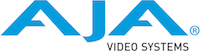 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
20 #define NTV2_BUFFER_LOCKING // IMPORTANT FOR 8K: Define this to pre-lock video/audio buffers in kernel
23 #define TCFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
24 #define TCWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
25 #define TCNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
26 #define TCINFO(_expr_) AJA_sINFO (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
27 #define TCDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
49 static const double gFrequencies [] = {250.0, 500.0, 1000.0, 2000.0};
63 mToneFrequency (440.0),
89 while (mProducerThread.
Active())
92 while (mConsumerThread.
Active())
95 #if defined(NTV2_BUFFER_LOCKING)
97 #endif // NTV2_BUFFER_LOCKING
130 cerr <<
"## ERROR: Cannot use channel '" <<
DEC(mConfig.
fOutputChannel+1) <<
"' -- device only supports channel 1"
131 << (maxNumChannels > 1 ? string(
" thru ") + string(1,
char(maxNumChannels+
'0')) :
"") << endl;
136 cerr <<
"## ERROR: 8K/UHD2 requires Ch1 or Ch3, not Ch" <<
DEC(mConfig.
fOutputChannel) << endl;
174 {cerr <<
"## ERROR: RenderTimeCodeFont failed for: " << mFormatDesc << endl;
return AJA_STATUS_UNSUPPORTED;}
179 if (mDevice.IsRemote())
180 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl;
182 #endif // defined(_DEBUG)
194 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
199 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
244 {cerr <<
"## WARNING: HDR Anc requested, but device can't do custom anc" << endl;
267 numAudioChannels = 8;
273 switch (mAudioSystem)
279 for (
UWord chan(0); chan < numChan; chan++)
337 if (mConfig.WithAudio())
340 PLFAIL(
"Failed to allocate " <<
xHEX0N(audioBufferSize,8) <<
"-byte audio buffer");
346 #ifdef NTV2_BUFFER_LOCKING
350 mFrameDataRing.
Add (&frameData);
360 vector<NTV2TestPatternSelect> testPatIDs;
378 mTestPatRasters.clear();
379 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
384 if (mFormatDesc.IsVANC())
388 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
392 {
PLFAIL(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": "
399 if (!testPatternGen.
DrawTestPattern (testPatIDs.at(tpNdx), mFormatDesc, mTestPatRasters.at(tpNdx)))
401 cerr <<
"## ERROR: DrawTestPattern " <<
DEC(tpNdx) <<
" failed: " << mFormatDesc << endl;
405 #ifdef NTV2_BUFFER_LOCKING
408 PLWARN(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": failed to pre-lock");
619 mConsumerThread.
Start();
642 ULWord goodXfers(0), badXfers(0), prodWaits(0), noRoomWaits(0);
643 const UWord numACFramesPerChannel(7);
666 uint32_t hdrPktSize (0);
671 PLWARN(
"HDR anc insertion disabled -- GenerateTransmitData failed");
677 #ifdef NTV2_BUFFER_LOCKING
684 const UWord endNum (mConfig.
fOutputChannel < 2 ? numACFramesPerChannel-1 : numACFramesPerChannel*2-1);
697 1 , startNum, endNum));
699 {
PLFAIL(
"AutoCirculateInitForOutput failed"); mGlobalQuit =
true;}
712 {prodWaits++;
continue;}
718 const CRP188 rp188Info (mCurrentFrame++, 0, 0, 10, tcFormat);
720 string timeCodeString;
753 PLNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
754 <<
DEC(prodWaits) <<
" starves, " <<
DEC(noRoomWaits) <<
" VBI waits");
768 mProducerThread.
Start();
785 ULWord freqNdx(0), testPatNdx(0), badTally(0);
786 double timeOfLastSwitch (0.0);
805 NTV2Buffer & testPatVidBuffer(mTestPatRasters.at(testPatNdx));
814 if (currentTime > timeOfLastSwitch + 4.0)
817 testPatNdx = (testPatNdx + 1) %
ULWord(mTestPatRasters.size());
819 timeOfLastSwitch = currentTime;
820 PLINFO(
"F" <<
DEC0N(mCurrentFrame,6) <<
": tone=" << mToneFrequency <<
"Hz, pattern='" << tpNames.at(testPatNdx) <<
"'");
827 PLNOTE(
"Thread completed: " <<
DEC(mCurrentFrame) <<
" frame(s) produced, " <<
DEC(badTally) <<
" failed");
849 ULWord bytesWritten(0), startSample(mCurrentSample);
852 mCurrentSample = startSample;
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
@ NTV2_XptFrameBuffer4YUV
virtual void StartConsumerThread(void)
Starts my consumer thread.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
static const ULWord gNumFrequencies(sizeof(gFrequencies)/sizeof(double))
bool fDoHDMIOutput
If true, enable HDMI output; otherwise, disable HDMI output.
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
virtual bool GetAncRegionOffsetFromBottom(ULWord &outByteOffsetFromBottom, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All)
Answers with the byte offset to the start of an ancillary data region within a device frame buffer,...
@ NTV2_TestPatt_CheckField
Declares the AJAAncillaryData_HDR_HLG class.
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
virtual AJAStatus SetUpHostBuffers(void)
Sets up my host video & audio buffers.
@ NTV2_XptFrameBuffer1_DS2YUV
#define NTV2_IS_VALID_TASK_MODE(__m__)
@ NTV2_XptSDIOut4InputDS2
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
virtual uint32_t AddTone(ULWord *audioBuffer)
Inserts audio tone (based on my current tone frequency) into the given audio buffer.
@ NTV2_XptFrameBuffer2RGB
@ NTV2_TestPatt_MultiBurst
virtual AJAStatus SetUpAudio(void)
Performs all audio setup.
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
@ NTV2_XptSDIOut3InputDS2
#define IS_KNOWN_AJAAncDataType(_x_)
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
bool GetRP188Reg(RP188_STRUCT &outRP188) const
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=false)
Applies the given routing table to the AJA device.
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
Header file for NTV2Player8K demonstration class.
ULWord GetByteCount(void) const
@ NTV2_AUDIOSYSTEM_4
This identifies the 4th Audio System.
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
@ NTV2_XptFrameBuffer3_DS2YUV
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
#define NTV2_IS_QUAD_QUAD_HFR_VIDEO_FORMAT(__f__)
virtual void Quit(void)
Gracefully stops me from running.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
@ NTV2_XptFrameBuffer4_DS2YUV
@ NTV2_XptFrameBuffer3YUV
bool Deallocate(void)
Deallocates my user-space storage (if I own it – i.e. from a prior call to Allocate).
virtual void StartProducerThread(void)
Starts my producer thread.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
virtual AJAStatus SetUpTestPatternBuffers(void)
Creates my test pattern buffers.
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
Declares the NTV2TestPatternGen class.
NTV2Buffer acAudioBuffer
The host audio buffer. This field is owned by the client application, and thus is responsible for all...
@ NTV2_TestPatt_ColorBars100
std::pair< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnection
#define DEC0N(__x__, __n__)
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
@ NTV2_AncRgn_Field2
Identifies the "normal" Field 2 ancillary data region.
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO3
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 3.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
@ NTV2_XptFrameBuffer1RGB
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
@ NTV2_XptSDIOut2InputDS2
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
bool NTV2DeviceCanDoFrameBufferFormat(const NTV2DeviceID inDeviceID, const NTV2FrameBufferFormat inFBFormat)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
bool SetOutputTimeCode(const NTV2_RP188 &inTimecode, const NTV2TCIndex inTCIndex=NTV2_TCINDEX_SDI1)
Intended for playout, sets one element of my acOutputTimeCodes member.
const char * NTV2FrameBufferFormatString(NTV2FrameBufferFormat fmt)
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
bool CanAcceptMoreOutputFrames(void) const
@ NTV2_XptFrameBuffer3RGB
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
NTV2FrameRate
Identifies a particular video frame rate.
This class handles "5251" Frame Status Information packets.
bool NTV2DeviceCanDoVideoFormat(const NTV2DeviceID inDeviceID, const NTV2VideoFormat inVideoFormat)
#define NTV2_IS_UHD2_FULL_VIDEO_FORMAT(__f__)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
@ NTV2_XptFrameBuffer2_DS2YUV
bool fDoTsiRouting
If true, enable TSI routing; otherwise route for square division (4K/8K)
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO1
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 1.
AJAAncDataType fTransmitHDRType
Specifies the HDR anc data packet to transmit, if any.
NTV2AudioSystemSet NTV2MakeAudioSystemSet(const NTV2AudioSystem inFirstAudioSystem, const UWord inCount=1)
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
virtual bool DMABufferUnlockAll()
Unlocks all previously-locked buffers used for DMA transfers.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
@ NTV2_XptFrameBuffer4_DS2RGB
I am an object that can play out an 8K or UHD2 test pattern (with timecode) to 4 x 12G SDI outputs of...
@ NTV2_XptFrameBuffer1_DS2RGB
virtual void ConsumeFrames(void)
My consumer thread that repeatedly plays frames using AutoCirculate (until quit).
@ NTV2_XptFrameBuffer2_DS2RGB
Declares the AJAProcess class.
virtual AJAStatus SetUpVideo(void)
Performs all video setup.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual bool GetNumberAudioChannels(ULWord &outNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current number of audio channels being captured or played by a given Audio System on the ...
@ NTV2_TestPatt_FlatField
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
@ NTV2_XptFrameBuffer1YUV
@ NTV2_XptDuallinkOut2DS2
@ NTV2_XptDuallinkOut1DS2
static size_t SetDefaultPageSize(void)
@ NTV2_XptDuallinkOut4DS2
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC=false, const bool inIsF2=false)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the producer thread's static callback function that gets called when the producer thread star...
std::set< NTV2AudioSystem > NTV2AudioSystemSet
A set of distinct NTV2AudioSystem values. New in SDK 16.2.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
virtual bool SetSDIOutRGBLevelAConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables an RGB-over-3G-level-A conversion at the SDI output widget (assuming the AJA devi...
UWord fNumAudioLinks
The number of audio systems to control for multi-link audio (4K/8K)
@ NTV2_TestPatt_ColorQuadrant
@ NTV2_TestPatt_ColorQuadrantBorder
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
NTV2VideoFormat fVideoFormat
The video format to use.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
@ NTV2_XptDualLinkOut4Input
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
NTV2Channel fOutputChannel
The device channel to use.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
This class handles "5251" Frame Status Information packets.
NTV2Buffer & AudioBuffer(void)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=false, bool inRDMA=false)
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
@ NTV2_TestPatt_SlantRamp
@ NTV2_XptSDIOut1InputDS2
UWord NTV2DeviceGetNumFrameStores(const NTV2DeviceID inDeviceID)
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
@ NTV2_TestPatt_ColorBars75
virtual bool IsDeviceReady(const bool inCheckValid=(0))
@ NTV2_TestPatt_ZonePlate
bool NTV2DeviceCanDoPlayback(const NTV2DeviceID inDeviceID)
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
virtual void ProduceFrames(void)
My producer thread that repeatedly produces video frames.
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
static const bool BUFFER_PAGE_ALIGNED(true)
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual bool SetHDMIOutAudioRate(const NTV2AudioRate inNewValue)
Sets the HDMI output's audio rate.
This class handles "5251" Frame Status Information packets.
bool AddAudioTone(ULWord &outNumBytesWritten, NTV2Buffer &inAudioBuffer, ULWord &inOutCurrentSample, const ULWord inNumSamples, const double inSampleRate, const double inAmplitude, const double inFrequency, const ULWord inNumBits, const bool inByteSwap, const ULWord inNumChannels)
Fills the given buffer with 32-bit (ULWord) audio tone samples.
virtual bool RouteOutputSignal(void)
Performs all widget/signal routing for playout.
static const uint32_t gAudMaxSizeBytes(256 *1024)
The maximum number of bytes of 48KHz audio that can be transferred for a single frame....
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
The NTV2 test pattern generator.
void * GetHostPointer(void) const
virtual std::string GetDescription(void) const
NTV2Buffer fAudioBuffer
Host audio buffer.
static ULWord gAncMaxSizeBytes(NTV2_ANCSIZE_MAX)
The maximum number of bytes of ancillary data that can be transferred for a single field....
virtual AJAStatus Run(void)
Runs me.
bool fDoRGBOnWire
If true, produce RGB on the wire; otherwise output YUV.
@ NTV2_AUDIOSYSTEM_3
This identifies the 3rd Audio System.
virtual ~NTV2Player8K(void)
@ NTV2_XptFrameBuffer4RGB
#define AUTOCIRCULATE_WITH_MULTILINK_AUDIO2
Use this to AutoCirculate with base audiosystem controlling base AudioSystem + 2.
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
Declares the AJAAncillaryData_HDR_HDR10 class.
std::string fDeviceSpec
The AJA device to use.
@ NTV2_XptDualLinkOut3Input
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
NTV2ChannelSet NTV2MakeChannelSet(const NTV2Channel inFirstChannel, const UWord inNumChannels=1)
@ NTV2_XptFrameBuffer2YUV
This struct replaces the old RP188_STRUCT.
NTV2Buffer acVideoBuffer
The host video buffer. This field is owned by the client application, and thus is responsible for all...
bool IsRGBFormat(const NTV2FrameBufferFormat format)
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread star...
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
ULWord GetAudioSamplesPerFrame(const NTV2FrameRate inFrameRate, const NTV2AudioRate inAudioRate, ULWord inCadenceFrame=0, bool inIsSMPTE372Enabled=false)
Returns the number of audio samples for a given video frame rate, audio sample rate,...
NTV2Player8K(const PlayerConfig &inConfig)
Constructs me using the given configuration settings.
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
virtual bool EnableChannels(const NTV2ChannelSet &inChannels, const bool inDisableOthers=false)
Enables the given FrameStore(s).
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual AJAStatus GenerateTransmitData(uint8_t *pBuffer, const size_t inMaxBytes, uint32_t &outPacketSize)
Generates "raw" ancillary data from my internal ancillary data (playback) – see SDI Anc Buffer Data F...
@ NTV2_XptDualLinkOut2Input
@ NTV2_AudioChannel1_8
This selects audio channels 1 thru 8.
virtual NTV2DeviceID GetDeviceID(void)
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
virtual void GetACStatus(AUTOCIRCULATE_STATUS &outStatus)
Provides status information about my output (playout) process.
double GetAudioSamplesPerSecond(const NTV2AudioRate inAudioRate)
Returns the audio sample rate as a number of audio samples per second.
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
@ NTV2_TestPatt_MultiPattern
Configures an NTV2Player instance.
virtual bool SetQuadQuadFrameEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables "quad-quad" 8K frame mode on the device.
virtual bool SetHDMIOutAudioSource8Channel(const NTV2Audio8ChannelSelect inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the HDMI output's 8-channel audio source.
NTV2PixelFormat fPixelFormat
The pixel format to use.
virtual bool SetQuadQuadSquaresEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables quad-quad-frame (8K) squares mode on the device.
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
static NTV2TestPatternNames getTestPatternNames(void)
@ NTV2_XptFrameBuffer3_DS2RGB
bool NTV2DeviceCanDoCustomAnc(const NTV2DeviceID inDeviceID)
double FramesToSeconds(int64_t frames) const
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
@ NTV2_TestPatt_LineSweep
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
Declares the AJAAncillaryData_HDR_SDR class.
virtual AJAStatus Start()
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
#define xHEX0N(__x__, __n__)
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
#define AJA_FAILURE(_status_)
@ NTV2_AUDIO_RATE_INVALID
static const double gFrequencies[]
bool NTV2DeviceCanDo2110(const NTV2DeviceID inDeviceID)
@ NTV2_TestPatt_LinearRamp
virtual bool SetHDMIOutAudioFormat(const NTV2AudioFormat inNewValue)
Sets the HDMI output's audio format.
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
NTV2Buffer acANCBuffer
The host ancillary data buffer. This field is owned by the client application, and thus is responsibl...
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
bool GetRP188Str(std::string &sRP188) const
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
NTV2StringList NTV2TestPatternNames
A list (std::vector) of pattern names.
@ AJAAncDataType_HDR_HDR10
bool Set(const void *pInUserPointer, const size_t inByteCount)
Sets (or resets) me from a client-supplied address and size.
@ NTV2_XptDualLinkOut1Input
@ NTV2_XptDuallinkOut3DS2
@ NTV2_AUDIOSYSTEM_INVALID
Declares the AJATimeBase class.