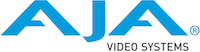 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
7 #ifndef __NTV2_CAPTION608TYPES_
8 #define __NTV2_CAPTION608TYPES_
49 #define IsValidLine21Row(__row__) ((__row__) >= NTV2_CC608_MinRow && (__row__) <= NTV2_CC608_MaxRow)
50 #define IsValidLine21Column(__col__) ((__col__) >= NTV2_CC608_MinCol && (__col__) <= NTV2_CC608_MaxCol)
66 #define IsValidLine21Field(_field_) ((_field_) == NTV2_CC608_Field1 || (_field_) == NTV2_CC608_Field2)
67 #define IsLine21Field1(_field_) ((_field_) == NTV2_CC608_Field1)
68 #define IsLine21Field2(_field_) ((_field_) == NTV2_CC608_Field2)
102 #define IsValidLine21Channel(_chan_) ((_chan_) >= NTV2_CC608_CC1 && (_chan_) < NTV2_CC608_ChannelMax)
103 #define IsLine21CaptionChannel(_chan_) ((_chan_) >= NTV2_CC608_CC1 && (_chan_) <= NTV2_CC608_CC4)
104 #define IsLine21TextChannel(_chan_) ((_chan_) >= NTV2_CC608_Text1 && (_chan_) <= NTV2_CC608_Text4)
105 #define IsLine21XDSChannel(_chan_) ((_chan_) == NTV2_CC608_XDS)
106 #define IsField1Line21CaptionChannel(_chan_) ((_chan_) == NTV2_CC608_CC1 || (_chan_) == NTV2_CC608_CC2 || (_chan_) == NTV2_CC608_Text1 || (_chan_) == NTV2_CC608_Text2)
107 #define IsField2Line21CaptionChannel(_chan_) ((_chan_) == NTV2_CC608_CC3 || (_chan_) == NTV2_CC608_CC4 || (_chan_) == NTV2_CC608_Text3 || (_chan_) == NTV2_CC608_Text4)
136 #define IsValidLine21Mode(_mode_) ((_mode_) >= NTV2_CC608_CapModePopOn && (_mode_) < NTV2_CC608_CapModeMax)
137 #define IsLine21PopOnMode(_mode_) ((_mode_) == NTV2_CC608_CapModePopOn)
138 #define IsLine21PaintOnMode(_mode_) ((_mode_) == NTV2_CC608_CapModePaintOn)
139 #define IsLine21RollUpMode(_mode_) ((_mode_) >= NTV2_CC608_CapModeRollUp2 && (_mode_) <= NTV2_CC608_CapModeRollUp4)
170 #define IsValidLine21Color(_color_) ((_color_) >= NTV2_CC608_White && (_color_) < NTV2_CC608_NumColors)
171 #define IsLine21WhiteColor(_color_) ((_color_) == NTV2_CC608_White)
172 #define IsLine21BlackColor(_color_) ((_color_) == NTV2_CC608_Black)
224 #define IsValidLine21Opacity(_opacity_) ((_opacity_) >= NTV2_CC608_Opaque && (_opacity_) < NTV2_CC608_NumOpacities)
225 #define IsLine21Transparent(_opacity_) ((_opacity_) == NTV2_CC608_Transparent)
226 #define IsLine21SemiTransparent(_opacity_) ((_opacity_) == NTV2_CC608_SemiTransparent)
227 #define IsLine21Opaque(_opacity_) ((_opacity_) == NTV2_CC608_Opaque)
258 #define IsValidLine21CharacterSet(_charset_) ((_charset_) >= NTV2_CC608_DefaultCharacterSet && (_charset_) < NTV2_CC608_NumCharacterSets)
335 return UByte ((inCodePoint & 0x00FF00) >> 8);
346 return UByte (inCodePoint & 0x000000FF);
378 const bool inItalics =
false,
379 const bool inUnderline =
false,
380 const bool inFlash =
false);
382 bItalic = inRHS.bItalic;
383 bUnderline = inRHS.bUnderline;
384 fgColor = inRHS.fgColor;
385 bgColor = inRHS.bgColor;
386 bgOpacity = inRHS.bgOpacity; }
388 bItalic = inRHS.bItalic;
389 bUnderline = inRHS.bUnderline;
390 fgColor = inRHS.fgColor;
391 bgColor = inRHS.bgColor;
392 bgOpacity = inRHS.bgOpacity;
return *
this;}
399 inline bool IsSet (
void)
const {
return bFlash || bItalic || bUnderline
520 inline void Clear (
void) { bFlash = bItalic = bUnderline =
false;
549 std::string GetHexString (
void)
const;
554 uint16_t GetHashKey (
void)
const;
564 unsigned bgOpacity :2;
673 explicit inline CaptionData () { bGotField1Data = bGotField2Data = bGotField3Data =
false; f1_char1 = f1_char2 = f2_char1 = f2_char2 = f3_char1 = f3_char2 = 0xFF; }
688 inline bool HasF1Data (
void)
const {
return (bGotField1Data && (f1_char1 != 0x80 || f1_char2 != 0x80));}
693 inline bool HasF2Data (
void)
const {
return (bGotField2Data && (f2_char1 != 0x80 || f2_char2 != 0x80));}
698 inline bool HasData (
void)
const {
return HasF1Data() || HasF2Data();}
703 inline bool IsF1Invalid (
void)
const {
return f1_char1 == 0xFF && f1_char2 == 0xFF;}
708 inline bool IsF2Invalid (
void)
const {
return f2_char1 == 0xFF && f2_char2 == 0xFF;}
713 inline bool IsError (
void)
const {
return IsF1Invalid() && IsF2Invalid();}
728 inline CaptionData &
SetF1Data (
const UByte inF1Char1,
const UByte inF1Char2) {f1_char1 = inF1Char1; f1_char2 = inF1Char2; bGotField1Data = inF1Char1 != 0xFF && inF1Char2 != 0xFF;
return *
this;}
743 inline CaptionData &
SetF2Data (
const UByte inF2Char1,
const UByte inF2Char2) {f2_char1 = inF2Char1; f2_char2 = inF2Char2; bGotField2Data = inF2Char1 != 0xFF && inF2Char2 != 0xFF;
return *
this;}
748 inline void Clear (
void) {bGotField1Data = bGotField2Data = bGotField3Data =
false; f1_char1 = f1_char2 = f2_char1 = f2_char2 = f3_char1 = f3_char2 = 0x80;}
753 inline void Zero (
void) {bGotField1Data = bGotField2Data = bGotField3Data =
false; f1_char1 = f1_char2 = f2_char1 = f2_char2 = f3_char1 = f3_char2 = 0x00;}
787 NTV2DecoderChange_None = 0,
788 NTV2DecoderChange_CurrentChannel = 1,
789 NTV2DecoderChange_CurrentRow = 2,
790 NTV2DecoderChange_CurrentColumn = 4,
791 NTV2DecoderChange_CurrentScreen = 8,
792 NTV2DecoderChange_CaptionMode = 16,
793 NTV2DecoderChange_ScreenCharacter = 32,
794 NTV2DecoderChange_ScreenAttribute = 64,
795 NTV2DecoderChange_DrawScreen = 128,
796 NTV2DecoderChange_All = 0xFFFF
797 } NTV2Caption608Change;
805 std::ostream & Print (std::ostream & inOutStrm)
const;
915 inline const NTV2Line21Attrs & operator [] (
const size_t inIndex)
const {
return GetPermutation(inIndex);}
922 #endif // __NTV2_CAPTION608TYPES_
NTV2CodePointSet::const_iterator NTV2CodePointSetConstIter
@ NTV2_CC608_PrivateCharacterSet1
void Clear(void)
Sets all of my "got data" fields to "false" and all my character values to 0x80.
@ NTV2_CC608_SemiTransparent
CaptionData()
Default constructor. Sets my caption bytes to 0xFF, and "gotFieldData" values to false.
struct CaptionData CaptionData
This structure encapsulates all possible CEA-608 caption data bytes that may be associated with a giv...
Defines the import/export macros for producing DLLs or LIBs.
NTV2Line21Channel mChannel
Caption channel being changed.
NTV2Line21Attributes StrToNTV2Line21Attributes(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21Attributes value.
bool HasF1Data(void) const
NTV2Line21Attributes & SetFlash(const bool inFlash)
Sets my flashing attribute setting.
NTV2Line21Opacity
The CEA-608 character opacity values: opaque, semi-transparent, and transparent.
@ NTV2_CC608_NumCharacterSets
const UWord NTV2_CC608_MinCol(1)
The minimum column index number (located at the left edge of the screen).
UByte f2_char1
Caption Byte 1 of Field 2.
NTV2Line21Attributes & AddUnderline(void)
Enables my underline attribute setting.
UWord mOld
Caption channel change. This is the old NTV2Line21Channel value. (The new, current value is in my mCh...
@ NTV2_CC608_NumOpacities
const std::string & NTV2Line21ColorToStr(const NTV2Line21Color inLine21Color, const bool inCompact=true)
Converts the given NTV2Line21Color value into a human-readable string.
UWord mOld
Current screen change. This is the old screen number.
bool IsF1Invalid(void) const
UWord NTV2CC608CodePointToUtf16Char(const NTV2_CC608_CodePoint in608CodePoint)
Returns the UTF-16 character that best represents the given CEA-608 code point.
CaptionData & SetF1Data(const UByte inF1Char1, const UByte inF1Char2)
Sets my F1 data bytes.
@ NTV2_CC608_CapModePaintOn
Paint-on caption mode.
bool IsF2Invalid(void) const
@ NTV2_CC608_CC2
Caption channel 2, the secondary caption channel.
@ NTV2_CC608_TextChannelOffset
bool IsSet(void) const
Returns true if I'm any different from the default, i.e., if IsFlashing(), IsItalicized(),...
NTV2Line21Attributes & SetBGColor(const NTV2Line21Color inBGColor)
Sets my background color attribute.
NTV2Line21Color
The CEA-608 color values: white, green, blue, cyan, red, yellow, magenta, and black.
bool operator<(const value_t lhs, const value_t rhs) noexcept
comparison operator for JSON types
UByte Get608Byte1(const NTV2_CC608_CodePoint inCodePoint)
Extracts the first CEA-608 byte from the given NTV2_CC608_CodePoint.
UWord mColumn
The column number that contains the character whose attributes are changing.
bool operator==(const json_pointer< RefStringTypeLhs > &lhs, const json_pointer< RefStringTypeRhs > &rhs) noexcept
bool NTV2Line21ColorToRGB8(const NTV2Line21Color inLine21Color, UByte &outR, UByte &outG, UByte &outB, const bool inIsHD=false)
Converts a given CEA-608 color value into three 8-bit RGB component values.
NTV2Line21Attributes & SetItalics(const bool inItalics)
Sets my italics setting.
NTV2Line21AttrsPtr NTV2Line21AttributesPtr
UByte f1_char2
Caption Byte 2 of Field 1.
@ NTV2_CC608_DefaultCharacterSet
@ NTV2_CC608_Field_Invalid
bool IsUnderlined(void) const
Returns true if I'm underlined; otherwise returns false.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
NTV2Line21Attributes & SetColor(const NTV2Line21Color inFGColor)
Sets my foreground color attribute.
bool IsItalicized(void) const
Returns true if I'm italicized; otherwise returns false.
UWord mScreenNum
The number of the screen backbuffer that's changing.
NTV2Line21Opacity StrToNTV2Line21Opacity(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21Opacity value.
UByte Get608Byte2(const NTV2_CC608_CodePoint inCodePoint)
Extracts the second CEA-608 byte from the given NTV2_CC608_CodePoint.
uint16_t GetHashKey(void) const
Returns my magnitude used to implement operator < for sorting or using a Line21 attribute as an index...
This class is used to respond to dynamic events that occur during CEA-608 caption decoding.
UWord mNew
Current NTV2Line21Mode change. This is the new NTV2Line21Mode value.
UByte f3_char2
Caption Byte 2 of Field 3.
@ NTV2_CC608_ChannelInvalid
CaptionData & SetF2Data(const CaptionData &inRHS)
Copies my F2 data bytes from the given CaptionData instance.
@ NTV2_CC608_CapModeUnknown
Unknown or invalid caption mode.
void Zero(void)
Sets all of my "got data" fields to "false" and all my character values to zero.
void Clear(void)
Clears all of my attributes, returning me to a default state, i.e., no flashing, no italics,...
const std::string & NTV2Line21ModeToStr(const NTV2Line21Mode inLine21Mode)
Converts the given NTV2Line21Mode value into a human-readable string.
enum NTV2Line21CharacterSet NTV2Line21CharSet
bool IsFlashing(void) const
Returns true if I'm flashing; otherwise returns false.
const std::string & NTV2Line21OpacityToStr(const NTV2Line21Opacity inLine21Opacity, const bool inCompact=true)
Converts the given NTV2Line21Opacity value into a human-readable string.
NTV2Line21CharacterSet GetLine21CharacterSet(const NTV2_CC608_CodePoint inCodePoint)
Extracts the NTV2Line21CharacterSet from the given NTV2_CC608_CodePoint.
uint16_t mNew
The new character NTV2Line21Attributes.
NTV2Line21Color StrToNTV2Line21Color(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21Color value.
NTV2Line21Channel StrToNTV2Line21Channel(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21Channel value.
NTV2Line21Attributes & SetOpacity(const NTV2Line21Opacity inOpacity)
Sets my background opacity attribute.
Declares numerous NTV2 utility functions.
@ NTV2_CC608_DoubleSizeCharacterSet
bool bGotField3Data
True if Field 3 bytes have been set. This is used only when translating 30fps video to 24fps and remo...
UWord mRow
Display character change. The row number that contains the character that's being changed.
UWord mWhatChanged
Bit mask that indicates what changed.
NTV2Line21Field StrToNTV2Line21Field(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21Field value.
@ NTV2_CC608_CapModeRollUp4
4-row roll-up from bottom
UWord mNew
Current screen change. This is the new screen number.
std::string NTV2CC608CodePointToUtf8String(const NTV2_CC608_CodePoint in608CodePoint)
Returns a string containing the UTF-8 character sequence that best represents the given CEA-608 code ...
bool bGotField2Data
True if Field 2 bytes have been set; otherwise false.
An ordered set of all possible NTV2Line21Attributes permutations (excluding the "flashing" attribute,...
CaptionData & SetF2Data(const UByte inF2Char1, const UByte inF2Char2)
Sets my F2 data bytes.
const std::string & NTV2Line21CharacterSetToStr(const NTV2Line21CharacterSet inLine21CharSet)
Converts the given NTV2Line21CharacterSet value into a human-readable string.
ULWord size(void) const
Returns my size (the total number of attribute permutations that I have).
bool NTV2Line21ColorToYUV8(const NTV2Line21Color inLine21Color, UByte &outY, UByte &outCb, UByte &outCr)
Converts a given CEA-608 color value into three 8-bit Y, Cb, Cr component values.
NTV2Line21Attributes(const NTV2Line21Attributes &inRHS)
UWord mColumn
Display character change. The column number that contains the character that's being changed.
UWord mScreenNum
Display character change. This is the number of the screen backbuffer that's changing.
const UWord NTV2_CC608_MaxCol(32)
The maximum column index number (located at the right edge of the screen).
const std::string & NTV2Line21FieldToStr(const NTV2Line21Field inLine21Field)
Converts the given NTV2Line21Field value into a human-readable string.
NTV2Line21Color GetBGColor(void) const
Returns my background color.
UWord mOld
Current column change. This is the old column number.
struct NTV2Line21Attributes NTV2Line21Attributes
CEA-608 Character Attributes.
@ NTV2_CC608_CapModeRollUp2
2-row roll-up from bottom
CaptionData & SetF1Data(const CaptionData &inRHS)
Copies my F1 data bytes from the given CaptionData instance.
NTV2_CC608_CodePoint Make608CodePoint(const UByte in608Byte1, const UByte in608Byte2, const NTV2Line21CharacterSet inCharSet=NTV2_CC608_DefaultCharacterSet)
Constructs a unique CEA-608 caption character code point from its three components.
ULWord NTV2_CC608_CodePoint
Describes a unique CEA-608 caption character code point in 32 bits: 0xSS00XXYY, where....
void() NTV2Caption608Changed(void *pInstance, const NTV2Caption608ChangeInfo &inChangeInfo)
This callback is used to respond to dynamic events that occur during CEA-608 caption decoding.
@ NTV2_CC608_RegisteredCharacterSet1
NTV2Line21Attributes & RemoveUnderline(void)
Disables my underline attribute setting.
@ NTV2_CC608_PrivateCharacterSet2
bool operator!=(const json_pointer< RefStringTypeLhs > &lhs, const json_pointer< RefStringTypeRhs > &rhs) noexcept
const std::string & NTV2Line21ChannelToStr(const NTV2Line21Channel inLine21Channel, const bool inCompact=true)
Converts the given NTV2Line21Channel value into a human-readable string.
NTV2Line21Mode
The CEA-608 modes: pop-on, roll-up (2, 3 and 4-line), and paint-on.
NTV2Line21Mode StrToNTV2Line21Mode(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21Mode value.
@ NTV2_CC608_CC1
Caption channel 1, the primary caption channel.
uint16_t mOld
The old character NTV2Line21Attributes.
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
UWord mOld
Current row change. This is the old row number.
UWord mOld
Current NTV2Line21Mode change. This is the old NTV2Line21Mode value.
const UWord NTV2_CC608_MinRow(1)
The minimum row index number (located at the top of the screen).
@ NTV2_CC608_CapModeRollUp3
3-row roll-up from bottom
struct NTV2Line21Attributes NTV2Line21Attrs
NTV2Line21Attributes & RemoveItalics(void)
Removes italics.
NTV2Line21Attributes & SetUnderline(const bool inUnderline)
Sets my underline attribute setting.
@ NTV2_CC608_PRChinaCharacterSet
NTV2Line21Attributes & AddFlash(void)
Enables my flashing attribute setting.
NTV2Line21CharacterSet StrToNTV2Line21CharacterSet(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21CharacterSet value.
std::ostream & operator<<(std::ostream &inOutStream, const NTV2Line21Attrs &inData)
Writes a human-readable rendition of the given NTV2Line21Attributes into the given output stream.
NTV2Line21Color GetColor(void) const
Returns my foreground color.
NTV2Line21Attrs * NTV2Line21AttrsPtr
std::string NTV2CodePointSetToString(const NTV2CodePointSet &inSet)
NTV2Line21Opacity GetOpacity(void) const
Returns my background opacity.
NTV2_CC608_CodePoint mNew
Display character change. The new character NTV2_CC608_CodePoint value.
bool bGotField1Data
True if Field 1 bytes have been set; otherwise false.
@ NTV2_CC608_KoreanCharacterSet
NTV2Line21Field
The two CEA-608 interlace fields.
CEA-608 Character Attributes.
This structure encapsulates all possible CEA-608 caption data bytes that may be associated with a giv...
NTV2_CC608_CodePoint mOld
Display character change. The old character NTV2_CC608_CodePoint value.
UByte f2_char2
Caption Byte 2 of Field 2.
NTV2Line21CharacterSet
The available CEA-608 character sets.
UWord mNew
Current column change. This is the new column number.
std::ostream & Print(std::ostream &inOutStrm) const
bool HasF2Data(void) const
NTV2Line21Attributes & AddItalics(void)
Enables italics.
UByte f3_char1
Caption Byte 1 of Field 3.
@ NTV2_CC608_CapModePopOn
Pop-on caption mode.
UWord mRow
The row number that contains the character whose attributes are changing.
NTV2Line21Attributes & RemoveFlash(void)
Disables my flashing attribute setting.
NTV2Caption608Change
Used to determine what changed. Also can be used to choose which changes to pay attention to.
UByte f1_char1
Caption Byte 1 of Field 1.
UWord mNew
Current row change. This is the new row number.
std::string NTV2Line21AttributesToStr(const NTV2Line21Attributes inLine21Attributes)
Converts the given NTV2Line21Attributes value into a human-readable string.
std::set< NTV2_CC608_CodePoint > NTV2CodePointSet
A set of unique CEA-608 caption character code points.
const UWord NTV2_CC608_MaxRow(15)
The maximum permissible row index number (located at the bottom of the screen).