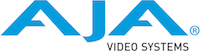 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
36 #define NTV2UTILS_ENUM_CASE_RETURN_STR(enum_name) case(enum_name): return #enum_name
38 #define NTV2UTILS_ENUM_CASE_RETURN_VAL_OR_ENUM_STR(condition, retail_name, enum_name)\
39 case(enum_name): return condition ? retail_name : #enum_name
47 uint32_t rowBytes = 0;
49 switch (inPixelFormat)
53 rowBytes = inPixelWidth * 2;
58 rowBytes = (( inPixelWidth % 48 == 0 ) ? inPixelWidth : (((inPixelWidth / 48 ) + 1) * 48)) * 8 / 3;
68 rowBytes = inPixelWidth * 4;
73 rowBytes = inPixelWidth * 3;
77 rowBytes = inPixelWidth * 2/4;
81 rowBytes = inPixelWidth * 6;
85 rowBytes = inPixelWidth * 36 / 8;
90 rowBytes = inPixelWidth * 20 / 16;
95 rowBytes = inPixelWidth;
121 out16BitYUVLine.clear ();
122 const ULWord * pInputLine (
reinterpret_cast <const ULWord *
> (pIn10BitYUVLine));
129 inNumPixels -= inNumPixels % 6;
131 const ULWord totalULWords (inNumPixels * 4 / 6);
133 for (
ULWord inputCount (0); inputCount < totalULWords; inputCount++)
135 out16BitYUVLine.push_back ((pInputLine [inputCount] ) & 0x3FF);
136 out16BitYUVLine.push_back ((pInputLine [inputCount] >> 10) & 0x3FF);
137 out16BitYUVLine.push_back ((pInputLine [inputCount] >> 20) & 0x3FF);
145 out16BitYUVLine.clear ();
146 const ULWord * pInputLine (
reinterpret_cast <const ULWord *
> (pIn10BitYUVLine));
152 if (inFormatDesc.GetRasterWidth () < 6)
157 for (
ULWord inputCount (0); inputCount < inFormatDesc.
linePitch; inputCount++)
159 out16BitYUVLine.push_back ((pInputLine [inputCount] ) & 0x3FF);
160 out16BitYUVLine.push_back ((pInputLine [inputCount] >> 10) & 0x3FF);
161 out16BitYUVLine.push_back ((pInputLine [inputCount] >> 20) & 0x3FF);
171 for ( uint32_t sampleCount = 0, dataCount = 0;
172 sampleCount < (numPixels*2) ;
173 sampleCount+=3,dataCount++ )
175 ycbcrBuffer[sampleCount] = packedBuffer[dataCount]&0x3FF;
176 ycbcrBuffer[sampleCount+1] = (packedBuffer[dataCount]>>10)&0x3FF;
177 ycbcrBuffer[sampleCount+2] = (packedBuffer[dataCount]>>20)&0x3FF;
186 for ( uint32_t inputCount=0, outputCount=0;
187 inputCount < (numPixels*2);
188 outputCount += 4, inputCount += 12 )
190 packedBuffer[outputCount] = uint32_t (ycbcrBuffer[inputCount+0]) + uint32_t (ycbcrBuffer[inputCount+1]<<10) + uint32_t (ycbcrBuffer[inputCount+2]<<20);
191 packedBuffer[outputCount+1] = uint32_t (ycbcrBuffer[inputCount+3]) + uint32_t (ycbcrBuffer[inputCount+4]<<10) + uint32_t (ycbcrBuffer[inputCount+5]<<20);
192 packedBuffer[outputCount+2] = uint32_t (ycbcrBuffer[inputCount+6]) + uint32_t (ycbcrBuffer[inputCount+7]<<10) + uint32_t (ycbcrBuffer[inputCount+8]<<20);
193 packedBuffer[outputCount+3] = uint32_t (ycbcrBuffer[inputCount+9]) + uint32_t (ycbcrBuffer[inputCount+10]<<10) + uint32_t (ycbcrBuffer[inputCount+11]<<20);
200 for ( uint32_t count = 0; count < numPixels*2; count+=4 )
204 buffer[count+2] = Cr;
214 for (uint32_t pixel(0); pixel < numPixels * 2; pixel++)
215 ycbcr8BitBuffer[pixel] = uint8_t(ycbcr10BitBuffer[pixel] >> 2);
224 bool bUseSmpteRange,
bool bAlphaFromLuma)
335 switch ( signalMask )
338 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
347 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
355 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
364 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
372 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
382 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
390 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
413 void StackQuadrants(uint8_t* pSrc, uint32_t srcWidth, uint32_t srcHeight, uint32_t srcRowBytes,
419 uint32_t copyRowBytes = srcRowBytes/2;
420 uint32_t copyHeight = srcHeight/2;
421 uint32_t dstRowBytes = copyRowBytes;
422 uint32_t dstHeight = srcHeight/2;
426 uint32_t srcLHSQuadrantRowBytes = srcRowBytes/2;
428 for (uint32_t quadrant=0; quadrant<4; quadrant++)
434 case 0: srcSample = 0;
break;
435 case 1: srcSample = srcLHSQuadrantRowBytes;
break;
436 case 2: srcSample = (srcRowBytes*copyHeight);
break;
437 case 3: srcSample = (srcRowBytes*copyHeight) + srcLHSQuadrantRowBytes;
break;
441 dstSample = quadrant * dstRowBytes * dstHeight;
443 for (uint32_t row=0; row<copyHeight; row++)
445 memcpy(&pDst[dstSample], &pSrc[srcSample], copyRowBytes);
446 dstSample += dstRowBytes;
447 srcSample += srcRowBytes;
454 void CopyFromQuadrant(uint8_t* srcBuffer, uint32_t srcHeight, uint32_t srcRowBytes, uint32_t srcQuadrant, uint8_t* dstBuffer, uint32_t quad13Offset)
458 ULWord dstHeight = srcHeight / 2;
459 ULWord dstRowBytes = srcRowBytes / 2;
465 case 0: srcSample = 0;
break;
466 case 1: srcSample = dstRowBytes - quad13Offset;
break;
467 case 2: srcSample = srcRowBytes*dstHeight;
break;
468 case 3: srcSample = srcRowBytes*dstHeight + dstRowBytes - quad13Offset;
break;
472 for (
ULWord i=0; i<dstHeight; i++)
474 memcpy(&dstBuffer[dstSample], &srcBuffer[srcSample], dstRowBytes);
475 dstSample += dstRowBytes;
476 srcSample += srcRowBytes;
482 void CopyToQuadrant(uint8_t* srcBuffer, uint32_t srcHeight, uint32_t srcRowBytes, uint32_t dstQuadrant, uint8_t* dstBuffer, uint32_t quad13Offset)
486 ULWord dstRowBytes = srcRowBytes * 2;
492 case 0: dstSample = 0;
break;
493 case 1: dstSample = srcRowBytes - quad13Offset;
break;
494 case 2: dstSample = dstRowBytes*srcHeight;
break;
495 case 3: dstSample = dstRowBytes*srcHeight + srcRowBytes - quad13Offset;
break;
499 for (
ULWord i=0; i<srcHeight; i++)
501 memcpy(&dstBuffer[dstSample], &srcBuffer[srcSample], srcRowBytes);
502 dstSample += dstRowBytes;
503 srcSample += srcRowBytes;
513 NTV2_ASSERT (pIn10BitYUVLine && pOut16BitYUVLine &&
"UnpackLine_10BitYUVto16BitYUV -- NULL buffer pointer(s)");
514 NTV2_ASSERT (inNumPixels &&
"UnpackLine_10BitYUVto16BitYUV -- Zero pixel count");
516 for (
ULWord outputCount = 0, inputCount = 0;
517 outputCount < (inNumPixels * 2);
518 outputCount += 3, inputCount++)
520 pOut16BitYUVLine [outputCount ] = pIn10BitYUVLine [inputCount] & 0x3FF;
521 pOut16BitYUVLine [outputCount + 1] = (pIn10BitYUVLine [inputCount] >> 10) & 0x3FF;
522 pOut16BitYUVLine [outputCount + 2] = (pIn10BitYUVLine [inputCount] >> 20) & 0x3FF;
529 NTV2_ASSERT (pIn16BitYUVLine && pOut10BitYUVLine &&
"PackLine_16BitYUVto10BitYUV -- NULL buffer pointer(s)");
530 NTV2_ASSERT (inNumPixels &&
"PackLine_16BitYUVto10BitYUV -- Zero pixel count");
532 for (
ULWord inputCount = 0, outputCount = 0;
533 inputCount < (inNumPixels * 2);
534 outputCount += 4, inputCount += 12)
536 pOut10BitYUVLine [outputCount ] =
ULWord (pIn16BitYUVLine [inputCount + 0]) + (
ULWord (pIn16BitYUVLine [inputCount + 1]) << 10) + (
ULWord (pIn16BitYUVLine [inputCount + 2]) << 20);
537 pOut10BitYUVLine [outputCount + 1] =
ULWord (pIn16BitYUVLine [inputCount + 3]) + (
ULWord (pIn16BitYUVLine [inputCount + 4]) << 10) + (
ULWord (pIn16BitYUVLine [inputCount + 5]) << 20);
538 pOut10BitYUVLine [outputCount + 2] =
ULWord (pIn16BitYUVLine [inputCount + 6]) + (
ULWord (pIn16BitYUVLine [inputCount + 7]) << 10) + (
ULWord (pIn16BitYUVLine [inputCount + 8]) << 20);
539 pOut10BitYUVLine [outputCount + 3] =
ULWord (pIn16BitYUVLine [inputCount + 9]) + (
ULWord (pIn16BitYUVLine [inputCount +10]) << 10) + (
ULWord (pIn16BitYUVLine [inputCount +11]) << 20);
546 if (!pOut10BitYUVLine)
550 if (
ULWord(in16BitYUVLine.size()) < inNumPixels*2)
553 for (
ULWord inputCount = 0, outputCount = 0;
554 inputCount < (inNumPixels * 2);
555 outputCount += 4, inputCount += 12)
557 pOut10BitYUVLine[outputCount ] =
ULWord(in16BitYUVLine[inputCount + 0]) + (
ULWord(in16BitYUVLine[inputCount + 1]) << 10) + (
ULWord(in16BitYUVLine[inputCount + 2]) << 20);
558 pOut10BitYUVLine[outputCount + 1] =
ULWord(in16BitYUVLine[inputCount + 3]) + (
ULWord(in16BitYUVLine[inputCount + 4]) << 10) + (
ULWord(in16BitYUVLine[inputCount + 5]) << 20);
559 pOut10BitYUVLine[outputCount + 2] =
ULWord(in16BitYUVLine[inputCount + 6]) + (
ULWord(in16BitYUVLine[inputCount + 7]) << 10) + (
ULWord(in16BitYUVLine[inputCount + 8]) << 20);
560 pOut10BitYUVLine[outputCount + 3] =
ULWord(in16BitYUVLine[inputCount + 9]) + (
ULWord(in16BitYUVLine[inputCount +10]) << 10) + (
ULWord(in16BitYUVLine[inputCount +11]) << 20);
569 if (inYCbCrLine.size() < 12)
571 if (inFrameBuffer.
IsNULL())
580 const uint32_t pixPerLineX2 (inDescriptor.GetRasterWidth() * 2);
581 uint32_t * pOutPackedLine (
AJA_NULL);
589 for (uint32_t inputCount = 0, outputCount = 0; inputCount < pixPerLineX2; outputCount += 4, inputCount += 12)
591 if ((inputCount+11) >= uint32_t(inYCbCrLine.size()))
593 #if defined(_DEBUG) // 'at' throws upon bad index values
594 pOutPackedLine[outputCount] = uint32_t(inYCbCrLine.at(inputCount+0)) | uint32_t(inYCbCrLine.at(inputCount+ 1)<<10) | uint32_t(inYCbCrLine.at(inputCount+ 2)<<20);
595 pOutPackedLine[outputCount+1] = uint32_t(inYCbCrLine.at(inputCount+3)) | uint32_t(inYCbCrLine.at(inputCount+ 4)<<10) | uint32_t(inYCbCrLine.at(inputCount+ 5)<<20);
596 pOutPackedLine[outputCount+2] = uint32_t(inYCbCrLine.at(inputCount+6)) | uint32_t(inYCbCrLine.at(inputCount+ 7)<<10) | uint32_t(inYCbCrLine.at(inputCount+ 8)<<20);
597 pOutPackedLine[outputCount+3] = uint32_t(inYCbCrLine.at(inputCount+9)) | uint32_t(inYCbCrLine.at(inputCount+10)<<10) | uint32_t(inYCbCrLine.at(inputCount+11)<<20);
598 #else // 'operator[]' doesn't throw
599 pOutPackedLine[outputCount] = uint32_t(inYCbCrLine[inputCount+0]) | uint32_t(inYCbCrLine[inputCount+ 1]<<10) | uint32_t(inYCbCrLine[inputCount+ 2]<<20);
600 pOutPackedLine[outputCount+1] = uint32_t(inYCbCrLine[inputCount+3]) | uint32_t(inYCbCrLine[inputCount+ 4]<<10) | uint32_t(inYCbCrLine[inputCount+ 5]<<20);
601 pOutPackedLine[outputCount+2] = uint32_t(inYCbCrLine[inputCount+6]) | uint32_t(inYCbCrLine[inputCount+ 7]<<10) | uint32_t(inYCbCrLine[inputCount+ 8]<<20);
602 pOutPackedLine[outputCount+3] = uint32_t(inYCbCrLine[inputCount+9]) | uint32_t(inYCbCrLine[inputCount+10]<<10) | uint32_t(inYCbCrLine[inputCount+11]<<20);
612 outYCbCrLine.clear();
613 if (inFrameBuffer.
IsNULL())
621 if (inDescriptor.GetRasterWidth () < 6)
626 for (
ULWord inputCount(0); inputCount < inDescriptor.
linePitch; inputCount++)
628 outYCbCrLine.push_back((pInputLine[inputCount] ) & 0x3FF);
629 outYCbCrLine.push_back((pInputLine[inputCount] >> 10) & 0x3FF);
630 outYCbCrLine.push_back((pInputLine[inputCount] >> 20) & 0x3FF);
639 for (
UWord count = 0; count < numULWords; count++)
641 ULWord value = (packedycbcrLine[count])<<2;
642 value = (value<<24) + ((value>>24)&0x000000FF) + ((value<<8)&0x00FF0000) + ((value>>8)&0x0000FF00);
644 packedycbcrLine[count] = value;
650 for (
ULWord pixel=0;pixel<numPixels;pixel++)
652 ULWord value = DPXLinebuffer[pixel];
655 rgba10BitBuffer[pixel].
Red =
UWord((value&0xC0)>>14) +
UWord((value&0xFF)<<2);
656 rgba10BitBuffer[pixel].
Green =
UWord((value&0x3F00)>>4) +
UWord((value&0xF00000)>>20);
657 rgba10BitBuffer[pixel].
Blue =
UWord((value&0xFC000000)>>26) +
UWord((value&0xF0000)>>12);
661 rgba10BitBuffer[pixel].
Red = (value>>22)&0x3FF;
662 rgba10BitBuffer[pixel].
Green = (value>>12)&0x3FF;
663 rgba10BitBuffer[pixel].
Blue = (value>>2)&0x3FF;
672 for (
ULWord pixel=0;pixel<numPixels;pixel++)
674 ULWord value = DPXLinebuffer[pixel];
675 rawrp215Buffer[pixel] = ((value&0x3F00)>>4) + ((value&0xF00000)>>20);
682 for (
ULWord pixel=0;pixel<numPixels;pixel++)
684 ULWord value = DPXLinebuffer[pixel];
685 rawrp215Buffer[pixel] = ((value&0x3F)>>4) + ((value&0xF00000)>>20);
696 switch ( signalMask )
699 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
708 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
716 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
725 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
733 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
743 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
751 for ( pixelCount = 0; pixelCount < (numPixels*2); pixelCount += 4 )
771 if (pOutLineData && inNumPixels)
772 for (
ULWord count(0); count < inNumPixels; count++)
784 if (pOutLineData && inNumPixels)
785 for (
ULWord count(0); count < inNumPixels; count++)
795 if (pOutLineData && inNumPixels)
796 for (
ULWord count(0); count < inNumPixels*2; count+=4)
798 pOutLineData[count] = Cb;
799 pOutLineData[count+1] = Y;
800 pOutLineData[count+2] = Cr;
801 pOutLineData[count+3] = Y;
811 if (!pBaseVideoAddress)
815 UWord lineBuffer[2048*2];
816 ULWord * pBaseAddress (
reinterpret_cast<ULWord*
>(pBaseVideoAddress));
833 for ( uint32_t count = 0; count < numPixels*2; count+=2 )
842 for ( uint32_t count = 0; count < numPixels*2; count+=2 )
856 for ( uint32_t count = 0; count < numPixels*2; count+=2 )
865 for ( uint32_t count = 0; count < numPixels*2; count+=2 )
879 for (
ULWord count = 0; count < numPixels*2; count += 4)
881 lineData[count] = Cb;
882 lineData[count+1] = Y;
883 lineData[count+2] = Cr;
884 lineData[count+3] = Y;
889 for (
ULWord count = 0; count < numPixels*2; count += 4)
892 lineData[count+1] = Cb;
893 lineData[count+2] = Y;
894 lineData[count+3] = Cr;
903 if (!pBaseVideoAddress)
907 UByte * pBaseAddress (
reinterpret_cast<UByte*
>(pBaseVideoAddress));
953 ULWord topPad = 0, bottomPad = 0, leftPad = 0, rightPad = 0;
956 ULWord* pSrc = pSrcBuffer;
957 ULWord* pDst = pDstBuffer;
959 if (dstHeight > srcHeight)
961 topPad = (dstHeight - srcHeight) / 2;
962 bottomPad = dstHeight - topPad - srcHeight;
965 pSrc += ((srcHeight - dstHeight) / 2) * srcWidth;
967 if (dstWidth > srcWidth)
969 leftPad = (dstWidth - srcWidth) / 2;
970 rightPad = dstWidth - srcWidth - leftPad;
973 pSrc += (srcWidth - dstWidth) / 2;
976 contentHeight = dstHeight - topPad - bottomPad;
977 contentWidth = dstWidth - leftPad - rightPad;
980 memset(pDst, 0, topPad * dstWidth * 4);
981 pDst += topPad * dstWidth;
984 while (contentHeight--)
987 memset(pDst, 0, leftPad * 4);
991 memcpy(pDst, pSrc, contentWidth * 4);
992 pDst += contentWidth;
996 memset(pDst, 0, rightPad * 4);
1001 memset(pDst, 0, bottomPad * dstWidth * 4);
1006 const ULWord inDstBytesPerLine,
1007 const UWord inDstTotalLines)
1009 const ULWord dstMaxPixelWidth (inDstBytesPerLine / 2);
1010 UByte * pLine (pDstBuffer);
1012 for (
UWord lineNum(0); lineNum < inDstTotalLines; lineNum++)
1015 pLine += inDstBytesPerLine;
1022 const ULWord inDstBytesPerLine,
1023 const UWord inDstTotalLines)
1029 NTV2Buffer dstBuffer (pDstBuffer,
ULWord(inDstTotalLines) * inDstBytesPerLine);
1030 const ULWord dstMaxPixelWidth (inDstBytesPerLine / 16 * 6);
1033 for (
UWord lineNum(1); lineNum < inDstTotalLines; lineNum++)
1034 if (!dstBuffer.
CopyFrom (dstBuffer,
1036 ULWord(lineNum) * inDstBytesPerLine,
1044 const ULWord inDstBytesPerLine,
1045 const UWord inDstTotalLines)
1047 const ULWord dstMaxPixelWidth (inDstBytesPerLine / 2);
1048 UByte * pLine (pDstBuffer);
1050 for (
UWord lineNum(0); lineNum < inDstTotalLines; lineNum++)
1053 pLine += inDstBytesPerLine;
1060 const ULWord inDstBytesPerLine,
1061 const UWord inDstTotalLines)
1067 NTV2Buffer dstBuffer (pDstBuffer,
ULWord(inDstTotalLines) * inDstBytesPerLine);
1068 const ULWord dstMaxPixelWidth (inDstBytesPerLine / 16 * 6);
1071 for (
UWord lineNum(1); lineNum < inDstTotalLines; lineNum++)
1072 if (!dstBuffer.
CopyFrom (dstBuffer,
1074 ULWord(lineNum) * inDstBytesPerLine,
1083 const ULWord inDstBytesPerLine,
1084 const UWord inDstTotalLines)
1088 if (inDstBytesPerLine == 0)
1090 if (inDstTotalLines == 0)
1093 switch (inPixelFormat)
1143 const ULWord inDstBytesPerLine,
1144 const UWord inDstTotalLines)
1148 if (inDstBytesPerLine == 0)
1150 if (inDstTotalLines == 0)
1153 switch (inPixelFormat)
1203 const UByte * pResult (pInFrameBuffer);
1206 pResult += inBytesPerVertLine *
ULWord(inVertLineOffset);
1207 pResult +=
ULWord(inBytesPerHorzPixel) *
ULWord(inHorzPixelOffset);
1214 UByte * pResult (pInFrameBuffer);
1217 pResult += inBytesPerVertLine *
ULWord(inVertLineOffset);
1218 pResult +=
ULWord(inBytesPerHorzPixel) *
ULWord(inHorzPixelOffset);
1225 const ULWord inDstBytesPerLine,
1226 const UWord inDstTotalLines,
1227 const UWord inDstVertLineOffset,
1228 const UWord inDstHorzPixelOffset,
1229 const UByte * pSrcBuffer,
1230 const ULWord inSrcBytesPerLine,
1231 const UWord inSrcTotalLines,
1232 const UWord inSrcVertLineOffset,
1233 const UWord inSrcVertLinesToCopy,
1234 const UWord inSrcHorzPixelOffset,
1235 const UWord inSrcHorzPixelsToCopy)
1237 if (inDstHorzPixelOffset & 1)
1239 if (inSrcHorzPixelOffset & 1)
1242 const ULWord TWO_BYTES_PER_PIXEL (2);
1243 const ULWord dstMaxPixelWidth (inDstBytesPerLine / TWO_BYTES_PER_PIXEL);
1244 const ULWord srcMaxPixelWidth (inSrcBytesPerLine / TWO_BYTES_PER_PIXEL);
1245 UWord numHorzPixelsToCopy (inSrcHorzPixelsToCopy);
1246 UWord numVertLinesToCopy (inSrcVertLinesToCopy);
1248 if (inDstHorzPixelOffset >= dstMaxPixelWidth)
1250 if (inSrcHorzPixelOffset >= srcMaxPixelWidth)
1252 if (
ULWord(inSrcHorzPixelOffset + inSrcHorzPixelsToCopy) > srcMaxPixelWidth)
1253 numHorzPixelsToCopy -= inSrcHorzPixelOffset + inSrcHorzPixelsToCopy - srcMaxPixelWidth;
1254 if (inSrcVertLineOffset + inSrcVertLinesToCopy > inSrcTotalLines)
1255 numVertLinesToCopy -= inSrcVertLineOffset + inSrcVertLinesToCopy - inSrcTotalLines;
1256 if (numVertLinesToCopy + inDstVertLineOffset >= inDstTotalLines)
1258 if (numVertLinesToCopy + inDstVertLineOffset > inDstTotalLines)
1259 numVertLinesToCopy -= numVertLinesToCopy + inDstVertLineOffset - inDstTotalLines;
1264 const UByte * pSrc (::
GetReadAddress_2vuy (pSrcBuffer, inSrcBytesPerLine, inSrcVertLineOffset, inSrcHorzPixelOffset, TWO_BYTES_PER_PIXEL));
1265 UByte * pDst (::
GetWriteAddress_2vuy (pDstBuffer, inDstBytesPerLine, inDstVertLineOffset, inDstHorzPixelOffset, TWO_BYTES_PER_PIXEL));
1267 for (
UWord srcLinesToCopy (numVertLinesToCopy); srcLinesToCopy > 0; srcLinesToCopy--)
1269 UWord dstPixelsCopied (0);
1270 const UByte * pSavedSrc (pSrc);
1271 UByte * pSavedDst (pDst);
1272 for (
UWord hPixelsToCopy (numHorzPixelsToCopy); hPixelsToCopy > 0; hPixelsToCopy--)
1277 if (dstPixelsCopied + inDstHorzPixelOffset >=
UWord(dstMaxPixelWidth))
1279 pDst += TWO_BYTES_PER_PIXEL;
1280 pSrc += TWO_BYTES_PER_PIXEL;
1284 pSrc += inSrcBytesPerLine;
1285 pDst += inDstBytesPerLine;
1294 const ULWord inDstBytesPerLine,
1295 const UWord inDstTotalLines,
1296 const UWord inDstVertLineOffset,
1297 const UWord inDstHorzPixelOffset,
1298 const UByte * pSrcBuffer,
1299 const ULWord inSrcBytesPerLine,
1300 const UWord inSrcTotalLines,
1301 const UWord inSrcVertLineOffset,
1302 const UWord inSrcVertLinesToCopy,
1303 const UWord inSrcHorzPixelOffset,
1304 const UWord inSrcHorzPixelsToCopy)
1306 if (inDstHorzPixelOffset % 6)
1308 if (inSrcHorzPixelOffset % 6)
1310 if (inDstBytesPerLine % 16)
1312 if (inSrcBytesPerLine % 16)
1314 if (inSrcHorzPixelsToCopy % 6)
1317 const ULWord dstMaxPixelWidth (inDstBytesPerLine / 16 * 6);
1318 const ULWord srcMaxPixelWidth (inSrcBytesPerLine / 16 * 6);
1319 ULWord numHorzPixelsToCopy (inSrcHorzPixelsToCopy);
1320 UWord numVertLinesToCopy (inSrcVertLinesToCopy);
1322 if (inDstHorzPixelOffset >= dstMaxPixelWidth)
1324 if (inSrcHorzPixelOffset >= srcMaxPixelWidth)
1326 if (inSrcHorzPixelOffset + inSrcHorzPixelsToCopy >
UWord(srcMaxPixelWidth))
1327 numHorzPixelsToCopy -= inSrcHorzPixelOffset + inSrcHorzPixelsToCopy - srcMaxPixelWidth;
1328 if (inDstHorzPixelOffset + numHorzPixelsToCopy > dstMaxPixelWidth)
1329 numHorzPixelsToCopy = inDstHorzPixelOffset + numHorzPixelsToCopy - dstMaxPixelWidth;
1331 if (inSrcVertLineOffset + inSrcVertLinesToCopy > inSrcTotalLines)
1332 numVertLinesToCopy -= inSrcVertLineOffset + inSrcVertLinesToCopy - inSrcTotalLines;
1333 if (numVertLinesToCopy + inDstVertLineOffset >= inDstTotalLines)
1335 if (numVertLinesToCopy + inDstVertLineOffset > inDstTotalLines)
1336 numVertLinesToCopy -= numVertLinesToCopy + inDstVertLineOffset - inDstTotalLines;
1341 for (
UWord lineNdx (0); lineNdx < numVertLinesToCopy; lineNdx++)
1343 const UByte * pSrcLine (pSrcBuffer + inSrcBytesPerLine * (inSrcVertLineOffset + lineNdx) + inSrcHorzPixelOffset * 16 / 6);
1344 UByte * pDstLine (pDstBuffer + inDstBytesPerLine * (inDstVertLineOffset + lineNdx) + inDstHorzPixelOffset * 16 / 6);
1345 ::memcpy (pDstLine, pSrcLine, numHorzPixelsToCopy * 16 / 6);
1355 const ULWord inDstBytesPerLine,
1356 const UWord inDstTotalLines,
1357 const UWord inDstVertLineOffset,
1358 const UWord inDstHorzPixelOffset,
1359 const UByte * pSrcBuffer,
1360 const ULWord inSrcBytesPerLine,
1361 const UWord inSrcTotalLines,
1362 const UWord inSrcVertLineOffset,
1363 const UWord inSrcVertLinesToCopy,
1364 const UWord inSrcHorzPixelOffset,
1365 const UWord inSrcHorzPixelsToCopy)
1367 if (inDstHorzPixelOffset % 16)
1369 if (inSrcHorzPixelOffset % 16)
1371 if (inDstBytesPerLine % 20)
1373 if (inSrcBytesPerLine % 20)
1375 if (inSrcHorzPixelsToCopy % 16)
1378 const ULWord dstMaxPixelWidth (inDstBytesPerLine / 20 * 16);
1379 const ULWord srcMaxPixelWidth (inSrcBytesPerLine / 20 * 16);
1380 ULWord numHorzPixelsToCopy (inSrcHorzPixelsToCopy);
1381 UWord numVertLinesToCopy (inSrcVertLinesToCopy);
1383 if (inDstHorzPixelOffset >= dstMaxPixelWidth)
1385 if (inSrcHorzPixelOffset >= srcMaxPixelWidth)
1387 if (inSrcHorzPixelOffset + inSrcHorzPixelsToCopy >
UWord(srcMaxPixelWidth))
1388 numHorzPixelsToCopy -= inSrcHorzPixelOffset + inSrcHorzPixelsToCopy - srcMaxPixelWidth;
1389 if (inDstHorzPixelOffset + numHorzPixelsToCopy > dstMaxPixelWidth)
1390 numHorzPixelsToCopy = inDstHorzPixelOffset + numHorzPixelsToCopy - dstMaxPixelWidth;
1392 if (inSrcVertLineOffset + inSrcVertLinesToCopy > inSrcTotalLines)
1393 numVertLinesToCopy -= inSrcVertLineOffset + inSrcVertLinesToCopy - inSrcTotalLines;
1394 if (numVertLinesToCopy + inDstVertLineOffset >= inDstTotalLines)
1396 if (numVertLinesToCopy + inDstVertLineOffset > inDstTotalLines)
1397 numVertLinesToCopy -= numVertLinesToCopy + inDstVertLineOffset - inDstTotalLines;
1402 for (
UWord lineNdx (0); lineNdx < numVertLinesToCopy; lineNdx++)
1404 const UByte * pSrcLine (pSrcBuffer + inSrcBytesPerLine * (inSrcVertLineOffset + lineNdx) + inSrcHorzPixelOffset * 20 / 16);
1405 UByte * pDstLine (pDstBuffer + inDstBytesPerLine * (inDstVertLineOffset + lineNdx) + inDstHorzPixelOffset * 20 / 16);
1406 ::memcpy (pDstLine, pSrcLine, numHorzPixelsToCopy * 20 / 16);
1415 const ULWord inDstBytesPerLine,
1416 const UWord inDstTotalLines,
1417 const UWord inDstVertLineOffset,
1418 const UWord inDstHorzPixelOffset,
1419 const UByte * pSrcBuffer,
1420 const ULWord inSrcBytesPerLine,
1421 const UWord inSrcTotalLines,
1422 const UWord inSrcVertLineOffset,
1423 const UWord inSrcVertLinesToCopy,
1424 const UWord inSrcHorzPixelOffset,
1425 const UWord inSrcHorzPixelsToCopy)
1427 if (inDstHorzPixelOffset % 8)
1429 if (inSrcHorzPixelOffset % 8)
1431 if (inDstBytesPerLine % 36)
1433 if (inSrcBytesPerLine % 36)
1435 if (inSrcHorzPixelsToCopy % 8)
1438 const ULWord dstMaxPixelWidth (inDstBytesPerLine / 36 * 8);
1439 const ULWord srcMaxPixelWidth (inSrcBytesPerLine / 36 * 8);
1440 ULWord numHorzPixelsToCopy (inSrcHorzPixelsToCopy);
1441 UWord numVertLinesToCopy (inSrcVertLinesToCopy);
1443 if (inDstHorzPixelOffset >= dstMaxPixelWidth)
1445 if (inSrcHorzPixelOffset >= srcMaxPixelWidth)
1447 if (inSrcHorzPixelOffset + inSrcHorzPixelsToCopy >
UWord(srcMaxPixelWidth))
1448 numHorzPixelsToCopy -= inSrcHorzPixelOffset + inSrcHorzPixelsToCopy - srcMaxPixelWidth;
1449 if (inDstHorzPixelOffset + numHorzPixelsToCopy > dstMaxPixelWidth)
1450 numHorzPixelsToCopy = inDstHorzPixelOffset + numHorzPixelsToCopy - dstMaxPixelWidth;
1452 if (inSrcVertLineOffset + inSrcVertLinesToCopy > inSrcTotalLines)
1453 numVertLinesToCopy -= inSrcVertLineOffset + inSrcVertLinesToCopy - inSrcTotalLines;
1454 if (numVertLinesToCopy + inDstVertLineOffset >= inDstTotalLines)
1456 if (numVertLinesToCopy + inDstVertLineOffset > inDstTotalLines)
1457 numVertLinesToCopy -= numVertLinesToCopy + inDstVertLineOffset - inDstTotalLines;
1462 for (
UWord lineNdx (0); lineNdx < numVertLinesToCopy; lineNdx++)
1464 const UByte * pSrcLine (pSrcBuffer + inSrcBytesPerLine * (inSrcVertLineOffset + lineNdx) + inSrcHorzPixelOffset * 36 / 8);
1465 UByte * pDstLine (pDstBuffer + inDstBytesPerLine * (inDstVertLineOffset + lineNdx) + inDstHorzPixelOffset * 36 / 8);
1466 ::memcpy (pDstLine, pSrcLine, numHorzPixelsToCopy * 36 / 8);
1476 const ULWord inDstBytesPerLine,
1477 const UWord inDstTotalLines,
1478 const UWord inDstVertLineOffset,
1479 const UWord inDstHorzPixelOffset,
1480 const UByte * pSrcBuffer,
1481 const ULWord inSrcBytesPerLine,
1482 const UWord inSrcTotalLines,
1483 const UWord inSrcVertLineOffset,
1484 const UWord inSrcVertLinesToCopy,
1485 const UWord inSrcHorzPixelOffset,
1486 const UWord inSrcHorzPixelsToCopy)
1488 const UWord FOUR_BYTES_PER_PIXEL (4);
1490 if (inDstBytesPerLine % FOUR_BYTES_PER_PIXEL)
1492 if (inSrcBytesPerLine % FOUR_BYTES_PER_PIXEL)
1495 const ULWord dstMaxPixelWidth (inDstBytesPerLine / FOUR_BYTES_PER_PIXEL);
1496 const ULWord srcMaxPixelWidth (inSrcBytesPerLine / FOUR_BYTES_PER_PIXEL);
1497 ULWord numHorzPixelsToCopy (inSrcHorzPixelsToCopy);
1498 UWord numVertLinesToCopy (inSrcVertLinesToCopy);
1500 if (inDstHorzPixelOffset >= dstMaxPixelWidth)
1502 if (inSrcHorzPixelOffset >= srcMaxPixelWidth)
1504 if (inSrcHorzPixelOffset + inSrcHorzPixelsToCopy >
UWord(srcMaxPixelWidth))
1505 numHorzPixelsToCopy -= inSrcHorzPixelOffset + inSrcHorzPixelsToCopy - srcMaxPixelWidth;
1506 if (inDstHorzPixelOffset + numHorzPixelsToCopy > dstMaxPixelWidth)
1507 numHorzPixelsToCopy = inDstHorzPixelOffset + numHorzPixelsToCopy - dstMaxPixelWidth;
1508 if (inSrcVertLineOffset + inSrcVertLinesToCopy > inSrcTotalLines)
1509 numVertLinesToCopy -= inSrcVertLineOffset + inSrcVertLinesToCopy - inSrcTotalLines;
1510 if (numVertLinesToCopy + inDstVertLineOffset >= inDstTotalLines)
1512 if (numVertLinesToCopy + inDstVertLineOffset > inDstTotalLines)
1513 numVertLinesToCopy -= numVertLinesToCopy + inDstVertLineOffset - inDstTotalLines;
1518 for (
UWord lineNdx (0); lineNdx < numVertLinesToCopy; lineNdx++)
1520 const UByte * pSrcLine (pSrcBuffer + inSrcBytesPerLine * (inSrcVertLineOffset + lineNdx) + inSrcHorzPixelOffset * FOUR_BYTES_PER_PIXEL);
1521 UByte * pDstLine (pDstBuffer + inDstBytesPerLine * (inDstVertLineOffset + lineNdx) + inDstHorzPixelOffset * FOUR_BYTES_PER_PIXEL);
1522 ::memcpy (pDstLine, pSrcLine, numHorzPixelsToCopy * FOUR_BYTES_PER_PIXEL);
1532 const ULWord inDstBytesPerLine,
1533 const UWord inDstTotalLines,
1534 const UWord inDstVertLineOffset,
1535 const UWord inDstHorzPixelOffset,
1536 const UByte * pSrcBuffer,
1537 const ULWord inSrcBytesPerLine,
1538 const UWord inSrcTotalLines,
1539 const UWord inSrcVertLineOffset,
1540 const UWord inSrcVertLinesToCopy,
1541 const UWord inSrcHorzPixelOffset,
1542 const UWord inSrcHorzPixelsToCopy)
1544 const UWord THREE_BYTES_PER_PIXEL (3);
1546 if (inDstBytesPerLine % THREE_BYTES_PER_PIXEL)
1548 if (inSrcBytesPerLine % THREE_BYTES_PER_PIXEL)
1551 const ULWord dstMaxPixelWidth (inDstBytesPerLine / THREE_BYTES_PER_PIXEL);
1552 const ULWord srcMaxPixelWidth (inSrcBytesPerLine / THREE_BYTES_PER_PIXEL);
1553 ULWord numHorzPixelsToCopy (inSrcHorzPixelsToCopy);
1554 UWord numVertLinesToCopy (inSrcVertLinesToCopy);
1556 if (inDstHorzPixelOffset >= dstMaxPixelWidth)
1558 if (inSrcHorzPixelOffset >= srcMaxPixelWidth)
1560 if (inSrcHorzPixelOffset + inSrcHorzPixelsToCopy >
UWord(srcMaxPixelWidth))
1561 numHorzPixelsToCopy -= inSrcHorzPixelOffset + inSrcHorzPixelsToCopy - srcMaxPixelWidth;
1562 if (inDstHorzPixelOffset + numHorzPixelsToCopy > dstMaxPixelWidth)
1563 numHorzPixelsToCopy = inDstHorzPixelOffset + numHorzPixelsToCopy - dstMaxPixelWidth;
1564 if (inSrcVertLineOffset + inSrcVertLinesToCopy > inSrcTotalLines)
1565 numVertLinesToCopy -= inSrcVertLineOffset + inSrcVertLinesToCopy - inSrcTotalLines;
1566 if (numVertLinesToCopy + inDstVertLineOffset >= inDstTotalLines)
1568 if (numVertLinesToCopy + inDstVertLineOffset > inDstTotalLines)
1569 numVertLinesToCopy -= numVertLinesToCopy + inDstVertLineOffset - inDstTotalLines;
1574 for (
UWord lineNdx (0); lineNdx < numVertLinesToCopy; lineNdx++)
1576 const UByte * pSrcLine (pSrcBuffer + inSrcBytesPerLine * (inSrcVertLineOffset + lineNdx) + inSrcHorzPixelOffset * THREE_BYTES_PER_PIXEL);
1577 UByte * pDstLine (pDstBuffer + inDstBytesPerLine * (inDstVertLineOffset + lineNdx) + inDstHorzPixelOffset * THREE_BYTES_PER_PIXEL);
1578 ::memcpy (pDstLine, pSrcLine, numHorzPixelsToCopy * THREE_BYTES_PER_PIXEL);
1588 const ULWord inDstBytesPerLine,
1589 const UWord inDstTotalLines,
1590 const UWord inDstVertLineOffset,
1591 const UWord inDstHorzPixelOffset,
1592 const UByte * pSrcBuffer,
1593 const ULWord inSrcBytesPerLine,
1594 const UWord inSrcTotalLines,
1595 const UWord inSrcVertLineOffset,
1596 const UWord inSrcVertLinesToCopy,
1597 const UWord inSrcHorzPixelOffset,
1598 const UWord inSrcHorzPixelsToCopy)
1600 const UWord SIX_BYTES_PER_PIXEL (6);
1602 if (inDstBytesPerLine % SIX_BYTES_PER_PIXEL)
1604 if (inSrcBytesPerLine % SIX_BYTES_PER_PIXEL)
1607 const ULWord dstMaxPixelWidth (inDstBytesPerLine / SIX_BYTES_PER_PIXEL);
1608 const ULWord srcMaxPixelWidth (inSrcBytesPerLine / SIX_BYTES_PER_PIXEL);
1609 ULWord numHorzPixelsToCopy (inSrcHorzPixelsToCopy);
1610 UWord numVertLinesToCopy (inSrcVertLinesToCopy);
1612 if (inDstHorzPixelOffset >= dstMaxPixelWidth)
1614 if (inSrcHorzPixelOffset >= srcMaxPixelWidth)
1616 if (inSrcHorzPixelOffset + inSrcHorzPixelsToCopy >
UWord(srcMaxPixelWidth))
1617 numHorzPixelsToCopy -= inSrcHorzPixelOffset + inSrcHorzPixelsToCopy - srcMaxPixelWidth;
1618 if (inDstHorzPixelOffset + numHorzPixelsToCopy > dstMaxPixelWidth)
1619 numHorzPixelsToCopy = inDstHorzPixelOffset + numHorzPixelsToCopy - dstMaxPixelWidth;
1620 if (inSrcVertLineOffset + inSrcVertLinesToCopy > inSrcTotalLines)
1621 numVertLinesToCopy -= inSrcVertLineOffset + inSrcVertLinesToCopy - inSrcTotalLines;
1622 if (numVertLinesToCopy + inDstVertLineOffset >= inDstTotalLines)
1624 if (numVertLinesToCopy + inDstVertLineOffset > inDstTotalLines)
1625 numVertLinesToCopy -= numVertLinesToCopy + inDstVertLineOffset - inDstTotalLines;
1630 for (
UWord lineNdx (0); lineNdx < numVertLinesToCopy; lineNdx++)
1632 const UByte * pSrcLine (pSrcBuffer + inSrcBytesPerLine * (inSrcVertLineOffset + lineNdx) + inSrcHorzPixelOffset * SIX_BYTES_PER_PIXEL);
1633 UByte * pDstLine (pDstBuffer + inDstBytesPerLine * (inDstVertLineOffset + lineNdx) + inDstHorzPixelOffset * SIX_BYTES_PER_PIXEL);
1634 ::memcpy (pDstLine, pSrcLine, numHorzPixelsToCopy * SIX_BYTES_PER_PIXEL);
1644 const ULWord inDstBytesPerLine,
1645 const UWord inDstTotalLines,
1646 const UWord inDstVertLineOffset,
1647 const UWord inDstHorzPixelOffset,
1648 const UByte * pSrcBuffer,
1649 const ULWord inSrcBytesPerLine,
1650 const UWord inSrcTotalLines,
1651 const UWord inSrcVertLineOffset,
1652 const UWord inSrcVertLinesToCopy,
1653 const UWord inSrcHorzPixelOffset,
1654 const UWord inSrcHorzPixelsToCopy)
1660 if (pDstBuffer == pSrcBuffer)
1662 if (inDstBytesPerLine == 0)
1664 if (inSrcBytesPerLine == 0)
1666 if (inDstTotalLines == 0)
1668 if (inSrcTotalLines == 0)
1670 if (inDstVertLineOffset >= inDstTotalLines)
1672 if (inSrcVertLineOffset >= inSrcTotalLines)
1674 switch (inPixelFormat)
1678 pSrcBuffer, inSrcBytesPerLine, inSrcTotalLines, inSrcVertLineOffset, inSrcVertLinesToCopy,
1679 inSrcHorzPixelOffset, inSrcHorzPixelsToCopy);
1683 pSrcBuffer, inSrcBytesPerLine, inSrcTotalLines, inSrcVertLineOffset, inSrcVertLinesToCopy,
1684 inSrcHorzPixelOffset, inSrcHorzPixelsToCopy);
1692 pSrcBuffer, inSrcBytesPerLine, inSrcTotalLines, inSrcVertLineOffset, inSrcVertLinesToCopy,
1693 inSrcHorzPixelOffset, inSrcHorzPixelsToCopy);
1697 pSrcBuffer, inSrcBytesPerLine, inSrcTotalLines, inSrcVertLineOffset, inSrcVertLinesToCopy,
1698 inSrcHorzPixelOffset, inSrcHorzPixelsToCopy);
1701 pSrcBuffer, inSrcBytesPerLine, inSrcTotalLines, inSrcVertLineOffset, inSrcVertLinesToCopy,
1702 inSrcHorzPixelOffset, inSrcHorzPixelsToCopy);
1705 pSrcBuffer, inSrcBytesPerLine, inSrcTotalLines, inSrcVertLineOffset, inSrcVertLinesToCopy,
1706 inSrcHorzPixelOffset, inSrcHorzPixelsToCopy);
1708 pSrcBuffer, inSrcBytesPerLine, inSrcTotalLines, inSrcVertLineOffset, inSrcVertLinesToCopy,
1709 inSrcHorzPixelOffset, inSrcHorzPixelsToCopy);
1739 switch (inFrameRate)
1755 #if !defined(NTV2_DEPRECATE_16_0)
1768 return 30.0 / 1.001;
1774 switch (inFrameRate)
1777 case NTV2_FRAMERATE_11988: outFractionNumerator = 120000; outFractionDenominator = 1001;
break;
1779 case NTV2_FRAMERATE_5994: outFractionNumerator = 60000; outFractionDenominator = 1001;
break;
1782 case NTV2_FRAMERATE_4795: outFractionNumerator = 48000; outFractionDenominator = 1001;
break;
1784 case NTV2_FRAMERATE_2997: outFractionNumerator = 30000; outFractionDenominator = 1001;
break;
1787 case NTV2_FRAMERATE_2398: outFractionNumerator = 24000; outFractionDenominator = 1001;
break;
1789 case NTV2_FRAMERATE_1498: outFractionNumerator = 15000; outFractionDenominator = 1001;
break;
1790 #if !defined(NTV2_DEPRECATE_16_0)
1792 case NTV2_FRAMERATE_1898: outFractionNumerator = 19000; outFractionDenominator = 1001;
break;
1794 case NTV2_FRAMERATE_1798: outFractionNumerator = 18000; outFractionDenominator = 1001;
break;
1795 #endif // !defined(NTV2_DEPRECATE_16_0)
1800 default: outFractionNumerator = 0; outFractionDenominator = 0;
return false;
1809 switch (inScanGeometry)
1826 const UWord inHeightLines,
1827 const UWord inWidthPixels,
1828 const bool inIsInterlaced,
1829 const bool inIsLevelB,
1837 if (inIsPSF == ::
IsPSF(fmt))
1848 switch (inVideoFormat)
2028 return quarterSizedFormat;
2034 switch (inVideoFormat)
2214 return inVideoFormat;
2225 default:
return inGeometry;
2238 default:
return inGeometry;
2254 default:
return inStandard;
2272 default:
return inStandard;
2281 switch (inVideoFormat)
2450 #if defined (_DEBUG)
2503 switch (inVideoFormat)
2535 default: result = inVideoFormat;
break;
2549 switch (inVideoFormat)
2736 #if defined (_DEBUG)
2779 ulSize = ((ulSize / 4096) + 1) * 4096;
2792 ULWord audioSamplesPerFrame(0);
2793 inCadenceFrame %= 5;
2795 if (inIsSMPTE372Enabled)
2799 switch (inFrameRate)
2816 audioSamplesPerFrame = 400;
2819 switch (inCadenceFrame)
2823 case 4: audioSamplesPerFrame = 400;
break;
2826 case 3: audioSamplesPerFrame = 401;
break;
2830 audioSamplesPerFrame = 800;
2833 switch (inCadenceFrame)
2835 case 0: audioSamplesPerFrame = 800;
break;
2840 case 4: audioSamplesPerFrame = 801;
break;
2849 switch (inCadenceFrame)
2853 case 4: audioSamplesPerFrame = 1602;
break;
2856 case 3: audioSamplesPerFrame = 1601;
break;
2865 switch (inCadenceFrame)
2867 case 0: audioSamplesPerFrame = 3204;
break;
2872 case 4: audioSamplesPerFrame = 3203;
break;
2875 #if !defined(NTV2_DEPRECATE_16_0)
2881 case NTV2_FRAMERATE_UNKNOWN:
2883 audioSamplesPerFrame = 0;
2892 audioSamplesPerFrame = 800;
2895 switch (inCadenceFrame)
2900 case 3: audioSamplesPerFrame = 801;
break;
2902 case 4: audioSamplesPerFrame = 800;
break;
2907 switch (inCadenceFrame)
2911 case 4: audioSamplesPerFrame = 1602;
break;
2914 case 3: audioSamplesPerFrame = 1601;
break;
2923 switch (inCadenceFrame)
2925 case 0: audioSamplesPerFrame = 3204;
break;
2930 case 4: audioSamplesPerFrame = 3203;
break;
2939 switch (inCadenceFrame)
2941 case 0: audioSamplesPerFrame = 3204*2;
break;
2946 case 4: audioSamplesPerFrame = 3203*2;
break;
2949 #if !defined(NTV2_DEPRECATE_16_0)
2955 case NTV2_FRAMERATE_UNKNOWN:
2957 audioSamplesPerFrame = 0*2;
2966 audioSamplesPerFrame = 1600;
2969 switch (inCadenceFrame)
2973 case 4: audioSamplesPerFrame = 1602;
break;
2976 case 3: audioSamplesPerFrame = 1601;
break;
2980 audioSamplesPerFrame = 3200;
2983 switch (inCadenceFrame)
2985 case 0: audioSamplesPerFrame = 3204;
break;
2990 case 4: audioSamplesPerFrame = 3203;
break;
2999 switch (inCadenceFrame)
3002 case 1: audioSamplesPerFrame = 6407;
break;
3006 case 4: audioSamplesPerFrame = 6406;
break;
3015 switch (inCadenceFrame)
3020 case 3: audioSamplesPerFrame = 12813;
break;
3022 case 4: audioSamplesPerFrame = 12812;
break;
3025 #if !defined(NTV2_DEPRECATE_16_0)
3031 case NTV2_FRAMERATE_UNKNOWN:
3033 audioSamplesPerFrame = 0*2;
3038 return audioSamplesPerFrame;
3049 LWord64 numAudioSamplesFromWholeGroups;
3051 ULWord numWholeGroupsOfFive;
3052 ULWord numAudioSamplesFromRemainder;
3055 numWholeGroupsOfFive = inFrameNumNonInclusive / 5;
3056 remainder = inFrameNumNonInclusive % 5;
3058 numTotalAudioSamples = 0;
3059 numAudioSamplesFromWholeGroups = 0;
3060 numAudioSamplesFromRemainder = 0;
3064 switch (inFrameRate)
3067 numTotalAudioSamples = 400 * inFrameNumNonInclusive;
3070 numAudioSamplesFromWholeGroups = ((2*401) + (3*400)) * numWholeGroupsOfFive;
3071 numAudioSamplesFromRemainder = (remainder == 0) ? 0 : ((400 * remainder) + remainder/2);
3072 numTotalAudioSamples = numAudioSamplesFromWholeGroups + numAudioSamplesFromRemainder;
3075 numTotalAudioSamples = 800 * inFrameNumNonInclusive;
3079 numAudioSamplesFromWholeGroups = ((1*800) + (4*801)) * numWholeGroupsOfFive;
3080 numAudioSamplesFromRemainder = (remainder == 0) ? 0 : ((801 * remainder) - 1);
3081 numTotalAudioSamples = numAudioSamplesFromWholeGroups + numAudioSamplesFromRemainder;
3084 numTotalAudioSamples = 1920/2 * inFrameNumNonInclusive;
3087 numTotalAudioSamples = 1000 * inFrameNumNonInclusive;
3090 numTotalAudioSamples = 1001 * inFrameNumNonInclusive;
3093 numTotalAudioSamples = 1600 * inFrameNumNonInclusive;
3097 numAudioSamplesFromWholeGroups = ((3*1602) + (2*1601)) * numWholeGroupsOfFive;
3098 numAudioSamplesFromRemainder = (remainder == 0) ? 0 : ((1602 * remainder) - remainder/2);
3099 numTotalAudioSamples = numAudioSamplesFromWholeGroups + numAudioSamplesFromRemainder;
3102 numTotalAudioSamples = 1920 * inFrameNumNonInclusive;
3105 numTotalAudioSamples = 2000 * inFrameNumNonInclusive;
3108 numTotalAudioSamples = 2002 * inFrameNumNonInclusive;
3111 numTotalAudioSamples = 3200 * inFrameNumNonInclusive;
3115 numAudioSamplesFromWholeGroups = ((1*3204) + (4*3203)) * numWholeGroupsOfFive;
3116 numAudioSamplesFromRemainder = (remainder == 0) ? 0 : ((3203 * remainder) + 1);
3117 numTotalAudioSamples = numAudioSamplesFromWholeGroups + numAudioSamplesFromRemainder;
3119 #if !defined(NTV2_DEPRECATE_16_0)
3125 case NTV2_FRAMERATE_UNKNOWN:
3127 numTotalAudioSamples = 0;
3133 switch (inFrameRate)
3136 numTotalAudioSamples = 800 * inFrameNumNonInclusive;
3139 numAudioSamplesFromWholeGroups = ((4*801) + (1*800)) * numWholeGroupsOfFive;
3140 numAudioSamplesFromRemainder = (remainder == 0) ? 0 : (801 * remainder);
3141 numTotalAudioSamples = numAudioSamplesFromWholeGroups + numAudioSamplesFromRemainder;
3144 numTotalAudioSamples = (800*2) * inFrameNumNonInclusive;
3147 numAudioSamplesFromWholeGroups = ((3*1602) + (2*1601)) * numWholeGroupsOfFive;
3148 numAudioSamplesFromRemainder = (remainder == 0) ? 0 : ((1602 * remainder) - remainder/2);
3149 numTotalAudioSamples = numAudioSamplesFromWholeGroups + numAudioSamplesFromRemainder;
3152 numTotalAudioSamples = 1920 * inFrameNumNonInclusive;
3155 numTotalAudioSamples = 2000 * inFrameNumNonInclusive;
3158 numTotalAudioSamples = 2002 * inFrameNumNonInclusive;
3161 numTotalAudioSamples = (1600*2) * inFrameNumNonInclusive;
3165 numAudioSamplesFromWholeGroups = ((1*3204) + (4*3203)) * numWholeGroupsOfFive;
3166 numAudioSamplesFromRemainder = (remainder == 0) ? 0 : ((3203 * remainder) + 1);
3167 numTotalAudioSamples = numAudioSamplesFromWholeGroups + numAudioSamplesFromRemainder;
3170 numTotalAudioSamples = (1920*2) * inFrameNumNonInclusive;
3173 numTotalAudioSamples = (2000*2) * inFrameNumNonInclusive;
3176 numTotalAudioSamples = (2002*2) * inFrameNumNonInclusive;
3179 numTotalAudioSamples = (3200*2) * inFrameNumNonInclusive;
3183 numAudioSamplesFromWholeGroups = ((1*3204*2) + (4*3203*2)) * numWholeGroupsOfFive;
3184 numAudioSamplesFromRemainder = (remainder == 0) ? 0 : (((3203*2) * remainder) + 2);
3185 numTotalAudioSamples = numAudioSamplesFromWholeGroups + numAudioSamplesFromRemainder;
3187 #if !defined(NTV2_DEPRECATE_16_0)
3193 case NTV2_FRAMERATE_UNKNOWN:
3195 numTotalAudioSamples = 0*2;
3200 return numTotalAudioSamples;
3205 static const ULWord sSamplesPerSecond [] = {48000, 96000, 192000, 0};
3208 return double(sSamplesPerSecond[inAudioRate]);
3222 switch (inSequenceRate)
3229 switch (inCadenceFrame % 2)
3241 switch (inCadenceFrame % 5)
3259 switch (inCadenceFrame % 4)
3273 switch (inCadenceFrame % 5)
3295 switch (inSequenceRate)
3302 switch (inCadenceFrame % 2)
3317 switch (inCadenceFrame % 4)
3338 switch (inSequenceRate)
3356 switch (inFrameRate)
3372 #if !defined(NTV2_DEPRECATE_16_0)
3378 case NTV2_FRAMERATE_UNKNOWN: break;
3397 if (duration == 100)
3417 else if (duration == 0)
3419 result = playFrameRate;
3423 float scaleFloat = scale / duration * float(100.0);
3424 long scaleInt = long(scaleFloat);
3433 switch (playFrameRate)
3443 if (scaleInt <= 1500 + 100)
3445 else if (scaleInt <= 2400 + 50)
3447 else if (scaleInt <= 2500 + 100)
3449 else if (scaleInt <= 3000 + 100)
3451 else if (scaleInt <= 4800 + 100)
3453 else if (scaleInt <= 5000 + 100)
3455 else if (scaleInt <= 6000 + 100)
3467 if (scaleInt <= 1498 + 100)
3469 else if (scaleInt <= 2398 + 100)
3471 else if (scaleInt <= 2997 + 100)
3473 else if (scaleInt <= 4795 + 100)
3475 else if (scaleInt <= 5994 + 100)
3490 if (inDenominator == 100)
3491 switch (inNumerator)
3511 const ULWord denominator(inDenominator == 1 ? inDenominator * 1000ULL : inDenominator);
3512 const ULWord numerator(inDenominator == 1 ? inNumerator * 1000ULL : inNumerator);
3533 switch ( videoFormat )
3732 #if defined (_DEBUG)
3757 switch (inFrameGeometry)
3769 return inFrameGeometry;
3793 #if defined (_DEBUG)
3812 switch (inFrameGeometry)
3846 return inFrameGeometry;
3847 #if defined (_DEBUG)
3892 #if defined (_DEBUG)
3944 result.insert(inFG);
3946 #if defined (_DEBUG)
3994 #if defined (_DEBUG)
4047 #if defined (_DEBUG)
4078 if (fr == inFrameRate && std == inStandard && fg == geo)
4093 return fd.GetRasterWidth();
4101 return fd.GetRasterWidth();
4124 return firstFieldTop ? smpteFirstActiveLine : smpteSecondActiveLine;
4126 return firstFieldTop ? smpteSecondActiveLine : smpteFirstActiveLine;
4133 inOutStream <<
"INVALID ";
4134 inOutStream <<
"SMPTELineNumber(";
4136 inOutStream <<
"1st=" << smpteFirstActiveLine << (firstFieldTop ?
"(top)" :
"")
4137 <<
", 2nd=" << smpteSecondActiveLine << (firstFieldTop ?
"" :
"(top)")
4140 inOutStream <<
"INVALID)";
4149 oss <<
"F" << (inRasterFieldID == 0 ?
"1" :
"2") <<
" ";
4150 oss <<
"L" << dec << inLineOffset+GetFirstActiveLine(inRasterFieldID);
4159 return "Frame count/range not specified";
4160 const bool hasCount(inStr.find(
'@') != string::npos);
4161 const bool hasRange(inStr.find(
'-') != string::npos);
4163 if (hasCount && hasRange)
4164 return "'@' and '-' cannot both be specified";
4170 strs.push_back(inStr);
4172 return "No frame count/range values parsed";
4173 if (strs.size() > 2)
4174 return "More than 2 frame count/range values parsed";
4175 if (hasCount || hasRange)
4176 if (strs.size() != 2)
4177 return "Expected exactly 2 frame count/range values";
4180 for (
size_t strNdx(0); strNdx < strs.size(); strNdx++)
4181 {
string str(strs.at(strNdx));
4183 return "Expected unsigned decimal integer value";
4184 for (
size_t chNdx(0); chNdx < str.length(); chNdx++)
4185 if (!isdigit(str.at(chNdx)))
4186 return "Non-digit character encountered in '" + str +
"'";
4195 bool isValid(
false);
4197 isValid = setRangeWithCount(numbers[0], numbers[1]);
4199 isValid = setExactRange(numbers[0], numbers[1]);
4201 isValid = setCountOnly(numbers[0]);
4202 return isValid ?
"" :
"First frame past last frame";
4212 else if (isFrameRange())
4215 oss <<
DEC(count()) <<
"@" <<
DEC(firstFrame());
4217 oss <<
DEC(firstFrame()) <<
"-" <<
DEC(lastFrame());
4220 oss <<
DEC(count());
4226 else if (isFrameRange())
4227 oss <<
"Frames " <<
DEC(firstFrame()) <<
"-" <<
DEC(lastFrame()) <<
" (" <<
DEC(lastFrame()-firstFrame()+1) <<
"@" <<
DEC(firstFrame()) <<
")";
4229 oss <<
DEC(count()) <<
" frames (auto-allocated)";
4238 "FILE 720x480 420 Planar 8 Bit 59.94i",
4239 "FILE 720x480 420 Planar 8 Bit 59.94p",
4240 "FILE 720x480 420 Planar 8 Bit 60i",
4241 "FILE 720x480 420 Planar 8 Bit 60p",
4242 "FILE 720x480 422 Planar 10 Bit 59.94i",
4243 "FILE 720x480 422 Planar 10 Bit 59.94p",
4244 "FILE 720x480 422 Planar 10 Bit 60i",
4245 "FILE 720x480 422 Planar 10 Bit 60p",
4247 "FILE 720x576 420 Planar 8 Bit 50i",
4248 "FILE 720x576 420 Planar 8 Bit 50p",
4249 "FILE 720x576 422 Planar 10 Bit 50i",
4250 "FILE 720x576 422 Planar 10 Bit 50p",
4252 "FILE 1280x720 420 Planar 8 Bit 2398p",
4253 "FILE 1280x720 420 Planar 8 Bit 24p",
4254 "FILE 1280x720 420 Planar 8 Bit 25p",
4255 "FILE 1280x720 420 Planar 8 Bit 29.97p",
4256 "FILE 1280x720 420 Planar 8 Bit 30p",
4257 "FILE 1280x720 420 Planar 8 Bit 50p",
4258 "FILE 1280x720 420 Planar 8 Bit 59.94p",
4259 "FILE 1280x720 420 Planar 8 Bit 60p",
4261 "FILE 1280x720 422 Planar 10 Bit 2398p",
4262 "FILE 1280x720 422 Planar 10 Bit 25p",
4263 "FILE 1280x720 422 Planar 10 Bit 25p",
4264 "FILE 1280x720 422 Planar 10 Bit 29.97p",
4265 "FILE 1280x720 422 Planar 10 Bit 30p",
4266 "FILE 1280x720 422 Planar 10 Bit 50p",
4267 "FILE 1280x720 422 Planar 10 Bit 59.94p",
4268 "FILE 1280x720 422 Planar 10 Bit 60p",
4270 "FILE 1920x1080 420 Planar 8 Bit 2398p",
4271 "FILE 1920x1080 420 Planar 8 Bit 24p",
4272 "FILE 1920x1080 420 Planar 8 Bit 25p",
4273 "FILE 1920x1080 420 Planar 8 Bit 29.97p",
4274 "FILE 1920x1080 420 Planar 8 Bit 30p",
4275 "FILE 1920x1080 420 Planar 8 Bit 50i",
4276 "FILE 1920x1080 420 Planar 8 Bit 50p",
4277 "FILE 1920x1080 420 Planar 8 Bit 59.94i",
4278 "FILE 1920x1080 420 Planar 8 Bit 59.94p",
4279 "FILE 1920x1080 420 Planar 8 Bit 60i",
4280 "FILE 1920x1080 420 Planar 8 Bit 60p",
4282 "FILE 1920x1080 422 Planar 10 Bit 2398p",
4283 "FILE 1920x1080 422 Planar 10 Bit 24p",
4284 "FILE 1920x1080 422 Planar 10 Bit 25p",
4285 "FILE 1920x1080 422 Planar 10 Bit 29.97p",
4286 "FILE 1920x1080 422 Planar 10 Bit 30p",
4287 "FILE 1920x1080 422 Planar 10 Bit 50i",
4288 "FILE 1920x1080 422 Planar 10 Bit 50p",
4289 "FILE 1920x1080 422 Planar 10 Bit 59.94i",
4290 "FILE 1920x1080 422 Planar 10 Bit 59.94p",
4291 "FILE 1920x1080 422 Planar 10 Bit 60i",
4292 "FILE 1920x1080 422 Planar 10 Bit 60p",
4294 "FILE 2048x1080 420 Planar 8 Bit 2398p",
4295 "FILE 2048x1080 420 Planar 8 Bit 24p",
4296 "FILE 2048x1080 420 Planar 8 Bit 25p",
4297 "FILE 2048x1080 420 Planar 8 Bit 29.97p",
4298 "FILE 2048x1080 420 Planar 8 Bit 30p",
4299 "FILE 2048x1080 420 Planar 8 Bit 50p",
4300 "FILE 2048x1080 420 Planar 8 Bit 59.94p",
4301 "FILE 2048x1080 420 Planar 8 Bit 60p",
4303 "FILE 2048x1080 422 Planar 10 Bit 2398p",
4304 "FILE 2048x1080 422 Planar 10 Bit 24p",
4305 "FILE 2048x1080 422 Planar 10 Bit 25p",
4306 "FILE 2048x1080 422 Planar 10 Bit 29.97p",
4307 "FILE 2048x1080 422 Planar 10 Bit 30p",
4308 "FILE 2048x1080 422 Planar 10 Bit 50p",
4309 "FILE 2048x1080 422 Planar 10 Bit 59.94p",
4310 "FILE 2048x1080 422 Planar 10 Bit 60p",
4312 "FILE 3840x2160 420 Planar 8 Bit 2398p",
4313 "FILE 3840x2160 420 Planar 8 Bit 24p",
4314 "FILE 3840x2160 420 Planar 8 Bit 25p",
4315 "FILE 3840x2160 420 Planar 8 Bit 29.97p",
4316 "FILE 3840x2160 420 Planar 8 Bit 30p",
4317 "FILE 3840x2160 420 Planar 8 Bit 50p",
4318 "FILE 3840x2160 420 Planar 8 Bit 59.94p",
4319 "FILE 3840x2160 420 Planar 8 Bit 60p",
4321 "FILE 3840x2160 420 Planar 10 Bit 50p",
4322 "FILE 3840x2160 420 Planar 10 Bit 59.94p",
4323 "FILE 3840x2160 420 Planar 10 Bit 60p",
4325 "FILE 3840x2160 422 Planar 8 Bit 2398p",
4326 "FILE 3840x2160 422 Planar 8 Bit 24p",
4327 "FILE 3840x2160 422 Planar 8 Bit 25p",
4328 "FILE 3840x2160 422 Planar 8 Bit 29.97p",
4329 "FILE 3840x2160 422 Planar 8 Bit 30p",
4330 "FILE 3840x2160 422 Planar 8 Bit 50p",
4331 "FILE 3840x2160 422 Planar 8 Bit 59.94p",
4332 "FILE 3840x2160 422 Planar 8 Bit 60p",
4334 "FILE 3840x2160 422 Planar 10 Bit 2398p",
4335 "FILE 3840x2160 422 Planar 10 Bit 24p",
4336 "FILE 3840x2160 422 Planar 10 Bit 25p",
4337 "FILE 3840x2160 422 Planar 10 Bit 29.97p",
4338 "FILE 3840x2160 422 Planar 10 Bit 30p",
4339 "FILE 3840x2160 422 Planar 10 Bit 50p",
4340 "FILE 3840x2160 422 Planar 10 Bit 59.94p",
4341 "FILE 3840x2160 422 Planar 10 Bit 60p",
4343 "FILE 4096x2160 420 Planar 10 Bit 5994p",
4344 "FILE 4096x2160 420 Planar 10 Bit 60p",
4345 "FILE 4096x2160 422 Planar 10 Bit 50p",
4346 "FILE 4096x2160 422 Planar 10 Bit 5994p IOnly",
4347 "FILE 4096x2160 422 Planar 10 Bit 60p IOnly",
4349 "VIF 720x480 420 Planar 8 Bit 59.94i",
4350 "VIF 720x480 420 Planar 8 Bit 59.94p",
4351 "VIF 720x480 420 Planar 8 Bit 60i",
4352 "VIF 720x480 420 Planar 8 Bit 60p",
4353 "VIF 720x480 422 Planar 10 Bit 59.94i",
4354 "VIF 720x480 422 Planar 10 Bit 59.94p",
4355 "VIF 720x480 422 Planar 10 Bit 60i",
4356 "VIF 720x480 422 Planar 10 Bit 60p",
4358 "VIF 720x576 420 Planar 8 Bit 50i",
4359 "VIF 720x576 420 Planar 8 Bit 50p",
4360 "VIF 720x576 422 Planar 10 Bit 50i",
4361 "VIF 720x576 422 Planar 10 Bit 50p",
4363 "VIF 1280x720 420 Planar 8 Bit 50p",
4364 "VIF 1280x720 420 Planar 8 Bit 59.94p",
4365 "VIF 1280x720 420 Planar 8 Bit 60p",
4366 "VIF 1280x720 422 Planar 10 Bit 50p",
4367 "VIF 1280x720 422 Planar 10 Bit 59.94p",
4368 "VIF 1280x720 422 Planar 10 Bit 60p",
4370 "VIF 1920x1080 420 Planar 8 Bit 50i",
4371 "VIF 1920x1080 420 Planar 8 Bit 50p",
4372 "VIF 1920x1080 420 Planar 8 Bit 59.94i",
4373 "VIF 1920x1080 420 Planar 8 Bit 59.94p",
4374 "VIF 1920x1080 420 Planar 8 Bit 60i",
4375 "VIF 1920x1080 420 Planar 8 Bit 60p",
4376 "VIF 1920x1080 420 Planar 10 Bit 50i",
4377 "VIF 1920x1080 420 Planar 10 Bit 50p",
4378 "VIF 1920x1080 420 Planar 10 Bit 59.94i",
4379 "VIF 1920x1080 420 Planar 10 Bit 59.94p",
4380 "VIF 1920x1080 420 Planar 10 Bit 60i",
4381 "VIF 1920x1080 420 Planar 10 Bit 60p",
4382 "VIF 1920x1080 422 Planar 10 Bit 59.94i",
4383 "VIF 1920x1080 422 Planar 10 Bit 59.94p",
4384 "VIF 1920x1080 422 Planar 10 Bit 60i",
4385 "VIF 1920x1080 422 Planar 10 Bit 60p",
4387 "VIF 3840x2160 420 Planar 8 Bit 30p",
4388 "VIF 3840x2160 420 Planar 8 Bit 50p",
4389 "VIF 3840x2160 420 Planar 8 Bit 59.94p",
4390 "VIF 3840x2160 420 Planar 8 Bit 60p",
4391 "VIF 3840x2160 420 Planar 10 Bit 50p",
4392 "VIF 3840x2160 420 Planar 10 Bit 59.94p",
4393 "VIF 3840x2160 420 Planar 10 Bit 60p",
4395 "VIF 3840x2160 422 Planar 10 Bit 30p",
4396 "VIF 3840x2160 422 Planar 10 Bit 50p",
4397 "VIF 3840x2160 422 Planar 10 Bit 59.94p",
4398 "VIF 3840x2160 422 Planar 10 Bit 60p",
4404 int iNumChans,
bool bKeepAudio24Bits)
4420 UWord * puwOut =
reinterpret_cast<UWord*
>(pSR);
4424 int iNumSamples = iNumBytes / SAMPLE_SIZE;
4425 int iMod = (iNumBytes % SAMPLE_SIZE) / 4;
4443 if (bKeepAudio24Bits)
4445 for (
int s = 0; s < iNumSamples; s++)
4447 for (
int c = iChan0; c < iChan0 + iNumChans; c++)
4457 for (
int s = 0; s < iNumSamples; s++)
4459 for (
int c = iChan0; c < iChan0 + iNumChans; c++)
4461 *puwOut++ =
UWord(pIn[c] >> 16);
4474 #define M_PI (3.14159265358979323846)
4480 ULWord & inOutCurrentSample,
4481 const ULWord inNumSamples,
4482 const double inSampleRate,
4483 const double inAmplitude,
4484 const double inFrequency,
4485 const ULWord inNumBitsPerSample,
4486 const bool inByteSwap,
4487 const ULWord inNumChannels)
4489 outNumBytesWritten = 0;
4490 if (inAudioBuffer.
IsNULL())
4493 const ULWord numBytes (4 * inNumSamples * inNumChannels);
4497 double j (inOutCurrentSample);
4498 const double cycleLength (inSampleRate / inFrequency);
4499 const double scale (
double(
ULWord(1 << (inNumBitsPerSample - 1))) - 1.0);
4500 ULWord * pAudioBuffer(inAudioBuffer);
4503 for (
ULWord i(0); i < inNumSamples; i++)
4505 const double nextFloat = double(::sin (j / cycleLength * (
M_PI * 2.0)) * inAmplitude);
4506 LWord value =
LWord((nextFloat * scale) +
double(0.5));
4511 for (
ULWord channel(0); channel < inNumChannels; channel++)
4512 *pAudioBuffer++ =
ULWord(value);
4515 if (j > cycleLength)
4517 inOutCurrentSample++;
4520 outNumBytesWritten = numBytes;
4527 ULWord & inOutCurrentSample,
4528 const ULWord inNumSamples,
4529 const double inSampleRate,
4530 const double inAmplitude,
4531 const double inFrequency,
4533 const bool inByteSwap,
4534 const ULWord inNumChannels)
4536 double j (inOutCurrentSample);
4537 const double cycleLength (inSampleRate / inFrequency);
4538 const double scale (
double (
ULWord (1 << (inNumBits - 1))) - 1.0);
4542 for (
ULWord i = 0; i < inNumSamples; i++)
4544 const double nextFloat = double(::sin (j / cycleLength * (
M_PI * 2.0)) * inAmplitude);
4545 LWord value =
LWord((nextFloat * scale) +
double(0.5));
4550 for (
ULWord channel = 0; channel < inNumChannels; channel++)
4551 *pAudioBuffer++ =
ULWord(value);
4554 if (j > cycleLength)
4556 inOutCurrentSample++;
4560 return inNumSamples * 4 * inNumChannels;
4566 ULWord & inOutCurrentSample,
4567 const ULWord inNumSamples,
4568 const double inSampleRate,
4569 const double inAmplitude,
4570 const double inFrequency,
4572 const bool inByteSwap,
4573 const ULWord inNumChannels)
4575 double j (inOutCurrentSample);
4576 const double cycleLength (inSampleRate / inFrequency);
4577 const double scale (
double (
ULWord (1 << (inNumBits - 1))) - 1.0);
4581 for (
ULWord i(0); i < inNumSamples; i++)
4583 const double nextFloat = double(::sin (j / cycleLength * (
M_PI * 2.0)) * inAmplitude);
4584 Word value =
Word((nextFloat * scale) +
double(0.5));
4589 for (
ULWord channel(0); channel < inNumChannels; channel++)
4590 *pAudioBuffer++ =
UWord(value);
4593 if (j > cycleLength)
4595 inOutCurrentSample++;
4599 return inNumSamples * 4 * inNumChannels;
4605 ULWord & inOutCurrentSample,
4606 const ULWord inNumSamples,
4607 const double inSampleRate,
4608 const double * pInAmplitudes,
4609 const double * pInFrequencies,
4611 const bool inByteSwap,
4612 const ULWord inNumChannels)
4616 const double scale (
double(
ULWord (1 << (inNumBits - 1))) - 1.0);
4618 for (
ULWord channel(0); channel < inNumChannels; channel++)
4620 cycleLength[channel] = inSampleRate / pInFrequencies[channel];
4621 j [channel] = inOutCurrentSample;
4624 if (pAudioBuffer && pInAmplitudes && pInFrequencies)
4626 for (
ULWord i(0); i < inNumSamples; i++)
4628 for (
ULWord channel(0); channel < inNumChannels; channel++)
4630 const double nextFloat = double(::sin(j[channel] / cycleLength[channel] * (
M_PI * 2.0)) * pInAmplitudes[channel]);
4631 LWord value =
LWord((nextFloat * scale) +
double(0.5));
4636 *pAudioBuffer++ =
ULWord(value);
4639 if (j[channel] > cycleLength[channel])
4640 j[channel] -= cycleLength[channel];
4643 inOutCurrentSample++;
4647 return inNumSamples * 4 * inNumChannels;
4653 ULWord & inOutCurrentSample,
4654 const ULWord inNumSamples,
4656 const bool inEndianConvert,
4657 const ULWord inNumChannels)
4660 for (
ULWord i(0); i < inNumSamples; i++)
4662 ULWord value ((inOutCurrentSample % inModulus) << 16);
4663 if (inEndianConvert)
4665 for (
ULWord channel(0); channel < inNumChannels; channel++)
4666 *pAudioBuffer++ = value;
4667 inOutCurrentSample++;
4669 return inNumSamples * 4 * inNumChannels;
4743 case DEVICE_ID_TTAP:
return inForRetailDisplay ?
"T-TAP" :
"TTap";
4751 return inForRetailDisplay ?
"Unknown" :
"???";
4768 switch (inCrosspointChannel)
4952 return gInputSourceToEmbeddedAudioInputs [inInputSource];
4978 return gChannelToInputChannelSpec [inChannel];
4989 return gChannelToOutputChannelSpec [inChannel];
5074 return gInputSourceToChannelSpec [inInputSource];
5098 return gInputSourceToReferenceSource [inInputSource];
5122 return gInputSourceToChannel [inInputSource];
5146 return gInputSourceToAudioSystem [inInputSource];
5184 return inEmbeddedLTC ? gInputSourceToLTCIndex [inInputSource] : gInputSourceToTCIndex [inInputSource];
5202 switch (inSourceType)
5221 return gOutputDestToChannel [inOutputDest];
5233 return gChannelToOutputDest [inChannel];
5242 return inTargetFormat;
5252 if (inFormat1 == inFormat2)
5263 default:
return false;
5278 return sSDIInputSources[inIndex0];
5282 return sHDMIInputSources[inIndex0];
5286 return sANLGInputSources[inIndex0];
5308 static const ULWord sInputSourcesIndexes [] = { 0,
5310 0, 1, 2, 3, 4, 5, 6, 7 };
5311 if (
static_cast <size_t> (inValue) <
sizeof (sInputSourcesIndexes) /
sizeof (
ULWord))
5312 return sInputSourcesIndexes [inValue];
5321 static const ULWord gFrameSizeToByteCount[] = { 2 , 4 , 8 , 16 ,
5327 return gFrameSizeToByteCount [inFrameSize] * 1024 * 1024;
5336 static const ULWord gBufferSizeToByteCount[] = { 1 * 1024*1024, 4 * 1024*1024, 2 * 1024*1024, 3 * 1024*1024, 0 };
5338 return gBufferSizeToByteCount[inBufferSize];
5382 if (iter != family.end())
5383 return *(family.begin());
5391 if (inFrameRate1 == inFrameRate2)
5403 return frFamily1 == frFamily2;
5659 switch( conversionMode )
5703 switch( conversionMode )
5743 return outputFormat;
5749 return inOutStream << inFrameDimensions.Width() <<
"Wx" << inFrameDimensions.Height() <<
"H";
5755 return inSmpteLineNumber.
Print (inOutStream);
5781 oss << (inCompactDisplay ?
"AudSys" :
"NTV2_AUDIOSYSTEM_") << (inValue + 1);
5783 oss << (inCompactDisplay ?
"NoAudio" :
"NTV2_AUDIOSYSTEM_INVALID");
5839 std::ostringstream oss;
6182 #if !defined(NTV2_DEPRECATE_16_0)
6186 #if !defined(_DEBUG)
6395 inOutStr <<
"[" << inObj.size () <<
" regs: ";
6399 if (++iter != inObj.end ())
6402 return inOutStr <<
"]";
6408 inOutSet.insert (inRegisterNumber);
6454 oss << (inCompactDisplay ?
"" :
"NTV2_AudioChannel") <<
DEC(inValue * 2 + 1) << (inCompactDisplay ?
"-" :
"_") <<
DEC(inValue * 2 + 2);
6455 else if (!inCompactDisplay)
6456 oss <<
"NTV2_AUDIO_CHANNEL_PAIR_INVALID";
6465 oss << (inCompactDisplay ?
"" :
"NTV2_AudioChannel") << (inValue * 4 + 1) << (inCompactDisplay ?
"-" :
"_") << (inValue * 4 + 4);
6466 else if (!inCompactDisplay)
6467 oss <<
"NTV2_AUDIO_CHANNEL_QUAD_INVALID";
6476 oss << (inCompactDisplay ?
"" :
"NTV2_AudioChannel") << (inValue * 8 + 1) << (inCompactDisplay ?
"-" :
"_") << (inValue * 8 + 8);
6477 else if (!inCompactDisplay)
6478 oss <<
"NTV2_AUDIO_CHANNEL_OCTET_INVALID";
6909 default:
return "Unknown";
6984 if (inForRetailDisplay)
7203 #if !defined(NTV2_DEPRECATE_16_0)
7209 case NTV2_NUM_FRAMERATES: return ""; //special case
7302 switch(inInterruptEnumValue)
7352 switch (inIpErrorEnumValue)
7392 default:
return "Unknown IP error";
7398 return inOutStream <<
"DBB=0x" << hex << setw (8) << setfill (
'0') << inObj.
DBB
7399 <<
"|HI=0x" << hex << setw (8) << setfill (
'0') << inObj.
High
7400 <<
"|LO=0x" << hex << setw (8) << setfill (
'0') << inObj.
Low
7407 bool useWindowsName = !useOemNameOnWindows;
7408 #if defined (AJAMac) || defined (AJALinux)
7409 useWindowsName =
false;
7414 case DEVICE_ID_CORVID1:
return useWindowsName ?
"corvid1_pcie.bit" :
"corvid1pcie.bit";
7415 case DEVICE_ID_CORVID22:
return useWindowsName ?
"corvid22_pcie.bit" :
"Corvid22.bit";
7416 case DEVICE_ID_CORVID24:
return useWindowsName ?
"corvid24_pcie.bit" :
"corvid24_quad.bit";
7417 case DEVICE_ID_CORVID3G:
return useWindowsName ?
"corvid3G_pcie.bit" :
"corvid1_3gpcie.bit";
7418 case DEVICE_ID_CORVID44:
return useWindowsName ?
"corvid44_pcie.bit" :
"corvid_44.bit";
7419 case DEVICE_ID_CORVID88:
return useWindowsName ?
"corvid88_pcie.bit" :
"corvid_88.bit";
7421 case DEVICE_ID_IO4K:
return useWindowsName ?
"io4k_pcie.bit" :
"IO_XT_4K.bit";
7422 case DEVICE_ID_IO4KUFC:
return useWindowsName ?
"io4k_ufc_pcie.bit" :
"IO_XT_4K_UFC.bit";
7423 case DEVICE_ID_IOEXPRESS:
return useWindowsName ?
"ioexpress_pcie.bit" :
"chekov_00_pcie.bit";
7424 case DEVICE_ID_IOXT:
return useWindowsName ?
"ioxt_pcie.bit" :
"top_io_tx.bit";
7425 case DEVICE_ID_KONA3G:
return useWindowsName ?
"kona3g_pcie.bit" :
"k3g_top.bit";
7427 case DEVICE_ID_KONA4:
return useWindowsName ?
"kona4_pcie.bit" :
"kona_4_quad.bit";
7428 case DEVICE_ID_KONA4UFC:
return useWindowsName ?
"kona4_ufc_pcie.bit" :
"kona_4_ufc.bit";
7435 case DEVICE_ID_KONALHI:
return useWindowsName ?
"lhi_pcie.bit" :
"top_pike.bit";
7436 case DEVICE_ID_TTAP:
return useWindowsName ?
"ttap_pcie.bit" :
"t_tap_top.bit";
7443 case DEVICE_ID_KONAHDMI:
return useWindowsName ?
"kona_hdmi_4rx.bit" :
"kona_hdmi_4rx.bit";
7444 case DEVICE_ID_KONA1:
return useWindowsName ?
"kona1_pcie.bit" :
"kona1.bit";
7489 if (inBitfileName == deviceBitfileName)
7512 typedef map <string, NTV2DeviceID> BitfileName2DeviceID;
7513 static BitfileName2DeviceID sBitfileName2DeviceID;
7514 if (sBitfileName2DeviceID.empty ())
7523 for (
unsigned ndx (0); ndx <
sizeof (sDeviceIDs) /
sizeof (
NTV2DeviceID); ndx++)
7526 return sBitfileName2DeviceID [inBitfileName];
7532 #if defined (AJAMac)
7533 return "/Library/Application Support/AJA/Firmware";
7534 #elif defined (MSWindows)
7536 DWORD bufferSize (1024);
7537 char * lpData (
new char [bufferSize]);
7539 if (RegOpenKeyExA (HKEY_LOCAL_MACHINE,
"Software\\AJA",
NULL, KEY_READ, &hKey) == ERROR_SUCCESS
7540 && RegQueryValueExA (hKey,
"firmwarePath",
NULL,
NULL, (LPBYTE) lpData, &bufferSize) == ERROR_SUCCESS)
7541 return string (lpData);
7544 #elif defined (AJALinux)
7545 return "/opt/aja/firmware";
7627 for (
unsigned ndx(0); ndx <
sizeof(sValidDeviceIDs) /
sizeof(
NTV2DeviceID); ndx++)
7632 bool insertIt (
false);
7660 result.insert (deviceID);
7694 switch (inVersionComponent)
7813 return "(bad bitfile type)";
7918 if (it != inList.end())
7946 if (++it != inData.end())
7947 inOutStream <<
", ";
7957 if (++it != inData.end())
7958 inOutStream <<
", ";
7976 result.insert (it->registerNumber);
7982 outGone.clear(); outSame.clear(); outNew.clear();
7983 set_difference (inBefore.begin(), inBefore.end(), inAfter.begin(), inAfter.end(), std::inserter(outGone, outGone.begin()));
7984 set_difference (inAfter.begin(), inAfter.end(), inBefore.begin(), inBefore.end(), std::inserter(outNew, outNew.begin()));
7985 set_intersection (inBefore.begin(), inBefore.end(), inAfter.begin(), inAfter.end(), std::inserter(outSame, outSame.begin()));
7992 if (&inBefore == &inAfter)
7994 if (inBefore.size() != inAfter.size())
7997 set_intersection (before.begin(), before.end(), after.begin(), after.end(),
7998 std::inserter(commonRegNums, commonRegNums.begin()));
8003 if (beforeIt != inBefore.end() && afterIt != inAfter.end() && beforeIt->registerValue != afterIt->registerValue)
8004 outChanged.insert(*it);
8007 else if (inBefore.at(0).registerNumber == inAfter.at(0).registerNumber
8008 && inBefore.at(inBefore.size()-1).registerNumber == inAfter.at(inAfter.size()-1).registerNumber)
8010 for (
size_t ndx(0); ndx < inBefore.size(); ndx++)
8011 if (inBefore[ndx].registerValue != inAfter[ndx].registerValue)
8012 outChanged.insert(inBefore[ndx].registerNumber);
8014 else for (
size_t ndx(0); ndx < inBefore.size(); ndx++)
8026 if (it != inAfter.end())
8031 return !outChanged.empty();
8036 { ostringstream oss;
8037 for (
size_t ndx(0); ndx < inStr.size(); ndx++)
8039 const char chr(inStr.at(
size_t(ndx)));
8040 if (::isalnum(chr) || chr ==
'-' || chr ==
'_' || chr ==
'.' || chr ==
'~')
8043 oss <<
"%" <<
HEX0N(
unsigned(chr),2);
8049 { ostringstream oss;
8050 unsigned hexNum(0), state(0);
8051 for (
size_t ndx(0); ndx < inStr.size(); ndx++)
8053 const char chr(inStr.at(
size_t(ndx)));
8057 if (::isalnum(chr) || chr ==
'-' || chr ==
'_' || chr ==
'.' || chr ==
'~')
8063 if (chr >=
'A' && chr <=
'F')
8064 hexNum = unsigned(chr + 10 -
'A') << 4;
8065 else if (chr >=
'a' && chr <=
'f')
8066 hexNum = unsigned(chr + 10 -
'a') << 4;
8067 else if (chr >=
'0' && chr <=
'9')
8068 hexNum = unsigned(chr -
'0') << 4;
8074 if (chr >=
'A' && chr <=
'F')
8075 hexNum += unsigned(chr + 10 -
'A');
8076 else if (chr >=
'a' && chr <=
'f')
8077 hexNum += unsigned(chr + 10 -
'a');
8078 else if (chr >=
'0' && chr <=
'9')
8079 hexNum += unsigned(chr -
'0');
8080 oss << char(hexNum);
8093 if (inSerNumStr.length() < 8 || inSerNumStr.length() > 9)
8095 string serNumStr(inSerNumStr);
8096 if (inSerNumStr.length() == 9)
8097 serNumStr.erase(0,1);
8099 for (
size_t ndx(0); ndx < serNumStr.length(); ndx++)
8101 const char ch (serNumStr.at(ndx));
8103 if ( ! ( ( (ch >=
'0') && (ch <=
'9') ) ||
8104 ( (ch >=
'A') && (ch <=
'Z') ) ||
8105 ( (ch >=
'a') && (ch <=
'z') ) ||
8106 (ch ==
' ') || (ch ==
'-') ) )
8108 serNum |= uint64_t(ch) << (ndx*8);
8116 const ULWord serialNumHigh (inSerNum >> 32);
8117 const ULWord serialNumLow (inSerNum & 0x00000000FFFFFFFF);
8120 serialNum[0] = char((serialNumLow & 0x000000FF) );
8121 serialNum[1] = char((serialNumLow & 0x0000FF00) >> 8);
8122 serialNum[2] = char((serialNumLow & 0x00FF0000) >> 16);
8123 serialNum[3] = char((serialNumLow & 0xFF000000) >> 24);
8124 serialNum[4] = char((serialNumHigh & 0x000000FF) );
8125 serialNum[5] = char((serialNumHigh & 0x0000FF00) >> 8);
8126 serialNum[6] = char((serialNumHigh & 0x00FF0000) >> 16);
8127 serialNum[7] = char((serialNumHigh & 0xFF000000) >> 24);
8128 serialNum[8] =
'\0';
8130 for (
unsigned ndx(0); ndx < 8; ndx++)
8132 if (serialNum[ndx] == 0)
8140 if ( ! ( ( (serialNum[ndx] >=
'0') && (serialNum[ndx] <=
'9') ) ||
8141 ( (serialNum[ndx] >=
'A') && (serialNum[ndx] <=
'Z') ) ||
8142 ( (serialNum[ndx] >=
'a') && (serialNum[ndx] <=
'z') ) ||
8143 (serialNum[ndx] ==
' ') || (serialNum[ndx] ==
'-') ) )
8149 #if defined (AJAMac)
8159 outMaj = outMin = outPt = outBld = outType = 0;
8166 const string key(
"<key>CFBundleShortVersionString</key>");
8167 for (
string line; std::getline(ifs, line); )
8169 size_t keyPos(line.find(key));
8170 if (keyPos == string::npos)
8172 if (!std::getline(ifs, line))
8175 size_t startPos(line.find(
"<string>")), endPos(line.find(
"</string>"));
8176 if (startPos == string::npos || endPos == string::npos)
8179 if (endPos < startPos)
8181 string versStr(line.substr(startPos, endPos-startPos));
8184 if (versComps.size() < 3 || versComps.size() > 4)
8186 const string sBuildTypes(
"_bad");
8187 for (
size_t bt(1); bt < 4; bt++)
8188 if (versComps.at(2).find(sBuildTypes[bt]) != string::npos)
8190 aja::split(versComps.at(2), sBuildTypes[bt], lastComps);
8191 versComps.erase(versComps.begin()+2);
8192 versComps.push_back(lastComps.at(0));
8194 outType =
UWord(bt);
8197 for (
size_t ndx(0); ndx < versComps.size(); ndx++)
8199 if (versComps.at(ndx).empty())
8208 else if (ndx == 3 && outType == 0)
@ NTV2_XptFrameBuffer6YUV
@ NTV2_1080i_5000to1080psf_2500
@ NTV2_XptFrameBuffer8_DS2RGB
@ M31_FILE_1280X720_420_8_30p
@ NTV2_FORMAT_FIRST_UHD2_DEF_FORMAT
@ NTV2_MAX_NUM_DownConvertModes
NTV2FrameRate GetFrameRateFamily(const NTV2FrameRate inFrameRate)
void CopyToQuadrant(uint8_t *srcBuffer, uint32_t srcHeight, uint32_t srcRowBytes, uint32_t dstQuadrant, uint8_t *dstBuffer, uint32_t quad13Offset)
#define NTV2_IS_TALLER_VANC_GEOMETRY(__g__)
@ NTV2_REFERENCE_HDMI_INPUT2
Specifies the HDMI In 2 connector.
bool NTV2DeviceCanDoCapture(const NTV2DeviceID inDeviceID)
bool NTV2IsCompatibleBitfileName(const string &inBitfileName, const NTV2DeviceID inDeviceID)
@ NTV2CROSSPOINT_CHANNEL4
@ NTV2_FORMAT_3840x2160p_6000
std::string NTV2IpErrorEnumToString(const NTV2IpError inIpErrorEnumValue)
NTV2InputSource NTV2TimecodeIndexToInputSource(const NTV2TCIndex inTCIndex)
Converts the given NTV2TCIndex value into the appropriate NTV2InputSource value.
@ NTV2_XptFrameBuffer4YUV
@ NTV2_FBF_10BIT_YCBCR_420PL3_LE
See 3-Plane 10-Bit YCbCr 4:2:0 ('I420_10LE' a.k.a. 'YUV-P420-L10').
@ NTV2_XptDualLinkIn3DSInput
@ NTV2_FORMAT_4096x2160psf_2500
bool NTV2DeviceCanDo12GSDI(const NTV2DeviceID inDeviceID)
@ NTV2IpErrCannotGetMacAddress
void UnPack10BitDPXtoForRP215(UWord *rawrp215Buffer, ULWord *DPXLinebuffer, ULWord numPixels)
@ DEVICE_ID_KONALHIDVI
See KONA LHi.
@ NTV2_XptMixer4BGKeyInput
unsigned long stoul(const std::string &str, std::size_t *idx, int base)
@ NTV2_BITFILE_KONA5_OE3_MAIN
@ NTV2_XptMixer3FGVidInput
@ NTV2_FORMAT_4x1920x1080p_6000
uint16_t maxContentLightLevel
@ NTV2WidgetType_HDMIOutV5
@ NTV2_1080i_5994to1080psf_2997
@ M31_FILE_3840X2160_420_8_5994p
string NTV2M31VideoPresetToString(const M31VideoPreset inValue, const bool inForRetailDisplay)
NTV2RegReadsConstIter FindFirstMatchingRegisterNumber(const uint32_t inRegNum, const NTV2RegReads &inRegInfos)
Returns a const iterator to the first entry in the NTV2RegInfo collection with a matching register nu...
@ NTV2_INPUTSOURCE_SDI4
Identifies the 4th SDI video input.
string NTV2GetBitfileName(const NTV2DeviceID inBoardID, const bool useOemNameOnWindows)
string NTV2AudioChannelQuadToString(const NTV2Audio4ChannelSelect inValue, const bool inCompactDisplay)
@ NTV2_FG_4x2048x1080
4096x2160, for 4K, NTV2_VANCMODE_OFF
@ NTV2_BITFILE_KONAIP_2110
@ NTV2_FORMAT_3840x2160psf_2500
void PackRGB10BitFor10BitRGBPacked(RGBAlpha10BitPixel *pBuffer, const ULWord inNumPixels)
@ NTV2_FORMAT_1080psf_2398
static const NTV2TCIndex gChanVITC2[]
@ NTV2_TCINDEX_SDI4
SDI 4 embedded VITC.
@ NTV2WidgetType_DCIMixer
@ NTV2_XptMultiLinkOut2DS3
New in SDK 16.0.
@ M31_FILE_1280X720_420_8_60p
@ NTV2_REFERENCE_INPUT3
Specifies the SDI In 3 connector.
@ NTV2_BITFILE_KONA5_3DLUT_MAIN
#define AJA_NTV2_SDK_BUILD_DATETIME
The date and time the SDK was built, in ISO-8601 format.
@ NTV2_XptDualLinkOut7Input
@ NTV2_FORMAT_4096x2160p_2400
@ NTV2CROSSPOINT_CHANNEL8
@ NTV2_WgtStereoCompressor
@ M31_FILE_1920X1080_422_10_24p
void UnpackLine_10BitYUVto16BitYUV(const ULWord *pIn10BitYUVLine, UWord *pOut16BitYUVLine, const ULWord inNumPixels)
Unpacks a line of 10-bit-per-component YCbCr video into 16-bit-per-component YCbCr (NTV2_FBF_10BIT_YC...
@ M31_FILE_1920X1080_420_8_50i
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
@ M31_FILE_2048X1080_422_10_50p
ULWordSetConstIter NTV2RegNumSetConstIter
A const iterator that iterates over a set of distinct NTV2RegisterNumbers.
@ NTV2_XptDuallinkOut8DS2
@ NTV2_FBF_ARGB
See 8-Bit ARGB, RGBA, ABGR Formats.
@ NTV2WidgetType_DualLinkV1In
@ NTV2_FBF_10BIT_YCBCR_420PL2
10-Bit 4:2:0 2-Plane YCbCr
@ DEVICE_ID_KONAIP_2110
See KONA IP.
static const UByte * GetReadAddress_2vuy(const UByte *pInFrameBuffer, const ULWord inBytesPerVertLine, const UWord inVertLineOffset, const UWord inHorzPixelOffset, const UWord inBytesPerHorzPixel)
uint8_t electroOpticalTransferFunction
@ NTV2_BITFILE_KONAIP_4CH_2SFP
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
bool IsMultiFormatCompatible(const NTV2FrameRate inFrameRate1, const NTV2FrameRate inFrameRate2)
Compares two frame rates and returns true if they are "compatible" (with respect to a multiformat-cap...
@ NTV2_DEVICEKIND_OUTPUT
Specifies devices that output (playout).
bool AddAudioTone(ULWord &outNumBytesWritten, NTV2Buffer &inAudioBuffer, ULWord &inOutCurrentSample, const ULWord inNumSamples, const double inSampleRate, const double inAmplitude, const double inFrequency, const ULWord inNumBitsPerSample, const bool inByteSwap, const ULWord inNumChannels)
Fills the given buffer with 32-bit (ULWord) audio tone samples.
@ NTV2_720p_5994to1080i_5994
@ NTV2_FORMAT_4096x2160p_12000
UWord NTV2DeviceGetNumHDMIVideoInputs(const NTV2DeviceID inDeviceID)
bool IsRaw(const NTV2FrameBufferFormat frameBufferFormat)
@ M31_VIF_3840X2160_422_10_50p
@ NTV2_XptStereoLeftInput
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
@ DEVICE_ID_KONAHDMI
See KONA HDMI.
@ NTV2MIXERINPUTCONTROL_UNSHAPED
@ NTV2_XptMultiLinkOut2Input
New in SDK 16.0.
@ NTV2_INPUTSOURCE_SDI6
Identifies the 6th SDI video input.
NTV2DeviceIDList::const_iterator NTV2DeviceIDListConstIter
A convenient const iterator for NTV2DeviceIDList.
#define NTV2_IS_VALID_NTV2FrameGeometry(__s__)
@ AUTOCIRCVIDPROCMODE_HORZWIPE
@ NTV2_DEVICEKIND_RELAYS
Specifies devices that have bypass relays.
@ NTV2_STANDARD_2Kx1080p
Identifies SMPTE HD 2K1080p.
@ NTV2_BITFILE_SOJI_OE7_MAIN
@ M31_FILE_3840X2160_420_8_60p
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
@ NTV2_FORMAT_4x4096x2160p_4800
@ NTV2WidgetType_UpDownConverter
@ NTV2_REGWRITE_IMMEDIATE
Register changes take effect immediately, without waiting for a field or frame VBI.
void Convert8BitYCbCrToYUY2(UByte *ycbcrBuffer, ULWord numPixels)
@ NTV2_XptFrameBuffer1_DS2YUV
NTV2HDMIBitDepth
Indicates or specifies the HDMI video bit depth.
@ NTV2_XptSDIOut4InputDS2
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
@ NTV2_FORMAT_END_UHD2_FULL_DEF_FORMATS
NTV2OutputDestination
Identifies a specific video output destination.
@ DEVICE_ID_CORVID44_2X4K
See Corvid 44 12G.
@ NTV2MIXERMODE_INVALID
Invalid/uninitialized.
@ NTV2_REFERENCE_INPUT1
Specifies the SDI In 1 connector.
double GetAudioSamplesPerSecond(const NTV2AudioRate inAudioRate)
Returns the audio sample rate as a number of audio samples per second.
NTV2AudioSource NTV2InputSourceToAudioSource(const NTV2InputSource inInputSource)
ULWord GetDisplayHeight(const NTV2VideoFormat inVideoFormat)
@ M31_FILE_3840X2160_420_8_50p
@ NTV2_AUDIOSYSTEM_7
This identifies the 7th Audio System.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ NTV2_FORMAT_4x4096x2160p_2398
#define NTV2_IS_VALID_AUDIO_CHANNEL_OCTET(__p__)
NTV2RegNumSet ToRegNumSet(const NTV2RegisterReads &inRegReads)
@ NTV2_FBF_12BIT_RGB_PACKED
See 12-Bit Packed RGB.
@ DEVICE_ID_KONA5_OE9
See KONA 5.
ULWord GetFirstActiveLine(const NTV2FieldID inRasterFieldID=NTV2_FIELD0) const
@ NTV2_REGWRITE_SYNCTOFIELD_AFTER10LINES
Register changes take effect after 10 lines after the next field VBI (not commonly used).
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_4
@ NTV2_625_2500to720p_5000
@ M31_VIF_3840X2160_420_10_50p
@ NTV2_DEVICEKIND_ANALOG
Specifies devices with analog video connectors.
@ NTV2_HDMIAudio2Channels
2 audio channels
@ NTV2_1080i2398to525_2398
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
@ NTV2_XptDualLinkIn2DSInput
@ NTV2_XptFrameBuffer2RGB
@ NTV2WidgetType_SDIIn12G
@ NTV2_XptMixer2BGKeyInput
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC, const bool inIsF2)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
@ NTV2_MAX_NUM_AudioSystemEnums
Declares a number of pixel format transcoder functions.
@ M31_FILE_3840X2160_422_8_2997p
@ NTV2WidgetType_SMPTE425Mux
@ NTV2_FG_1920x1114
1920x1080, NTV2_VANCMODE_TALLER
#define NTV2_VIDEO_FORMAT_IS_J2K_SUPPORTED(__f__)
@ NTV2_NUM_REFERENCE_INPUTS
@ NTV2_MAX_NUM_EmbeddedAudioInputs
string NTV2MixerInputControlToString(const NTV2MixerKeyerInputControl inValue, const bool inCompactDisplay)
@ NTV2IpErrSFP2NotConfigured
Declares device capability functions.
@ NTV2_FG_720x576
720x576, for PAL 625i, NTV2_VANCMODE_OFF
string NTV2MixerKeyerModeToString(const NTV2MixerKeyerMode inValue, const bool inCompactDisplay)
@ NTV2_FG_720x508
720x486, for NTSC 525i, NTV2_VANCMODE_TALL
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inSourceType)
@ NTV2_XptCompressionModule
@ NTV2_XptMixer2FGKeyInput
@ NTV2_1080i_2500to625_2500
@ NTV2_FORMAT_4096x2160p_11988
@ NTV2_XptSDIOut3InputDS2
std::set< std::string > NTV2StringSet
@ M31_VIF_720X480_420_8_60i
std::string & strip(std::string &str, const std::string &ws)
ULWord registerValue
My register value to use in a ReadRegister or WriteRegister call.
@ NTV2MIXERMODE_MIX
Overlays foreground video on top of background video.
#define AJA_NTV2_SDK_VERSION_POINT
The SDK "point" release version, an unsigned decimal integer.
@ NTV2_FBF_PRORES_HDV
Apple ProRes HDV.
@ M31_FILE_1280X720_420_8_25p
@ NTV2_FORMAT_4096x2160p_2500
@ AUTOCIRCVIDPROCMODE_KEY
#define NTV2_FBF_IS_RAW(__fbf__)
NTV2Standard GetQuarterSizedStandard(const NTV2Standard inStandard)
@ NTV2_FRAMERATE_1500
15 frames per second
@ NTV2_BITFILE_SOJI_OE4_MAIN
@ NTV2_TCINDEX_SDI2_2
SDI 2 embedded VITC 2.
std::set< NTV2TCIndex > NTV2TCIndexes
A set of distinct NTV2TCIndex values.
@ NTV2_FRAMERATE_6000
60 frames per second
#define NTV2UTILS_ENUM_CASE_RETURN_VAL_OR_ENUM_STR(condition, retail_name, enum_name)
@ NTV2_INPUTSOURCE_SDI7
Identifies the 7th SDI video input.
@ M31_FILE_720X480_420_8_5994i
std::vector< NTV2FrameRates > NTV2FrameRateFamilies
@ M31_VIF_3840X2160_422_10_30p
string NTV2InputCrosspointIDToString(const NTV2InputCrosspointID inValue, const bool inForRetailDisplay)
#define NTV2_VIDEO_FORMAT_HAS_PROGRESSIVE_PICTURE(__f__)
@ NTV2_REFERENCE_SFP2_PCR
Specifies the PCR source on SFP 2.
void ConvertARGBYCbCrToABGR(UByte *rgbaBuffer, ULWord numPixels)
@ NTV2_BITFILE_KONA5_OE6_MAIN
@ NTV2_FORMAT_525psf_2997
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
NTV2HDMIColorSpace
Indicates or specifies HDMI Color Space.
@ NTV2_FG_4x4096x2160
8192x4320, for 8K, NTV2_VANCMODE_OFF
@ NTV2WidgetType_DualLinkV2In
void Convert16BitARGBTo16BitRGB(RGBAlpha16BitPixel *rgbaLineBuffer, UWord *rgbLineBuffer, ULWord numPixels)
bool Fill8BitYCbCrVideoFrame(void *pBaseVideoAddress, const NTV2Standard inStandard, const NTV2FrameBufferFormat inFBF, const YCbCrPixel inPixelColor, const NTV2VANCMode inVancMode)
@ NTV2_525_2398to1080i_2398
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
@ NTV2_FORMAT_4x2048x1080p_4795
@ NTV2WidgetType_AnalogCompositeOut
Declares common audio macros and structs used in the SDK.
@ NTV2CROSSPOINT_CHANNEL1
string NTV2ModeToString(const NTV2Mode inValue, const bool inCompactDisplay)
@ NTV2_REFERENCE_INPUT7
Specifies the SDI In 7 connector.
@ NTV2_BITFILE_KONA5_OE11_MAIN
@ NTV2_XptDualLinkIn1Input
@ NTV2_FBF_10BIT_DPX_LE
10-Bit DPX Little-Endian
@ M31_FILE_3840X2160_420_8_2997p
static const INTERRUPT_ENUMS gChannelToInputInterrupt[]
ULWordSet NTV2RegisterNumberSet
A set of distinct ULWord values.
@ NTV2_XptSDIOut5InputDS2
@ NTV2_FORMAT_4096x2160psf_2398
static const NTV2TCIndex gChanATCLTC[]
@ M31_FILE_4096X2160_422_10_50p
@ NTV2_525_5994to525psf_2997
@ M31_FILE_1920X1080_422_10_2398p
@ NTV2_FORMAT_3840x2160p_5994_B
@ NTV2_BITFILE_KONA5_8K_MAIN
NTV2VideoFormat GetQuadSizedVideoFormat(const NTV2VideoFormat inVideoFormat, const bool isSquareDivision)
@ DEVICE_ID_KONA5
See KONA 5.
void split(const std::string &str, const char delim, std::vector< std::string > &elems)
@ M31_FILE_1920X1080_422_10_60p
@ M31_FILE_1280X720_420_8_24p
NTV2Standard GetStandardFromGeometry(const NTV2FrameGeometry inGeometry, const bool inIsProgressive)
ULWord GetByteCount(void) const
@ NTV2_AUDIOSYSTEM_4
This identifies the 4th Audio System.
@ NTV2_TCINDEX_SDI7_LTC
SDI 7 embedded ATC LTC.
#define NTV2_ASSERT(_expr_)
@ NTV2_DownConvertAnamorphic
string NTV2RegNumSetToString(const NTV2RegisterNumberSet &inObj)
@ NTV2_STANDARD_1080
Identifies SMPTE HD 1080i or 1080psf.
@ NTV2_STANDARD_3840x2160p
Identifies Ultra-High-Definition (UHD)
ULWord GetScaleFromFrameRate(const NTV2FrameRate inFrameRate)
string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay)
@ M31_FILE_3840X2160_422_8_5994p
virtual bool IsValid(void) const
@ M31_FILE_3840X2160_422_10_5994p
@ NTV2_FRAMERATE_2997
Fractional rate of 30,000 frames per 1,001 seconds.
@ NTV2_BITFILE_NUMBITFILETYPES
enum NTV2TCIndex NTV2TimecodeIndex
@ NTV2_TCINDEX_SDI8_2
SDI 8 embedded VITC 2.
@ NTV2_XptFrameBuffer3_DS2YUV
@ NTV2_VIDEOLIMITING_LEGALSDI
Identifies the "Legal SDI" mode (Ymax=0x3AC, Cmax=0x3C0)
@ NTV2_FORMAT_1080p_2K_6000_B
bool IsAlphaChannelFormat(const NTV2FrameBufferFormat format)
@ NTV2_FORMAT_4096x2160p_5000_B
@ M31_FILE_1280X720_422_10_24p
@ NTV2_FBF_RGBA
See 8-Bit ARGB, RGBA, ABGR Formats.
@ NTV2_STANDARD_625
Identifies SMPTE SD 625i.
@ NTV2_FORMAT_1080psf_2K_2398
string NTV2HDMIAudioChannelsToString(const NTV2HDMIAudioChannels inValue, const bool inCompact)
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_1
@ M31_FILE_4096X2160_420_10_5994p
@ NTV2_BITFILE_CORVID44_8K_MAIN
bool IsProgressiveTransport(const NTV2VideoFormat format)
@ NTV2_FORMAT_FIRST_4K_TSI_DEF_FORMAT
@ DEVICE_ID_IOX3
See IoX3.
@ NTV2_XptMixer3BGKeyInput
@ M31_FILE_2048X1080_422_10_5994p
static NTV2FrameRateFamilies sFRFamilies
@ NTV2_XptMultiLinkOut1Input
New in SDK 16.0.
NTV2Crosspoint GetNTV2CrosspointForIndex(const ULWord index)
@ NTV2_FG_2048x1080
2048x1080, for 2Kx1080p, NTV2_VANCMODE_OFF
bool PackLine_UWordSequenceTo10BitYUV(const UWordSequence &in16BitYUVLine, ULWord *pOut10BitYUVLine, const ULWord inNumPixels)
Packs a line of 16-bit-per-component YCbCr (NTV2_FBF_10BIT_YCBCR) video into 10-bit-per-component YCb...
@ NTV2_STANDARD_4096HFR
Identifies high frame-rate 4K.
@ NTV2_STANDARD_4096x2160p
Identifies 4K.
@ NTV2_1080i2398to525_2997
@ NTV2_BITFILE_KONA5_MAIN
@ M31_FILE_3840X2160_422_8_25p
@ NTV2_XptDualLinkIn8Input
@ NTV2_FORMAT_1080p_2K_4800_A
@ NTV2_FORMAT_4x2048x1080p_11988
@ NTV2_XptDualLinkIn5DSInput
@ NTV2_XptDuallinkOut7DS2
string PercentDecode(const string &inStr)
@ NTV2_AUDIO_FORMAT_DOLBY
@ NTV2_Wgt4KDownConverter
@ NTV2_XptFrameBuffer8YUV
@ NTV2_XptFrameBuffer4_DS2YUV
@ NTV2_XptFrameBuffer3YUV
@ NTV2_FRAMERATE_12000
120 frames per second
@ NTV2_DownConvertLetterbox
@ NTV2_FBF_48BIT_RGB
See 48-Bit RGB.
@ NTV2_OUTPUTDESTINATION_SDI2
ULWord AddAudioTestPattern(ULWord *pAudioBuffer, ULWord &inOutCurrentSample, const ULWord inNumSamples, const ULWord inModulus, const bool inEndianConvert, const ULWord inNumChannels)
std::string PrintLineNumber(const ULWord inLineOffset=0, const NTV2FieldID inRasterFieldID=NTV2_FIELD0) const
string NTV2FrameRateToString(const NTV2FrameRate inValue, const bool inForRetailDisplay)
@ NTV2_XptFrameBuffer3DS2Input
@ NTV2_AUDIO_MIC
Obtain audio samples from the device microphone input, if available.
@ DEVICE_ID_CORVID44_8KMK
See Corvid 44 12G.
@ NTV2_OUTPUTDESTINATION_SDI3
@ M31_VIF_3840X2160_422_10_5994p
@ DEVICE_ID_KONAIP_2110_RGB12
See KONA IP.
@ NTV2_BITFILE_IOIP_2110_RGB12
@ DEVICE_ID_CORVID22
See Corvid 22.
@ NTV2_FORMAT_4x1920x1080p_6000_B
@ DEVICE_ID_IOIP_2022
See Io IP.
#define NTV2_IS_VALID_TIMECODE_INDEX(__x__)
Defines a number of handy byte-swapping macros.
static bool CopyRaster20BytesPer16Pixels(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
@ NTV2_BITFILE_KONA5_8KMK_MAIN
NTV2HDMIAudioChannels
Indicates or specifies the HDMI audio channel count.
string NTV2DownConvertModeToString(const NTV2DownConvertMode inValue, const bool inCompact)
@ NTV2_MAX_NUM_BreakoutTypes
@ NTV2_FG_NUMFRAMEGEOMETRIES
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
@ NTV2_SIGNALMASK_NONE
Output Black.
@ NTV2_WgtModuleTypeCount
@ NTV2_FORMAT_4x1920x1080psf_3000
#define AJA_NTV2_SDK_BUILD_TYPE
The SDK build type, where "a"=alpha, "b"=beta, "d"=development, ""=release.
UWord NTV2DeviceGetNumAnalogVideoInputs(const NTV2DeviceID inDeviceID)
@ DEVICE_ID_CORVIDHEVC
See Corvid HEVC.
@ NTV2_BITFILE_SOJI_OE1_MAIN
@ NTV2_FBF_8BIT_HDV
See 8-Bit HDV.
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_8
@ NTV2_BreakoutCableBNC
Identifies the AES/EBU audio breakout cable that has BNC connectors.
NTV2FrameGeometry GetQuarterSizedGeometry(const NTV2FrameGeometry inGeometry)
@ NTV2_FORMAT_1080p_2K_3000
@ NTV2_BITFILE_CORVID44_8KMK_MAIN
@ NTV2_FORMAT_4096x2160psf_2997
@ NTV2_FORMAT_4x2048x1080p_4795_B
@ M31_FILE_3840X2160_420_8_30p
@ NTV2_FORMAT_4x2048x1080p_2997
NTV2FrameGeometry GetGeometryFromStandard(const NTV2Standard inStandard)
NTV2Crosspoint NTV2InputSourceToChannelSpec(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Crosspoint value.
#define NTV2_VIDEO_FORMAT_IS_B(__f__)
@ NTV2_FBF_10BIT_ARGB
10-Bit ARGB
@ AUTOCIRCVIDPROCMODE_VERTWIPE
@ M31_VIF_1920X1080_422_10_60i
@ NTV2_FBF_10BIT_YCBCRA
10-Bit YCbCrA
static const INTERRUPT_ENUMS gChannelToOutputInterrupt[]
bool IsRGBFormat(const NTV2FrameBufferFormat format)
#define NTV2_IS_VALID_AUDIO_BUFFER_SIZE(_x_)
@ DEVICE_ID_KONA5_8KMK
See KONA 5.
@ NTV2_BITFILE_IOEXPRESS_MAIN
#define NTV2_IS_2K_VIDEO_FORMAT(__f__)
@ NTV2_FORMAT_4x4096x2160p_2500
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
@ NTV2_TCINDEX_SDI4_2
SDI 4 embedded VITC 2.
@ NTV2_BITFILE_CORVID44_2X4K_MAIN
@ NTV2_XptFrameBuffer7_DS2RGB
@ NTV2_Xpt3DLUT1Input
New in SDK 16.0.
@ M31_FILE_1920X1080_420_8_25p
string NTV2AudioSourceToString(const NTV2AudioSource inValue, const bool inCompactDisplay)
@ NTV2_HDMIRangeSMPTE
Levels are 16 - 235 (SMPTE)
@ NTV2_IOKINDS_HDMI
Specifies HDMI input/output kinds.
@ NTV2_FORMAT_4x4096x2160p_4795
@ NTV2_AncRgn_MonField1
Identifies the "monitor" or "auxiliary" Field 1 ancillary data region.
@ NTV2_XptAnalogOutCompositeOut
ULWord GetVideoActiveSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode)
@ NTV2_FORMAT_4x1920x1080p_2997
@ NTV2_FORMAT_4x1920x1080p_2500
@ NTV2_AncRgn_Field2
Identifies the "normal" Field 2 ancillary data region.
@ NTV2_XptMixer3FGKeyInput
NTV2FieldID
These values are used to identify fields for interlaced video. See Field/Frame Interrupts and CNTV2Ca...
@ NTV2_FORMAT_4x2048x1080p_4800
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ NTV2_FORMAT_4x3840x2160p_2500
void ConvertLineto16BitRGB(UWord *ycbcrBuffer, RGBAlpha16BitPixel *rgbaBuffer, ULWord numPixels, bool fUseSDMatrix, bool fUseSMPTERange=false)
@ M31_VIF_3840X2160_420_8_50p
@ NTV2IpErrUllNotSupported
#define NTV2_VIDEO_FORMAT_IS_A(__f__)
@ NTV2_REFERENCE_SFP2_PTP
Specifies the PTP source on SFP 2.
Declares common video macros and structs used in the SDK.
@ NTV2_TCINDEX_SDI6
SDI 6 embedded VITC.
@ NTV2_REFERENCE_ANALOG_INPUT1
Specifies the Analog In 1 connector.
@ NTV2_FG_720x486
720x486, for NTSC 525i and 525p60, NTV2_VANCMODE_OFF
@ NTV2_525_5994to525_5994
ULWord GetIndexForNTV2CrosspointChannel(const NTV2Crosspoint channel)
@ NTV2_XptFrameBuffer1RGB
@ NTV2_FORMAT_FIRST_HIGH_DEF_FORMAT
#define NTV2UTILS_ENUM_CASE_RETURN_STR(enum_name)
string NTV2FramesizeToString(const NTV2Framesize inValue, const bool inCompactDisplay)
@ M31_VIF_1280X720_420_8_5994p
@ NTV2_XptDuallinkOut6DS2
@ NTV2_FORMAT_FIRST_UHD2_FULL_DEF_FORMAT
NTV2DeviceIDSet::const_iterator NTV2DeviceIDSetConstIter
A convenient const iterator for NTV2DeviceIDSet.
@ NTV2_AUDIOSYSTEM_8
This identifies the 8th Audio System.
#define NTV2_IS_2K_1080_VIDEO_FORMAT(__f__)
@ NTV2_FBF_10BIT_DPX
See 10-Bit RGB - DPX Format.
@ NTV2_XptSDIOut2InputDS2
#define NTV2_IS_VALID_OUTPUT_DEST(_dest_)
@ NTV2_1080p_2500to1080i_2500
@ M31_VIF_3840X2160_420_10_5994p
@ NTV2_XptFrameBuffer4Input
bool UnpackLine_10BitYUVtoU16s(vector< uint16_t > &outYCbCrLine, const NTV2Buffer &inFrameBuffer, const NTV2FormatDescriptor &inDescriptor, const UWord inLineOffset)
@ NTV2WidgetType_HDMIInV5
@ NTV2_FORMAT_4x3840x2160p_5000_B
void Make8BitBlackLine(UByte *lineData, ULWord numPixels, NTV2FrameBufferFormat fbFormat)
enum NTV2InputSourceKinds NTV2IOKinds
@ NTV2_XptSDIOut8InputDS2
bool SetRasterLinesBlack(const NTV2PixelFormat inPixelFormat, UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines)
Sets all or part of a destination raster image to legal black.
@ NTV2_XptFrameBuffer3Input
@ NTV2_INVALID_HDMI_AUDIO_CHANNELS
bool StringToSerialNum64(const string &inSerNumStr, uint64_t &outSerNum)
string NTV2UpConvertModeToString(const NTV2UpConvertMode inValue, const bool inCompact)
@ M31_FILE_720X480_420_8_60p
@ NTV2_FORMAT_4x2048x1080p_6000_B
@ NTV2_XptMultiLinkOut1DS1
New in SDK 16.0.
std::string setFromString(const std::string &inStr)
@ NTV2_XptDualLinkOut5Input
@ NTV2_SIGNALMASK_Y
Output Y if set, else Output Y=0x40.
@ NTV2_IOKINDS_ANALOG
Specifies analog input/output kinds.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
@ NTV2_XptFrameBuffer6_DS2RGB
@ DEVICE_ID_KONAIP_1RX_1TX_1SFP_J2K
See KONA IP.
@ NTV2WidgetType_Compression
NTV2FrameRate GetNTV2FrameRateFromNumeratorDenominator(const ULWord inNumerator, const ULWord inDenominator)
@ NTV2_XptMixer4BGVidInput
@ M31_FILE_2048X1080_420_8_60p
NTV2StringSet::const_iterator NTV2StringSetConstIter
@ NTV2_FORMAT_4x3840x2160p_2398
@ M31_VIF_3840X2160_420_8_30p
@ NTV2_FBF_24BIT_RGB
See 24-Bit RGB.
@ NTV2CROSSPOINT_CHANNEL5
string NTV2HDMIColorSpaceToString(const NTV2HDMIColorSpace inValue, const bool inCompact)
@ NTV2_BreakoutNone
No identifiable breakout hardware appears to be attached.
void UnPack10BitDPXtoForRP215withEndianSwap(UWord *rawrp215Buffer, ULWord *DPXLinebuffer, ULWord numPixels)
@ NTV2_FORMAT_4x4096x2160p_6000_B
@ NTV2_BITFILE_KONAIP_2TX_1SFP_J2K
@ NTV2_FIELD0
Identifies the first field in time for an interlaced video frame, or the first and only field in a pr...
@ NTV2_XptFrameBuffer3RGB
#define NTV2_IS_VALID_AUDIO_RATE(_x_)
uint8_t staticMetadataDescriptorID
@ NTV2CROSSPOINT_CHANNEL7
@ NTV2_XptFrameBuffer7DS2Input
string NTV2IsoConvertModeToString(const NTV2IsoConvertMode inValue, const bool inCompact)
@ NTV2_FRAMERATE_2500
25 frames per second
@ NTV2_BITFILE_KONA5_OE2_MAIN
NTV2HDMIRange
Indicates or specifies the HDMI RGB range.
#define CCIR601_10BIT_WHITE
@ NTV2_DEVICEKIND_HDMI
Specifies devices with HDMI connectors.
@ DEVICE_ID_IO4KUFC
See Io4K (UFC Mode).
@ M31_VIF_3840X2160_420_8_5994p
static const string AJAMacDriverInfoPlistPath("/Library/Extensions/AJANTV2.kext/Contents/Info.plist")
@ NTV2_FORMAT_1080p_2K_4795_A
bool IsNTV2CrosspointOutput(const NTV2Crosspoint inChannel)
#define NTV2_IS_TALL_VANC_GEOMETRY(__g__)
@ NTV2_FORMAT_3840x2160p_2500
@ NTV2_HDMIAudio8Channels
8 audio channels
@ NTV2_VIDEOLIMITING_LEGALBROADCAST
Identifies the "Legal Broadcast" mode (Ymax=0x340, Cmax=0x340)
@ NTV2_720p_5000to625_2500
NTV2FrameRate
Identifies a particular video frame rate.
@ NTV2_INPUTSOURCE_HDMI3
Identifies the 3rd HDMI video input.
@ DEVICE_ID_CORVID1
See Corvid, Corvid 3G.
void Make8BitLine(UByte *lineData, UByte Y, UByte Cb, UByte Cr, ULWord numPixels, NTV2FrameBufferFormat fbFormat)
bool UnpackLine_10BitYUVtoUWordSequence(const void *pIn10BitYUVLine, UWordSequence &out16BitYUVLine, ULWord inNumPixels)
Unpacks a line of NTV2_FBF_10BIT_YCBCR video into 16-bit-per-component YUV data.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
string NTV2AudioBufferSizeToString(const NTV2AudioBufferSize inValue, const bool inForRetailDisplay)
bool HasVANCGeometries(const NTV2FrameGeometry inFG)
@ M31_FILE_720X576_420_8_50p
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
uint16_t maxFrameAverageLightLevel
@ NTV2_EMBEDDED_AUDIO_INPUT_INVALID
@ M31_FILE_1920X1080_420_8_2398p
@ NTV2_1080psf_2398to1080i_5994
@ M31_FILE_3840X2160_420_10_60p
@ NTV2_FORMAT_4096x2160p_6000_B
@ M31_FILE_720X480_422_10_60p
@ NTV2_XptDualLinkIn7DSInput
@ NTV2_XptFrameBuffer6Input
bool NTV2DeviceCanDoVideoFormat(const NTV2DeviceID inDeviceID, const NTV2VideoFormat inVideoFormat)
@ NTV2_XptDualLinkIn3Input
ULWord GetNTV2FrameGeometryWidth(const NTV2FrameGeometry inGeometry)
bool NTV2DeviceCanDoFormat(const NTV2DeviceID inDeviceID, const NTV2FrameRate inFrameRate, const NTV2FrameGeometry inFrameGeometry, const NTV2Standard inStandard)
@ NTV2_FORMAT_4x4096x2160p_2997
NTV2RegisterNumberSet NTV2RegNumSet
A set of distinct NTV2RegisterNumbers.
@ NTV2_FRAMERATE_4800
48 frames per second
string NTV2RegisterNumberToString(const NTV2RegisterNumber inValue)
@ M31_VIF_720X480_422_10_5994i
void PackRGB10BitFor10BitRGB(RGBAlpha10BitPixel *pBuffer, const ULWord inNumPixels)
@ M31_FILE_2048X1080_420_8_25p
@ M31_VIF_720X480_420_8_60p
@ M31_FILE_1920X1080_422_10_50i
NTV2MixerKeyerInputControl
These enum values identify the Mixer/Keyer foreground and background input control values.
@ NTV2_HDMIProtocolDVI
DVI protocol.
@ NTV2_FORMAT_4096x2160psf_2400
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
NTV2EmbeddedAudioInput NTV2InputSourceToEmbeddedAudioInput(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2EmbeddedAudioInput value.
uint16_t minMasteringLuminance
@ NTV2_XptFrameBuffer2_DS2YUV
@ DEVICE_ID_CORVID44_8K
See Corvid 44 12G.
@ DEVICE_ID_KONAIP_2TX_1SFP_J2K
See KONA IP.
@ NTV2_XptMixer4FGVidInput
@ NTV2_REGWRITE_SYNCTOFRAME
Frame Mode: Register changes take effect at the next frame VBI (power-up default).
@ NTV2_XptDualLinkIn7Input
@ NTV2_TCINDEX_SDI5_2
SDI 5 embedded VITC 2.
string NTV2RegisterWriteModeToString(const NTV2RegisterWriteMode inValue, const bool inForRetailDisplay)
@ M31_VIF_1920X1080_420_8_60i
@ NTV2_UpConvertAnamorphic
@ M31_VIF_720X576_420_8_50i
@ NTV2WidgetType_MultiLinkOut
string NTV2EmbeddedAudioInputToString(const NTV2EmbeddedAudioInput inValue, const bool inCompactDisplay)
@ NTV2_525_5994to1080i_5994
NTV2VideoFormat GetQuarterSizedVideoFormat(const NTV2VideoFormat inVideoFormat)
@ M31_VIF_720X480_420_8_5994p
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
@ NTV2_FRAMERATE_2400
24 frames per second
NTV2DeviceIDSet NTV2GetSupportedDevices(const NTV2DeviceKinds inKinds)
Returns an NTV2DeviceIDSet of devices supported by the SDK.
NTV2EmbeddedAudioClock
This enum value determines/states the device audio clock reference source. It was important to set th...
string AutoCircVidProcModeToString(const AutoCircVidProcMode inValue, const bool inCompactDisplay)
@ NTV2_BITFILE_CORVID3G_MAIN
@ M31_FILE_3840X2160_422_10_25p
string NTV2FrameGeometryToString(const NTV2FrameGeometry inValue, const bool inForRetailDisplay)
@ M31_FILE_3840X2160_422_10_30p
@ M31_FILE_1920X1080_422_10_60i
@ NTV2_FORMAT_4x2048x1080psf_2398
@ NTV2_FBF_10BIT_RGB_PACKED
10-Bit Packed RGB
@ NTV2_BITFILE_TYPE_INVALID
@ NTV2_INPUTSOURCE_ANALOG1
Identifies the first analog video input.
@ NTV2_XptMultiLinkOut1DS2
New in SDK 16.0.
#define NTV2_IS_3Gb_FORMAT(__f__)
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
string NTV2BreakoutTypeToString(const NTV2BreakoutType inValue, const bool inCompactDisplay)
@ DEVICE_ID_KONAIP_4CH_2SFP
See KONA IP.
@ NTV2IpErrGrandMasterInfo
@ NTV2_BITFILE_KONA5_OE1_MAIN
@ NTV2_FORMAT_4x2048x1080p_2398
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
void PackLine_16BitYUVto10BitYUV(const UWord *pIn16BitYUVLine, ULWord *pOut10BitYUVLine, const ULWord inNumPixels)
Packs a line of 16-bit-per-component YCbCr (NTV2_FBF_10BIT_YCBCR) video into 10-bit-per-component YCb...
string NTV2HDMIRangeToString(const NTV2HDMIRange inValue, const bool inCompact)
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
@ NTV2_BITFILE_KONA4UFC_MAIN
@ NTV2_NUM_OUTPUTDESTINATIONS
string PercentEncode(const string &inStr)
string NTV2AudioLoopBackToString(const NTV2AudioLoopBack inValue, const bool inForRetailDisplay)
@ NTV2_XptFrameBuffer4_DS2RGB
@ NTV2_REFERENCE_HDMI_INPUT4
Specifies the HDMI In 4 connector.
NTV2GeometrySet GetRelatedGeometries(const NTV2FrameGeometry inFG)
@ M31_FILE_3840X2160_420_8_24p
@ M31_VIF_1920X1080_420_8_50p
@ NTV2_TCINDEX_SDI2_LTC
SDI 2 embedded ATC LTC.
@ NTV2_FORMAT_END_UHD2_DEF_FORMATS
@ NTV2_XptFrameBuffer1_DS2RGB
NTV2FrameGeometry GetVANCFrameGeometry(const NTV2FrameGeometry inFrameGeometry, const NTV2VANCMode inVancMode)
@ NTV2_FORMAT_1080psf_2K_2500
string NTV2WidgetTypeToString(const NTV2WidgetType inValue, const bool inCompactDisplay)
@ NTV2_OUTPUTDESTINATION_SDI1
@ NTV2_REFERENCE_INPUT5
Specifies the SDI In 5 connector.
#define NTV2_IS_FBF_RGB(__fbf__)
@ NTV2_FORMAT_4x2048x1080psf_2400
@ M31_FILE_3840X2160_422_10_2398p
@ NTV2_TCINDEX_SDI1_LTC
SDI 1 embedded ATC LTC.
@ NTV2_FBF_10BIT_RAW_RGB
10-Bit Raw RGB
@ M31_FILE_1920X1080_420_8_60p
@ NTV2_XptFrameBuffer2_DS2RGB
@ M31_VIF_1920X1080_420_8_50i
ULWord GetIndexForNTV2Crosspoint(const NTV2Crosspoint channel)
@ NTV2_XptFrameBuffer7Input
@ NTV2_XptMultiLinkOut2DS1
New in SDK 16.0.
#define NTV2_IS_VALID_AUDIO_CHANNEL_QUAD(__p__)
bool IsProgressivePicture(const NTV2VideoFormat format)
@ M31_VIF_1280X720_422_10_60p
ULWord registerNumber
My register number to use in a ReadRegister or WriteRegister call.
@ NTV2_FORMAT_1080p_5994_B
@ NTV2_XptFrameBuffer1Input
ostream & operator<<(ostream &inOutStream, const NTV2FrameDimensions inFrameDimensions)
@ NTV2_FORMAT_1080psf_3000_2
@ NTV2_TCINDEX_SDI1
SDI 1 embedded VITC.
@ NTV2_FORMAT_1080p_2K_2400
@ NTV2_TCINDEX_SDI2
SDI 2 embedded VITC.
string NTV2BitfileTypeToString(const NTV2BitfileType inValue, const bool inCompactDisplay)
@ NTV2_FG_2048x1588
2048x1556, for 2Kx1556psf film format, NTV2_VANCMODE_TALL
enum _NTV2DeviceKinds NTV2DeviceKinds
These enum values are used for device selection/filtering.
@ NTV2_EMBEDDED_AUDIO_CLOCK_REFERENCE
Audio clock derived from the device reference.
NTV2TimecodeIndex NTV2InputSourceToTimecodeIndex(const NTV2InputSource inInputSource, const bool inEmbeddedLTC)
Converts a given NTV2InputSource to its equivalent NTV2TimecodeIndex value.
@ NTV2_BITFILE_KONA5_OE4_MAIN
@ NTV2_VANCMODE_TALL
This identifies the "tall" mode in which there are some VANC lines in the frame buffer.
@ NTV2_REFERENCE_INPUT4
Specifies the SDI In 4 connector.
@ NTV2_FORMAT_4x2048x1080p_3000
@ NTV2_XptDualLinkIn2Input
@ NTV2_TCINDEX_SDI5
SDI 5 embedded VITC.
@ M31_FILE_2048X1080_422_10_60p
NTV2Standard
Identifies a particular video standard.
@ M31_VIF_3840X2160_420_10_60p
@ NTV2_DEVICEKIND_CUSTOM_ANC
Specifies devices that have custom Anc inserter/extractor firmware.
#define NTV2EndianSwap16(__val__)
@ NTV2_AUDIOSYSTEM_2
This identifies the 2nd Audio System.
@ NTV2IpErrInvalidChannel
std::ostream & Print(std::ostream &inOutStream) const
Writes a human-readable description of me into the given output stream.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
void Make10BitLine(UWord *pOutLineData, const UWord Y, const UWord Cb, const UWord Cr, const ULWord inNumPixels)
@ NTV2_FORMAT_4096x2160p_4795
@ NTV2_625_2500to1080i_2500
bool IsVideoFormatA(const NTV2VideoFormat format)
@ NTV2_1080p_2400to1080i_3000
@ NTV2_XptFrameBuffer7_DS2YUV
Declares the AJALock class.
@ NTV2_FRAMERATE_2398
Fractional rate of 24,000 frames per 1,001 seconds.
@ DEVICE_ID_KONA5_8K
See KONA 5.
@ NTV2_WgtAnalogCompositeOut1
string NTV2InputSourceToString(const NTV2InputSource inValue, const bool inForRetailDisplay)
ULWord GetIndexForNTV2Channel(const NTV2Channel inChannel)
@ NTV2IpErrInvalidMBResponseNoMac
@ DEVICE_ID_KONA3G
See KONA 3G (UFC Mode).
@ M31_FILE_1280X720_422_10_5994p
@ NTV2_FORMAT_4x4096x2160p_3000
@ NTV2_XptMultiLinkOut1InputDS2
New in SDK 16.0.
@ NTV2_FORMAT_4x2048x1080p_5000_B
ULWord NTV2FramesizeToByteCount(const NTV2Framesize inFrameSize)
Converts the given NTV2Framesize value into an exact byte count.
uint16_t maxMasteringLuminance
string NTV2AudioSystemToString(const NTV2AudioSystem inValue, const bool inCompactDisplay)
@ NTV2WidgetType_FrameSync
@ NTV2WidgetType_TestPattern
@ M31_VIF_1280X720_420_8_60p
@ NTV2WidgetType_4KDownConverter
@ DEVICE_ID_KONAXM
See KONA XM™.
@ M31_FILE_1920X1080_420_8_5994p
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
@ NTV2_XptMixer1FGKeyInput
NTV2VideoFormat GetSupportedNTV2VideoFormatFromInputVideoFormat(const NTV2VideoFormat inVideoFormat)
@ NTV2WidgetType_HDMIInV2
string NTV2AudioRateToString(const NTV2AudioRate inValue, const bool inForRetailDisplay)
@ M31_FILE_1920X1080_422_10_30p
@ DEVICE_ID_CORVID3G
See Corvid, Corvid 3G.
@ DEVICE_ID_KONAX
See KONA X.
@ NTV2_1080i_5994to525_5994
@ NTV2_TCINDEX_SDI7_2
SDI 7 embedded VITC 2.
@ NTV2_FORMAT_4x2048x1080psf_3000
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
@ M31_VIF_1920X1080_420_10_50p
@ NTV2WidgetType_AnalogOut
@ DEVICE_ID_KONA5_OE5
See KONA 5.
string NTV2TaskModeToString(const NTV2EveryFrameTaskMode inValue, const bool inCompactDisplay)
@ NTV2_625_5000to625psf_2500
@ NTV2_DEVICEKIND_12G
Specifies devices that have 12G SDI connectors.
static std::string GetDisplayName(const uint32_t inRegNum)
NTV2ReferenceSource
These enum values identify a specific source for the device's (output) reference clock.
@ NTV2_FORMAT_1080p_2K_4795_B
@ NTV2_AncRgn_Field1
Identifies the "normal" Field 1 ancillary data region.
@ NTV2_XptFrameBuffer1YUV
#define NTV2EndianSwap32(__val__)
@ DEVICE_ID_KONA4UFC
See KONA 4 (UFC Mode).
@ NTV2WidgetType_FrameStore
#define NTV2_IS_INPUT_CROSSPOINT(__x__)
@ NTV2_XptDuallinkOut2DS2
@ NTV2_FG_720x612
720x576, for PAL 625i, NTV2_VANCMODE_TALLER
@ NTV2_FRAMERATE_1498
Fractional rate of 15,000 frames per 1,001 seconds.
@ M31_FILE_1280X720_420_8_2997p
@ NTV2_XptDuallinkOut1DS2
@ NTV2_XptDuallinkOut4DS2
@ NTV2CROSSPOINT_CHANNEL2
@ NTV2_1080i_5994to720p_5994
@ NTV2_FORMAT_4x3840x2160p_5994
@ DEVICE_ID_KONAIP_1RX_1TX_2110
See KONA IP.
void RePackLineDataForYCbCrDPX(ULWord *packedycbcrLine, ULWord numULWords)
@ NTV2_FORMAT_4096x2160p_2398
std::vector< uint16_t > UWordSequence
An ordered sequence of UWord (uint16_t) values.
std::set< NTV2FrameGeometry > NTV2GeometrySet
A set of distinct NTV2FrameGeometry values.
@ M31_VIF_720X480_422_10_60p
@ NTV2_FORMAT_END_STANDARD_DEF_FORMATS
NTV2Mode
Used to identify the mode of a Frame Store, or the direction of an AutoCirculate stream: either Captu...
NTV2HDMIProtocol
Indicates or specifies the HDMI protocol.
@ NTV2_INPUTSOURCE_INVALID
The invalid video input.
@ NTV2_DEVICEKIND_4K
Specifies devices that can do 4K video.
@ M31_VIF_1920X1080_420_8_5994i
@ M31_FILE_1920X1080_420_8_30p
@ NTV2MIXERINPUTCONTROL_SHAPED
@ NTV2_XptWaterMarker1Input
@ NTV2IpErrAcquireMBTimeout
@ NTV2_MAX_NUM_VIDEO_FORMATS
@ M31_VIF_1920X1080_420_10_60p
float minMasteringLuminance
@ NTV2IpErrWriteCountToMB
@ NTV2_BITFILE_IO4KPLUS_MAIN
@ M31_VIF_720X480_422_10_5994p
@ NTV2_FORMAT_3840x2160psf_3000
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
@ NTV2_FORMAT_4x1920x1080p_5000_B
@ NTV2_XptFrameBuffer8_DS2YUV
NTV2FrameRate GetFrameRateFromScale(long scale, long duration, NTV2FrameRate playFrameRate)
@ NTV2CROSSPOINT_CHANNEL6
@ NTV2_INPUTSOURCE_SDI5
Identifies the 5th SDI video input.
std::string NTV2InterruptEnumToString(const INTERRUPT_ENUMS inInterruptEnumValue)
NTV2RegWritesConstIter NTV2RegisterReadsConstIter
@ NTV2_XptConversionModInput
@ NTV2WidgetType_HDMIInV4
@ NTV2_FORMAT_4x2048x1080p_5000
@ M31_FILE_3840X2160_420_10_50p
NTV2VideoFormat GetFirstMatchingVideoFormat(const NTV2FrameRate inFrameRate, const UWord inHeightLines, const UWord inWidthPixels, const bool inIsInterlaced, const bool inIsLevelB, const bool inIsPSF)
@ NTV2IpErrInvalidUllLevels
uint16_t maxContentLightLevel
void CopyRGBAImageToFrame(ULWord *pSrcBuffer, ULWord srcWidth, ULWord srcHeight, ULWord *pDstBuffer, ULWord dstWidth, ULWord dstHeight)
@ NTV2_FBF_24BIT_BGR
See 24-Bit BGR.
@ NTV2_OUTPUTDESTINATION_SDI6
ULWord GetIndexForNTV2CrosspointInput(const NTV2Crosspoint channel)
@ NTV2_FORMAT_FIRST_4K_DEF_FORMAT2
@ NTV2_XptConversionModule
@ NTV2_BITFILE_SOJI_DIAGS_MAIN
string NTV2HDMIProtocolToString(const NTV2HDMIProtocol inValue, const bool inCompact)
@ NTV2_HDMIColorSpaceYCbCr
YCbCr color space.
UWord NTV2DeviceGetNumVideoInputs(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumHDMIVideoOutputs(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4096x2160p_6000
@ NTV2_REFERENCE_INPUT6
Specifies the SDI In 6 connector.
@ M31_VIF_1280X720_420_8_50p
@ NTV2_FG_720x514
720x486, for NTSC 525i and 525p60, NTV2_VANCMODE_TALLER
NTV2AudioChannelPair
Identifies a pair of audio channels.
@ NTV2_1080p_3000to720p_6000
@ M31_FILE_720X480_422_10_60i
NTV2RegisterReads FromRegNumSet(const NTV2RegNumSet &inRegNumSet)
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
string NTV2CrosspointToString(const NTV2Crosspoint inChannel)
@ DEVICE_ID_TTAP_PRO
See T-Tap Pro.
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
std::set< NTV2FrameRate > NTV2FrameRates
bool CopyFrom(const void *pInSrcBuffer, const ULWord inByteCount)
Replaces my contents from the given memory buffer, resizing me to the new byte count.
@ NTV2_STANDARD_1080p
Identifies SMPTE HD 1080p.
@ NTV2_BITFILE_KONA5_OE10_MAIN
NTV2RegWrites NTV2RegisterReads
bool NTV2DeviceCanDo4KVideo(const NTV2DeviceID inDeviceID)
NTV2VideoFormat GetInputForConversionMode(const NTV2ConversionMode conversionMode)
string NTV2AudioChannelPairToString(const NTV2AudioChannelPair inValue, const bool inCompactDisplay)
void CopyFromQuadrant(uint8_t *srcBuffer, uint32_t srcHeight, uint32_t srcRowBytes, uint32_t srcQuadrant, uint8_t *dstBuffer, uint32_t quad13Offset)
@ NTV2_FORMAT_4x1920x1080p_3000
@ NTV2IpErrInvalidIGMPVersion
@ NTV2_XptFrameBuffer6_DS2YUV
#define NTV2_IS_VANCMODE_ON(__v__)
string NTV2VideoLimitingToString(const NTV2VideoLimiting inValue, const bool inCompactDisplay)
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
@ NTV2_FORMAT_4x3840x2160p_2997
@ NTV2_TCINDEX_SDI7
SDI 7 embedded VITC.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
@ NTV2_XptDualLinkOut4Input
@ NTV2MIXERINPUTCONTROL_INVALID
@ NTV2_EMBEDDED_AUDIO_CLOCK_VIDEO_INPUT
Audio clock derived from the video input.
@ NTV2_OUTPUTDESTINATION_SDI8
Declares numerous NTV2 utility functions.
@ NTV2_FORMAT_1080p_5000_B
NTV2Framesize
Kona2/Xena2 specific enums.
@ NTV2_XptFrameBuffer8DS2Input
NTV2AudioSystem NTV2InputSourceToAudioSystem(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2AudioSystem value.
@ NTV2_XptMixer2FGVidInput
@ M31_FILE_720X576_422_10_50i
@ NTV2_TCINDEX_SDI5_LTC
SDI 5 embedded ATC LTC.
static bool SetRasterLinesWhite8BitYCbCr(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines)
@ NTV2_BITFILE_KONAIP_1RX_1TX_1SFP_J2K
NTV2BreakoutType
Identifies the Breakout Boxes and Cables that may be attached to an AJA NTV2 device.
@ M31_FILE_3840X2160_422_8_2398p
NTV2FrameRates::const_iterator NTV2FrameRatesConstIter
@ NTV2_FORMAT_4x3840x2160p_5000
@ M31_FILE_1920X1080_422_10_25p
@ DEVICE_ID_KONAIP_2022
See KONA IP.
@ M31_VIF_3840X2160_422_10_60p
@ NTV2_FORMAT_1080p_2K_5994_A
#define NTV2_INPUT_SOURCE_IS_HDMI(_inpSrc_)
@ NTV2_BITFILE_KONA5_2X4K_MAIN
NTV2InputCrosspointID
Identifies a widget input that potentially can accept a signal emitted from another widget's output (...
bool IsVideoFormatB(const NTV2VideoFormat format)
@ DEVICE_ID_IOXT
See IoXT.
ULWord GetDisplayWidth(const NTV2VideoFormat inVideoFormat)
@ NTV2_XptFrameBuffer5_DS2RGB
string NTV2ColorCorrectionModeToString(const NTV2ColorCorrectionMode inValue, const bool inCompactDisplay)
@ M31_FILE_3840X2160_422_10_50p
@ NTV2MIXERMODE_FOREGROUND_ON
Passes only foreground video + key to the Mixer output.
@ NTV2_FORMAT_4x2048x1080p_12000
@ NTV2_DEVICEKIND_ALL
Specifies any/all devices.
@ NTV2_HDMIRangeFull
Levels are 0 - 255 (Full)
@ DEVICE_ID_KONA5_8K_MV_TX
See KONA 5.
bool convertHDRRegisterToFloatValues(const HDRRegValues &inRegisterValues, HDRFloatValues &outFloatValues)
@ NTV2_XptSDIOut1InputDS2
@ NTV2_AUDIO_LOOPBACK_INVALID
void setHDRDefaultsForBT2020(HDRRegValues &outRegisterValues)
#define NTV2_IS_FBF_8BIT(__fbf__)
UWord NTV2DeviceGetNumAnalogVideoOutputs(const NTV2DeviceID inDeviceID)
@ M31_FILE_3840X2160_420_8_2398p
static bool CopyRaster36BytesPer8Pixels(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
@ M31_FILE_3840X2160_422_8_60p
@ DEVICE_ID_KONA5_OE8
See KONA 5.
@ M31_FILE_4096X2160_422_10_60p_IF
@ NTV2_DEVICEKIND_INPUT
Specifies devices that input (capture).
string NTV2WidgetIDToString(const NTV2WidgetID inValue, const bool inCompactDisplay)
@ NTV2WidgetType_HDMIInV1
@ M31_FILE_1280X720_420_8_5994p
@ NTV2_BITFILE_KONA3G_MAIN
@ NTV2_XptFrameBuffer1DS2Input
@ NTV2_FBF_10BIT_YCBCR_422PL2
10-Bit 4:2:2 2-Plane YCbCr
@ NTV2_REFERENCE_HDMI_INPUT1
Specifies the HDMI In 1 connector.
void PackTo10BitYCbCrBuffer(const uint16_t *ycbcrBuffer, uint32_t *packedBuffer, const uint32_t numPixels)
@ NTV2_FORMAT_625psf_2500
@ DEVICE_ID_KONALHEPLUS
See KONA LHe Plus.
@ DEVICE_ID_KONA5_OE1
See KONA 5.
@ NTV2_INPUTSOURCE_HDMI4
Identifies the 4th HDMI video input.
@ NTV2_DEVICEKIND_EXTERNAL
Specifies external devices (e.g. Thunderbolt).
NTV2AudioLoopBack
This enum value determines/states if an audio output embedder will embed silence (zeroes) or de-embed...
#define CCIR601_10BIT_BLACK
@ M31_FILE_1920X1080_420_8_2997p
ULWord GetVaricamRepeatCount(const NTV2FrameRate inSequenceRate, const NTV2FrameRate inPlayRate, const ULWord inCadenceFrame)
NTV2Channel NTV2TimecodeIndexToChannel(const NTV2TCIndex inTCIndex)
Converts the given NTV2TCIndex value into the appropriate NTV2Channel value.
@ DEVICE_ID_CORVID88
See Corvid 88.
@ M31_FILE_2048X1080_420_8_50p
bool NTV2DeviceCanDoPlayback(const NTV2DeviceID inDeviceID)
@ NTV2_FG_1280x720
1280x720, for 720p, NTV2_VANCMODE_OFF
@ M31_FILE_3840X2160_422_8_50p
@ NTV2_FORMAT_1080p_2K_2500
@ NTV2WidgetType_HDMIOutV4
@ NTV2IpErrInvalidMBResponse
NTV2VANCMode GetVANCModeForGeometry(const NTV2FrameGeometry inFG)
@ NTV2_1080psf_2400to1080i_3000
@ NTV2_FORMAT_4x4096x2160p_4800_B
@ NTV2_FORMAT_4x1920x1080psf_2997
@ M31_FILE_2048X1080_420_8_2398p
Defines for the NTV2 SDK version number, used by ajantv2/includes/ntv2enums.h. See the ajantv2/includ...
@ NTV2_CONVERSIONMODE_UNKNOWN
@ NTV2_FORMAT_END_4K_DEF_FORMATS2
bool convertHDRFloatToRegisterValues(const HDRFloatValues &inFloatValues, HDRRegValues &outRegisterValues)
@ NTV2_FORMAT_1080p_6000_A
bool NTV2DeviceIsExternalToHost(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_3840x2160psf_2398
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
@ NTV2_FORMAT_FIRST_UHD_TSI_DEF_FORMAT
NTV2TCIndexes GetTCIndexesForSDIConnector(const NTV2Channel inSDI)
@ NTV2_TCINDEX_SDI4_LTC
SDI 4 embedded ATC LTC.
@ NTV2_Xpt4KDownConverterOutRGB
@ NTV2_BITFILE_KONALHE_PLUS
void MaskYCbCrLine(UWord *ycbcrLine, UWord signalMask, ULWord numPixels)
string NTV2TCIndexToString(const NTV2TCIndex inValue, const bool inCompactDisplay)
@ NTV2_STANDARD_TASKS
1: Standard/Retail: Device is completely controlled by AJA ControlPanel, service/daemon,...
@ NTV2_TCINDEX_SDI3_2
SDI 3 embedded VITC 2.
@ NTV2_FRAMERATE_11988
Fractional rate of 120,000 frames per 1,001 seconds.
@ NTV2_FORMAT_1080p_2K_2398
#define AJA_NTV2_SDK_VERSION_MAJOR
The SDK major version number, an unsigned decimal integer.
void ConvertLineTo8BitYCbCr(const uint16_t *ycbcr10BitBuffer, uint8_t *ycbcr8BitBuffer, const uint32_t numPixels)
uint16_t maxMasteringLuminance
@ NTV2_FORMAT_3840x2160p_2997
@ NTV2_FORMAT_4096x2160p_4800
@ M31_FILE_720X480_420_8_60i
std::vector< std::string > NTV2StringList
LWord64 GetTotalAudioSamplesFromFrameNbrZeroUpToFrameNbr(const NTV2FrameRate inFrameRate, const NTV2AudioRate inAudioRate, const ULWord inFrameNumNonInclusive)
@ NTV2_MAX_NUM_Framesizes
void Make10BitBlackLine(UWord *pOutLineData, const ULWord inNumPixels)
Writes a line of unpacked, legal SMPTE 10-bit Y/C black values into the given buffer.
@ NTV2_AncRgn_MonField2
Identifies the "monitor" or "auxiliary" Field 2 ancillary data region.
NTV2VANCMode
These enum values identify the available VANC modes.
@ NTV2_INVALID_HDMI_PROTOCOL
bool IsNTV2CrosspointInput(const NTV2Crosspoint inChannel)
@ NTV2_XptMultiLinkOut2DS2
New in SDK 16.0.
@ M31_FILE_720X480_420_8_5994p
@ NTV2_STANDARD_4096i
Identifies 4K psf.
bool GetInstalledMacDriverVersion(UWord &outMaj, UWord &outMin, UWord &outPt, UWord &outBld, UWord &outType)
@ NTV2_INVALID_HDMI_RANGE
@ NTV2_INPUT_CROSSPOINT_INVALID
@ NTV2_FORMAT_3840x2160psf_2400
static bool CopyRaster4BytesPerPixel(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
string NTV2OutputCrosspointIDToString(const NTV2OutputCrosspointID inValue, const bool inForRetailDisplay)
@ NTV2_FORMAT_1080psf_2K_2400
#define NTV2_IS_PSF_VIDEO_FORMAT(__f__)
@ M31_VIF_1280X720_422_10_50p
@ NTV2_BreakoutCableXLR
Identifies the AES/EBU audio breakout cable that has XLR connectors.
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_6
void Make8BitWhiteLine(UByte *lineData, ULWord numPixels, NTV2FrameBufferFormat fbFormat)
@ NTV2_XptFrameSync2Input
@ NTV2_XptFrameBuffer4DS2Input
@ M31_FILE_720X576_422_10_50p
NTV2Channel NTV2CrosspointToNTV2Channel(const NTV2Crosspoint inCrosspointChannel)
void ConvertLinetoRGB(UByte *ycbcrBuffer, RGBAlphaPixel *rgbaBuffer, ULWord numPixels, bool fUseSDMatrix, bool fUseSMPTERange=false)
NTV2OutputDestination NTV2ChannelToOutputDestination(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its ordinary equivalent NTV2OutputDestination.
#define NTV2_IS_VALID_CHANNEL(__x__)
@ NTV2_DISABLE_TASKS
0: Disabled: Device is completely configured by controlling application(s) – no driver involvement.
@ M31_FILE_1920X1080_420_8_24p
@ M31_FILE_1920X1080_420_8_50p
@ M31_FILE_3840X2160_422_10_24p
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
bool IsTransportCompatibleFormat(const NTV2VideoFormat inFormat1, const NTV2VideoFormat inFormat2)
@ NTV2_FORMAT_3840x2160p_5994
@ NTV2_FORMAT_4x4096x2160p_2400
@ M31_VIF_1920X1080_422_10_5994p
@ NTV2_FBF_8BIT_YCBCR_YUY2
See Alternate 8-Bit YCbCr ('YUY2').
@ M31_FILE_720X576_420_8_50i
@ NTV2WidgetType_SDIOut3G
#define CCIR601_8BIT_BLACK
@ NTV2_BITFILE_SOJI_OE2_MAIN
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
@ NTV2IpErrSoftwareMismatch
@ NTV2IpErrNoResponseFromMB
@ NTV2_INPUTSOURCE_HDMI2
Identifies the 2nd HDMI video input.
@ NTV2_FORMAT_4x4096x2160p_6000
NTV2OutputCrosspointID
Identifies a widget output, a signal source, that potentially can drive another widget's input (ident...
@ NTV2_FORMAT_3840x2160p_2398
void * GetHostPointer(void) const
@ NTV2_FORMAT_4x2048x1080p_5994_B
@ NTV2_FG_1920x1112
1920x1080, for 1080i and 1080p, NTV2_VANCMODE_TALL
@ NTV2_INVALID_HDMIBitDepth
NTV2InputSource
Identifies a specific video input source.
@ DEVICE_ID_IOIP_2110
See Io IP.
@ NTV2_1080p_2398to1080i_5994
@ DEVICE_ID_KONA5_3DLUT
See KONA 5.
@ NTV2_XptDualLinkIn4Input
string NTV2GetFirmwareFolderPath(void)
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
std::string toString(const bool inNormalized=false) const
@ NTV2_DEVICEKIND_SDI
Specifies devices with SDI connectors.
@ NTV2_BITFILE_KONA5_OE5_MAIN
@ NTV2_MAX_NUM_IsoConvertModes
void ConvertARGBToRGB(UByte *rgbaLineBuffer, UByte *rgbLineBuffer, ULWord numPixels)
@ NTV2WidgetType_SDIOut12G
NTV2Crosspoint GetNTV2CrosspointChannelForIndex(const ULWord index)
@ NTV2_FORMAT_END_4K_TSI_DEF_FORMATS
@ NTV2_IOKINDS_NONE
Doesn't specify any kind of input/output.
NTV2ConversionMode GetConversionMode(const NTV2VideoFormat inFormat, const NTV2VideoFormat outFormat)
#define NTV2_IS_QUAD_QUAD_FORMAT(__f__)
NTV2OutputCrosspointIDs::const_iterator NTV2OutputCrosspointIDsConstIter
A convenient const iterator for NTV2OutputCrosspointIDs.
@ NTV2_Xpt4KDownConverterOut
@ NTV2_AUDIO_AES
Obtain audio samples from the device AES inputs, if available.
@ NTV2_FORMAT_4x1920x1080psf_2398
@ NTV2_FG_FIRST
The ordinally first geometry (New in SDK 16.0)
bool YUVComponentsTo10BitYUVPackedBuffer(const vector< uint16_t > &inYCbCrLine, NTV2Buffer &inFrameBuffer, const NTV2FormatDescriptor &inDescriptor, const UWord inLineOffset)
string NTV2OutputDestinationToString(const NTV2OutputDestination inValue, const bool inForRetailDisplay)
@ M31_FILE_4096X2160_420_10_60p
NTV2FrameGeometry GetNormalizedFrameGeometry(const NTV2FrameGeometry inFrameGeometry)
@ NTV2_AUDIO_HDMI
Obtain audio samples from the device HDMI input, if available.
bool IsYCbCrFormat(const NTV2FrameBufferFormat format)
@ NTV2_FORMAT_1080p_2K_6000_A
@ NTV2_XptFrameBuffer8RGB
NTV2Channel NTV2OutputDestinationToChannel(const NTV2OutputDestination inOutputDest)
Converts a given NTV2OutputDestination to its equivalent NTV2Channel value.
@ NTV2_XptMixer1FGVidInput
static const char * m31Presets[M31_NUMVIDEOPRESETS]
@ NTV2_FG_720x598
720x576, for PAL 625i, NTV2_VANCMODE_TALL
@ NTV2_BITFILE_KONAIP_2022
@ NTV2_BITFILE_IO4KUFC_MAIN
#define AJA_NTV2_SDK_BUILD_NUMBER
The SDK build number, an unsigned decimal integer.
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
@ NTV2_TCINDEX_SDI1_2
SDI 1 embedded VITC 2.
void PackRGB10BitFor10BitDPX(RGBAlpha10BitPixel *pBuffer, const ULWord inNumPixels, const bool bigEndian=true)
@ NTV2_XptFrameBuffer8Input
@ NTV2_1080i_2398to720p_2398
@ M31_VIF_720X480_422_10_60i
@ NTV2_FBF_PRORES_DVCPRO
Apple ProRes DVC Pro.
@ NTV2_XptMixer4FGKeyInput
void Fill4k8BitYCbCrVideoFrame(PULWord _baseVideoAddress, NTV2FrameBufferFormat frameBufferFormat, YCbCrPixel color, bool vancEnabled, bool b4k, bool wideVANC)
@ M31_FILE_1280X720_422_10_50p
@ NTV2_XptStereoRightInput
@ NTV2_VANCMODE_TALLER
This identifies the mode in which there are some + extra VANC lines in the frame buffer.
NTV2Standard Get4xSizedStandard(const NTV2Standard inStandard, const bool bIs4k)
@ M31_FILE_1280X720_422_10_30p
@ NTV2IpErrInvalidBitdepth
Used to describe Start of Active Video (SAV) location and field dominance for a given NTV2Standard....
bool NTV2DeviceHasSDIRelays(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4x1920x1080p_5994_B
@ NTV2_TCINDEX_SDI3
SDI 3 embedded VITC.
@ NTV2_DEVICEKIND_SFP
Specifies devices with SFP connectors.
@ NTV2_XptFrameSync1Input
#define NTV2_NUMAUDIO_CHANNELS
@ NTV2_HDMIProtocolHDMI
HDMI protocol.
@ NTV2_FORMAT_3840x2160p_5000_B
@ NTV2_FORMAT_4096x2160p_3000
void UnPack10BitDPXtoRGBAlpha10BitPixel(RGBAlpha10BitPixel *rgba10BitBuffer, const ULWord *DPXLinebuffer, ULWord numPixels, bool bigEndian)
@ M31_FILE_3840X2160_422_8_30p
ULWord GetIndexForNTV2InputSource(const NTV2InputSource inValue)
NTV2FrameGeometry GetNTV2FrameGeometryFromVideoFormat(const NTV2VideoFormat inVideoFormat)
NTV2FrameGeometry GetGeometryFromFrameDimensions(const NTV2FrameDimensions &inFD)
@ NTV2_1080psf_2500to1080i_2500
@ NTV2_XptFrameBuffer6RGB
void StackQuadrants(uint8_t *pSrc, uint32_t srcWidth, uint32_t srcHeight, uint32_t srcRowBytes, uint8_t *pDst)
@ NTV2_XptDualLinkIn6DSInput
@ M31_FILE_1920X1080_422_10_50p
@ NTV2_XptFrameBuffer2Input
NTV2VideoLimiting
These enum values identify the available SDI video output limiting modes.
@ NTV2_AUDIOSYSTEM_3
This identifies the 3rd Audio System.
@ NTV2CROSSPOINT_CHANNEL3
@ NTV2_SIGNALMASK_Cb
Output Cb if set, elso Output Cb to 0x200.
@ NTV2_FORMAT_4x1920x1080psf_2500
@ NTV2_FORMAT_END_2K_DEF_FORMATS
@ NTV2_1080i_2500to720p_5000
NTV2Channel GetNTV2ChannelForIndex(const ULWord inIndex)
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_7
@ NTV2_FRAMERATE_5994
Fractional rate of 60,000 frames per 1,001 seconds.
@ NTV2_OUTPUTDESTINATION_HDMI
@ NTV2_XptDualLinkIn8DSInput
@ NTV2_XptFrameBuffer4RGB
bool NTV2DeviceCanDoIP(const NTV2DeviceID inDeviceID)
NTV2FrameGeometry Get4xSizedGeometry(const NTV2FrameGeometry inGeometry)
Private include file for all ajabase sources.
@ DEVICE_ID_CORVID24
See Corvid 24.
@ NTV2_FORMAT_4x3840x2160p_2400
@ NTV2_XptMixer1BGVidInput
@ NTV2_VIDEOLIMITING_INVALID
@ NTV2_STANDARD_525
Identifies SMPTE SD 525i.
@ NTV2_FORMAT_4x4096x2160p_5000_B
@ M31_VIF_720X576_420_8_50p
static bool SetRasterLinesBlack10BitYCbCr(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines)
@ NTV2_UpConvertPillarbox4x3
@ NTV2_FBF_10BIT_RAW_YCBCR
See 10-Bit Raw YCbCr (CION).
@ DEVICE_ID_KONA1
See KONA 1.
bool IsVideoFormatJ2KSupported(const NTV2VideoFormat format)
INTERRUPT_ENUMS NTV2ChannelToOutputInterrupt(const NTV2Channel inChannel)
Converts the given NTV2Channel value into the equivalent output INTERRUPT_ENUMS value.
@ NTV2_XptMultiLinkOut2InputDS2
New in SDK 16.0.
@ NTV2_XptMixer3BGVidInput
@ NTV2_DEVICEKIND_6G
Specifies devices that have 6G SDI connectors.
bool Is2KFormat(const NTV2VideoFormat format)
#define HEX0N(__x__, __n__)
@ NTV2_OUTPUTDESTINATION_INVALID
std::vector< NTV2OutputCrosspointID > NTV2OutputCrosspointIDs
An ordered sequence of NTV2OutputCrosspointID values.
@ M31_FILE_2048X1080_420_8_30p
void ConvertARGBToBGR(const UByte *pInRGBALineBuffer, UByte *pOutRGBLineBuffer, const ULWord inNumPixels)
@ NTV2_BITFILE_KONA5_OE12_MAIN
@ NTV2_AUDIO_LOOPBACK_ON
Embeds SDI input source audio into the data stream.
@ NTV2_FORMAT_4x2048x1080p_2500
static bool CopyRaster16BytesPer6Pixels(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
void Make10BitWhiteLine(UWord *pOutLineData, const ULWord inNumPixels)
Writes a line of unpacked, legal SMPTE 10-bit Y/C white values into the given buffer.
@ NTV2_FORMAT_4x1920x1080p_5994
static UByte * GetWriteAddress_2vuy(UByte *pInFrameBuffer, const ULWord inBytesPerVertLine, const UWord inVertLineOffset, const UWord inHorzPixelOffset, const UWord inBytesPerHorzPixel)
@ NTV2_FORMAT_4x4096x2160p_5000
NTV2RegisterWriteMode
These values are used to determine when certain register writes actually take effect....
@ M31_VIF_1920X1080_420_10_5994p
@ M31_FILE_4096X2160_422_10_5994p_IF
@ NTV2_BITFILE_CORVID24_MAIN
@ M31_VIF_1920X1080_420_8_60p
@ NTV2_MODE_CAPTURE
Capture (input) mode, which writes into device SDRAM.
@ DEVICE_ID_IO4KPLUS
See Io4K Plus.
@ NTV2_XptStereoCompressorOut
@ NTV2_INPUTSOURCE_SDI8
Identifies the 8th SDI video input.
@ NTV2_FORMAT_4x3840x2160p_6000
@ NTV2_BITFILE_CORVIDHEVC
@ NTV2_FORMAT_4x2048x1080p_4800_B
@ NTV2MIXERINPUTCONTROL_FULLRASTER
@ NTV2_XptCompressionModInput
@ NTV2_XptDualLinkOut3Input
@ NTV2_STANDARD_3840HFR
Identifies high frame-rate UHD.
@ NTV2_FBF_10BIT_YCBCR_DPX
See 10-Bit YCbCr - DPX Format.
@ NTV2_BITFILE_KONA5_OE9_MAIN
@ NTV2_525_5994to720p_5994
@ NTV2_720p_6000to1080i_3000
@ M31_FILE_1280X720_422_10_2997p
@ NTV2_INPUTSOURCE_SDI2
Identifies the 2nd SDI video input.
@ NTV2IpErrInvalidMBResponseSize
@ NTV2_XptFrameBuffer2YUV
#define NTV2_IS_4K_QUADHD_VIDEO_FORMAT(__f__)
bool GetChangedRegisters(const NTV2RegisterReads &inBefore, const NTV2RegisterReads &inAfter, NTV2RegNumSet &outChanged)
@ DEVICE_ID_CORVID44
See Corvid 44.
@ M31_FILE_1280X720_422_10_2398p
@ NTV2_FBF_10BIT_YCBCR_422PL3_LE
See 3-Plane 10-Bit YCbCr 4:2:2 ('I422_10LE' a.k.a. 'YUV-P-L10').
@ M31_FILE_2048X1080_420_8_2997p
@ NTV2_BITFILE_KONA5_OE8_MAIN
@ NTV2_TCINDEX_LTC2
Analog LTC 2.
string NTV2StandardToString(const NTV2Standard inValue, const bool inForRetailDisplay)
@ NTV2_XptFrameBuffer5RGB
string NTV2ReferenceSourceToString(const NTV2ReferenceSource inValue, const bool inForRetailDisplay)
string NTV2VANCModeToString(const NTV2VANCMode inValue, const bool inCompactDisplay)
@ NTV2_XptFrameBuffer5DS2Input
void Convert16BitARGBTo12BitRGBPacked(RGBAlpha16BitPixel *rgbaLineBuffer, UByte *rgbLineBuffer, ULWord numPixels)
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
@ NTV2_XptFrameBuffer5_DS2YUV
@ M31_FILE_1280X720_420_8_2398p
@ NTV2WidgetType_HDMIInV3
@ NTV2_STANDARD_2Kx1080i
Identifies SMPTE HD 2K1080psf.
@ NTV2_FRAMERATE_UNKNOWN
Represents an unknown or invalid frame rate.
@ NTV2_XptFrameBuffer7YUV
INTERRUPT_ENUMS NTV2ChannelToInputInterrupt(const NTV2Channel inChannel)
Converts the given NTV2Channel value into the equivalent input INTERRUPT_ENUMS value.
#define NTV2_IS_VALID_FIELD(__x__)
@ NTV2_XptDualLinkIn5Input
@ NTV2_FORMAT_1080p_5000_A
@ DEVICE_ID_KONA4
See KONA 4 (Quad Mode).
@ NTV2_FORMAT_4x2048x1080p_6000
int RecordCopyAudio(PULWord pAja, PULWord pSR, int iStartSample, int iNumBytes, int iChan0, int iNumChans, bool bKeepAudio24Bits)
@ M31_VIF_720X576_422_10_50p
#define CCIR601_8BIT_CHROMAOFFSET
@ NTV2_FORMAT_3840x2160psf_2997
@ M31_FILE_2048X1080_422_10_30p
@ NTV2_XptMixer2BGVidInput
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ NTV2_STANDARD_8192
Identifies 8K.
#define CCIR601_10BIT_CHROMAOFFSET
@ NTV2_FORMAT_4096x2160p_5000
@ NTV2_MAX_NUM_AudioBufferSizes
@ NTV2_TCINDEX_DEFAULT
The "default" timecode (mostly used by the AJA "Retail" service and Control Panel)
string NTV2AudioFormatToString(const NTV2AudioFormat inValue, const bool inCompact)
double GetFramesPerSecond(const NTV2FrameRate inFrameRate)
@ NTV2_TCINDEX_SDI6_2
SDI 6 embedded VITC 2.
void UnPack10BitYCbCrBuffer(uint32_t *packedBuffer, uint16_t *ycbcrBuffer, uint32_t numPixels)
@ NTV2WidgetType_WaterMarker
@ NTV2_XptDuallinkOut5DS2
@ NTV2_XptDualLinkIn6Input
NTV2InputSource GetNTV2HDMIInputSourceForIndex(const ULWord inIndex0)
@ NTV2_BITFILE_SOJI_OE5_MAIN
ULWord GetAudioSamplesPerFrame(const NTV2FrameRate inFrameRate, const NTV2AudioRate inAudioRate, ULWord inCadenceFrame, const bool inIsSMPTE372Enabled)
Returns the number of audio samples for a given video frame rate, audio sample rate,...
@ NTV2_BITFILE_CORVID1_MAIN
@ NTV2_FORMAT_4x1920x1080p_5000
@ M31_FILE_720X480_422_10_5994p
@ NTV2_FBF_8BIT_YCBCR_420PL3
See 3-Plane 8-Bit YCbCr 4:2:0 ('I420' a.k.a. 'YUV-P420').
@ NTV2_BITFILE_KONA4_MAIN
@ NTV2_STANDARD_720
Identifies SMPTE HD 720p.
string NTV2EmbeddedAudioClockToString(const NTV2EmbeddedAudioClock inValue, const bool inForRetailDisplay)
@ NTV2_FORMAT_4x1920x1080p_2398
string NTV2ChannelToString(const NTV2Channel inValue, const bool inForRetailDisplay)
@ NTV2_FG_2048x1112
2048x1080, for 2Kx1080p, NTV2_VANCMODE_TALL
@ DEVICE_ID_TTAP
See T-Tap.
@ NTV2_XptDualLinkOut6Input
NTV2Crosspoint GetNTV2CrosspointInputForIndex(const ULWord index)
void setHDRDefaultsForDCIP3(HDRRegValues &outRegisterValues)
NTV2FrameGeometry
Identifies a particular video frame geometry.
@ M31_VIF_720X576_422_10_50i
@ NTV2_XptDualLinkOut2Input
@ NTV2_FORMAT_1080p_6000_B
@ NTV2_FORMAT_4x4096x2160p_5994_B
@ NTV2_IOKINDS_ALL
Specifies any/all input/output kinds.
@ NTV2_XptCSC1KeyFromInput2
@ DEVICE_ID_KONA5_OE3
See KONA 5.
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
std::set< NTV2DeviceID > NTV2DeviceIDSet
A set of NTV2DeviceIDs.
@ NTV2_FORMAT_4096x2160p_2997
@ NTV2_REGWRITE_SYNCTOFIELD
Field Mode: Register changes take effect at the next field VBI.
@ M31_FILE_1280X720_422_10_60p
@ NTV2_XptDualLinkIn4DSInput
@ NTV2_XptFrameBuffer6DS2Input
@ NTV2_TCINDEX_SDI3_LTC
SDI 3 embedded ATC LTC.
@ NTV2_OUTPUTDESTINATION_SDI7
@ NTV2_BITFILE_LHI_T_MAIN
@ NTV2_REFERENCE_INPUT2
Specifies the SDI In 2 connector.
@ NTV2_FBF_8BIT_DVCPRO
See 8-Bit DVCPro.
@ NTV2_FORMAT_4096x2160p_5994_B
@ NTV2_FORMAT_4x3840x2160p_5994_B
@ NTV2WidgetType_AnalogIn
@ DEVICE_ID_KONA5_OE7
See KONA 5.
@ NTV2_WgtUpDownConverter2
@ NTV2_FORMAT_1080p_5994_A
@ NTV2_FORMAT_4x3840x2160p_6000_B
@ NTV2_FG_2048x1114
2048x1080, NTV2_VANCMODE_TALLER
ULWord GetNTV2FrameGeometryHeight(const NTV2FrameGeometry inGeometry)
@ NTV2_FBF_8BIT_YCBCR_422PL2
8-Bit 4:2:2 2-Plane YCbCr
@ NTV2_720p_2398to1080i_2398
@ NTV2_BITFILE_CORVID22_MAIN
@ NTV2_FORMAT_END_HIGH_DEF_FORMATS2
@ DEVICE_ID_KONA5_OE10
See KONA 5.
NTV2Audio4ChannelSelect
Identifies a contiguous, adjacent group of four audio channels.
@ NTV2_FORMAT_4x2048x1080p_5994
@ NTV2_OUTPUTDESTINATION_SDI4
@ M31_VIF_720X480_420_8_5994i
@ M31_FILE_1920X1080_422_10_2997p
static const NTV2TCIndex gChanVITC1[]
NTV2StringList::const_iterator NTV2StringListConstIter
@ M31_VIF_1920X1080_422_10_5994i
@ NTV2IpErrInvalidUllHeight
@ NTV2WidgetType_HDMIOutV3
static bool CheckFrameRateFamiliesInitialized(void)
@ NTV2_DEVICEKIND_NONE
Doesn't specify any kind of device.
@ M31_FILE_1280X720_422_10_25p
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat videoFormat)
@ M31_VIF_1280X720_422_10_5994p
@ NTV2_BITFILE_TTAP_PRO_MAIN
@ NTV2_FG_4x1920x1080
3840x2160, for UHD, NTV2_VANCMODE_OFF
@ NTV2_XptFrameBuffer5Input
@ NTV2WidgetType_StereoCompressor
@ NTV2_FORMAT_4x3840x2160p_3000
#define AJA_NTV2_SDK_VERSION_MINOR
The SDK minor version number, an unsigned decimal integer.
@ NTV2_FORMAT_1080p_2K_5994_B
@ NTV2_AUDIOSYSTEM_5
This identifies the 5th Audio System.
@ NTV2_INVALID_HDMI_COLORSPACE
@ M31_FILE_3840X2160_422_10_2997p
@ M31_VIF_1920X1080_420_10_5994i
@ NTV2WidgetType_DualLinkV2Out
@ NTV2_BITFILE_KONA3G_QUAD
@ NTV2_FBF_16BIT_ARGB
16-Bit ARGB
string NTV2VideoFormatToString(const NTV2VideoFormat inFormat, const bool inUseFrameRate)
#define NTV2_IS_4K_4096_VIDEO_FORMAT(__f__)
@ NTV2_MODE_INVALID
The invalid mode.
@ NTV2_MAX_NUM_UpConvertModes
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
enum NTV2AncillaryDataRegion NTV2AncDataRgn
NTV2Crosspoint NTV2ChannelToInputCrosspoint(const NTV2Channel inChannel)
#define NTV2_IS_VANCMODE_TALL(__v__)
@ NTV2_IOKINDS_SDI
Specifies SDI input/output kinds.
static bool SetRasterLinesWhite10BitYCbCr(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines)
@ M31_FILE_1920X1080_422_10_5994i
bool Fill(const T &inValue)
Fills me with the given scalar value.
@ NTV2_FBF_8BIT_YCBCR_422PL3
See 3-Plane 8-Bit YCbCr 4:2:2 (Weitek 'Y42B' a.k.a. 'YUV-P8').
@ DEVICE_ID_KONA3GQUAD
See KONA 3G (Quad Mode).
@ M31_FILE_3840X2160_422_10_60p
@ DEVICE_ID_CORVIDHBR
See Corvid HB-R.
bool CopyRaster(const NTV2PixelFormat inPixelFormat, UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
Copies all or part of a source raster image into a destination raster at a given position.
ULWord GetVideoWriteSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode)
Identical to the GetVideoActiveSize function, except rounds the result up to the nearest 4K page size...
@ NTV2_TCINDEX_SDI8
SDI 8 embedded VITC.
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
@ NTV2_HDMIColorSpaceRGB
RGB color space.
@ M31_FILE_2048X1080_422_10_2398p
@ NTV2_FORMAT_3840x2160p_5000
@ M31_FILE_1920X1080_420_8_60i
@ NTV2_XptFrameBuffer3_DS2RGB
#define NTV2_IS_OUTPUT_CROSSPOINT(__x__)
bool Is8BitFrameBufferFormat(const NTV2FrameBufferFormat format)
@ NTV2_BITFILE_KONAIP_1RX_1TX_2110
@ NTV2_FORMAT_4096x2160psf_3000
@ NTV2_XptSDIOut7InputDS2
bool NTV2DeviceCanDoCustomAnc(const NTV2DeviceID inDeviceID)
@ NTV2_BITFILE_SOJI_3DLUT_MAIN
@ NTV2IpErrSFP1NotConfigured
@ NTV2WidgetType_SDIMonOut
@ NTV2_AUDIO_ANALOG
Obtain audio samples from the device analog input(s), if available.
@ NTV2_XptWaterMarker2Input
@ M31_FILE_3840X2160_422_8_24p
@ NTV2_FG_1280x740
1280x720, for 720p, NTV2_VANCMODE_TALL
@ NTV2WidgetType_DualLinkV1Out
@ NTV2_FORMAT_1080p_2K_5000_A
@ NTV2_AUDIO_BUFFER_STANDARD
@ NTV2_TCINDEX_SDI8_LTC
SDI 8 embedded ATC LTC.
@ NTV2_FRAMERATE_5000
50 frames per second
void ConvertARGBYCbCrToRGBA(UByte *rgbaBuffer, ULWord numPixels)
@ NTV2_FORMAT_4x4096x2160p_4795_B
@ NTV2_XptMultiLinkOut1DS4
New in SDK 16.0.
@ NTV2_TCINDEX_SDI6_LTC
SDI 6 embedded ATC LTC.
@ NTV2_AUDIO_FORMAT_INVALID
std::vector< NTV2DeviceID > NTV2DeviceIDList
An ordered list of NTV2DeviceIDs.
#define CCIR601_8BIT_WHITE
@ NTV2_FORMAT_4x2048x1080psf_2500
@ M31_FILE_2048X1080_422_10_2997p
@ NTV2_FBF_10BIT_RGB
See 10-Bit RGB Format.
@ NTV2_625_2500to625_2500
@ DEVICE_ID_KONA5_OE11
See KONA 5.
@ NTV2_INPUTSOURCE_SDI3
Identifies the 3rd SDI video input.
NTV2FrameRateFamilies::const_iterator NTV2FrameRateFamiliesConstIter
@ NTV2_FG_2048x1556
2048x1556, for 2Kx1556psf film format, NTV2_VANCMODE_OFF
@ M31_VIF_1920X1080_420_8_5994p
string SerialNum64ToString(const uint64_t &inSerNum)
@ NTV2_BITFILE_KONA5_8K_MV_TX_MAIN
@ NTV2_REFERENCE_EXTERNAL
Specifies the External Reference connector.
std::string NTV2GetVersionString(const bool inDetailed)
@ NTV2_FRAMERATE_4795
Fractional rate of 48,000 frames per 1,001 seconds.
NTV2EmbeddedAudioInput NTV2ChannelToEmbeddedAudioInput(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2EmbeddedAudioInput.
string NTV2AncDataRgnToStr(const NTV2AncDataRgn inValue, const bool inCompactDisplay)
@ NTV2_XptMultiLinkOut2DS4
New in SDK 16.0.
@ M31_FILE_2048X1080_422_10_25p
NTV2DeviceID NTV2GetDeviceIDFromBitfileName(const string &inBitfileName)
NTV2EmbeddedAudioInput
This enum value determines/states which SDI video input will be used to supply audio samples to an au...
@ NTV2_FRAMERATE_3000
30 frames per second
@ AUTOCIRCVIDPROCMODE_INVALID
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay)
bool Is8KFormat(const NTV2VideoFormat format)
static bool CopyRaster3BytesPerPixel(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
@ M31_FILE_720X480_422_10_5994i
uint16_t maxFrameAverageLightLevel
#define NTV2_IS_VALID_AUDIO_CHANNEL_PAIR(__p__)
@ M31_VIF_1920X1080_420_10_60i
uint32_t CalcRowBytesForFormat(const NTV2FrameBufferFormat inPixelFormat, const uint32_t inPixelWidth)
bool SetRasterLinesWhite(const NTV2PixelFormat inPixelFormat, UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines)
Sets all or part of a destination raster image to legal white.
@ M31_FILE_1280X720_420_8_50p
bool Is4KFormat(const NTV2VideoFormat format)
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
@ NTV2_FORMAT_1080p_2K_5000_B
@ NTV2_AUDIO_RATE_INVALID
@ NTV2_XptFrameBuffer5YUV
@ AUTOCIRCVIDPROCMODE_MIX
#define NTV2_IS_SUPPORTED_NTV2FrameRate(__r__)
@ M31_FILE_1920X1080_422_10_5994p
@ DEVICE_ID_IOIP_2110_RGB12
See Io IP.
#define NTV2_IS_VALID_AUDIO_SYSTEM(__x__)
@ NTV2_FG_4x3840x2160
7680x4320, for UHD2, NTV2_VANCMODE_OFF
@ NTV2_FORMAT_FIRST_4K_DEF_FORMAT
@ DEVICE_ID_KONA5_2X4K
See KONA 5.
@ M31_FILE_2048X1080_422_10_24p
static bool SetRasterLinesBlack8BitYCbCr(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines)
@ M31_VIF_1920X1080_420_10_50i
@ NTV2_XptMixer1BGKeyInput
@ NTV2_FORMAT_1080psf_2997_2
@ NTV2_REFERENCE_INPUT8
Specifies the SDI In 8 connector.
@ NTV2_TCINDEX_LTC1
Analog LTC 1.
#define NTV2_INPUT_SOURCE_IS_ANALOG(_inpSrc_)
#define NTV2_IS_VALID_VANCMODE(__v__)
@ NTV2_FORMAT_4x4096x2160p_5994
@ NTV2_FORMAT_4x2048x1080p_2400
@ NTV2_EMBEDDED_AUDIO_CLOCK_INVALID
@ NTV2_AUDIOSYSTEM_6
This identifies the 6th Audio System.
@ NTV2IpErrTimeoutNoBytecount
NTV2MixerKeyerMode
These enum values identify the mixer mode.
@ M31_FILE_1920X1080_420_8_5994i
NTV2Audio8ChannelSelect
Identifies a contiguous, adjacent group of eight audio channels.
@ NTV2_BITFILE_KONA5_OE7_MAIN
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
@ NTV2_XptMultiLinkOut1DS3
New in SDK 16.0.
@ NTV2_FORMAT_1080p_2K_4800_B
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_2
@ NTV2_REFERENCE_HDMI_INPUT3
Specifies the HDMI In 3 connector.
@ DEVICE_ID_KONA5_OE2
See KONA 5.
void MakeUnPacked10BitYCbCrBuffer(uint16_t *buffer, uint16_t Y, uint16_t Cb, uint16_t Cr, uint32_t numPixels)
@ NTV2_XptFrameBuffer2DS2Input
NTV2AudioSource
This enum value determines/states where an audio system will obtain its audio samples.
@ NTV2_STANDARD_7680
Identifies UHD2.
void ConvertLineto10BitRGB(UWord *ycbcrBuffer, RGBAlpha10BitPixel *rgbaBuffer, ULWord numPixels, bool fUseSDMatrix, bool fUseSMPTERange=false)
@ NTV2_FORMAT_4x1920x1080p_2400
Declares the CNTV2RegisterExpert class.
bool IsPSF(const NTV2VideoFormat format)
@ NTV2_FORMAT_4x1920x1080psf_2400
@ M31_FILE_3840X2160_420_8_25p
@ NTV2_FG_1920x1080
1920x1080, for 1080i and 1080p, NTV2_VANCMODE_OFF
@ NTV2_OUTPUTDESTINATION_ANALOG
@ NTV2_HDMIColorSpaceAuto
Automatic (not for OEM use)
NTV2Crosspoint
Logically, these are an NTV2Channel combined with an NTV2Mode.
@ NTV2_FORMAT_3840x2160p_6000_B
@ NTV2_XptFrameBuffer7RGB
NTV2VideoFormat GetOutputForConversionMode(const NTV2ConversionMode conversionMode)
#define NTV2_AUDIOSAMPLESIZE
@ NTV2_BITFILE_KONAIP_2110_RGB12
string NTV2AudioChannelOctetToString(const NTV2Audio8ChannelSelect inValue, const bool inCompactDisplay)
@ NTV2_FORMAT_1080psf_2500_2
@ DEVICE_ID_IO4K
See Io4K (Quad Mode).
@ NTV2_BITFILE_SOJI_OE3_MAIN
NTV2InputSource GetNTV2InputSourceForIndex(const ULWord inIndex0, const NTV2IOKinds inKinds)
@ NTV2_FORMAT_4096x2160p_4795_B
@ NTV2_720p_5994to525_5994
@ NTV2_XptWaterMarker2RGB
uint8_t staticMetadataDescriptorID
@ DEVICE_ID_KONALHI
See KONA LHi.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
void MaskUnPacked10BitYCbCrBuffer(uint16_t *ycbcrUnPackedBuffer, uint16_t signalMask, uint32_t numPixels)
@ NTV2_WgtUpDownConverter1
@ NTV2_FORMAT_4096x2160p_4800_B
@ NTV2_FORMAT_3840x2160p_2400
void ConvertUnpacked10BitYCbCrToPixelFormat(uint16_t *unPackedBuffer, uint32_t *packedBuffer, uint32_t numPixels, NTV2FrameBufferFormat pixelFormat, bool bUseSmpteRange, bool bAlphaFromLuma)
static AJALock sFRFamMutex
@ M31_VIF_1920X1080_422_10_60p
NTV2AudioBufferSize
Represents the size of the audio buffer used by a device audio system for storing captured samples or...
@ M31_FILE_2048X1080_420_8_24p
@ DEVICE_ID_KONA5_OE4
See KONA 5.
@ NTV2_AncRgn_All
Identifies "all" ancillary data regions.
uint8_t electroOpticalTransferFunction
@ NTV2_FBF_ABGR
See 8-Bit ARGB, RGBA, ABGR Formats.
@ NTV2_AUDIO_SOURCE_INVALID
bool GetRegNumChanges(const NTV2RegNumSet &inBefore, const NTV2RegNumSet &inAfter, NTV2RegNumSet &outGone, NTV2RegNumSet &outSame, NTV2RegNumSet &outNew)
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
@ M31_FILE_3840X2160_420_10_5994p
#define NTV2_IS_VALID_VIDEO_FORMAT(__f__)
@ NTV2_OUTPUTDESTINATION_SDI5
@ NTV2_720p_5000to1080i_2500
@ NTV2_STANDARD_3840i
Identifies Ultra-High-Definition (UHD) psf.
NTV2Crosspoint NTV2ChannelToOutputCrosspoint(const NTV2Channel inChannel)
@ M31_VIF_3840X2160_420_8_60p
@ NTV2_1080i_6000to1080psf_3000
ULWord NTV2AudioBufferSizeToByteCount(const NTV2AudioBufferSize inBufferSize)
Converts the given NTV2BufferSize value into its exact byte count.
@ NTV2_XptSDIOut6InputDS2
@ NTV2_FORMAT_3840x2160p_3000
@ NTV2MIXERMODE_FOREGROUND_OFF
Passes only background video + key to the Mixer output.
@ NTV2_FORMAT_1080psf_2400
#define NTV2_IS_PROGRESSIVE_STANDARD(__s__)
@ NTV2WidgetType_HDMIOutV1
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
UWord NTV2GetSDKVersionComponent(const int inVersionComponent)
Returns an SDK version component value.
@ NTV2_XptDualLinkIn1DSInput
@ NTV2_BITFILE_SOJI_OE6_MAIN
@ NTV2_BITFILE_CORVID44_PLNR_MAIN
@ M31_FILE_2048X1080_420_8_5994p
@ NTV2_FORMAT_4096x2160p_5994
#define NTV2_FBF_HAS_ALPHA(__fbf__)
@ NTV2WidgetType_HDMIOutV2
@ NTV2_FORMAT_END_HIGH_DEF_FORMATS
@ DEVICE_ID_KONA5_OE6
See KONA 5.
static bool CopyRaster4BytesPer2Pixels(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
@ DEVICE_ID_KONA5_OE12
See KONA 5.
string NTV2HDMIBitDepthToString(const NTV2HDMIBitDepth inValue, const bool inCompact)
@ NTV2_REFERENCE_SFP1_PCR
Specifies the PCR source on SFP 1.
bool Fill10BitYCbCrVideoFrame(void *pBaseVideoAddress, const NTV2Standard inStandard, const NTV2FrameBufferFormat inFBF, const YCbCr10BitPixel inPixelColor, const NTV2VANCMode inVancMode)
@ NTV2_XptDualLinkOut1Input
@ DEVICE_ID_CORVID44_PLNR
See Corvid 44 12G.
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_5
@ NTV2_XptDuallinkOut3DS2
NTV2Standard GetNTV2StandardFromScanGeometry(const UByte inScanGeometry, const bool inIsProgressiveTransport)
@ NTV2_FORMAT_4x2048x1080psf_2997
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_3
@ NTV2_XptDualLinkOut8Input
@ NTV2_UpConvertZoomLetterbox
@ NTV2_VIDEOLIMITING_OFF
Disables normal FrameBuffer Y/C value read limiting (NOT RECOMMENDED).
@ NTV2_AUDIOSYSTEM_INVALID
NTV2VideoFormat GetTransportCompatibleFormat(const NTV2VideoFormat inFormat, const NTV2VideoFormat inTargetFormat)
@ NTV2_FORMAT_1080p_2K_2997
@ NTV2_1080i_3000to720p_6000
static bool CopyRaster6BytesPerPixel(UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
@ NTV2_SIGNALMASK_Cr
Output Cr if set, elso Output Cr to 0x200.
@ DEVICE_ID_IOEXPRESS
See Io Express.