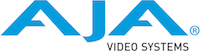 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
8 #ifndef NTV2DEVICESCANNER_H
9 #define NTV2DEVICESCANNER_H
218 static bool GetDeviceAtIndex (
const ULWord inDeviceIndexNumber,
CNTV2Card & outDevice);
235 static bool GetFirstDeviceWithName (
const std::string & inNameSubString,
CNTV2Card & outDevice);
244 static bool GetFirstDeviceWithSerial (
const std::string & inSerialStr,
CNTV2Card & outDevice);
252 static bool GetDeviceWithSerial (
const uint64_t inSerialNumber,
CNTV2Card & outDevice);
266 static bool GetFirstDeviceFromArgument (
const std::string & inArgument,
CNTV2Card & outDevice);
285 static std::string GetDeviceRefName (
CNTV2Card & inDevice);
291 static bool IsLegalDecimalNumber (
const std::string & inStr,
const size_t inMaxLength = 2);
292 static uint64_t IsLegalHexSerialNumber (
const std::string & inStr);
293 static bool IsHexDigit (
const char inChr);
294 static bool IsDecimalDigit (
const char inChr);
295 static bool IsAlphaNumeric (
const char inStr);
301 static bool IsAlphaNumeric (
const std::string & inStr);
307 static bool IsLegalSerialNumber (
const std::string & inStr);
337 virtual void ScanHardware (
void);
338 virtual void ScanHardware (
UWord inDeviceMask);
346 virtual inline size_t GetNumDevices (
void)
const {
return GetDeviceInfoList ().size (); }
354 virtual bool DeviceIDPresent (
const NTV2DeviceID inDeviceID,
const bool inRescan =
false);
363 virtual bool GetDeviceInfo (
const ULWord inDeviceIndexNumber,
NTV2DeviceInfo & outDeviceInfo,
const bool inRescan =
false);
382 virtual void SortDeviceInfoList (
void);
399 #endif // NTV2DEVICESCANNER_H
std::ostream & operator<<(std::ostream &inOutStr, const NTV2AudioSampleRateList &inList)
Streams the NTV2AudioSampleRateList to the given output stream in a human-readable format.
NTV2AudioSourceList audioOutSourceList
My supported audio output destinations (AES, etc.)
bool quarterExpandSupport
UWord numAnalogAudioInputChannels
Total number of analog audio input channels.
bool biDirectionalSDI
Supports Bi-directional SDI.
NTV2AudioBitsPerSampleList audioBitsPerSampleList
My supported audio bits-per-sample.
UWord numDownConverters
Total number of down-converters.
UWord numAnlgVidOutputs
Total number of analog video outputs.
bool has3GLevelConversion
Supports 3G Level Conversion?
This class is used to enumerate AJA devices that are attached and known to the local host computer.
UWord numHDMIVidInputs
Total number of HDMI inputs.
bool sdi12GSupport
Supports 12G?
NTV2DeviceInfoList::iterator NTV2DeviceInfoListIter
Declares common audio macros and structs used in the SDK.
uint64_t deviceSerialNumber
Unique device serial number.
bool ltcInSupport
Accepts LTC input?
NTV2AudioSourceList::const_iterator NTV2AudioSourceListConstIter
virtual const NTV2DeviceInfoList & GetDeviceInfoList(void) const
Returns an NTV2DeviceInfoList that can be "walked" using standard C++ vector iteration techniques.
UWord numHDMIVidOutputs
Total number of HDMI outputs.
std::vector< NTV2DeviceInfo > NTV2DeviceInfoList
I am an ordered list of NTV2DeviceInfo structs.
UWord numSerialPorts
Total number of serial ports.
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
virtual NTV2DeviceInfoList & GetDeviceInfoList(void)
Returns an NTV2DeviceInfoList that can be "walked" using standard C++ vector iteration techniques.
UWord numHDMIAudioOutputChannels
Total number of HDMI audio output channels.
UWord numAnlgVidInputs
Total number of analog video inputs.
NTV2AudioChannelsPerFrameList::const_iterator NTV2AudioChannelsPerFrameListConstIter
std::vector< AudioBitsPerSampleEnum > NTV2AudioBitsPerSampleList
std::vector< AudioSampleRateEnum > NTV2AudioSampleRateList
UWord numVidOutputs
Total number of video outputs – analog, digital, whatever.
std::string deviceIdentifier
Device name as seen in Control Panel, Watcher, Cables, etc.
NTV2AudioSourceList audioInSourceList
My supported audio input sources (AES, ADAT, etc.)
bool has4KSupport
Supports 4K formats?
UWord numOutputConverters
Total number of output converters.
Declares the CNTV2Card class.
NTV2AudioSourceList::iterator NTV2AudioSourceListIter
virtual ~CNTV2DeviceScanner()
AudioChannelsPerFrameEnum
UWord numDMAEngines
Total number of DMA engines.
I interrogate and control an AJA video/audio capture/playout device.
bool rgbAlphaOutputSupport
Supports RGB alpha channel?
UWord numAESAudioOutputChannels
Total number of AES audio output channels.
NTV2AudioPhysicalFormatList::iterator NTV2AudioPhysicalFormatListIter
bool has2KSupport
Supports 2K formats?
bool proResSupport
Supports ProRes?
bool stereoOutSupport
Supports stereo output?
UWord numUpConverters
Total number of up-converters.
UWord numEmbeddedAudioInputChannels
Total number of embedded (SDI) audio input channels.
bool programmableCSCSupport
Programmable color space converter?
NTV2DeviceInfoList::const_iterator NTV2DeviceInfoListConstIter
bool multiFormat
Supports multiple video formats?
NTV2AudioSampleRateList::iterator NTV2AudioSampleRateListIter
ULWord deviceIndex
Device index number – this will be phased out someday.
UWord numAnalogAudioOutputChannels
Total number of analog audio output channels.
UWord numHDMIAudioInputChannels
Total number of HDMI audio input channels.
UWord numVidInputs
Total number of video inputs – analog, digital, whatever.
bool has8KSupport
Supports 8K formats?
std::vector< AudioSourceEnum > NTV2AudioSourceList
NTV2AudioPhysicalFormatList::const_iterator NTV2AudioPhysicalFormatListConstIter
virtual size_t GetNumDevices(void) const
Returns the number of AJA devices found on the local host.
NTV2AudioSampleRateList audioSampleRateList
My supported audio sample rates.
NTV2AudioBitsPerSampleList::iterator NTV2AudioBitsPerSampleListIter
bool operator==(const NTV2DeviceInfo &rhs) const
ULWord pciSlot
PCI slot (if applicable and/or known)
NTV2AudioSampleRateList::const_iterator NTV2AudioSampleRateListConstIter
bool ltcOutSupport
Supports LTC output?
NTV2AudioChannelsPerFrameList::iterator NTV2AudioChannelsPerFrameListIter
UWord numInputConverters
Total number of input converters.
bool ipSupport
Supports IP IO?
bool colorCorrectionSupport
Supports color correction?
bool sdi3GSupport
Supports 3G?
bool breakoutBoxSupport
Can support a breakout box?
struct NTV2DeviceInfo NTV2DeviceInfo
std::vector< NTV2AudioPhysicalFormat > NTV2AudioPhysicalFormatList
I am an ordered list of NTV2AudioPhysicalFormat structs.
bool ltcInOnRefPort
Supports LTC on reference input?
NTV2AudioChannelsPerFrameList audioNumChannelsList
My supported number of audio channels per frame.
std::vector< AudioChannelsPerFrameEnum > NTV2AudioChannelsPerFrameList
bool stereoInSupport
Supports stereo input?
UWord numAESAudioInputChannels
Total number of AES audio input channels.
NTV2DeviceID deviceID
Device ID/species (e.g., DEVICE_ID_KONA3G, DEVICE_ID_IOXT, etc.)
NTV2AudioBitsPerSampleList::const_iterator NTV2AudioBitsPerSampleListConstIter
UWord numAudioStreams
Maximum number of independent audio streams.
bool dualLinkSupport
Supports dual-link?
UWord numEmbeddedAudioOutputChannels
Total number of embedded (SDI) audio output channels.