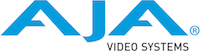 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
28 return outputs > 0 ?
true :
false;
37 return inputs > 0 ?
true :
false;
48 default:
return false;
64 default:
return false;
76 default:
return false;
86 default:
return false;
102 default:
return false;
114 default:
return false;
130 default:
return false;
234 #if (defined(__CPLUSPLUS__) || defined(__cplusplus)) && !defined(NTV2_BUILDING_DRIVER)
264 switch (inFrameGeometry)
333 switch (inFrameGeometry)
412 #if (defined(__CPLUSPLUS__) || defined(__cplusplus)) && !defined(NTV2_BUILDING_DRIVER)
424 ULWord hwBytesPerFrame = 0;
425 ULWord videoMemorySize = 0;
432 hwBytesPerFrame = gFrameSizeToByteCount [inFrameSize] * 1024 * 1024;
437 videoMemorySize = activeMemorySize - numAudioBytes;
441 return videoMemorySize/hwBytesPerFrame;
444 #if (defined(__CPLUSPLUS__) || defined(__cplusplus)) && !defined(NTV2_BUILDING_DRIVER)
528 switch (inFrameGeometry)
597 switch (inFrameGeometry)
635 #if (defined(__CPLUSPLUS__) || defined(__cplusplus)) && !defined(NTV2_BUILDING_DRIVER)
643 #if (defined(__CPLUSPLUS__) || defined(__cplusplus)) && !defined(NTV2_BUILDING_DRIVER)
649 #if (defined(__CPLUSPLUS__) || defined(__cplusplus)) && !defined(NTV2_BUILDING_DRIVER)
653 #else // #if defined(__CPLUSPLUS__) || defined(__cplusplus)
657 #endif // #if defined(__CPLUSPLUS__) || defined(__cplusplus)
661 #if (defined(__CPLUSPLUS__) || defined(__cplusplus)) && !defined(NTV2_BUILDING_DRIVER)
667 #if (defined(__CPLUSPLUS__) || defined(__cplusplus)) && !defined(NTV2_BUILDING_DRIVER)
670 #else // #if defined(__CPLUSPLUS__) || defined(__cplusplus)
673 #endif // #if defined(__CPLUSPLUS__) || defined(__cplusplus)
693 const ULWord inIsSMPTE372Enabled)
702 const ULWord inIsSMPTE372Enabled,
703 const bool inIsProgressivePicture)
712 const ULWord inIsSMPTE372Enabled,
713 const bool inIsProgressivePicture,
714 const bool inIsSquareDivision)
729 default:
return false;
745 default:
return false;
755 if (inIsSMPTE372Enabled)
767 if (inIsSMPTE372Enabled)
779 if (inIsSMPTE372Enabled)
796 if (inIsSMPTE372Enabled)
811 if (inIsSMPTE372Enabled)
838 default:
return false;
998 default:
return false;
1006 #define MAX_OF(__a__,__b__) ((__a__) > (__b__) ? (__a__) : (__b__))
1145 #if !defined(NTV2_DEPRECATE_17_0)
1165 #endif // !defined(NTV2_DEPRECATE_17_0)
UWord NTV2DeviceGetNumLTCInputs(const NTV2DeviceID inDeviceID)
bool NTV2DeviceHasSPIv5(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_3840x2160p_6000
@ NTV2_FORMAT_4096x2160psf_2500
@ DEVICE_ID_KONALHIDVI
See KONA LHi.
@ NTV2_FORMAT_4x1920x1080p_6000
@ NTV2_FG_4x2048x1080
4096x2160, for 4K, NTV2_VANCMODE_OFF
UWord NTV2DeviceGetNumAnalogAudioOutputChannels(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_3840x2160psf_2500
@ NTV2_FORMAT_1080psf_2398
@ NTV2_TCINDEX_SDI4
SDI 4 embedded VITC.
@ NTV2_FORMAT_4096x2160p_2400
@ NTV2_FBF_ARGB
See 8-Bit ARGB, RGBA, ABGR Formats.
@ DEVICE_ID_KONAIP_2110
See KONA IP.
@ NTV2_FORMAT_4096x2160p_12000
@ DEVICE_ID_KONAHDMI
See KONA HDMI.
@ NTV2_STANDARD_2Kx1080p
Identifies SMPTE HD 2K1080p.
#define XENA2_FRAMEBUFFER_SIZE
@ NTV2_FORMAT_4x4096x2160p_4800
NTV2OutputDestination
Identifies a specific video output destination.
@ DEVICE_ID_CORVID44_2X4K
See Corvid 44 12G.
@ NTV2_FORMAT_4x4096x2160p_2398
@ NTV2_FBF_12BIT_RGB_PACKED
See 12-Bit Packed RGB.
@ DEVICE_ID_KONA5_OE9
See KONA 5.
@ NTV2_FG_1920x1114
1920x1080, NTV2_VANCMODE_TALLER
Declares device capability functions.
UWord NTV2DeviceGetGenlockVersion(const NTV2DeviceID inDeviceID)
@ NTV2_FG_720x576
720x576, for PAL 625i, NTV2_VANCMODE_OFF
@ NTV2_FG_720x508
720x486, for NTSC 525i, NTV2_VANCMODE_TALL
bool NTV2DeviceCanDoOutputDestination(const NTV2DeviceID inDeviceID, const NTV2OutputDestination inOutputDest)
@ NTV2_FORMAT_4096x2160p_11988
@ NTV2_FORMAT_4096x2160p_2500
@ NTV2_FRAMERATE_1500
15 frames per second
@ NTV2_TCINDEX_SDI2_2
SDI 2 embedded VITC 2.
@ NTV2_FRAMERATE_6000
60 frames per second
@ NTV2_FORMAT_525psf_2997
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
@ NTV2_FG_4x4096x2160
8192x4320, for 8K, NTV2_VANCMODE_OFF
@ NTV2_FORMAT_4x2048x1080p_4795
@ NTV2_FBF_10BIT_DPX_LE
10-Bit DPX Little-Endian
@ NTV2_FORMAT_4096x2160psf_2398
@ DEVICE_ID_KONA5
See KONA 5.
bool NTV2DeviceCanDo3GOut(NTV2DeviceID boardID, UWord index0)
@ NTV2_TCINDEX_SDI7_LTC
SDI 7 embedded ATC LTC.
#define NTV2_ASSERT(_expr_)
@ NTV2_STANDARD_1080
Identifies SMPTE HD 1080i or 1080psf.
@ NTV2_STANDARD_3840x2160p
Identifies Ultra-High-Definition (UHD)
@ NTV2_FRAMERATE_2997
Fractional rate of 30,000 frames per 1,001 seconds.
@ NTV2_TCINDEX_SDI8_2
SDI 8 embedded VITC 2.
@ NTV2_FORMAT_1080p_2K_6000_B
@ NTV2_FBF_RGBA
See 8-Bit ARGB, RGBA, ABGR Formats.
@ NTV2_STANDARD_625
Identifies SMPTE SD 625i.
@ NTV2_FORMAT_1080psf_2K_2398
@ DEVICE_ID_IOX3
See IoX3.
@ NTV2_FG_2048x1080
2048x1080, for 2Kx1080p, NTV2_VANCMODE_OFF
@ NTV2_STANDARD_4096HFR
Identifies high frame-rate 4K.
@ NTV2_STANDARD_4096x2160p
Identifies 4K.
@ NTV2_FORMAT_1080p_2K_4800_A
@ NTV2_FORMAT_4x2048x1080p_11988
ULWord NTV2DeviceGetActiveMemorySize(const NTV2DeviceID inDeviceID)
ULWord NTV2DeviceGetFrameBufferSize(NTV2DeviceID boardID, NTV2FrameGeometry inFrameGeometry, NTV2FrameBufferFormat frameFormat)
@ NTV2_FRAMERATE_12000
120 frames per second
@ NTV2_FBF_48BIT_RGB
See 48-Bit RGB.
@ NTV2_OUTPUTDESTINATION_SDI2
ULWord NTV2DeviceGetAudioFrameBuffer_Ex(NTV2DeviceID boardID)
@ DEVICE_ID_CORVID44_8KMK
See Corvid 44 12G.
@ NTV2_OUTPUTDESTINATION_SDI3
@ DEVICE_ID_KONAIP_2110_RGB12
See KONA IP.
@ DEVICE_ID_CORVID22
See Corvid 22.
@ DEVICE_ID_IOIP_2022
See Io IP.
@ NTV2_FORMAT_4x1920x1080psf_3000
@ DEVICE_ID_CORVIDHEVC
See Corvid HEVC.
@ NTV2_FORMAT_1080p_2K_3000
@ NTV2_FORMAT_4096x2160psf_2997
@ NTV2_FORMAT_4x2048x1080p_2997
@ NTV2_FBF_10BIT_ARGB
10-Bit ARGB
#define NTV2_IS_QUAD_QUAD_FRAME_GEOMETRY(geom)
@ NTV2_FBF_10BIT_YCBCRA
10-Bit YCbCrA
@ DEVICE_ID_KONA5_8KMK
See KONA 5.
@ NTV2_FORMAT_4x4096x2160p_2500
bool NTV2DeviceCanDoAudioOut(const NTV2DeviceID inDeviceID)
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
@ NTV2_TCINDEX_SDI4_2
SDI 4 embedded VITC 2.
@ NTV2_FORMAT_4x4096x2160p_4795
@ NTV2_FORMAT_4x1920x1080p_2997
@ NTV2_FORMAT_4x1920x1080p_2500
@ NTV2_FORMAT_4x2048x1080p_4800
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ NTV2_FORMAT_4x3840x2160p_2500
@ NTV2_TCINDEX_SDI6
SDI 6 embedded VITC.
@ NTV2_FG_720x486
720x486, for NTSC 525i and 525p60, NTV2_VANCMODE_OFF
#define NTV2_IS_ATC_VITC2_TIMECODE_INDEX(__x__)
UWord NTV2DeviceGetNumCSCs(const NTV2DeviceID inDeviceID)
bool NTV2DeviceHasSPIv2(const NTV2DeviceID inDeviceID)
@ NTV2_FBF_10BIT_DPX
See 10-Bit RGB - DPX Format.
@ DEVICE_ID_KONAIP_1RX_1TX_1SFP_J2K
See KONA IP.
@ NTV2_FORMAT_4x3840x2160p_2398
ULWord NTV2DeviceGetNumberFrameBuffers(NTV2DeviceID boardID, NTV2FrameGeometry inFrameGeometry, NTV2FrameBufferFormat frameFormat)
@ NTV2_FRAMERATE_2500
25 frames per second
@ DEVICE_ID_IO4KUFC
See Io4K (UFC Mode).
bool work_around_erroneous_compiler_warning(void)
@ NTV2_FORMAT_1080p_2K_4795_A
@ NTV2_FORMAT_3840x2160p_2500
NTV2FrameRate
Identifies a particular video frame rate.
@ DEVICE_ID_CORVID1
See Corvid, Corvid 3G.
bool NTV2DeviceCanDo292Out(NTV2DeviceID boardID, UWord index0)
@ NTV2_FORMAT_4x4096x2160p_2997
bool NTV2DeviceCanDoTCIndex(const NTV2DeviceID inDeviceID, const NTV2TCIndex inTCIndex)
@ NTV2_FRAMERATE_4800
48 frames per second
bool NTV2DeviceCanDoLTCInN(const NTV2DeviceID devID, UWord index0)
@ NTV2_FORMAT_4096x2160psf_2400
UWord NTV2DeviceGetNumSerialPorts(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_CORVID44_8K
See Corvid 44 12G.
@ DEVICE_ID_KONAIP_2TX_1SFP_J2K
See KONA IP.
@ NTV2_TCINDEX_SDI5_2
SDI 5 embedded VITC 2.
@ NTV2_FRAMERATE_2400
24 frames per second
@ NTV2_FORMAT_4x2048x1080psf_2398
@ NTV2_FBF_10BIT_RGB_PACKED
10-Bit Packed RGB
@ DEVICE_ID_KONAIP_4CH_2SFP
See KONA IP.
@ NTV2_FORMAT_4x2048x1080p_2398
#define MAX_OF(__a__, __b__)
bool NTV2DeviceCanDoInputTCIndex(const NTV2DeviceID inDeviceID, const NTV2TCIndex inTCIndex)
@ NTV2_TCINDEX_SDI2_LTC
SDI 2 embedded ATC LTC.
@ NTV2_FORMAT_1080psf_2K_2500
@ NTV2_OUTPUTDESTINATION_SDI1
@ NTV2_FORMAT_4x2048x1080psf_2400
@ NTV2_TCINDEX_SDI1_LTC
SDI 1 embedded ATC LTC.
@ NTV2_FORMAT_1080p_5994_B
@ NTV2_FORMAT_1080psf_3000_2
@ NTV2_TCINDEX_SDI1
SDI 1 embedded VITC.
@ NTV2_FORMAT_1080p_2K_2400
@ NTV2_TCINDEX_SDI2
SDI 2 embedded VITC.
@ NTV2_FG_2048x1588
2048x1556, for 2Kx1556psf film format, NTV2_VANCMODE_TALL
@ NTV2_FORMAT_4x2048x1080p_3000
@ NTV2_TCINDEX_SDI5
SDI 5 embedded VITC.
NTV2Standard
Identifies a particular video standard.
@ NTV2_FORMAT_4096x2160p_4795
@ NTV2_FRAMERATE_2398
Fractional rate of 24,000 frames per 1,001 seconds.
@ DEVICE_ID_KONA5_8K
See KONA 5.
@ DEVICE_ID_KONA3G
See KONA 3G (UFC Mode).
@ NTV2_FORMAT_4x4096x2160p_3000
bool NTV2DeviceCanDoAudioMixer(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoWidget(const NTV2DeviceID inDeviceID, const NTV2WidgetID inWidgetID)
@ DEVICE_ID_CORVID3G
See Corvid, Corvid 3G.
@ DEVICE_ID_KONAX
See KONA X.
@ NTV2_TCINDEX_SDI7_2
SDI 7 embedded VITC 2.
@ NTV2_FORMAT_4x2048x1080psf_3000
@ DEVICE_ID_KONA5_OE5
See KONA 5.
@ NTV2_FORMAT_1080p_2K_4795_B
bool NTV2DeviceCanDoAudioN(const NTV2DeviceID devID, UWord index0)
@ DEVICE_ID_KONA4UFC
See KONA 4 (UFC Mode).
@ NTV2_FG_720x612
720x576, for PAL 625i, NTV2_VANCMODE_TALLER
@ NTV2_FRAMERATE_1498
Fractional rate of 15,000 frames per 1,001 seconds.
UWord Get8MBFrameSizeFactor(const NTV2FrameGeometry inFG, const NTV2FrameBufferFormat inFBF)
@ NTV2_FORMAT_4x3840x2160p_5994
@ DEVICE_ID_KONAIP_1RX_1TX_2110
See KONA IP.
NTV2AudioSystem NTV2DeviceGetHostAudioSystem(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoAudio6Channels(const NTV2DeviceID devID)
@ NTV2_FORMAT_4096x2160p_2398
NTV2AudioSystem NTV2DeviceGetAudioMixerSystem(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_3840x2160psf_3000
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
bool NTV2DeviceCanDo12GOut(NTV2DeviceID boardID, UWord index0)
bool NTV2DeviceCanDoColorCorrection(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4x2048x1080p_5000
@ NTV2_OUTPUTDESTINATION_SDI6
UWord NTV2DeviceGetNumVideoInputs(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumHDMIVideoOutputs(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4096x2160p_6000
@ NTV2_FG_720x514
720x486, for NTSC 525i and 525p60, NTV2_VANCMODE_TALLER
UWord NTV2DeviceGetNumLUTs(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_TTAP_PRO
See T-Tap Pro.
bool NTV2DeviceHasColorSpaceConverterOnChannel2(const NTV2DeviceID devID)
@ NTV2_STANDARD_1080p
Identifies SMPTE HD 1080p.
bool NTV2DeviceGetVideoFormatFromState(NTV2VideoFormat *pOutValue, const NTV2FrameRate inFrameRate, const NTV2FrameGeometry inFrameGeometry, const NTV2Standard inStandard, const ULWord inIsSMPTE372Enabled)
@ NTV2_FORMAT_4x1920x1080p_3000
@ NTV2_FORMAT_4x3840x2160p_2997
@ NTV2_TCINDEX_SDI7
SDI 7 embedded VITC.
@ NTV2_OUTPUTDESTINATION_SDI8
@ NTV2_FORMAT_1080p_5000_B
NTV2Framesize
Kona2/Xena2 specific enums.
@ NTV2_TCINDEX_SDI5_LTC
SDI 5 embedded ATC LTC.
@ NTV2_FORMAT_4x3840x2160p_5000
@ DEVICE_ID_KONAIP_2022
See KONA IP.
@ NTV2_FORMAT_1080p_2K_5994_A
ULWord NTV2DeviceGetNumberFrameBuffers_Ex(NTV2DeviceID boardID)
@ DEVICE_ID_IOXT
See IoXT.
UWord NTV2DeviceGetNumHDMIAudioInputChannels(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4x2048x1080p_12000
@ DEVICE_ID_KONA5_8K_MV_TX
See KONA 5.
UWord NTV2DeviceGetNumAnalogVideoOutputs(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_KONA5_OE8
See KONA 5.
@ NTV2_FORMAT_625psf_2500
@ DEVICE_ID_KONALHEPLUS
See KONA LHe Plus.
UWord NTV2DeviceGetNumLTCOutputs(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_KONA5_OE1
See KONA 5.
@ DEVICE_ID_CORVID88
See Corvid 88.
@ NTV2_FG_1280x720
1280x720, for 720p, NTV2_VANCMODE_OFF
@ NTV2_FORMAT_1080p_2K_2500
@ NTV2_FORMAT_4x1920x1080psf_2997
@ NTV2_FORMAT_1080p_6000_A
@ NTV2_FORMAT_3840x2160psf_2398
@ NTV2_TCINDEX_SDI4_LTC
SDI 4 embedded ATC LTC.
@ NTV2_TCINDEX_SDI3_2
SDI 3 embedded VITC 2.
@ NTV2_FRAMERATE_11988
Fractional rate of 120,000 frames per 1,001 seconds.
@ NTV2_FORMAT_1080p_2K_2398
ULWord NTV2DeviceGetAudioFrameBuffer(NTV2DeviceID boardID, NTV2FrameGeometry inFrameGeometry, NTV2FrameBufferFormat frameFormat)
@ NTV2_FORMAT_3840x2160p_2997
@ NTV2_FORMAT_4096x2160p_4800
@ NTV2_MAX_NUM_Framesizes
@ NTV2_STANDARD_4096i
Identifies 4K psf.
@ NTV2_FORMAT_3840x2160psf_2400
@ NTV2_FORMAT_1080psf_2K_2400
bool NTV2DeviceHasSPIv4(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_3840x2160p_5994
@ NTV2_FORMAT_4x4096x2160p_2400
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4x4096x2160p_6000
@ NTV2_FORMAT_3840x2160p_2398
@ NTV2_FG_1920x1112
1920x1080, for 1080i and 1080p, NTV2_VANCMODE_TALL
@ DEVICE_ID_IOIP_2110
See Io IP.
@ DEVICE_ID_KONA5_3DLUT
See KONA 5.
bool NTV2DeviceCanDoAudio2Channels(const NTV2DeviceID devID)
bool NTV2DeviceCanDoAudio8Channels(const NTV2DeviceID devID)
@ NTV2_FORMAT_4x1920x1080psf_2398
bool NTV2DeviceCanDo12gRouting(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_1080p_2K_6000_A
@ NTV2_FG_720x598
720x576, for PAL 625i, NTV2_VANCMODE_TALL
@ NTV2_TCINDEX_SDI1_2
SDI 1 embedded VITC 2.
@ NTV2_TCINDEX_SDI3
SDI 3 embedded VITC.
#define NTV2_IS_QUAD_FRAME_GEOMETRY(geom)
@ NTV2_FORMAT_4096x2160p_3000
ULWord NTV2DeviceGetAudioFrameBuffer2(NTV2DeviceID boardID, NTV2FrameGeometry inFrameGeometry, NTV2FrameBufferFormat frameFormat)
bool NTV2DeviceCanDo292In(NTV2DeviceID boardID, UWord index0)
@ NTV2_FORMAT_4x1920x1080psf_2500
@ NTV2_FRAMERATE_5994
Fractional rate of 60,000 frames per 1,001 seconds.
@ NTV2_OUTPUTDESTINATION_HDMI
bool NTV2DeviceHasSPIv3(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoRS422N(const NTV2DeviceID devID, const NTV2Channel ch)
@ DEVICE_ID_CORVID24
See Corvid 24.
@ NTV2_FORMAT_4x3840x2160p_2400
@ NTV2_STANDARD_525
Identifies SMPTE SD 525i.
@ DEVICE_ID_KONA1
See KONA 1.
@ NTV2_FORMAT_4x2048x1080p_2500
@ NTV2_FORMAT_4x1920x1080p_5994
@ NTV2_FORMAT_4x4096x2160p_5000
@ DEVICE_ID_IO4KPLUS
See Io4K Plus.
@ NTV2_FORMAT_4x3840x2160p_6000
bool NTV2DeviceGetVideoFormatFromState_Ex2(NTV2VideoFormat *pOutValue, const NTV2FrameRate inFrameRate, const NTV2FrameGeometry inFrameGeometry, const NTV2Standard inStandard, const ULWord inIsSMPTE372Enabled, const bool inIsProgressivePicture, const bool inIsSquareDivision)
@ NTV2_STANDARD_3840HFR
Identifies high frame-rate UHD.
@ DEVICE_ID_CORVID44
See Corvid 44.
@ NTV2_TCINDEX_LTC2
Analog LTC 2.
@ NTV2_STANDARD_2Kx1080i
Identifies SMPTE HD 2K1080psf.
bool NTV2DeviceCanDo3GIn(NTV2DeviceID boardID, UWord index0)
@ NTV2_FORMAT_1080p_5000_A
@ DEVICE_ID_KONA4
See KONA 4 (Quad Mode).
@ NTV2_FORMAT_4x2048x1080p_6000
@ NTV2_FORMAT_3840x2160psf_2997
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ NTV2_STANDARD_8192
Identifies 8K.
Contains implementations of NTV2DeviceCanDo... and NTV2DeviceGetNum... functions. This module is incl...
@ NTV2_FORMAT_4096x2160p_5000
@ NTV2_TCINDEX_DEFAULT
The "default" timecode (mostly used by the AJA "Retail" service and Control Panel)
@ NTV2_TCINDEX_SDI6_2
SDI 6 embedded VITC 2.
@ NTV2_FORMAT_4x1920x1080p_5000
bool NTV2DeviceCanDoStackedAudio(const NTV2DeviceID inDeviceID)
@ NTV2_STANDARD_720
Identifies SMPTE HD 720p.
@ NTV2_FORMAT_4x1920x1080p_2398
@ NTV2_FG_2048x1112
2048x1080, for 2Kx1080p, NTV2_VANCMODE_TALL
@ DEVICE_ID_TTAP
See T-Tap.
NTV2FrameGeometry
Identifies a particular video frame geometry.
@ NTV2_FORMAT_1080p_6000_B
@ DEVICE_ID_KONA5_OE3
See KONA 5.
@ NTV2_FORMAT_4096x2160p_2997
@ NTV2_TCINDEX_SDI3_LTC
SDI 3 embedded ATC LTC.
ULWord NTV2DeviceGetNumberVideoFrameBuffers(NTV2DeviceID inDeviceID, NTV2FrameGeometry inFrameGeometry, NTV2Framesize inFrameSize)
@ NTV2_OUTPUTDESTINATION_SDI7
bool NTV2DeviceGetVideoFormatFromState_Ex(NTV2VideoFormat *pOutValue, const NTV2FrameRate inFrameRate, const NTV2FrameGeometry inFrameGeometry, const NTV2Standard inStandard, const ULWord inIsSMPTE372Enabled, const bool inIsProgressivePicture)
@ DEVICE_ID_KONA5_OE7
See KONA 5.
UWord NTV2DeviceGetNumHDMIAudioOutputChannels(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_1080p_5994_A
@ NTV2_FG_2048x1114
2048x1080, NTV2_VANCMODE_TALLER
@ DEVICE_ID_KONA5_OE10
See KONA 5.
@ NTV2_FORMAT_4x2048x1080p_5994
@ NTV2_OUTPUTDESTINATION_SDI4
bool NTV2DeviceHasGenlockv3(const NTV2DeviceID devID)
@ NTV2_FG_4x1920x1080
3840x2160, for UHD, NTV2_VANCMODE_OFF
@ NTV2_FORMAT_4x3840x2160p_3000
@ NTV2_FORMAT_1080p_2K_5994_B
@ NTV2_FBF_16BIT_ARGB
16-Bit ARGB
ULWord NTV2DeviceGetAudioFrameBuffer2_Ex(NTV2DeviceID boardID)
UWord NTV2DeviceGetNumAudioStreams(const NTV2DeviceID devID)
bool NTV2DeviceCanDoLTCEmbeddedN(NTV2DeviceID boardID, UWord index0)
@ DEVICE_ID_KONA3GQUAD
See KONA 3G (Quad Mode).
@ DEVICE_ID_CORVIDHBR
See Corvid HB-R.
@ NTV2_TCINDEX_SDI8
SDI 8 embedded VITC.
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_3840x2160p_5000
@ NTV2_FORMAT_4096x2160psf_3000
bool NTV2DeviceHasGenlockv2(const NTV2DeviceID devID)
@ NTV2_FG_1280x740
1280x720, for 720p, NTV2_VANCMODE_TALL
@ NTV2_FORMAT_1080p_2K_5000_A
@ NTV2_TCINDEX_SDI8_LTC
SDI 8 embedded ATC LTC.
@ NTV2_FRAMERATE_5000
50 frames per second
@ NTV2_TCINDEX_SDI6_LTC
SDI 6 embedded ATC LTC.
UWord NTV2DeviceGetNumAnalogAudioInputChannels(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4x2048x1080psf_2500
@ NTV2_FBF_10BIT_RGB
See 10-Bit RGB Format.
@ DEVICE_ID_KONA5_OE11
See KONA 5.
@ NTV2_FG_2048x1556
2048x1556, for 2Kx1556psf film format, NTV2_VANCMODE_OFF
@ NTV2_FRAMERATE_4795
Fractional rate of 48,000 frames per 1,001 seconds.
@ NTV2_FRAMERATE_3000
30 frames per second
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
@ NTV2_FORMAT_1080p_2K_5000_B
@ DEVICE_ID_IOIP_2110_RGB12
See Io IP.
@ NTV2_FG_4x3840x2160
7680x4320, for UHD2, NTV2_VANCMODE_OFF
@ DEVICE_ID_KONA5_2X4K
See KONA 5.
@ NTV2_FORMAT_1080psf_2997_2
bool NTV2DeviceCanDoProgrammableCSC(const NTV2DeviceID inDeviceID)
@ NTV2_TCINDEX_LTC1
Analog LTC 1.
@ NTV2_FORMAT_4x4096x2160p_5994
@ NTV2_FORMAT_4x2048x1080p_2400
bool NTV2DeviceCanDoAudioIn(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_1080p_2K_4800_B
@ DEVICE_ID_KONA5_OE2
See KONA 5.
@ NTV2_STANDARD_7680
Identifies UHD2.
@ NTV2_FORMAT_4x1920x1080p_2400
@ NTV2_FORMAT_4x1920x1080psf_2400
@ NTV2_FG_1920x1080
1920x1080, for 1080i and 1080p, NTV2_VANCMODE_OFF
bool NTV2DeviceCanDo12GIn(NTV2DeviceID boardID, UWord index0)
@ NTV2_OUTPUTDESTINATION_ANALOG
@ NTV2_FORMAT_1080psf_2500_2
@ DEVICE_ID_IO4K
See Io4K (Quad Mode).
@ DEVICE_ID_KONALHI
See KONA LHi.
@ NTV2_FORMAT_3840x2160p_2400
@ DEVICE_ID_KONA5_OE4
See KONA 5.
@ NTV2_FBF_ABGR
See 8-Bit ARGB, RGBA, ABGR Formats.
bool NTV2DeviceCanDoLTCOutN(const NTV2DeviceID devID, UWord index0)
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
@ NTV2_OUTPUTDESTINATION_SDI5
@ NTV2_STANDARD_3840i
Identifies Ultra-High-Definition (UHD) psf.
@ NTV2_FORMAT_3840x2160p_3000
@ NTV2_FORMAT_1080psf_2400
ULWord NTV2DeviceGetFrameBufferSize_Ex(NTV2DeviceID boardID)
bool NTV2DeviceROMHasBankSelect(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4096x2160p_5994
@ DEVICE_ID_KONA5_OE6
See KONA 5.
@ DEVICE_ID_KONA5_OE12
See KONA 5.
UWord NTV2DeviceGetSPIFlashVersion(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoVITC2(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_CORVID44_PLNR
See Corvid 44 12G.
@ NTV2_FORMAT_4x2048x1080psf_2997
@ NTV2_AUDIOSYSTEM_INVALID
@ NTV2_FORMAT_1080p_2K_2997
@ DEVICE_ID_IOEXPRESS
See Io Express.