NTV2VANCMode GetVANCModeFromString (
const std::string & inStr);
703 const bool isInputRGB =
false);
715 const bool isInputRGB =
false);
728 const bool isInputRGB =
false);
741 static std::string ToLower (
const std::string & inStr);
748 static std::string StripFormatString (
const std::string & inStr);
754 static char ReadCharacterPress (
void);
759 static void WaitForEnterKeyPress (
void);
776 static const char * GetGlobalMutexName (
void);
795 static size_t SetDefaultPageSize (
void);
804 Popt (
const int inArgc,
const char ** pArgs,
const PoptOpts * pInOptionsTable);
807 virtual inline bool isGood (
void)
const {
return parseResult() == -1;}
808 virtual inline operator bool()
const {
return isGood();}
809 virtual inline const std::string &
errorStr (
void)
const {
return mError;}
818 static bool BFT(
void);
825 #if defined(AJA_RAW_AUDIO_RECORD)
832 #define AJA_NTV2_AUDIO_RECORD_BEGIN ostringstream _filename; \
833 _filename << ::NTV2DeviceString(mDeviceID) << "-" << mDevice.GetIndexNumber() \
834 << "." << ::NTV2ChannelToString(mConfig.fInputChannel,true) \
835 << "." << ::NTV2InputSourceToString(mConfig.fInputSource, true) \
836 << "." << ::NTV2VideoFormatToString(mVideoFormat) \
837 << "." << ::NTV2AudioSystemToString(mAudioSystem, true) \
838 << "." << AJAProcess::GetPid() \
840 ofstream _ostrm(_filename.str(), ios::binary);
842 #define AJA_NTV2_AUDIO_RECORD_DO if (NTV2_IS_VALID_AUDIO_SYSTEM(mAudioSystem)) \
843 if (pFrameData->fAudioBuffer) \
844 _ostrm.write(pFrameData->AudioBuffer(), \
845 streamsize(pFrameData->NumCapturedAudioBytes()));
847 #define AJA_NTV2_AUDIO_RECORD_END
848 #elif defined(AJA_WAV_AUDIO_RECORD)
851 #define AJA_NTV2_AUDIO_RECORD_BEGIN ostringstream _wavfilename; \
852 _wavfilename << ::NTV2DeviceString(mDeviceID) << "-" << mDevice.GetIndexNumber() \
853 << "." << ::NTV2ChannelToString(mConfig.fInputChannel,true) \
854 << "." << ::NTV2InputSourceToString(mConfig.fInputSource, true) \
855 << "." << ::NTV2VideoFormatToString(mVideoFormat) \
856 << "." << ::NTV2AudioSystemToString(mAudioSystem, true) \
857 << "." << AJAProcess::GetPid() \
859 const int _wavMaxNumAudChls(mDevice.features().GetMaxAudioChannels()); \
860 AJAWavWriter _wavWriter (_wavfilename.str(), \
861 AJAWavWriterAudioFormat(_wavMaxNumAudChls, 48000, 32)); \
864 #define AJA_NTV2_AUDIO_RECORD_DO if (NTV2_IS_VALID_AUDIO_SYSTEM(mAudioSystem)) \
865 if (pFrameData->fAudioBuffer) \
866 if (_wavWriter.IsOpen()) \
867 _wavWriter.write(pFrameData->AudioBuffer(), pFrameData->NumCapturedAudioBytes());
869 #define AJA_NTV2_AUDIO_RECORD_END if (_wavWriter.IsOpen()) \
872 #define AJA_NTV2_AUDIO_RECORD_BEGIN
873 #define AJA_NTV2_AUDIO_RECORD_DO
874 #define AJA_NTV2_AUDIO_RECORD_END
878 #if defined(AJA_RECORD_MLAUDIO)
880 #define AJA_NTV2_MLAUDIO_RECORD_BEGIN ofstream ofs1, ofs2; \
881 if (mConfig.fNumAudioLinks > 1) \
883 ofs1.open("temp1.raw", ios::out | ios::trunc | ios::binary); \
884 ofs2.open("temp2.raw", ios::out | ios::trunc | ios::binary); \
887 #define AJA_NTV2_MLAUDIO_RECORD_DO if (mConfig.fNumAudioLinks > 1) \
888 { const ULWord halfBytes (pFrameData->NumCapturedAudioBytes() / 2); \
889 ofs1.write(pFrameData->AudioBuffer(), halfBytes); \
890 NTV2Buffer lastHalf (pFrameData->fAudioBuffer.GetHostAddress(halfBytes), halfBytes); \
891 ofs2.write(lastHalf, lastHalf.GetByteCount()); \
894 #define AJA_NTV2_MLAUDIO_RECORD_END if (mConfig.fNumAudioLinks > 1) \
900 #define AJA_NTV2_MLAUDIO_RECORD_BEGIN
901 #define AJA_NTV2_MLAUDIO_RECORD_DO
902 #define AJA_NTV2_MLAUDIO_RECORD_END
905 #endif // _NTV2DEMOCOMMON_H
std::set< NTV2InputSource > NTV2InputSourceSet
A set of distinct NTV2InputSource values.
ULWord NumCapturedAnc2Bytes(void) const
uint32_t fFrameFlags
Frame data flags.
bool fDoMultiFormat
If true, use multi-format/multi-channel mode, if device supports it; otherwise normal mode.
uint32_t fFrameFlags
Frame data flags.
virtual const NTV2StringList & otherArgs(void) const
bool fDoHDMIOutput
If true, enable HDMI output; otherwise, disable HDMI output.
Declares the AJASystemInfo class.
RP188_STRUCT fRP188Data2
For future use.
PlayerConfig(const std::string &inDeviceSpecifier="0")
Constructs a default Player configuration.
Declares the AJAAncillaryData class.
std::set< NTV2FrameBufferFormat > NTV2FrameBufferFormatSet
A set of distinct NTV2FrameBufferFormat values.
NTV2OutputDestination
Identifies a specific video output destination.
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
RP188_STRUCT fRP188Data
For future use.
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
bool fIsFieldMode
True if Field Mode, otherwise Frame Mode.
std::set< NTV2TCIndex > NTV2TCIndexes
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
NTV2FrameDataArray::const_iterator NTV2FrameDataArrayConstIter
Handy const iterator.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
ULWord fNumAncBytes
Actual number of captured F1 anc bytes.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
NTV2ACFrameRange ACFrameRange
ULWord GetByteCount(void) const
std::set< NTV2VideoFormat > NTV2VideoFormatSet
A set of distinct NTV2VideoFormat values.
This structure encapsulates the video, audio and anc buffers used in the AutoCirculate demos....
NTV2Buffer & AncBuffer2(void)
Declares the AJATime class.
Declares the AJAWavWriter class.
@ NTV2_OUTPUTDESTINATION_SDI2
#define NTV2_IS_VALID_TIMECODE_INDEX(__x__)
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
bool fSuppressAudio
If true, suppress audio; otherwise include audio.
NTV2TCIndex fTimecodeSource
Timecode source to use.
NTV2PixelFormat fPixelFormat
Pixel format to use.
AJAAncDataType
Identifies the ancillary data types that are known to this module.
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
NTV2Buffer fAncBuffer2
Additional "F2" host anc buffer.
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
std::string fAncDataFilePath
Optional path to Anc binary data file.
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
bool fSuppressVideo
If true, suppress video; otherwise include video.
enum NTV2InputSourceKinds NTV2IOKinds
NTV2Buffer fAncBuffer
Host ancillary data buffer.
NTV2VANCMode fVancMode
VANC mode to use.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
bool fDoLinkGrouping
If true, enables 6/12G output mode on IoX3/Kona5 (4K/8K)
virtual int parseResult(void) const
NTV2FrameRate
Identifies a particular video frame rate.
enum NTV2OutputDestination NTV2OutputDest
bool fWithAnc
If true, also capture Anc.
NTV2Buffer & VideoBuffer(void)
bool fDoTsiRouting
If true, enable TSI routing; otherwise route for square division (4K/8K)
AJAAncDataType fTransmitHDRType
Specifies the HDR anc data packet to transmit, if any.
NTV2PixelFormat fPixelFormat
The pixel format to use.
Bouncer(const T inUpperLimit, const T inLowerLimit=T(0), const T inStartValue=T(0), const bool inStartAscend=(!(0)))
NTV2ACFrameRange fOutputFrames
Playout frame count or range.
ULWord NumCapturedAncBytes(void) const
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
uint32_t fAudioRecordSize
For future use.
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
@ NTV2_TCINDEX_SDI1
SDI 1 embedded VITC.
enum _NTV2DeviceKinds NTV2DeviceKinds
These enum values are used for device selection/filtering.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
bool HasValidTimecode(const NTV2TCIndex inTCNdx) const
ULWord NumCapturedAudioBytes(void) const
NTV2Buffer fVideoBuffer
Host video buffer.
This class is used to configure an NTV2Capture instance.
uint8_t * fVideoBufferUnaligned
For future use.
ULWord VideoBufferSize(void) const
bool fSuppressAudio
If true, suppress audio; otherwise generate audio tone.
struct BurnConfig BurnConfig
Configures an NTV2Burn or NTV2FieldBurn instance.
AutoCirculate Frame Range.
bool fWithHanc
If true, capture & play HANC data, including audio (LLBurn). Defaults to false.
@ NTV2_INPUTSOURCE_INVALID
The invalid video input.
std::set< NTV2AudioSystem > NTV2AudioSystemSet
A set of distinct NTV2AudioSystem values. New in SDK 16.2.
Declares the CNTV2Card class.
uint32_t fAudioBufferSize
Size of host audio buffer, in bytes.
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
bool fSuppressVideo
If true, suppress video; otherwise generate test patterns.
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
ULWord AncBufferSize(void) const
UWord fNumAudioLinks
The number of audio systems to control for multi-link audio (4K/8K)
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
NTV2VideoFormat fVideoFormat
The video format to use.
ULWord AudioBufferSize(void) const
ULWord AncBuffer2Size(void) const
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
NTV2Channel fOutputChannel
The device channel to use.
std::string fDeviceSpec
The AJA device to use.
Declares numerous NTV2 utility functions.
AJALabelValuePairs Get(const bool inCompact=(0)) const
Renders a human-readable representation of me.
virtual const std::string & errorStr(void) const
std::string fAncDataFilePath
Optional path to Anc binary data file to playout.
NTV2Buffer & AudioBuffer(void)
I interrogate and control an AJA video/audio capture/playout device.
@ NTV2_DEVICEKIND_ALL
Specifies any/all devices.
uint32_t fAncF2BufferSize
Size of "Field 2" ANC buffer, in bytes.
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
NTV2Buffer fVideoBuffer2
Additional host video buffer, usually F2.
bool fWithAnc
If true, capture & play anc data (LLBurn). Defaults to false.
std::string fDeviceSpec2
Second AJA device to use (Burn4KQuadrant or BurnBoardToBoard only)
std::vector< NTV2FrameData > NTV2FrameDataArray
A vector of NTV2FrameData elements.
bool fDoABConversion
If true, do level-A/B conversion; otherwise don't.
enum _NTV2PixelFormatKinds NTV2PixelFormatKinds
std::vector< std::string > NTV2StringList
NTV2VANCMode
These enum values identify the available VANC modes.
bool HasTimecode(const NTV2TCIndex inTCNdx) const
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
uint32_t * fAudioBuffer
Pointer to host audio buffer.
NTV2Channel fInputChannel
The input channel to use.
NTV2Channel fInputChannel
The device channel to use.
uint32_t * fVideoBuffer
Pointer to host video buffer.
A set of common convenience functions used by the NTV2 Demonstration Applications....
std::vector< NTV2Channel > NTV2ChannelList
An ordered sequence of NTV2Channel values.
std::string fDeviceSpec
The AJA device to use.
NTV2InputSource
Identifies a specific video input source.
NTV2InputSource fInputSource
The device input connector to use.
NTV2Buffer fAudioBuffer
Host audio buffer.
uint32_t * fVideoBuffer2
Pointer to an additional host video buffer, usually field 2.
bool fTransmitLTC
If true, embed LTC; otherwise embed VITC.
uint32_t fAncBufferSize
Size of ANC buffer, in bytes.
NTV2Buffer & VideoBuffer2(void)
@ VIDEO_FORMATS_NON_4KUHD
bool fDoRGBOnWire
If true, produce RGB on the wire; otherwise output YUV.
NTV2OutputDest fOutputDest
The desired output connector to use.
Private include file for all ajabase sources.
AJACircularBuffer< NTV2FrameData * > FrameDataRingBuffer
Buffer ring of NTV2FrameData's.
std::string fDeviceSpec
The AJA device to use.
uint32_t * fAncF2Buffer
Pointer to "Field 2" ANC buffer.
Declares the enumeration constants used in the ajabase library.
bool fDoTSIRouting
If true, do TSI routing; otherwise squares.
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
std::map< NTV2TCIndex, NTV2_RP188 > NTV2TimeCodes
A mapping of NTV2TCIndex enum values to NTV2_RP188 structures.
ULWord VideoBufferSize2(void) const
NTV2FrameDataArray::iterator NTV2FrameDataArrayIter
Handy non-const iterator.
virtual bool isGood(void) const
This struct replaces the old RP188_STRUCT.
AJALabelValuePairs Get(const bool inCompact=(0)) const
Renders a human-readable representation of me.
uint32_t fVideoBufferSize
Size of host video buffer, in bytes.
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
ULWord fNumAnc2Bytes
Actual number of captured F2 anc bytes.
A handy class that makes it easy to "bounce" an unsigned integer value between a minimum and maximum ...
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
@ NTV2_IOKINDS_ALL
Specifies any/all input/output kinds.
Declares the CRP188 class. See SMPTE RP188 standard for details.
void swap(nlohmann::basic_json< ObjectType, ArrayType, StringType, BooleanType, NumberIntegerType, NumberUnsignedType, NumberFloatType, AllocatorType, JSONSerializer, BinaryType, CustomBaseClass > &j1, nlohmann::basic_json< ObjectType, ArrayType, StringType, BooleanType, NumberIntegerType, NumberUnsignedType, NumberFloatType, AllocatorType, JSONSerializer, BinaryType, CustomBaseClass > &j2) noexcept(//NOLINT(readability-inconsistent-declaration-parameter-name, cert-dcl58-cpp) is_nothrow_move_constructible< nlohmann::basic_json< ObjectType, ArrayType, StringType, BooleanType, NumberIntegerType, NumberUnsignedType, NumberFloatType, AllocatorType, JSONSerializer, BinaryType, CustomBaseClass > >::value &&//NOLINT(misc-redundant-expression, cppcoreguidelines-noexcept-swap, performance-noexcept-swap) is_nothrow_move_assignable< nlohmann::basic_json< ObjectType, ArrayType, StringType, BooleanType, NumberIntegerType, NumberUnsignedType, NumberFloatType, AllocatorType, JSONSerializer, BinaryType, CustomBaseClass > >::value)
exchanges the values of two JSON objects
uint32_t fAncRecordSize
For future use.
void SetIncrement(const T inValue)
uint32_t * fAncBuffer
Pointer to ANC buffer.
UWord fNumAudioLinks
Number of audio links to capture.
CaptureConfig(const std::string &inDeviceSpec="0")
Constructs a default NTV2Capture configuration.
Configures an NTV2Player instance.
bool fDoABConversion
If true, do level-A/B conversion; otherwise don't.
NTV2PixelFormat fPixelFormat
The pixel format to use.
bool Fill(const T &inValue)
Fills me with the given scalar value.
Declares enums and structs used by all platform drivers and the SDK.
std::ostream & operator<<(std::ostream &ioStrm, const CaptureConfig &inObj)
Declaration of AJACircularBuffer template class.
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
NTV2ACFrameRange fInputFrames
Ingest frame count or range.
Configures an NTV2Burn or NTV2FieldBurn instance.
NTV2Buffer & AncBuffer(void)
enum _NTV2VideoFormatKinds NTV2VideoFormatKinds
struct PlayerConfig PlayerConfig
Configures an NTV2Player instance.
enum _NTV2TCIndexKinds NTV2TCIndexKinds
BurnConfig(const std::string &inDeviceSpecifier="0")
Constructs a default Player configuration.
NTV2InputSource fInputSource
The device input connector to use.
NTV2Channel fOutputChannel
The output channel to use.
Declares the AJADebug class.
NTV2TimeCodes fTimecodes
Map of TC indexes to NTV2_RP188 values.
bool fWithAudio
If true, also capture Audio.