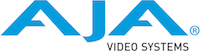 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
10 #ifndef AJA_DEBUGSHARE_H
11 #define AJA_DEBUGSHARE_H
164 #define AJA_DEBUG_DESTINATION_NONE 0
166 #define AJA_DEBUG_DESTINATION_DEBUG 0x00000001
167 #define AJA_DEBUG_DESTINATION_CONSOLE 0x00000002
168 #define AJA_DEBUG_DESTINATION_LOG 0x00000004
169 #define AJA_DEBUG_DESTINATION_DRIVER 0x00000008
178 #define AJA_DEBUG_MAGIC_ID AJA_FOURCC('D','B','U','G')
180 #define AJA_DEBUG_VERSION 110
181 #define AJA_DEBUG_UNIT_ARRAY_SIZE 65536
182 #define AJA_DEBUG_SEVERITY_ARRAY_SIZE 64
183 #define AJA_DEBUG_MAX_NUM_STATS 256
184 #define AJA_DEBUG_STAT_DEQUE_SIZE 11
185 #define AJA_DEBUG_MESSAGE_MAX_SIZE 512
186 #define AJA_DEBUG_MESSAGE_RING_SIZE 4096
187 #define AJA_DEBUG_FILE_NAME_MAX_SIZE 512
188 #define AJA_DEBUG_SHARE_NAME "aja-shm-debug"
189 #define AJA_DEBUG_TICK_RATE 1000000
190 #define AJA_DEBUG_STATE_FILE_VERSION 510
199 #pragma pack(push, 16)
284 inline bool operator != (
const AJADebugStat & inRHS)
const {
return !(*
this == inRHS);}
286 double Average(
void)
const;
298 bool IncrementCount (
const uint32_t inIncrement = 1,
const bool inRollOver =
true);
305 bool DecrementCount (
const uint32_t inDecrement = 1,
const bool inRollUnder =
true);
307 inline bool IsSimpleCounter (
void)
const {
return fMin == 0xFFFFFFFF && !fMax;}
308 void SetValue (
const uint32_t inValue);
309 inline uint32_t GetCurrentValue (
void)
const {
return fCount ? fValues[CurrentValueIndex()] : 0;}
311 inline uint32_t GetValue (
void)
const {
return IsSimpleCounter() ? fCount : GetCurrentValue();}
315 static std::string StatKeyName (
const int inKey);
316 static inline bool StatKeyHasName (
const int inKey) {
return !StatKeyName(inKey).empty();}
317 static bool SetStatKeyName (
const int inKey,
const std::string & inName);
318 static std::vector<int> NamedStatKeys (
void);
352 #endif // AJA_DEBUGSHARE_H
@ AJA_DebugStat_GetInterruptCount
@ AJA_DebugStat_DMATransferEx
@ AJA_DebugUnit_Unused_76
@ AJA_DebugUnit_StatsGeneric
@ AJA_DebugUnit_AudioGeneric
@ AJA_DebugUnit_FirstUnused
uint64_t volatile statsMessagesAccepted
@ AJA_DebugSeverity_Assert
@ AJA_DebugUnit_CC608DecodeScreen
@ AJA_DebugUnit_Unused_82
@ AJA_DebugSeverity_Warning
@ AJA_DebugStat_HEVCSendMessage
@ AJA_DebugUnit_App_User2
@ AJA_DebugStat_ReadRegister
@ AJA_DebugStat_NTV2Message
@ AJA_DebugUnit_Unused_68
@ AJA_DebugUnit_Unused_60
@ AJA_DebugUnit_Unused_67
enum _AJADebugSeverity AJADebugSeverity
@ AJA_DebugStat_AutoCirculate
@ AJA_DebugStat_WaitForInterruptOthers
@ AJA_DebugUnit_Unused_62
@ AJA_DebugUnit_ControlPanel
@ AJA_DebugSeverity_Error
@ AJA_DebugStat_WaitForInterruptIn2
@ AJA_DebugSeverity_Alert
@ AJA_DebugUnit_App_Screen
@ AJA_DebugUnit_Unused_78
enum _AJADebugUnit AJADebugUnit
@ AJA_DebugUnit_AJAAncData
@ AJA_DebugUnit_AJAAncList
struct _AJADebugShare AJADebugShare
@ AJA_DebugStat_WaitForInterruptIn8
@ AJA_DebugUnit_DriverInterface
uint32_t messageTextCapacity
@ AJA_DebugStat_ACXferRPCDecode
@ AJA_DebugUnit_Unused_70
@ AJA_DebugUnit_ServiceGeneric
@ AJA_DebugUnit_Unused_61
uint32_t reserved[128 - 1 - 1 - 2 *256/64]
@ AJA_DebugUnit_Unused_65
@ AJA_DebugUnit_App_Decode
@ AJA_DebugStat_DMATransferP2P
@ AJA_DebugStat_NUM_STATS
#define AJA_DEBUG_MAX_NUM_STATS
@ AJA_DebugUnit_Anc2110Xmit
@ AJA_DebugUnit_CC608DecodeChannel
uint64_t volatile writeIndex
enum _AJADebugStats AJADebugStats
@ AJA_DebugUnit_Unused_72
@ AJA_DebugUnit_Persistence
@ AJA_DebugUnit_App_DiskWrite
AJADebugMessage messageRing[4096]
uint32_t messageRingCapacity
@ AJA_DebugUnit_Unused_74
@ AJA_DebugStat_WaitForInterruptUartTx1
@ AJA_DebugStat_AutoCirculateXfer
@ AJA_DebugUnit_RoutingGeneric
@ AJA_DebugStat_WaitForInterruptUartTx2
@ AJA_DebugUnit_DemoPlayout
int32_t volatile clientRefCount
@ AJA_DebugSeverity_Debug
@ AJA_DebugUnit_Unused_83
@ AJA_DebugUnit_CC708Decode
@ AJA_DebugSeverity_Notice
@ AJA_DebugUnit_App_User1
@ AJA_DebugUnit_Unused_84
@ AJA_DebugUnit_Unused_64
uint64_t volatile statsMessagesIgnored
uint32_t unitArray[65536]
@ AJA_DebugUnit_App_Encode
@ AJA_DebugUnit_Unused_75
@ AJA_DebugUnit_UserGeneric
@ AJA_DebugStat_WaitForInterruptUartRx2
static bool StatKeyHasName(const int inKey)
@ AJA_DebugUnit_App_Alloc
@ AJA_DebugUnit_Unused_63
@ AJA_DebugUnit_CCLine21Decode
@ AJA_DebugUnit_AncGeneric
@ AJA_DebugStat_WaitForInterruptIn7
@ AJA_DebugUnit_CC608Encode
@ AJA_DebugStat_WaitForInterruptIn6
uint32_t volatile statAllocChanges
@ AJA_DebugStat_WaitForInterruptUartRx1
@ AJA_DebugUnit_Unused_66
@ AJA_DebugUnit_CC608Decode
#define AJA_DEBUG_STAT_DEQUE_SIZE
@ AJA_DebugUnit_Enumeration
@ AJA_DebugUnit_Unused_79
@ AJA_DebugUnit_CC608MsgQueue
@ AJA_DebugStat_WaitForInterruptOut1
#define AJA_DEBUG_UNIT_ARRAY_SIZE
@ AJA_DebugUnit_CC608DataQueue
@ AJA_DebugUnit_Anc2110Rcv
uint64_t statAllocMask[256/64]
@ AJA_DebugUnit_CC708Encode
@ AJA_DebugUnit_Application
@ AJA_DebugSeverity_Emergency
@ AJA_DebugUnit_Unused_77
@ AJA_DebugUnit_TimecodeGeneric
@ AJA_DebugUnit_CC708Window
@ AJA_DebugUnit_CC708ServiceBlockQueue
@ AJA_DebugStat_WriteRegister
@ AJA_DebugUnit_Unused_80
@ AJA_DebugUnit_RPCClient
@ AJA_DebugUnit_RPCServer
@ AJA_DebugStat_DMATransfer
@ AJA_DebugUnit_Unused_81
@ AJA_DebugUnit_Unused_71
@ AJA_DebugUnit_Unused_73
@ AJA_DebugUnit_DriverGeneric
@ AJA_DebugUnit_CC708Service
@ AJA_DebugUnit_CCLine21Encode
@ AJA_DebugStat_WaitForInterruptIn1
@ AJA_DebugUnit_VideoGeneric
@ AJA_DebugStat_WaitForInterruptIn3
#define AJA_DEBUG_MESSAGE_RING_SIZE
@ AJA_DebugStat_WaitForInterruptIn4
@ AJA_DebugUnit_QuickTime
@ AJA_DebugUnit_Unused_69
@ AJA_DebugUnit_App_DiskRead
@ AJA_DebugUnit_DemoCapture
@ AJA_DebugUnit_AutoCirculate
#define AJA_DEBUG_MESSAGE_MAX_SIZE
struct _AJADebugMessage AJADebugMessage
@ AJA_DebugStat_WaitForInterruptIn5
uint32_t messageFileNameCapacity
@ AJA_DebugStat_ACXferRPCEncode
Declares system-dependent import/export macros and libraries.
#define AJA_DEBUG_FILE_NAME_MAX_SIZE