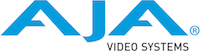 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
28 string hostName(inHostName);
95 string dateStr, timeStr;
99 oss << dateStr <<
" at " << timeStr;
101 oss <<
"Unavailable";
110 return ReadRegister (48, status) ? ((status >> 8) & 0xFF) : -1;
118 oss << hex << version;
125 static const string sDriverBuildTypes [] = {
"",
"b",
"a",
"d"};
126 UWord versions[4] = {0, 0, 0, 0};
133 const string & dabr (sDriverBuildTypes[versBits >> 30]);
135 oss <<
DEC(versions[0]) <<
"." <<
DEC(versions[1]) <<
"." <<
DEC(versions[2]);
137 oss <<
"." <<
DEC(versions[3]);
139 oss << dabr <<
DEC(versions[3]);
147 outMajor = outMinor = outPoint = outBuild = 0;
148 ULWord driverVersionULWord (0);
151 if (!driverVersionULWord)
208 const uint64_t result((hi << 32) | lo);
224 if (outSerialNumberString.empty())
226 outSerialNumberString =
"INVALID?";
232 outSerialNumberString =
"5" + outSerialNumberString;
236 outSerialNumberString =
"6" + outSerialNumberString;
238 outSerialNumberString =
"7" + outSerialNumberString;
242 ULWord serialArray[] = {0,0,0,0};
249 outSerialNumberString.clear();
250 for (
int serialIndex = 0; serialIndex < 4; serialIndex++)
252 if (serialArray[serialIndex] != 0xffffffff)
254 for (
int i = 0; i < 4; i++)
256 char tempChar = ((serialArray[serialIndex] >> (i*8)) & 0xff);
257 if (tempChar > 0 && tempChar !=
'.')
258 outSerialNumberString.push_back(tempChar);
277 ::memset (&bitFileInfo, 0,
sizeof (bitFileInfo));
285 outDateStr =
reinterpret_cast <char *
> (&bitFileInfo.
dateStr [0]);
286 outTimeStr =
reinterpret_cast <char *
> (&bitFileInfo.
timeStr [0]);
295 oss << inBitFileInfo.
dateStr <<
" " << inBitFileInfo.
timeStr <<
" ";
305 outIsSafeBoot =
false;
314 outCanWarmBoot =
false;
322 outCanWarmBoot =
true;
334 const bool bPhonyKBox (
false);
382 #if !defined(NTV2_DEPRECATE_16_3)
496 #endif // !defined(NTV2_DEPRECATE_16_3)
504 inOutStr <<
"(none)";
531 inOutStr << *iter << endl;
540 inOutStr <<
xHEX0N(uint16_t(*it),2);
541 if (++it != inDIDs.end())
557 if (!inDevice.IsOpen())
562 mNumFrames =
UWord(totalBytes / m8MB);
563 if (totalBytes % m8MB)
564 {mNumFrames++; cerr <<
DEC(totalBytes % m8MB) <<
" leftover/spare bytes -- last frame is partial frame" << endl;}
565 for (
UWord frm(0); frm < mNumFrames; frm++)
573 for (FrameTagsConstIter it(mFrameTags.begin()); it != mFrameTags.end(); ++it)
576 oss <<
DEC0N(it->first,3) <<
": " <<
aja::join(tags,
", ") << endl;
584 for (
size_t ndx(0); ndx < inRgn1.size(); ndx++)
585 if (result.find(inRgn1.at(ndx)) == result.end())
586 result.insert(inRgn1.at(ndx));
587 for (
size_t ndx(0); ndx < inRgn2.size(); ndx++)
588 if (result.find(inRgn2.at(ndx)) == result.end())
589 result.insert(inRgn2.at(ndx));
590 for (
size_t ndx(0); ndx < inRgn3.size(); ndx++)
591 if (result.find(inRgn3.at(ndx)) == result.end())
592 result.insert(inRgn3.at(ndx));
604 const ULWord rgnInfo(*it);
605 const UWord startBlk(rgnInfo >> 16), numBlks(
UWord(rgnInfo & 0x0000FFFF));
609 oss <<
"Frms " <<
DEC0N(startBlk,3) <<
"-" <<
DEC0N(startBlk+numBlks-1,3) <<
" : ";
611 oss <<
"Frm " <<
DEC0N(startBlk,3) <<
" : ";
623 outFree.clear(); outUsed.clear(); outBad.clear();
624 FrameTagsConstIter it(mFrameTags.begin());
625 if (it == mFrameTags.end())
627 UWord frmStart(it->first), lastFrm(frmStart);
629 while (++it != mFrameTags.end())
636 if (frmStart != lastFrm)
637 outFree.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
639 outFree.push_back((
ULWord(frmStart) << 16) |
ULWord(1));
641 else if (runTags.size() > 1)
643 if (frmStart != lastFrm)
644 outBad.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
650 if (frmStart != lastFrm)
651 outUsed.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
653 outUsed.push_back((
ULWord(frmStart) << 16) |
ULWord(1));
655 frmStart = lastFrm = it->first;
663 if (frmStart != lastFrm)
664 outFree.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
666 outFree.push_back((
ULWord(frmStart) << 16) |
ULWord(1));
668 else if (runTags.size() > 1)
670 if (frmStart != lastFrm)
671 outBad.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
677 if (frmStart != lastFrm)
678 outUsed.push_back((
ULWord(frmStart) << 16) |
ULWord(lastFrm-frmStart+1));
680 outUsed.push_back((
ULWord(frmStart) << 16) |
ULWord(1));
688 FrameTagsConstIter it(mFrameTags.find(inIndex));
689 if (it == mFrameTags.end())
691 outTags = it->second;
697 FrameTagsConstIter it(mFrameTags.find(inIndex));
698 if (it == mFrameTags.end())
700 return it->second.size();
706 if (inIsQuad && inIsQuadQuad)
708 if (inSrcRgns.empty())
710 const UWord _8MB_frames_per_dest_frame(
UWord(GetIntrinsicFrameByteCount() / m8MB) * (inIsQuad?4:1) * (inIsQuadQuad?16:1));
711 if (!_8MB_frames_per_dest_frame)
713 if (_8MB_frames_per_dest_frame == 1)
714 {outDestRgns = inSrcRgns;
return true;}
717 for (
size_t ndx(0); ndx < inSrcRgns.size(); ndx++)
718 {
const ULWord val(inSrcRgns.at(ndx));
719 ULWord startBlkOffset(val >> 16), lengthBlks(val & 0x0000FFFF);
720 startBlkOffset = startBlkOffset / _8MB_frames_per_dest_frame + (startBlkOffset % _8MB_frames_per_dest_frame ? 1 : 0);
721 lengthBlks = lengthBlks / _8MB_frames_per_dest_frame;
722 outDestRgns.push_back((startBlkOffset << 16) | lengthBlks);
730 bool isReading(
false), isWriting(
false);
735 tag <<
"Aud" <<
DEC(audSys+1);
740 TagMemoryBlock(addr, m8MB, inMarkStoppedAudioBuffersFree && !isReading && !isWriting ?
string() : tag.str());
752 bool isEnabled(
false), isMultiFormat(
false), isQuad(
false), isQuadQuad(
false), isSquares(
false), isTSI(
false);
754 uint64_t addr(0), len(0);
755 if (skipChannels.find(chan) != skipChannels.end())
762 tag <<
"AC" <<
DEC(chan+1) << (acStatus.
IsInput() ?
" Write" :
" Read");
774 inDevice.
GetDeviceFrameInfo (
UWord(frameNum), chan, mIntrinsicSize, isMultiFormat, isQuad, isQuadQuad, isSquares, isTSI, addr, len);
776 tag <<
"MR" <<
DEC(chan+1);
778 tag <<
"Ch" <<
DEC(chan+1);
786 else if (isQuad && !isQuadQuad && isTSI)
809 if (inStartAddr % m8MB)
811 if (inByteLength % m8MB)
815 const UWord startFrm(
UWord(inStartAddr / m8MB)), frmCnt(
UWord(inByteLength / m8MB));
816 for (
UWord frm(0); frm < frmCnt; frm++)
818 UWord frameNum(startFrm + frm);
820 if (tags.find(inTag) == tags.end())
823 if (frameNum >= mNumFrames)
824 tags.insert(
"Invalid");
virtual ULWord DeviceGetAudioFrameBuffer(void)
virtual bool IsAudioOutputRunning(const NTV2AudioSystem inAudioSystem, bool &outIsRunning)
Answers whether or not the playout side of the given NTV2AudioSystem is currently running.
virtual bool IS_INPUT_SPIGOT_INVALID(const UWord inInputSpigot) const
bool TagMemoryBlock(const ULWord inStartAddr, const ULWord inByteLength, const std::string &inTag)
virtual bool SetFrameBufferSize(const NTV2Framesize inSize)
Sets the device's intrinsic frame buffer size.
virtual bool DeviceCanDoAudioMixer(void)
bool NTV2DeviceCanDoConversionMode(const NTV2DeviceID inDeviceID, const NTV2ConversionMode inConversionMode)
virtual ULWord GetSerialNumberHigh(void)
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
@ DEVICE_ID_KONAHDMI
See KONA HDMI.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
Declares device capability functions.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
bool TagAudioBuffers(CNTV2Card &inDevice, const bool inMarkStoppedAudioBuffersFree)
std::set< std::string > NTV2StringSet
std::string & strip(std::string &str, const std::string &ws)
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
bool _boardOpened
True if I'm open and connected to the device.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
virtual bool IS_CHANNEL_INVALID(const NTV2Channel inChannel) const
NTV2AudioChannelOctets::const_iterator NTV2AudioChannelOctetsConstIter
Handy const iterator to iterate over a set of distinct NTV2AudioChannelOctet values.
std::string join(const std::vector< std::string > &parts, const std::string &delim)
@ DEVICE_ID_KONA5
See KONA 5.
virtual bool DeviceCanDoHDMIQuadRasterConversion(void)
virtual bool IsBreakoutBoardConnected(void)
virtual Word GetDeviceVersion(void)
Answers with this device's version number.
@ DEVICE_ID_IOX3
See IoX3.
ULWord NTV2DeviceGetActiveMemorySize(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanReportFailSafeLoaded(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_IOIP_2022
See Io IP.
bool GetTagsForFrameIndex(const UWord inIndex, NTV2StringSet &outTags) const
Answers with the list of tags for the given frame number.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
virtual bool DeviceIsDNxIV(void)
@ NTV2_BreakoutCableBNC
Identifies the AES/EBU audio breakout cable that has BNC connectors.
virtual bool GetFrameBufferSize(const NTV2Channel inChannel, NTV2Framesize &outValue)
Answers with the frame size currently being used on the device.
@ DEVICE_ID_KONA5_8KMK
See KONA 5.
virtual bool DeviceCanDo3GOut(const UWord index0)
ULWord _ulNumFrameBuffers
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
#define DEC0N(__x__, __n__)
virtual bool GetInputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current input frame index number for the given FrameStore. This identifies which par...
virtual bool DeviceCanDoConversionMode(const NTV2ConversionMode inCM)
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
virtual bool GetInstalledBitfileInfo(ULWord &outNumBytes, std::string &outDateStr, std::string &outTimeStr)
Returns the size and time/date stamp of the device's currently-installed firmware.
virtual bool DeviceCanDoFrameBufferFormat(const NTV2PixelFormat inPF)
ULWord _ulFrameBufferSize
static std::string SerialNum64ToString(const uint64_t inSerialNumber)
Returns a string containing the decoded, human-readable device serial number.
@ kDeviceCanDoAudioMixer
True if device has a firmware audio mixer.
bool NTV2DeviceCanDoFrameBufferFormat(const NTV2DeviceID inDeviceID, const NTV2FrameBufferFormat inFBFormat)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool DeviceCanDoFormat(const NTV2FrameRate inFR, const NTV2FrameGeometry inFG, const NTV2Standard inStd)
@ NTV2_BreakoutNone
No identifiable breakout hardware appears to be attached.
virtual bool DeviceCanDoWidget(const NTV2WidgetID inWgtID)
virtual bool GetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat &outValue)
Returns the current frame buffer format for the given FrameStore on the AJA device.
NTV2DeviceID _boardID
My cached device ID.
NTV2FrameRate
Identifies a particular video frame rate.
ULWord NTV2FramesizeToByteCount(const NTV2Framesize inFrameSize)
Converts the given NTV2Framesize value into an exact byte count.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
std::string NTV2AudioChannelQuadToString(const NTV2Audio4ChannelSelect inValue, const bool inCompactDisplay=false)
@ kDeviceGetNumBufferedAudioSystems
The total number of audio systems on the device that can read/write audio buffer memory....
bool NTV2DeviceCanDoVideoFormat(const NTV2DeviceID inDeviceID, const NTV2VideoFormat inVideoFormat)
virtual std::string GetDeviceVersionString(void)
Answers with this device's version number as a human-readable string.
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
ULWordSet::const_iterator ULWordSetConstIter
virtual bool IsSupported(const NTV2BoolParamID inParamID)
#define NTV2DriverVersionDecode_Point(__vers__)
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
virtual std::string GetModelName(void)
Answers with this device's model name.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
static bool isEnabled(CNTV2Card &device, const NTV2Channel inChannel)
virtual std::string GetBitfileInfoString(const BITFILE_INFO_STRUCT &inBitFileInfo)
Generates and returns an info string with date, time and name for the given inBifFileInfo.
virtual bool IsAudioInputRunning(const NTV2AudioSystem inAudioSystem, bool &outIsRunning)
Answers whether or not the capture side of the given NTV2AudioSystem is currently running.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
std::ostream & DumpBlocks(std::ostream &oss) const
Dumps all 8MB blocks/frames and their tags, if any, into the given stream.
virtual bool DeviceHasMicInput(void)
std::set< UByte > NTV2DIDSet
A set of distinct NTV2DID values.
virtual bool GetOutputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current output frame number for the given FrameStore (expressed as an NTV2Channel).
NTV2Standard
Identifies a particular video standard.
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
virtual std::string GetPCIFPGAVersionString(void)
@ DEVICE_ID_KONA5_8K
See KONA 5.
std::string NTV2AudioChannelPairToString(const NTV2AudioChannelPair inValue, const bool inCompactDisplay=false)
@ DEVICE_ID_KONA3G
See KONA 3G (UFC Mode).
#define NTV2DriverVersionDecode_Major(__vers__)
static NTV2Buffer NULL_POINTER
Used for default empty NTV2Buffer parameters – do not modify.
bool NTV2DeviceCanDoWidget(const NTV2DeviceID inDeviceID, const NTV2WidgetID inWidgetID)
NTV2DoubleArray::const_iterator NTV2DoubleArrayConstIter
Handy const iterator to iterate over an NTV2DoubleArray.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
virtual bool DeviceCanDoInputSource(const NTV2InputSource inSrc)
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
virtual ULWord DeviceGetFrameBufferSize(void)
@ DEVICE_ID_KONAX
See KONA X.
NTV2AudioChannelQuads::const_iterator NTV2AudioChannelQuadsConstIter
Handy const iterator to iterate over a set of distinct NTV2AudioChannelQuad values.
@ DEVICE_ID_KONA4UFC
See KONA 4 (UFC Mode).
std::set< NTV2AudioChannelPair > NTV2AudioChannelPairs
A set of distinct NTV2AudioChannelPair values.
virtual ULWord DeviceGetNumberFrameBuffers(void)
NTV2Mode
Used to identify the mode of a Frame Store, or the direction of an AutoCirculate stream: either Captu...
std::ostream & RawDump(std::ostream &oss) const
Dumps a human-readable list of regions into the given stream.
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
bool NTV2DeviceCanDo3GOut(NTV2DeviceID boardID, UWord index0)
Declares the CNTV2Card class.
@ NTV2_BITFILE_IO4KPLUS_MAIN
virtual bool GetDeviceFrameInfo(const UWord inFrameNumber, const NTV2Channel inChannel, uint64_t &outAddress, uint64_t &outLength)
Answers with the address and size of the given frame.
virtual bool IsFailSafeBitfileLoaded(bool &outIsFailSafe)
Answers whether or not the "fail-safe" (aka "safe-boot") bitfile is currently loaded and running in t...
size_t GetTagCount(const UWord inIndex) const
virtual bool GetSerialNumberString(std::string &outSerialNumberString)
Answers with a string that contains my human-readable serial number.
UWord NTV2DeviceGetNumVideoInputs(const NTV2DeviceID inDeviceID)
ULWord NTV2DeviceGetAudioFrameBuffer2(NTV2DeviceID boardID, NTV2FrameGeometry frameGeometry, NTV2FrameBufferFormat frameFormat)
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
virtual uint64_t GetSerialNumber(void)
Answers with my serial number.
Declares numerous NTV2 utility functions.
NTV2Framesize
Kona2/Xena2 specific enums.
NTV2BreakoutType
Identifies the Breakout Boxes and Cables that may be attached to an AJA NTV2 device.
virtual bool DeviceCanDoLTCEmbeddedN(const UWord index0)
I interrogate and control an AJA video/audio capture/playout device.
@ DEVICE_ID_KONA5_8K_MV_TX
See KONA 5.
virtual bool CanWarmBootFPGA(bool &outCanWarmBoot)
Answers whether or not the FPGA can be reloaded without powering off.
bool IsStopped(void) const
@ DEVICE_ID_KONALHEPLUS
See KONA LHe Plus.
@ DEVICE_ID_KONA5_OE1
See KONA 5.
std::string NTV2AudioChannelOctetToString(const NTV2Audio8ChannelSelect inValue, const bool inCompactDisplay=false)
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
uint16_t GetEndFrame(void) const
virtual ULWord GetNumSupported(const NTV2NumericParamID inParamID)
virtual bool GetMode(const NTV2Channel inChannel, NTV2Mode &outValue)
Answers with the current NTV2Mode of the given FrameStore on the AJA device.
@ NTV2_BreakoutCableXLR
Identifies the AES/EBU audio breakout cable that has XLR connectors.
@ kDeviceGetNumMicInputs
The number of microphone inputs on the device.
#define NTV2_IS_VALID_CHANNEL(__x__)
virtual std::string GetDriverVersionString(void)
Answers with this device's driver's version as a human-readable string.
virtual NTV2BreakoutType GetBreakoutHardware(void)
@ kDeviceGetNumHDMIVideoInputs
The number of HDMI video inputs on the device.
NTV2InputSource
Identifies a specific video input source.
@ DEVICE_ID_IOIP_2110
See Io IP.
@ DEVICE_ID_KONA5_3DLUT
See KONA 5.
virtual bool DeviceCanDoVideoFormat(const NTV2VideoFormat inVF)
virtual bool GetFrameGeometry(NTV2FrameGeometry &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
std::set< NTV2AudioChannelOctet > NTV2AudioChannelOctets
A set of distinct NTV2AudioChannelOctet values.
bool GetRegions(ULWordSequence &outFree, ULWordSequence &outUsed, ULWordSequence &outBad) const
Answers with the lists of free, in-use and conflicting 8MB memory blocks. Each ULWord represents a re...
bool TranslateRegions(ULWordSequence &outRgns, const ULWordSequence &inRgns, const bool inIsQuad, const bool inIsQuadQuad) const
Translates an 8MB-chunked list of regions into another list of regions with frame indexes and sizes e...
virtual bool IS_OUTPUT_SPIGOT_INVALID(const UWord inOutputSpigot) const
@ kDeviceGetNumHDMIVideoOutputs
The number of HDMI video outputs on the device.
Private include file for all ajabase sources.
virtual ~CNTV2Card()
My destructor.
#define NTV2DriverVersionDecode_Minor(__vers__)
@ DEVICE_ID_IO4KPLUS
See Io4K Plus.
bool NTV2DeviceCanDoInputSource(const NTV2DeviceID inDeviceID, const NTV2InputSource inInputSource)
bool NTV2DeviceCanDoDSKMode(const NTV2DeviceID inDeviceID, const NTV2DSKMode inDSKMode)
virtual bool DeviceCanDoDSKMode(const NTV2DSKMode inDSKM)
bool NTV2DeviceCanDoFormat(const NTV2DeviceID inDevID, const NTV2FrameRate inFR, const NTV2FrameGeometry inFG, const NTV2Standard inStd)
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
@ DEVICE_ID_KONA4
See KONA 4 (Quad Mode).
uint16_t GetStartFrame(void) const
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ kDeviceHasMicrophoneInput
True if device has a microphone input connector.
bool TagVideoFrames(CNTV2Card &inDevice)
ULWord NTV2DeviceGetNumVideoChannels(const NTV2DeviceID inDeviceID)
NTV2FrameGeometry
Identifies a particular video frame geometry.
virtual bool IsChannelEnabled(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the given FrameStore is enabled.
virtual ULWord GetFrameBufferSize(void) const
CNTV2Card()
My default constructor.
static ULWordSet CoalesceRegions(const ULWordSequence &inRgn1, const ULWordSequence &inRgn2, const ULWordSequence &inRgn3)
virtual NTV2DeviceID GetDeviceID(void)
virtual bool GetDriverVersionComponents(UWord &outMajor, UWord &outMinor, UWord &outPoint, UWord &outBuild)
Answers with the individual version components of this device's driver.
virtual bool IsMultiRasterWidgetChannel(const NTV2Channel inChannel)
virtual bool IsBufferSizeSetBySW(void)
bool NTV2DeviceCanDoWarmBootFPGA(const NTV2DeviceID inDeviceID)
virtual std::string GetFPGAVersionString(const NTV2XilinxFPGA inFPGA=eFPGAVideoProc)
std::set< NTV2AudioChannelQuad > NTV2AudioChannelQuads
A set of distinct NTV2AudioChannelQuad values.
std::vector< double > NTV2DoubleArray
An array of double-precision floating-point values.
@ NTV2_MODE_INVALID
The invalid mode.
@ DEVICE_ID_KONA3GQUAD
See KONA 3G (Quad Mode).
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
#define NTV2DriverVersionDecode_Build(__vers__)
std::string NTV2BitfileTypeToString(const NTV2BitfileType inValue, const bool inCompactDisplay=false)
#define NTV2_IS_INPUT_MODE(__mode__)
virtual bool GetAudioMemoryOffset(const ULWord inOffsetBytes, ULWord &outAbsByteOffset, const NTV2AudioSystem inAudioSystem, const bool inCaptureBuffer=false)
Answers with the byte offset in device SDRAM into the specified Audio System's audio buffer.
virtual ULWord GetSerialNumberLow(void)
@ kVRegDriverVersion
Packed driver version – use NTV2DriverVersionEncode, NTV2DriverVersionDecode* macros to encode/decode...
#define xHEX0N(__x__, __n__)
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
bool NTV2DeviceCanDoLTCEmbeddedN(NTV2DeviceID boardID, UWord index0)
@ DEVICE_ID_IOIP_2110_RGB12
See Io IP.
ULWord NTV2DeviceGetFrameBufferSize(NTV2DeviceID boardID, NTV2FrameGeometry frameGeometry, NTV2FrameBufferFormat frameFormat)
@ DEVICE_ID_KONA5_2X4K
See KONA 5.
virtual bool HasMultiRasterWidget(void)
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
virtual bool Open(const UWord inDeviceIndex)
Opens a local/physical AJA device so it can be monitored/controlled.
@ DEVICE_ID_IO4K
See Io4K (Quad Mode).
ostream & operator<<(ostream &inOutStr, const NTV2AudioChannelPairs &inSet)
bool AssessDevice(CNTV2Card &inDevice, const bool inIgnoreStoppedAudioBuffers=false)
Assesses the given device.
NTV2DIDSet::const_iterator NTV2DIDSetConstIter
Handy const iterator to iterate over an NTV2DIDSet.
virtual bool DriverGetBitFileInformation(BITFILE_INFO_STRUCT &outBitFileInfo, const NTV2BitFileType inBitFileType=NTV2_VideoProcBitFile)
Answers with the currently-installed bitfile information.
@ DEVICE_ID_KONALHI
See KONA LHi.
virtual ULWord DeviceGetAudioFrameBuffer2(void)
NTV2AudioChannelPairs::const_iterator NTV2AudioChannelPairsConstIter
Handy const iterator to iterate over a set of distinct NTV2AudioChannelPair values.
ULWord NTV2DeviceGetNumberFrameBuffers(NTV2DeviceID boardID, NTV2FrameGeometry frameGeometry, NTV2FrameBufferFormat frameFormat)
std::string SerialNum64ToString(const uint64_t &inSerNum)
virtual Word GetPCIFPGAVersion(void)
virtual bool Close(void)
Closes me, releasing host resources that may have been allocated in a previous Open call.
UWord NTV2DeviceGetSPIFlashVersion(const NTV2DeviceID inDeviceID)
ULWord NTV2DeviceGetAudioFrameBuffer(NTV2DeviceID boardID, NTV2FrameGeometry frameGeometry, NTV2FrameBufferFormat frameFormat)