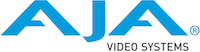 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
9 #ifndef _NTV2DOLBYPLAYER_H
10 #define _NTV2DOLBYPLAYER_H
19 #define DOLBY_FULL_PARSER // If defined, parse EC3 files with multiple sync frames per HDMI burst; otherwise parse with single sync frame per HDMI burst.
74 virtual void Quit (
void);
76 virtual bool IsRunning (
void)
const {
return !mGlobalQuit;}
221 #ifdef DOLBY_FULL_PARSER
229 virtual bool GetDolbyFrame (uint16_t * pInDolbyBuffer, uint32_t & numSamples);
247 virtual void SetBitBuffer (uint8_t * pBuffer, uint32_t size);
255 virtual bool GetBits (uint32_t & data, uint32_t inBitCount);
282 typedef std::vector<NTV2Buffer> NTV2Buffers;
291 double mToneFrequency;
299 NTV2Buffers mTestPatRasters;
302 uint16_t mRampSample;
303 uint32_t mBurstIndex;
304 uint32_t mBurstSamples;
305 uint16_t * mBurstBuffer;
307 uint32_t mBurstOffset;
309 uint16_t * mDolbyBuffer;
311 uint32_t mDolbyBlocks;
312 uint8_t * mBitBuffer;
317 #endif // _NTV2DOLBY_H
enum NTV2EveryFrameTaskMode NTV2TaskMode
virtual ~NTV2DolbyPlayer(void)
virtual bool RouteOutputSignal(void)
Performs all widget/signal routing for playout.
std::string fDolbyFilePath
Optional path to Dolby audio source file.
std::set< NTV2TCIndex > NTV2TCIndexes
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
AJALabelValuePairs Get(const bool inCompact=(0)) const
virtual void ConsumeFrames(void)
My consumer thread that repeatedly plays frames using AutoCirculate (until quit).
virtual AJAStatus SetUpHostBuffers(void)
Sets up my host video & audio buffers.
uint8_t mixdatabuffer[64]
virtual AJAStatus Run(void)
Runs me.
virtual void SetBitBuffer(uint8_t *pBuffer, uint32_t size)
Set the bitstream buffer for bit retrieval.
virtual AJAStatus SetUpAudio(void)
Performs all audio setup.
Declares the AJATimeCodeBurn class.
NTV2DolbyPlayer(const DolbyPlayerConfig &inConfigData)
Constructs me using the given configuration settings.
I am similar to NTV2Player, but I demonstrate how to play/output 8 channels of audio tone (or ramp da...
virtual AJAStatus SetUpVideo(void)
Performs all video setup.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
std::ostream & operator<<(std::ostream &ioStrm, const DolbyPlayerConfig &inObj)
Configures an NTV2DolbyPlayer instance.
uint32_t blkmixcfginfo[6]
I interrogate and control an AJA video/audio capture/playout device.
virtual uint32_t AddRamp(NTV2FrameData &inFrameData)
Inserts audio test ramp into the given NTV2FrameData's audio buffer.
std::vector< NTV2FrameData > NTV2FrameDataArray
A vector of NTV2FrameData elements.
virtual void ProduceFrames(void)
My producer thread that repeatedly produces video frames.
virtual uint32_t AddDolby(NTV2FrameData &inFrameData)
Inserts dolby audio into the given NTV2FrameData's audio buffer.
Declares the AJAFileIO class.
virtual void Quit(void)
Gracefully stops me from running.
DolbyPlayerConfig DolbyPlayerConfig
Configures an NTV2DolbyPlayer instance.
This file contains some structures, constants, classes and functions that are used in some of the dem...
virtual bool GetDolbyFrame(uint16_t *pInDolbyBuffer, uint32_t &numSamples)
Get a dolby audio frame from the input file.
virtual uint32_t AddTone(NTV2FrameData &inFrameData)
Inserts audio tone (based on my current tone frequency) into the given NTV2FrameData's audio buffer.
virtual bool GetBits(uint32_t &data, uint32_t inBitCount)
Retreive the specified number of bits from the bitstream buffer.
virtual bool ParseBSI(uint16_t *pInDolbyBuffer, uint32_t numSamples, NTV2DolbyBSI *pBsi)
Parse the dolby audio bit stream information block.
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the producer thread's static callback function that gets called when the producer thread star...
DolbyPlayerConfig(const std::string &inDeviceSpecifier="0")
Constructs a default DolbyPlayer configuration.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
Configures an NTV2Player instance.
virtual void StartProducerThread(void)
Starts my producer thread.
virtual void StartConsumerThread(void)
Starts my consumer thread.
virtual AJAStatus SetUpTestPatternBuffers(void)
Creates my test pattern buffers.
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
Declares the AJAThread class.
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread star...
virtual void GetACStatus(AUTOCIRCULATE_STATUS &outStatus)
Provides status information about my output (playout) process.
bool fDoRamp
If true, use audio ramp pattern instead of tone.