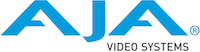 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
19 #define AJA_FD_BEGIN NTV2FormatDescriptor (
21 #define AJA_FD_NOTSUPPORTED NTV2FormatDescriptor ()
22 #define AJA_FD_TBD NTV2FormatDescriptor ()
24 #define AsConstUBytePtr(_x_) reinterpret_cast<const UByte*>(_x_)
25 #define AsUBytePtr(_x_) reinterpret_cast<UByte*>(_x_)
30 {
"YCbCr",
"",
"",
""},
31 {
"YCbCr",
"",
"",
""},
35 {
"YCbCr",
"",
"",
""},
38 {
"YCbCr",
"",
"",
""},
40 {
"Y",
"Cb",
"Cr",
""},
44 {
"YCbCrA",
"",
"",
""},
53 {
"Y",
"Cb",
"Cr",
""},
55 {
"YCbCr",
"",
"",
""},
56 {
"Y",
"Cb",
"Cr",
""},
57 {
"Y",
"Cb",
"Cr",
""},
58 {
"Y",
"CbCr",
"",
""},
59 {
"Y",
"CbCr",
"",
""},
60 {
"Y",
"CbCr",
"",
""},
669 mStandard = inStandard;
670 mPixelFormat = inFrameBufferFormat;
671 mVancMode = inVancMode;
680 const ULWord numActiveLines (numLines);
696 default: numLines = numActiveLines;
break;
698 firstActiveLine = numLines - numActiveLines;
707 void NTV2FormatDescriptor::FinalizePlanar (
void)
711 switch (mPixelFormat)
719 mLinePitch[0] = linePitch * 4;
720 mLinePitch[1] = linePitch * 4;
728 mLinePitch[0] = numPixels;
729 const ULWord lumaRasterBytes (numLines * numPixels);
730 ULWord chromaRasterBytes (lumaRasterBytes / 2);
731 if (lumaRasterBytes % 4)
732 {MakeInvalid();
break;}
733 mLinePitch[1] = mLinePitch[2] = chromaRasterBytes / numLines;
734 if (chromaRasterBytes % numLines)
743 mLinePitch[0] = numPixels *
sizeof(uint16_t);
744 const ULWord lumaRasterBytes (numLines * mLinePitch[0]);
745 ULWord chromaRasterBytes (lumaRasterBytes / 2);
746 mLinePitch[1] = mLinePitch[2] = chromaRasterBytes / numLines;
747 if (chromaRasterBytes % numLines)
752 default: MakeInvalid();
break;
774 mVideoFormat = inVideoFormat;
775 mStandard = inStandard;
776 mPixelFormat = inFrameBufferFormat;
777 mVancMode = inVancMode;
786 const ULWord numActiveLines (numLines);
802 default: numLines = numActiveLines;
break;
804 firstActiveLine = numLines - numActiveLines;
819 : numLines (inNumLines),
820 numPixels (inNumPixels),
821 linePitch (inLinePitch),
822 firstActiveLine (inFirstActiveLine),
830 mLinePitch[0] = inLinePitch * 4;
831 mLinePitch[1] = mLinePitch[2] = mLinePitch[3] = 0;
845 mLinePitch[0] = mLinePitch[1] = mLinePitch[2] = mLinePitch[3] = 0;
848 mNumBitsLuma = mNumBitsChroma = mNumBitsAlpha = 0;
858 }
while (++plane < GetNumPlanes());
864 static const ULWord s4K(0x00001000), s64K(0x00010000);
866 if (inPageSizeBytes != s4K)
868 pageSizeBytes = s64K;
871 if (pageSizeBytes & inPageSizeBytes)
874 }
while (pageSizeBytes > s4K);
876 if (result % pageSizeBytes)
877 result = ((result / pageSizeBytes) + 1) * pageSizeBytes;
883 if (inPlaneIndex0 >= mNumPlanes)
885 switch (mPixelFormat)
891 return inPlaneIndex0 ? 2 : 1;
912 && mLinePitch[0] == inRHS.mLinePitch[0];
918 static string emptyString;
935 if (inByteOffset < byteOffset)
937 }
while (++plane < GetNumPlanes());
945 if (origPlane == 0xFFFF)
947 ULWord byteOffsetToStartOfPlane (0);
948 UWord plane (origPlane);
951 NTV2_ASSERT(inByteOffset >= byteOffsetToStartOfPlane);
952 const ULWord lineOffset ((inByteOffset - byteOffsetToStartOfPlane) /
GetBytesPerRow(origPlane));
953 return UWord(lineOffset);
962 if (plane >= GetNumPlanes())
976 if (inPlaneIndex0 >= mNumPlanes)
978 if (inFrameBuffer.
IsNULL())
982 if (inPlaneIndex0 > 0)
984 if (inPlaneIndex0 > 1)
986 if (inPlaneIndex0 > 2)
990 byteOffset += inRowIndex0 * bytesPerRow;
991 if (byteOffset+bytesPerRow > inFrameBuffer.
GetByteCount())
994 return inOutRowBuffer.
Set(inFrameBuffer.
GetHostAddress(byteOffset), bytesPerRow);
1001 if (inPlaneIndex0 >= mNumPlanes)
1003 if (!pInStartAddress)
1008 if (inPlaneIndex0 > 0)
1010 if (inPlaneIndex0 > 1)
1012 if (inPlaneIndex0 > 2)
1022 if (inPlaneIndex0 >= mNumPlanes)
1026 if (inPlaneIndex0 > 0)
1028 if (inPlaneIndex0 > 1)
1030 if (inPlaneIndex0 > 2)
1040 if (inPlaneIndex0 >= mNumPlanes)
1044 if (inPlaneIndex0 > 0)
1046 if (inPlaneIndex0 > 1)
1048 if (inPlaneIndex0 > 2)
1056 outFirstChangedRowNum = 0xFFFFFFFF;
1057 if (!pInStartAddress1)
1059 if (!pInStartAddress2)
1061 if (pInStartAddress1 == pInStartAddress2)
1069 for (outFirstChangedRowNum = 0; outFirstChangedRowNum <
GetFullRasterHeight(); outFirstChangedRowNum++)
1072 }
while (++plane < GetNumPlanes());
1073 outFirstChangedRowNum = 0xFFFFFFFF;
1083 if (!pInBuffer1 || !pInBuffer2)
1091 if (pInBuffer1 == pInBuffer2)
1100 for (
ULWord rowOffset(0); rowOffset < maxLines; rowOffset++)
1102 outDiffs.push_back(rowOffset);
1103 }
while (++plane < GetNumPlanes());
1114 <<
" px/line=" <<
DEC(GetRasterWidth());
1118 oss <<
" PL" << plane <<
":";
1120 }
while (++plane < GetNumPlanes());
1130 }
while (++plane < GetNumPlanes());
1142 oss <<
" bitsC" <<
DEC(
UWord(GetNumBitsChroma()));
1144 oss <<
" bitsY" <<
DEC(
UWord(GetNumBitsLuma()));
1146 oss <<
"A" <<
DEC(
UWord(GetNumBitsAlpha()));
1155 static const ULWord LineNumbersF1 [] = { 21, 26, 21, 23, 42, 211, 42, 21, 42, 42, 42, 42, 42, 42, 0 };
1156 static const ULWord LineNumbersF2 [] = { 584, 27, 283, 336, 43, 1201, 43, 584, 43, 43, 43, 43, 43, 43, 0 };
1158 static const ULWord LineNumbersF1t [] = { 5, 6, 10, 12, 10, 211, 10, 5, 10, 10, 10, 10, 10, 10, 0 };
1159 static const ULWord LineNumbersF2t [] = { 568, 7, 272, 325, 11, 1201, 11, 568, 11, 11, 11, 11, 11, 11, 0 };
1161 static const ULWord LineNumbersF1tt [] = { 4, 6, 7, 5, 8, 211, 8, 4, 8, 8, 8, 8, 8, 8, 0 };
1162 static const ULWord LineNumbersF2tt [] = { 567, 7, 269, 318, 9, 1201, 9, 567, 9, 9, 9, 9, 9, 9, 0 };
1164 static const ULWord LineNumbersF1Last[] = { 560, 745, 263, 310, 1121, 0, 1121, 0, 2201, 2201, 2201, 2201, 4361, 4361, 0 };
1165 static const ULWord LineNumbersF2Last[] = { 1123, 745, 525, 623, 1121, 0, 1121, 0, 0, 0, 0, 0, 0, 0, 0 };
1170 outIsField2 =
false;
1183 outIsField2 = (inLineOffset & 1) ? !is525i : is525i;
1195 outSMPTELine = inLineOffset/divisor + smpteLine;
1202 outLineOffset = 0xFFFFFFFF;
1210 ULWord firstF1Line(0), firstF2Line(0);
1223 if (inSMPTELine < firstF1Line)
1233 outLineOffset = inSMPTELine - firstF1Line;
1234 else if (inSMPTELine >= firstF2Line)
1235 outLineOffset = (inSMPTELine - firstF2Line) * 2 + not525i;
1237 outLineOffset = (inSMPTELine - firstF1Line) * 2 + is525i;
1249 inOutStream <<
"F" << (isF2 ?
"2" :
"1") << (inForTextMode ?
"" :
" ");
1251 inOutStream <<
"L" <<
DEC0N(smpteLine,4);
1253 inOutStream <<
"L" <<
DEC(smpteLine);
1282 return inSegmentInfo;
1312 ULWord current (0xFFFFFFFF);
1313 ULWord previous (0xFFFFFFFF);
1314 ULWord first (0xFFFFFFFF);
1315 ULWord last (0xFFFFFFFF);
1320 while (iter != inObj.end())
1325 iter = inObj.begin();
1328 while (iter != inObj.end())
1331 if (previous == 0xFFFFFFFF)
1332 previous = first = last = current;
1333 else if (current == (previous + 1))
1334 last = previous = current;
1335 else if (current == previous)
1343 oss << first <<
"-" << last;
1344 pieces.push_back (oss.str ());
1346 first = last = previous = current;
1351 if (first != 0xFFFFFFFF && last != 0xFFFFFFFF)
1357 oss << first <<
"-" << last;
1358 pieces.push_back (oss.str ());
1364 if (++it != pieces.end())
@ NTV2_FBF_10BIT_YCBCR_420PL3_LE
See 3-Plane 10-Bit YCbCr 4:2:0 ('I420_10LE' a.k.a. 'YUV-P420-L10').
#define HD_NUMCOMPONENTPIXELS_1080_DVCPRO
NTV2SegmentedXferInfo & setDestOffset(const ULWord inOffset)
Sets my destination offset.
#define HD_NUMCOMPONENTPIXELS_2K
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
#define NTV2_IS_VANCMODE_TALLER(__v__)
@ NTV2_FBF_10BIT_YCBCR_420PL2
10-Bit 4:2:0 2-Plane YCbCr
NTV2SegmentedXferInfo & setSegmentCount(const ULWord inNumSegments)
Sets my segment count.
#define HD_NUMCOMPONENTPIXELS_720_DVCPRO
#define RGB12PLINEPITCH_3840
#define RGB48LINEPITCH_7680
@ NTV2_STANDARD_2Kx1080p
Identifies SMPTE HD 2K1080p.
#define RGB12PLINEPITCH_525
#define HD_YCBCRLINEPITCH_1080
NTV2SegmentedXferInfo & setSourceOffset(const ULWord inOffset)
Sets my source offset.
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
#define NTV2_IS_FBF_PLANAR(__s__)
#define HD_YCBCRLINEPITCH_1080_DVCPRO
#define RGB12PLINEPITCH_4096
NTV2FrameGeometry GetNTV2FrameGeometryFromVideoFormat(const NTV2VideoFormat inVideoFormat)
NTV2SegmentedXferInfo & setSourcePitch(const ULWord inPitch)
Sets my source pitch.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
ULWord GetLastLine(const NTV2FieldID inRasterFieldID=NTV2_FIELD0) const
#define NTV2_IS_2K1080_STANDARD(__s__)
ULWord GetByteCount(void) const
#define NTV2_ASSERT(_expr_)
@ NTV2_STANDARD_1080
Identifies SMPTE HD 1080i or 1080psf.
@ NTV2_STANDARD_625
Identifies SMPTE SD 625i.
#define FD_YCBCRLINEPITCH_UHD2
NTV2SegmentedXferInfo & setSegmentLength(const ULWord inNumElements)
Sets my segment length.
#define RGB24LINEPITCH_625
#define HD_NUMACTIVELINES_2K
#define RGB12PLINEPITCH_8192
#define RGB12PLINEPITCH_7680
bool Deallocate(void)
Deallocates my user-space storage (if I own it – i.e. from a prior call to Allocate).
#define DEC0N(__x__, __n__)
NTV2FieldID
These values are used to identify fields for interlaced video. See Field/Frame Interrupts and CNTV2Ca...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
NTV2FrameGeometry GetVANCFrameGeometry(const NTV2FrameGeometry inFrameGeometry, const NTV2VANCMode inVancMode)
#define NTV2_IS_2K_1080_VIDEO_FORMAT(__f__)
NTV2SmpteLineNumber(const NTV2Standard inStandard=NTV2_STANDARD_INVALID)
Constructs me from a given NTV2Standard.
@ NTV2_FIELD0
Identifies the first field in time for an interlaced video frame, or the first and only field in a pr...
#define HD_YCBCRLINEPITCH_2K
#define RGB24LINEPITCH_720
Describes a segmented data transfer (copy or move) from a source memory location to a destination loc...
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
#define RGB48LINEPITCH_8192
#define HD_YCBCRLINEPITCH_720
@ NTV2_VANCMODE_TALL
This identifies the "tall" mode in which there are some VANC lines in the frame buffer.
NTV2Standard
Identifies a particular video standard.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
#define RGB48LINEPITCH_3840
#define NUMACTIVELINES_525
#define HD_NUMACTIVELINES_720
#define NTV2_IS_VALID_STANDARD(__s__)
#define NUMACTIVELINES_625
#define FD_NUMCOMPONENTPIXELS_UHD2
#define RGB48LINEPITCH_2048
#define RGB24LINEPITCH_3840
#define RGB12PLINEPITCH_1080
std::string NTV2StandardToString(const NTV2Standard inValue, const bool inForRetailDisplay=false)
#define HD_NUMCOMPONENTPIXELS_QUADHD
#define RGB24LINEPITCH_8192
@ NTV2_STANDARD_1080p
Identifies SMPTE HD 1080p.
NTV2SegmentedXferInfo & setDestPitch(const ULWord inPitch)
Sets my destination pitch.
#define NTV2_IS_VANCMODE_ON(__v__)
Declares numerous NTV2 utility functions.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
#define FD_NUMCOMPONENTPIXELS_8K
@ NTV2_FBF_10BIT_YCBCR_422PL2
10-Bit 4:2:2 2-Plane YCbCr
#define HD_YCBCRLINEPITCH_720_HDV
#define RGB48LINEPITCH_525
std::vector< std::string > NTV2StringList
NTV2VANCMode
These enum values identify the available VANC modes.
#define RGB48LINEPITCH_4096
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
#define HD_YCBCRLINEPITCH_1080_HDV
#define HD_NUMCOMPONENTPIXELS_720_HDV
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
#define RGB12PLINEPITCH_625
NTV2SegmentedXferInfo & setElementLength(const ULWord inBytesPerElement)
Sets my element length.
#define RGB48LINEPITCH_625
@ NTV2_VANCMODE_TALLER
This identifies the mode in which there are some + extra VANC lines in the frame buffer.
#define RGB48LINEPITCH_720
@ NTV2_STANDARD_525
Identifies SMPTE SD 525i.
#define RGB24LINEPITCH_7680
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
#define HD_YCBCRLINEPITCH_720_DVCPRO
#define YCBCRLINEPITCH_SD
@ NTV2_FBF_10BIT_YCBCR_422PL3_LE
See 3-Plane 10-Bit YCbCr 4:2:2 ('I422_10LE' a.k.a. 'YUV-P-L10').
#define RGB48LINEPITCH_1080
@ NTV2_STANDARD_2Kx1080i
Identifies SMPTE HD 2K1080psf.
#define NTV2_IS_VALID_FIELD(__x__)
#define RGB24LINEPITCH_4096
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
@ NTV2_FBF_8BIT_YCBCR_420PL3
See 3-Plane 8-Bit YCbCr 4:2:0 ('I420' a.k.a. 'YUV-P420').
#define HD_NUMCOMPONENTPIXELS_1080
NTV2FrameGeometry GetGeometryFromStandard(const NTV2Standard inStandard)
@ NTV2_STANDARD_720
Identifies SMPTE HD 720p.
#define HD_NUMCOMPONENTPIXELS_720
@ NTV2_FBF_8BIT_YCBCR_422PL2
8-Bit 4:2:2 2-Plane YCbCr
#define HD_YCBCRLINEPITCH_3840
NTV2StringList::const_iterator NTV2StringListConstIter
#define RGB12PLINEPITCH_2048
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
@ NTV2_FBF_8BIT_YCBCR_422PL3
See 3-Plane 8-Bit YCbCr 4:2:2 (Weitek 'Y42B' a.k.a. 'YUV-P8').
#define HD_YCBCRLINEPITCH_4K
#define HD_NUMCOMPONENTPIXELS_4K
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define RGB12PLINEPITCH_720
#define xHEX0N(__x__, __n__)
bool firstFieldTop
True if the first field on the wire is the top-most field in the raster (field dominance)
#define HD_NUMCOMPONENTPIXELS_1080_HDV
#define NTV2_IS_VALID_VANCMODE(__v__)
#define NUMCOMPONENTPIXELS
#define FD_YCBCRLINEPITCH_8K
#define RGB24LINEPITCH_1080
#define RGB24LINEPITCH_525
#define HD_NUMACTIVELINES_1080
#define NTV2_IS_VALID_VIDEO_FORMAT(__f__)
#define RGB24LINEPITCH_2048
#define NTV2_IS_PROGRESSIVE_STANDARD(__s__)
bool Set(const void *pInUserPointer, const size_t inByteCount)
Sets (or resets) me from a client-supplied address and size.