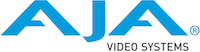 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
22 #define TCFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
23 #define TCWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
24 #define TCNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
25 #define TCINFO(_expr_) AJA_sINFO (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
26 #define TCDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
49 static const double gFrequencies [] = {250.0, 500.0, 1000.0, 2000.0};
51 static const double gAmplitudes [] = { 0.10, 0.15, 0.20, 0.25, 0.30, 0.35, 0.40, 0.45, 0.50, 0.55, 0.60, 0.65, 0.70, 0.75, 0.80, 0.85,
52 0.85, 0.80, 0.75, 0.70, 0.65, 0.60, 0.55, 0.50, 0.45, 0.40, 0.35, 0.30, 0.25, 0.20, 0.15, 0.10};
62 mToneFrequency (440.0),
94 delete [] mDolbyBuffer;
100 delete [] mBurstBuffer;
112 while (mProducerThread.
Active())
115 while (mConsumerThread.
Active())
122 #if defined(NTV2_BUFFER_LOCKING)
124 #endif // NTV2_BUFFER_LOCKING
144 if (!mDevice.
features().CanDoPlayback())
146 if (!mDevice.
features().GetNumHDMIVideoOutputs())
149 const UWord maxNumChannels (mDevice.
features().GetNumFrameStores());
157 <<
" -- only supports Ch1" << (maxNumChannels > 1 ? string(
"-Ch") + string(1,
char(maxNumChannels+
'0')) :
"") << endl;
169 if (mDevice.
features().CanDoMultiFormat())
178 {cerr <<
"## ERROR: Could not open file: " << mConfig.
fDolbyFilePath << endl;
return status;}
204 {cerr <<
"## ERROR: RenderTimeCodeFont failed for: " << mFormatDesc << endl;
return AJA_STATUS_UNSUPPORTED;}
209 if (mDevice.IsRemote())
210 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl;
212 #endif // defined(_DEBUG)
225 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
230 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
267 const uint16_t numberOfAudioChannels (8);
270 if (mDevice.
features().GetNumAudioSystems() > 1)
274 if (!mDevice.
features().CanDoFrameStore1Display())
319 if (mConfig.WithVideo())
325 #ifdef NTV2_BUFFER_LOCKING
331 if (mConfig.WithAudio())
334 PLFAIL(
"Failed to allocate " <<
xHEX0N(AUDIOBYTES_MAX,8) <<
"-byte audio buffer");
337 #ifdef NTV2_BUFFER_LOCKING
341 mFrameDataRing.
Add (&frameData);
344 if (mDolbyFileIO.
IsOpen())
347 mBurstSamples = 6144;
348 mBurstMax = mBurstSamples * 2;
349 mBurstBuffer =
new uint16_t [mBurstMax];
350 mDolbyBuffer =
new uint16_t [mBurstMax];
359 vector<NTV2TestPatternSelect> testPatIDs;
369 mTestPatRasters.clear();
370 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
375 if (mFormatDesc.IsVANC())
379 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
383 {
PLFAIL(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": "
390 if (!testPatternGen.
DrawTestPattern (testPatIDs.at(tpNdx), mFormatDesc, mTestPatRasters.at(tpNdx)))
392 cerr <<
"## ERROR: DrawTestPattern " <<
DEC(tpNdx) <<
" failed: " << mFormatDesc << endl;
396 #ifdef NTV2_BUFFER_LOCKING
399 PLWARN(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": failed to pre-lock");
440 mConsumerThread.
Start ();
461 ULWord goodXfers(0), badXfers(0), starves(0), noRoomWaits(0);
473 {
PLFAIL(
"AutoCirculateInitForOutput failed"); mGlobalQuit =
true;}
474 else if (!mConfig.WithVideo())
497 {starves++;
continue;}
529 PLNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
530 <<
DEC(starves) <<
" starves, " <<
DEC(noRoomWaits) <<
" VBI waits");
544 mProducerThread.
Start();
561 ULWord freqNdx(0), testPatNdx(0), badTally(0);
562 double timeOfLastSwitch (0.0);
589 const CRP188 rp188Info (mCurrentFrame++, 0, 0, 10, tcFormat);
598 TCDBG(
"F" <<
DEC0N(mCurrentFrame-1,6) <<
": " << tcF1 <<
": " << tcString);
603 if (mDolbyFileIO.
IsOpen())
616 if (currentTime > timeOfLastSwitch + 4.0)
619 testPatNdx = (testPatNdx + 1) %
ULWord(mTestPatRasters.size());
621 timeOfLastSwitch = currentTime;
622 PLINFO(
"F" <<
DEC0N(mCurrentFrame,6) <<
": " << tcString <<
": tone=" << mToneFrequency <<
"Hz, pattern='" << tpNames.at(testPatNdx) <<
"'");
629 PLNOTE(
"Thread completed: " <<
DEC(mCurrentFrame) <<
" frame(s) produced, " <<
DEC(badTally) <<
" failed");
651 pFrequencies [0] = (mToneFrequency / 2.0);
652 for (
ULWord chan (1); chan < numChannels; chan++)
655 pFrequencies [chan] = pFrequencies [chan - 1] * 1.154782;
690 for (uint32_t samp(0); samp < numSamples; samp++)
692 for (uint32_t ch(0); ch < numChannels; ch++)
694 *audioBuffer = uint32_t(mRampSample) << 16;
700 return numSamples * numChannels * 4;
703 #if defined(DOLBY_FULL_PARSER)
720 if (!mDolbyFileIO.
IsOpen() || !mDolbyBuffer)
724 while (sampleOffset < numSamples)
726 if ((mBurstSize != 0) && (mBurstIndex < mBurstSamples))
728 if(mBurstOffset < mBurstSize)
732 if (mBurstIndex == 0)
738 else if (mBurstIndex == 1)
741 data0 = ((bsi.
bsmod & 0x7) << 8) | 0x0015;
742 data1 = mBurstSize * 2;
747 data0 = (uint32_t)(mBurstBuffer[mBurstOffset]);
748 data0 = ((data0 & 0x00ff) << 8) | ((data0 & 0xff00) >> 8);
750 data1 = (uint32_t)(mBurstBuffer[mBurstOffset]);
751 data1 = ((data1 & 0x00ff) << 8) | ((data1 & 0xff00) >> 8);
758 for (
ULWord i = 0; i < numChannels; i += 2)
760 audioBuffer[sampleOffset * numChannels + i] = data0;
761 audioBuffer[sampleOffset * numChannels + i + 1] = data1;
767 for (
ULWord i = 0; i < numChannels; i++)
769 audioBuffer[sampleOffset * numChannels + i] = 0;
791 cerr <<
"## ERROR: Dolby frame not found" << endl;
792 mDolbyFileIO.
Close();
796 if (!
ParseBSI(&mDolbyBuffer[1], sampleCount - 1, &bsi))
806 mDolbySize = sampleCount;
809 case 0: mDolbyBlocks = 1;
break;
810 case 1: mDolbyBlocks = 2;
break;
811 case 2: mDolbyBlocks = 3;
break;
812 case 3: mDolbyBlocks = 6;
break;
813 default:
goto silence;
817 while (numBlocks <= 6)
820 while (dolbyOffset < mDolbySize)
823 if (burstOffset >= mBurstMax)
825 cerr <<
"## ERROR: Dolby burst too large" << endl;
826 mDolbyFileIO.
Close();
831 mBurstBuffer[burstOffset] = mDolbyBuffer[dolbyOffset];
842 cerr <<
"## ERROR: Dolby frame not found" << endl;
843 mDolbyFileIO.
Close();
849 if (!
ParseBSI(&mDolbyBuffer[1], sampleCount - 1, &bsi))
855 cerr <<
"## ERROR: Dolby frame bad bsid = " << bsi.
bsid << endl;
859 mDolbySize = sampleCount;
866 numBlocks += mDolbyBlocks;
870 case 0: mDolbyBlocks = 1;
break;
871 case 1: mDolbyBlocks = 2;
break;
872 case 2: mDolbyBlocks = 3;
break;
873 case 3: mDolbyBlocks = 6;
break;
875 cerr <<
"## ERROR: Dolby frame bad numblkscod = " << bsi.
numblkscod << endl;
887 cerr <<
"## ERROR: Dolby frame unexpected convsync = " << bsi.
convsync << endl;
893 mBurstSize = burstOffset;
900 return numSamples * numChannels * 4;
904 memset(&audioBuffer[sampleOffset * numChannels], 0, (numSamples - sampleOffset) * numChannels * 4);
905 return numSamples * numChannels * 4;
916 bytes = mDolbyFileIO.
Read((uint8_t*)(&pInDolbyBuffer[0]), 2);
925 if ((mDolbyBuffer[0] == 0x7705) ||
926 (mDolbyBuffer[0] == 0x770b))
931 bytes = mDolbyFileIO.
Read((uint8_t*)(&pInDolbyBuffer[1]), 4);
936 uint32_t size = (uint32_t)mDolbyBuffer[1];
937 size = (((size & 0x00ff) << 8) | ((size & 0xff00) >> 8));
938 size = (size & 0x7ff) + 1;
941 uint32_t len = (size - 3) * 2;
942 bytes = mDolbyFileIO.
Read((uint8_t*)(&pInDolbyBuffer[3]), len);
954 if ((pInDolbyBuffer ==
NULL) || (pBsi ==
NULL))
975 if (pBsi->
acmod == 0x0)
995 if (pBsi->
acmod > 0x2)
999 if ((pBsi->
acmod & 0x1) && (pBsi->
acmod > 0x2))
1004 if (pBsi->
acmod & 0x4)
1024 if (pBsi->
acmod == 0x0)
1044 else if (pBsi->
mixdef == 0x2)
1048 else if (pBsi->
mixdef == 0x3)
1126 uint32_t size = 8 * (pBsi->
mixdeflen + 2);
1127 size = (size + 7) / 8 * 8;
1131 if (!
GetBits(data, (size > 8)? 8 : size))
return false;
1137 if (pBsi->
acmod < 0x2)
1145 if (pBsi->
acmod == 0x0)
1174 for(blk = 0; blk < numblk; blk++)
1192 if (pBsi->
acmod == 0x2)
1197 if (pBsi->
acmod >= 0x6)
1208 if (pBsi->
acmod == 0x0)
1218 if (pBsi->
fscod < 0x3)
1248 uint32_t size = 8 * (pBsi->
addbsil + 1);
1252 if (!
GetBits(data, 8))
return false;
1264 mBitBuffer = pBuffer;
1272 static uint8_t bitMask[] = { 0x00, 0x01, 0x03, 0x07, 0x0f, 0x1f, 0x3f, 0x7f, 0xff };
1292 data |= ((*mBitBuffer >> mBitIndex) & bitMask[cb]) << bits;
1298 #else // DOLBY_FULL_PARSER
1312 if ((mConfig.fDolbyFile ==
NULL) || (mDolbyBuffer ==
NULL))
1316 while (sampleOffset < numSamples)
1319 if (mBurstIndex >= mBurstSamples)
1323 if (mBurstIndex == 0)
1326 uint32_t bytes = mConfig.fDolbyFile->Read((uint8_t*)(&mDolbyBuffer[0]), 2);
1331 bytes = mConfig.fDolbyFile->Read((uint8_t*)(&mDolbyBuffer[0]), 2);
1337 if ((mDolbyBuffer[0] != 0x7705) &&
1338 (mDolbyBuffer[0] != 0x770b))
1342 bytes = mConfig.fDolbyFile->Read((uint8_t*)(&mDolbyBuffer[1]), 4);
1347 uint32_t size = (uint32_t)mDolbyBuffer[1];
1348 size = (((size & 0x00ff) << 8) | ((size & 0xff00) >> 8)) + 1;
1351 uint32_t len = (size - 3) * 2;
1352 bytes = mConfig.fDolbyFile->Read((uint8_t*)(&mDolbyBuffer[3]), len);
1362 if (mBurstOffset < mBurstSize)
1366 if (mBurstIndex == 0)
1372 else if (mBurstIndex == 1)
1376 data1 = mBurstSize * 2;
1381 data0 = (uint32_t)(mDolbyBuffer[mBurstOffset]);
1382 data0 = ((data0 & 0x00ff) << 8) | ((data0 & 0xff00) >> 8);
1384 data1 = (uint32_t)(mDolbyBuffer[mBurstOffset]);
1385 data1 = ((data1 & 0x00ff) << 8) | ((data1 & 0xff00) >> 8);
1392 for (
ULWord i = 0; i < numChannels; i += 2)
1394 pInAudioBuffer[sampleOffset * numChannels + i] = data0;
1395 pInAudioBuffer[sampleOffset * numChannels + i + 1] = data1;
1401 for (
ULWord i = 0; i < numChannels; i++)
1403 pInAudioBuffer[sampleOffset * numChannels + i] = 0;
1411 return numSamples * numChannels * 4;
1415 memset(&pInAudioBuffer[sampleOffset * numChannels], 0, (numSamples - sampleOffset) * numChannels * 4);
1416 return numSamples * numChannels * 4;
1418 #endif // DOLBY_FULL_PARSER
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
virtual ~NTV2DolbyPlayer(void)
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
virtual bool RouteOutputSignal(void)
Performs all widget/signal routing for playout.
@ NTV2_TestPatt_CheckField
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
#define NTV2_IS_VALID_TASK_MODE(__m__)
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
UWord firstFrame(void) const
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
@ NTV2_TestPatt_MultiBurst
std::string fDolbyFilePath
Optional path to Dolby audio source file.
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
bool GetRP188Reg(RP188_STRUCT &outRP188) const
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
Declares common audio macros and structs used in the SDK.
ULWord GetByteCount(void) const
AJALabelValuePairs Get(const bool inCompact=(0)) const
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual void ConsumeFrames(void)
My consumer thread that repeatedly plays frames using AutoCirculate (until quit).
@ NTV2_AUDIO_FORMAT_DOLBY
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
static const uint32_t AUDIOBYTES_MAX_48K(201 *1024)
The maximum number of bytes of 48KHz audio that can be transferred for a single frame....
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
Declares the NTV2TestPatternGen class.
@ NTV2_TestPatt_ColorBars100
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
#define DEC0N(__x__, __n__)
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
virtual AJAStatus SetUpHostBuffers(void)
Sets up my host video & audio buffers.
uint8_t mixdatabuffer[64]
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
virtual AJAStatus Run(void)
Runs me.
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
Header file for NTV2DolbyPlayer demonstration class.
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual void SetBitBuffer(uint8_t *pBuffer, uint32_t size)
Set the bitstream buffer for bit retrieval.
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
const char * NTV2FrameBufferFormatString(NTV2FrameBufferFormat fmt)
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
bool CanAcceptMoreOutputFrames(void) const
NTV2FrameRate
Identifies a particular video frame rate.
AJAStatus Open(const std::string &fileName, const int flags, const int properties)
NTV2Buffer & VideoBuffer(void)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=(0), bool inRDMA=(0))
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
virtual class DeviceCapabilities & features(void)
virtual AJAStatus SetUpAudio(void)
Performs all audio setup.
static const ULWord gNumFrequencies(sizeof(gFrequencies)/sizeof(double))
NTV2DolbyPlayer(const DolbyPlayerConfig &inConfigData)
Constructs me using the given configuration settings.
virtual bool DMABufferUnlockAll()
Unlocks all previously-locked buffers used for DMA transfers.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
I am similar to NTV2Player, but I demonstrate how to play/output 8 channels of audio tone (or ramp da...
Declares the AJAProcess class.
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
virtual AJAStatus SetUpVideo(void)
Performs all video setup.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool GetNumberAudioChannels(ULWord &outNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current number of audio channels being captured or played by a given Audio System on the ...
@ NTV2_TestPatt_FlatField
static AJALabelValuePairs & append(AJALabelValuePairs &inOutTable, const std::string &inLabel, const std::string &inValue=std::string())
A convenience function that appends the given label and value strings to the provided AJALabelValuePa...
virtual std::string GetDescription(void) const
AJAStatus Seek(const int64_t distance, const AJAFileSetFlag flag) const
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
ULWord VideoBufferSize(void) const
bool SetOutputTimeCodes(const NTV2TimeCodes &inValues)
Intended for playout, replaces the contents of my acOutputTimeCodes member.
static size_t SetDefaultPageSize(void)
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
bool CopyFrom(const void *pInSrcBuffer, const ULWord inByteCount)
Replaces my contents from the given memory buffer, resizing me to the new byte count.
NTV2VideoFormat fVideoFormat
The video format to use.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
NTV2Channel fOutputChannel
The device channel to use.
AJALabelValuePairs Get(const bool inCompact=(0)) const
Renders a human-readable representation of me.
Configures an NTV2DolbyPlayer instance.
uint32_t blkmixcfginfo[6]
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
NTV2Buffer & AudioBuffer(void)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
virtual uint32_t AddRamp(NTV2FrameData &inFrameData)
Inserts audio test ramp into the given NTV2FrameData's audio buffer.
@ NTV2_TestPatt_ColorBars75
virtual bool IsDeviceReady(const bool inCheckValid=(0))
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
uint16_t GetEndFrame(void) const
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
std::vector< std::string > NTV2StringList
virtual void ProduceFrames(void)
My producer thread that repeatedly produces video frames.
virtual uint32_t AddDolby(NTV2FrameData &inFrameData)
Inserts dolby audio into the given NTV2FrameData's audio buffer.
virtual bool SetHDMIOutAudioRate(const NTV2AudioRate inNewValue)
Sets the HDMI output's audio rate.
bool AddAudioTone(ULWord &outNumBytesWritten, NTV2Buffer &inAudioBuffer, ULWord &inOutCurrentSample, const ULWord inNumSamples, const double inSampleRate, const double inAmplitude, const double inFrequency, const ULWord inNumBits, const bool inByteSwap, const ULWord inNumChannels)
Fills the given buffer with 32-bit (ULWord) audio tone samples.
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
The NTV2 test pattern generator.
NTV2Buffer fAudioBuffer
Host audio buffer.
uint32_t Read(uint8_t *pBuffer, const uint32_t length)
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=(0))
Connects the given widget signal input (sink) to the given widget signal output (source).
virtual bool SetHDMIOutAudioSource2Channel(const NTV2AudioChannelPair inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the HDMI output's 2-channel audio source.
virtual void Quit(void)
Gracefully stops me from running.
@ NTV2_OUTPUTDESTINATION_HDMI
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
virtual bool GetDolbyFrame(uint16_t *pInDolbyBuffer, uint32_t &numSamples)
Get a dolby audio frame from the input file.
UWord lastFrame(void) const
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
std::string fDeviceSpec
The AJA device to use.
virtual uint32_t AddTone(NTV2FrameData &inFrameData)
Inserts audio tone (based on my current tone frequency) into the given NTV2FrameData's audio buffer.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool GetBits(uint32_t &data, uint32_t inBitCount)
Retreive the specified number of bits from the bitstream buffer.
This struct replaces the old RP188_STRUCT.
bool IsRGBFormat(const NTV2FrameBufferFormat format)
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
ULWord GetAudioSamplesPerFrame(const NTV2FrameRate inFrameRate, const NTV2AudioRate inAudioRate, ULWord inCadenceFrame=0, bool inIsSMPTE372Enabled=false)
Returns the number of audio samples for a given video frame rate, audio sample rate,...
uint16_t GetStartFrame(void) const
virtual bool ParseBSI(uint16_t *pInDolbyBuffer, uint32_t numSamples, NTV2DolbyBSI *pBsi)
Parse the dolby audio bit stream information block.
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the producer thread's static callback function that gets called when the producer thread star...
static const double gFrequencies[]
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
double GetAudioSamplesPerSecond(const NTV2AudioRate inAudioRate)
Returns the audio sample rate as a number of audio samples per second.
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
@ NTV2_TestPatt_MultiPattern
virtual void StartProducerThread(void)
Starts my producer thread.
NTV2PixelFormat fPixelFormat
The pixel format to use.
enum NTV2OutputCrosspointID NTV2OutputXptID
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
static NTV2TestPatternNames getTestPatternNames(void)
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
double FramesToSeconds(int64_t frames) const
virtual void StartConsumerThread(void)
Starts my consumer thread.
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
std::ostream & operator<<(std::ostream &ioStrm, const DolbyPlayerConfig &inObj)
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
@ NTV2_TestPatt_LineSweep
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
virtual AJAStatus SetUpTestPatternBuffers(void)
Creates my test pattern buffers.
virtual AJAStatus Start()
static const bool BUFFER_PAGE_ALIGNED((!(0)))
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
#define xHEX0N(__x__, __n__)
#define AJA_FAILURE(_status_)
@ NTV2_AUDIO_RATE_INVALID
@ NTV2_AudioChannel1_2
This selects audio channels 1 and 2 (Group 1 channels 1 and 2)
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
virtual bool SetHDMIOutAudioFormat(const NTV2AudioFormat inNewValue)
Sets the HDMI output's audio format.
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread star...
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
static const double gAmplitudes[]
bool GetRP188Str(std::string &sRP188) const
virtual void GetACStatus(AUTOCIRCULATE_STATUS &outStatus)
Provides status information about my output (playout) process.
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
NTV2TimeCodes fTimecodes
Map of TC indexes to NTV2_RP188 values.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
static const uint32_t AUDIOBYTES_MAX_192K(824 *1024)
The maximum number of bytes of 192KHz audio that can be transferred for a single frame....
bool fDoRamp
If true, use audio ramp pattern instead of tone.
@ NTV2_AUDIOSYSTEM_INVALID
Declares the AJATimeBase class.