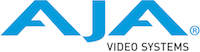 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
8 #ifndef AJA_CIRCULAR_BUFFER_H
9 #define AJA_CIRCULAR_BUFFER_H
29 template <
typename FrameDataPtr>
53 mAbortFlag = pAbortFlag;
63 return mCircBufferCount;
82 return (
unsigned int) mFrames.size ();
94 mFrames.push_back(pInFrameData);
96 mLocks.push_back(lock);
113 if( !WaitForLockOrAbort(&mDataBufferLock) )
116 if ( mCircBufferCount == mFrames.size() )
119 if( !WaitForEventOrAbort(&mNotFullEvent) )
126 if( !WaitForLockOrAbort(mLocks[mHead]) )
return NULL;
128 mHead = (mHead+1)%((
unsigned int)(mFrames.size()));
130 if( mCircBufferCount == mFrames.size() )
135 return mFrames[mFillIndex];
157 if( !WaitForLockOrAbort(&mDataBufferLock) )
160 if ( mCircBufferCount == 0 )
163 if( !WaitForEventOrAbort(&mNotEmptyEvent) )
171 if( !WaitForLockOrAbort(mLocks[mTail]) )
175 mTail = (mTail+1)%((
unsigned int)mFrames.size());
177 if( mCircBufferCount == 0 )
181 return mFrames[mEmptyIndex];
202 typedef std::vector <AJALock *> AJALockVector;
204 std::vector <FrameDataPtr> mFrames;
205 AJALockVector mLocks;
209 unsigned int mCircBufferCount;
214 unsigned int mFillIndex;
215 unsigned int mEmptyIndex;
217 const bool * mAbortFlag;
224 bool WaitForEventOrAbort (
AJAEvent * ajaEvent);
232 bool WaitForLockOrAbort (
AJALock * ajaEvent);
238 template <
typename FrameDataPtr>
242 mCircBufferCount (0),
249 template <
typename FrameDataPtr>
258 template<
typename FrameDataPtr>
261 mLocks[mFillIndex]->Unlock();
262 mNotEmptyEvent.SetState(
true);
265 template<
typename FrameDataPtr>
268 mLocks[mEmptyIndex]->Unlock();
269 mNotFullEvent.SetState(
true);
272 template<
typename FrameDataPtr>
275 const unsigned int timeout = 100;
292 template<
typename FrameDataPtr>
295 const unsigned int timeout = 100;
313 template<
typename FrameDataPtr>
316 for (AJALockVector::iterator iter (mLocks.begin()); iter != mLocks.end(); ++iter)
322 mHead = mTail = mFillIndex = mEmptyIndex = mCircBufferCount = 0;
327 #endif // AJA_CIRCULAR_BUFFER_H
AJACircularBuffer()
My default constructor.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
virtual AJAStatus WaitForSignal(uint32_t timeout=0xffffffff)
Master header for the ajabase library.
unsigned int GetCircBufferCount(void) const
Retrieves the size count of the circular buffer, i.e. how far the tail pointer is behind the head poi...
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
Declares the AJALock class.
virtual AJAStatus SetState(bool signaled=true)
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
bool IsEmpty(void) const
Returns "true" if I'm empty – i.e., if my tail and head are in the same place.
Declares the AJAEvent class.
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
unsigned int GetNumFrames(void) const
Returns my frame storage capacity, which reflects how many times my Add method has been called.
virtual AJAStatus Lock(uint32_t timeout=0xffffffff)
void Clear(void)
Clears my frame collection, their locks, everything.
virtual ~AJACircularBuffer()
My destructor.
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
virtual AJAStatus Unlock()
I am a circular frame buffer that simplifies implementing a type-safe producer/consumer model for pro...