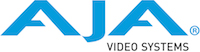 |
AJA NTV2 SDK
17.6.0.1688
NTV2 SDK 17.6.0.1688
|
Go to the documentation of this file.
19 #define TCFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
20 #define TCWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
21 #define TCNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
22 #define TCINFO(_expr_) AJA_sINFO (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
23 #define TCDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
57 while (mProducerThread.
Active())
60 while (mConsumerThread.
Active())
83 if (!mDevice.
features().CanDoPlayback())
85 if (!mDevice.
features().CanDoStreamingDMA())
88 const UWord maxNumChannels (mDevice.
features().GetNumFrameStores());
96 <<
" -- only supports Ch1" << (maxNumChannels > 1 ? string(
"-Ch") + string(1,
char(maxNumChannels+
'0')) :
"") << endl;
108 if (mDevice.
features().CanDoMultiFormat())
132 {cerr <<
"## ERROR: RenderTimeCodeFont failed for: " << mFormatDesc << endl;
return AJA_STATUS_UNSUPPORTED;}
136 cerr << mConfig << endl;
137 #endif // defined(_DEBUG)
149 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
154 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
163 {cerr <<
"## ERROR: No CSC for channel " <<
DEC(mConfig.
fOutputChannel+1) <<
" to convert RGB pixel format" << endl;
208 mHostBuffers.push_back(FrameData());
209 FrameData & frameData(mHostBuffers.back());
212 if (mConfig.WithVideo())
220 frameData.fDataReady =
false;
233 vector<NTV2TestPatternSelect> testPatIDs;
244 mTestPatRasters.clear();
245 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
250 if (mFormatDesc.IsVANC())
254 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
258 {
PLFAIL(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": "
265 if (!testPatternGen.
DrawTestPattern (testPatIDs.at(tpNdx), mFormatDesc, mTestPatRasters.at(tpNdx)))
267 cerr <<
"## ERROR: DrawTestPattern " <<
DEC(tpNdx) <<
" failed: " << mFormatDesc << endl;
280 const UWord numSDIOutputs (mDevice.
features().GetNumVideoOutputs());
282 const bool canVerify (mDevice.
features().HasCrosspointConnectROM());
283 UWord connectFailures (0);
289 if (!mDevice.
Connect (cscInputXpt, fsVidOutXpt, canVerify))
295 if (mDevice.
features().HasBiDirectionalSDI())
306 const UWord numFrameStores(mDevice.
features().GetNumFrameStores());
310 if (!mDevice.
Connect (cscInputXpt, fsVidOutXpt, canVerify))
317 if (mDevice.
features().HasBiDirectionalSDI())
327 if (mDevice.
features().GetNumAnalogVideoOutputs())
332 if (mDevice.
features().GetNumHDMIVideoOutputs())
337 PLWARN(
DEC(connectFailures) <<
" 'Connect' call(s) failed");
338 return connectFailures == 0;
362 mConsumerThread.
Start();
383 ULWord goodQueue(0), badQueue(0), goodRelease(0), badRelease(0), starves(0), noRoomWaits(0), status(0);
387 for (FrameDataArrayIter iterHost (mHostBuffers.begin()); iterHost != mHostBuffers.end(); ++iterHost)
388 if (iterHost->fVideoBuffer)
395 cerr <<
"## ERROR: Stream initialize failed: " << status << endl;
404 {cerr <<
"## ERROR: Stream status failed: " << status << endl;
return;}
408 FrameData * pFrameData (&mHostBuffers[mConsumerIndex]);
409 if (pFrameData->fDataReady)
421 cerr <<
"## ERROR: Stream buffer add failed: " << status << endl;
429 {cerr <<
"## ERROR: Stream stop failed: " << status << endl;
return;}
433 while (!strStatus.
IsIdle())
437 for (
int i = 0; i < 30; i++)
443 {cerr <<
"## ERROR: Stream start failed: " << status << endl;
return;}
477 {cerr <<
"## ERROR: Stream initialize failed: " << status << endl;
return;}
495 cerr <<
"## ERROR: Stream release failed: " << status << endl;
499 PLNOTE(
"Thread completed: " <<
DEC(goodQueue) <<
" queued, " <<
DEC(badQueue) <<
" failed, "
500 <<
DEC(starves) <<
" starves, " <<
DEC(noRoomWaits) <<
" waits, "
501 <<
DEC(goodRelease) <<
" releases (" <<
DEC(badRelease) <<
" failed)");
515 mProducerThread.
Start();
531 ULWord testPatNdx(0), badTally(0);
532 double timeOfLastSwitch (0.0);
545 FrameData * pFrameData (&mHostBuffers[mProducerIndex]);
546 if (pFrameData->fDataReady)
554 pFrameData->fVideoBuffer.CopyFrom (mTestPatRasters.at(testPatNdx),
557 pFrameData->fVideoBuffer.GetByteCount());
559 const CRP188 rp188Info (mCurrentFrame++, 0, 0, 10, tcFormat);
570 if (isPAL) tcF2.
fHi |=
BIT(27);
else tcF2.
fLo |=
BIT(27);
573 if (pFrameData->fVideoBuffer.GetHostAddress(0))
575 mTCBurner.
BurnTimeCode (pFrameData->fVideoBuffer.GetHostAddress(0), tcString.c_str(), 80);
577 TCDBG(
"F" <<
DEC0N(mCurrentFrame-1,6) <<
": " << tcF1 <<
": " << tcString);
581 if (currentTime > timeOfLastSwitch + 4.0)
583 testPatNdx = (testPatNdx + 1) %
ULWord(mTestPatRasters.size());
584 timeOfLastSwitch = currentTime;
585 PLINFO(
"F" <<
DEC0N(mCurrentFrame,6) <<
": " << tcString <<
"pattern='" << tpNames.at(testPatNdx) <<
"'");
589 pFrameData->fDataReady =
true;
593 PLNOTE(
"Thread completed: " <<
DEC(mCurrentFrame) <<
" frame(s) produced, " <<
DEC(badTally) <<
" failed");
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
virtual ULWord StreamBufferQueue(const NTV2Channel inChannel, NTV2Buffer &inBuffer, ULWord64 bufferCookie, NTV2StreamBuffer &status)
Queue a buffer to the stream. The bufferCookie is a user defined identifier of the buffer used by the...
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
bool CanDoVideoFormat(const NTV2VideoFormat inVF)
@ NTV2_OUTPUTDESTINATION_HDMI1
@ NTV2_TestPatt_CheckField
ULWord64 mBufferCookie
Buffer User cookie.
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
#define NTV2_IS_VALID_TASK_MODE(__m__)
@ NTV2_CHANNEL2
Specifies channel or FrameStore 2 (or the 2nd item).
@ AJA_ThreadPriority_High
static const bool BUFFER_PAGE_ALIGNED((!(0)))
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
@ NTV2_TestPatt_MultiBurst
bool GetRP188Reg(RP188_STRUCT &outRP188) const
#define NTV2_VIDEO_FORMAT_HAS_PROGRESSIVE_PICTURE(__f__)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
Describes a user-space buffer on the host computer. I have an address and a length,...
virtual AJAStatus SetUpHostBuffers(void)
Sets up my host video & audio buffers.
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
ULWord GetQueueDepth(void)
Gets the queue depth.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
Declares the NTV2TestPatternGen class.
virtual bool DisableChannel(const NTV2Channel inChannel)
Disables the given FrameStore.
@ NTV2_TestPatt_ColorBars100
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
#define DEC0N(__x__, __n__)
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
virtual ULWord StreamChannelRelease(const NTV2Channel inChannel)
Release a stream. Releases all buffers remaining in the queue.
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
I play out SD or HD test pattern (with timecode) to an output of an AJA device with or without audio ...
enum NTV2InputCrosspointID NTV2InputXptID
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
const char * NTV2FrameBufferFormatString(NTV2FrameBufferFormat fmt)
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
bool IsIdle(void)
Is the stream idle.
virtual void ConsumeFrames(void)
My consumer thread that repeatedly plays frames using AutoCirculate (until quit).
virtual void Quit(void)
Gracefully stops me from running.
NTV2FrameRate
Identifies a particular video frame rate.
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=(0), bool inRDMA=(0))
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
virtual class DeviceCapabilities & features(void)
virtual ~NTV2StreamPlayer(void)
virtual std::string GetDisplayName(void)
Answers with this device's display name.
ULWord fLo
| BG 4 | Secs10 | BG 3 | Secs 1 | BG 2 | Frms10 | BG 1 | Frms 1 |
virtual void StartConsumerThread(void)
Starts my consumer thread.
Declares the AJAProcess class.
virtual AJAStatus SetUpVideo(void)
Performs all video setup.
ULWord fHi
| BG 8 | Hrs 10 | BG 7 | Hrs 1 | BG 6 | Mins10 | BG 5 | Mins 1 |
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
NTV2Standard
Identifies a particular video standard.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual AJAStatus SetUpTestPatternBuffers(void)
Creates my test pattern buffers.
@ NTV2_TestPatt_FlatField
Declares the CNTV2DeviceScanner class.
virtual ULWord StreamChannelStart(const NTV2Channel inChannel, NTV2StreamChannel &status)
Start a stream. Put the stream is the active state to start processing queued buffers....
virtual ULWord StreamChannelStatus(const NTV2Channel inChannel, NTV2StreamChannel &status)
Get the current stream status.
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=(!(0)))
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
static size_t SetDefaultPageSize(void)
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread star...
NTV2VideoFormat fVideoFormat
The video format to use.
#define NTV2_IS_PAL_VIDEO_FORMAT(__f__)
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
NTV2Channel fOutputChannel
The device channel to use.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
virtual ULWord StreamBufferRelease(const NTV2Channel inChannel, NTV2StreamBuffer &status)
Remove the oldest buffer released by the streaming engine from the buffer queue.
bool IsVideoFormatA(const NTV2VideoFormat format)
@ NTV2_TestPatt_ColorBars75
virtual bool IsDeviceReady(const bool inCheckValid=(0))
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
std::vector< std::string > NTV2StringList
@ NTV2_INPUT_CROSSPOINT_INVALID
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
The NTV2 test pattern generator.
virtual AJAStatus Run(void)
Runs me.
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=(0))
Connects the given widget signal input (sink) to the given widget signal output (source).
virtual ULWord StreamChannelStop(const NTV2Channel inChannel, NTV2StreamChannel &status)
Stop a stream. Put the stream is the idle state. For frame based video, the stream will idle on the b...
virtual bool SetSDIOutputStandard(const UWord inOutputSpigot, const NTV2Standard inValue)
Sets the SDI output spigot's video standard.
virtual ULWord StreamChannelWait(const NTV2Channel inChannel, NTV2StreamChannel &status)
Wait for any stream event. Returns for any state or buffer change.
std::string fDeviceSpec
The AJA device to use.
#define GetFrameBufferOutputXptFromChannel
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the producer thread's static callback function that gets called when the producer thread star...
This struct replaces the old RP188_STRUCT.
bool IsRGBFormat(const NTV2FrameBufferFormat format)
static AJA_PixelFormat GetAJAPixelFormat(const NTV2PixelFormat inFormat)
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
#define NTV2_STREAM_STATUS_SUCCESS
Used in NTV2Stream success.
virtual NTV2DeviceID GetDeviceID(void)
virtual ULWord StreamChannelInitialize(const NTV2Channel inChannel)
Initialize a stream. Put the stream queue and hardware in a known good state ready for use....
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
@ NTV2_TestPatt_MultiPattern
Configures an NTV2Player instance.
bool fDoABConversion
If true, do level-A/B conversion; otherwise don't.
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
NTV2PixelFormat fPixelFormat
The pixel format to use.
enum NTV2OutputCrosspointID NTV2OutputXptID
virtual AJAStatus SetPriority(AJAThreadPriority priority)
static NTV2TestPatternNames getTestPatternNames(void)
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
double FramesToSeconds(int64_t frames) const
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
@ NTV2_TestPatt_LineSweep
virtual AJAStatus Start()
#define xHEX0N(__x__, __n__)
#define AJA_FAILURE(_status_)
virtual bool RouteOutputSignal(void)
Performs all widget/signal routing for playout.
NTV2StreamPlayer(const PlayerConfig &inConfig)
Constructs me using the given configuration settings.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
virtual void StartProducerThread(void)
Starts my producer thread.
virtual void GetStreamStatus(NTV2StreamChannel &outStatus)
Provides status information about my output (playout) process.
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
bool GetRP188Str(std::string &sRP188) const
@ NTV2_OEM_TASKS
2: OEM (recommended): device configured by client application(s) with some driver involvement.
@ NTV2_OUTPUTDESTINATION_ANALOG1
Declares the AJATimeBase class.
virtual void ProduceFrames(void)
My producer thread that repeatedly produces video frames.