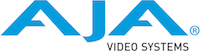 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
17 static string ToLower (
const string & inStr)
23 static string ToUpper (
const string & inStr)
30 {
static const string sHexDigits(
"0123456789ABCDEFabcdef");
31 return sHexDigits.find(inChr) != string::npos;
35 {
static const string sDecDigits(
"0123456789");
36 return sDecDigits.find(inChr) != string::npos;
40 {
static const string sLegalChars(
"0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz");
41 return sLegalChars.find(inChr) != string::npos;
46 if (inStr.length() > inMaxLength)
48 for (
size_t ndx(0); ndx < inStr.size(); ndx++)
49 if (!IsDecimalDigit(inStr.at(ndx)))
56 if (inStr.length() < 3)
58 string hexStr(::
ToLower(inStr));
59 if (hexStr[0] ==
'0' && hexStr[1] ==
'x')
61 if (hexStr.length() > 16)
63 for (
size_t ndx(0); ndx < hexStr.size(); ndx++)
64 if (!IsHexDigit(hexStr.at(ndx)))
66 while (hexStr.length() != 16)
67 hexStr =
'0' + hexStr;
68 istringstream iss(hexStr);
76 for (
size_t ndx(0); ndx < inStr.size(); ndx++)
77 if (!IsAlphaNumeric(inStr.at(ndx)))
84 if (inStr.length() != 8 && inStr.length() != 9)
86 return IsAlphaNumeric(inStr);
106 if (
this != &boardScan)
123 _deviceInfoList.clear();
126 for (
NTV2DeviceInfoListConstIter bilIter (boardScan._deviceInfoList.begin ()); bilIter != boardScan._deviceInfoList.end (); ++bilIter)
132 boardInfo.
deviceID = bilIter->deviceID;
133 boardInfo.
pciSlot = bilIter->pciSlot;
139 for (NTV2AudioSampleRateList::const_iterator asrIter (bilIter->audioSampleRateList.begin()); asrIter != bilIter->audioSampleRateList.end(); ++asrIter)
143 for (NTV2AudioChannelsPerFrameList::const_iterator ncIter (bilIter->audioNumChannelsList.begin()); ncIter != bilIter->audioNumChannelsList.end(); ++ncIter)
147 for (NTV2AudioBitsPerSampleList::const_iterator bpsIter (bilIter->audioBitsPerSampleList.begin()); bpsIter != bilIter->audioBitsPerSampleList.end(); ++bpsIter)
151 for (NTV2AudioSourceList::const_iterator aslIter (bilIter->audioInSourceList.begin()); aslIter != bilIter->audioInSourceList.end(); ++aslIter)
155 for (NTV2AudioSourceList::const_iterator aoslIter (bilIter->audioOutSourceList.begin()); aoslIter != bilIter->audioOutSourceList.end(); ++aoslIter)
159 _deviceInfoList.push_back(boardInfo);
165 { (void) inDeviceMask;
170 GetDeviceInfoList().clear();
172 for (
UWord boardNum(0); ; boardNum++)
175 if (tmpDevice.IsOpen())
190 oss <<
", Slot " << info.
pciSlot;
194 SetVideoAttributes(info);
195 SetAudioAttributes(info, tmpDevice);
196 GetDeviceInfoList().push_back(info);
214 if (iter->deviceID == inDeviceID)
228 if (inDeviceIndexNumber < deviceList.size())
230 outDeviceInfo = deviceList[inDeviceIndexNumber];
231 return outDeviceInfo.
deviceIndex == inDeviceIndexNumber;
252 if (iter->deviceID == inDeviceID)
262 if (!IsAlphaNumeric(inNameSubString))
264 if (inNameSubString.find(
":") != string::npos)
265 return outDevice.
Open(inNameSubString);
270 string nameSubString(::
ToLower(inNameSubString));
275 const string deviceName(::
ToLower(iter->deviceIdentifier));
276 if (deviceName.find(nameSubString) != string::npos)
279 if (nameSubString ==
"io4kplus")
281 nameSubString =
"avid dnxiv";
284 const string deviceName(::
ToLower(iter->deviceIdentifier));
285 if (deviceName.find(nameSubString) != string::npos)
298 const string searchSerialStr(::
ToLower(inSerialStr));
307 if (serNumStr.find(searchSerialStr) != string::npos)
321 if (iter->deviceSerialNumber == inSerialNumber)
330 if (inArgument.empty())
336 string upperArg(::
ToUpper(inArgument));
337 if (upperArg ==
"LIST" || upperArg ==
"?")
339 if (infoList.empty())
340 cout <<
"No devices detected" << endl;
342 cout <<
DEC(infoList.size()) <<
" available " << (infoList.size() == 1 ?
"device:" :
"devices:") << endl;
348 cout <<
" | " << setw(9) << serNum <<
" | " <<
HEX0N(iter->deviceSerialNumber,8);
362 inOutStr <<
" " << *iter;
389 return diffs ?
false :
true;
402 outBoardsAdded.clear ();
403 outBoardsRemoved.clear ();
407 if (oldIter == inOldList.end () && newIter == inNewList.end ())
410 if (oldIter != inOldList.end () && newIter != inNewList.end ())
414 if (oldInfo != newInfo)
417 outBoardsRemoved.push_back (oldInfo);
421 outBoardsAdded.push_back (newInfo);
430 if (oldIter != inOldList.end () && newIter == inNewList.end ())
432 outBoardsRemoved.push_back (*oldIter);
437 if (oldIter == inOldList.end () && newIter != inNewList.end ())
439 if (newIter->deviceID && newIter->deviceID !=
NTV2DeviceID(0xFFFFFFFF))
440 outBoardsAdded.push_back (*newIter);
450 return !outBoardsAdded.empty () || !outBoardsRemoved.empty ();
457 if (!inDevice.IsOpen())
460 if (!inDevice.GetHostName().empty() && inDevice.IsRemote())
461 return inDevice.GetHostName();
470 if (!str.empty() && str !=
"???")
474 ostringstream oss; oss <<
DEC(inDevice.GetIndexNumber());
482 inOutStr <<
" " << *iter;
491 inOutStr <<
" " << *iter;
509 return inOutStr <<
" ???";
516 inOutStr <<
" " << *iter;
525 <<
" Device Index Number: " << inInfo.
deviceIndex << endl
526 <<
" Device ID: 0x" << hex << inInfo.
deviceID << dec << endl
528 <<
" PCI Slot: 0x" << hex << inInfo.
pciSlot << dec << endl
542 <<
" Qrez: " << (inInfo.
qrezSupport ?
"Y" :
"N") << endl
543 <<
" HDV: " << (inInfo.
hdvSupport ?
"Y" :
"N") << endl
559 <<
" SDI 3G: " << (inInfo.
sdi3GSupport ?
"Y" :
"N") << endl
560 <<
" SDI 12G: " << (inInfo.
sdi12GSupport ?
"Y" :
"N") << endl
561 <<
" IP: " << (inInfo.
ipSupport ?
"Y" :
"N") << endl
563 <<
" LTC In: " << (inInfo.
ltcInSupport ?
"Y" :
"N") << endl
564 <<
" LTC Out: " << (inInfo.
ltcOutSupport ?
"Y" :
"N") << endl
565 <<
" LTC In on Ref Port: " << (inInfo.
ltcInOnRefPort ?
"Y" :
"N") << endl
594 inOutStr <<
"AudioPhysicalFormat:" << endl
595 <<
" boardNumber: " << inFormat.
boardNumber << endl
596 <<
" sampleRate: " << inFormat.
sampleRate << endl
597 <<
" numChannels: " << inFormat.
numChannels << endl
599 #if defined (DEBUG) || defined (AJA_DEBUG)
600 <<
" sourceIn: 0x" << hex << inFormat.
sourceIn << dec << endl
601 <<
" sourceOut: 0x" << hex << inFormat.
sourceOut << dec << endl
602 #endif // DEBUG or AJA_DEBUG
622 void CNTV2DeviceScanner::SetVideoAttributes (
NTV2DeviceInfo & info)
694 if (audioControl &
BIT(21))
735 std::sort (_deviceInfoList.begin (), _deviceInfoList.end (),
gCompareSlot);
static uint64_t IsLegalHexSerialNumber(const std::string &inStr)
UWord NTV2DeviceGetNumLTCInputs(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDo12GSDI(const NTV2DeviceID inDeviceID)
bool CanDoAudio2Channels(void)
UWord GetNumAESAudioOutputChannels(void)
UWord NTV2DeviceGetNumDownConverters(const NTV2DeviceID inDeviceID)
NTV2AudioSourceList audioOutSourceList
My supported audio output destinations (AES, etc.)
UWord NTV2DeviceGetNumHDMIVideoInputs(const NTV2DeviceID inDeviceID)
bool quarterExpandSupport
UWord numAnalogAudioInputChannels
Total number of analog audio input channels.
bool biDirectionalSDI
Supports Bi-directional SDI.
UWord GetNumAnalogAudioOutputChannels(void)
NTV2AudioBitsPerSampleList audioBitsPerSampleList
My supported audio bits-per-sample.
bool CanDoAudio8Channels(void)
UWord numDownConverters
Total number of down-converters.
UWord numAnlgVidOutputs
Total number of analog video outputs.
Declares device capability functions.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
bool has3GLevelConversion
Supports 3G Level Conversion?
This class is used to enumerate AJA devices that are attached and known to the local host computer.
UWord GetNumAudioSystems(void)
UWord numHDMIVidInputs
Total number of HDMI inputs.
bool sdi12GSupport
Supports 12G?
uint64_t deviceSerialNumber
Unique device serial number.
#define NTV2_ASSERT(_expr_)
bool ltcInSupport
Accepts LTC input?
NTV2AudioSourceList::const_iterator NTV2AudioSourceListConstIter
UWord numHDMIVidOutputs
Total number of HDMI outputs.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
static bool GetFirstDeviceWithID(const NTV2DeviceID inDeviceID, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device found on the host t...
std::vector< NTV2DeviceInfo > NTV2DeviceInfoList
I am an ordered list of NTV2DeviceInfo structs.
UWord NTV2DeviceGetNumAnalogVideoInputs(const NTV2DeviceID inDeviceID)
UWord numSerialPorts
Total number of serial ports.
UWord NTV2DeviceGetNumInputConverters(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
virtual bool GetDeviceInfo(const ULWord inDeviceIndexNumber, NTV2DeviceInfo &outDeviceInfo, const bool inRescan=false)
Returns detailed information about the AJA device having the given zero-based index number.
bool NTV2DeviceCanDoDualLink(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoProgrammableCSC(const NTV2DeviceID inDeviceID)
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
UWord GetNumAnalogAudioInputChannels(void)
bool NTV2DeviceCanDoHDV(const NTV2DeviceID inDeviceID)
static std::string SerialNum64ToString(const uint64_t inSerialNumber)
Returns a string containing the decoded, human-readable device serial number.
UWord GetNumEmbeddedAudioInputChannels(void)
static bool GetFirstDeviceWithSerial(const std::string &inSerialStr, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device whose serial number...
virtual NTV2DeviceInfoList & GetDeviceInfoList(void)
Returns an NTV2DeviceInfoList that can be "walked" using standard C++ vector iteration techniques.
UWord numHDMIAudioOutputChannels
Total number of HDMI audio output channels.
virtual void SortDeviceInfoList(void)
Sorts my device list by ascending PCI slot number.
bool NTV2DeviceCanDoStereoOut(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumSerialPorts(const NTV2DeviceID inDeviceID)
UWord numAnlgVidInputs
Total number of analog video inputs.
NTV2AudioChannelsPerFrameList::const_iterator NTV2AudioChannelsPerFrameListConstIter
bool NTV2DeviceCanDoProRes(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoStereoIn(const NTV2DeviceID inDeviceID)
virtual class DeviceCapabilities & features(void)
ULWord NTV2DeviceGetPingLED(const NTV2DeviceID inDeviceID)
std::vector< AudioBitsPerSampleEnum > NTV2AudioBitsPerSampleList
std::vector< AudioSampleRateEnum > NTV2AudioSampleRateList
static bool CompareDeviceInfoLists(const NTV2DeviceInfoList &inOldList, const NTV2DeviceInfoList &inNewList, NTV2DeviceInfoList &outDevicesAdded, NTV2DeviceInfoList &outDevicesRemoved)
Compares two NTV2DeviceInfoLists and returns a list of additions and a list of removals.
UWord numVidOutputs
Total number of video outputs – analog, digital, whatever.
std::string & lower(std::string &str)
std::string deviceIdentifier
Device name as seen in Control Panel, Watcher, Cables, etc.
NTV2AudioSourceList audioInSourceList
My supported audio input sources (AES, ADAT, etc.)
bool NTV2DeviceCanDoIsoConvert(const NTV2DeviceID inDeviceID)
bool has4KSupport
Supports 4K formats?
bool NTV2DeviceCanDoRateConvert(const NTV2DeviceID inDeviceID)
Declares the CNTV2DeviceScanner class.
#define AsNTV2DriverInterfaceRef(_x_)
UWord numOutputConverters
Total number of output converters.
bool NTV2DeviceHasBiDirectionalSDI(const NTV2DeviceID inDeviceID)
UWord GetNumEmbeddedAudioOutputChannels(void)
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
bool NTV2DeviceCanDo3GOut(NTV2DeviceID boardID, UWord index0)
UWord NTV2DeviceGetDownConverterDelay(const NTV2DeviceID inDeviceID)
virtual void ScanHardware(void)
Re-scans the local host for connected AJA devices.
CNTV2DeviceScanner(const bool inScanNow=true)
Constructs me.
virtual bool GetSerialNumberString(std::string &outSerialNumberString)
Answers with a string that contains my human-readable serial number.
UWord NTV2DeviceGetNumVideoInputs(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumHDMIVideoOutputs(const NTV2DeviceID inDeviceID)
static bool IsLegalDecimalNumber(const std::string &inStr, const size_t inMaxLength=2)
virtual bool DeviceIDPresent(const NTV2DeviceID inDeviceID, const bool inRescan=false)
Returns true if one or more AJA devices having the specified device identifier are attached and known...
bool CanDoAudio6Channels(void)
std::string & upper(std::string &str)
bool NTV2DeviceCanDo4KVideo(const NTV2DeviceID inDeviceID)
virtual uint64_t GetSerialNumber(void)
Answers with my serial number.
Declares numerous NTV2 utility functions.
UWord numDMAEngines
Total number of DMA engines.
bool NTV2DeviceCanDo3GLevelConversion(const NTV2DeviceID inDeviceID)
static bool GetFirstDeviceWithName(const std::string &inNameSubString, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device whose device identi...
static bool GetDeviceWithSerial(const uint64_t inSerialNumber, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the first AJA device whose serial number...
I interrogate and control an AJA video/audio capture/playout device.
UWord NTV2DeviceGetNumAnalogVideoOutputs(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoQuarterExpand(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumLTCOutputs(const NTV2DeviceID inDeviceID)
bool rgbAlphaOutputSupport
Supports RGB alpha channel?
bool NTV2DeviceCanDoColorCorrection(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDo2KVideo(const NTV2DeviceID inDeviceID)
UWord numAESAudioOutputChannels
Total number of AES audio output channels.
static std::string GetDeviceRefName(CNTV2Card &inDevice)
bool CanDoAnalogAudio(void)
static string ToUpper(const string &inStr)
bool has2KSupport
Supports 2K formats?
bool proResSupport
Supports ProRes?
bool stereoOutSupport
Supports stereo output?
UWord numUpConverters
Total number of up-converters.
static bool gCompareSlot(const NTV2DeviceInfo &b1, const NTV2DeviceInfo &b2)
bool NTV2DeviceCanDoDVCProHD(const NTV2DeviceID inDeviceID)
UWord numEmbeddedAudioInputChannels
Total number of embedded (SDI) audio input channels.
bool NTV2DeviceCanDoVideoProcessing(const NTV2DeviceID inDeviceID)
static bool IsHexDigit(const char inChr)
bool programmableCSCSupport
Programmable color space converter?
NTV2DeviceInfoList::const_iterator NTV2DeviceInfoListConstIter
bool multiFormat
Supports multiple video formats?
ULWord deviceIndex
Device index number – this will be phased out someday.
UWord numAnalogAudioOutputChannels
Total number of analog audio output channels.
UWord numHDMIAudioInputChannels
Total number of HDMI audio input channels.
UWord numVidInputs
Total number of video inputs – analog, digital, whatever.
bool has8KSupport
Supports 8K formats?
std::vector< AudioSourceEnum > NTV2AudioSourceList
bool NTV2DeviceCanDoIP(const NTV2DeviceID inDeviceID)
Private include file for all ajabase sources.
static bool GetDeviceAtIndex(const ULWord inDeviceIndexNumber, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device having the given zero-bas...
ULWord NTV2DeviceGetNumDMAEngines(const NTV2DeviceID inDeviceID)
#define HEX0N(__x__, __n__)
ostream & operator<<(ostream &inOutStr, const NTV2DeviceInfoList &inList)
NTV2AudioPhysicalFormatList::const_iterator NTV2AudioPhysicalFormatListConstIter
static string ToLower(const string &inStr)
NTV2AudioSampleRateList audioSampleRateList
My supported audio sample rates.
bool NTV2DeviceCanDoLTCInOnRefPort(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumOutputConverters(const NTV2DeviceID inDeviceID)
virtual NTV2DeviceID GetDeviceID(void)
bool operator==(const NTV2DeviceInfo &rhs) const
ULWord pciSlot
PCI slot (if applicable and/or known)
bool NTV2DeviceCanDoRGBPlusAlphaOut(const NTV2DeviceID inDeviceID)
NTV2AudioSampleRateList::const_iterator NTV2AudioSampleRateListConstIter
bool ltcOutSupport
Supports LTC output?
virtual CNTV2DeviceScanner & operator=(const CNTV2DeviceScanner &inDeviceScanner)
Assigns an existing CNTV2DeviceScanner instance to me.
UWord NTV2DeviceGetNumUpConverters(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
UWord numInputConverters
Total number of input converters.
bool ipSupport
Supports IP IO?
bool NTV2DeviceCanDo8KVideo(const NTV2DeviceID inDeviceID)
bool colorCorrectionSupport
Supports color correction?
bool sdi3GSupport
Supports 3G?
bool breakoutBoxSupport
Can support a breakout box?
std::vector< NTV2AudioPhysicalFormat > NTV2AudioPhysicalFormatList
I am an ordered list of NTV2AudioPhysicalFormat structs.
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
bool ltcInOnRefPort
Supports LTC on reference input?
NTV2AudioChannelsPerFrameList audioNumChannelsList
My supported number of audio channels per frame.
std::vector< AudioChannelsPerFrameEnum > NTV2AudioChannelsPerFrameList
virtual bool Open(const UWord inDeviceIndex)
Opens a local/physical AJA device so it can be monitored/controlled.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
bool stereoInSupport
Supports stereo input?
static bool IsDecimalDigit(const char inChr)
UWord numAESAudioInputChannels
Total number of AES audio input channels.
UWord GetNumAESAudioInputChannels(void)
NTV2DeviceID deviceID
Device ID/species (e.g., DEVICE_ID_KONA3G, DEVICE_ID_IOXT, etc.)
virtual bool Close(void)
Closes me, releasing host resources that may have been allocated in a previous Open call.
static bool IsAlphaNumeric(const char inStr)
static bool IsLegalSerialNumber(const std::string &inStr)
NTV2AudioBitsPerSampleList::const_iterator NTV2AudioBitsPerSampleListConstIter
UWord GetNumHDMIAudioInputChannels(void)
UWord numAudioStreams
Maximum number of independent audio streams.
bool dualLinkSupport
Supports dual-link?
bool NTV2DeviceCanDoQREZ(const NTV2DeviceID inDeviceID)
#define DECN(__x__, __n__)
UWord numEmbeddedAudioOutputChannels
Total number of embedded (SDI) audio output channels.
UWord GetNumHDMIAudioOutputChannels(void)
bool NTV2DeviceCanDoBreakoutBox(const NTV2DeviceID inDeviceID)