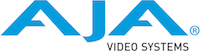 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
18 #if defined (AJAMac) || defined (AJALinux)
95 typedef pair <string, NTV2VideoFormat> String2VideoFormatPair;
96 typedef pair <string, NTV2FrameBufferFormat> String2PixelFormatPair;
97 typedef pair <string, NTV2AudioSystem> String2AudioSystemPair;
98 typedef pair <string, NTV2VANCMode> String2VANCModePair;
99 typedef pair <string, NTV2InputSource> String2InputSourcePair;
100 typedef pair <string, NTV2OutputDestination> String2OutputDestPair;
124 if (str.at (str.length () - 1) ==
'a')
125 str.erase (str.length () - 1);
127 if (str.find (
".00") != string::npos)
128 str.erase (str.find (
".00"), 3);
130 while (str.find (
" ") != string::npos)
131 str.erase (str.find (
" "), 1);
133 if (str.find (
".") != string::npos)
134 str.erase (str.find (
"."), 1);
211 while (str.find (
" ") != string::npos)
212 str.erase (str.find (
" "), 1);
214 while (str.find (
"-") != string::npos)
215 str.erase (str.find (
"-"), 1);
217 if (str.find (
"compatible") != string::npos)
218 str.erase (str.find (
"compatible"), 10);
220 if (str.find (
"ittle") != string::npos)
221 str.erase (str.find (
"ittle"), 5);
223 if (str.find (
"ndian") != string::npos)
224 str.erase (str.find (
"ndian"), 5);
231 if (str.find (
"NTV2_FBF_") == 0)
234 while (str.find (
" ") != string::npos)
235 str.erase (str.find (
" "), 1);
237 while (str.find (
"_") != string::npos)
238 str.erase (str.find (
"_"), 1);
283 for (uint8_t ndx (0); ndx < 8; ndx++)
356 string tpName(tpNames.at(tp));
359 ostringstream oss; oss <<
DEC(tp);
364 string colorName(*it);
371 string ULWordToString (
const ULWord inNum)
385 if (it != fTimecodes.end())
392 size_t errorCount(0);
413 size_t errorCount(0);
436 const string deviceSpec (inDeviceSpec.empty() ?
"0" : inDeviceSpec);
439 if (deviceSpec !=
"LIST" && deviceSpec !=
"list" && deviceSpec !=
"?")
440 cerr <<
"## ERROR: Device spec '" << deviceSpec <<
"' not valid" << endl;
456 strs.push_back(
"capture");
458 strs.push_back(
"playout");
460 strs.push_back(
"SDI");
462 strs.push_back(
"HDMI");
464 strs.push_back(
"analog video");
466 strs.push_back(
"IP/SFPs");
468 strs.push_back(
"Thunderbolt/PCMCIA");
470 strs.push_back(
"4K");
472 strs.push_back(
"12G SDI");
474 strs.push_back(
"6G SDI");
476 strs.push_back(
"custom Anc");
478 strs.push_back(
"SDI relays");
483 for (vector<string>::const_iterator it(strs.begin()); it != strs.end(); )
486 if (++it != strs.end())
495 ostringstream oss, hdr;
501 typedef map<NTV2DeviceID,UWord> DeviceTallies;
502 DeviceTallies tallies;
510 if (tallies.find(deviceID) == tallies.end())
512 tallies[deviceID] = 1;
518 const UWord num(tallies[deviceID]);
519 tallies.erase(deviceID);
520 tallies[deviceID] = num+1;
526 if (supportedDevices.find(deviceID) == supportedDevices.end())
527 oss <<
"\t## Doesn't support one of " << filterString;
532 return string(
"No devices\n");
533 hdr <<
DEC(ndx) <<
" local " << (ndx == 1 ?
"device" :
"devices") <<
" found:" << endl
534 << setw(16) << left <<
"Legal -d Values" << endl
535 << setw(16) << left <<
"----------------";
558 if (!inDeviceSpecifier.empty())
561 oss << setw(25) << left <<
"Video Format" <<
"\t" << setw(16) << left <<
"Legal -v Values" << endl
562 << setw(25) << left <<
"------------------------" <<
"\t" << setw(16) << left <<
"----------------" << endl;
567 if (*iter == it->second)
569 oss << setw(25) << left << formatName <<
"\t" << setw(16) << left << it->first;
610 if (!inDeviceSpecifier.empty ())
614 if (device.IsOpen ())
622 oss << setw (34) << left <<
"Frame Buffer Format" <<
"\t" << setw (32) << left <<
"Legal -p Values" << endl
623 << setw (34) << left <<
"----------------------------------" <<
"\t" << setw (32) << left <<
"--------------------------------" << endl;
628 if (*iter == it->second)
630 oss << setw (35) << left << formatName <<
"\t" << setw (25) << left << it->first;
632 oss <<
"\t## Incompatible with " << displayName;
690 if (!inDeviceSpecifier.empty ())
693 oss << setw (25) << left <<
"Input Source" <<
"\t" << setw (16) << left <<
"Legal -i Values" << endl
694 << setw (25) << left <<
"------------------------" <<
"\t" << setw (16) << left <<
"----------------" << endl;
699 if (*iter == it->second)
701 oss << setw (25) << left << sourceName <<
"\t" << setw (16) << left << it->first;
727 if (!inDeviceSpecifier.empty())
730 oss << setw (25) << left <<
"Output Destination" <<
"\t" << setw(16) << left <<
"Legal -o Values" << endl
731 << setw (25) << left <<
"------------------------" <<
"\t" << setw(16) << left <<
"----------------" << endl;
736 if (*iter == it->second)
738 oss << setw(25) << left << destName <<
"\t" << setw(16) << left << it->first;
781 const string inDeviceSpecifier,
782 const bool inIsInputOnly)
784 const NTV2TCIndexes & tcIndexes (GetSupportedTCIndexes (inKinds));
787 if (!inDeviceSpecifier.empty ())
790 oss << setw (25) << left <<
"Timecode Index" <<
"\t" << setw (16) << left <<
"Legal Values " << endl
791 << setw (25) << left <<
"------------------------" <<
"\t" << setw (16) << left <<
"----------------" << endl;
796 if (*iter == it->second)
798 oss << setw (25) << left << tcNdxName <<
"\t" << setw (16) << left << it->first;
799 if (!inDeviceSpecifier.empty () && theDevice.IsOpen ())
831 if (!inDeviceSpecifier.empty())
843 oss << setw(12) << left <<
"Audio System" << endl
844 << setw(12) << left <<
"------------" << endl;
845 for (
UWord ndx(0); ndx < 8; ndx++)
847 oss << setw(12) << left << (ndx+1);
848 if (!displayName.empty() && ndx >= numAudioSystems)
849 oss <<
"\t## Incompatible with " << displayName;
864 typedef map<string,string> NTV2StringMap;
869 if (keys.find(val) == keys.end())
873 NTV2StringMap legals;
874 for (NTV2StringSet::const_iterator kit(keys.begin()); kit != keys.end(); ++kit)
879 if (it->second == officialVM)
880 legalValues.push_back(it->first);
885 oss << setw(12) << left <<
"VANC Mode" <<
"\t" << setw(32) << left <<
"Legal --vanc Values " << endl
886 << setw(12) << left <<
"---------" <<
"\t" << setw(32) << left <<
"--------------------------------" << endl;
887 for (NTV2StringMap::const_iterator it(legals.begin()); it != legals.end(); ++it)
888 oss << setw(12) << left << it->first <<
"\t" << setw(32) << left << it->second << endl;
902 typedef map<string,string> NTV2StringMap;
905 if (keys.find(it->second) == keys.end())
906 keys.insert(it->second);
908 NTV2StringMap legals;
909 for (NTV2StringSet::const_iterator kit(keys.begin()); kit != keys.end(); ++kit)
911 const string & officialPatName(*kit);
914 if (it->second == officialPatName)
915 legalValues.push_back(it->first);
916 legals[officialPatName] =
aja::join(legalValues,
", ");
920 oss << setw(25) << left <<
"Test Pattern or Color " <<
"\t" << setw(22) << left <<
"Legal --pattern Values" << endl
921 << setw(25) << left <<
"------------------------" <<
"\t" << setw(22) << left <<
"----------------------" << endl;
922 for (NTV2StringMap::const_iterator it(legals.begin()); it != legals.end(); ++it)
923 oss << setw(25) << left << it->first <<
"\t" << setw(22) << left << it->second << endl;
930 string tpName(inStr);
939 string result(inStr);
946 string result (inStr);
947 while (result.find (
" ") != string::npos)
948 result.erase (result.find (
" "), 1);
949 while (result.find (
"00") != string::npos)
950 result.erase (result.find (
"00"), 2);
951 while (result.find (
".") != string::npos)
952 result.erase (result.find (
"."), 1);
960 #if defined (AJAMac) || defined (AJALinux)
961 struct termios terminalStatus;
962 ::memset (&terminalStatus, 0,
sizeof (terminalStatus));
963 if (::tcgetattr (0, &terminalStatus) < 0)
964 cerr <<
"tcsetattr()";
965 terminalStatus.c_lflag &= ~uint32_t(ICANON);
966 terminalStatus.c_lflag &= ~uint32_t(ECHO);
967 terminalStatus.c_cc[VMIN] = 1;
968 terminalStatus.c_cc[VTIME] = 0;
969 if (::tcsetattr (0, TCSANOW, &terminalStatus) < 0)
970 cerr <<
"tcsetattr ICANON";
971 if (::read (0, &result, 1) < 0)
972 cerr <<
"read()" << endl;
973 terminalStatus.c_lflag |= ICANON;
974 terminalStatus.c_lflag |= ECHO;
975 if (::tcsetattr (0, TCSADRAIN, &terminalStatus) < 0)
976 cerr <<
"tcsetattr ~ICANON" << endl;
977 #elif defined (MSWindows) || defined (AJAWindows)
978 HANDLE hdl (GetStdHandle (STD_INPUT_HANDLE));
981 PeekConsoleInput (hdl, &buffer, 1, &nEvents);
984 ReadConsoleInput (hdl, &buffer, 1, &nEvents);
985 result = char (buffer.Event.KeyEvent.wVirtualKeyCode);
994 cout <<
"## Press Enter/Return key to exit: ";
1003 switch (inFrameRate)
1024 switch (inFrameRate)
1028 #if !defined(NTV2_DEPRECATE_16_0)
1034 case NTV2_FRAMERATE_5000: return AJA_FrameRate_5000;
1101 static struct VideoFormatPair
1105 } VideoFormatPairs[] = {
1133 for (
size_t formatNdx(0); formatNdx <
sizeof(VideoFormatPairs) /
sizeof(VideoFormatPair); formatNdx++)
1134 if (VideoFormatPairs[formatNdx].vIn == inOutVideoFormat)
1136 inOutVideoFormat = VideoFormatPairs[formatNdx].vOut;
1145 static struct VideoFormatPair
1149 } VideoFormatPairs[] = {
1177 for (
size_t formatNdx(0); formatNdx <
sizeof(VideoFormatPairs) /
sizeof(VideoFormatPair); formatNdx++)
1178 if (VideoFormatPairs[formatNdx].vIn == inOutVideoFormat)
1180 inOutVideoFormat = VideoFormatPairs[formatNdx].vOut;
1197 for (
size_t ndx(0); ndx <
sizeof(s425MuxerIDs)/
sizeof(
NTV2WidgetID); ndx++)
1208 UWord tsiMux(firstFramestoreIndex);
1210 if (totFrameStores > totTSIMuxers)
1211 tsiMux = firstFramestoreIndex/2;
1212 else if (totFrameStores < totTSIMuxers)
1213 tsiMux = firstFramestoreIndex*2;
1214 for (
UWord num(0); num < inCount; num++)
1222 const bool isInputRGB)
1231 if (isInputRGB && !isFrameRGB)
1236 else if (!isInputRGB && isFrameRGB)
1244 return !conns.empty();
1252 const bool isInputRGB)
1254 UWord sdi(0), mux(0), csc(0), fb(0), path(0);
1263 if (isInputRGB == isFrameRGB)
1265 for (path = 0; path < 4; path++)
1276 else if (isInputRGB && !isFrameRGB)
1278 for (path = 0; path < 4; path++)
1296 for (path = 0; path < 4; path++)
1315 cerr <<
"## ERROR: Ch5678 must be for Corvid88, but no HDMI on that device" << endl;
1329 {fb = 4; sdi = fb; mux = fb / 2; csc = fb;}
1334 for (path = 0; path < 4; path++)
1352 for (path = 0; path < 4; path++)
1369 for (path = 0; path < 4; path++)
1383 for (path = 0; path < 4; path++)
1393 return !conns.empty();
1401 const bool isInputRGB)
1402 { (void)isInputRGB; (void) devID;
1403 UWord fb(0), path(0);
1422 for (path = 0; path < 4; path++)
1438 else if (isQuadQuadHFR)
1457 for (path = 0; path < 4; path++)
1480 return !conns.empty();
1509 if (hwPageSizeBytes != sdkPageSizeBytes)
1512 cerr <<
"## NOTE: Page size changed from " <<
DEC(sdkPageSizeBytes/1024) <<
"K to " <<
DEC(hwPageSizeBytes/1024) <<
"K" << endl;
1514 cerr <<
"## WARNING: Failed to change page size from " <<
DEC(sdkPageSizeBytes/1024) <<
"K to " <<
DEC(hwPageSizeBytes/1024) <<
"K" << endl;
1516 return hwPageSizeBytes;
1525 { ostringstream oss;
1534 mOtherArgs.push_back(
string(pStr));
1548 typedef struct {
string fName;
NTV2VideoFormat fFormat;} FormatNameDictionary;
1549 static const FormatNameDictionary sVFmtDict[] = {
1667 cout << endl << endl;
1668 for (
unsigned ndx(0); !sVFmtDict[ndx].fName.empty(); ndx++)
1670 const string & str (sVFmtDict[ndx].fName);
1674 if (vFormat != vFormat2)
1756 if (fDeviceSpec2.empty())
std::set< NTV2InputSource > NTV2InputSourceSet
A set of distinct NTV2InputSource values.
static NTV2StringList getColorNames(void)
NTV2TCIndexes NTV2TCIndexSet
An alias to NTV2TCIndexes.
@ NTV2_FORMAT_3840x2160p_6000
@ NTV2_FBF_10BIT_YCBCR_420PL3_LE
See 3-Plane 10-Bit YCbCr 4:2:0 ('I420_10LE' a.k.a. 'YUV-P420-L10').
unsigned long stoul(const std::string &str, std::size_t *idx, int base)
AJALabelValuePairs Get(const bool inCompact=false) const
Renders a human-readable representation of me.
@ NTV2_FORMAT_4x1920x1080p_6000
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
@ NTV2_FORMAT_1080psf_2398
virtual bool DMABufferUnlock(const NTV2Buffer &inBuffer)
Unlocks the given host buffer that was previously locked using CNTV2Card::DMABufferLock.
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
static NTV2InputSource GetInputSourceFromString(const std::string &inStr)
Returns the NTV2InputSource that matches the given string.
static bool SetDefaultPageSize(const size_t inNewSize)
Changes the default page size for use in future page-aligned allocations.
@ NTV2_FORMAT_4096x2160p_2400
static std::string GetVideoFormatStrings(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_NON_4KUHD, const std::string inDeviceSpecifier=std::string())
static const string gGlobalMutexName("com.aja.ntv2.mutex.demo")
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
@ AJA_PixelFormat_YCBCR10_422PL
@ NTV2_FBF_ARGB
See 8-Bit ARGB, RGBA, ABGR Formats.
NTV2OutputXptID GetSDIInputOutputXptFromChannel(const NTV2Channel inSDIInput, const bool inIsDS2=false)
@ NTV2_FBF_10BIT_YCBCR_420PL2
10-Bit 4:2:0 2-Plane YCbCr
String2PixelFormatMap::const_iterator String2PixelFormatMapConstIter
static NTV2TCIndexSet gTCIndexesVITC1
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
static String2OutputDestMap gString2OutputDestMap
@ NTV2_DEVICEKIND_OUTPUT
Specifies devices that output (playout).
NTV2FrameBufferFormatSet::const_iterator NTV2FrameBufferFormatSetConstIter
A handy const iterator for iterating over an NTV2FrameBufferFormatSet.
#define NTV2_IS_ATC_VITC1_TIMECODE_INDEX(__x__)
@ NTV2_DEVICEKIND_RELAYS
Specifies devices that have bypass relays.
@ NTV2_FORMAT_4x4096x2160p_4800
std::set< NTV2FrameBufferFormat > NTV2FrameBufferFormatSet
A set of distinct NTV2FrameBufferFormat values.
NTV2OutputDestination
Identifies a specific video output destination.
static NTV2InputSourceSet gInputSources
@ NTV2_FORMAT_4x4096x2160p_2398
@ AJA_PixelFormat_YCBCR8_422PL3
@ NTV2_FBF_12BIT_RGB_PACKED
See 12-Bit Packed RGB.
@ NTV2_DEVICEKIND_ANALOG
Specifies devices with analog video connectors.
static NTV2FrameBufferFormatSet GetSupportedPixelFormats(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL)
map< string, NTV2FrameBufferFormat > String2PixelFormatMap
NTV2InputXptID GetDLInInputXptFromChannel(const NTV2Channel inChannel, const bool inLinkB=false)
std::set< NTV2OutputDestination > NTV2OutputDestinations
A set of distinct NTV2OutputDestination values.
static const NTV2VideoFormatSet & GetSupportedVideoFormats(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_NON_4KUHD)
Declares device capability functions.
static NTV2InputSourceSet gInputSourcesHDMI
static NTV2VANCMode GetVANCModeFromString(const std::string &inStr)
std::set< std::string > NTV2StringSet
#define NTV2_IS_FBF_PLANAR(__s__)
static std::string GetOutputDestinationStrings(const std::string inDeviceSpecifier=std::string())
std::string & strip(std::string &str, const std::string &ws)
NTV2TestPatternSelect
Identifies a predefined NTV2 test pattern.
@ NTV2_FBF_PRORES_HDV
Apple ProRes HDV.
@ NTV2_FORMAT_4096x2160p_2500
#define NTV2_FBF_IS_RAW(__fbf__)
@ NTV2_FRAMERATE_1500
15 frames per second
std::set< NTV2TCIndex > NTV2TCIndexes
A set of distinct NTV2TCIndex values.
@ NTV2_FRAMERATE_6000
60 frames per second
NTV2DeviceIDSet NTV2GetSupportedDevices(const NTV2DeviceKinds inKinds=NTV2_DEVICEKIND_ALL)
Returns an NTV2DeviceIDSet of devices supported by the SDK.
#define NTV2_IS_FBF_PRORES(__fbf__)
static char ReadCharacterPress(void)
Returns the character that represents the last key that was pressed on the keyboard without waiting f...
@ NTV2_FORMAT_525psf_2997
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
static NTV2FrameBufferFormatSet gPixelFormats
@ NTV2_FORMAT_4x2048x1080p_4795
static NTV2VideoFormatSet g4KFormats
@ NTV2_FBF_10BIT_DPX_LE
10-Bit DPX Little-Endian
std::string join(const std::vector< std::string > &parts, const std::string &delim)
@ NTV2_FORMAT_3840x2160p_5994_B
#define NTV2_ASSERT(_expr_)
std::set< NTV2VideoFormat > NTV2VideoFormatSet
A set of distinct NTV2VideoFormat values.
@ NTV2_FRAMERATE_2997
Fractional rate of 30,000 frames per 1,001 seconds.
@ NTV2_FORMAT_1080p_2K_6000_B
@ NTV2_FORMAT_4096x2160p_5000_B
const char * poptGetArg(poptContext con)
@ NTV2_FBF_RGBA
See 8-Bit ARGB, RGBA, ABGR Formats.
@ AJA_PixelFormat_BGR8_PACK
@ NTV2_FORMAT_1080psf_2K_2398
#define NTV2_IS_QUAD_QUAD_HFR_VIDEO_FORMAT(__f__)
static std::string GetAudioSystemStrings(const std::string inDeviceSpecifier=std::string())
@ NTV2_FORMAT_1080p_2K_4800_A
pair< string, NTV2TCIndex > String2TCIndexPair
@ NTV2_FORMAT_4x2048x1080p_11988
@ NTV2_FRAMERATE_12000
120 frames per second
@ NTV2_FBF_48BIT_RGB
See 48-Bit RGB.
map< string, NTV2InputSource > String2InputSourceMap
static bool GetInputRouting(NTV2XptConnections &outConnections, const CaptureConfig &inConfig, const bool isInputRGB=false)
Answers with the crosspoint connections needed to implement the given capture configuration.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
AJALabelValuePairs Get(const bool inCompact=false) const
static String2TPNamesMap gString2TPNamesMap
const char * poptStrerror(const int error)
NTV2OutputDestinations::const_iterator NTV2OutputDestinationsConstIter
A handy const iterator for iterating over an NTV2OutputDestinations.
@ AJA_PixelFormat_PRORES_DVPRO
@ NTV2_FORMAT_4x1920x1080psf_3000
String2VANCModeMap::const_iterator String2VANCModeMapConstIter
NTV2PixelFormat fPixelFormat
Pixel format to use.
NTV2Channel NTV2OutputDestinationToChannel(const NTV2OutputDestination inOutputDest)
Converts a given NTV2OutputDestination to its equivalent NTV2Channel value.
NTV2EmbeddedAudioInput NTV2InputSourceToEmbeddedAudioInput(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2EmbeddedAudioInput value.
@ NTV2_FBF_8BIT_HDV
See 8-Bit HDV.
@ AJA_PixelFormat_YCBCR8_422PL
Declares the NTV2TestPatternGen class.
@ NTV2_FORMAT_1080p_2K_3000
map< string, NTV2TCIndex > String2TCIndexMap
NTV2InputXptID GetFrameBufferInputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsBInput=false)
@ NTV2_FORMAT_4x2048x1080p_2997
@ NTV2_TestPatt_ColorBars100
@ NTV2_FBF_10BIT_ARGB
10-Bit ARGB
@ NTV2_FBF_10BIT_YCBCRA
10-Bit YCbCrA
const std::string & AJAAncDataTypeToString(const AJAAncDataType inValue, const bool inCompact=true)
@ NTV2_FORMAT_4x4096x2160p_2500
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
@ NTV2_IOKINDS_HDMI
Specifies HDMI input/output kinds.
@ NTV2_FORMAT_4x4096x2160p_4795
@ NTV2_FORMAT_4x1920x1080p_2997
@ NTV2_FORMAT_4x1920x1080p_2500
@ NTV2_FORMAT_4x2048x1080p_4800
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ NTV2_FORMAT_4x3840x2160p_2500
#define NTV2_IS_ATC_VITC2_TIMECODE_INDEX(__x__)
#define NTV2_IS_ATC_LTC_TIMECODE_INDEX(__x__)
static NTV2FrameBufferFormatSet gFBFsProRes
@ NTV2_FBF_10BIT_DPX
See 10-Bit RGB - DPX Format.
@ NTV2_FORMAT_4x3840x2160p_5000_B
enum NTV2InputSourceKinds NTV2IOKinds
enum NTV2InputCrosspointID NTV2InputXptID
@ NTV2_OUTPUT_CROSSPOINT_INVALID
NTV2TimeCodes::const_iterator NTV2TimeCodesConstIter
A handy const interator for iterating over NTV2TCIndex/NTV2TimeCodeList pairs.
@ NTV2_IOKINDS_ANALOG
Specifies analog input/output kinds.
std::ostream & operator<<(std::ostream &ioStrm, const CaptureConfig &inObj)
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
bool NTV2DeviceCanDoFrameBufferFormat(const NTV2DeviceID inDeviceID, const NTV2FrameBufferFormat inFBFormat)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
@ NTV2_FORMAT_4x3840x2160p_2398
@ NTV2_FBF_24BIT_RGB
See 24-Bit RGB.
@ NTV2_FORMAT_4x4096x2160p_6000_B
@ AJA_PixelFormat_YCBCR8_420PL3
ULWord GetIndexForNTV2Channel(const NTV2Channel inChannel)
@ NTV2_FRAMERATE_2500
25 frames per second
@ NTV2_DEVICEKIND_HDMI
Specifies devices with HDMI connectors.
@ NTV2_FORMAT_1080p_2K_4795_A
static bool Get4KInputFormat(NTV2VideoFormat &inOutVideoFormat)
Given a video format, if all 4 inputs are the same and promotable to 4K, this function does the promo...
@ NTV2_FORMAT_3840x2160p_2500
NTV2FrameRate
Identifies a particular video frame rate.
map< string, string > String2TPNamesMap
@ NTV2_FORMAT_4096x2160p_6000_B
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
static NTV2TCIndex GetTCIndexFromString(const std::string &inStr)
Returns the NTV2TCIndex that matches the given string.
bool NTV2DeviceCanDoVideoFormat(const NTV2DeviceID inDeviceID, const NTV2VideoFormat inVideoFormat)
enum NTV2OutputDestination NTV2OutputDest
@ NTV2_FORMAT_4x4096x2160p_2997
@ NTV2_FRAMERATE_4800
48 frames per second
static NTV2VideoFormat GetVideoFormatFromString(const std::string &inStr, const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_NON_4KUHD)
Returns the NTV2VideoFormat that matches the given string.
#define NTV2_IS_4K_VIDEO_FORMAT(__f__)
static std::string GetDeviceStrings(const NTV2DeviceKinds inKinds=NTV2_DEVICEKIND_ALL)
static std::string GetTCIndexStrings(const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDeviceSpecifier=std::string(), const bool inIsInputOnly=true)
@ NTV2_FRAMERATE_2400
24 frames per second
static NTV2TCIndexSet gTCIndexesATCLTC
@ NTV2_FORMAT_4x2048x1080psf_2398
@ NTV2_FBF_10BIT_RGB_PACKED
10-Bit Packed RGB
NTV2TCIndexesConstIter NTV2TCIndexSetConstIter
An alias to NTV2TCIndexesConstIter.
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
pair< string, string > String2TPNamePair
@ NTV2_FORMAT_4x2048x1080p_2398
@ AJA_PixelFormat_RGB_DPX_LE
@ AJA_PixelFormat_YCbCr_DPX
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
#define NTV2_IS_ANALOG_TIMECODE_INDEX(__x__)
virtual std::string GetDisplayName(void)
Answers with this device's display name.
static bool IsValidDevice(const std::string &inDeviceSpec)
static NTV2VideoFormatSet gAllFormats
@ AJA_PixelFormat_RGB8_PACK
static size_t DefaultPageSize(void)
static NTV2AudioSystem GetAudioSystemFromString(const std::string &inStr)
Returns the NTV2AudioSystem that matches the given string.
@ NTV2_FORMAT_1080psf_2K_2500
String2TPNamesMap::const_iterator String2TPNamesMapConstIter
static std::string GetTestPatternStrings(void)
#define NTV2_IS_FBF_RGB(__fbf__)
@ NTV2_FORMAT_4x2048x1080psf_2400
std::string & lower(std::string &str)
@ NTV2_FBF_10BIT_RAW_RGB
10-Bit Raw RGB
std::string NTV2InputSourceToString(const NTV2InputSource inValue, const bool inForRetailDisplay=false)
Popt(const int inArgc, const char **pArgs, const PoptOpts *pInOptionsTable)
static NTV2TCIndexSet gTCIndexesVITC2
std::string NTV2TCIndexToString(const NTV2TCIndex inValue, const bool inCompactDisplay=false)
@ NTV2_FORMAT_1080p_5994_B
static NTV2FrameBufferFormatSet gFBFsRaw
@ NTV2_FORMAT_1080psf_3000_2
@ NTV2_FORMAT_1080p_2K_2400
enum _NTV2DeviceKinds NTV2DeviceKinds
These enum values are used for device selection/filtering.
@ NTV2_FORMAT_4x2048x1080p_3000
@ NTV2_DEVICEKIND_CUSTOM_ANC
Specifies devices that have custom Anc inserter/extractor firmware.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
@ NTV2_FORMAT_4096x2160p_4795
std::string & replace(std::string &str, const std::string &from, const std::string &to)
@ NTV2_FRAMERATE_2398
Fractional rate of 24,000 frames per 1,001 seconds.
static bool ConfigureAudioSystems(CNTV2Card &inDevice, const CaptureConfig &inConfig, const NTV2AudioSystemSet inAudioSystems)
Configures capture audio systems.
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
@ NTV2_FORMAT_4x4096x2160p_3000
bool NTV2DeviceCanDoWidget(const NTV2DeviceID inDeviceID, const NTV2WidgetID inWidgetID)
static AJALabelValuePairs & append(AJALabelValuePairs &inOutTable, const std::string &inLabel, const std::string &inValue=std::string())
A convenience function that appends the given label and value strings to the provided AJALabelValuePa...
Declares the CNTV2DeviceScanner class.
This class is used to configure an NTV2Capture instance.
static NTV2FrameBufferFormatSet gFBFsPacked
@ NTV2_FORMAT_4x2048x1080psf_3000
@ NTV2_DEVICEKIND_12G
Specifies devices that have 12G SDI connectors.
@ NTV2_FORMAT_1080p_2K_4795_B
@ AJA_PixelFormat_YCBCR10_420PL
@ NTV2_FRAMERATE_1498
Fractional rate of 15,000 frames per 1,001 seconds.
static size_t SetDefaultPageSize(void)
static NTV2FrameBufferFormat GetPixelFormatFromString(const std::string &inStr)
Returns the NTV2FrameBufferFormat that matches the given string.
NTV2_RP188 Timecode(const NTV2TCIndex inTCNdx) const
@ NTV2_FORMAT_4x3840x2160p_5994
@ NTV2_FORMAT_4096x2160p_2398
@ NTV2_INPUTSOURCE_INVALID
The invalid video input.
@ NTV2_DEVICEKIND_4K
Specifies devices that can do 4K video.
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
std::set< NTV2AudioSystem > NTV2AudioSystemSet
A set of distinct NTV2AudioSystem values. New in SDK 16.2.
@ NTV2_MAX_NUM_VIDEO_FORMATS
String2VideoFormatMap::const_iterator String2VideoFormatMapConstIter
NTV2TCIndex
These enum values are indexes into the capture/playout AutoCirculate timecode arrays.
map< string, NTV2AudioSystem > String2AudioSystemMap
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
static UWord GetNumTSIMuxers(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_4x2048x1080p_5000
@ NTV2_FBF_24BIT_BGR
See 24-Bit BGR.
virtual bool GetSerialNumberString(std::string &outSerialNumberString)
Answers with a string that contains my human-readable serial number.
@ AJA_PixelFormat_YCBCR10_422PL3LE
@ NTV2_FORMAT_4096x2160p_6000
std::string NTV2VANCModeToString(const NTV2VANCMode inValue, const bool inCompactDisplay=false)
@ AJA_PixelFormat_YCBCR10_420PL3LE
static NTV2ChannelList GetTSIMuxesForFrameStore(const NTV2DeviceID inDeviceID, const NTV2Channel in1stFrameStore, const UWord inCount)
static std::string GetVANCModeStrings(void)
static const NTV2TCIndexes GetSupportedTCIndexes(const NTV2TCIndexKinds inKinds)
@ NTV2_FORMAT_4x1920x1080p_3000
@ NTV2_FORMAT_4x3840x2160p_2997
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
Declares numerous NTV2 utility functions.
@ AJA_PixelFormat_RGB10_PACK
@ NTV2_FORMAT_1080p_5000_B
@ AJA_PixelFormat_RGB_DPX
poptContext poptFreeContext(poptContext con)
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
NTV2VideoFormatSet::const_iterator NTV2VideoFormatSetConstIter
A handy const iterator for iterating over an NTV2VideoFormatSet.
@ NTV2_FORMAT_4x3840x2160p_5000
@ NTV2_FORMAT_1080p_2K_5994_A
#define NTV2_INPUT_SOURCE_IS_HDMI(_inpSrc_)
@ NTV2_MAX_NUM_TIMECODE_INDEXES
static const DemoCommonInitializer gInitializer
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
I interrogate and control an AJA video/audio capture/playout device.
@ NTV2_FORMAT_4x2048x1080p_12000
@ NTV2_DEVICEKIND_ALL
Specifies any/all devices.
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=false, bool inRDMA=false)
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
UWord NTV2DeviceGetNumFrameStores(const NTV2DeviceID inDeviceID)
@ NTV2_DEVICEKIND_INPUT
Specifies devices that input (capture).
@ NTV2_FBF_10BIT_YCBCR_422PL2
10-Bit 4:2:2 2-Plane YCbCr
std::pair< NTV2InputXptID, NTV2OutputXptID > NTV2Connection
This links an NTV2InputXptID and an NTV2OutputXptID.
@ NTV2_FORMAT_625psf_2500
static void WaitForEnterKeyPress(void)
Prompts the user (via stdout) to press the Return or Enter key, then waits for it to happen.
@ NTV2_AUDIO_BUFFER_SIZE_4MB
@ NTV2_DEVICEKIND_EXTERNAL
Specifies external devices (e.g. Thunderbolt).
@ NTV2_FORMAT_1080p_2K_2500
@ NTV2_FORMAT_4x4096x2160p_4800_B
@ NTV2_FORMAT_4x1920x1080psf_2997
static std::string GetTestPatternNameFromString(const std::string &inStr)
@ NTV2_FORMAT_1080p_6000_A
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
NTV2OutputXptID GetDLInOutputXptFromChannel(const NTV2Channel inDLInput)
@ NTV2_FRAMERATE_11988
Fractional rate of 120,000 frames per 1,001 seconds.
static string DeviceFilterString(const NTV2DeviceKinds inKinds)
@ NTV2_FORMAT_1080p_2K_2398
@ NTV2_FORMAT_3840x2160p_2997
@ NTV2_FORMAT_4096x2160p_4800
enum _NTV2PixelFormatKinds NTV2PixelFormatKinds
std::vector< std::string > NTV2StringList
static String2VANCModeMap gString2VANCModeMap
NTV2VANCMode
These enum values identify the available VANC modes.
@ NTV2_INPUT_CROSSPOINT_INVALID
bool NTV2DeviceCanDoOutputDestination(const NTV2DeviceID inDeviceID, const NTV2OutputDestination inOutputDest)
@ NTV2_FORMAT_1080psf_2K_2400
@ AJA_PixelFormat_YCbCrA10
#define NTV2_OUTPUT_DEST_IS_SDI(_dest_)
static NTV2VideoFormatSet gNon4KFormats
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
@ NTV2_FORMAT_3840x2160p_5994
@ NTV2_FORMAT_4x4096x2160p_2400
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
@ NTV2_FBF_8BIT_YCBCR_YUY2
See Alternate 8-Bit YCbCr ('YUY2').
NTV2Channel fInputChannel
The device channel to use.
static NTV2OutputDestination GetOutputDestinationFromString(const std::string &inStr)
Returns the NTV2OutputDestination that matches the given string.
@ NTV2_FORMAT_4x4096x2160p_6000
std::vector< NTV2Channel > NTV2ChannelList
An ordered sequence of NTV2Channel values.
@ NTV2_FORMAT_3840x2160p_2398
@ AJA_PixelFormat_PRORES_HDV
NTV2InputSource
Identifies a specific video input source.
static String2VideoFormatMap gString2VideoFormatMap
static NTV2OutputDestinations gOutputDestinations
@ NTV2_DEVICEKIND_SDI
Specifies devices with SDI connectors.
#define NTV2_IS_QUAD_QUAD_FORMAT(__f__)
@ NTV2_FORMAT_4x1920x1080psf_2398
bool NTV2DeviceCanDo12gRouting(const NTV2DeviceID inDeviceID)
poptContext poptGetContext(const char *name, int argc, const char **argv, const struct poptOption *options, unsigned int flags)
@ NTV2_FORMAT_1080p_2K_6000_A
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
String2InputSourceMap::const_iterator String2InputSourceMapConstIter
@ NTV2_FBF_PRORES_DVCPRO
Apple ProRes DVC Pro.
static NTV2VideoFormatSet g8KFormats
@ VIDEO_FORMATS_NON_4KUHD
@ NTV2_DEVICEKIND_SFP
Specifies devices with SFP connectors.
@ NTV2_FORMAT_3840x2160p_5000_B
@ NTV2_FORMAT_4096x2160p_3000
@ NTV2_FORMAT_4x1920x1080psf_2500
@ NTV2_FRAMERATE_5994
Fractional rate of 60,000 frames per 1,001 seconds.
@ NTV2_OUTPUTDESTINATION_HDMI
int poptGetNextOpt(poptContext con)
This file contains some structures, constants, classes and functions that are used in some of the dem...
Private include file for all ajabase sources.
AJALabelValuePairs Get(const bool inCompact=false) const
Renders a human-readable representation of me.
@ NTV2_FORMAT_4x3840x2160p_2400
static bool GetDeviceAtIndex(const ULWord inDeviceIndexNumber, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device having the given zero-bas...
NTV2AudioSystemSet::const_iterator NTV2AudioSystemSetConstIter
A handy const iterator into an NTV2AudioSystemSet. New in SDK 16.2.
@ NTV2_FORMAT_4x4096x2160p_5000_B
@ AJA_PixelFormat_Unknown
NTV2TCIndexes::const_iterator NTV2TCIndexesConstIter
A handy const interator for iterating over an NTV2TCIndexes set.
@ NTV2_FBF_10BIT_RAW_YCBCR
See 10-Bit Raw YCbCr (CION).
@ NTV2_DEVICEKIND_6G
Specifies devices that have 6G SDI connectors.
static std::string GetInputSourceStrings(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDeviceSpecifier=std::string())
@ NTV2_OUTPUTDESTINATION_INVALID
map< string, NTV2VideoFormat > String2VideoFormatMap
@ NTV2_FORMAT_4x2048x1080p_2500
@ NTV2_FORMAT_4x1920x1080p_5994
@ NTV2_FORMAT_4x4096x2160p_5000
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
@ NTV2_FORMAT_4x3840x2160p_6000
bool fDoTSIRouting
If true, do TSI routing; otherwise squares.
bool NTV2DeviceGetSupportedOutputDests(const NTV2DeviceID inDeviceID, NTV2OutputDestinations &outOutputDests, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL)
Returns a set of distinct NTV2OutputDest values supported on the given device.
bool NTV2DeviceCanDoInputSource(const NTV2DeviceID inDeviceID, const NTV2InputSource inInputSource)
@ AJA_PixelFormat_YCbCr10
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
NTV2OutputXptID GetTSIMuxOutputXptFromChannel(const NTV2Channel inTSIMuxer, const bool inLinkB=false, const bool inIsRGB=false)
@ NTV2_FBF_10BIT_YCBCR_DPX
See 10-Bit YCbCr - DPX Format.
NTV2InputSourceSet::const_iterator NTV2InputSourceSetConstIter
A handy const iterator for iterating over an NTV2InputSourceSet.
This struct replaces the old RP188_STRUCT.
@ NTV2_FBF_10BIT_YCBCR_422PL3_LE
See 3-Plane 10-Bit YCbCr 4:2:2 ('I422_10LE' a.k.a. 'YUV-P-L10').
bool IsRGBFormat(const NTV2FrameBufferFormat format)
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
@ NTV2_FRAMERATE_UNKNOWN
Represents an unknown or invalid frame rate.
static string ToLower(const string &inStr)
@ NTV2_FORMAT_1080p_5000_A
@ NTV2_FORMAT_4x2048x1080p_6000
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ NTV2_FORMAT_4096x2160p_5000
static NTV2TCIndexSet gTCIndexesAnalog
@ NTV2_FORMAT_4x1920x1080p_5000
@ NTV2_FBF_8BIT_YCBCR_420PL3
See 3-Plane 8-Bit YCbCr 4:2:0 ('I420' a.k.a. 'YUV-P420').
@ NTV2_FORMAT_4x1920x1080p_2398
bool NTV2DeviceGetSupportedInputSources(const NTV2DeviceID inDeviceID, NTV2InputSourceSet &outInputSources, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL)
Returns a set of distinct NTV2InputSource values supported on the given device.
static NTV2InputSourceSet gInputSourcesSDI
@ NTV2_FORMAT_1080p_6000_B
@ NTV2_FORMAT_4x4096x2160p_5994_B
@ NTV2_IOKINDS_ALL
Specifies any/all input/output kinds.
static String2AudioSystemMap gString2AudioSystemMap
std::set< NTV2DeviceID > NTV2DeviceIDSet
A set of NTV2DeviceIDs.
@ NTV2_FORMAT_4096x2160p_2997
virtual NTV2DeviceID GetDeviceID(void)
@ NTV2_FBF_8BIT_DVCPRO
See 8-Bit DVCPro.
static NTV2StringList gTestPatternNames
@ NTV2_FORMAT_4096x2160p_5994_B
@ NTV2_FORMAT_4x3840x2160p_5994_B
@ NTV2_FORMAT_1080p_5994_A
@ NTV2_FORMAT_4x3840x2160p_6000_B
@ NTV2_FBF_8BIT_YCBCR_422PL2
8-Bit 4:2:2 2-Plane YCbCr
NTV2OutputXptID GetInputSourceOutputXpt(const NTV2InputSource inInputSource, const bool inIsSDI_DS2=false, const bool inIsHDMI_RGB=false, const UWord inHDMI_Quadrant=0)
static bool GetInputRouting8K(NTV2XptConnections &outConnections, const CaptureConfig &inConfig, const NTV2VideoFormat inVidFormat, const NTV2DeviceID inDevID=DEVICE_ID_INVALID, const bool isInputRGB=false)
Answers with the crosspoint connections needed to implement the given 8K/UHD2 capture configuration.
bool NTV2DeviceCanDoInputTCIndex(const NTV2DeviceID inDeviceID, const NTV2TCIndex inTCIndex)
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDeviceSpecifier=std::string())
@ NTV2_FORMAT_4x2048x1080p_5994
static NTV2FrameBufferFormatSet gFBFsRGB
NTV2StringList::const_iterator NTV2StringListConstIter
@ NTV2_DEVICEKIND_NONE
Doesn't specify any kind of device.
bool UnlockAll(CNTV2Card &inDevice)
static NTV2TCIndexSet gTCIndexesSDI
@ NTV2_FORMAT_4x3840x2160p_3000
@ NTV2_FORMAT_1080p_2K_5994_B
static String2InputSourceMap gString2InputSourceMap
@ NTV2_FBF_16BIT_ARGB
16-Bit ARGB
Configures an NTV2Player instance.
std::string NTV2ChannelToString(const NTV2Channel inValue, const bool inForRetailDisplay=false)
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
@ NTV2_IOKINDS_SDI
Specifies SDI input/output kinds.
enum NTV2OutputCrosspointID NTV2OutputXptID
@ NTV2_FBF_8BIT_YCBCR_422PL3
See 3-Plane 8-Bit YCbCr 4:2:2 (Weitek 'Y42B' a.k.a. 'YUV-P8').
static NTV2TestPatternNames getTestPatternNames(void)
@ NTV2_FORMAT_3840x2160p_5000
static const NTV2InputSourceSet GetSupportedInputSources(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL)
bool LockAll(CNTV2Card &inDevice)
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
@ NTV2_FORMAT_1080p_2K_5000_A
@ NTV2_FRAMERATE_5000
50 frames per second
@ NTV2_FORMAT_4x4096x2160p_4795_B
static size_t HostPageSize(void)
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
@ NTV2_FORMAT_4x2048x1080psf_2500
@ NTV2_FBF_10BIT_RGB
See 10-Bit RGB Format.
static NTV2FrameBufferFormatSet gFBFsAlpha
static std::string StripFormatString(const std::string &inStr)
static const char * GetGlobalMutexName(void)
const char * poptBadOption(poptContext con, unsigned int flags)
@ NTV2_FRAMERATE_4795
Fractional rate of 48,000 frames per 1,001 seconds.
std::string to_string(bool val)
@ NTV2_FRAMERATE_3000
30 frames per second
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
bool NTV2DeviceCanDoTCIndex(const NTV2DeviceID inDeviceID, const NTV2TCIndex inTCIndex)
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
@ NTV2_FORMAT_1080p_2K_5000_B
static String2PixelFormatMap gString2PixelFormatMap
static bool Get8KInputFormat(NTV2VideoFormat &inOutVideoFormat)
Given a video format, if all 4 inputs are the same and promotable to 8K, this function does the promo...
@ NTV2_FORMAT_1080psf_2997_2
map< string, NTV2OutputDestination > String2OutputDestMap
String2AudioSystemMap::const_iterator String2AudioSystemMapConstIter
@ NTV2_FORMAT_4x4096x2160p_5994
@ NTV2_FORMAT_4x2048x1080p_2400
static NTV2FrameBufferFormatSet gFBFsPlanar
@ NTV2_FORMAT_1080p_2K_4800_B
@ NTV2_FORMAT_4x1920x1080p_2400
@ NTV2_FORMAT_4x1920x1080psf_2400
enum _NTV2VideoFormatKinds NTV2VideoFormatKinds
static NTV2TCIndexSet gTCIndexesHDMI
@ NTV2_FORMAT_3840x2160p_6000_B
@ NTV2_FORMAT_1080psf_2500_2
enum _NTV2TCIndexKinds NTV2TCIndexKinds
@ NTV2_FORMAT_4096x2160p_4795_B
NTV2InputXptID GetTSIMuxInputXptFromChannel(const NTV2Channel inTSIMuxer, const bool inLinkB=false)
String2OutputDestMap::const_iterator String2OutputDestMapConstIter
static NTV2InputSourceSet gInputSourcesAnalog
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
@ NTV2_FORMAT_4096x2160p_4800_B
@ NTV2_FORMAT_3840x2160p_2400
map< string, NTV2VANCMode > String2VANCModeMap
@ NTV2_FBF_ABGR
See 8-Bit ARGB, RGBA, ABGR Formats.
NTV2InputSource fInputSource
The device input connector to use.
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
#define NTV2_IS_VALID_VIDEO_FORMAT(__f__)
static String2TCIndexMap gString2TCIndexMap
@ NTV2_FORMAT_3840x2160p_3000
@ NTV2_FORMAT_1080psf_2400
@ AJA_PixelFormat_YCBCR8_420PL
String2TCIndexMap::const_iterator String2TCIndexMapConstIter
@ NTV2_FORMAT_4096x2160p_5994
#define NTV2_FBF_HAS_ALPHA(__fbf__)
static bool GetInputRouting4K(NTV2XptConnections &outConnections, const CaptureConfig &inConfig, const NTV2DeviceID inDevID=DEVICE_ID_INVALID, const bool isInputRGB=false)
Answers with the crosspoint connections needed to implement the given 4K/UHD capture configuration.
static NTV2TCIndexSet gTCIndexes
const char * NTV2VideoFormatString(NTV2VideoFormat fmt)
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_FORMAT_4x2048x1080psf_2997
std::string NTV2OutputDestinationToString(const NTV2OutputDestination inValue, const bool inForRetailDisplay=false)
@ NTV2_AUDIOSYSTEM_INVALID
@ NTV2_FORMAT_1080p_2K_2997