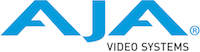 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
21 const string fileName,
22 const uint32_t frameWidth,
23 const uint32_t frameHeight,
34 mDeviceSpecifier (inDeviceSpecifier),
36 mFrameWidth (frameWidth),
37 mFrameHeight (frameHeight),
38 mInputChannel (inChannel),
48 mLastFrameInput (
false),
49 mLastFrameRaw (
false),
50 mLastFrameHevc (
false),
51 mLastFrameVideo (
false),
53 mVideoInputFrameCount (0),
54 mVideoProcessFrameCount (0),
55 mCodecRawFrameCount (0),
56 mCodecHevcFrameCount (0),
57 mVideoFileFrameCount (0)
59 ::memset (mFileInputBuffer, 0x0,
sizeof (mFileInputBuffer));
60 ::memset (mVideoRawBuffer, 0x0,
sizeof (mVideoRawBuffer));
61 ::memset (mVideoHevcBuffer, 0x0,
sizeof (mVideoHevcBuffer));
86 if (mFileInputBuffer[bufferNdx].pVideoBuffer)
91 if (mFileInputBuffer[bufferNdx].pInfoBuffer)
97 if (mVideoRawBuffer[bufferNdx].pVideoBuffer)
102 if (mVideoRawBuffer[bufferNdx].pInfoBuffer)
108 if (mVideoHevcBuffer[bufferNdx].pVideoBuffer)
113 if (mVideoHevcBuffer[bufferNdx].pInfoBuffer)
125 if (mM31 && !mLastFrame && !mGlobalQuit)
133 for (i = 0; i < timeout; i++)
135 if (mLastFrameVideo)
break;
139 { cerr <<
"## ERROR: Wait for last frame timeout" << endl; }
143 { cerr <<
"## ERROR: ChangeEHState ready to stop failed" << endl; }
146 { cerr <<
"## ERROR: ChangeEHState stop failed" << endl; }
150 { cerr <<
"## ERROR: ChangeVInState stop failed" << endl; }
156 { cerr <<
"## ERROR: ChangeMainState to init failed" << endl; }
163 while (mFileInputThread.
Active())
166 while (mVideoProcessThread.
Active())
169 while (mCodecRawThread.
Active())
172 while (mCodecHevcThread.
Active())
175 while (mVideoFileThread.
Active())
200 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return AJA_STATUS_OPEN; }
214 if (!mDevice.
features().HasHEVCM31())
216 cerr <<
"## ERROR: M31 not found" << endl;
221 mM31 =
new CNTV2m31 (&mDevice);
232 if (CNTV2m31::IsPresetVIF(mPreset))
236 mVideoFormat = CNTV2m31::GetPresetVideoFormat(mPreset);
237 mPixelFormat = CNTV2m31::GetPresetFrameBufferFormat(mPreset);
238 mQuad = CNTV2m31::IsPresetUHD(mPreset);
239 mInterlaced = CNTV2m31::IsPresetInterlaced(mPreset);
250 switch (mInputChannel)
261 status = mHevcCommon->
SetupHEVC (mM31, mPreset, mEncodeChannel, mMultiStream,
false);
272 cerr <<
"OpenYuv420File " << mFileName <<
" failed " << status << endl;
278 ostringstream fileName;
280 fileName <<
"raw_" << (mInputChannel+1) <<
".hevc";
282 fileName <<
"raw.hevc";
306 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
309 mFileInputBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
313 mFileInputBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
317 mFileInputCircularBuffer.
Add (& mFileInputBuffer[bufferNdx]);
322 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
325 mVideoRawBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
329 mVideoRawBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
333 mVideoRawCircularBuffer.
Add (& mVideoRawBuffer[bufferNdx]);
338 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
341 mVideoHevcBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
345 mVideoHevcBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mEncInfoBufferSize/4];
349 mVideoHevcCircularBuffer.
Add (& mVideoHevcBuffer[bufferNdx]);
374 mFileInputThread.
Start();
397 if (mVideoInputFrameCount < mHevcCommon->YuvNumFrames())
407 printf(
"Error reading frame %d\n", mVideoInputFrameCount);
414 if (mVideoInputFrameCount == mHevcCommon->
YuvNumFrames())
419 if(pVideoData->
lastFrame && !mLastFrameInput)
421 printf (
"Read last frame number %d\n", mVideoInputFrameCount );
422 mLastFrameInput =
true;
424 mVideoInputFrameCount++;
444 mVideoProcessThread.
Start();
475 mVideoProcessFrameCount++;
495 mCodecRawThread.
Start();
518 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
521 m31 =
new CNTV2m31 (&ntv2Device);
534 m31->RawTransfer(mPreset, mEncodeChannel,
539 m31->RawTransfer(mPreset, mEncodeChannel,
546 m31->RawTransfer(mEncodeChannel,
553 mLastFrameRaw =
true;
556 mCodecRawFrameCount++;
574 mCodecHevcThread.
Start();
597 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
600 m31 =
new CNTV2m31 (&ntv2Device);
613 uint8_t* pVideoBuffer = (uint8_t*)pFrameData->
pVideoBuffer;
614 uint8_t* pInfoBuffer = (uint8_t*)pFrameData->
pInfoBuffer;
619 m31->EncTransfer(mEncodeChannel,
646 m31->EncTransfer(mEncodeChannel,
669 m31->EncTransfer(mEncodeChannel,
692 mLastFrameHevc =
true;
695 mCodecHevcFrameCount++;
712 mVideoFileThread.
Start();
736 if (!mLastFrameVideo)
743 printf (
"Video file last frame number %d\n", mVideoFileFrameCount );
744 mLastFrameVideo =
true;
747 mVideoFileFrameCount++;
virtual void StartVideoFileThread(void)
Start the video file writer thread.
Declares the NTV2EncodeHEVCFile class.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
static void VideoFileThreadStatic(AJAThread *pThread, void *pContext)
This is the video file writer thread's static callback function that gets called when the thread star...
Declares device capability functions.
uint32_t videoDataSize2
Size of field 2 video data (bytes)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
void WriteHevcData(void *pBuffer, uint32_t bufferSize)
static void VideoInputThreadStatic(AJAThread *pThread, void *pContext)
This is the video input thread's static callback function that gets called when the thread starts....
virtual AJAStatus ProcessVideoFrame(AVHevcDataBuffer *pSrcFrame, AVHevcDataBuffer *pDstFrame)
Default do-nothing function for processing the captured frames.
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual void CodecRawWorker(void)
Repeatedly removes video frames from the raw video ring and transfers them to the codec.
bool lastFrame
Indicates last captured frame.
virtual ~NTV2EncodeHEVCFile()
virtual void GetStatus(AVHevcStatus &outStatus)
Provides status information about my input (capture) process.
Declares the AJATime class.
virtual AJAStatus Run(void)
Runs me.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
AJAStatus ConvertYuv420FrameToNV12(void *pSrcBuffer, void *pDstBuffer, uint32_t bufferSize)
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
virtual void StartCodecRawThread(void)
Start the codec raw thread.
uint32_t * pVideoBuffer
Pointer to host video buffer.
static void VideoProcessThreadStatic(AJAThread *pThread, void *pContext)
This is the video process thread's static callback function that gets called when the thread starts....
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
virtual void VideoFileWorker(void)
Repeatedly removes hevc frame from the hevc ring and writes them to the hevc output file.
virtual void CodecHevcWorker(void)
Repeatedly transfers hevc frames from the codec and adds them to the hevc ring.
uint32_t infoDataSize
Size of the information data (bytes)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
uint32_t * pInfoBuffer
Picture information (raw) or encode information (hevc)
uint32_t infoDataSize2
Size of the field 2 information data (bytes)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
virtual class DeviceCapabilities & features(void)
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
uint32_t videoDataSize
Size of video data (bytes)
Declares the AJAProcess class.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
void CloseYuv420File(void)
@ NTV2_MAX_NUM_VIDEO_FORMATS
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
Declares numerous NTV2 utility functions.
I interrogate and control an AJA video/audio capture/playout device.
static void CodecRawThreadStatic(AJAThread *pThread, void *pContext)
This is the codec raw thread's static callback function that gets called when the thread starts....
struct HevcPictureInfo HevcPictureInfo
ULWord GetVideoActiveSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
struct HevcEncodedInfo HevcEncodedInfo
@ NTV2_STANDARD_TASKS
1: Standard/Retail: Device is completely controlled by AJA ControlPanel, service/daemon,...
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual void StartVideoProcessThread(void)
Start the video process thread.
virtual void VideoProcessWorker(void)
Repeatedly removes video frames from the video input ring, calls a custom video process method and ad...
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
NTV2EncodeHEVCFile(const std::string inDeviceSpecifier="0", const NTV2Channel inChannel=NTV2_CHANNEL1, const std::string inFilePath="", const uint32_t inFrameWidth=0, const uint32_t inFrameHeight=0, const M31VideoPreset inM31Preset=M31_FILE_1920X1080_420_8_24p)
Constructs me using the given settings.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
@ Hevc_EhState_ReadyToStop
virtual NTV2DeviceID GetDeviceID(void)
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
AJAStatus CreateHevcFile(const std::string &inFileName, uint32_t maxFrames)
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
uint32_t videoBufferSize
Size of host video buffer (bytes)
virtual void StartVideoInputThread(void)
Start the video input thread.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
uint32_t infoBufferSize
Size of the host information buffer (bytes)
static void CodecHevcThreadStatic(AJAThread *pThread, void *pContext)
This is the codec hevc thread's static callback function that gets called when the thread starts....
virtual void Quit(void)
Gracefully stops me from running.
uint32_t AlignDataBuffer(void *pBuffer, uint32_t bufferSize, uint32_t dataSize, uint32_t alignBytes, uint8_t fill)
virtual AJAStatus Start()
virtual void VideoInputWorker(void)
Repeatedly captures video frames using AutoCirculate and add them to the video input ring.
This structure encapsulates the video and audio buffers used by the HEVC demo applications....
#define AJA_FAILURE(_status_)
AJAStatus ReadYuv420Frame(void *pBuffer, uint32_t numFrame)
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
AJAStatus OpenYuv420File(const std::string &inFileName, const uint32_t width, const uint32_t height)
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
virtual void StartCodecHevcThread(void)
Start the codec hevc thread.
AJAStatus SetupHEVC(CNTV2m31 *pM31, M31VideoPreset preset, M31Channel encodeChannel, bool multiStream, bool withInfo)