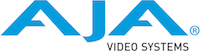 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
8 #ifndef NTV2DRIVERINTERFACE_H
9 #define NTV2DRIVERINTERFACE_H
18 #if defined(NTV2_WRITEREG_PROFILING) // Register Write Profiling
20 #endif // NTV2_WRITEREG_PROFILING Register Write Profiling
24 #ifdef AJA_USE_CPLUSPLUS11
25 #ifndef NTV2_USE_CPLUSPLUS11
26 #error "AJA_USE_CPLUSPLUS11 && !NTV2_USE_CPLUSPLUS11"
31 #ifdef NTV2_USE_CPLUSPLUS11
32 #error "!AJA_USE_CPLUSPLUS11 && NTV2_USE_CPLUSPLUS11"
38 #if defined(AJALinux ) || defined(AJAMac)
42 #elif defined(MSWindows)
48 #define AsNTV2DriverInterfaceRef(_x_) reinterpret_cast<CNTV2DriverInterface&>(_x_)
49 #define AsNTV2DriverInterfacePtr(_x_) reinterpret_cast<CNTV2DriverInterface*>(_x_)
69 static inline UWord MaxNumDevices (
void) {
return 32;}
76 static void SetShareMode (
const bool inSharedMode);
77 static bool GetShareMode (
void);
84 static void SetOverlappedMode (
const bool inOverlapMode);
85 static bool GetOverlappedMode (
void);
119 AJA_VIRTUAL inline bool IsOpen (
void)
const {
return _boardOpened;}
128 AJA_VIRTUAL bool IsDeviceReady (
const bool inCheckValid =
false);
152 AJA_VIRTUAL bool Open (
const std::string & inURLSpec);
225 bool result (ReadRegister(inRegNum, regValue, inMask, inShift));
227 outValue = T(regValue);
231 #if !defined(READREGMULTICHANGE)
239 #endif // !defined(READREGMULTICHANGE)
240 AJA_VIRTUAL bool RestoreHardwareProcampRegisters() = 0;
266 const ULWord inFrameNumber,
268 const ULWord inCardOffsetBytes,
269 const ULWord inTotalByteCount,
270 const bool inSynchronous =
true);
301 const ULWord inFrameNumber,
303 const ULWord inCardOffsetBytes,
304 const ULWord inTotalByteCount,
305 const ULWord inNumSegments,
306 const ULWord inHostPitchPerSeg,
307 const ULWord inCardPitchPerSeg,
308 const bool inSynchronous =
true) = 0;
313 const ULWord inFrameNumber,
314 const ULWord inCardOffsetBytes,
316 const ULWord inNumSegments,
317 const ULWord inSegmentHostPitch,
318 const ULWord inSegmentCardPitch,
412 AJA_VIRTUAL bool BitstreamWrite (
const NTV2Buffer & inBuffer,
const bool inFragment,
const bool inSwap);
413 AJA_VIRTUAL bool BitstreamReset (
const bool inConfiguration,
const bool inInterface);
415 AJA_VIRTUAL bool BitstreamLoad (
const bool inSuspend,
const bool inResume);
427 GetBoolParam (
ULWord(inParamID), value);
436 GetNumericParam (
ULWord(inParamID), value);
480 AJA_VIRTUAL bool AcquireStreamForApplicationWithReference (
const ULWord inAppType,
const int32_t inProcessID);
498 AJA_VIRTUAL bool ReleaseStreamForApplicationWithReference (
const ULWord inAppType,
const int32_t inProcessID);
518 AJA_VIRTUAL bool AcquireStreamForApplication (
const ULWord inAppType,
const int32_t inProcessID);
536 AJA_VIRTUAL bool ReleaseStreamForApplication (
const ULWord inAppType,
const int32_t inProcessID);
551 AJA_VIRTUAL bool SetStreamingApplication (
const ULWord inAppType,
const int32_t inProcessID);
565 AJA_VIRTUAL bool GetStreamingApplication (
ULWord & outAppType, int32_t & outProcessID);
569 AJA_VIRTUAL inline std::string GetHostName (
void)
const {
return IsRemote() ? _pRPCAPI->Name() :
"";}
570 AJA_VIRTUAL inline bool IsRemote (
void)
const {
return _pRPCAPI ?
true :
false;}
575 #if defined(NTV2_NUB_CLIENT_SUPPORT) && !defined(NTV2_DEPRECATE_16_0)
580 #if !defined(NTV2_DEPRECATE_16_0)
600 #endif // !defined(NTV2_DEPRECATE_16_0)
601 #if !defined(NTV2_DEPRECATE_16_3)
604 #endif // !defined(NTV2_DEPRECATE_16_3)
606 #if defined(NTV2_WRITEREG_PROFILING) // Register Write Profiling
612 AJA_VIRTUAL bool StartRecordRegisterWrites (
const bool inSkipActualWrites =
false);
613 AJA_VIRTUAL bool IsRecordingRegisterWrites (
void)
const;
616 AJA_VIRTUAL bool ResumeRecordRegisterWrites (
void);
618 #endif // NTV2_WRITEREG_PROFILING // Register Write Profiling
629 AJA_VIRTUAL bool OpenRemote (
const std::string & inURLSpec);
676 #if defined(NTV2_WRITEREG_PROFILING)
679 #endif // NTV2_WRITEREG_PROFILING
684 #if defined(NTV2_WRITEREG_PROFILING)
687 #endif // NTV2_WRITEREG_PROFILING
688 #if !defined(NTV2_DEPRECATE_16_0)
695 #endif // !defined(NTV2_DEPRECATE_16_0)
702 #endif // NTV2DRIVERINTERFACE_H
bool mSkipRegWrites
True if actual register writes are skipped while recording.
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
NTV2_DriverDebugMessageSet
Declares device capability functions.
ULWord * _pXena2FlashBaseAddress
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
bool _boardOpened
True if I'm open and connected to the device.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
ULWord _ulNumFrameBuffers
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
ULWord * _pCh2FrameBaseAddress
ULWord _ulFrameBufferSize
std::string packageNumber
UWord _boardNumber
My device index number.
NTV2DeviceID _boardID
My cached device ID.
virtual bool SuspendAudio(void)
_EventCounts mEventCounts
My event tallies, one for each interrupt type. Note that these.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
virtual bool IsSupported(const NTV2BoolParamID inParamID)
virtual bool HevcSendMessage(HevcMessageHeader *pMessage)
Sends an HEVC message to the NTV2 driver.
NTV2RegisterWrites mRegWrites
Stores WriteRegister data.
enum _NTV2NumericParamID NTV2NumericParamID
Used with CNTV2DriverInterface::GetNumericParam to determine device capabilities.
std::set< ULWord > ULWordSet
A collection of unique ULWord (uint32_t) values.
Declares the AJALock class.
ULWord NTV2NubProtocolVersion
bool ReadRegister(const ULWord inRegNum, T &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
This template function reads all or part of the 32-bit contents of a specific register (real or virtu...
enum _NTV2EnumsID NTV2EnumsID
Identifies NTV2 enumerated types, used in CNTV2DriverInterface::GetSupportedItems.
All new NTV2 structs start with this common header.
Enumerations for controlling NTV2 devices.
NTV2RegWrites NTV2RegisterReads
Declares numerous NTV2 utility functions.
std::vector< ULWord > NTV2ULWordVector
An ordered sequence of ULWords.
std::vector< std::string > NTV2StringList
virtual ULWord GetNumSupported(const NTV2NumericParamID inParamID)
enum _NTV2BoolParamID NTV2BoolParamID
Used with CNTV2DriverInterface::GetBoolParam to determine device capabilities.
std::vector< PULWord > _EventHandles
virtual std::string GetDescription(void) const
#define NTV2_SHOULD_BE_DEPRECATED(__f__)
#define NTV2_DEPRECATED_f(__f__)
virtual ULWord GetNumFrameBuffers(void) const
bool NTV2DeviceCanDoIP(const NTV2DeviceID inDeviceID)
std::vector< NTV2RegInfo > NTV2RegisterWrites
ULWord _pRegisterBaseAddressLength
ULWord * _pCh1FrameBaseAddress
virtual ULWord GetFrameBufferSize(void) const
_EventHandles mInterruptEventHandles
For subscribing to each possible event, one for each interrupt type.
bool mRecordRegWrites
True if recording; otherwise false when not recording.
struct PACKAGE_INFO_STRUCT * PPACKAGE_INFO_STRUCT
Declares data types and structures used in NTV2 "nub" packets.
std::vector< ULWord > _EventCounts
Declares enums and structs used by all platform drivers and the SDK.
ULWord * _pRegisterBaseAddress
AJALock mRegWritesLock
Guard mutex for mRegWrites.
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
Base class of objects that can connect to, and operate remote or fake devices. I have three general A...
ULWord * _pFrameBaseAddress
Declares NTV2 "nub" client functions.
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
virtual bool ResumeAudio(const ULWord inFBSize)
NTV2RPCAPI * _pRPCAPI
Points to remote or software device interface; otherwise NULL for local physical device.