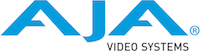 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
18 #if defined(AJA_LINUX) || defined(AJA_BAREMETAL)
30 static const std::string
sSeverityString[] = {
"emergency",
"alert",
"assert",
"error",
"warning",
"notice",
"info",
"debug"};
35 #define addDebugGroupToLabelVector(x) sGroupLabelVector.push_back(#x)
37 #define STAT_BIT_SHIFT (1ULL<<(inKey%64))
38 #define STAT_BIT_TEST (spShare->statAllocMask[inKey/(AJA_DEBUG_MAX_NUM_STATS/64)] & STAT_BIT_SHIFT)
39 #define IS_STAT_BAD !STAT_BIT_TEST
40 #define STAT_BIT_SET spShare->statAllocMask[inKey/(AJA_DEBUG_MAX_NUM_STATS/64)] |= STAT_BIT_SHIFT
41 #define STAT_BIT_CLEAR spShare->statAllocMask[inKey/(AJA_DEBUG_MAX_NUM_STATS/64)] &= 0xFFFFFFFFFFFFFFFF - STAT_BIT_SHIFT
53 #if defined(AJA_DEBUG)
106 if (incrementRefCount)
177 std::string name(
"AJA_DebugUnit_Unused_");
202 if (decrementRefCount)
224 uint32_t currentDestination = 0;
234 uint32_t currentDestination = 0;
332 inline uint64_t
report_common(int32_t index, int32_t severity,
const char* pFileName, int32_t lineNumber, uint64_t& writeIndex, int32_t& messageIndex)
334 static const char * spUnknown =
"unknown";
365 pFileName = spUnknown;
388 void AJADebug::Report (int32_t index, int32_t severity,
const char* pFileName, int32_t lineNumber, ...)
394 uint64_t writeIndex = 0;
395 int32_t messageIndex = 0;
396 if (
report_common(index, severity, pFileName, lineNumber, writeIndex, messageIndex))
400 va_start(vargs, lineNumber);
401 const char* pFormat = va_arg(vargs,
const char*);
405 pFormat = (
char*)
"no message";
423 void AJADebug::Report (int32_t index, int32_t severity,
const char* pFileName, int32_t lineNumber,
const std::string& message)
429 uint64_t writeIndex = 0;
430 int32_t messageIndex = 0;
431 if (
report_common(index, severity, pFileName, lineNumber, writeIndex, messageIndex))
448 #if defined(AJA_DEBUG)
459 if (pFileName ==
NULL)
461 pFileName = (
char*)
"unknown";
484 "assertion failed (file %s, line %d): %s\n",
485 pFileName, lineNumber, pExpression.c_str());
679 if (ppFileName ==
NULL)
837 if (severity < 0 || severity > 7)
838 return "severity range error";
843 {
static const std::string emptystr;
844 if (severity < 0 || severity > 7)
853 return "index range error";
862 static const std::string sRangeErr(
"<bad index>");
863 static const std::string sNoLabelErr(
"<empty>");
879 pFile = ::fopen(inFilePath.c_str(),
"w");
895 fprintf(pFile,
"CustomGroupDestination: %6d : %08x\n", i,
spShare->
unitArray[i]);
915 pFile = ::fopen(inFilePath.c_str(),
"r");
924 uint32_t destination;
928 count = fscanf(pFile,
" AJADebugVersion: %d", &intVersion);
929 version = intVersion;
935 count = fscanf(pFile,
" AJADebugStateFileVersion: %d", &intVersion);
936 version = intVersion;
946 count = fscanf(pFile,
" GroupDestination: %d : %x", &index, &destination);
949 count = fscanf(pFile,
" CustomGroupDestination: %d : %x", &index, &destination);
987 case AJA_STATUS_FAIL:
return inDetailed ?
"Failed" :
"AJA_STATUS_FAIL";
989 case AJA_STATUS_RANGE:
return inDetailed ?
"Out Of Range" :
"AJA_STATUS_RANGE";
992 case AJA_STATUS_OPEN:
return inDetailed ?
"Not Open" :
"AJA_STATUS_OPEN";
993 case AJA_STATUS_IO:
return inDetailed ?
"I/O Error" :
"AJA_STATUS_IO";
1001 case AJA_STATUS_MEMORY:
return inDetailed ?
"Out Of Memory" :
"AJA_STATUS_MEMORY";
1002 case AJA_STATUS_ALIGN:
return inDetailed ?
"Misaligned" :
"AJA_STATUS_ALIGN";
1018 #if !defined(_DEBUG)
1022 return "<bad AJAStatus>";
1174 #define HEX0N(__x__,__n__) std::hex << std::uppercase << std::setw(int(__n__)) << std::setfill('0') << (__x__) << std::dec << std::setfill(' ') << std::nouppercase
1189 {
const uint32_t * pU32(&stat.
fMin);
1190 for (
size_t num(0); num < 16; num++)
1191 std::cerr <<
" " <<
HEX0N(*pU32++,8);
1192 std::cerr << std::endl;
1253 outKeys.push_back(inKey);
1275 outKeys.insert(inKey);
1303 for (
size_t n(0); n < inNum; n++)
1310 uint32_t result(0xFFFFFFFF);
1315 for (
size_t n(0); n < inNum; n++)
1328 for (
size_t n(0); n < inNum; n++)
1363 if (!inRollOver &&
fCount == 0xFFFFFFFF)
1366 while (inIncrement--)
1376 if (!inRollUnder && !
fCount)
1379 while (inDecrement--)
1413 using namespace std;
1479 it->second = inName;
1492 result.push_back(it->first);
static AJAStatus GetClientReferenceCount(int32_t &outRefCount)
@ AJA_DebugStat_GetInterruptCount
@ AJA_STATUS_BADBUFFERSIZE
@ AJA_DebugStat_DMATransferEx
static AJAStatus SaveState(const std::string &inFilePath)
static AJAStatus GetMessageFileName(const uint64_t sequenceNumber, std::string &outFileName)
static AJAStatus StatReset(const uint32_t inKey)
static AJAStatus StatTimerStop(const uint32_t inKey)
static AJAStatus GetDestination(const int32_t inGroup, uint32_t &outDestination)
#define AJA_DEBUG_SHARE_NAME
@ AJA_DebugUnit_StatsGeneric
bool DecrementCount(const uint32_t inDecrement=1, const bool inRollUnder=true)
@ AJA_DebugUnit_AudioGeneric
static AJADebugShare * spShare
static AJAStatus GetMessageGroup(const uint64_t sequenceNumber, int32_t &outGroupIndex)
uint64_t report_common(int32_t index, int32_t severity, const char *pFileName, int32_t lineNumber, uint64_t &writeIndex, int32_t &messageIndex)
@ AJA_DebugUnit_FirstUnused
uint64_t volatile statsMessagesAccepted
@ AJA_DebugSeverity_Assert
static const std::string sSeverityString[]
@ AJA_DebugUnit_CC608DecodeScreen
StatKeyNameMap::iterator StatKeyNameMapIter
static StatKeyNameMap gStatKeyToStr
@ AJA_DebugSeverity_Warning
@ AJA_DebugStat_HEVCSendMessage
@ AJA_DebugUnit_App_User2
@ AJA_DebugStat_ReadRegister
@ AJA_DebugStat_NTV2Message
static AJAStatus StatTimerStart(const uint32_t inKey)
virtual bool IsValid(void) const
static std::string StatKeyName(const int inKey)
@ AJA_DebugStat_AutoCirculate
@ AJA_DebugStat_WaitForInterruptOthers
Declares the AJATime class.
@ AJA_DebugUnit_ControlPanel
static AJAStatus GetSequenceNumber(uint64_t &outSequenceNumber)
@ AJA_DebugStat_WaitForInterruptIn2
static uint64_t GetThreadId()
static AJAStatus SetDestination(int32_t index, uint32_t destination=AJA_DEBUG_DESTINATION_NONE)
static bool SetStatKeyName(const int inKey, const std::string &inName)
@ AJA_DebugUnit_App_Screen
StatKeyNameMap::const_iterator StatKeyNameMapConstIter
char * safer_strncpy(char *target, const char *source, size_t num, size_t maxSize)
bool operator==(const AJADebugStat &inRHS) const
pair< int, string > StatKeyNamePair
@ AJA_DebugUnit_AJAAncData
@ AJA_DebugUnit_AJAAncList
static AJAStatus Enable(int32_t index, uint32_t destination=AJA_DEBUG_DESTINATION_NONE)
static void Report(int32_t index, int32_t severity, const char *pFileName, int32_t lineNumber,...)
struct _AJADebugShare AJADebugShare
static uint64_t GetSystemMicroseconds(void)
Returns the current value of the host's high-resolution clock, in microseconds.
@ AJA_DebugStat_WaitForInterruptIn8
@ AJA_DebugUnit_DriverInterface
uint32_t messageTextCapacity
@ AJA_DebugStat_ACXferRPCDecode
@ AJA_DebugUnit_ServiceGeneric
uint32_t Maximum(size_t inNum=11) const
@ AJA_STATUS_STREAMCONFLICT
@ AJA_DebugUnit_App_Decode
@ AJA_DebugStat_DMATransferP2P
static AJAStatus GetMessageSeverity(const uint64_t sequenceNumber, int32_t &outSeverity)
Declares the AJAProcess class.
static const std::string & GroupName(const int32_t group)
static void * Exchange(void *volatile *pTarget, void *pValue)
@ AJA_DebugStat_NUM_STATS
#define AJA_DEBUG_MAX_NUM_STATS
@ AJA_DebugUnit_Anc2110Xmit
@ AJA_DebugUnit_CC608DecodeChannel
uint64_t volatile writeIndex
@ AJA_DebugUnit_Persistence
static AJAStatus RestoreState(const std::string &inFilePath)
#define addDebugGroupToLabelVector(x)
Declares the AJALock class.
static bool IsDebugBuild(void)
@ AJA_DebugUnit_App_DiskWrite
AJADebugMessage messageRing[4096]
static AJAStatus StatSetValue(const uint32_t inKey, const uint32_t inValue)
uint32_t messageRingCapacity
static const char * GetSeverityString(int32_t severity)
@ AJA_DebugStat_WaitForInterruptUartTx1
static void * GetPrivateDataLoc(void)
bool IncrementCount(const uint32_t inIncrement=1, const bool inRollOver=true)
static const char * GetGroupString(int32_t group)
static bool IsActive(int32_t index)
@ AJA_DebugStat_AutoCirculateXfer
static AJAStatus Close(bool decrementRefCount=false)
@ AJA_DebugUnit_RoutingGeneric
static void * AllocateShared(size_t *size, const char *pShareName, bool global=true)
@ AJA_STATUS_STREAMRUNNING
@ AJA_DebugStat_WaitForInterruptUartTx2
@ AJA_DebugUnit_DemoPlayout
void SetValue(const uint32_t inValue)
static AJAStatus GetMessagesAccepted(uint64_t &outCount)
int32_t volatile clientRefCount
static AJAStatus GetMessageSequenceNumber(const uint64_t sequenceNumber, uint64_t &outSequenceNumber)
static void InitStatKeyNames(void)
static AJAStatus SetClientReferenceCount(int32_t refCount)
static int64_t DebugTime(void)
static AJALock gStatKeyToStrLock
static void AssertWithMessage(const char *pFileName, int32_t lineNumber, const std::string &pExpression)
@ AJA_DebugUnit_CC708Decode
static AJAStatus StatAllocate(const uint32_t inKey)
static AJAStatus StatGetKeys(std::vector< uint32_t > &outKeys, uint32_t &outSeqNum)
#define AJA_DEBUG_MAGIC_ID
static AJAStatus GetThreadId(const uint64_t sequenceNumber, uint64_t &outTid)
static AJAStatus StatGetInfo(const uint32_t inKey, AJADebugStat &outInfo)
@ AJA_DebugUnit_App_User1
#define AJA_DEBUG_VERSION
static void FreeShared(void *pMemory)
uint64_t volatile statsMessagesIgnored
uint32_t unitArray[65536]
#define AJA_DEBUG_TICK_RATE
@ AJA_DebugUnit_App_Encode
double Average(void) const
@ AJA_DebugUnit_UserGeneric
static size_t GetPrivateDataLen(void)
@ AJA_STATUS_INVALID_TIME
#define AJA_DEBUG_DESTINATION_CONSOLE
static AJAStatus Open(bool incrementRefCount=false)
@ AJA_DebugStat_WaitForInterruptUartRx2
@ AJA_DebugUnit_App_Alloc
static AJAStatus StatCounterIncrement(const uint32_t inKey, const uint32_t inIncrement=1)
static uint32_t StatsCapacity(void)
@ AJA_DebugUnit_CCLine21Decode
System specific functions.
@ AJA_DebugUnit_AncGeneric
@ AJA_DebugStat_WaitForInterruptIn7
static std::vector< std::string > sGroupLabelVector
static int32_t Increment(int32_t volatile *pTarget)
@ AJA_DebugUnit_CC608Encode
@ AJA_DebugStat_WaitForInterruptIn6
uint32_t volatile statAllocChanges
@ AJA_DebugStat_WaitForInterruptUartRx1
Private include file for all ajabase sources.
@ AJA_DebugUnit_CC608Decode
static AJAStatus GetMessageDestination(const uint64_t sequenceNumber, uint32_t &outDestination)
static const std::string & SeverityName(const int32_t severity)
#define AJA_DEBUG_STAT_DEQUE_SIZE
static bool StatIsAllocated(const uint32_t inKey)
#define HEX0N(__x__, __n__)
static uint32_t Version(void)
@ AJA_DebugUnit_Enumeration
@ AJA_DebugUnit_CC608MsgQueue
@ AJA_DebugStat_WaitForInterruptOut1
#define AJA_DEBUG_UNIT_ARRAY_SIZE
@ AJA_DebugUnit_CC608DataQueue
static std::vector< int > NamedStatKeys(void)
@ AJA_DebugUnit_Anc2110Rcv
std::string AJAStatusToString(const AJAStatus inStatus, const bool inDetailed)
uint64_t Sum(size_t inNum=11) const
uint64_t statAllocMask[256/64]
@ AJA_DebugUnit_CC708Encode
@ AJA_DebugUnit_Application
static AJAStatus GetMessageTime(const uint64_t sequenceNumber, uint64_t &outTime)
Declares the AJAAtomic class.
static uint32_t TotalBytes(void)
static AJAStatus GetMessageLineNumber(const uint64_t sequenceNumber, int32_t &outLineNumber)
@ AJA_DebugUnit_TimecodeGeneric
@ AJA_DebugUnit_CC708Window
static AJAStatus GetProcessId(const uint64_t sequenceNumber, uint64_t &outPid)
@ AJA_DebugUnit_CC708ServiceBlockQueue
uint32_t Minimum(size_t inNum=11) const
static AJAStatus GetMessagesIgnored(uint64_t &outCount)
@ AJA_DebugStat_WriteRegister
static bool HasStats(void)
@ AJA_DebugUnit_RPCClient
@ AJA_DebugUnit_RPCServer
@ AJA_DebugStat_DMATransfer
std::ostream & operator<<(std::ostream &oss, const AJADebugStat &inStat)
#define AJA_DEBUG_DESTINATION_NONE
static AJAStatus Disable(int32_t index, uint32_t destination=AJA_DEBUG_DESTINATION_NONE)
@ AJA_STATUS_SURPRISE_REMOVAL
map< int, string > StatKeyNameMap
@ AJA_DebugUnit_DriverGeneric
std::string to_string(bool val)
static AJAStatus GetMessageText(const uint64_t sequenceNumber, std::string &outMessage)
static bool gStatKeyToStrReady(false)
@ AJA_DebugUnit_CC708Service
@ AJA_STATUS_BADBUFFERCOUNT
@ AJA_DebugUnit_CCLine21Encode
@ AJA_DebugStat_WaitForInterruptIn1
@ AJA_DebugUnit_VideoGeneric
Declares the AJAThread class.
@ AJA_DebugStat_WaitForInterruptIn3
static uint32_t MessageRingCapacity(void)
static AJAStatus GetMessageWallClockTime(const uint64_t sequenceNumber, int64_t &outTime)
#define AJA_DEBUG_MESSAGE_RING_SIZE
static int64_t GetSystemCounter(void)
Returns the current value of the host system's high-resolution time counter.
@ AJA_DebugStat_WaitForInterruptIn4
Declares the AJAMemory class.
@ AJA_STATUS_NOTINITIALIZED
@ AJA_DebugUnit_QuickTime
#define AJA_DEBUG_STATE_FILE_VERSION
static AJAStatus StatGetSequenceNum(uint32_t &outSeqNum)
static int64_t GetSystemFrequency(void)
Returns the frequency of the host system's high-resolution time counter.
@ AJA_DebugUnit_App_DiskRead
Declares the AJADebug class.
@ AJA_DebugUnit_DemoCapture
@ AJA_DebugUnit_AutoCirculate
#define AJA_DEBUG_MESSAGE_MAX_SIZE
static int32_t Decrement(int32_t volatile *pTarget)
@ AJA_DebugStat_WaitForInterruptIn5
uint32_t messageFileNameCapacity
@ AJA_DebugStat_ACXferRPCEncode
static AJAStatus StatFree(const uint32_t inKey)
#define AJA_DEBUG_FILE_NAME_MAX_SIZE