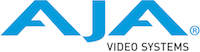 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
16 #define NTV2_BUFFER_LOCK
18 #define NTV2_AUDIOSIZE_MAX (401 * 1024)
19 #define NTV2_ANCILLARYSIZE_MAX (256 * 1024)
55 while (mConsumerThread.
Active())
58 while (mProducerThread.
Active())
83 if (!mDevice.
features().CanDoCapture())
87 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support '"
101 cerr <<
"## ERROR: Unable to acquire '" << mDevice.
GetDisplayName() <<
"' because another app (pid " << appPID <<
") owns it" << endl;
108 if (mDevice.
features().CanDoMultiFormat())
148 if (mDevice.IsRemote())
149 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl;
151 #endif // defined(_DEBUG)
169 {cerr <<
"## ERROR: No input signal or unknown format" << endl;
return AJA_STATUS_NOINPUT;}
217 ULWord F1AncSize(0), F2AncSize(0);
218 ULWord F1OffsetFromEnd(0), F2OffsetFromEnd(0);
222 F1AncSize = F2OffsetFromEnd > F1OffsetFromEnd ? 0 : F1OffsetFromEnd - F2OffsetFromEnd;
223 F2AncSize = F2OffsetFromEnd > F1OffsetFromEnd ? F2OffsetFromEnd - F1OffsetFromEnd : F2OffsetFromEnd;
236 mAVCircularBuffer.
Add(&frameData);
238 #ifdef NTV2_BUFFER_LOCK
286 mConsumerThread.
Start();
307 uint64_t ancTally(0);
308 uint64_t audioTally(0);
309 uint64_t dolbyTally(0);
329 if (pData[i] == 0xff)
333 cout <<
"f1 size reg " <<
DEC(pFrameData->
NumCapturedAncBytes()) <<
" ffs " <<
DEC(i) <<
" samples " <<
DEC(audioSize/4) << endl << flush;
342 if (pData[i] == 0xff)
351 if (pOFS && !ancTally++)
364 if (pAFS && !audioTally++)
367 pAFS->write(pFrameData->
AudioBuffer(), streamsize(audioSize));
372 pAFS->write(pFrameData->
AudioBuffer(), streamsize(audioSize));
378 if (pDFS && !dolbyTally++)
382 pDFS->write(pFrameData->
AncBuffer(), streamsize(dolbySize));
388 pDFS->write(pFrameData->
AncBuffer2(), streamsize(dolbySize));
396 {
delete pOFS; cerr <<
"Wrote " <<
DEC(ancTally) <<
" frames of raw anc data" << endl; }
398 {
delete pAFS; cerr <<
"Wrote " <<
DEC(audioTally) <<
" frames of raw audio data" << endl; }
400 {
delete pDFS; cerr <<
"Wrote " <<
DEC(dolbyTally) <<
" frames of dolby data" << endl; }
401 CAPNOTE(
"Thread completed, will exit");
414 mProducerThread.
Start();
435 ULWord acOptions (0), overruns(0);
495 {
bool overrun(
false);
498 {overruns++;
CAPWARN(overruns <<
" aux overrun(s)");}
502 stale.
Fill(uint8_t(0));
509 stale.
Fill(uint8_t(0));
527 CAPNOTE(
"Thread completed, will exit");
543 const uint8_t * pInAncData(inAncBuffer);
544 uint8_t * pOutAudioData(outAudioBuffer);
545 uint32_t audioSize(0);
548 for (uint32_t num(0); num < ancSize / 32; num++)
551 if (pInAncData[0] == 0x02)
554 if ((pInAncData[1] & 0x01))
557 if ((pInAncData[2] & 0x01))
559 *pOutAudioData++ = 0;
560 *pOutAudioData++ = 0;
561 *pOutAudioData++ = 0;
562 *pOutAudioData++ = 0;
566 *pOutAudioData++ = pInAncData[4];
567 *pOutAudioData++ = pInAncData[5];
568 *pOutAudioData++ = pInAncData[7];
569 *pOutAudioData++ = pInAncData[8];
575 if ((pInAncData[1] & 0x10) == 0)
578 if ((pInAncData[1] & 0x02))
581 if ((pInAncData[2] & 0x02))
583 *pOutAudioData++ = 0;
584 *pOutAudioData++ = 0;
585 *pOutAudioData++ = 0;
586 *pOutAudioData++ = 0;
590 *pOutAudioData++ = pInAncData[11];
591 *pOutAudioData++ = pInAncData[12];
592 *pOutAudioData++ = pInAncData[14];
593 *pOutAudioData++ = pInAncData[15];
599 if ((pInAncData[1] & 0x04))
602 if ((pInAncData[2] & 0x04))
604 *pOutAudioData++ = 0;
605 *pOutAudioData++ = 0;
606 *pOutAudioData++ = 0;
607 *pOutAudioData++ = 0;
611 *pOutAudioData++ = pInAncData[18];
612 *pOutAudioData++ = pInAncData[19];
613 *pOutAudioData++ = pInAncData[21];
614 *pOutAudioData++ = pInAncData[22];
620 if ((pInAncData[1] & 0x08))
623 if ((pInAncData[2] & 0x08))
625 *pOutAudioData++ = 0;
626 *pOutAudioData++ = 0;
627 *pOutAudioData++ = 0;
628 *pOutAudioData++ = 0;
632 *pOutAudioData++ = pInAncData[25];
633 *pOutAudioData++ = pInAncData[26];
634 *pOutAudioData++ = pInAncData[28];
635 *pOutAudioData++ = pInAncData[29];
648 const uint16_t * pInAudioData(inAudioBuffer);
649 uint16_t * pOutDolbyData(outDolbyBuffer);
650 uint32_t dolbySize(0);
653 for (uint32_t cnt(0); cnt < inAudioSize / 2; cnt++)
658 case 0: mDolbyState = (*pInAudioData == 0xf872) ? 1 : 0;
661 case 1: mDolbyState = (*pInAudioData == 0x4e1f) ? 2 : 0;
665 case 2: mDolbyState = ((*pInAudioData & 0xff) == 0x15) ? 3 : 0;
669 case 3: mDolbyLength = uint32_t(*pInAudioData / 2);
674 case 4:
if (mDolbyLength)
679 *pOutDolbyData = (*pInAudioData >> 8) & 0xff;
680 *pOutDolbyData |= (*pInAudioData & 0xff) << 8;
690 default: mDolbyState = 0;
695 return dolbySize * 2;
ULWord NumCapturedAnc2Bytes(void) const
bool fDoMultiFormat
If true, use multi-format/multi-channel mode, if device supports it; otherwise normal mode.
std::string fDolbyDataFilePath
Optional path to Dolby binary data file.
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the capture thread's static callback function that gets called when the capture thread runs....
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
Declares the NTV2DolbyCapture class.
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
bool fDoAudioFilter
If true, capture only audio anc.
@ AJA_ThreadPriority_High
NTV2DolbyCapture(const DolbyCaptureConfig &inConfig)
Constructs me using the given settings.
UWord firstFrame(void) const
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
#define NTV2_ANCILLARYSIZE_MAX
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
This class is used to configure an NTV2Capture instance.
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
#define NTV2_VIDEO_FORMAT_HAS_PROGRESSIVE_PICTURE(__f__)
ULWord fNumAncBytes
Actual number of captured F1 anc bytes.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
virtual void GetACStatus(ULWord &outGoodFrames, ULWord &outDroppedFrames, ULWord &outBufferLevel)
Provides status information about my input (capture) process.
virtual bool RouteInputSignal(void)
Sets up device routing for capture.
ULWord GetByteCount(void) const
virtual bool AuxExtractSetFilterInclusionMode(const UWord inHDMIInput, const bool inEnable)
Enables or disables HDMI AUX packet filtering for the given HDMI input.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
NTV2Buffer & AncBuffer2(void)
static bool GetInputRouting(NTV2XptConnections &outConnections, const CaptureConfig &inConfig, const bool isInputRGB=(0))
Answers with the crosspoint connections needed to implement the given capture configuration.
virtual void CaptureFrames(void)
Repeatedly captures frames using AutoCirculate (until global quit flag set).
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
ULWord GetProcessedFrameCount(void) const
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
NTV2PixelFormat fPixelFormat
Pixel format to use.
virtual ~NTV2DolbyCapture()
virtual bool AncSetFrameBufferSize(const ULWord inF1Size, const ULWord inF2Size)
Sets the capacity of the SDI ANC or HDMI AUX buffers in device frame memory. (Call NTV2DeviceCanDoCus...
ULWord GetBufferLevel(void) const
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
#define NTV2_AUDIOSIZE_MAX
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
@ NTV2_IOKINDS_HDMI
Specifies HDMI input/output kinds.
NTV2Buffer fAncBuffer2
Additional "F2" host anc buffer.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
std::string fAncDataFilePath
Optional path to Anc binary data file.
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
@ NTV2_LHIHDMIColorSpaceRGB
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=(0))
Applies the given routing table to the AJA device.
bool SetAncBuffers(ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my ancillary data buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
AJALabelValuePairs Get(const bool inCompact=(0)) const
NTV2Buffer fAncBuffer
Host ancillary data buffer.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
NTV2Buffer & VideoBuffer(void)
#define NTV2_IS_4K_VIDEO_FORMAT(__f__)
@ kVRegAncField2Offset
Anc Field2 byte offset from end of frame buffer (GUMP on all boards except RTP for SMPTE2022/IP)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=(0), bool inRDMA=(0))
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
virtual class DeviceCapabilities & features(void)
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
ULWord NumCapturedAncBytes(void) const
std::string fAudioDataFilePath
Optional path to Audio binary data file.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
std::string NTV2InputSourceToString(const NTV2InputSource inValue, const bool inForRetailDisplay=false)
Declares the AJAProcess class.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread runs...
AJALabelValuePairs Get(const bool inCompact=(0)) const
static AJALabelValuePairs & append(AJALabelValuePairs &inOutTable, const std::string &inLabel, const std::string &inValue=std::string())
A convenience function that appends the given label and value strings to the provided AJALabelValuePa...
virtual std::string GetDescription(void) const
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
ULWord VideoBufferSize(void) const
@ kVRegAncField1Offset
Anc Field1 byte offset from end of frame buffer (GUMP on all boards except RTP for SMPTE2022/IP)
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
virtual uint32_t RecoverAudio(const NTV2Buffer &inAncBuffer, const uint32_t inAncSize, NTV2Buffer &outAudioBuffer)
Recover audio from ancillary data.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
ULWord AncBufferSize(void) const
ULWord GetCapturedAncByteCount(const bool inField2=false) const
virtual void StartConsumerThread(void)
Starts my frame consumer thread.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord AncBuffer2Size(void) const
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
std::string fDeviceSpec
The AJA device to use.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
#define NTV2_INPUT_SOURCE_IS_HDMI(_inpSrc_)
NTV2Buffer & AudioBuffer(void)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
virtual bool AuxExtractSetPacketFilters(const UWord inHDMIInput, const NTV2DIDSet &inDIDs)
Replaces the HDMI packet types to be excluded (filtered) by the given HDMI input's Aux extractor.
virtual bool AuxExtractGetBufferOverrun(const UWord inHDMIInput, bool &outIsOverrun, const UWord inField=0)
Answers whether or not the given HDMI input's Aux extractor reached its buffer limits.
virtual bool IsDeviceReady(const bool inCheckValid=(0))
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
bool CanDoInputSource(const NTV2InputSource inSrc)
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
virtual void StartProducerThread(void)
Starts my capture thread.
virtual bool SetTsiFrameEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 two-sample interleave (Tsi) frame mode on the device.
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
#define NTV2_IS_VALID_CHANNEL(__x__)
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
@ NTV2_DISABLE_TASKS
0: Disabled: Device is completely configured by controlling application(s) – no driver involvement.
NTV2Channel fInputChannel
The device channel to use.
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
NTV2Buffer fAudioBuffer
Host audio buffer.
@ NTV2_LHIHDMIColorSpaceYCbCr
UWord lastFrame(void) const
bool IsRunning(void) const
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
bool fDoFrameStats
if true, output per frame statistics
virtual AJAStatus Run(void)
Runs me.
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
#define AUTOCIRCULATE_WITH_HDMIAUX
Use this to AutoCirculate with HDMI auxiliary data.
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
ULWord GetDroppedFrameCount(void) const
ULWord fNumAnc2Bytes
Actual number of captured F2 anc bytes.
virtual bool GetHDMIInputColor(NTV2LHIHDMIColorSpace &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current colorspace for the given HDMI input.
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual void Quit(void)
Gracefully stops me from running.
bool WithCustomAnc(void) const
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
I capture HDMI Dolby audio from an HDMI input of an AJA device.
std::ostream & operator<<(std::ostream &ioStrm, const DolbyCaptureConfig &inObj)
virtual AJAStatus Start()
std::set< UByte > NTV2DIDSet
A set of distinct NTV2DID values.
static NTV2DIDSet AuxExtractGetDefaultPacketFilters(void)
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
#define AJA_FAILURE(_status_)
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
NTV2Buffer & AncBuffer(void)
virtual uint32_t RecoverDolby(const NTV2Buffer &inAudioBuffer, const uint32_t inAudioSize, NTV2Buffer &outDolbyBuffer)
Recover Dolby data from the given audio data.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
NTV2InputSource fInputSource
The device input connector to use.
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
virtual void ConsumeFrames(void)
Repeatedly consumes frames from the circular buffer (until global quit flag set).