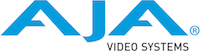 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
24 #define TCFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
25 #define TCWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
26 #define TCNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
27 #define TCINFO(_expr_) AJA_sINFO (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
28 #define TCDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
50 static const double gFrequencies [] = {250.0, 500.0, 1000.0, 2000.0};
52 static const double gAmplitudes [] = { 0.10, 0.15, 0.20, 0.25, 0.30, 0.35, 0.40, 0.45, 0.50, 0.55, 0.60, 0.65, 0.70, 0.75, 0.80, 0.85,
53 0.85, 0.80, 0.75, 0.70, 0.65, 0.60, 0.55, 0.50, 0.45, 0.40, 0.35, 0.30, 0.25, 0.20, 0.15, 0.10};
64 mToneFrequency (440.0),
91 while (mProducerThread.
Active())
94 while (mConsumerThread.
Active())
97 #if defined(NTV2_BUFFER_LOCKING)
99 #endif // NTV2_BUFFER_LOCKING
130 <<
" -- only supports Ch1" << (maxNumChannels > 1 ? string(
"-Ch") + string(1,
char(maxNumChannels+
'0')) :
"") << endl;
169 {cerr <<
"## ERROR: RenderTimeCodeFont failed for: " << mFormatDesc << endl;
return AJA_STATUS_UNSUPPORTED;}
174 if (mDevice.IsRemote())
175 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl;
177 #endif // defined(_DEBUG)
189 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
194 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
203 {cerr <<
"## ERROR: No CSC for channel " <<
DEC(mConfig.
fOutputChannel+1) <<
" to convert RGB pixel format" << endl;
235 {cerr <<
"## WARNING: HDR Anc requested, but device can't do custom anc" << endl;
258 numAudioChannels = 8;
310 if (mConfig.WithVideo())
316 #ifdef NTV2_BUFFER_LOCKING
322 if (mConfig.WithAudio())
328 #ifdef NTV2_BUFFER_LOCKING
332 mFrameDataRing.
Add (&frameData);
342 vector<NTV2TestPatternSelect> testPatIDs;
352 mTestPatRasters.clear();
353 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
358 if (mFormatDesc.IsVANC())
362 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
366 {
PLFAIL(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": "
373 if (!testPatternGen.
DrawTestPattern (testPatIDs.at(tpNdx), mFormatDesc, mTestPatRasters.at(tpNdx)))
375 cerr <<
"## ERROR: DrawTestPattern " <<
DEC(tpNdx) <<
" failed: " << mFormatDesc << endl;
379 #ifdef NTV2_BUFFER_LOCKING
382 PLWARN(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": failed to pre-lock");
396 const bool canVerify (mDevice.HasCanConnectROM());
397 UWord connectFailures (0);
408 if (!mDevice.
Connect (cscInputXpt, fsVidOutXpt, canVerify))
430 if (!mDevice.
Connect (cscInputXpt, fsVidOutXpt, canVerify))
450 if (mConfig.WithAudio())
466 PLWARN(
DEC(connectFailures) <<
" 'Connect' call(s) failed");
467 return connectFailures == 0;
491 mConsumerThread.
Start();
514 ULWord goodXfers(0), badXfers(0), starves(0), noRoomWaits(0);
538 uint32_t hdrPktSize (0);
543 PLWARN(
"HDR anc insertion disabled -- GenerateTransmitData failed");
552 if (pAncStrm->good())
569 #ifdef NTV2_BUFFER_LOCKING
570 if (outputXferInfo.acANCBuffer)
572 if (outputXferInfo.acANCField2Buffer)
580 {
PLFAIL(
"AutoCirculateInitForOutput failed"); mGlobalQuit =
true;}
581 else if (!mConfig.WithVideo())
603 {starves++;
continue;}
612 if (pAncStrm && pAncStrm->good() && outputXfer.
acANCBuffer)
640 PLNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
641 <<
DEC(starves) <<
" starves, " <<
DEC(noRoomWaits) <<
" VBI waits");
657 mProducerThread.
Start();
673 ULWord freqNdx(0), testPatNdx(0), badTally(0);
674 double timeOfLastSwitch (0.0);
699 const CRP188 rp188Info (mCurrentFrame++, 0, 0, 10, tcFormat);
710 if (isPAL) tcF2.
fHi |=
BIT(27);
else tcF2.
fLo |=
BIT(27);
719 TCDBG(
"F" <<
DEC0N(mCurrentFrame-1,6) <<
": " << tcF1 <<
": " << tcString);
727 if (currentTime > timeOfLastSwitch + 4.0)
730 testPatNdx = (testPatNdx + 1) %
ULWord(mTestPatRasters.size());
732 timeOfLastSwitch = currentTime;
733 PLINFO(
"F" <<
DEC0N(mCurrentFrame,6) <<
": " << tcString <<
": tone=" << mToneFrequency <<
"Hz, pattern='" << tpNames.at(testPatNdx) <<
"'");
740 PLNOTE(
"Thread completed: " <<
DEC(mCurrentFrame) <<
" frame(s) produced, " <<
DEC(badTally) <<
" failed");
757 pFrequencies[0] = (mToneFrequency / 2.0);
758 for (
ULWord chan(1); chan < numChannels; chan++)
761 pFrequencies[chan] = pFrequencies[chan-1] * 1.154782;
789 switch (inOutputDest)
813 if (regNum && mDevice.
ReadRegister(regNum, regValue) && regValue &
BIT(23))
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
virtual bool RouteOutputSignal(void)
Performs all widget/signal routing for playout.
virtual bool GetAncRegionOffsetFromBottom(ULWord &outByteOffsetFromBottom, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All)
Answers with the byte offset to the start of an ancillary data region within a device frame buffer,...
@ NTV2_TestPatt_CheckField
Declares the AJAAncillaryData_HDR_HLG class.
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
#define NTV2_IS_VALID_TASK_MODE(__m__)
NTV2OutputDestination
Identifies a specific video output destination.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
UWord firstFrame(void) const
bool HasBiDirectionalSDI(void)
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
virtual void StartConsumerThread(void)
Starts my consumer thread.
@ NTV2_TestPatt_MultiBurst
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
UWord GetNumAudioSystems(void)
#define IS_KNOWN_AJAAncDataType(_x_)
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
bool GetRP188Reg(RP188_STRUCT &outRP188) const
#define NTV2_VIDEO_FORMAT_HAS_PROGRESSIVE_PICTURE(__f__)
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
ULWord GetByteCount(void) const
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the producer thread's static callback function that gets called when the producer thread star...
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
static const uint32_t gAudMaxSizeBytes(256 *1024)
The maximum number of bytes of 48KHz audio that can be transferred for a single frame....
I play out SD or HD test pattern (with timecode) to an output of an AJA device with or without audio ...
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
bool Deallocate(void)
Deallocates my user-space storage (if I own it – i.e. from a prior call to Allocate).
@ NTV2_OUTPUTDESTINATION_SDI2
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
static const double gFrequencies[]
@ NTV2_OUTPUTDESTINATION_SDI3
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
Declares the NTV2TestPatternGen class.
virtual bool DisableChannel(const NTV2Channel inChannel)
Disables the given FrameStore.
@ NTV2_TestPatt_ColorBars100
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
#define DEC0N(__x__, __n__)
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
@ NTV2_AncRgn_Field2
Identifies the "normal" Field 2 ancillary data region.
UWord GetNumAnalogVideoOutputs(void)
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
#define NTV2_IS_ATC_VITC2_TIMECODE_INDEX(__x__)
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
#define NTV2_IS_2K_1080_VIDEO_FORMAT(__f__)
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
enum NTV2InputCrosspointID NTV2InputXptID
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
const char * NTV2FrameBufferFormatString(NTV2FrameBufferFormat fmt)
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
bool CanDoCustomAnc(void)
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
bool CanAcceptMoreOutputFrames(void) const
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
NTV2FrameRate
Identifies a particular video frame rate.
This class handles "5251" Frame Status Information packets.
bool CanDo3GLevelConversion(void)
NTV2Buffer & VideoBuffer(void)
AJAAncDataType fTransmitHDRType
Specifies the HDR anc data packet to transmit, if any.
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
UWord GetNumFrameStores(void)
virtual class DeviceCapabilities & features(void)
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
virtual bool DMABufferUnlockAll()
Unlocks all previously-locked buffers used for DMA transfers.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
virtual void StartProducerThread(void)
Starts my producer thread.
NTV2OutputDestination NTV2ChannelToOutputDestination(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its ordinary equivalent NTV2OutputDestination.
ULWord fLo
| BG 4 | Secs10 | BG 3 | Secs 1 | BG 2 | Frms10 | BG 1 | Frms 1 |
@ NTV2_OUTPUTDESTINATION_SDI1
Declares the AJAProcess class.
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
ULWord fHi
| BG 8 | Hrs 10 | BG 7 | Hrs 1 | BG 6 | Mins10 | BG 5 | Mins 1 |
UWord GetMaxAudioChannels(void)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
NTV2Standard
Identifies a particular video standard.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual bool GetNumberAudioChannels(ULWord &outNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current number of audio channels being captured or played by a given Audio System on the ...
UWord GetNumVideoOutputs(void)
@ NTV2_TestPatt_FlatField
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
ULWord VideoBufferSize(void) const
bool SetOutputTimeCodes(const NTV2TimeCodes &inValues)
Intended for playout, replaces the contents of my acOutputTimeCodes member.
static size_t SetDefaultPageSize(void)
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC=false, const bool inIsF2=false)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
virtual AJAStatus SetUpAudio(void)
Performs all audio setup.
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
virtual ~NTV2Player(void)
@ NTV2_OUTPUTDESTINATION_SDI6
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=false)
Connects the given widget signal input (sink) to the given widget signal output (source).
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
bool CopyFrom(const void *pInSrcBuffer, const ULWord inByteCount)
Replaces my contents from the given memory buffer, resizing me to the new byte count.
NTV2VideoFormat fVideoFormat
The video format to use.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
#define NTV2_IS_PAL_VIDEO_FORMAT(__f__)
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
NTV2Channel fOutputChannel
The device channel to use.
@ NTV2_OUTPUTDESTINATION_SDI8
std::string fAncDataFilePath
Optional path to Anc binary data file to playout.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
This class handles "5251" Frame Status Information packets.
NTV2Buffer & AudioBuffer(void)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=false, bool inRDMA=false)
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
bool IsVideoFormatA(const NTV2VideoFormat format)
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
@ NTV2_TestPatt_ColorBars75
UWord GetNumHDMIVideoOutputs(void)
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread star...
virtual bool IsDeviceReady(const bool inCheckValid=(0))
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
NTV2Buffer acANCField2Buffer
The host "Field 2" ancillary data buffer. This field is owned by the client application,...
uint16_t GetEndFrame(void) const
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
std::vector< std::string > NTV2StringList
virtual void ProduceFrames(void)
My producer thread that repeatedly produces video frames.
static const double gAmplitudes[]
@ NTV2_INPUT_CROSSPOINT_INVALID
virtual bool SetHDMIOutAudioRate(const NTV2AudioRate inNewValue)
Sets the HDMI output's audio rate.
This class handles "5251" Frame Status Information packets.
#define NTV2_OUTPUT_DEST_IS_SDI(_dest_)
bool AddAudioTone(ULWord &outNumBytesWritten, NTV2Buffer &inAudioBuffer, ULWord &inOutCurrentSample, const ULWord inNumSamples, const double inSampleRate, const double inAmplitude, const double inFrequency, const ULWord inNumBits, const bool inByteSwap, const ULWord inNumChannels)
Fills the given buffer with 32-bit (ULWord) audio tone samples.
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
The NTV2 test pattern generator.
virtual std::string GetDescription(void) const
NTV2Buffer fAudioBuffer
Host audio buffer.
virtual void DisableRP188Bypass(const NTV2OutputDestination inOutputDest)
Disables the given SDI output's RP188 bypass.
bool fTransmitLTC
If true, embed LTC; otherwise embed VITC.
virtual bool SetSDIOutputStandard(const UWord inOutputSpigot, const NTV2Standard inValue)
Sets the SDI output spigot's video standard.
@ NTV2_OUTPUTDESTINATION_HDMI
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
NTV2TCIndexes::const_iterator NTV2TCIndexesConstIter
A handy const interator for iterating over an NTV2TCIndexes set.
UWord lastFrame(void) const
Declares the AJAAncillaryData_HDR_HDR10 class.
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
virtual bool OutputDestHasRP188BypassEnabled(const NTV2OutputDestination inOutputDest)
std::string fDeviceSpec
The AJA device to use.
virtual AJAStatus SetUpTestPatternBuffers(void)
Creates my test pattern buffers.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual uint32_t AddTone(NTV2FrameData &inFrameData)
Inserts audio tone (based on my current tone frequency) into the given NTV2FrameData's audio buffer.
This struct replaces the old RP188_STRUCT.
static const ULWord gNumFrequencies(sizeof(gFrequencies)/sizeof(double))
ULWord GetRP188RegisterForOutput(const NTV2OutputDestination inOutputDest)
bool IsRGBFormat(const NTV2FrameBufferFormat format)
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
static ULWord gAncMaxSizeBytes(NTV2_ANCSIZE_MAX)
The maximum number of bytes of ancillary data that can be transferred for a single field....
ULWord GetAudioSamplesPerFrame(const NTV2FrameRate inFrameRate, const NTV2AudioRate inAudioRate, ULWord inCadenceFrame=0, bool inIsSMPTE372Enabled=false)
Returns the number of audio samples for a given video frame rate, audio sample rate,...
uint16_t GetStartFrame(void) const
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual AJAStatus GenerateTransmitData(uint8_t *pBuffer, const size_t inMaxBytes, uint32_t &outPacketSize)
Generates "raw" ancillary data from my internal ancillary data (playback) – see SDI Anc Buffer Data F...
virtual bool SetSDIOutputDS2AudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the Audio System that will supply audio for the given SDI output's audio embedder for Data Strea...
NTV2Player(const PlayerConfig &inConfig)
Constructs me using the given configuration settings.
@ NTV2_AudioChannel1_8
This selects audio channels 1 thru 8.
virtual void ConsumeFrames(void)
My consumer thread that repeatedly plays frames using AutoCirculate (until quit).
@ NTV2_OUTPUTDESTINATION_SDI7
virtual void Quit(void)
Gracefully stops me from running.
bool CanDoFrameStore1Display(void)
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
bool CanDoMultiFormat(void)
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
@ NTV2_OUTPUTDESTINATION_SDI4
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
double GetAudioSamplesPerSecond(const NTV2AudioRate inAudioRate)
Returns the audio sample rate as a number of audio samples per second.
virtual AJAStatus SetUpHostBuffers(void)
Sets up my host video & audio buffers.
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
@ NTV2_TestPatt_MultiPattern
Configures an NTV2Player instance.
bool fDoABConversion
If true, do level-A/B conversion; otherwise don't.
virtual bool SetHDMIOutAudioSource8Channel(const NTV2Audio8ChannelSelect inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the HDMI output's 8-channel audio source.
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
NTV2PixelFormat fPixelFormat
The pixel format to use.
enum NTV2OutputCrosspointID NTV2OutputXptID
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
static NTV2TestPatternNames getTestPatternNames(void)
virtual AJAStatus Run(void)
Runs me.
double FramesToSeconds(int64_t frames) const
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
@ NTV2_TestPatt_LineSweep
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
Declares the AJAAncillaryData_HDR_SDR class.
virtual AJAStatus Start()
virtual AJAStatus SetUpVideo(void)
Performs all video setup.
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
#define xHEX0N(__x__, __n__)
#define AJA_FAILURE(_status_)
@ NTV2_AUDIO_RATE_INVALID
static const bool BUFFER_PAGE_ALIGNED(true)
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
virtual bool SetHDMIOutAudioFormat(const NTV2AudioFormat inNewValue)
Sets the HDMI output's audio format.
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
NTV2Buffer acANCBuffer
The host ancillary data buffer. This field is owned by the client application, and thus is responsibl...
@ NTV2_OUTPUTDESTINATION_ANALOG
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
virtual void GetACStatus(AUTOCIRCULATE_STATUS &outStatus)
Provides status information about my output (playout) process.
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
@ NTV2_OUTPUTDESTINATION_SDI5
bool GetRP188Str(std::string &sRP188) const
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
@ AJAAncDataType_HDR_HDR10
NTV2TimeCodes fTimecodes
Map of TC indexes to NTV2_RP188 values.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID
Declares the AJATimeBase class.