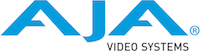 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
11 #if defined (MSWindows)
13 #elif defined (AJAMac)
15 #elif defined (AJALinux)
17 #elif defined (AJABareMetal)
58 #if defined(NTV2_INCLUDE_DEVICE_CAPABILITIES_API)
221 return itms.find(
ULWord(inChannel)) != itms.end();
225 return itms.find(
ULWord(inMode)) != itms.end();
229 return itms.find(
ULWord(inMode)) != itms.end();
233 return itms.find(
ULWord(inPF)) != itms.end();
237 return itms.find(
ULWord(inSrc)) != itms.end();
241 return itms.find(
ULWord(inDest)) != itms.end();
245 return itms.find(
ULWord(inVF)) != itms.end();
249 return itms.find(
ULWord(inWgtID)) != itms.end();
254 #endif // defined(NTV2_INCLUDE_DEVICE_CAPABILITIES_API)
259 #if defined (MSWindows)
261 #elif defined (AJAMac)
263 #elif defined (AJALinux)
265 #elif defined (AJABareMetal)
288 const std::string & inHostName = std::string());
371 #if defined(NTV2_INCLUDE_DEVICE_CAPABILITIES_API)
378 #endif // defined(NTV2_INCLUDE_DEVICE_CAPABILITIES_API)
379 #if !defined(NTV2_DEPRECATE_16_3)
403 #endif // defined(NTV2_DEPRECATE_16_3)
525 const ULWord inCardOffsetBytes,
526 const ULWord inSegmentByteCount,
527 const ULWord inNumSegments,
528 const ULWord inSegmentHostPitch,
529 const ULWord inSegmentCardPitch);
547 const ULWord * pFrameBuffer,
548 const ULWord inOffsetBytes,
549 const ULWord inSegmentByteCount,
550 const ULWord inNumSegments,
551 const ULWord inSegmentHostPitch,
552 const ULWord inSegmentCardPitch);
567 ULWord segmentTargetPitch,
577 const bool inToHost);
580 const bool inToHost);
599 const ULWord inOffsetBytes,
600 const ULWord inByteCount);
618 const ULWord * pInAudioBuffer,
619 const ULWord inOffsetBytes,
620 const ULWord inByteCount);
772 const UWord inEndFrameNumber,
787 uint64_t & outAddress, uint64_t & outLength);
790 bool & outMultiFmt,
bool & outQuad,
bool & outQuadQuad,
bool & outSquares,
bool & outTSI,
791 uint64_t & outAddress, uint64_t & outLength);
838 #define AJA_RETAIL_DEFAULT true
839 #else // else !defined (AJAMac)
840 #define AJA_RETAIL_DEFAULT false
841 #endif // !defined (AJAMac)
1434 #if !defined(NTV2_DEPRECATE_16_3)
1437 #endif // defined(NTV2_DEPRECATE_16_3)
1438 #if !defined(NTV2_DEPRECATE_16_2)
1445 #endif // !defined(NTV2_DEPRECATE_16_2)
1446 #if !defined(NTV2_DEPRECATE_16_0)
1450 #define Set425FrameEnable SetTsiFrameEnable // Replace calls to Set425FrameEnable with calls to SetTsiFrameEnable instead
1451 #define Get425FrameEnable GetTsiFrameEnable // Replace calls to Get425FrameEnable with calls to GetTsiFrameEnable instead
1452 #endif // NTV2_DEPRECATE_16_0
1845 const NTV2AudioSystem inAudioSystem,
const bool inCaptureBuffer =
false);
2516 #if !defined(NTV2_DEPRECATE_16_0)
2523 #endif // !defined(NTV2_DEPRECATE_16_0)
2524 #if !defined(NTV2_DEPRECATE_16_1)
2530 #endif // !defined(NTV2_DEPRECATE_16_1)
2531 #if !defined(NTV2_DEPRECATE_16_3)
2533 #endif // !defined(NTV2_DEPRECATE_16_1)
2534 #if !defined(NTV2_DEPRECATE_17_0)
2536 #endif // !defined(NTV2_DEPRECATE_17_0)
2727 #if !defined(NTV2_DEPRECATE_16_3)
2730 #endif // !defined(NTV2_DEPRECATE_16_3)
2739 AJA_VIRTUAL bool ProgramMainFlash(
const std::string & inFileName,
const bool bInForceUpdate =
false,
const bool bInQuiet =
false);
2818 #if !defined(NTV2_DEPRECATE_16_0)
2824 #endif // !defined(NTV2_DEPRECATE_16_0)
2826 #if !defined(NTV2_DEPRECATE_17_0)
2837 #endif // !defined(NTV2_DEPRECATE_17_0)
3351 #if !defined (NTV2_DEPRECATE_16_0)
3354 #endif // NTV2_DEPRECATE_16_0
3413 const UWord inFrameCount = 7,
3415 const ULWord inOptionFlags = 0,
3416 const UByte inNumChannels = 1,
3417 const UWord inStartFrameNumber = 0,
3418 const UWord inEndFrameNumber = 0);
3463 const UWord inFrameCount = 7,
3465 const ULWord inOptionFlags = 0,
3466 const UByte inNumChannels = 1,
3467 const UWord inStartFrameNumber = 0,
3468 const UWord inEndFrameNumber = 0);
3701 #define NTV2_STREAM_SUCCESS(__status__) (__status__ == NTV2_STREAM_SUCCESS)
3702 #define NTV2_STREAM_FAIL(__status__) (__status__ != NTV2_STREAM_SUCCESS)
3782 #if defined(READREGMULTICHANGE)
3794 #endif // defined(READREGMULTICHANGE)
4426 #if !defined(R2_DEPRECATE)
4680 #if !defined(NTV2_DEPRECATE_17_0)
4682 #endif // !defined(NTV2_DEPRECATE_17_0)
4741 #if !defined(R2_DEPRECATE)
5249 #if !defined(NTV2_DEPRECATE_16_3)
5254 #endif // !defined(NTV2_DEPRECATE_16_3)
5751 const bool inVancY,
const bool inVancC,
5752 const bool inHancY,
const bool inHancC);
5865 const bool inVancY,
const bool inVancC,
5866 const bool inHancY,
const bool inHancC);
5942 uint64_t & outF1StartAddr, uint64_t & outF1EndAddr,
5943 uint64_t & outF2StartAddr, uint64_t & outF2EndAddr);
6435 #if !defined(NTV2_DEPRECATE_16_1)
6438 #endif // NTV2_DEPRECATE_16_1
6477 #if !defined(NTV2_DEPRECATE_16_2)
6484 #endif // !defined(NTV2_DEPRECATE_16_2)
6486 #define SetTablesToHardware LoadLUTTables
6487 #define GetTablesFromHardware GetLUTTables
6604 bool HasFrameIndex (
const UWord inIndex)
const {
return mFrameTags.find(inIndex) != mFrameTags.end();}
6608 inline bool HasTag (
const UWord inIndex)
const {
return GetTagCount(inIndex) > 0;}
6609 inline bool HasConflicts (
const UWord inIndex)
const {
return GetTagCount(inIndex) > 1;}
6610 inline ULWord GetIntrinsicFrameByteCount (
void)
const {
return mIntrinsicSize;}
6629 std::ostream &
RawDump (std::ostream & oss)
const;
6636 std::ostream &
DumpBlocks (std::ostream & oss)
const;
6649 bool TagMemoryBlock (
const uint64_t inStartAddr,
const uint64_t inByteLength,
const std::string & inTag)
6655 typedef std::pair<UWord, NTV2StringSet> FrameTag;
6656 typedef std::map<UWord, NTV2StringSet> FrameTags;
6657 typedef FrameTags::const_iterator FrameTagsConstIter;
6660 FrameTags mFrameTags;
6666 #endif // NTV2CARD_H
ULWord GetHDMIVersion(void)
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual ULWord DeviceGetAudioFrameBuffer(void)
@ kDeviceCanDoMSI
True if device DMA hardware supports MSI (Message Signaled Interrupts).
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
CNTV2Card CNTV2TestPattern
@ kDeviceGetNumVideoInputs
The number of SDI video inputs on the device.
virtual bool SetSDIInLevelBtoLevelAConversion(const NTV2ChannelSet &inSDIInputs, const bool inEnable)
Enables or disables 3G level B to 3G level A conversion at the SDI input(s).
virtual bool GetConnections(NTV2XptConnections &outConnections)
Answers with the device's current widget routing connections.
virtual bool IsAudioOutputRunning(const NTV2AudioSystem inAudioSystem, bool &outIsRunning)
Answers whether or not the playout side of the given NTV2AudioSystem is currently running.
virtual bool SetMixerFGMatteEnabled(const UWord inWhichMixer, const bool inIsEnabled)
Answers if the given mixer/keyer's foreground matte is enabled or not.
virtual bool StopAudioInput(const NTV2AudioSystem inAudioSystem)
Stops the capture side of the given NTV2AudioSystem, and resets the capture position (i....
@ kDeviceHasBiDirectionalSDI
True if device SDI connectors are bi-directional.
virtual bool SetColorSpaceMakeAlphaFromKey(const bool inMakeAlphaFromKey, const NTV2Channel inChannel=NTV2_CHANNEL1)
Specifies whether or not the given CSC will produce alpha channel data from its key input.
bool CanDoAudio2Channels(void)
virtual bool IS_INPUT_SPIGOT_INVALID(const UWord inInputSpigot) const
static NTV2VideoFormat GetNTV2VideoFormat(NTV2FrameRate frameRate, UByte inputGeometry, bool progressiveTransport, bool isThreeG, bool progressivePicture=false)
@ kDeviceCanDoAudio6Channels
@ kDeviceCanDoPIO
True if device supports Programmed I/O.
virtual bool GetDieTemperature(double &outTemp, const NTV2DieTempScale inTempScale=NTV2DieTempScale_Celsius)
Reads the current die temperature of the device.
UWord GetNumAESAudioOutputChannels(void)
virtual bool SetHDMIInAudioChannel34Swap(const bool inIsSwapped, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the given HDMI input's audio channel 3/4 swap state.
virtual bool GetHDMIHDREnabled(void)
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
virtual bool GetSDIInput3GPresent(bool &outValue, const NTV2Channel channel)
virtual bool EnableHDMIHDR(const bool inEnableHDMIHDR)
Enables or disables HDMI HDR.
bool TagMemoryBlock(const ULWord inStartAddr, const ULWord inByteLength, const std::string &inTag)
#define NTV2VANCModeFromBools(_tall_, _taller_)
virtual bool GetHDMIOutDownstreamBitDepth(NTV2HDMIBitDepth &outValue)
UWord GetNumUpConverters(void)
@ kDeviceCanDoStackedAudio
True if device uses a "stacked" arrangement of its audio buffers.
virtual bool DMABufferUnlock(const NTV2Buffer &inBuffer)
Unlocks the given host buffer that was previously locked using CNTV2Card::DMABufferLock.
bool CanDoLTCInOnRefPort(void)
virtual bool GetSDIRelayPosition(NTV2RelayState &outValue, const UWord inIndex0)
Answers if the bypass relays between connectors 1/2 or 3/4 are currently in bypass or routing the sig...
virtual bool Download12BitLUTToHW(const NTV2DoubleArray &inRedLUT, const NTV2DoubleArray &inGreenLUT, const NTV2DoubleArray &inBlueLUT, const NTV2Channel inLUT, const int inBank)
virtual bool SetFrameBufferSize(const NTV2Framesize inSize)
Sets the device's intrinsic frame buffer size.
@ kDeviceGetNumMixers
The number of mixer/keyer widgets on the device.
virtual bool GetHDMIOutTsiIO(bool &tsiEnabled)
NTV2ColorCorrectionHostAccessBank
bool CanDoCustomAux(void)
virtual bool GetSDIOut12GEnable(const NTV2Channel inChannel, bool &outIsEnabled)
virtual bool SetAudioOutputDelay(const NTV2AudioSystem inAudioSystem, const ULWord inDelay)
Sets the audio output delay for the given Audio System on the device.
virtual bool GetStereoCompressorFlipLeftHorz(ULWord &outValue)
@ kDeviceGetNumFrameStores
The number of FrameStores on the device.
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
virtual bool GetHDMIOutPrefer420(bool &outValue)
virtual bool GetInputAudioChannelPairsWithPCM(const NTV2Channel inSDIInputConnector, NTV2AudioChannelPairs &outChannelPairs)
For the given SDI input (specified as a channel number), returns the set of audio channel pairs that ...
virtual ULWord StreamBufferQueue(const NTV2Channel inChannel, NTV2_POINTER inBuffer, ULWord64 bufferCookie, NTV2StreamBuffer &status)
Queue a buffer to the stream. The bufferCookie is a user defined identifier of the buffer used by the...
virtual bool DMAStreamStop(const NTV2Channel inChannel, const bool inToHost)
virtual bool GetRS422Parity(const NTV2Channel inSerialPort, NTV2_RS422_PARITY &outParity)
Answers with the current parity control for the specified RS422 serial port.
virtual bool WriteSDSaturationAdjustment(const ULWord inNewValue)
std::map< ULWord, ULWord > NTV2RegisterValueMap
A mapping of distinct NTV2RegisterNumbers to their corresponding ULWord values.
virtual bool DeviceCanDoAudioMixer(void)
virtual bool LoadLUTTables(const NTV2DoubleArray &inRedLUT, const NTV2DoubleArray &inGreenLUT, const NTV2DoubleArray &inBlueLUT)
Writes the LUT tables.
virtual bool SetHDMIHDRStaticMetadataDescriptorID(const uint8_t inSMDId)
Declares the MSWindows-specific flavor of CNTV2DriverInterface.
@ kDeviceCanMeasureTemperature
True if device can measure its FPGA die temperature.
std::set< NTV2InputXptID > NTV2InputCrosspointIDSet
virtual ULWord GetSerialNumberHigh(void)
virtual bool ReadRegisters(NTV2RegisterReads &inOutValues)
Reads the register(s) specified by the given NTV2RegInfo sequence.
virtual bool LoadDynamicDevice(const NTV2DeviceID inDeviceID)
Quickly, dynamically loads the given device ID firmware.
virtual bool GetAncRegionOffsetFromBottom(ULWord &outByteOffsetFromBottom, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All)
Answers with the byte offset to the start of an ancillary data region within a device frame buffer,...
virtual bool GetLTCInputPresent(bool &outIsPresent, const UWord inLTCInputNdx=0)
Answers whether or not a valid analog LTC signal is being applied to the device's analog LTC input co...
virtual bool GetHDMIHDRWhitePointX(uint16_t &outWhitePointX)
Answers with the Display Mastering data for White Point X as defined in SMPTE ST 2086....
virtual bool EnableHDMIOutCenterCrop(const bool inEnable)
Controls the 4k/2k -> UHD/HD HDMI center cropping feature.
virtual bool SetStandard(NTV2Standard inValue, NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool GetHDMIOutStatus(NTV2HDMIOutputStatus &outStatus)
Answers with the current HDMI output status.
virtual bool SetStereoCompressorRightSource(const NTV2OutputCrosspointID inNewValue)
UWord GetTotalNumAudioSystems(void)
bool CanDoStereoOut(void)
UWord GetNumAnalogAudioOutputChannels(void)
virtual bool GetColorSpaceCustomCoefficients12Bit(NTV2CSCCustomCoeffs &outCustomCoefficients, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool GetHDMIInAudioSampleRateConverterEnable(bool &outIsEnabled, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool GetRegisterWriteMode(NTV2RegisterWriteMode &outValue, const NTV2Channel inFrameStore=NTV2_CHANNEL1)
Answers with the FrameStore's current NTV2RegisterWriteMode setting, which determines when CNTV2Card:...
@ kDeviceHasSPIv4
Use kDeviceGetSPIVersion instead.
NTV2LutBitDepth
This specifies the LUT bit depth.
@ kDeviceCanDoIP
True if device has SFP connectors.
virtual bool GetOutputFieldID(const NTV2Channel channel, NTV2FieldID &outFieldID)
Returns the current field ID of the specified output channel.
NTV2HDMIBitDepth
Indicates or specifies the HDMI video bit depth.
bool CanDoAudio8Channels(void)
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
NTV2OutputDestination
Identifies a specific video output destination.
virtual bool SetWarmBootFirmwareReload(bool enable)
virtual bool SetSDIOut2Kx1080Enable(const NTV2Channel inChannel, const bool inIsEnabled)
virtual bool AncInsertSetEnable(const UWord inSDIOutput, const bool inIsEnabled)
Enables or disables the given SDI output's Anc inserter frame buffer reads. (Call NTV2DeviceCanDoCust...
virtual bool SetUCAutoLine21(const ULWord inValue)
virtual bool WaitForOutputFieldID(const NTV2FieldID inFieldID, const NTV2Channel inChannel=NTV2_CHANNEL1)
Efficiently sleeps the calling thread/process until the next output VBI for the given field and outpu...
virtual bool SetQuadFrameEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables quad-frame mode on the device.
virtual bool SetAudioOutputMonitorSource(const NTV2AudioChannelPair inChannelPair, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the audio monitor output source to a specified audio system and channel pair....
@ kDeviceCanDoHDMIMultiView
True if device can rasterize 4 HD signals into a single HDMI output.
bool CanDoRateConvert(void)
virtual bool SetHDMIOutVideoStandard(const NTV2Standard inNewValue)
virtual bool StartAudioInput(const NTV2AudioSystem inAudioSystem, const bool inWaitForVBI=false)
Starts the capture side of the given NTV2AudioSystem, writing incoming audio samples into the Audio S...
virtual bool GetForce64(ULWord *force64)
@ kDeviceCanDoVideoProcessing
True if device can do video processing.
UWord GetNum2022ChannelsSFP2(void)
virtual bool SetSDIOut3GEnable(const NTV2Channel inChannel, const bool inEnable)
virtual bool KickSDIWatchdog(void)
Restarts the countdown timer to prevent the watchdog timer from timing out.
virtual bool AutoCirculateSetActiveFrame(const NTV2Channel inChannel, const ULWord inNewActiveFrame)
Immediately changes the Active Frame for the given channel.
bool HasLEDAudioMeters(void)
bool CanDoAnalogVideoIn(void)
bool HasBiDirectionalSDI(void)
virtual bool SetLHIVideoDACMode(NTV2LHIVideoDACMode value)
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
virtual bool Set4kSquaresEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 "2K quadrants" mode for the given FrameStore bank on the device....
virtual bool GetHDMIOutColorSpace(NTV2HDMIColorSpace &outValue)
virtual bool HevcReadRegister(ULWord address, ULWord *pValue, ULWord mask=0xffffffff, ULWord shift=0)
Read an hevc register.
@ kNTV2EnumsID_VideoFormat
Identifies the NTV2VideoFormat enumerated type.
virtual bool SetSDIWatchdogEnable(const bool inEnable, const UWord inIndex0)
Sets the connector pair relays to be under watchdog timer control or manual control.
virtual bool SetFrameBufferQuarterSizeMode(NTV2Channel inChannel, NTV2QuarterSizeExpandMode inValue)
virtual bool GetSDIOut3GEnable(const NTV2Channel inChannel, bool &outIsEnabled)
virtual bool GetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, NTV2AudioSource &outAudioSource, NTV2EmbeddedAudioInput &outEmbeddedSource)
Answers with the device's current NTV2AudioSource (and also possibly its NTV2EmbeddedAudioInput) for ...
virtual bool GetRegisterBaseAddress(ULWord regNumber, ULWord **pRegAddress)
virtual bool SetEmbeddedAudioClock(const NTV2EmbeddedAudioClock inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2EmbeddedAudioClock setting for the given NTV2AudioSystem.
virtual bool GetVideoHOffset(int &outHOffset, const UWord inOutputSpigot=0)
Answers with the current horizontal timing offset, in pixels, for the given SDI output connector.
virtual bool AcquireMailBoxLock(void)
virtual bool AncInsertInit(const UWord inSDIOutput, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Standard inStandard=NTV2_STANDARD_INVALID)
Initializes the given SDI output's Anc inserter for custom Anc packet insertion. (Call NTV2DeviceCanD...
virtual bool SetRP188Mode(const NTV2Channel inChannel, const NTV2_RP188Mode inMode)
Sets the current RP188 mode – NTV2_RP188_INPUT or NTV2_RP188_OUTPUT – for the given channel.
virtual bool DMAWriteSegments(const ULWord inFrameNumber, const ULWord *pFrameBuffer, const ULWord inOffsetBytes, const ULWord inSegmentByteCount, const ULWord inNumSegments, const ULWord inSegmentHostPitch, const ULWord inSegmentCardPitch)
Performs a segmented data transfer from the host to the AJA device.
virtual bool SetAudioMixerInputChannelsMute(const NTV2AudioMixerInput inMixerInput, const NTV2AudioChannelsMuted16 inMutes)
Mutes (or enables) the given output audio channel of the Audio Mixer.
virtual bool GetVPIDValidA(const NTV2Channel inChannel)
@ kDeviceGetUFCVersion
The version number of the UFC on the device.
bool TagAudioBuffers(CNTV2Card &inDevice, const bool inMarkStoppedAudioBuffersFree)
std::set< std::string > NTV2StringSet
UWord GetNumAudioSystems(void)
@ kDeviceHasXptConnectROM
True if device has a crosspoint connection ROM (New in SDK 17.0)
virtual bool AncExtractGetWriteInfo(const UWord inSDIInput, uint64_t &outF1StartAddr, uint64_t &outF1EndAddr, uint64_t &outF2StartAddr, uint64_t &outF2EndAddr)
Answers with the given SDI input's current Anc extractor info. (Call NTV2DeviceCanDoCustomAnc to dete...
UWord GetDownConverterDelay(void)
virtual bool GetColorSpaceRGBBlackRange(NTV2_CSC_RGB_Range &outRange, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current RGB range being used by a given CSC.
virtual bool S2110DeviceAncFromXferBuffers(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &inOutXferInfo)
bool HasColorSpaceConverterOnChannel2(void)
@ kDeviceGetNumDownConverters
The number of down-converters on the device.
virtual bool SetAudioMixerInputChannelSelect(const NTV2AudioMixerInput inMixerInput, const NTV2AudioChannelPair inChannelPair)
Specifies the Audio Channel Pair that will drive the given input of the Audio Mixer.
virtual bool SetUCPassLine21(const ULWord inValue)
@ kDeviceCanDoHDV
True if device can squeeze/stretch between 1920x1080 and 1440x1080.
UWord GetNumCrossConverters(void)
virtual bool Set3DLUTTableLocation(const ULWord inFrameNumber, ULWord inLUTIndex=0)
virtual bool GetHDMIHDRDolbyVisionEnabled(void)
virtual bool GetColorCorrectionOutputBank(const NTV2Channel inLUTWidget, ULWord &outBank)
Answers with the current LUT bank in use for the given LUT.
bool CanDoAudioMixer(void)
virtual bool FindUnallocatedFrames(const UWord inFrameCount, LWord &outStartFrame, LWord &outEndFrame, const NTV2Channel inFrameStore=NTV2_CHANNEL_INVALID)
Returns the device frame buffer numbers of the first unallocated contiguous band of frame buffers hav...
virtual bool GetMixerSyncStatus(const UWord inWhichMixer, bool &outIsSyncOK)
Returns the current sync state of the given mixer/keyer.
virtual bool SetSDIOutVPID(const ULWord inValueA, const ULWord inValueB, const UWord inOutputSpigot=NTV2_CHANNEL1)
@ kDeviceCanDoSDIErrorChecks
True if device can perform SDI error checking.
virtual bool GetColorSpaceVideoKeySyncFail(bool &outVideoKeySyncFail, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers whether or not the video signal present at the CSC’s Key Input is in sync with the video sign...
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=false)
Applies the given routing table to the AJA device.
virtual bool GetHDMIOutSampleStructure(NTV2HDMISampleStructure &outValue)
virtual bool GetMixerBGInputControl(const UWord inWhichMixer, NTV2MixerKeyerInputControl &outInputControl)
Returns the current background input control value for the given mixer/keyer.
virtual bool ReadHDSaturationAdjustmentCr(ULWord &outValue)
virtual bool SetColorSpaceCustomCoefficients12Bit(const NTV2CSCCustomCoeffs &inCustomCoefficients, const NTV2Channel inChannel=NTV2_CHANNEL1)
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
NTV2HDMIColorSpace
Indicates or specifies HDMI Color Space.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
virtual bool IsProgressiveStandard(bool &outIsProgressive, NTV2Channel inChannel=NTV2_CHANNEL1)
NTV2AudioMixerInput
Identifies the Audio Mixer's audio inputs.
virtual bool GetDieVoltage(double &outVoltage)
Reads the current "Vcc" voltage of the device.
virtual bool IS_CHANNEL_INVALID(const NTV2Channel inChannel) const
virtual bool SetColorCorrectionSaturation(const NTV2Channel inChannel, const ULWord inValue)
virtual bool GetStandard(NTV2Standard &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
NTV2AudioChannelOctets::const_iterator NTV2AudioChannelOctetsConstIter
Handy const iterator to iterate over a set of distinct NTV2AudioChannelOctet values.
virtual bool GetSDIOutputStandard(const UWord inOutputSpigot, NTV2Standard &outValue)
Answers with the current video standard of the given SDI output spigot.
virtual bool GetVPIDRGBRange(NTV2VPIDRGBRange &outValue, const NTV2Channel inChannel)
virtual bool SetFrameBufferOrientation(const NTV2Channel inChannel, const NTV2FBOrientation inValue)
Sets the frame buffer orientation for the given NTV2Channel.
@ kDeviceGetNumAnalogAudioInputChannels
The number of analog audio input channels on the device.
virtual bool DeviceCanDoHDMIQuadRasterConversion(void)
virtual bool IsBreakoutBoardConnected(void)
std::set< NTV2VideoFormat > NTV2VideoFormatSet
A set of distinct NTV2VideoFormat values.
virtual bool StopAudioOutput(const NTV2AudioSystem inAudioSystem)
Stops the playout side of the given NTV2AudioSystem, parking the "Read Head" at the start of the play...
virtual bool WriteHDContrastAdjustment(const ULWord inNewValue)
virtual bool Write12BitLUTTables(const UWordSequence &inRedLUT, const UWordSequence &inGreenLUT, const UWordSequence &inBlueLUT)
virtual bool SetSmpte372(ULWord inValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables the device's SMPTE-372 (dual-link) mode (used for older 3G-levelB-capable devices...
virtual bool SetStereoCompressorStandard(const NTV2Standard inNewValue)
bool CanDoAudioDelay(void)
virtual bool GetHDMIInBitDepth(NTV2HDMIBitDepth &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current bit depth setting.
virtual Word GetDeviceVersion(void)
Answers with this device's version number.
virtual bool GetAudioBufferSize(NTV2AudioBufferSize &outSize, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Retrieves the size of the input or output audio buffer being used for a given Audio System on the AJA...
virtual bool SetMixerCoefficient(const UWord inWhichMixer, const ULWord inMixCoefficient)
Sets the current mix coefficient of the given mixer/keyer.
virtual bool GetEnable4KPSFOutMode(bool &outIsEnabled)
@ kDeviceGetNumEmbeddedAudioOutputChannels
The number of SDI-embedded output audio channels supported by the device.
bool SoftwareCanChangeFrameBufferSize(void)
virtual bool GetVideoVOffset(int &outVOffset, const UWord inOutputSpigot=0)
Answers with the current vertical timing offset, in lines, for the given SDI output connector.
virtual bool ProgramMainFlash(const std::string &inFileName, const bool bInForceUpdate=false, const bool bInQuiet=false)
virtual bool SetMixerBGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the background input control value for the given mixer/keyer.
virtual bool GetSDI1OutHTiming(ULWord *value)
virtual bool ReadSDIInVPID(const NTV2Channel inSDIInput, ULWord &outValueA, ULWord &outValueB)
virtual bool SetHDMIOutRange(const NTV2HDMIRange inNewValue)
virtual bool GetHDMIOutVideoFPS(NTV2FrameRate &outValue)
virtual bool HevcDebugInfo(HevcDeviceDebug *pDebug)
Get debug data from the hevc device.
virtual bool SetSuspendHostAudio(const bool inSuspend)
Suspends or resumes host OS audio (e.g. CoreAudio on MacOS) for the AJA device.
@ kDeviceCanDoAudio192K
True if Audio System(s) support a 192kHz sample rate.
virtual bool IsXilinxProgrammed()
virtual bool SetRP188Data(const NTV2Channel inSDIOutput, const NTV2_RP188 &inRP188Data)
Writes the raw RP188 data into the DBB/Low/Hi registers for the given SDI output. These values are la...
bool HasRetailSupport(void)
virtual bool Load1DLUTTable(const NTV2Channel inChannel)
bool CanDoDSKMode(const NTV2DSKMode inMode)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool WriteSDCbOffsetAdjustment(const ULWord inNewValue)
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
virtual bool SetFrameRate(NTV2FrameRate inNewValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the AJA device's frame rate.
virtual bool GetQuadFrameEnable(bool &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the device's current quad-frame mode, whether it's enabled or not.
virtual bool GetMixerMode(const UWord inWhichMixer, NTV2MixerKeyerMode &outMode)
Returns the current operating mode of the given mixer/keyer.
virtual bool ReadAnalogLTCInput(const UWord inLTCInput, RP188_STRUCT &outRP188Data)
Reads the current contents of the device's analog LTC input registers.
virtual bool GetVPIDValidB(const NTV2Channel inChannel)
virtual bool SetConverterInRate(const NTV2FrameRate inValue)
@ kDeviceIsSupported
True if device is supported by this SDK.
bool CanDoSDIErrorChecks(void)
virtual bool DisableOutputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Prevents the CNTV2Card instance from waiting for and responding to vertical blanking interrupts origi...
virtual bool GetInputVideoSelect(NTV2InputVideoSelect &outInputSelect)
virtual bool GetColorCorrectionHostAccessBank(NTV2ColorCorrectionHostAccessBank &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool GetHDMIOutAudioChannel34Swap(bool &outIsSwapped, const NTV2Channel inWhichHDMIOut=NTV2_CHANNEL1)
Answers with the HDMI output's current audio channel 3/4 swap setting.
virtual bool HevcSendCommand(HevcDeviceCommand *pCommand)
Send a command to the hevc device. See the hevc codec documentation for details on commands.
virtual bool AutoCirculatePreRoll(const NTV2Channel inChannel, const ULWord inPreRollFrames)
Tells AutoCirculate how many frames to skip before playout starts for the given channel.
virtual bool GetCurrentInterruptMasks(NTV2InterruptMask &outIntMask1, NTV2Interrupt2Mask &outIntMask2)
virtual bool GetStereoCompressorFlipMode(ULWord &outValue)
virtual bool EnableHDMIOutUserOverride(const bool inEnable)
Enables or disables override of HDMI parameters.
virtual bool GetAudioMixerInputAudioSystem(const NTV2AudioMixerInput inMixerInput, NTV2AudioSystem &outAudioSystem)
Answers with the Audio System that's currently driving the given input of the Audio Mixer.
virtual bool DmaP2PTargetFrame(NTV2Channel channel, ULWord frameNumber, ULWord frameOffset, PCHANNEL_P2P_STRUCT pP2PData)
DirectGMA p2p transfers (not GPUDirect: see DMABufferLock)
@ kDeviceCanDoCustomAux
True if device supports HDMI AUX data insertion/extraction.
virtual bool S2110DeviceAncFromBuffers(const NTV2Channel inChannel, NTV2Buffer &ancF1, NTV2Buffer &ancF2)
@ kDeviceHasGenlockv3
True if device has version 3 genlock hardware and/or firmware.
virtual bool GetVideoLimiting(NTV2VideoLimiting &outValue)
virtual bool SetHDMIOutVideoFPS(const NTV2FrameRate inNewValue)
@ kDeviceCanDoEnhancedCSC
True if device has enhanced CSCs.
@ kDeviceHasHeadphoneJack
True if device has a headphone jack.
virtual bool DMAWrite(const ULWord inFrameNumber, const ULWord *pFrameBuffer, const ULWord inOffsetBytes, const ULWord inByteCount)
Transfers data from the host to the AJA device.
virtual bool GetHDMIOutDecimateMode(bool &outIsEnabled)
virtual bool GetAudioWrapAddress(ULWord &outWrapAddress, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the wrap address, the threshold at which input/record or out...
virtual bool DMARead(const ULWord inFrameNumber, ULWord *pFrameBuffer, const ULWord inOffsetBytes, const ULWord inByteCount)
Transfers data from the AJA device to the host.
virtual bool SetConverterPulldown(const ULWord inValue)
virtual bool GetRP188BypassSource(const NTV2Channel inSDIOutput, UWord &outSDIInput)
For the given SDI output that's in RP188 bypass mode (E-E), answers with the SDI input that's current...
virtual bool GetHDMIOut3DMode(NTV2HDMIOut3DMode &outValue)
virtual bool SetSDIOut6GEnable(const NTV2Channel inChannel, const bool inEnable)
virtual NTV2VideoFormat GetAnalogInputVideoFormat(void)
Returns the video format of the signal that is present on the device's analog video input.
Implements the MacOS-specific flavor of CNTV2DriverInterface.
virtual bool ReadProcAmpC1CRAdjustment(ULWord &outValue)
virtual bool GetEncodedAudioMode(NTV2EncodedAudioMode &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
virtual bool GetEnable4KDCYCC444Mode(bool &outIsEnabled)
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Standard st, const NTV2FrameGeometry fg, const NTV2Channel inChannel=NTV2_CHANNEL1)
NTV2HDMIAudioChannels
Indicates or specifies the HDMI audio channel count.
CNTV2Card CNTV2ColorCorrection
bool GetTagsForFrameIndex(const UWord inIndex, NTV2StringSet &outTags) const
Answers with the list of tags for the given frame number.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
virtual bool DeviceIsDNxIV(void)
virtual bool ReadHDCbOffsetAdjustment(ULWord &outValue)
virtual bool DMAReadAnc(const ULWord inFrameNumber, NTV2Buffer &outAncF1Buffer, NTV2Buffer &outAncF2Buffer=NULL_POINTER, const NTV2Channel inChannel=NTV2_CHANNEL1)
Transfers the contents of the ancillary data buffer(s) from a given frame on the AJA device to the ho...
NTV2LutType
This specifies what function(s) are currently loaded into the LUTs.
@ kDeviceCanDo4KVideo
True if the device can handle 4K/UHD video.
@ kNTV2EnumsID_OutputDest
Identifies the NTV2OutputDest enumerated type.
@ kDeviceGetNumReferenceVideoInputs
The number of external reference video inputs on the device.
virtual bool AncSetFrameBufferSize(const ULWord inF1Size, const ULWord inF2Size)
Sets the capacity of the ANC buffers in device frame memory. (Call NTV2DeviceCanDoCustomAnc to determ...
@ kDeviceCanReportRunningFirmwareDate
True if device can report its running (and not necessarily installed) firmware date.
virtual bool GetRunningFirmwareDate(UWord &outYear, UWord &outMonth, UWord &outDay)
Reports the (local Pacific) build date of the currently-running firmware.
virtual bool EnableBOBAnalogAudioIn(bool inEnable)
Enables breakout board analog audio XLR inputs.
virtual bool Set12BitLUTPlaneSelect(const NTV2LUTPlaneSelect inLUTPlane)
Sets the LUT plane.
virtual NTV2DeviceID GetBaseDeviceID()
UWord GetNumAnalogVideoInputs(void)
virtual bool SetProgressivePicture(ULWord value)
bool CanDoMultiLinkAudio(void)
virtual bool GetSDIWatchdogEnable(bool &outIsEnabled, const UWord inIndex0)
Answers true if the given connector pair relays are under watchdog timer control, or false if they're...
virtual bool DisableChannel(const NTV2Channel inChannel)
Disables the given FrameStore.
virtual bool IsConnected(const NTV2InputCrosspointID inInputXpt, bool &outIsConnected)
Answers whether or not the given widget signal input (sink) is connected to another output (source).
virtual bool GetUCAutoLine21(ULWord &outValue)
ULWord GetNumVideoChannels(void)
@ kDeviceCanDoAudio96K
True if Audio System(s) support a 96kHz sample rate.
@ kDeviceCanDo3GLevelConversion
True if device can do 3G level B to 3G level A conversion.
virtual bool GetUCPassLine21(ULWord &outValue)
bool GetFreeRegions(ULWordSequence &outBlks) const
Answers with the list of free memory regions.
virtual bool GetSDIInput12GPresent(bool &outValue, const NTV2Channel channel)
virtual bool GetSDIOut6GEnable(const NTV2Channel inChannel, bool &outIsEnabled)
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
@ kDeviceHasAudioMonitorRCAJacks
True if device has a pair of unbalanced RCA audio monitor output connectors.
virtual bool GetFramePulseReference(NTV2ReferenceSource &outRefSource)
Answers with the device's current frame pulse reference source.
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
@ kDeviceCanDoQuarterExpand
True if device can handle quarter-sized frames (pixel-halving and line-halving during input,...
virtual bool SetColorCorrectionOutputBank(const NTV2Channel inLUTWidget, const ULWord inBank)
Sets the LUT bank to be used for the given LUT.
virtual bool SetHDMIHDRBT2020(void)
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
virtual ULWord StreamChannelFlush(const NTV2Channel inChannel, NTV2StreamChannel &status)
Flush a stream. Remove all the buffers from the stream except a currently active idle buffer....
virtual bool GetHDMIOutAudioRate(NTV2AudioRate &outValue)
Answers with the HDMI output's current audio rate.
virtual bool SetHDMIInAudioSampleRateConverterEnable(const bool inNewValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool GetHDMIHDRBluePrimaryY(uint16_t &outBluePrimaryY)
Answers with the Display Mastering data for Blue Primary Y as defined in SMPTE ST 2086....
virtual bool GetInputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current input frame index number for the given FrameStore. This identifies which par...
virtual bool WriteProcAmpC1CBAdjustment(const ULWord inNewValue)
virtual bool SetDualLinkInputEnable(const bool inIsEnabled)
virtual bool GetColorSpaceMakeAlphaFromKey(ULWord &outMakeAlphaFromKey, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers whether or not the given CSC is set to produce alpha channel data from its key input.
virtual bool AddDynamicDirectory(const std::string &inDirectory)
Adds all bitfiles found in the given host file directory to the list of available dynamic bitfiles.
@ kDeviceGetNumVideoOutputs
The number of SDI video outputs on the device.
virtual bool AutoCirculateResume(const NTV2Channel inChannel, const bool inClearDropCount=false)
Resumes AutoCirculate for the given channel, picking up at the next frame without loss of audio synch...
virtual bool WriteSDProcAmpControlsInitialized(const ULWord inNewValue=1)
@ kDeviceGetNum4kQuarterSizeConverters
The number of quarter-size 4K/UHD down-converters on the device.
virtual bool EnableRP188Bypass(const NTV2Channel inSDIOutput)
Configures the SDI output's embedder to embed SMPTE 12M timecode obtained from an SDI input,...
virtual bool SetOutputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the output frame index number for the given FrameStore. This identifies which frame in device SD...
NTV2FieldID
These values are used to identify fields for interlaced video. See Field/Frame Interrupts and CNTV2Ca...
virtual bool GetStereoCompressorLeftSource(NTV2OutputCrosspointID &outValue)
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
UWord GetNumAnalogVideoOutputs(void)
ULWord GetLUTVersion(void)
UWord GetNumAnalogAudioInputChannels(void)
virtual bool GetInstalledBitfileInfo(ULWord &outNumBytes, std::string &outDateStr, std::string &outTimeStr)
Returns the size and time/date stamp of the device's currently-installed firmware.
UWord GetNumReferenceVideoInputs(void)
virtual bool GetAudioMixerOutputLevels(const NTV2AudioChannelPairs &inChannelPairs, std::vector< uint32_t > &outLevels)
Answers with the Audio Mixer's current audio output levels.
bool CanDoRGBLevelAConversion(void)
@ kDeviceCanDoFramePulseSelect
True if device supports frame pulse source independent of reference source.
virtual bool AncExtractSetFilterDIDs(const UWord inSDIInput, const NTV2DIDSet &inDIDs)
Replaces the DIDs to be excluded (filtered) by the given SDI input's Anc extractor....
virtual bool SetStereoCompressorFlipMode(const ULWord inNewValue)
virtual bool HevcVideoTransfer(HevcDeviceTransfer *pTransfer)
Transfer video to/from the hevc device.
virtual bool SetLUTControlSelect(const NTV2LUTControlSelect inLUTSelect)
@ kDeviceCanDo12gRouting
True if device supports 12G routing crosspoints.
virtual bool GetHDMIHDRGreenPrimaryY(uint16_t &outGreenPrimaryY)
Answers with the Display Mastering data for Green Primary Y as defined in SMPTE ST 2086....
virtual bool SetHDMIOutAudioChannel34Swap(const bool inIsSwapped, const NTV2Channel inWhichHDMIOut=NTV2_CHANNEL1)
Sets the HDMI output's audio channel 3/4 swap state.
@ kDeviceHasSPIv2
Use kDeviceGetSPIVersion instead.
virtual bool AncExtractGetFilterDIDs(const UWord inSDIInput, NTV2DIDSet &outDIDs)
Answers with the DIDs currently being excluded (filtered) by the SDI input's Anc extractor....
virtual bool SetHDMIHDRConstantLuminance(const bool inEnableConstantLuminance)
Enables or disables BT.2020 Y'cC'bcC'rc versus BT.2020 Y'C'bC'r or R'G'B'.
bool CanDoHDMIHDROut(void)
@ kDeviceCanThermostat
True if device fan can be thermostatically controlled.
virtual bool Load3DLUTTable(void)
Convenience class/API for inquiring about device capabilities of physical and virtual devices....
virtual ULWord StreamChannelRelease(const NTV2Channel inChannel)
Release a stream. Releases all buffers remaining in the queue.
virtual bool SetDeinterlaceMode(const ULWord inValue)
virtual bool SetVPIDRGBRange(const NTV2VPIDRGBRange inValue, const NTV2Channel inChannel)
bool CanDoThunderbolt(void)
bool CanChangeFrameBufferSize(void)
virtual bool WriteHDProcAmpControlsInitialized(const ULWord inNewValue=1)
virtual bool GetVANCMode(NTV2VANCMode &outVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Retrieves the current VANC mode for the given FrameStore.
ULWord GetActiveMemorySize(void)
virtual bool GetMultiFormatMode(bool &outIsEnabled)
Answers if the device is operating in multiple-format per channel (independent channel) mode or not....
virtual bool GetSDIRelayManualControl(NTV2RelayState &outValue, const UWord inIndex0)
Answers if the bypass relays between connectors 1 and 2 would be in bypass or would route signals thr...
virtual bool SupportsP2PTransfer(void)
virtual bool GetAudioOutputAESSyncModeBit(const NTV2AudioSystem inAudioSystem, bool &outAESSyncModeBitSet)
Answers with the current state of the AES Sync Mode bit for the given Audio System's output.
@ kDeviceGetNumAESAudioOutputChannels
The number of AES/EBU audio output channels on the device.
@ kDeviceCanDoAudioMixer
True if device has a firmware audio mixer.
@ kDeviceCanDoFrameStore1Display
True if device can display/output video from FrameStore 1.
virtual bool MixerHasRGBModeSupport(const UWord inWhichMixer, bool &outIsSupported)
Answers if the given mixer/keyer's has RGB mode support.
virtual bool GetEnableHDMIOutCenterCrop(bool &outIsEnabled)
Answers if the HDMI 4k/2k -> UHD/HD center cropping is enabled or not.
virtual bool AncInsertGetReadInfo(const UWord inSDIOutput, uint64_t &outF1StartAddr, uint64_t &outF2StartAddr)
Answers where, in device SDRAM, the given SDI connector's Anc inserter is currently reading Anc data ...
UWord GetNumEmbeddedAudioInputChannels(void)
@ kDeviceHasPCIeGen2
True if device supports 2nd-generation PCIe.
virtual bool WriteHDCrOffsetAdjustment(const ULWord inNewValue)
@ kDeviceCanDoDualLink
True if device supports 10-bit RGB input/output over 2-wire SDI.
virtual bool SetLUTV2HostAccessBank(const NTV2ColorCorrectionHostAccessBank inValue)
bool CanDoProgrammableRS422(void)
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
virtual bool WriteOutputTimingControl(const ULWord inValue, const UWord inOutputSpigot=0)
Adjusts the output timing for the given SDI output connector.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool ReadFlashProgramControl(ULWord &outValue)
virtual bool WriteProcAmpOffsetYAdjustment(const ULWord inNewValue)
virtual bool ReadHDCrOffsetAdjustment(ULWord &outValue)
NTV2RelayState
This enumerated data type identifies the two possible states of the bypass relays....
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool GetAudioInputDelay(const NTV2AudioSystem inAudioSystem, ULWord &outDelay)
Answers with the audio input delay for the given Audio System on the device.
bool CanDoCustomAnc(void)
virtual bool GetSDITransmitEnable(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the specified SDI connector is currently acting as a transmitter (i....
virtual bool SetHDMIOutSampleStructure(const NTV2HDMISampleStructure inNewValue)
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
virtual bool GetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat &outValue)
Returns the current frame buffer format for the given FrameStore on the AJA device.
@ kDeviceCanDoWarmBootFPGA
True if device can warm-boot to load updated firmware.
virtual bool ReadHDBrightnessAdjustment(ULWord &outValue)
virtual bool GetEnable4KDCPSFInMode(bool &outIsEnabled)
Audits an NTV2 device's SDRAM utilization, and can report contiguous regions of SDRAM,...
virtual bool SetHDMIHDRGreenPrimaryX(const uint16_t inGreenPrimaryX)
Sets the Display Mastering data for Green Primary X as defined in SMPTE ST 2086. This is Byte 3 and 4...
UWord GetNumLTCOutputs(void)
virtual bool GetXena2FlashBaseAddress(ULWord **pXena2FlashAddress)
@ kDeviceCanChangeFrameBufferSize
True if frame buffer sizes are not fixed.
bool CanDoOutputDestination(const NTV2OutputDestination inDest)
virtual bool WriteHDSaturationAdjustmentCr(const ULWord inNewValue)
virtual bool GetSDIOut2Kx1080Enable(const NTV2Channel inChannel, bool &outIsEnabled)
virtual bool GetHDMIInAudioChannel34Swap(bool &outIsSwapped, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current audio channel 3/4 swap setting.
virtual bool SetColorSpaceCustomCoefficients(const NTV2CSCCustomCoeffs &inCustomCoefficients, const NTV2Channel inChannel=NTV2_CHANNEL1)
NTV2HDMIRange
Indicates or specifies the HDMI RGB range.
virtual bool Set1DLUTTableLocation(const NTV2Channel inChannel, const ULWord inFrameNumber, ULWord inLUTIndex=0)
virtual bool GetQuadQuadSquaresEnable(bool &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the device's current "quad-quad-squares" frame mode, whether it's enabled or not.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
@ kDeviceGetNumAnalogAudioOutputChannels
The number of analog audio output channels on the device.
virtual bool Enable4KDCYCC444Mode(bool inEnable)
Sets 4K Down Convert YCC 444 mode.
Reports HDMI output status information.
Declares the CNTV2LinuxDriverInterface class.
virtual bool GetHDMIOutAudioChannels(NTV2HDMIAudioChannels &outValue)
virtual bool GetEnableConverter(bool &outValue)
virtual bool Get12BitLUTTables(NTV2DoubleArray &outRedLUT, NTV2DoubleArray &outGreenLUT, NTV2DoubleArray &outBlueLUT)
NTV2FrameRate
Identifies a particular video frame rate.
virtual bool AncExtractSetField2WriteParams(const UWord inSDIInput, const ULWord inFrameNumber, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Framesize inFrameSize=NTV2_FRAMESIZE_INVALID)
Configures the given SDI input's Anc extractor to receive the next frame's F2 Anc data....
virtual bool SetAudioMixerOutputGain(const ULWord inGainValue)
Sets the gain for the output of the Audio Mixer.
@ kDeviceGetNumDMAEngines
The number of DMA engines on the device.
virtual bool GetHDMIOutDownstreamColorSpace(NTV2LHIHDMIColorSpace &outValue)
This is returned by the CNTV2Card::AutoCirculateGetFrameStamp function, and is also embedded in the A...
bool CanDo3GLevelConversion(void)
virtual bool SetAudioPlayCaptureModeEnable(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Enables or disables a special mode for the given Audio System whereby its embedder and deembedder bot...
@ kDeviceGetNumBufferedAudioSystems
The total number of audio systems on the device that can read/write audio buffer memory....
@ kDeviceGetNumLUTs
The number of LUT widgets on the device.
const NTV2AudioChannelsMuted16 NTV2AudioChannelsMuteAll
All 16 audio channels muted/disabled.
virtual bool SetConverterOutRate(const NTV2FrameRate inValue)
virtual bool WriteRegisters(const NTV2RegisterWrites &inRegWrites)
Writes the given sequence of NTV2RegInfo's.
virtual bool SetFrameBufferQuality(NTV2Channel inChannel, NTV2FrameBufferQuality inValue)
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
virtual bool ReadSDCbOffsetAdjustment(ULWord &outValue)
virtual bool GetMixerBGMatteEnabled(const UWord inWhichMixer, bool &outIsEnabled)
Answers if the given mixer/keyer's background matte is enabled or not.
virtual bool IsAudioChannelPairPresent(const NTV2AudioSystem inAudioSystem, const NTV2AudioChannelPair inChannelPair, bool &outIsPresent)
Answers whether or not the given NTV2AudioChannelPair in the given NTV2AudioSystem on the device is p...
virtual bool SetOutputVerticalEventCount(const ULWord inCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Resets my output interrupt event tally for the given channel.
@ kDeviceHasSDIRelays
True if device has bypass relays on its SDI connectors.
NTV2RegisterNumberSet NTV2RegNumSet
A set of distinct NTV2RegisterNumbers.
Declares CNTV2SignalRouter class.
virtual bool GetRunningFirmwareUserID(ULWord &outUserID)
Reports the UserID number of the currently-running firmware.
virtual bool ReadSDProcAmpControlsInitialized(ULWord &outValue)
virtual bool GetAudioCaptureEnable(const NTV2AudioSystem inAudioSystem, bool &outEnable)
Answers whether or not the Audio System is configured to write captured audio samples into device aud...
NTV2MixerKeyerInputControl
These enum values identify the Mixer/Keyer foreground and background input control values.
virtual bool GetEnableHDMIOutUserOverride(bool &outIsEnabled)
Answers if override of HDMI parameters is enabled or not.
virtual std::string GetDeviceVersionString(void)
Answers with this device's version number as a human-readable string.
@ kDeviceCanDoAudioDelay
True if Audio System(s) support an adjustable delay.
virtual bool ReadSDBrightnessAdjustment(ULWord &outValue)
virtual bool EnableOutputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to output vertical blanking interrupts originat...
virtual bool GetHDMIHDRBluePrimaryX(uint16_t &outBluePrimaryX)
Answers with the Display Mastering data for Blue Primary X as defined in SMPTE ST 2086....
virtual bool GetAncRegionOffsetAndSize(ULWord &outByteOffset, ULWord &outByteCount, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All)
Answers with the offset and size of an ancillary data region within a device frame buffer.
virtual bool GetLUTControlSelect(NTV2LUTControlSelect &outLUTSelect)
@ kDeviceGetDACVersion
The version number of the DAC on the device.
virtual bool WriteProcAmpC2CBAdjustment(const ULWord inNewValue)
virtual bool GetAudioMixerLevelsSampleCount(ULWord &outSampleCount)
Answers with the Audio Mixer's current sample count used for measuring audio levels.
enum NTV2VPIDTransferCharacteristics NTV2HDRXferChars
NTV2_RS422_PARITY
These enum values identify RS-422 serial port parity configuration.
virtual bool SetEnableConverter(const bool inValue)
virtual bool GetDetectedAudioChannelPairs(const NTV2AudioSystem inAudioSystem, NTV2AudioChannelPairs &outDetectedChannelPairs)
Answers which audio channel pairs are present in the given Audio System's input stream.
virtual bool GetSDIOutputDS2AudioSystem(const NTV2Channel inSDIOutputConnector, NTV2AudioSystem &outAudioSystem)
Answers with the device's Audio System that is currently providing audio for the given SDI output's a...
virtual bool DownloadLUTToHW(const NTV2DoubleArray &inRedLUT, const NTV2DoubleArray &inGreenLUT, const NTV2DoubleArray &inBlueLUT, const NTV2Channel inLUT, const int inBank)
Sends the given color lookup tables (LUTs) to the given LUT and bank.
virtual bool AncInsertSetComponents(const UWord inSDIOutput, const bool inVancY, const bool inVancC, const bool inHancY, const bool inHancC)
Enables or disables individual Anc insertion components for the given SDI output. (Call NTV2DeviceCan...
virtual bool SetHDMIHDRMaxContentLightLevel(const uint16_t inMaxContentLightLevel)
Sets the Display Mastering data for the Max Content Light Level(Max CLL) value. This is Byte 23 and 2...
virtual bool GetSDILock(const NTV2Channel inChannel)
virtual bool IsSupported(const NTV2BoolParamID inParamID)
bool IsDirectAddressable(void)
@ kDeviceCanDoLTC
True if device can read LTC (Linear TimeCode) from one of its inputs.
UWord GetNumFrameStores(void)
virtual bool GetColorSpaceMethod(NTV2ColorSpaceMethod &outMethod, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current operating mode of the given color space converter.
virtual std::string GetModelName(void)
Answers with this device's model name.
NTV2EmbeddedAudioClock
This enum value determines/states the device audio clock reference source. It was important to set th...
bool CanDoIsoConvert(void)
virtual bool HevcGetDeviceInfo(HevcDeviceInfo *pInfo)
Returns the driver version and time/date stamp of the hevc device's currently-installed firmware.
virtual bool GetColorSpaceCustomCoefficients(NTV2CSCCustomCoeffs &outCustomCoefficients, const NTV2Channel inChannel=NTV2_CHANNEL1)
@ kDeviceCanDoDVCProHD
True if device can squeeze/stretch between 1920x1080/1280x1080 and 1280x720/960x720.
virtual bool GetSDIInput6GPresent(bool &outValue, const NTV2Channel channel)
virtual class DeviceCapabilities & features(void)
virtual bool DMAWriteAudio(const NTV2AudioSystem inAudioSystem, const ULWord *pInAudioBuffer, const ULWord inOffsetBytes, const ULWord inByteCount)
Synchronously transfers audio data from the specified host buffer to the given Audio System's buffer ...
@ kDeviceCanDoHFRRGB
True if device supports 1080p RGB at more than 50Hz frame rates.
virtual bool GetHDMIOutAudioSource8Channel(NTV2Audio8ChannelSelect &outValue, NTV2AudioSystem &outAudioSystem)
Answers with the HDMI output's current 8-channel audio source.
virtual bool SetVPIDTransferCharacteristics(const NTV2VPIDTransferCharacteristics inValue, const NTV2Channel inChannel)
virtual bool Enable4KDCPSFInMode(bool inEnable)
Sets 4K Down Convert PSF in mode.
virtual bool SetHDMIOutLevelBMode(const bool inEnable)
Enables or disables level-B mode on the device's HDMI rasterizer.
virtual bool Enable4KDCRGBMode(bool inEnable)
Sets 4K Down Convert RGB mode.
virtual bool SetAudioOutputEraseMode(const NTV2AudioSystem inAudioSystem, const bool &inEraseModeEnabled)
Enables or disables output erase mode for the given Audio System, which, when enabled,...
virtual bool GetPulldownMode(NTV2Channel inChannel, bool &outValue)
virtual std::string GetBitfileInfoString(const BITFILE_INFO_STRUCT &inBitFileInfo)
Generates and returns an info string with date, time and name for the given inBifFileInfo.
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
virtual bool IsAudioInputRunning(const NTV2AudioSystem inAudioSystem, bool &outIsRunning)
Answers whether or not the capture side of the given NTV2AudioSystem is currently running.
virtual bool DMABufferUnlockAll()
Unlocks all previously-locked buffers used for DMA transfers.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
virtual bool GetColorSpaceMatrixSelect(NTV2ColorSpaceMatrixType &outType, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current matrix type being used for the given CSC.
virtual NTV2VideoFormat GetReferenceVideoFormat(void)
Returns the video format of the signal that is present on the device's reference input.
@ kDeviceCanDoAnalogAudio
virtual bool GetAnalogLTCInClockChannel(const UWord inLTCInput, NTV2Channel &outChannel)
Answers with the (SDI) input channel that's providing the clock reference being used by the given dev...
bool CanDoStackedAudio(void)
std::ostream & DumpBlocks(std::ostream &oss) const
Dumps all 8MB blocks/frames and their tags, if any, into the given stream.
virtual bool SetMixerBGMatteEnabled(const UWord inWhichMixer, const bool inIsEnabled)
Answers if the given mixer/keyer's background matte is enabled or not.
virtual bool GetIsoConvertMode(NTV2IsoConvertMode &outValue)
NTV2DoubleArray::iterator NTV2DoubleArrayIter
Handy non-const iterator to iterate over an NTV2DoubleArray.
virtual bool DeviceHasMicInput(void)
virtual bool ReadHDProcAmpControlsInitialized(ULWord &outValue)
virtual bool SetColorCorrectionMode(const NTV2Channel inChannel, const NTV2ColorCorrectionMode inMode)
virtual bool GetMixerMatteColor(const UWord inWhichMixer, YCbCr10BitPixel &outYCbCrValue)
Answers with the given mixer/keyer's current matte color value being used.
virtual bool GetMixerFGInputControl(const UWord inWhichMixer, NTV2MixerKeyerInputControl &outInputControl)
Returns the current foreground input control value for the given mixer/keyer.
virtual bool GetHDMIHDRMaxFrameAverageLightLevel(uint16_t &outMaxFrameAverageLightLevel)
Answers with the Display Mastering data for the Max Frame Average Light Level(Max FALL) value....
virtual bool GetPossibleConnections(NTV2PossibleConnections &outConnections)
Answers with the implemented crosspoint connections (if known).
virtual bool GetVideoDACMode(NTV2VideoDACMode &outValue)
virtual bool EnableInterrupt(const INTERRUPT_ENUMS inEventCode)
virtual bool GetStereoCompressorFlipRightVert(ULWord &outValue)
virtual bool SetVideoLimiting(const NTV2VideoLimiting inValue)
virtual bool GetRP188Data(const NTV2Channel inSDIInput, NTV2_RP188 &outRP188Data)
Reads the raw RP188 data from the DBB/Low/Hi registers for the given SDI input. On newer devices with...
virtual bool DMAReadSegments(const ULWord inFrameNumber, ULWord *pFrameBuffer, const ULWord inCardOffsetBytes, const ULWord inSegmentByteCount, const ULWord inNumSegments, const ULWord inSegmentHostPitch, const ULWord inSegmentCardPitch)
Performs a segmented data transfer from the AJA device to the host.
virtual bool SetRP188SourceFilter(const NTV2Channel inSDIInput, const UWord inFilterValue)
Sets the RP188 DBB filter for the given SDI input.
virtual bool AncExtractGetField1Size(const UWord inSDIInput, ULWord &outF1Size)
Answers with the number of bytes of field 1 ANC extracted. (Call NTV2DeviceCanDoCustomAnc to determin...
@ kDeviceCanDoProgrammableRS422
True if device has at least one RS-422 serial port, and it (they) can be programmed (for baud rate,...
virtual bool AncExtractSetWriteParams(const UWord inSDIInput, const ULWord inFrameNumber, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Framesize inFrameSize=NTV2_FRAMESIZE_INVALID)
Configures the given SDI input's Anc extractor to receive the next frame's F1 Anc data....
@ kDeviceGetNumAudioSystems
The number of independent Audio Systems on the device.
virtual bool GetHDMIOutProtocol(NTV2HDMIProtocol &outValue)
virtual bool SetHDMIOutAudioChannels(const NTV2HDMIAudioChannels inNewValue)
@ kDeviceHasSPIv3
Use kDeviceGetSPIVersion instead.
@ kDeviceSoftwareCanChangeFrameBufferSize
True if device frame buffer size can be changed.
virtual bool GetHDMIOutLevelBMode(bool &outIsEnabled)
virtual bool GetHDMIOutRange(NTV2HDMIRange &outValue)
std::set< UByte > NTV2DIDSet
A set of distinct NTV2DID values.
@ kDeviceCanDoThunderbolt
True if device connects to the host using a Thunderbolt cable.
virtual bool GetOutputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current output frame number for the given FrameStore (expressed as an NTV2Channel).
bool CanDoBreakoutBox(void)
UWord GetMaxAudioChannels(void)
virtual bool SetUpConvertMode(const NTV2UpConvertMode inValue)
@ kDeviceCanDo2110
True if device supports SMPTE ST2110.
virtual bool AncInsertSetField2ReadParams(const UWord inSDIOutput, const ULWord inFrameNumber, const ULWord inF2Size, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Framesize inFrameSize=NTV2_FRAMESIZE_INVALID)
Configures the Anc inserter for the next frame's F2 Anc data to embed/transmit. (Call NTV2DeviceCanDo...
virtual bool GetTsiMuxSyncFail(bool &outSyncFailed, const NTV2Channel inWhichTsiMux)
Answers if the SMPTE 425 two-sample-interleave mux/demux input sync has failed or not.
@ kDeviceCanDoRGBLevelAConversion
True if the device can do RGB over 3G Level A.
@ kDeviceGetNum2022ChannelsSFP1
The number of 2022 channels configured on SFP 1 on the device.
bool NeedsRoutingSetup(void)
NTV2Standard
Identifies a particular video standard.
virtual bool AutoCirculateGetFrameStamp(const NTV2Channel inChannel, const ULWord inFrameNumber, FRAME_STAMP &outFrameInfo)
Returns precise timing information for the given frame and channel that's currently AutoCirculating.
@ kDeviceHasRotaryEncoder
True if device has a rotary encoder volume control.
virtual bool SetHDMIHDRDCIP3(void)
virtual bool SetSecondaryVideoFormat(NTV2VideoFormat inFormat)
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual bool SupportsP2PTarget(void)
virtual bool GetNumberAudioChannels(ULWord &outNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current number of audio channels being captured or played by a given Audio System on the ...
@ kDeviceHasSPIFlashSerial
True if device has serial SPI flash hardware.
virtual bool GetRP188SourceFilter(const NTV2Channel inSDIInput, UWord &outFilterValue)
Returns the current RP188 filter setting for the given SDI input.
virtual bool GetLHIVideoDACStandard(NTV2Standard &outValue)
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
virtual bool SetHDMIOut3DPresent(const bool inIs3DPresent)
virtual std::string GetPCIFPGAVersionString(void)
virtual bool IsDynamicFirmwareLoaded(void)
@ kDeviceCanDoHDMIHDROut
True if device supports HDMI HDR output.
virtual bool GetVideoFormat(NTV2VideoFormat &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool DMABufferUnlock(const ULWord *pInBuffer, const ULWord inByteCount)
Unlocks the given host buffer that was previously locked using CNTV2Card::DMABufferLock.
UWord GetNumVideoOutputs(void)
virtual bool SetConversionMode(const NTV2ConversionMode inConversionMode)
virtual bool SubscribeEvent(const INTERRUPT_ENUMS inEventCode)
Causes me to be notified when the given event/interrupt is triggered for the AJA device.
static NTV2Buffer NULL_POINTER
Used for default empty NTV2Buffer parameters – do not modify.
bool CanDoHDMIMultiView(void)
@ kDeviceCanDoProRes
True if device can can accommodate Apple ProRes-compressed video in its frame buffers.
@ kDeviceGetNumVideoChannels
The number of video channels supported on the device.
virtual NTV2DeviceIDSet GetDynamicDeviceIDs(void)
NTV2DoubleArray::const_iterator NTV2DoubleArrayConstIter
Handy const iterator to iterate over an NTV2DoubleArray.
virtual bool SetAnalogLTCOutClockChannel(const UWord inLTCOutput, const NTV2Channel inChannel)
Sets the (SDI) output channel that is to provide the clock reference to be used by the given analog L...
virtual bool GetSDIWatchdogTimeout(ULWord &outValue)
Answers with the amount of time that must elapse before the watchdog timer times out.
@ kDeviceGetNumAnalogVideoInputs
The number of analog video inputs on the device.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
virtual bool GetQuadQuadFrameEnable(bool &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the device's current "quad-quad" frame mode, whether it's enabled or not.
bool CanDoPCMDetection(void)
virtual bool SetColorCorrectionHostAccessBank(const NTV2ColorCorrectionHostAccessBank inValue)
virtual bool GetSDI2OutHTiming(ULWord *value)
virtual bool GetAudioOutputEraseMode(const NTV2AudioSystem inAudioSystem, bool &outEraseModeEnabled)
Answers with the current state of the audio output erase mode for the given Audio System....
std::ostream & operator<<(std::ostream &inOutStr, const NTV2AudioChannelPairs &inSet)
Handy ostream writer for NTV2AudioChannelPairs.
virtual bool GetSDIOutVPID(ULWord &outValueA, ULWord &outValueB, const UWord inOutputSpigot=NTV2_CHANNEL1)
virtual bool ReadProcAmpOffsetYAdjustment(ULWord &outValue)
virtual bool AncExtractGetField2Size(const UWord inSDIInput, ULWord &outF2Size)
Answers with the number of bytes of field 2 ANC extracted. (Call NTV2DeviceCanDoCustomAnc to determin...
virtual bool GetRawAudioTimer(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Answers with the current value of the 48kHz audio clock counter.
virtual ULWord DeviceGetFrameBufferSize(void)
virtual bool ReleaseMailBoxLock(void)
virtual bool WriteVirtualData(const ULWord inTag, const void *inVirtualData, const ULWord inVirtualDataSize)
Writes the block of virtual data.
virtual bool GetAudioReadOffset(ULWord &outReadOffset, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset from the start of the audio buffer to the fi...
@ kNTV2EnumsID_ConversionMode
Identifies the NTV2ConversionMode enumerated type.
virtual bool GetAudioMixerOutputGain(ULWord &outGainValue)
Answers with the current gain setting for the Audio Mixer's output.
virtual bool GetRunningFirmwareTime(UWord &outHours, UWord &outMinutes, UWord &outSeconds)
Reports the (local Pacific) build time of the currently-running firmware.
virtual ULWord StreamChannelStart(const NTV2Channel inChannel, NTV2StreamChannel &status)
Start a stream. Put the stream is the active state to start processing queued buffers....
virtual NTV2DeviceIDList GetDynamicDeviceList(void)
NTV2ReferenceSource
These enum values identify a specific source for the device's (output) reference clock.
UWord GetNumMicInputs(void)
NTV2AudioChannelQuads::const_iterator NTV2AudioChannelQuadsConstIter
Handy const iterator to iterate over a set of distinct NTV2AudioChannelQuad values.
@ kDeviceGetLUTVersion
The version number of the LUT(s) on the device.
virtual bool SetLEDState(ULWord inValue)
The four on-board LEDs can be set by writing 0-15.
virtual bool WriteHDSaturationAdjustmentCb(const ULWord inNewValue)
std::set< NTV2AudioChannelPair > NTV2AudioChannelPairs
A set of distinct NTV2AudioChannelPair values.
virtual ULWord StreamChannelStatus(const NTV2Channel inChannel, NTV2StreamChannel &status)
Get the current stream status.
@ kDeviceCanDoHDMIOutStereo
True if device supports 3D/stereo HDMI video output.
virtual ULWord DeviceGetNumberFrameBuffers(void)
virtual bool GetAESOutputSource(const NTV2Audio4ChannelSelect inAESAudioChannels, NTV2AudioSystem &outSrcAudioSystem, NTV2Audio4ChannelSelect &outSrcAudioChannels)
Answers with the current audio source for a given quad of AES audio output channels....
virtual bool GetSDIOut3GbEnable(const NTV2Channel inChannel, bool &outIsEnabled)
std::vector< uint16_t > UWordSequence
An ordered sequence of UWord (uint16_t) values.
NTV2Mode
Used to identify the mode of a Frame Store, or the direction of an AutoCirculate stream: either Captu...
NTV2HDMIProtocol
Indicates or specifies the HDMI protocol.
virtual bool GetInputAudioChannelPairsWithoutPCM(const NTV2Channel inSDIInputConnector, NTV2AudioChannelPairs &outChannelPairs)
For the given SDI input (specified as a channel number), returns the set of audio channel pairs that ...
std::ostream & RawDump(std::ostream &oss) const
Dumps a human-readable list of regions into the given stream.
UWord GetNumEmbeddedAudioOutputChannels(void)
@ kDeviceCanDoLTCInOnRefPort
True if device can read LTC (Linear TimeCode) from its reference input.
virtual bool GetRunningFirmwarePackageRevision(ULWord &outRevision)
Reports the revision number of the currently-running firmware package. KonaIP style boards have a pac...
virtual bool SetHDMIOutForceConfig(const bool inNewValue)
virtual bool ReadSDCrOffsetAdjustment(ULWord &outValue)
std::set< NTV2AudioSystem > NTV2AudioSystemSet
A set of distinct NTV2AudioSystem values. New in SDK 16.2.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
virtual bool SetForce64(ULWord force64)
virtual bool GetHDMIOutVideoStandard(NTV2Standard &outValue)
virtual bool SetSDIOutRGBLevelAConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables an RGB-over-3G-level-A conversion at the SDI output widget (assuming the AJA devi...
virtual bool SetVPIDColorimetry(const NTV2VPIDColorimetry inValue, const NTV2Channel inChannel)
virtual bool Read12BitLUTTables(UWordSequence &outRedLUT, UWordSequence &outGreenLUT, UWordSequence &outBlueLUT)
virtual bool WriteProcAmpC2CRAdjustment(const ULWord inNewValue)
virtual bool GetEncodeAsPSF(NTV2Channel inChannel, NTV2EncodeAsPSF &outValue)
virtual bool IsConnectedTo(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, bool &outIsConnected)
Answers whether or not the given widget signal input (sink) is connected to another output (source).
CNTV2Card CNTV2Device
Instances of this class are able to interrogate and control an NTV2 AJA video/audio capture/playout d...
virtual bool GetHDMIHDRMinMasteringLuminance(uint16_t &outMinMasteringLuminance)
Answers with the Display Mastering data for the Min Mastering Luminance value as defined in SMPTE ST ...
@ kDeviceCanDoPCMDetection
True if device can detect which audio channel pairs are not carrying PCM (Pulse Code Modulation) audi...
@ kDeviceIs64Bit
True if device is 64-bit addressable.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
@ kDeviceGetHDMIVersion
The HDMI input(s) and/or output(s) on the device.
virtual bool AncInsertSetIPParams(const UWord inSDIOutput, const UWord ancChannel, const ULWord payloadID, const ULWord ssrc)
Configures the Anc inserter IP specific params.
bool CanDoRGBPlusAlphaOut(void)
@ kDeviceCanDisableUFC
True if there's at least one UFC, and it can be disabled.
virtual bool SetAnalogLTCInClockChannel(const UWord inLTCInput, const NTV2Channel inChannel)
Sets the (SDI) input channel that is to provide the clock reference to be used by the given analog LT...
virtual bool SetIsoConvertMode(const NTV2IsoConvertMode inValue)
virtual bool GetDeviceFrameInfo(const UWord inFrameNumber, const NTV2Channel inChannel, uint64_t &outAddress, uint64_t &outLength)
Answers with the address and size of the given frame.
virtual bool GetAudioAnalogLevel(NTV2AudioLevel &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
@ kDeviceAudioCanWaitForVBI
True if device audio systems can wait for VBI before starting. (New in SDK 17.0)
virtual bool GetEnable4KDCRGBMode(bool &outIsEnabled)
virtual bool SetHDMIHDRElectroOpticalTransferFunction(const uint8_t inEOTFByte)
ULWord GetUFCVersion(void)
virtual bool SetMixerMatteColor(const UWord inWhichMixer, const YCbCr10BitPixel inYCbCrValue)
Sets the matte color to use for the given mixer/keyer.
virtual bool IsFailSafeBitfileLoaded(bool &outIsFailSafe)
Answers whether or not the "fail-safe" (aka "safe-boot") bitfile is currently loaded and running in t...
virtual bool GetAnalogLTCOutClockChannel(const UWord inLTCOutput, NTV2Channel &outChannel)
Answers with the (SDI) output channel that's providing the clock reference being used by the given de...
@ kDeviceHasXilinxDMA
True if device has Xilinx DMA hardware.
virtual bool SetRP188BypassSource(const NTV2Channel inSDIOutput, const UWord inSDIInput)
For the given SDI output that's in RP188 bypass mode (E-E), specifies the SDI input to be used as a t...
@ kDeviceGetMaxRegisterNumber
The highest register number for the device.
virtual bool ReadProcAmpC1YAdjustment(ULWord &outValue)
virtual bool GetAudioOutputDelay(const NTV2AudioSystem inAudioSystem, ULWord &outDelay)
Answers with the audio output delay for the given Audio System on the device.
size_t GetTagCount(const UWord inIndex) const
Linux implementation of CNTV2DriverInterface.
virtual bool ReadAudioLastIn(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset to the last byte of the latest chunk of 4-by...
virtual bool GetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, NTV2AudioSystem &outAudioSystem)
Answers with the device's NTV2AudioSystem that is currently providing audio for the given SDI output'...
virtual bool GetHDRData(HDRFloatValues &outFloatValues)
virtual bool GetInputFieldID(const NTV2Channel channel, NTV2FieldID &outFieldID)
Returns the current field ID of the specified input channel.
virtual bool GetConverterOutRate(NTV2FrameRate &outValue)
virtual bool GetSerialNumberString(std::string &outSerialNumberString)
Answers with a string that contains my human-readable serial number.
@ kDeviceCanDoVITC2
True if device can insert or extract RP-188/VITC2.
virtual bool WriteLUTTables(const UWordSequence &inRedLUT, const UWordSequence &inGreenLUT, const UWordSequence &inBlueLUT)
Writes the LUT tables.
virtual bool SetFramePulseReference(const NTV2ReferenceSource inRefSource)
Sets the device's frame pulse reference source. See Device Clocking and Synchronization for more info...
virtual bool GetHDMIHDRElectroOpticalTransferFunction(uint8_t &outEOTFByte)
virtual bool ReadAudioLastOut(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset of the tail end of the last chunk of audio s...
NTV2AudioChannelPair
Identifies a pair of audio channels.
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=false)
Connects the given widget signal input (sink) to the given widget signal output (source).
virtual bool Get12BitLUTPlaneSelect(NTV2LUTPlaneSelect &outLUTPlane)
Answers with the current LUT plane.
virtual bool GetAudioOutputEmbedderState(const NTV2Channel inSDIOutputConnector, bool &outIsEnabled)
Answers with the current state of the audio output embedder for the given SDI output connector (speci...
virtual bool SetVideoHOffset(const int inHOffset, const UWord inOutputSpigot=0)
Adjusts the horizontal timing offset, in pixels, for the given SDI output connector.
bool CanDoAudio6Channels(void)
bool CanReportRunningFirmwareDate(void)
virtual bool SetLTCInputEnable(const bool inEnable)
Enables or disables the ability for the device to read analog LTC on the reference input connector.
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
@ kDeviceHasHEVCM31
True if device has an HEVC M31 encoder.
@ kDeviceGetNumInputConverters
The number of input converter widgets on the device.
virtual bool GetLEDState(ULWord &outValue)
Answers with the current state of the four on-board LEDs.
virtual bool DMAWriteAnc(const ULWord inFrameNumber, NTV2Buffer &inAncF1Buffer, NTV2Buffer &inAncF2Buffer=NULL_POINTER, const NTV2Channel inChannel=NTV2_CHANNEL1)
Transfers the contents of the ancillary data buffer(s) from the host to a given frame on the AJA devi...
virtual bool GetLHIHDMIOutColorSpace(NTV2LHIHDMIColorSpace &outValue)
virtual bool ReadProcAmpC1CBAdjustment(ULWord &outValue)
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
virtual bool GetEmbeddedAudioClock(NTV2EmbeddedAudioClock &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given NTV2AudioSystem, answers with the current NTV2EmbeddedAudioClock setting.
virtual bool SetHDMIOutBitDepth(const NTV2HDMIBitDepth inNewValue)
virtual uint64_t GetSerialNumber(void)
Answers with my serial number.
virtual ULWord StreamBufferStatus(const NTV2Channel inChannel, ULWord64 bufferCookie, NTV2StreamBuffer &status)
Get the status of the buffer identified by the bufferCookie.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
Physical device implementations of CNTV2DriverInterface methods through AJA Windows driver.
@ kNTV2EnumsID_InputSource
Identifies the NTV2InputSource enumerated type.
Declares numerous NTV2 utility functions.
UWord GetNumSerialPorts(void)
virtual bool GetHDMIInDolbyVision(bool &outIsDolbyVision, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's Dolby Vision flag is set.
@ kDeviceHasSPIv5
Use kDeviceGetSPIVersion instead.
NTV2Framesize
Kona2/Xena2 specific enums.
virtual bool Disconnect(const NTV2InputCrosspointID inInputXpt)
Disconnects the given widget signal input (sink) from whatever output (source) it may be connected.
virtual bool GetOutputVerticalEventCount(ULWord &outCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the number of output interrupt events that I successfully waited for on the given channe...
@ kDeviceCanDoSDVideo
True if device can handle SD (Standard Definition) video.
virtual NTV2VideoFormat GetAnalogCompositeInputVideoFormat(void)
Returns the video format of the signal that is present on the device's composite video input.
virtual bool GetAnalogAudioTransmitEnable(const NTV2Audio4ChannelSelect inChannelQuad, bool &outEnabled)
Answers whether or not the specified bidirectional XLR audio connectors are collectively acting as in...
virtual bool SetHDMIOutPrefer420(const bool inNewValue)
virtual bool GetHDMIInputAudioChannels(NTV2HDMIAudioChannels &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current number of audio channels being received on the given HDMI input.
virtual bool S2110DeviceAncToBuffers(const NTV2Channel inChannel, NTV2Buffer &ancF1, NTV2Buffer &ancF2)
@ kDeviceCanDoMultiLinkAudio
True if device supports grouped audio system control.
virtual bool GetDefaultVideoOutMode(ULWord &outMode)
NTV2BreakoutType
Identifies the Breakout Boxes and Cables that may be attached to an AJA NTV2 device.
virtual ULWord GetSDIUnlockCount(const NTV2Channel inChannel)
virtual bool CanLoadDynamicDevice(const NTV2DeviceID inDeviceID)
virtual bool AutoCirculatePause(const NTV2Channel inChannel, const UWord inAtFrameNum=0xFFFF)
Pauses AutoCirculate for the given channel. Once paused, AutoCirculate can be resumed later by callin...
virtual bool GetHDMIInProtocol(NTV2HDMIProtocol &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's protocol.
virtual bool EnableHDMIHDRDolbyVision(const bool inEnable)
Enables or disables HDMI HDR Dolby Vision.
NTV2InputCrosspointID
Identifies a widget input that potentially can accept a signal emitted from another widget's output (...
virtual bool GetAudio20BitMode(const NTV2AudioSystem inAudioSystem, bool &outEnable)
Answers whether or not the device's NTV2AudioSystem is currently operating in 20-bit mode....
bool CanMeasureTemperature(void)
virtual bool GetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, bool &outIsEnabled)
Answers with the device's current 3G level A to 3G level B conversion setting for the given SDI outpu...
@ kDeviceGetMaxTransferCount
The maximum number of 32-bit words that the DMA engine can move at a time on the device.
virtual bool GetStereoCompressorFlipRightHorz(ULWord &outValue)
virtual bool InputAudioChannelPairHasPCM(const NTV2Channel inSDIInputConnector, const NTV2AudioChannelPair inAudioChannelPair, bool &outIsPCM)
For the given SDI input (specified as a channel number), answers if the specified audio channel pair ...
virtual bool DMAReadFrame(const ULWord inFrameNumber, ULWord *pOutFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the AJA device to the host.
virtual ULWord StreamBufferRelease(const NTV2Channel inChannel, NTV2StreamBuffer &status)
Remove the oldest buffer released by the streaming engine from the buffer queue.
I interrogate and control an AJA video/audio capture/playout device.
virtual bool DMAWriteLUTTable(const ULWord inFrameNumber, const ULWord *pInLUTBuffer, const ULWord inLUTIndex, const ULWord inByteCount=LUTTablePartitionSize)
Synchronously transfers LUT data from the specified host buffer to the given buffer memory on the AJA...
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=false, bool inRDMA=false)
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
virtual bool SetAnalogInputADCMode(const NTV2LSVideoADCMode inValue)
virtual bool GetAudioOutputMonitorSource(NTV2AudioChannelPair &outChannelPair, NTV2AudioSystem &outAudioSystem)
Answers with the current audio monitor source. The audio output monitor is typically a pair of RCA ja...
virtual bool SetAudioOutputEmbedderState(const NTV2Channel inSDIOutputConnector, const bool &inEnable)
Enables or disables the audio output embedder for the given SDI output connector (specified as a chan...
virtual bool CanWarmBootFPGA(bool &outCanWarmBoot)
Answers whether or not the FPGA can be reloaded without powering off.
SDRAMAuditor()
My default constructor. I am not ready for use until my SDRAMAuditor::Assess method has been called.
virtual bool GetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode &outValue)
Retrieves the current "VANC Shift Mode" feature for the given channel.
virtual bool SetEncodedAudioMode(const NTV2EncodedAudioMode value, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
SDRAMAuditor(CNTV2Card &inDevice)
Constructs me and automatically assesses the given device.
@ NTV2DieTempScale_Celsius
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
UWord GetNumHDMIVideoOutputs(void)
@ kDeviceCanDoPCMControl
True if device can mark specific audio channel pairs as not carrying PCM (Pulse Code Modulation) audi...
bool HasSPIFlashSerial(void)
virtual bool WriteSDCrOffsetAdjustment(const ULWord inNewValue)
virtual bool SetHDMIHDRMaxMasteringLuminance(const uint16_t inMaxMasteringLuminance)
Sets the Display Mastering data for the Max Mastering Luminance value as defined in SMPTE ST 2086....
virtual bool SetConverterOutStandard(const NTV2Standard inValue)
NTV2AudioLoopBack
This enum value determines/states if an audio output embedder will embed silence (zeroes) or de-embed...
virtual bool DMAReadAudio(const NTV2AudioSystem inAudioSystem, ULWord *pOutAudioBuffer, const ULWord inOffsetBytes, const ULWord inByteCount)
Synchronously transfers audio data from a given Audio System's buffer memory on the AJA device to the...
virtual bool GetUpConvertMode(NTV2UpConvertMode &outValue)
virtual bool CanConnect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, bool &outCanConnect)
Answers whether or not the given widget signal input (sink) can legally be connected to the given sig...
virtual bool GetEmbeddedAudioInput(NTV2EmbeddedAudioInput &outEmbeddedSource, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Answers with the device's current embedded (SDI) audio source for the given NTV2AudioSystem.
DeviceCapabilities(CNTV2DriverInterface &inDev)
static UWord AncExtractGetMaxNumFilterDIDs(void)
bool CanDoInputSource(const NTV2InputSource inSrc)
@ kDeviceCanDoAnalogVideoOut
virtual bool GetColorCorrectionMode(const NTV2Channel inChannel, NTV2ColorCorrectionMode &outMode)
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
virtual bool GetSecondaryVideoFormat(NTV2VideoFormat &outFormat)
enum NTV2VPIDColorimetry NTV2HDRColorimetry
virtual bool SetHDMIInColorSpace(const NTV2HDMIColorSpace inNewValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the given HDMI input's color space.
virtual bool SetTsiFrameEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 two-sample interleave (Tsi) frame mode on the device.
virtual bool SetLHIVideoDACStandard(const NTV2Standard inValue)
virtual bool SetHDMIHDRMinMasteringLuminance(const uint16_t inMinMasteringLuminance)
Sets the Display Mastering data for the Min Mastering Luminance value as defined in SMPTE ST 2086....
@ kDeviceHasColorSpaceConverterOnChannel2
Calculate based on if NTV2_WgtCSC2 is present.
virtual bool GetReference(NTV2ReferenceSource &outRefSource)
Answers with the device's current clock reference source.
virtual bool S2110DeviceAncToXferBuffers(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &inOutXferInfo)
virtual NTV2FrameRate GetSDIInputRate(const NTV2Channel channel)
This class is a collection of widget input-to-output connections that can be applied all-at-once to a...
virtual bool SetDitherFor8BitInputs(const NTV2Channel inChannel, const ULWord inDither)
virtual bool GetAudioMixerOutputChannelsMute(NTV2AudioChannelsMuted16 &outMutes)
Answers with a std::bitset that indicates which output audio channels of the Audio Mixer are currentl...
@ kDeviceCanDoBreakoutBox
True if device supports an AJA breakout box.
@ kNTV2EnumsID_WidgetID
Identifies the NTV2AudioWidgetID enumerated type.
NTV2QuarterSizeExpandMode
virtual bool SetHDMIInBitDepth(const NTV2HDMIBitDepth inNewValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the given HDMI input's bit depth.
@ kDeviceIsDirectAddressable
True if device is direct addressable.
@ kDeviceGetPingLED
The highest bit number of the LED bits in the Global Control Register on the device.
virtual bool ReadSDHueAdjustment(ULWord &outValue)
@ kDeviceCanDoStereoIn
True if device supports 3D video input over dual-stream SDI.
virtual bool SetAudio20BitMode(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Enables or disables 20-bit mode for the NTV2AudioSystem.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual bool SetAudioOutputAESSyncModeBit(const NTV2AudioSystem inAudioSystem, const bool &inAESSyncModeBitSet)
Sets or clears the AES Sync Mode bit for the given Audio System's output.
NTV2VANCMode
These enum values identify the available VANC modes.
virtual bool SetSDIWatchdogTimeout(const ULWord inValue)
Specifies the amount of time that must elapse before the watchdog timer times out.
bool CanDoAnalogAudio(void)
UWord GetNumFrameSyncs(void)
bool CanDoWarmBootFPGA(void)
virtual bool SetHDMIOutAudioRate(const NTV2AudioRate inNewValue)
Sets the HDMI output's audio rate.
virtual bool GetMode(const NTV2Channel inChannel, NTV2Mode &outValue)
Answers with the current NTV2Mode of the given FrameStore on the AJA device.
@ kDeviceGetNumMicInputs
The number of microphone inputs on the device.
UWord GetNum4kQuarterSizeConverters(void)
@ kDeviceGetDownConverterDelay
The down-converter delay on the device.
virtual bool GetLUTV2OutputBank(const NTV2Channel inLUTWidget, ULWord &outBank)
virtual bool GetHDMIV2Mode(NTV2HDMIV2Mode &outMode)
Answers with the current HDMI V2 mode of the device.
virtual bool GetMultiLinkAudioMode(const NTV2AudioSystem inAudioSystem, bool &outEnabled)
Answers with the current multi-link audio mode for the given audio system.
virtual ULWord GetCRCErrorCountB(const NTV2Channel inChannel)
virtual std::string GetDriverVersionString(void)
Answers with this device's driver's version as a human-readable string.
bool HasRotaryEncoder(void)
virtual bool SetMixerFGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the foreground input control value for the given mixer/keyer.
virtual NTV2BreakoutType GetBreakoutHardware(void)
bool CanDoAudio192K(void)
@ kDeviceGetNumAESAudioInputChannels
The number of AES/EBU audio input channels on the device.
virtual bool GetDeinterlaceMode(ULWord &outValue)
virtual bool RemoveConnections(const NTV2XptConnections &inConnections)
Removes the given widget routing connections from the AJA device.
virtual bool GetConnectedInputs(const NTV2OutputCrosspointID inOutputXpt, NTV2InputCrosspointIDSet &outInputXpts)
Answers with all of the NTV2InputCrosspointIDs that are connected to the given NTV2OutputCrosspointID...
virtual bool GetAudioPlayCaptureModeEnable(const NTV2AudioSystem inAudioSystem, bool &outEnable)
Answers whether or not the device's Audio System is currently operating in a special mode in which it...
virtual bool GetHDMIInputStatus(ULWord &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1, const bool in12BitDetection=false)
Answers with the contents of the HDMI Input status register for the given HDMI input.
@ kDeviceHasRetailSupport
True if device is supported by AJA "retail" software (AJA ControlPanel & ControlRoom).
virtual bool Get64BitAutodetect(ULWord *autodetect64)
@ kDeviceHasGenlockv2
True if device has version 2 genlock hardware and/or firmware.
virtual bool HevcGetStatus(HevcDeviceStatus *pStatus)
Get the status of the hevc device.
virtual bool GetHDMIOut3DPresent(bool &outIs3DPresent)
NTV2OutputCrosspointID
Identifies a widget output, a signal source, that potentially can drive another widget's input (ident...
virtual bool SetHDMIHDRRedPrimaryX(const uint16_t inRedPrimaryX)
Sets the Display Mastering data for Red Primary X as defined in SMPTE ST 2086. This is Byte 11 and 12...
@ kDeviceGetNumFrameSyncs
The number of frame sync widgets on the device.
@ kDeviceGetNumHDMIVideoInputs
The number of HDMI video inputs on the device.
NTV2InputSource
Identifies a specific video input source.
NTV2HDMIColorimetry
Indicates or specifies the HDMI colorimetry.
virtual bool SetStereoCompressorOutputMode(const NTV2StereoCompressorOutputMode inNewValue)
virtual bool GetHDMIInVideoRange(NTV2HDMIRange &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's video black/white range.
virtual bool AncInsertSetReadParams(const UWord inSDIOutput, const ULWord inFrameNumber, const ULWord inF1Size, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Framesize inFrameSize=NTV2_FRAMESIZE_INVALID)
Configures the Anc inserter for the next frame's F1 Anc data to embed/transmit. (Call NTV2DeviceCanDo...
virtual bool GetEnableFramePulseReference(bool &outEnabled)
Answers whether or not the device's current frame pulse reference source is enabled....
virtual bool SetHDMIHDRWhitePointX(const uint16_t inWhitePointX)
Sets the Display Mastering data for White Point X as defined in SMPTE ST 2086. This is Byte 15 and 16...
virtual bool GetMultiRasterBypassEnable(bool &outEnabled)
virtual bool GetMixerVancOutputFromForeground(const UWord inWhichMixer, bool &outIsFromForegroundSource)
Answers whether or not the VANC source for the given mixer/keyer is currently the foreground video....
virtual bool GetFrameGeometry(NTV2FrameGeometry &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
UWord GetNumOutputConverters(void)
virtual bool GetHDMIInColorSpace(NTV2HDMIColorSpace &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current color space setting.
@ kRegSDI5678Input3GStatus
virtual bool GetColorCorrectionSaturation(const NTV2Channel inChannel, ULWord &outValue)
virtual bool ReadSDSaturationAdjustment(ULWord &outValue)
@ kDeviceCanDoAnalogVideoIn
ULWord GetMaxRegisterNumber(void)
virtual bool ReadOutputTimingControl(ULWord &outValue, const UWord inOutputSpigot=0)
Returns the current output timing control value for the given SDI output connector.
std::set< NTV2AudioChannelOctet > NTV2AudioChannelOctets
A set of distinct NTV2AudioChannelOctet values.
ULWord GetMaxTransferCount(void)
virtual bool GetRouting(CNTV2SignalRouter &outRouting)
Answers with the current signal routing between any and all widgets on the AJA device.
virtual bool Load12BitLUTTables(const NTV2DoubleArray &inRedLUT, const NTV2DoubleArray &inGreenLUT, const NTV2DoubleArray &inBlueLUT)
virtual bool ReadHDContrastAdjustment(ULWord &outValue)
virtual bool SetAudioMixerInputAudioSystem(const NTV2AudioMixerInput inMixerInput, const NTV2AudioSystem inAudioSystem)
Sets the Audio System that will drive the given input of the Audio Mixer.
bool GetUsedRegions(ULWordSequence &outBlks) const
Answers with the list of used memory regions.
virtual bool AutoCirculateFlush(const NTV2Channel inChannel, const bool inClearDropCount=false)
Flushes AutoCirculate for the given channel.
@ kDeviceGetNumCSCs
The number of colorspace converter widgets on the device.
bool GetRegions(ULWordSequence &outFree, ULWordSequence &outUsed, ULWordSequence &outBad) const
Answers with the lists of free, in-use and conflicting 8MB memory blocks. Each ULWord represents a re...
bool TranslateRegions(ULWordSequence &outRgns, const ULWordSequence &inRgns, const bool inIsQuad, const bool inIsQuadQuad) const
Translates an 8MB-chunked list of regions into another list of regions with frame indexes and sizes e...
@ kDeviceCanDoRGBPlusAlphaOut
True if device has CSCs capable of splitting the key (alpha) and YCbCr (fill) from RGB frame buffers ...
virtual bool DMABufferLock(const ULWord *pInBuffer, const ULWord inByteCount, bool inMap=false, bool inRDMA=false)
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
virtual bool GetHDMIHDRMaxContentLightLevel(uint16_t &outMaxContentLightLevel)
Answers with the Display Mastering data for the Max Content Light Level(Max CLL) value....
virtual bool GetConverterPulldown(ULWord &outValue)
virtual NTV2VideoFormat GetHDMIInputVideoFormat(NTV2Channel inHDMIInput=NTV2_CHANNEL1)
UWord GetNumHDMIVideoInputs(void)
virtual bool ReadProcAmpC2CRAdjustment(ULWord &outValue)
virtual bool GetHDMIHDRGreenPrimaryX(uint16_t &outGreenPrimaryX)
Answers with the Display Mastering data for Green Primary X as defined in SMPTE ST 2086....
virtual bool SetRS422Parity(const NTV2Channel inSerialPort, const NTV2_RS422_PARITY inParity)
Sets the parity control on the specified RS422 serial port.
virtual bool GetHDMIHDRWhitePointY(uint16_t &outWhitePointY)
Answers with the Display Mastering data for White Point Y as defined in SMPTE ST 2086....
virtual bool SetVideoDACMode(NTV2VideoDACMode inValue)
UWord GetNumInputConverters(void)
virtual bool GetHDMIOutAudioSource2Channel(NTV2AudioChannelPair &outValue, NTV2AudioSystem &outAudioSystem)
Answers with the HDMI output's current 2-channel audio source.
virtual bool EnableFramePulseReference(const bool inEnable)
Enables the device's frame pulse reference select.
virtual bool GetSDITRSError(const NTV2Channel inChannel)
virtual bool IS_CHANNEL_VALID(const NTV2Channel inChannel) const
virtual bool SetHDMIHDRBluePrimaryX(const uint16_t inBluePrimaryX)
Sets the Display Mastering data for Blue Primary X as defined in SMPTE ST 2086. This is Byte 7 and 8 ...
bool CanDoAESAudioIn(void)
virtual bool SetHDMIOutAudioSource2Channel(const NTV2AudioChannelPair inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the HDMI output's 2-channel audio source.
virtual bool GetDualLinkInputEnable(bool &outIsEnabled)
@ kNTV2EnumsID_DSKMode
Identifies the NTV2DSKMode enumerated type.
virtual bool GetDualLinkOutputEnable(bool &outIsEnabled)
virtual bool SetMixerRGBRange(const UWord inWhichMixer, const NTV2MixerRGBRange inRGBRange)
Sets the RGB range for the given mixer/keyer.
virtual bool GetAudioOutputPause(const NTV2AudioSystem inAudioSystem, bool &outIsPaused)
Answers if the device's Audio System is currently paused or not.
virtual ULWord StreamChannelStop(const NTV2Channel inChannel, NTV2StreamChannel &status)
Stop a stream. Put the stream is the idle state. For frame based video, the stream will idle on the b...
virtual bool GetInputVerticalEventCount(ULWord &outCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the number of input interrupt events that I successfully waited for on the given channel...
#define NTV2_SHOULD_BE_DEPRECATED(__f__)
virtual bool IS_OUTPUT_SPIGOT_INVALID(const UWord inOutputSpigot) const
virtual bool GetDownConvertMode(NTV2DownConvertMode &outValue)
virtual bool GetLUTTables(NTV2DoubleArray &outRedLUT, NTV2DoubleArray &outGreenLUT, NTV2DoubleArray &outBlueLUT)
Reads the LUT tables (as double-precision floating point values).
virtual bool SetStereoCompressorFlipLeftVert(const ULWord inNewValue)
virtual bool SetSDIOutputStandard(const UWord inOutputSpigot, const NTV2Standard inValue)
Sets the SDI output spigot's video standard.
@ kDeviceCanReportFrameSize
True if device can report its frame size.
virtual bool SetEmbeddedAudioInput(const NTV2EmbeddedAudioInput inEmbeddedSource, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the embedded (SDI) audio source for the given NTV2AudioSystem on the device.
NTV2VideoLimiting
These enum values identify the available SDI video output limiting modes.
#define NTV2_DEPRECATED_f(__f__)
virtual bool WriteHDCbOffsetAdjustment(const ULWord inNewValue)
@ kDeviceGetNumHDMIVideoOutputs
The number of HDMI video outputs on the device.
This is used by the CNTV2Card::ReadSDIStatistics function.
virtual ULWord StreamChannelWait(const NTV2Channel inChannel, NTV2StreamChannel &status)
Wait for any stream event. Returns for any state or buffer change.
@ kDeviceHasLEDAudioMeters
True if device has LED audio meters.
virtual bool DisableChannels(const NTV2ChannelSet &inChannels)
Disables the given FrameStore(s).
virtual bool GetAudioLoopBack(NTV2AudioLoopBack &outMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Answers if NTV2AudioLoopBack mode is currently on or off for the given NTV2AudioSystem.
virtual bool GetHDMIHDRRedPrimaryX(uint16_t &outRedPrimaryX)
Answers with the Display Mastering data for Red Primary X as defined in SMPTE ST 2086....
@ kDeviceCanChangeEmbeddedAudioClock
virtual ~CNTV2Card()
My destructor.
virtual bool Enable4KPSFOutMode(bool inEnable)
Sets 4K Down Convert PSF out Mode.
virtual bool SetLUTEnable(const bool inEnable, const NTV2Channel inLUT)
Enables or disables the given LUT.
virtual bool GetHDMIOutForceConfig(bool &outValue)
std::vector< NTV2RegInfo > NTV2RegisterWrites
@ kDeviceGetNumHDMIAudioOutputChannels
The number of HDMI audio output channels on the device.
UWord GetNumLTCInputs(void)
virtual bool SetSDIOut12GEnable(const NTV2Channel inChannel, const bool inEnable)
virtual bool BankSelectReadRegister(const NTV2RegInfo &inBankSelect, NTV2RegInfo &inOutRegInfo)
Reads the given set of registers from the bank specified in position 0.
virtual bool WriteSDIInVPID(const NTV2Channel inChannel, const ULWord inValA, const ULWord inValB)
virtual ULWord GetCRCErrorCountA(const NTV2Channel inChannel)
@ kDeviceHasSPIFlash
True if device has SPI flash hardware.
virtual bool Get4kSquaresEnable(bool &outIsEnabled, const NTV2Channel inChannel)
Answers whether the FrameStore bank's current SMPTE 425 "4K squares" (i.e., "2K quadrants") mode is e...
virtual bool GetDetectedAESChannelPairs(NTV2AudioChannelPairs &outDetectedChannelPairs)
Answers which AES/EBU audio channel pairs are present on the device.
@ kDeviceGetNumHDMIAudioInputChannels
The number of HDMI audio input channels on the device.
virtual bool GetFrameBufferQuarterSizeMode(NTV2Channel inChannel, NTV2QuarterSizeExpandMode &outValue)
virtual bool AncExtractInit(const UWord inSDIInput, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Standard inStandard=NTV2_STANDARD_INVALID)
Initializes the given SDI input's Anc extractor for custom Anc packet detection and de-embedding....
virtual bool GetHDMIInIsLocked(bool &outIsLocked, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers if the given HDMI input is genlocked or not.
virtual bool GetProgressivePicture(ULWord &outValue)
@ kDeviceNeedsRoutingSetup
True if device widget routing can be queried or changed.
NTV2RegisterWriteMode
These values are used to determine when certain register writes actually take effect....
virtual bool GetBaseAddress(NTV2Channel channel, ULWord **pBaseAddress)
virtual bool GetAnalogOutHTiming(ULWord &outValue)
std::bitset< 16 > NTV2AudioChannelsMuted16
Per-audio-channel mute state for up to 16 audio channels.
virtual bool GetHDMIHDRConstantLuminance(void)
virtual bool GetStereoCompressorOutputMode(NTV2StereoCompressorOutputMode &outValue)
@ kDeviceCanDoJ2K
True if device supports JPEG 2000 codec.
virtual bool SetAudioPCMControl(const NTV2AudioSystem inAudioSystem, const bool inIsNonPCM)
Determines whether or not all outgoing audio channel pairs are to be flagged as non-PCM for the given...
virtual bool DmaP2PTransferFrame(NTV2DMAEngine DMAEngine, ULWord frameNumber, ULWord frameOffset, ULWord transferSize, ULWord numSegments, ULWord segmentTargetPitch, ULWord segmentCardPitch, PCHANNEL_P2P_STRUCT pP2PData)
virtual bool GetAudioMixerInputChannelSelect(const NTV2AudioMixerInput inMixerInput, NTV2AudioChannelPair &outChannelPair)
Answers with the Audio Channel Pair that's currently driving the given input of the Audio Mixer.
virtual bool GetConverterOutStandard(NTV2Standard &outValue)
bool CanDoFramePulseSelect(void)
virtual bool GetHDMIHDRStaticMetadataDescriptorID(uint8_t &outSMDId)
virtual bool WriteSDContrastAdjustment(const ULWord inNewValue)
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
virtual bool AddDynamicBitfile(const std::string &inBitfilePath)
Adds the given bitfile to the list of available dynamic bitfiles.
virtual bool GetHDMIInColorimetry(NTV2HDMIColorimetry &outColorimetry, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current colorimetry.
UWord GetNum2022ChannelsSFP1(void)
virtual bool GetProgramStatus(SSC_GET_FIRMWARE_PROGRESS_STRUCT *statusStruct)
virtual bool SetHDMIOutProtocol(const NTV2HDMIProtocol inNewValue)
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool WriteSDHueAdjustment(const ULWord inNewValue)
@ kDeviceIsExternalToHost
True if device connects to the host with a cable.
virtual bool GetSDIInLevelBtoLevelAConversion(const UWord inInputSpigot, bool &outIsEnabled)
Answers with the device's current 3G level B to 3G level A conversion setting for the given SDI input...
virtual bool SetHeadphoneOutputGain(const ULWord inGainValue)
Sets the gain for the headphone out.
virtual bool SetAnalogAudioTransmitEnable(const NTV2Audio4ChannelSelect inChannelQuad, const bool inEnable)
Sets the specified bidirectional XLR audio connectors to collectively act as inputs or outputs.
virtual bool GetLHIVideoDACMode(NTV2LHIVideoDACMode &outValue)
virtual bool SetInputVerticalEventCount(const ULWord inCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Resets my input interrupt event tally for the given channel.
This struct replaces the old RP188_STRUCT.
virtual bool WriteHDBrightnessAdjustment(const ULWord inNewValue)
virtual bool SetMixerMode(const UWord inWhichMixer, const NTV2MixerKeyerMode inMode)
Sets the mode for the given mixer/keyer.
virtual bool SetHDMIHDRRedPrimaryY(const uint16_t inRedPrimaryY)
Sets the Display Mastering data for Red Primary Y as defined in SMPTE ST 2086. This is Byte 13 and 14...
bool CanDoEnhancedCSC(void)
virtual bool GetDisabledChannels(NTV2ChannelSet &outChannels)
Answers with the set of channels that are currently disabled.
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
virtual bool SetStereoCompressorLeftSource(const NTV2OutputCrosspointID inNewValue)
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
virtual bool SetHDMIOutTsiIO(const bool inTsiEnable)
Enables or disables two sample interleave I/O mode on the device's HDMI rasterizer.
virtual bool SetAESOutputSource(const NTV2Audio4ChannelSelect inAESAudioChannels, const NTV2AudioSystem inSrcAudioSystem, const NTV2Audio4ChannelSelect inSrcAudioChannels)
Changes the audio source for the given quad of AES audio output channels. By default,...
virtual bool GetStereoCompressorRightSource(NTV2OutputCrosspointID &outValue)
@ kDeviceCanDo8KVideo
True if device supports 8K video formats.
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
virtual bool SetHDMIHDRWhitePointY(const uint16_t inWhitePointY)
Sets the Display Mastering data for White Point Y as defined in SMPTE ST 2086. This is Byte 17 and 18...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
virtual bool EnableChannels(const NTV2ChannelSet &inChannels, const bool inDisableOthers=false)
Enables the given FrameStore(s).
virtual bool SetAudioOutputPause(const NTV2AudioSystem inAudioSystem, const bool inPausePlayout)
Pauses or resumes output of audio samples and advancement of the audio buffer pointer ("play head") o...
virtual bool SetSDIRelayManualControl(const NTV2RelayState inValue, const UWord inIndex0)
Sets the state of the given connector pair relays to NTV2_DEVICE_BYPASSED (or NTV2_THROUGH_DEVICE if ...
@ kDeviceHasMicrophoneInput
True if device has a microphone input connector.
virtual bool SetRS422BaudRate(const NTV2Channel inSerialPort, const NTV2_RS422_BAUD_RATE inBaudRate)
Sets the baud rate of the specified RS422 serial port.
virtual bool AncInsertIsEnabled(const UWord inSDIOutput, bool &outIsEnabled)
Answers with the run state of the given Anc inserter – i.e. if its "memory reader" is enabled or not....
virtual bool GetHDMIInputColor(NTV2LHIHDMIColorSpace &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current colorspace for the given HDMI input.
virtual bool AncExtractIsEnabled(const UWord inSDIInput, bool &outIsEnabled)
Answers whether the given SDI input's Anc extractor is enabled/active or not. (Call NTV2DeviceCanDoCu...
bool TagVideoFrames(CNTV2Card &inDevice)
static bool GenerateGammaTable(const NTV2LutType inLUTType, const int inBank, NTV2DoubleArray &outTable, const NTV2LutBitDepth inBitDepth=NTV2_LUT10Bit)
ULWord GetNumDMAEngines(void)
virtual bool SetSDIOut3GbEnable(const NTV2Channel inChannel, const bool inEnable)
virtual bool SetMultiRasterBypassEnable(const bool inEnable)
virtual bool SetAudioMixerOutputChannelsMute(const NTV2AudioChannelsMuted16 inMutes)
Mutes or enables the individual output audio channels of the Audio Mixer.
std::multimap< NTV2InputXptID, NTV2OutputXptID > NTV2PossibleConnections
A map of zero or more one-to-many possible NTV2InputXptID to NTV2OutputXptID connections.
UByte NTV2DID
An ancillary Data IDentifier.
virtual bool GetHDMIOutBitDepth(NTV2HDMIBitDepth &outValue)
virtual NTV2VideoFormat GetSDIInputVideoFormat(NTV2Channel inChannel, bool inIsProgressive=false)
Returns the video format of the signal that is present on the given SDI input source.
virtual bool GetHDMIHDRRedPrimaryY(uint16_t &outRedPrimaryY)
Answers with the Display Mastering data for Red Primary Y as defined in SMPTE ST 2086....
virtual bool AbortMailBoxLock(void)
NTV2VPIDTransferCharacteristics
virtual bool ReadVirtualData(const ULWord inTag, void *outVirtualData, const ULWord inVirtualDataSize)
Reads the block of virtual data for a specific tag.
virtual bool SetSDIOutputDS2AudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the Audio System that will supply audio for the given SDI output's audio embedder for Data Strea...
NTV2FrameGeometry
Identifies a particular video frame geometry.
virtual bool IsChannelEnabled(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the given FrameStore is enabled.
@ kDeviceCanDo425Mux
True if the device supports SMPTE 425 mux control.
virtual bool WriteAnalogLTCOutput(const UWord inLTCOutput, const RP188_STRUCT &inRP188Data)
Writes the given timecode to the specified analog LTC output register.
virtual bool SetColorSpaceUseCustomCoefficient(const ULWord inUseCustomCoefficient, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool SetMultiLinkAudioMode(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Sets the multi-link audio mode for the given audio system.
bool CanDoWidget(const NTV2WidgetID inWgtID)
virtual ULWord GetFrameBufferSize(void) const
A Mac-specific implementation of CNTV2DriverInterface.
virtual bool SetHDMIOutColorSpace(const NTV2HDMIColorSpace inNewValue)
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
virtual bool ReadSDContrastAdjustment(ULWord &outValue)
std::set< NTV2DeviceID > NTV2DeviceIDSet
A set of NTV2DeviceIDs.
virtual bool SetVPIDLuminance(const NTV2VPIDLuminance inValue, const NTV2Channel inChannel)
CNTV2Card()
My default constructor.
virtual bool GetMixerRGBRange(const UWord inWhichMixer, NTV2MixerRGBRange &outRGBRange)
Answers with the given mixer/keyer's current RGB Range.
@ kNTV2EnumsID_Channel
Identifies the NTV2Channel enumerated type.
static ULWordSet CoalesceRegions(const ULWordSequence &inRgn1, const ULWordSequence &inRgn2, const ULWordSequence &inRgn3)
NTV2ConversionMode GetConversionMode(const NTV2VideoFormat inFormat, const NTV2VideoFormat outFormat)
NTV2DIDSet::iterator NTV2DIDSetIter
Handy non-const iterator to iterate over an NTV2DIDSet.
virtual ULWord StreamChannelInitialize(const NTV2Channel inChannel)
Initialize a stream. Put the stream queue and hardware in a known good state ready for use....
virtual bool SetInputVideoSelect(NTV2InputVideoSelect inInputSelect)
bool CanDoFrameStore1Display(void)
@ kDeviceGetMaxAudioChannels
The maximum number of audio channels that a single Audio System can support on the device.
virtual bool StartAudioOutput(const NTV2AudioSystem inAudioSystem, const bool inWaitForVBI=false)
Starts the playout side of the given NTV2AudioSystem, reading outgoing audio samples from the Audio S...
virtual bool GetDriverVersionComponents(UWord &outMajor, UWord &outMinor, UWord &outPoint, UWord &outBuild)
Answers with the individual version components of this device's driver.
virtual bool SetStereoCompressorFlipRightHorz(const ULWord inNewValue)
virtual bool UnsubscribeEvent(const INTERRUPT_ENUMS inEventCode)
Unregisters me so I'm no longer notified when the given event/interrupt is triggered on the AJA devic...
@ kDeviceCanDoRP188
True if device can insert and/or extract RP-188/VITC.
virtual bool DeviceAddressToFrameNumber(const uint64_t inAddress, UWord &outFrameNumber, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the frame number that contains the given address.
@ kDeviceCanDoAudio2Channels
virtual bool IsMultiRasterWidgetChannel(const NTV2Channel inChannel)
virtual bool SetMixerVancOutputFromForeground(const UWord inWhichMixer, const bool inFromForegroundSource=true)
Sets the VANC source for the given mixer/keyer to the foreground video (or not). See the Ancillary Da...
bool CanDoConversionMode(const NTV2ConversionMode inMode)
virtual bool SetDefaultVideoOutMode(ULWord mode)
virtual bool GetMixerFGMatteEnabled(const UWord inWhichMixer, bool &outIsEnabled)
Answers if the given mixer/keyer's foreground matte is enabled or not.
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual bool IsBufferSizeSetBySW(void)
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
UWord GetNumDownConverters(void)
virtual bool GetPCIDeviceID(ULWord &outPCIDeviceID)
Answers with my PCI device ID.
virtual std::string GetFPGAVersionString(const NTV2XilinxFPGA inFPGA=eFPGAVideoProc)
@ kDeviceCanDo12GSDI
True if device has 12G SDI connectors.
@ kDeviceCanDoHDVideo
True if device can handle HD (High Definition) video.
bool CanDoMultiFormat(void)
virtual bool SetAudioMixerInputGain(const NTV2AudioMixerInput inMixerInput, const NTV2AudioMixerChannel inChannel, const ULWord inGainValue)
Sets the gain for the given input of the Audio Mixer.
virtual bool SetConverterInStandard(const NTV2Standard inValue)
NTV2Audio4ChannelSelect
Identifies a contiguous, adjacent group of four audio channels.
virtual bool SetHDMIInputRange(const NTV2HDMIRange inNewValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the given HDMI input's input range.
std::set< NTV2AudioChannelQuad > NTV2AudioChannelQuads
A set of distinct NTV2AudioChannelQuad values.
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
virtual bool SetInputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the input frame index number for the given FrameStore. This identifies which frame in device SDR...
@ kDeviceCanDoCustomAnc
True if device has ANC inserter/extractor firmware.
virtual bool GetColorSpaceUseCustomCoefficient(ULWord &outUseCustomCoefficient, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
virtual bool SetHDMIV2Mode(const NTV2HDMIV2Mode inMode)
Sets HDMI V2 mode for the device.
virtual bool GetLUTV2HostAccessBank(NTV2ColorCorrectionHostAccessBank &outValue, const NTV2Channel inChannel)
@ kDeviceGetActiveMemorySize
The size, in bytes, of the device's active RAM available for video and audio.
virtual bool SetEnableVANCData(const bool inVANCenabled, const bool inTallerVANC, const NTV2Standard inStandard, const NTV2FrameGeometry inGeometry, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool GetVPIDTransferCharacteristics(NTV2VPIDTransferCharacteristics &outValue, const NTV2Channel inChannel)
virtual bool Has12BitLUTSupport(void)
virtual bool GetHDMIInputStatusRegNum(ULWord &outRegNum, const NTV2Channel inChannel=NTV2_CHANNEL1, const bool in12BitDetection=false)
Answers with the HDMI Input status register number for the given HDMI input.
bool CanDoHDMIOutStereo(void)
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=false)
Returns the video format of the signal that is present on the given input source.
virtual bool IsRP188BypassEnabled(const NTV2Channel inSDIOutput, bool &outIsBypassEnabled)
Answers if the SDI output's RP-188 bypass mode is enabled or not.
virtual bool DMAClearAncRegion(const UWord inStartFrameNumber, const UWord inEndFrameNumber, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All, const NTV2Channel=NTV2_CHANNEL1)
Clears the ancillary data region in the device frame buffer for the specified frames.
virtual bool DisableRP188Bypass(const NTV2Channel inSDIOutput)
Configures the SDI output's embedder to embed SMPTE 12M timecode specified in calls to CNTV2Card::Set...
virtual bool GetConverterInStandard(NTV2Standard &outValue)
enum NTV2VPIDLuminance NTV2HDRLuminance
virtual bool GetVPIDLuminance(NTV2VPIDLuminance &outValue, const NTV2Channel inChannel)
virtual bool ReadProcAmpC2CBAdjustment(ULWord &outValue)
virtual bool SetStereoCompressorFlipLeftHorz(const ULWord inNewValue)
virtual bool GetHeadphoneOutputGain(ULWord &outGainValue)
Answers with the current gain setting for the headphone out.
virtual bool SetHDMIOutDecimateMode(const bool inEnable)
Enables or disables decimate mode on the device's HDMI rasterizer, which halves the output frame rate...
std::vector< double > NTV2DoubleArray
An array of double-precision floating-point values.
virtual bool SetQuadQuadFrameEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables "quad-quad" 8K frame mode on the device.
virtual bool SetStereoCompressorFlipRightVert(const ULWord inNewValue)
virtual bool SetHDMIOutAudioSource8Channel(const NTV2Audio8ChannelSelect inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the HDMI output's 8-channel audio source.
@ kDeviceGetTotalNumAudioSystems
The total number of audio systems on the device, including host audio and mixer audio systems,...
bool HasHeadphoneJack(void)
virtual bool DMAStreamStart(ULWord *inBuffer, const ULWord inByteCount, const NTV2Channel inChannel, const bool inToHost)
Streaming transfers.
UWord GetNumVideoInputs(void)
virtual bool SetQuadQuadSquaresEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables quad-quad-frame (8K) squares mode on the device.
UWord GetNumLUTBanks(void)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
@ kDeviceHasNWL
True if device has NorthWest Logic DMA hardware.
virtual bool GetOutputVerticalInterruptCount(ULWord &outCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the number of output vertical interrupts handled by the driver for the given output chan...
bool CanChangeEmbeddedAudioClock(void)
virtual bool GetAnalogInputADCMode(NTV2LSVideoADCMode &outValue)
virtual bool SetAnalogOutHTiming(ULWord inValue)
@ kDeviceCanReportFailSafeLoaded
True if device can report if its "fail-safe" firmware is loaded/running.
const NTV2AudioChannelsMuted16 NTV2AudioChannelsEnableAll
All 16 audio channels unmuted/enabled.
virtual bool DMABufferAutoLock(const bool inEnable, const bool inMap=false, const ULWord64 inMaxLockSize=0)
Enables or disables automatic buffer locking.
bool CanReportFrameSize(void)
virtual bool GetStereoCompressorFlipLeftVert(ULWord &outValue)
virtual bool IsSDStandard(bool &outIsStandardDef, NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool SetHDMIHDRGreenPrimaryY(const uint16_t inGreenPrimaryY)
Sets the Display Mastering data for Green Primary Y as defined in SMPTE ST 2086. This is Byte 5 and 6...
virtual bool GetDitherFor8BitInputs(const NTV2Channel inChannel, ULWord &outDither)
UWord GetDACVersion(void)
@ kDeviceCanDoRateConvert
True if device can do frame rate conversion.
virtual bool WaitForInputFieldID(const NTV2FieldID inFieldID, const NTV2Channel inChannel=NTV2_CHANNEL1)
Efficiently sleeps the calling thread/process until the next input VBI for the given field and input ...
virtual bool GetSDIInputIsProgressive(const NTV2Channel channel)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetHDMIHDRBluePrimaryY(const uint16_t inBluePrimaryY)
Sets the Display Mastering data for Blue Primary Y as defined in SMPTE ST 2086. This is Byte 9 and 10...
std::vector< NTV2DeviceID > NTV2DeviceIDList
An ordered list of NTV2DeviceIDs.
virtual bool GetLTCInputEnable(bool &outIsEnabled)
Answers true if the device is currently configured to read analog LTC from the reference input connec...
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
virtual bool GetSupportedVideoFormats(NTV2VideoFormatSet &outFormats)
Returns a std::set of NTV2VideoFormat values that I support.
virtual bool GetRS422BaudRate(const NTV2Channel inSerialPort, NTV2_RS422_BAUD_RATE &outBaudRate)
Answers with the current baud rate of the specified RS422 serial port.
virtual bool GetSuspendHostAudio(bool &outIsSuspended)
Answers as to whether or not the host OS audio services for the AJA device (e.g. CoreAudio on MacOS) ...
virtual bool SetHDMIHDRMaxFrameAverageLightLevel(const uint16_t inMaxFrameAverageLightLevel)
Sets the Display Mastering data for the Max Frame Average Light Level(Max FALL) value....
virtual bool GetRP188Mode(const NTV2Channel inChannel, NTV2_RP188Mode &outMode)
Returns the current RP188 mode – NTV2_RP188_INPUT or NTV2_RP188_OUTPUT – for the given channel.
@ kDeviceCanDoAudio8Channels
#define AJA_RETAIL_DEFAULT
virtual bool GetAudioMemoryOffset(const ULWord inOffsetBytes, ULWord &outAbsByteOffset, const NTV2AudioSystem inAudioSystem, const bool inCaptureBuffer=false)
Answers with the byte offset in device SDRAM into the specified Audio System's audio buffer.
NTV2AncillaryDataRegion
These enumerations identify the various ancillary data regions located at the bottom of each frame bu...
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=true)
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
virtual bool SetPulldownMode(NTV2Channel inChannel, bool inValue)
NTV2EmbeddedAudioInput
This enum value determines/states which SDI video input will be used to supply audio samples to an au...
virtual bool GetSDIWatchdogStatus(NTV2RelayState &outValue)
Answers if the watchdog timer would put the SDI relays into NTV2_DEVICE_BYPASSED or NTV2_THROUGH_DEVI...
@ kDeviceHasHEVCM30
True if device has an HEVC M30 encoder/decoder.
virtual bool GetHDMIInputRange(NTV2HDMIRange &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's current input range setting.
virtual ULWord GetSerialNumberLow(void)
bool GetBadRegions(ULWordSequence &outBlks) const
Answers with the list of colliding and illegal memory regions.
bool HasBiDirectionalAnalogAudio(void)
virtual bool SetHDRData(const HDRFloatValues &inFloatValues)
virtual bool SetAudioInputDelay(const NTV2AudioSystem inAudioSystem, const ULWord inDelay)
Sets the audio input delay for the given Audio System on the device.
@ kDeviceCanDo2KVideo
True if device can handle 2Kx1556 (film) video.
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
virtual bool GetSDIOutRGBLevelAConversion(const UWord inOutputSpigot, bool &outIsEnabled)
Answers with the device's current RGB-over-3G-level-A conversion at the given SDI output spigot (assu...
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
virtual bool GetNominalMinMaxHV(int &outNominalH, int &outMinH, int &outMaxH, int &outNominalV, int &outMinV, int &outMaxV)
virtual bool SetDualLinkOutputEnable(const bool inIsEnabled)
virtual bool GetRunningFirmwareRevision(UWord &outRevision)
Reports the revision number of the currently-running firmware.
virtual bool IsDynamicDevice(void)
virtual bool DisableInterrupt(const INTERRUPT_ENUMS inEventCode)
bool TagMemoryBlock(const uint64_t inStartAddr, const uint64_t inByteLength, const std::string &inTag)
@ kDeviceGetNumUpConverters
The number of up-converters on the device.
@ kDeviceGetNumLUTBanks
The number of LUT banks on the device. (New in SDK 17.0)
virtual bool SetAlphaFromInput2Bit(ULWord inValue)
virtual bool AncExtractSetEnable(const UWord inSDIInput, const bool inIsEnabled)
Enables or disables the given SDI input's Anc extractor. (Call NTV2DeviceCanDoCustomAnc to determine ...
virtual bool HasMultiRasterWidget(void)
bool CanDo12gRouting(void)
@ kDeviceHasBiDirectionalAnalogAudio
True if device has a bi-directional analog audio connector.
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
bool IsExternalToHost(void)
NTV2HDMIOut3DMode
This specifies the HDMI Out Stereo 3D Mode.
virtual bool GetEnabledChannels(NTV2ChannelSet &outChannels)
Answers with the set of channels that are currently enabled.
virtual bool SetColorSpaceMethod(const NTV2ColorSpaceMethod inCSCMethod, const NTV2Channel inChannel)
Selects the color space converter operation method.
virtual bool GetHDMIOutAudioFormat(NTV2AudioFormat &outValue)
Answers with the HDMI output's current audio format.
virtual bool SetRegisterWriteMode(const NTV2RegisterWriteMode inValue, const NTV2Channel inFrameStore=NTV2_CHANNEL1)
Sets the FrameStore's NTV2RegisterWriteMode, which determines when CNTV2Card::SetInputFrame or CNTV2C...
virtual bool ReadHDSaturationAdjustmentCb(ULWord &outValue)
virtual bool GetRoutingForChannel(const NTV2Channel inChannel, CNTV2SignalRouter &outRouting)
Answers with the current signal routing for the given channel.
NTV2MixerKeyerMode
These enum values identify the mixer mode.
NTV2Audio8ChannelSelect
Identifies a contiguous, adjacent group of eight audio channels.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
virtual bool SetHDMIV2TxBypass(const bool inBypass)
virtual bool SetAudioCaptureEnable(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Enables or disables the writing of incoming audio into the given Audio System's capture buffer.
virtual NTV2FrameGeometry GetSDIInputGeometry(const NTV2Channel channel)
@ kDeviceGetNumAnalogVideoOutputs
The number of analog video outputs on the device.
bool CanDoDSKOpacity(void)
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
virtual bool GetAudioPCMControl(const NTV2AudioSystem inAudioSystem, bool &outIsNonPCM)
Answers whether or not all outgoing audio channel pairs are currently being flagged as non-PCM for th...
NTV2AudioSource
This enum value determines/states where an audio system will obtain its audio samples.
@ kDeviceGetNum2022ChannelsSFP2
The number of 2022 channels configured on SFP 2 on the device.
virtual bool SetAudioAnalogLevel(const NTV2AudioLevel value, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
virtual bool GetSmpte372(ULWord &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the device's current SMPTE-372 (dual-link) mode, whether it's enabled or not.
virtual bool WriteProcAmpC1CRAdjustment(const ULWord inNewValue)
virtual bool GetTsiFrameEnable(bool &outIsEnabled, const NTV2Channel inChannel)
Returns the current SMPTE 425 two-sample-interleave frame mode on the device, whether it's enabled or...
virtual bool SetHDMIOutAudioFormat(const NTV2AudioFormat inNewValue)
Sets the HDMI output's audio format.
@ kDeviceCanDoDSKOpacity
True if device mixer/keyer supports adjustable opacity.
bool HasAudioMonitorRCAJacks(void)
bool CanDoVideoProcessing(void)
NTV2Crosspoint
Logically, these are an NTV2Channel combined with an NTV2Mode.
@ kDeviceGetNumOutputConverters
The number of output converter widgets on the device.
bool CanDoQuarterExpand(void)
NTV2_RS422_BAUD_RATE
These enum values identify RS-422 serial port baud rate configuration.
@ kDeviceGetNumEmbeddedAudioInputChannels
The number of SDI-embedded input audio channels supported by the device.
virtual bool HevcWriteRegister(ULWord address, ULWord value, ULWord mask=0xffffffff, ULWord shift=0)
Write an hevc register.
@ kDeviceGetNumSerialPorts
The number of RS-422 serial ports on the device.
virtual bool SetLHIHDMIOutColorSpace(const NTV2LHIHDMIColorSpace inNewValue)
@ NTV2_VPID_Luminance_YCbCr
bool AssessDevice(CNTV2Card &inDevice, const bool inIgnoreStoppedAudioBuffers=false)
Assesses the given device.
virtual bool SetEncodeAsPSF(NTV2Channel inChannel, NTV2EncodeAsPSF inValue)
NTV2DIDSet::const_iterator NTV2DIDSetConstIter
Handy const iterator to iterate over an NTV2DIDSet.
virtual bool ReadLUTTables(UWordSequence &outRedLUT, UWordSequence &outGreenLUT, UWordSequence &outBlueLUT)
Reads the LUT tables (as raw, unsigned 10-bit integers).
virtual bool GetAudioMixerInputLevels(const NTV2AudioMixerInput inMixerInput, const NTV2AudioChannelPairs &inChannelPairs, std::vector< uint32_t > &outLevels)
Answers with the Audio Mixer's current audio input levels.
virtual bool ReadSDIStatistics(NTV2SDIInStatistics &outStats)
For devices that support it (see the NTV2DeviceCanDoSDIErrorChecks function in "ntv2devicefeatures....
virtual bool GetSDIInput3GbPresent(bool &outValue, const NTV2Channel channel)
@ kDeviceCanDoQREZ
True if device can handle QRez.
virtual bool SetHDMIOut3DMode(const NTV2HDMIOut3DMode inValue)
NTV2AudioBufferSize
Represents the size of the audio buffer used by a device audio system for storing captured samples or...
virtual ULWord DeviceGetAudioFrameBuffer2(void)
virtual bool GetMixerCoefficient(const UWord inWhichMixer, ULWord &outMixCoefficient)
Returns the current mix coefficient the given mixer/keyer.
bool CanReportFailSafeLoaded(void)
virtual bool GetStereoCompressorStandard(NTV2Standard &outValue)
@ NTV2_AncRgn_All
Identifies "all" ancillary data regions.
virtual bool GetConverterInRate(NTV2FrameRate &outValue)
bool CanDoChannel(const NTV2Channel inChannel)
virtual bool GetTransmitSDIs(NTV2ChannelSet &outXmitSDIs)
Answers with the transmitting/output SDI connectors.
virtual bool GetHDMIInDynamicRange(HDRRegValues &outRegValues, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the given HDMI input's video dynamic range and mastering information.
@ kDeviceGetNumCrossConverters
The number of cross-converters on the device.
@ kDeviceCanDoIsoConvert
True if device can do ISO conversion.
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
enum NTV2VideoFrameBufferOrientation NTV2FBOrientation
@ kDeviceCanDoMultiFormat
True if device can simultaneously handle different video formats on more than one SDI input or output...
UWord GetNumAESAudioInputChannels(void)
virtual bool SetLUTV2OutputBank(const NTV2Channel inLUTWidget, const ULWord inBank)
virtual bool GetHDMIHDRMaxMasteringLuminance(uint16_t &outMaxMasteringLuminance)
Answers with the Display Mastering data for the Max Mastering Luminance value as defined in SMPTE ST ...
virtual bool SetAudioMixerLevelsSampleCount(const ULWord inSampleCount)
Sets the Audio Mixer's sample count it uses for measuring audio levels.
virtual bool SetColorSpaceRGBBlackRange(const NTV2_CSC_RGB_Range inRange, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the RGB range for the given CSC.
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
bool CanDoPCMControl(void)
virtual bool SetColorSpaceMatrixSelect(const NTV2ColorSpaceMatrixType inType, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the matrix type to be used for the given CSC, typically NTV2_Rec601Matrix or NTV2_Rec709Matrix.
virtual bool WriteSDBrightnessAdjustment(const ULWord inNewValue)
virtual bool GetAudioMixerInputChannelsMute(const NTV2AudioMixerInput inMixerInput, NTV2AudioChannelsMuted16 &outMutes)
Answers with a std::bitset that indicates which input audio channels of the given Audio Mixer input a...
NTV2AudioChannelPairs::const_iterator NTV2AudioChannelPairsConstIter
Handy const iterator to iterate over a set of distinct NTV2AudioChannelPair values.
std::string SerialNum64ToString(const uint64_t &inSerNum)
virtual bool WriteProcAmpC1YAdjustment(const ULWord inNewValue)
virtual Word GetPCIFPGAVersion(void)
virtual bool BankSelectWriteRegister(const NTV2RegInfo &inBankSelect, const NTV2RegInfo &inRegInfo)
Writes the given set of registers to the bank specified at position 0.
virtual bool DisableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Prevents the CNTV2Card instance from waiting for and responding to vertical blanking interrupts origi...
bool CanDoAnalogVideoOut(void)
@ kDeviceGetNumLTCOutputs
The number of analog LTC outputs on the device.
virtual bool SetVideoVOffset(const int inVOffset, const UWord inOutputSpigot=0)
Adjusts the vertical timing offset, in lines, for the given SDI output connector.
virtual bool SetDownConvertMode(const NTV2DownConvertMode inValue)
const ULWord LUTTablePartitionSize
virtual bool GetConnectedOutput(const NTV2InputCrosspointID inInputXpt, NTV2OutputCrosspointID &outOutputXpt)
Answers with the currently connected NTV2OutputCrosspointID for the given NTV2InputCrosspointID.
UWord GetNumHDMIAudioInputChannels(void)
virtual bool SetFrameGeometry(NTV2FrameGeometry inGeometry, bool inIsRetail=true, NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the frame geometry of the given channel.
virtual bool GetInputVerticalInterruptCount(ULWord &outCount, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the number of input vertical interrupts handled by the driver for the given input channe...
virtual bool GetAlphaFromInput2Bit(ULWord &outValue)
virtual bool AncExtractGetBufferOverrun(const UWord inSDIInput, bool &outIsOverrun, const UWord inField=0)
Answers whether or not the given SDI input's Anc extractor reached its buffer limits....
@ kDeviceCanDoStereoOut
True if device supports 3D video output over dual-stream SDI.
virtual bool GetConnectedInput(const NTV2OutputCrosspointID inOutputXpt, NTV2InputCrosspointID &outInputXpt)
Answers with a connected NTV2InputCrosspointID for the given NTV2OutputCrosspointID.
virtual bool ReadLineCount(ULWord &outValue)
Answers with the line offset into the frame currently being read (NTV2_MODE_DISPLAY) or written (NTV2...
@ kDeviceGetNumLTCInputs
The number of analog LTC inputs on the device.
UWord GetNumBufferedAudioSystems(void)
virtual bool GetFrameBufferOrientation(const NTV2Channel inChannel, NTV2FBOrientation &outValue)
Answers with the current frame buffer orientation for the given NTV2Channel.
virtual bool GetAudioMixerInputGain(const NTV2AudioMixerInput inMixerInput, const NTV2AudioMixerChannel inChannel, ULWord &outGainValue)
Answers with the current gain setting for the Audio Mixer's given input.
@ kNTV2EnumsID_PixelFormat
Identifies the NTV2PixelFormat enumerated type.
static NTV2DIDSet AncExtractGetDefaultDIDs(const bool inHDAudio=true)
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
UWord GetNumHDMIAudioOutputChannels(void)
virtual bool GetVPIDColorimetry(NTV2VPIDColorimetry &outValue, const NTV2Channel inChannel)
virtual bool AncExtractSetComponents(const UWord inSDIInput, const bool inVancY, const bool inVancC, const bool inHancY, const bool inHancC)
Enables or disables the Anc extraction components for the given SDI input. (Call NTV2DeviceCanDoCusto...
NTV2StereoCompressorOutputMode
@ NTV2_AUDIOSYSTEM_INVALID
virtual bool GetFrameBufferQuality(NTV2Channel inChannel, NTV2FrameBufferQuality &outValue)