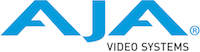 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
26 mSingleDevice (
false),
63 while (mPlayThread.
Active())
66 while (mCaptureThread.
Active())
82 if (!mInputDevice.
features().CanDo4KVideo())
94 if (!mOutputDevice.
features().CanDo4KVideo())
102 if (mInputDevice.
features().GetNumFrameStores() < 8)
109 {cerr <<
"## ERROR: Input device '" << mConfig.
fDeviceSpec <<
"' is in use by another application" << endl;
return AJA_STATUS_BUSY;}
116 {cerr <<
"## ERROR: Output device '" << mConfig.
fDeviceSpec2 <<
"' is in use by another application" << endl;
return AJA_STATUS_BUSY;}
122 if (mInputDevice.
features().CanDoMultiFormat())
124 if (mOutputDevice.
features().CanDoMultiFormat())
128 switch (mInputDevice.
features().GetNumFrameStores())
145 switch (mOutputDevice.
features().GetNumFrameStores())
194 if (mInputDevice.IsRemote())
195 cerr <<
"Input Device Desc: " << mInputDevice.
GetDescription() << endl;
196 if (mOutputDevice.IsRemote())
197 cerr <<
"Output Device Desc: " << mOutputDevice.
GetDescription() << endl;
200 BURNINFO(
"Configuration: " << mConfig);
223 if (mInputDevice.
features().HasBiDirectionalSDI())
266 if (mOutputDevice.
features().HasBiDirectionalSDI())
354 const uint16_t numberOfAudioChannels (mInputDevice.
features().GetMaxAudioChannels());
382 const uint16_t numberOfAudioChannels (mOutputDevice.
features().GetMaxAudioChannels());
441 BURNFAIL(
"Failed to allocate " <<
xHEX0N(vidBuffSizeBytes,8) <<
"-byte video buffer");
446 if (mConfig.WithAudio())
456 mFrameDataRing.
Add(&frameData);
584 ULWord goodXfers(0), badXfers(0), starves(0), noRoomWaits(0);
608 {
BURNFAIL(
"AutoCirculateInitForOutput failed"); mGlobalQuit =
true;}
621 {starves++;
continue;}
651 BURNNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
652 <<
DEC(starves) <<
" ring starves, " <<
DEC(noRoomWaits) <<
" device starves");
669 mCaptureThread.
Start();
692 ULWord goodXfers(0), badXfers(0), ringFulls(0), devWaits(0);
717 {
BURNFAIL(
"AutoCirculateInitForInput failed"); mGlobalQuit =
true;}
734 {ringFulls++;
continue;}
779 BURNNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
780 <<
DEC(ringFulls) <<
" ring full(s), " <<
DEC(devWaits) <<
" device waits");
798 switch (inInputSource)
819 if (regNum && mInputDevice.
ReadRegister(regNum, regValue) && regValue &
BIT(16))
@ NTV2_XptFrameBuffer6YUV
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual AJAStatus SetupOutputAudio(void)
Sets up everything I need for playing audio.
@ NTV2_XptFrameBuffer4YUV
virtual void RouteOutputSignal(void)
Sets up board routing for playout.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
@ NTV2_INPUTSOURCE_SDI4
Identifies the 4th SDI video input.
static void PlayThreadStatic(AJAThread *pThread, void *pContext)
This is the playout thread's static callback function that gets called when the playout thread runs....
const AUTOCIRCULATE_TRANSFER_STATUS & GetTransferStatus(void) const
Returns a constant reference to my AUTOCIRCULATE_TRANSFER_STATUS.
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
ULWord GetCapturedAudioByteCount(void) const
@ NTV2_REFERENCE_INPUT1
Specifies the SDI In 1 connector.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
UWord firstFrame(void) const
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
ULWord GetProcessedFrameCount(void) const
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
@ NTV2_XptFrameBuffer2RGB
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
Declares common types used in the ajabase library.
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2Burn4KQuadrant(const BurnConfig &inConfig)
Constructs me using the given configuration settings.
bool GetRP188Reg(RP188_STRUCT &outRP188) const
virtual void StartPlayThread(void)
Starts my playout thread.
virtual void StartCaptureThread(void)
Starts my capture thread.
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_1
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
@ NTV2_XptFrameBuffer8YUV
@ NTV2_XptFrameBuffer3YUV
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
NTV2TCIndex fTimecodeSource
Timecode source to use.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
virtual void CaptureFrames(void)
Repeatedly captures frames using AutoCirculate (until global quit flag set).
@ NTV2_XptFrameBuffer1RGB
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
bool fSuppressVideo
If true, suppress video; otherwise include video.
@ NTV2_XptFrameBuffer4Input
@ NTV2_XptFrameBuffer3Input
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
bool SetOutputTimeCode(const NTV2_RP188 &inTimecode, const NTV2TCIndex inTCIndex=NTV2_TCINDEX_SDI1)
Intended for playout, sets one element of my acOutputTimeCodes member.
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
bool CanAcceptMoreOutputFrames(void) const
virtual void Quit(void)
Gracefully stops me from running.
@ NTV2_XptFrameBuffer3RGB
static bool Get4KInputFormat(NTV2VideoFormat &inOutVideoFormat)
Given a video format, if all 4 inputs are the same and promotable to 4K, this function does the promo...
NTV2FrameRate
Identifies a particular video frame rate.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
NTV2Buffer & VideoBuffer(void)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
virtual bool EnableOutputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to output vertical blanking interrupts originat...
NTV2PixelFormat fPixelFormat
The pixel format to use.
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
virtual class DeviceCapabilities & features(void)
NTV2ACFrameRange fOutputFrames
Playout frame count or range.
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
static size_t DefaultPageSize(void)
virtual AJAStatus SetupOutputVideo(void)
Sets up everything I need for playing 4K video.
@ NTV2_TCINDEX_SDI1_LTC
SDI 1 embedded ATC LTC.
Declares the AJAProcess class.
virtual bool SetRP188SourceFilter(const NTV2Channel inSDIInput, const UWord inFilterValue)
Sets the RP188 DBB filter for the given SDI input.
@ NTV2_XptFrameBuffer1Input
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_TCINDEX_SDI1
SDI 1 embedded VITC.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
bool HasValidTimecode(const NTV2TCIndex inTCNdx) const
virtual std::string GetDescription(void) const
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
ULWord VideoBufferSize(void) const
@ NTV2_XptFrameBuffer1YUV
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=(!(0)))
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
static size_t SetDefaultPageSize(void)
NTV2_RP188 Timecode(const NTV2TCIndex inTCNdx) const
virtual void RouteInputSignal(void)
Sets up board routing for capture.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
virtual bool SetLTCInputEnable(const bool inEnable)
Enables or disables the ability for the device to read analog LTC on the reference input connector.
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
Header file for the NTV2Burn4KQuadrant demonstration class.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord AudioBufferSize(void) const
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
NTV2Buffer & AudioBuffer(void)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
virtual AJAStatus SetupInputAudio(void)
Sets up everything I need for capturing audio.
virtual bool IsDeviceReady(const bool inCheckValid=(0))
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
std::string fDeviceSpec2
Second AJA device to use (Burn4KQuadrant or BurnBoardToBoard only)
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
virtual AJAStatus Run(void)
Runs me.
#define NTV2_AUDIOSIZE_MAX
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
NTV2Channel fInputChannel
The input channel to use.
std::string fDeviceSpec
The AJA device to use.
NTV2InputSource
Identifies a specific video input source.
virtual AJAStatus SetupInputVideo(void)
Sets up everything I need for capturing 4K video.
NTV2Buffer fAudioBuffer
Host audio buffer.
virtual void PlayFrames(void)
Repeatedly plays out frames using AutoCirculate (until global quit flag set).
@ NTV2_XptFrameBuffer8RGB
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=(0))
Connects the given widget signal input (sink) to the given widget signal output (source).
static ULWord GetRP188RegisterForInput(const NTV2InputSource inInputSource)
Returns the RP188 DBB register number to use for the given NTV2InputSource.
virtual AJAStatus SetupHostBuffers(void)
Sets up my circular buffers.
@ NTV2_XptFrameBuffer6RGB
@ NTV2_XptFrameBuffer2Input
@ NTV2_XptFrameBuffer4RGB
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
UWord lastFrame(void) const
bool IsRunning(void) const
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
@ NTV2_INPUTSOURCE_SDI2
Identifies the 2nd SDI video input.
@ NTV2_XptFrameBuffer2YUV
This struct replaces the old RP188_STRUCT.
@ NTV2_XptFrameBuffer5RGB
bool IsRGBFormat(const NTV2FrameBufferFormat format)
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
@ NTV2_XptFrameBuffer7YUV
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
Instances of me can capture 4K/UHD video from one 4-channel AJA device, burn timecode into one quadra...
virtual bool SetEnableVANCData(const bool inVANCenabled, const bool inTallerVANC, const NTV2Standard inStandard, const NTV2FrameGeometry inGeometry, const NTV2Channel inChannel=NTV2_CHANNEL1)
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
@ NTV2_AUDIOSYSTEM_5
This identifies the 5th Audio System.
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
virtual void GetACStatus(AUTOCIRCULATE_STATUS &outInputStatus, AUTOCIRCULATE_STATUS &outOutputStatus)
Provides status information about my input (capture) and output (playout) processes.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
virtual ~NTV2Burn4KQuadrant()
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
bool GetInputTimeCodes(NTV2TimeCodeList &outValues) const
Intended for capture, answers with the timecodes captured in my acTransferStatus member's acFrameStam...
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
@ NTV2_INPUTSOURCE_SDI3
Identifies the 3rd SDI video input.
virtual AJAStatus Start()
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
#define xHEX0N(__x__, __n__)
#define AJA_FAILURE(_status_)
NTV2ACFrameRange fInputFrames
Ingest frame count or range.
@ NTV2_XptFrameBuffer5YUV
Configures an NTV2Burn or NTV2FieldBurn instance.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
@ NTV2_XptFrameBuffer7RGB
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
virtual bool InputSignalHasTimecode(void)
Returns true if the current input signal has timecode embedded in it; otherwise returns false.
bool GetRP188Str(std::string &sRP188) const
NTV2Channel fOutputChannel
The output channel to use.
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
NTV2TimeCodes fTimecodes
Map of TC indexes to NTV2_RP188 values.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
static void CaptureThreadStatic(AJAThread *pThread, void *pContext)
This is the capture thread's static callback function that gets called when the capture thread runs....