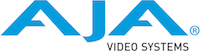 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
15 #pragma warning(disable: 4800)
21 #define AUDFAIL(__x__) AJA_sERROR (AJA_DebugUnit_AudioGeneric, " " << HEX0N(uint64_t(this),16) << "::" << AJAFUNC << ": " << __x__)
22 #define AUDWARN(__x__) AJA_sWARNING(AJA_DebugUnit_AudioGeneric, " " << HEX0N(uint64_t(this),16) << "::" << AJAFUNC << ": " << __x__)
23 #define AUDNOTE(__x__) AJA_sNOTICE (AJA_DebugUnit_AudioGeneric, " " << HEX0N(uint64_t(this),16) << "::" << AJAFUNC << ": " << __x__)
24 #define AUDINFO(__x__) AJA_sINFO (AJA_DebugUnit_AudioGeneric, " " << HEX0N(uint64_t(this),16) << "::" << AJAFUNC << ": " << __x__)
25 #define AUDDBUG(__x__) AJA_sDEBUG (AJA_DebugUnit_AudioGeneric, " " << HEX0N(uint64_t(this),16) << "::" << AJAFUNC << ": " << __x__)
153 if (regAudControl == 0)
155 else if (inNumChannels == 6 || inNumChannels == 8)
163 else if (inNumChannels == 16)
175 size_t numFailures(0);
177 if (!SetNumberAudioChannels (inNumChannels, *it))
179 return numFailures == 0;
189 if (regAudControl == 0)
239 if ((rateLow == 0) && (rateHigh == 0))
241 else if ((rateLow == 1) && (rateHigh == 0))
243 else if ((rateLow == 0) && (rateHigh == 1))
263 size_t numFailures(0);
265 if (!SetAudioBufferSize (inMode, *it))
267 return numFailures == 0;
286 if (IsBreakoutBoardConnected())
296 if (IsBreakoutBoardConnected())
316 size_t numFailures(0);
318 if (!SetAudioLoopBack (inMode, *it))
320 return numFailures == 0;
360 switch (inAudioInput)
370 default:
return false;
393 ULWord sparse1(0), sparse2(0);
396 if (!sparse1 && !sparse2)
398 else if (sparse1 && !sparse2)
400 else if (!sparse1 && sparse2)
402 else if (sparse1 && sparse2)
439 if (!GetAudioBufferSize (bufferSize, inAudioSystem))
455 if (!GetAudioBufferSize (bufferSize, inAudioSystem))
482 #if !defined(NTV2_DEPRECATE_16_0)
485 #endif // !defined(NTV2_DEPRECATE_16_0)
493 static const ULWord sAudioSourceToRegValues [] = { 0x1,
502 sAudioSourceToRegValues [inAudioSource],
507 if (SetEmbeddedAudioInput (inEmbeddedSource, inAudioSystem))
513 result = EnableBOBAnalogAudioIn(
true);
515 result = EnableBOBAnalogAudioIn(
false);
536 switch (regValue & 0x0000000F)
543 default:
return false;
547 GetEmbeddedAudioInput(outEmbeddedSource, inAudioSystem);
559 ULWord b2(0), b1(0), b0(0);
561 if (!ReadRegister (regNum, b2,
BIT(18), 18))
563 if (!ReadRegister (regNum, b1,
BIT(28), 28))
565 if (!ReadRegister (regNum, b0,
BIT(30), 30))
580 ULWord value (inAudioSystem);
602 size_t numFailures(0);
603 for (NTV2ChannelSet::const_iterator it(inSDIOutputs.begin()); it != inSDIOutputs.end(); ++it)
604 if (!(inDS2 ? SetSDIOutputDS2AudioSystem(*it, inAudioSystem) : SetSDIOutputAudioSystem(*it, inAudioSystem)))
606 return numFailures == 0;
616 ULWord b2(0), b1(0), b0(0);
618 if (!ReadRegister (regNum, b2,
BIT(19), 19))
620 if (!ReadRegister (regNum, b1,
BIT(29), 29))
622 if (!ReadRegister (regNum, b0,
BIT(31), 31))
637 ULWord value (inAudioSystem);
763 vector<uint32_t> & outLevels)
775 if (inChannelPairs.empty())
778 chanPairs.insert(chPr);
781 chanPairs = inChannelPairs;
785 std::set<ULWord> regsToRead;
791 uint32_t regNum(gAudMxrMainOutLvlRegs[chanPair]);
792 regsToRead.insert(regNum);
794 for (std::set<ULWord>::const_iterator it(regsToRead.begin()); it != regsToRead.end(); ++it)
798 const bool result(ReadRegisters(regs));
802 ULWord rawLevels(it->IsValid() ? it->registerValue : 0);
807 while (outLevels.size() < chanPairs.size() * 2)
808 outLevels.push_back(0);
835 unsigned long ulongvalue(0);
876 vector<uint32_t> & outLevels)
890 if (inChannelPairs.empty())
896 chanPairs.insert(chPr);
899 chanPairs = inChannelPairs;
903 std::set<ULWord> regsToRead;
909 uint32_t regNum(gAudMxrMainInLvlRegs[chanPair]);
918 regsToRead.insert(regNum);
920 for (std::set<ULWord>::const_iterator it(regsToRead.begin()); it != regsToRead.end(); ++it)
924 const bool result(ReadRegisters(regs));
928 ULWord rawLevels(it->IsValid() ? it->registerValue : 0);
933 while (outLevels.size() < chanPairs.size() * 2)
934 outLevels.push_back(0);
943 outSampleCount = 1 << outSampleCount;
951 if (inSampleCount > 0x00008000)
953 ULWord result(0), sampleCount(inSampleCount);
954 while (sampleCount >>= 1)
981 ULWord channelSelect =
static_cast<ULWord>(inValue) % 4;
989 const ULWord encoding ((
ULWord (inAudioSystem) << 4) | inValue);
1000 ULWord engineSelect (0), channelSelect(0), bankSelect(0);
1052 bool result =
false;
1055 ULWord engineSelect (0), channelSelect(0);
1075 outAudioSystem =
static_cast <NTV2AudioSystem> ((encoding & 0xC) >> 2);
1112 const ULWord encoding ((
ULWord(inAudioSystem) << 4) | inChannelPair);
1150 for (
size_t ndx(0); ndx < badRgns.size(); ndx++)
1151 {
const ULWord rgnInfo(badRgns.at(ndx));
1152 const UWord startBlk(rgnInfo >> 16), numBlks(
UWord(rgnInfo & 0x0000FFFF));
1155 const string infoStr (
aja::join(tags,
", "));
1156 ostringstream acLabel; acLabel <<
"Aud" <<
DEC(inAudioSystem+1);
1157 if (infoStr.find(acLabel.str()) != string::npos)
1158 { ostringstream warning;
1160 warning <<
"8MB Frms " <<
DEC0N(startBlk,3) <<
"-" <<
DEC0N(startBlk+numBlks-1,3);
1162 warning <<
"8MB Frm " <<
DEC0N(startBlk,3);
1163 AUDWARN(
"Aud" <<
DEC(inAudioSystem+1) <<
" memory overlap/interference: " << warning.str() <<
": " << infoStr);
1178 bool isStopped (
true);
1183 outIsRunning = !isStopped;
1239 for (
size_t ndx(0); ndx < badRgns.size(); ndx++)
1240 {
const ULWord rgnInfo(badRgns.at(ndx));
1241 const UWord startBlk(rgnInfo >> 16), numBlks(
UWord(rgnInfo & 0x0000FFFF));
1244 const string infoStr (
aja::join(tags,
", "));
1245 ostringstream acLabel; acLabel <<
"Aud" <<
DEC(inAudioSystem+1);
1246 if (infoStr.find(acLabel.str()) != string::npos)
1247 { ostringstream warning;
1249 warning <<
"8MB Frms " <<
DEC0N(startBlk,3) <<
"-" <<
DEC0N(startBlk+numBlks-1,3);
1251 warning <<
"8MB Frm " <<
DEC0N(startBlk,3);
1252 AUDWARN(
"Aud" <<
DEC(inAudioSystem+1) <<
" memory overlap/interference: " << warning.str() <<
": " << infoStr);
1269 bool isStopped (
true);
1274 outIsRunning = !isStopped;
1377 const bool isNonPCM (inNonPCMChannelPairs.find (chanPair) != inNonPCMChannelPairs.end ());
1402 ULWord numAudioChannels (0);
1403 bool isNonPCM (
false);
1405 outNonPCMChannelPairs.clear ();
1408 if (!GetNumberAudioChannels (numAudioChannels, inAudioSystem))
1412 if (!GetAudioPCMControl (inAudioSystem, isNonPCM))
1417 for (
UWord chPair (0); chPair <= maxPair; chPair++)
1428 if (regVal &
BIT(inAudioSystem * 8 + chanPair))
1429 outNonPCMChannelPairs.insert (chanPair);
1448 outIsPresent =
false;
1449 if (!GetDetectedAudioChannelPairs (inAudioSystem, activeChannelPairs))
1451 if (activeChannelPairs.find (inChannelPair) != activeChannelPairs.end ())
1452 outIsPresent =
true;
1459 outDetectedChannelPairs.clear ();
1469 if (detectBits &
BIT(bitGroup * 8 + chanPair))
1470 outDetectedChannelPairs.insert (chanPair);
1477 uint32_t valLo8(0), valHi8(0);
1478 outDetectedChannelPairs.clear ();
1486 const uint32_t detectBits (((valLo8 >> 24) & 0x0000000F) | ((valHi8 >> 24) & 0x000000F0));
1488 if (!(detectBits &
BIT(chPair)))
1489 outDetectedChannelPairs.insert(chPair);
1544 const UWord maxNumAudioChannelsForQuad ((inAESAudioChannels + 1) * 4);
1549 if (numAESAudioOutputChannels < 4)
1551 if (maxNumAudioChannelsForQuad > numAESAudioOutputChannels)
1583 if (inBitMask &
BIT (channelPair))
1584 result.insert (channelPair);
1585 if (inExtendedBitMask)
1587 if (inExtendedBitMask &
BIT (channelPair))
1588 result.insert (channelPair);
1604 if (!GetInputAudioChannelPairsWithPCM (inSDIInputChannel, withPCMs))
1607 outHasPCM = withPCMs.find (inAudioChannelPair) != withPCMs.end ();
1614 outPCMPairs.clear ();
1624 const bool isExtended (numChannels > 16);
1628 if (!ReadRegister (regNum, mask))
1631 if (!ReadRegister (regNum + 1, extMask))
1642 outNonPCMPairs.clear ();
1652 const bool isExtended (numChannels > 16);
1656 if (!ReadRegister (regNum, mask))
1659 if (!ReadRegister (regNum + 1, extMask))
1673 outIsEnabled =
true;
1684 outIsEnabled = value ?
false :
true;
1703 outEraseModeEnabled =
false;
1739 outXmitEnabled =
false;
1749 outXmitEnabled = !outXmitEnabled;
1769 #if !defined(NTV2_DEPRECATE_16_1)
1780 bool xlr14Xmit(
false), xlr58Xmit(
false);
1785 if (xlr14Xmit && xlr58Xmit)
1787 else if (xlr14Xmit && !xlr58Xmit)
1789 else if (!xlr14Xmit && xlr58Xmit)
1795 #endif // !defined(NTV2_DEPRECATE_16_1)
1806 outAESSyncModeBitSet = regValue ?
true :
false;
1834 #pragma warning(default: 4800)
@ kRegShiftAud4PlayCapMode
@ kRegMaskPCMControlA2P9_10
@ kK2RegShiftAudioBufferSize
@ kRegShiftPCMControlA7P15_16
@ kRegMaskPCMControlA5P1_2
@ kRegShiftEmbeddedAudioInput
virtual bool IsAudioOutputRunning(const NTV2AudioSystem inAudioSystem, bool &outIsRunning)
Answers whether or not the playout side of the given NTV2AudioSystem is currently running.
@ kRegMaskPCMControlA5P13_14
virtual bool StopAudioInput(const NTV2AudioSystem inAudioSystem)
Stops the capture side of the given NTV2AudioSystem, and resets the capture position (i....
@ kRegMaskPCMControlA3P11_12
@ kRegShiftPCMControlA7P11_12
@ kRegShiftPCMControlA8P9_10
@ kRegMaskPCMControlA6P15_16
virtual bool SetAudioOutputDelay(const NTV2AudioSystem inAudioSystem, const ULWord inDelay)
Sets the audio output delay for the given Audio System on the device.
@ kRegMaskAudioMixerChannelSelect
virtual bool GetInputAudioChannelPairsWithPCM(const NTV2Channel inSDIInputConnector, NTV2AudioChannelPairs &outChannelPairs)
For the given SDI input (specified as a channel number), returns the set of audio channel pairs that ...
@ kRegMaskPCMControlA6P11_12
@ kRegMaskHDMIOutAudioFormat
@ kRegShiftPCMControlA4P13_14
@ kRegMaskPCMControlA1P7_8
UWord NTV2DeviceGetNumHDMIVideoInputs(const NTV2DeviceID inDeviceID)
static const ULWord kAudCtrlRegsForSDIOutputs[]
@ kRegShiftOutputStartAtVBI
@ kRegShiftHDMIOutAudioCh
@ kRegMaskAud6PlayCapMode
@ kRegMaskPCMControlA2P5_6
static const ULWord gChannelToAudioOutLastAddrRegNum[]
@ kRegMaskAudioMixerMainInputSelect
@ kRegShiftAud2PlayCapMode
@ kRegAudioMixerAux1GainCh2
@ kRegMaskPCMControlA5P5_6
virtual bool SetAudioOutputMonitorSource(const NTV2AudioChannelPair inChannelPair, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the audio monitor output source to a specified audio system and channel pair....
@ kRegShiftAudioMixerOutputChannelsMute
virtual bool StartAudioInput(const NTV2AudioSystem inAudioSystem, const bool inWaitForVBI=false)
Starts the capture side of the given NTV2AudioSystem, writing incoming audio samples into the Audio S...
@ NTV2_AudioChannel13_16
This selects audio channels 13 thru 16.
#define NTV2_IS_VALID_AUDIO_CHANNEL_OCTET(__p__)
@ kRegShiftPCMControlA6P9_10
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_4
@ NTV2_HDMIAudio2Channels
2 audio channels
@ kRegAudioMixerAux1InputLevels
virtual bool GetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, NTV2AudioSource &outAudioSource, NTV2EmbeddedAudioInput &outEmbeddedSource)
Answers with the device's current NTV2AudioSource (and also possibly its NTV2EmbeddedAudioInput) for ...
virtual bool SetEmbeddedAudioClock(const NTV2EmbeddedAudioClock inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2EmbeddedAudioClock setting for the given NTV2AudioSystem.
Declares device capability functions.
virtual bool SetAudioMixerInputChannelsMute(const NTV2AudioMixerInput inMixerInput, const NTV2AudioChannelsMuted16 inMutes)
Mutes (or enables) the given output audio channel of the Audio Mixer.
std::set< std::string > NTV2StringSet
@ kRegShiftPCMControlA4P1_2
@ kRegMaskRotaryEncoderGain
@ kRegShiftPCMControlA7P5_6
static const ULWord gAudioPlayCaptureModeShifts[]
virtual bool SetAudioMixerInputChannelSelect(const NTV2AudioMixerInput inMixerInput, const NTV2AudioChannelPair inChannelPair)
Specifies the Audio Channel Pair that will drive the given input of the Audio Mixer.
@ kRegMaskAud8PlayCapMode
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
@ kRegShiftAudioMixerChannelSelect
@ kRegAudioMixerAux1GainCh1
@ kRegMaskPCMControlA1P5_6
NTV2AudioMixerInput
Identifies the Audio Mixer's audio inputs.
Declares common audio macros and structs used in the SDK.
@ kRegShiftAud6PlayCapMode
@ kRegShiftPCMControlA5P9_10
std::string join(const std::vector< std::string > &parts, const std::string &delim)
static const ULWord sAudioMixerInputSelectMasks[]
@ kRegShiftAudioMixerAux2InputEnable
#define NTV2_ASSERT(_expr_)
@ kRegMaskPCMControlA4P3_4
virtual bool StopAudioOutput(const NTV2AudioSystem inAudioSystem)
Stops the playout side of the given NTV2AudioSystem, parking the "Read Head" at the start of the play...
@ NTV2_MAX_NUM_AudioChannelPair
virtual bool GetAudioBufferSize(NTV2AudioBufferSize &outSize, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Retrieves the size of the input or output audio buffer being used for a given Audio System on the AJA...
@ kRegShiftPCMControlA2P1_2
virtual bool SetSuspendHostAudio(const bool inSuspend)
Suspends or resumes host OS audio (e.g. CoreAudio on MacOS) for the AJA device.
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_1
static const ULWord sAudioMixerInputGainCh1Regs[]
@ kRegMaskHDMIOutAudioSource
@ kRegShiftBOBAnalogInputSelect
@ NTV2_AUDIO_CHANNEL_PAIR_INVALID
@ kRegShiftPCMControlA5P3_4
@ kRegMaskPCMControlA4P13_14
virtual bool GetAudioMixerInputAudioSystem(const NTV2AudioMixerInput inMixerInput, NTV2AudioSystem &outAudioSystem)
Answers with the Audio System that's currently driving the given input of the Audio Mixer.
@ kRegMaskPCMControlA2P13_14
#define NTV2_AUDIO_WRAPADDRESS_BIG
@ kRegMaskPCMControlA6P3_4
@ kRegShiftPCMControlA8P13_14
@ NTV2_AUDIO_MIC
Obtain audio samples from the device microphone input, if available.
@ kRegMaskPCMControlA8P13_14
@ kRegShiftHDMIOut8ChGroupSelect
bool NTV2DeviceCanDoMultiLinkAudio(const NTV2DeviceID inDeviceID)
virtual bool GetAudioWrapAddress(ULWord &outWrapAddress, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the wrap address, the threshold at which input/record or out...
@ kRegMaskAnalogIOControl_58
@ kRegMaskAudioMixerAux2x2CHInput
static const PCM_CONTROL_INFO gAudioEngineChannelPairToFieldInformation[][8]
@ NTV2_AudioMixerChannel1
@ NTV2_AudioChannel17_18
This selects audio channels 17 and 18.
virtual bool GetEncodedAudioMode(NTV2EncodedAudioMode &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
NTV2HDMIAudioChannels
Indicates or specifies the HDMI audio channel count.
bool GetTagsForFrameIndex(const UWord inIndex, NTV2StringSet &outTags) const
Answers with the list of tags for the given frame number.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
@ kRegMaskAudioMixerAux1InputEnable
@ kRegShiftAud1PlayCapMode
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_8
virtual bool EnableBOBAnalogAudioIn(bool inEnable)
Enables breakout board analog audio XLR inputs.
@ kRegMaskAud7PlayCapMode
@ kRegShiftHDMIOutAudioFormat
@ kRegShiftPCMControlA2P15_16
static const ULWord gAudioRateHighMask[]
@ kRegShiftPCMControlA3P9_10
virtual bool GetHDMIOutAudioRate(NTV2AudioRate &outValue)
Answers with the HDMI output's current audio rate.
#define DEC0N(__x__, __n__)
@ kRegMaskHDMIOutAudioRate
@ kRegMaskBOBAnalogLevelControl
@ kRegMaskPCMControlA3P3_4
@ kRegShiftPCMControlA2P5_6
@ kRegAudioOutputSourceMap
@ kRegMaskPCMControlA3P15_16
virtual bool GetAudioMixerOutputLevels(const NTV2AudioChannelPairs &inChannelPairs, std::vector< uint32_t > &outLevels)
Answers with the Audio Mixer's current audio output levels.
@ kRegShiftPCMControlA3P15_16
@ kRegShiftPCMControlA1P15_16
@ kRegShiftAudio16Channel
@ kRegMaskPCMControlA8P5_6
@ kRegShiftHDMIOutSourceSelect
@ kRegMaskPCMControlA5P11_12
@ kRegAudioMixerAux2GainCh1
@ kRegShiftAud8PlayCapMode
@ kRegShiftPCMControlA6P5_6
static const ULWord sAudioMixerInputSelectShifts[]
virtual bool GetAudioOutputAESSyncModeBit(const NTV2AudioSystem inAudioSystem, bool &outAESSyncModeBitSet)
Answers with the current state of the AES Sync Mode bit for the given Audio System's output.
@ kRegAudioMixerMainOutputLevelsPair0
@ kDeviceCanDoAudioMixer
True if device has a firmware audio mixer.
@ kRegMaskPCMControlA8P3_4
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ kRegAudioMixerMainOutputLevelsPair6
bool NTV2DeviceHasBiDirectionalAnalogAudio(const NTV2DeviceID inDeviceID)
virtual bool GetAudioInputDelay(const NTV2AudioSystem inAudioSystem, ULWord &outDelay)
Answers with the audio input delay for the given Audio System on the device.
@ kRegAudioMixerMainInputLevelsPair1
@ kRegMaskPCMControlA6P5_6
@ kRegShiftEmbeddedAudioClock
Audits an NTV2 device's SDRAM utilization, and can report contiguous regions of SDRAM,...
@ kRegAudioMixerMainInputLevelsPair5
@ kRegShiftPCMControlA1P3_4
virtual bool SetAnalogAudioIOConfiguration(const NTV2AnalogAudioIO inConfig)
#define NTV2_IS_AUDIO_MIXER_CHANNELS_1_OR_2(__p__)
virtual bool GetHDMIOutAudioChannels(NTV2HDMIAudioChannels &outValue)
@ kRegShiftPCMControlA8P3_4
@ NTV2_HDMIAudio8Channels
8 audio channels
virtual bool SetAudioMixerOutputGain(const ULWord inGainValue)
Sets the gain for the output of the Audio Mixer.
@ kRegShiftAud5PlayCapMode
@ NTV2_EMBEDDED_AUDIO_INPUT_INVALID
virtual bool SetAudioPlayCaptureModeEnable(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Enables or disables a special mode for the given Audio System whereby its embedder and deembedder bot...
@ kDeviceGetNumBufferedAudioSystems
The total number of audio systems on the device that can read/write audio buffer memory....
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
@ kRegShiftInputStartAtVBI
virtual bool IsAudioChannelPairPresent(const NTV2AudioSystem inAudioSystem, const NTV2AudioChannelPair inChannelPair, bool &outIsPresent)
Answers whether or not the given NTV2AudioChannelPair in the given NTV2AudioSystem on the device is p...
@ kRegMaskPCMControlA4P1_2
virtual bool GetAudioCaptureEnable(const NTV2AudioSystem inAudioSystem, bool &outEnable)
Answers whether or not the Audio System is configured to write captured audio samples into device aud...
static NTV2RegisterNumber GetNonPCMDetectRegisterNumber(const NTV2Channel inSDIInputChannel, const bool inIsExtended=(0))
@ kRegAudioMixerAux2GainCh2
virtual bool GetAudioMixerLevelsSampleCount(ULWord &outSampleCount)
Answers with the Audio Mixer's current sample count used for measuring audio levels.
virtual bool GetDetectedAudioChannelPairs(const NTV2AudioSystem inAudioSystem, NTV2AudioChannelPairs &outDetectedChannelPairs)
Answers which audio channel pairs are present in the given Audio System's input stream.
virtual bool GetSDIOutputDS2AudioSystem(const NTV2Channel inSDIOutputConnector, NTV2AudioSystem &outAudioSystem)
Answers with the device's Audio System that is currently providing audio for the given SDI output's a...
@ kRegMaskBOBAnalogInputSelect
NTV2EmbeddedAudioClock
This enum value determines/states the device audio clock reference source. It was important to set th...
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
#define NTV2_IS_EXTENDED_AUDIO_CHANNEL_PAIR(__p__)
ULWord NTV2DeviceGetHDMIVersion(const NTV2DeviceID inDeviceID)
virtual bool GetHDMIOutAudioSource8Channel(NTV2Audio8ChannelSelect &outValue, NTV2AudioSystem &outAudioSystem)
Answers with the HDMI output's current 8-channel audio source.
@ kRegShiftPCMControlA5P7_8
virtual bool SetAudioOutputEraseMode(const NTV2AudioSystem inAudioSystem, const bool &inEraseModeEnabled)
Enables or disables output erase mode for the given Audio System, which, when enabled,...
@ NTV2_AudioChannel9_10
This selects audio channels 9 and 10 (Group 3 channels 1 and 2)
virtual bool IsAudioInputRunning(const NTV2AudioSystem inAudioSystem, bool &outIsRunning)
Answers whether or not the capture side of the given NTV2AudioSystem is currently running.
@ kRegShiftPCMControlA4P11_12
@ kRegMaskAudioMixerInputLeftLevel
@ kRegShiftPCMControlA8P5_6
@ kRegMaskPCMControlA7P9_10
@ kRegMaskPCMControlA3P5_6
@ kRegShiftResetAudioOutput
@ kRegShiftPCMControlA2P3_4
@ kRegShiftPCMControlA2P11_12
virtual bool SetHDMIOutAudioChannels(const NTV2HDMIAudioChannels inNewValue)
@ kRegMaskPCMControlA2P15_16
@ NTV2_EMBEDDED_AUDIO_CLOCK_REFERENCE
Audio clock derived from the device reference.
@ kRegShiftPCMControlA2P9_10
@ NTV2_AnalogAudioIO_4Out_4In
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual bool GetNumberAudioChannels(ULWord &outNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current number of audio channels being captured or played by a given Audio System on the ...
@ kRegMaskPCMControlA4P5_6
@ kRegShiftAudioMixerInputRightLevel
@ kRegShiftPCMControlA3P13_14
@ kRegMaskPCMControlA1P9_10
@ kRegShiftPCMControlA6P15_16
@ kRegShiftPCMControlA5P1_2
virtual bool GetAudioOutputEraseMode(const NTV2AudioSystem inAudioSystem, bool &outEraseModeEnabled)
Answers with the current state of the audio output erase mode for the given Audio System....
@ kRegMaskPCMControlA3P7_8
@ kRegMaskInputStartAtVBI
@ kRegShiftEncodedAudioMode
virtual bool GetRawAudioTimer(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Answers with the current value of the 48kHz audio clock counter.
virtual bool GetAudioReadOffset(ULWord &outReadOffset, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset from the start of the audio buffer to the fi...
virtual bool GetAudioMixerOutputGain(ULWord &outGainValue)
Answers with the current gain setting for the Audio Mixer's output.
@ kRegShiftPCMControlA3P11_12
@ kRegMaskPCMControlA1P3_4
@ kRegMaskPCMControlA1P11_12
#define NTV2_IS_VALID_AUDIO_MIXER_INPUT(__p__)
@ kRegShiftPCMControlA7P9_10
std::set< NTV2AudioChannelPair > NTV2AudioChannelPairs
A set of distinct NTV2AudioChannelPair values.
@ kRegShiftPCMControlA3P3_4
@ kRegShiftPCMControlA6P11_12
@ kRegAudioMixerMainOutputLevelsPair5
@ kRegMaskAudioMixerLevelSampleCount
@ kRegFirstNonPCMAudioDetectRegister
virtual bool GetAESOutputSource(const NTV2Audio4ChannelSelect inAESAudioChannels, NTV2AudioSystem &outSrcAudioSystem, NTV2Audio4ChannelSelect &outSrcAudioChannels)
Answers with the current audio source for a given quad of AES audio output channels....
@ kRegShiftPCMControlA8P11_12
virtual bool GetInputAudioChannelPairsWithoutPCM(const NTV2Channel inSDIInputConnector, NTV2AudioChannelPairs &outChannelPairs)
For the given SDI input (specified as a channel number), returns the set of audio channel pairs that ...
@ kRegMaskPCMControlA7P15_16
std::set< NTV2AudioSystem > NTV2AudioSystemSet
A set of distinct NTV2AudioSystem values. New in SDK 16.2.
@ kRegShiftAudioMixerLevelSampleCount
@ kRegShiftPCMControlA1P11_12
@ kRegMaskPCMControlA5P15_16
Declares the CNTV2Card class.
@ kRegShiftEmbeddedOutputSupressCh1
@ kRegMaskHDMIOutAudio2ChannelSelect
@ NTV2_AudioMixerInputAux1
This selects the Audio Mixer's 1st Auxiliary input.
@ kRegShiftPCMControlA1P5_6
@ kRegShiftHDMIOutAudio2ChannelSelect
virtual bool GetAudioAnalogLevel(NTV2AudioLevel &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
@ kDeviceAudioCanWaitForVBI
True if device audio systems can wait for VBI before starting. (New in SDK 17.0)
static const ULWord gChannelToSDIOutControlRegNum[]
@ kRegMaskAud1PlayCapMode
NTV2RegWritesConstIter NTV2RegisterReadsConstIter
@ kRegShiftPCMControlA2P7_8
virtual bool GetAudioOutputDelay(const NTV2AudioSystem inAudioSystem, ULWord &outDelay)
Answers with the audio output delay for the given Audio System on the device.
virtual bool ReadAudioLastIn(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset to the last byte of the latest chunk of 4-by...
virtual bool GetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, NTV2AudioSystem &outAudioSystem)
Answers with the device's NTV2AudioSystem that is currently providing audio for the given SDI output'...
@ kRegShiftPCMControlA6P7_8
UWord NTV2DeviceGetNumVideoInputs(const NTV2DeviceID inDeviceID)
@ kRegMaskPCMControlA1P1_2
#define NTV2_AUDIO_READBUFFEROFFSET
virtual bool ReadAudioLastOut(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset of the tail end of the last chunk of audio s...
@ kRegAudioMixerInputSelects
NTV2AudioChannelPair
Identifies a pair of audio channels.
virtual bool GetAudioOutputEmbedderState(const NTV2Channel inSDIOutputConnector, bool &outIsEnabled)
Answers with the current state of the audio output embedder for the given SDI output connector (speci...
@ kRegShiftPCMControlA6P13_14
NTV2RegWrites NTV2RegisterReads
@ kRegAudioMixerMainInputLevelsPair7
virtual bool GetEmbeddedAudioClock(NTV2EmbeddedAudioClock &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given NTV2AudioSystem, answers with the current NTV2EmbeddedAudioClock setting.
@ kRegMaskPCMControlA3P9_10
@ kRegShiftPCMControlA3P7_8
@ kRegShiftPCMControlA5P13_14
@ NTV2_EMBEDDED_AUDIO_CLOCK_VIDEO_INPUT
Audio clock derived from the video input.
Declares numerous NTV2 utility functions.
@ kRegShiftPCMControlA7P3_4
virtual bool GetAnalogAudioTransmitEnable(const NTV2Audio4ChannelSelect inChannelQuad, bool &outEnabled)
Answers whether or not the specified bidirectional XLR audio connectors are collectively acting as in...
@ NTV2_AnalogAudioIO_4In_4Out
virtual bool ReadAudioSource(ULWord &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
@ kRegShiftMultiLinkAudio
@ NTV2_AUDIO_BUFFER_SIZE_1MB
@ kRegMaskPCMControlA2P1_2
virtual bool GetAudio20BitMode(const NTV2AudioSystem inAudioSystem, bool &outEnable)
Answers whether or not the device's NTV2AudioSystem is currently operating in 20-bit mode....
bool NTV2DeviceCanDoAudioDelay(const NTV2DeviceID inDeviceID)
virtual bool InputAudioChannelPairHasPCM(const NTV2Channel inSDIInputConnector, const NTV2AudioChannelPair inAudioChannelPair, bool &outIsPCM)
For the given SDI input (specified as a channel number), answers if the specified audio channel pair ...
@ kRegMaskPCMControlA1P15_16
@ kRegShiftEmbeddedOutputSupressCh2
@ kRegShiftPCMControlA4P15_16
@ kRegShiftPCMControlA7P13_14
@ NTV2_AUDIO_LOOPBACK_INVALID
virtual bool GetAudioOutputMonitorSource(NTV2AudioChannelPair &outChannelPair, NTV2AudioSystem &outAudioSystem)
Answers with the current audio monitor source. The audio output monitor is typically a pair of RCA ja...
virtual bool SetAudioOutputEmbedderState(const NTV2Channel inSDIOutputConnector, const bool &inEnable)
Enables or disables the audio output embedder for the given SDI output connector (specified as a chan...
virtual bool SetEncodedAudioMode(const NTV2EncodedAudioMode value, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
@ kRegShiftPCMControlA4P9_10
@ NTV2_AUDIO_BUFFER_SIZE_4MB
NTV2AudioLoopBack
This enum value determines/states if an audio output embedder will embed silence (zeroes) or de-embed...
@ kRegMaskAudioMixerMainInputEnable
PCM_CONTROL_INFO(ULWord regNum, ULWord mask, ULWord shift)
virtual bool GetEmbeddedAudioInput(NTV2EmbeddedAudioInput &outEmbeddedSource, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Answers with the device's current embedded (SDI) audio source for the given NTV2AudioSystem.
#define NTV2_IS_AUDIO_MIXER_INPUT_MAIN(__p__)
@ kRegAudioMixerMainInputLevelsPair0
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
@ kRegAudioMixerMainOutputLevelsPair3
@ kRegShiftPCMControlA4P5_6
@ kRegShiftAudioMixerMainInputSelect
virtual bool GetAudioMixerOutputChannelsMute(NTV2AudioChannelsMuted16 &outMutes)
Answers with a std::bitset that indicates which output audio channels of the Audio Mixer are currentl...
@ kRegShiftRotaryEncoderGain
@ kRegMaskPCMControlA4P11_12
@ kRegShiftAud7PlayCapMode
static const ULWord gAudioRateHighShift[]
virtual bool SetAudio20BitMode(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Enables or disables 20-bit mode for the NTV2AudioSystem.
@ kRegShiftAudioMixerMainInputEnable
virtual bool SetAudioOutputAESSyncModeBit(const NTV2AudioSystem inAudioSystem, const bool &inAESSyncModeBitSet)
Sets or clears the AES Sync Mode bit for the given Audio System's output.
@ kRegMaskPCMControlA7P11_12
static const ULWord sAudioMixerInputMuteShifts[]
@ kRegMaskPCMControlA4P15_16
struct NTV2RegInfo NTV2RegInfo
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
@ kRegMaskPCMControlA4P7_8
@ kRegShiftAud3PlayCapMode
virtual bool SetHDMIOutAudioRate(const NTV2AudioRate inNewValue)
Sets the HDMI output's audio rate.
#define NTV2_IS_VALID_AUDIO_LOOPBACK(_x_)
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_6
@ kRegShiftPCMControlA5P5_6
@ kRegMaskAud2PlayCapMode
#define NTV2_IS_VALID_CHANNEL(__x__)
virtual bool GetAnalogAudioIOConfiguration(NTV2AnalogAudioIO &outConfig)
virtual bool GetMultiLinkAudioMode(const NTV2AudioSystem inAudioSystem, bool &outEnabled)
Answers with the current multi-link audio mode for the given audio system.
@ kRegAudioMixerMainOutputLevelsPair4
#define NTV2_IS_VALID_AUDIO_SOURCE(_x_)
static const unsigned sAudioDetectGroups[]
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
#define NTV2_AUDIO_WRAPADDRESS
virtual bool GetAudioPlayCaptureModeEnable(const NTV2AudioSystem inAudioSystem, bool &outEnable)
Answers whether or not the device's Audio System is currently operating in a special mode in which it...
@ NTV2_AudioChannel5_8
This selects audio channels 5 thru 8.
@ kRegMaskResetAudioOutput
@ kRegMaskEmbeddedAudioClock
@ kRegMaskPCMControlA7P7_8
@ kRegAudioMixerMainInputLevelsPair6
@ kRegAudioMixerMainInputLevelsPair2
@ kRegAudioMixerChannelSelect
@ kVRegSuspendSystemAudio
@ kRegShiftPCMControlA6P3_4
virtual bool SetAudioMixerInputAudioSystem(const NTV2AudioMixerInput inMixerInput, const NTV2AudioSystem inAudioSystem)
Sets the Audio System that will drive the given input of the Audio Mixer.
@ kRegMaskAud3PlayCapMode
bool NTV2DeviceCanDoPCMDetection(const NTV2DeviceID inDeviceID)
@ kRegMaskPCMControlA1P13_14
@ kRegMaskHDMIOutSourceSelect
@ NTV2_AUDIO_AES
Obtain audio samples from the device AES inputs, if available.
static const ULWord gAudioDelayRegisterNumbers[]
@ kRegShiftPCMControlA4P3_4
@ NTV2_AUDIO_HDMI
Obtain audio samples from the device HDMI input, if available.
@ kRegMaskPCMControlA3P1_2
virtual bool GetHDMIOutAudioSource2Channel(NTV2AudioChannelPair &outValue, NTV2AudioSystem &outAudioSystem)
Answers with the HDMI output's current 2-channel audio source.
@ kRegMaskPCMControlA7P5_6
@ kRegShiftBOBAnalogLevelControl
virtual bool SetHDMIOutAudioSource2Channel(const NTV2AudioChannelPair inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the HDMI output's 2-channel audio source.
virtual bool GetAudioOutputPause(const NTV2AudioSystem inAudioSystem, bool &outIsPaused)
Answers if the device's Audio System is currently paused or not.
@ NTV2_AudioChannel9_16
This selects audio channels 9 thru 16.
@ kRegMaskOutputStartAtVBI
virtual bool WriteAudioSource(const ULWord inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual bool SetEmbeddedAudioInput(const NTV2EmbeddedAudioInput inEmbeddedSource, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the embedded (SDI) audio source for the given NTV2AudioSystem on the device.
@ kRegMaskPCMControlA5P3_4
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_7
virtual bool GetAudioLoopBack(NTV2AudioLoopBack &outMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Answers if NTV2AudioLoopBack mode is currently on or off for the given NTV2AudioSystem.
bool NTV2DeviceCanDoIP(const NTV2DeviceID inDeviceID)
Private include file for all ajabase sources.
NTV2AudioSystemSet::const_iterator NTV2AudioSystemSetConstIter
A handy const iterator into an NTV2AudioSystemSet. New in SDK 16.2.
@ kRegShiftAudioAutoErase
@ kRegAudioMixerAux2InputLevels
virtual bool GetDetectedAESChannelPairs(NTV2AudioChannelPairs &outDetectedChannelPairs)
Answers which AES/EBU audio channel pairs are present on the device.
@ NTV2_AUDIO_LOOPBACK_ON
Embeds SDI input source audio into the data stream.
bool NTV2DeviceCanDoAESAudioIn(const NTV2DeviceID inDeviceID)
@ kRegShiftResetAudioInput
@ kRegShiftPCMControlA1P7_8
std::bitset< 16 > NTV2AudioChannelsMuted16
Per-audio-channel mute state for up to 16 audio channels.
virtual bool SetAudioPCMControl(const NTV2AudioSystem inAudioSystem, const bool inIsNonPCM)
Determines whether or not all outgoing audio channel pairs are to be flagged as non-PCM for the given...
virtual bool GetAudioMixerInputChannelSelect(const NTV2AudioMixerInput inMixerInput, NTV2AudioChannelPair &outChannelPair)
Answers with the Audio Channel Pair that's currently driving the given input of the Audio Mixer.
@ kRegMaskEmbeddedAudioInput
@ kRegShiftPCMControlA5P11_12
@ kRegMaskPCMControlA2P3_4
@ NTV2_AUDIO_CHANNEL_QUAD_INVALID
virtual bool SetHeadphoneOutputGain(const ULWord inGainValue)
Sets the gain for the headphone out.
@ kRegMaskEncodedAudioMode
virtual bool SetAnalogAudioTransmitEnable(const NTV2Audio4ChannelSelect inChannelQuad, const bool inEnable)
Sets the specified bidirectional XLR audio connectors to collectively act as inputs or outputs.
@ kRegShiftPCMControlA1P1_2
bool NTV2DeviceCanDoBreakoutBoard(const NTV2DeviceID inDeviceID)
@ kRegMaskPCMControlA2P7_8
#define NTV2_IS_WITHIN_AUDIO_CHANNELS_1_TO_16(__p__)
static const unsigned gAESChannelMappingShifts[4]
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
@ kRegMaskPCMControlA6P13_14
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
@ kRegShiftPCMControlA1P9_10
virtual bool SetAESOutputSource(const NTV2Audio4ChannelSelect inAESAudioChannels, const NTV2AudioSystem inSrcAudioSystem, const NTV2Audio4ChannelSelect inSrcAudioChannels)
Changes the audio source for the given quad of AES audio output channels. By default,...
@ kRegMaskEmbeddedOutputSupressCh1
@ kRegShiftPCMControlA6P1_2
static const ULWord sAudioMixerInputGainCh2Regs[]
virtual bool SetAudioOutputPause(const NTV2AudioSystem inAudioSystem, const bool inPausePlayout)
Pauses or resumes output of audio samples and advancement of the audio buffer pointer ("play head") o...
#define NTV2_IS_NORMAL_AUDIO_CHANNEL_QUAD(__p__)
@ kRegShiftPCMControlA4P7_8
@ kRegMaskAnalogIOControl_14
@ kRegAudioMixerMainOutputLevelsPair1
virtual bool SetAudioMixerOutputChannelsMute(const NTV2AudioChannelsMuted16 inMutes)
Mutes or enables the individual output audio channels of the Audio Mixer.
@ kRegMaskPCMControlA6P9_10
bool NTV2DeviceCanDoStackedAudio(const NTV2DeviceID inDeviceID)
@ kRegShiftAudioMixerInputLeftLevel
virtual bool SetSDIOutputDS2AudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the Audio System that will supply audio for the given SDI output's audio embedder for Data Strea...
@ kRegMaskPCMControlA6P1_2
@ kRegMaskPCMControlA8P15_16
static const ULWord gAudioSystemToSrcSelectRegNum[]
virtual bool SetMultiLinkAudioMode(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Sets the multi-link audio mode for the given audio system.
@ kRegMaskResetAudioInput
@ kRegShiftAudioMixerAux1x2CHInput
@ kRegMaskPCMControlA8P11_12
@ kRegAudioMixerMainOutputLevelsPair7
@ kRegMaskPCMControlA8P1_2
@ NTV2_AudioChannel1_8
This selects audio channels 1 thru 8.
@ kRegMaskPCMControlA4P9_10
static const ULWord sAudioMixerInputMuteMasks[]
@ NTV2_AnalogAudioIO_8Out
virtual bool StartAudioOutput(const NTV2AudioSystem inAudioSystem, const bool inWaitForVBI=false)
Starts the playout side of the given NTV2AudioSystem, reading outgoing audio samples from the Audio S...
@ kRegMaskHDMIOut8ChGroupSelect
@ kRegMaskAudioMixerOutputChannelsMute
@ kRegMaskPCMControlA8P7_8
@ NTV2_AUDIO_BUFFER_INVALID
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
virtual bool SetAudioMixerInputGain(const NTV2AudioMixerInput inMixerInput, const NTV2AudioMixerChannel inChannel, const ULWord inGainValue)
Sets the gain for the given input of the Audio Mixer.
NTV2Audio4ChannelSelect
Identifies a contiguous, adjacent group of four audio channels.
@ kRegMaskEmbeddedOutputSupressCh2
bool NTV2DeviceHasRotaryEncoder(const NTV2DeviceID inDeviceID)
@ kRegShiftEmbeddedAudioInput2
@ kRegMaskAud4PlayCapMode
@ kRegMaskPCMControlA8P9_10
@ NTV2_AUDIOSYSTEM_5
This identifies the 5th Audio System.
virtual bool GetHeadphoneOutputGain(ULWord &outGainValue)
Answers with the current gain setting for the headphone out.
static const ULWord sAudioDetectRegs[]
@ kRegMaskPCMControlA2P11_12
virtual bool SetHDMIOutAudioSource8Channel(const NTV2Audio8ChannelSelect inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the HDMI output's 8-channel audio source.
@ kDeviceGetTotalNumAudioSystems
The total number of audio systems on the device, including host audio and mixer audio systems,...
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
@ NTV2_AUDIO_ANALOG
Obtain audio samples from the device analog input(s), if available.
@ kRegShiftPCMControlA2P13_14
@ kRegShiftPCMControlA7P1_2
static const ULWord gAudioSystemToAudioControlRegNum[]
@ kRegShiftPCMControlA8P1_2
@ NTV2_AudioChannel15_16
This selects audio channels 15 and 16 (Group 4 channels 3 and 4)
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
@ kRegAudioMixerMainInputLevelsPair3
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
UWord NTV2DeviceGetNumAESAudioOutputChannels(const NTV2DeviceID inDeviceID)
virtual bool GetSuspendHostAudio(bool &outIsSuspended)
Answers as to whether or not the host OS audio services for the AJA device (e.g. CoreAudio on MacOS) ...
@ kRegMaskEmbeddedAudioInput2
@ kRegShiftPCMControlA7P7_8
NTV2EmbeddedAudioInput
This enum value determines/states which SDI video input will be used to supply audio samples to an au...
bool GetBadRegions(ULWordSequence &outBlks) const
Answers with the list of colliding and illegal memory regions.
#define NTV2_IS_VALID_AUDIO_CHANNEL_PAIR(__p__)
@ kRegMaskPCMControlA5P9_10
virtual bool SetAudioInputDelay(const NTV2AudioSystem inAudioSystem, const ULWord inDelay)
Sets the audio input delay for the given Audio System on the device.
@ kRegAudioMixerMainOutputLevelsPair2
@ kRegShiftPCMControlA3P5_6
@ NTV2_AudioChannel1_4
This selects audio channels 1 thru 4.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
#define NTV2_IS_VALID_AUDIO_SYSTEM(__x__)
@ kRegShiftPCMControlA3P1_2
@ NTV2_AudioChannel1_2
This selects audio channels 1 and 2 (Group 1 channels 1 and 2)
static NTV2AudioChannelPairs BitMasksToNTV2AudioChannelPairs(const ULWord inBitMask, const ULWord inExtendedBitMask)
@ kRegMaskPCMControlA6P7_8
@ NTV2_AudioChannel9_12
This selects audio channels 9 thru 12.
virtual bool GetHDMIOutAudioFormat(NTV2AudioFormat &outValue)
Answers with the HDMI output's current audio format.
@ kK2RegMaskAudioBufferSize
NTV2Audio8ChannelSelect
Identifies a contiguous, adjacent group of eight audio channels.
virtual bool SetAudioCaptureEnable(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Enables or disables the writing of incoming audio into the given Audio System's capture buffer.
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_2
virtual bool GetAudioPCMControl(const NTV2AudioSystem inAudioSystem, bool &outIsNonPCM)
Answers whether or not all outgoing audio channel pairs are currently being flagged as non-PCM for th...
NTV2AudioSource
This enum value determines/states where an audio system will obtain its audio samples.
static const ULWord gChannelToAudioInLastAddrRegNum[]
virtual bool SetAudioAnalogLevel(const NTV2AudioLevel value, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
@ kRegShiftPCMControlA1P13_14
@ kRegShiftAudioMixerAux2x2CHInput
virtual bool SetHDMIOutAudioFormat(const NTV2AudioFormat inNewValue)
Sets the HDMI output's audio format.
@ kRegMaskAudioMixerInputRightLevel
#define NTV2_AUDIO_READBUFFEROFFSET_BIG
@ kRegMaskPCMControlA3P13_14
@ kRegShiftPCMControlA8P7_8
@ kRegShiftHDMIOutAudioSource
@ kRegShiftAudioMixerAux1InputEnable
bool AssessDevice(CNTV2Card &inDevice, const bool inIgnoreStoppedAudioBuffers=false)
Assesses the given device.
virtual bool GetAudioMixerInputLevels(const NTV2AudioMixerInput inMixerInput, const NTV2AudioChannelPairs &inChannelPairs, std::vector< uint32_t > &outLevels)
Answers with the Audio Mixer's current audio input levels.
bool NTV2DeviceCanDoPCMControl(const NTV2DeviceID inDeviceID)
@ kRegMaskPCMControlA5P7_8
NTV2AudioBufferSize
Represents the size of the audio buffer used by a device audio system for storing captured samples or...
@ NTV2_AUDIO_SOURCE_INVALID
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
virtual bool SetAudioMixerLevelsSampleCount(const ULWord inSampleCount)
Sets the Audio Mixer's sample count it uses for measuring audio levels.
@ kRegAudioMixerMainInputLevelsPair4
virtual bool GetAudioMixerInputChannelsMute(const NTV2AudioMixerInput inMixerInput, NTV2AudioChannelsMuted16 &outMutes)
Answers with a std::bitset that indicates which input audio channels of the given Audio Mixer input a...
NTV2AudioChannelPairs::const_iterator NTV2AudioChannelPairsConstIter
Handy const iterator to iterate over a set of distinct NTV2AudioChannelPair values.
Declares the AJADebug class.
@ kRegMaskAudioMixerAux2InputEnable
@ kRegMaskPCMControlA7P1_2
@ kRegMaskPCMControlA7P13_14
@ kRegMaskAud5PlayCapMode
@ kRegMaskPCMControlA7P3_4
static const ULWord gAudioPlayCaptureModeMasks[]
@ kRegShiftPCMControlA5P15_16
@ kRegShiftHDMIOutAudioRate
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_5
virtual bool GetAudioMixerInputGain(const NTV2AudioMixerInput inMixerInput, const NTV2AudioMixerChannel inChannel, ULWord &outGainValue)
Answers with the current gain setting for the Audio Mixer's given input.
@ kRegMaskAudioMixerAux1x2CHInput
@ kRegShiftPCMControlA8P15_16
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_3
@ NTV2_AUDIOSYSTEM_INVALID