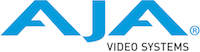 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
9 #ifndef _NTV2ENCODEHEVCFILE_H
10 #define _NTV2ENCODEHEVCFILE_H
28 #define VIDEO_RING_SIZE 60
30 #define RAWFILEPATH "ElephantsDream.yuv"
31 #define RAWFILEWIDTH 1920
32 #define RAWFILEHEIGHT 1080
33 #define M31PRESET M31_FILE_1920X1080_420_8_24p
59 const std::string inFilePath =
"",
60 const uint32_t inFrameWidth = 0,
61 const uint32_t inFrameHeight = 0,
80 virtual void Quit (
void);
215 const std::string mDeviceSpecifier;
216 const std::string mFileName;
217 uint32_t mFrameWidth;
218 uint32_t mFrameHeight;
231 bool mLastFrameInput;
234 bool mLastFrameVideo;
237 uint32_t mVideoBufferSize;
238 uint32_t mPicInfoBufferSize;
239 uint32_t mEncInfoBufferSize;
250 uint32_t mVideoInputFrameCount;
251 uint32_t mVideoProcessFrameCount;
252 uint32_t mCodecRawFrameCount;
253 uint32_t mCodecHevcFrameCount;
254 uint32_t mVideoFileFrameCount;
262 #endif // _NTV2ENCODEHEVCFILE_H
virtual void StartVideoFileThread(void)
Start the video file writer thread.
static void VideoFileThreadStatic(AJAThread *pThread, void *pContext)
This is the video file writer thread's static callback function that gets called when the thread star...
Declares device capability functions.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
static void VideoInputThreadStatic(AJAThread *pThread, void *pContext)
This is the video input thread's static callback function that gets called when the thread starts....
This file contains some structures, constants, classes and functions that are used in some of the hev...
virtual AJAStatus ProcessVideoFrame(AVHevcDataBuffer *pSrcFrame, AVHevcDataBuffer *pDstFrame)
Default do-nothing function for processing the captured frames.
virtual void CodecRawWorker(void)
Repeatedly removes video frames from the raw video ring and transfers them to the codec.
virtual ~NTV2EncodeHEVCFile()
virtual void GetStatus(AVHevcStatus &outStatus)
Provides status information about my input (capture) process.
virtual AJAStatus Run(void)
Runs me.
virtual void StartCodecRawThread(void)
Start the codec raw thread.
static void VideoProcessThreadStatic(AJAThread *pThread, void *pContext)
This is the video process thread's static callback function that gets called when the thread starts....
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
Declares the AJATimeCode class.
virtual void VideoFileWorker(void)
Repeatedly removes hevc frame from the hevc ring and writes them to the hevc output file.
virtual void CodecHevcWorker(void)
Repeatedly transfers hevc frames from the codec and adds them to the hevc ring.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
Declares the AJATimeCodeBurn class.
Declares the CNTV2DeviceScanner class.
Enumerations for controlling NTV2 devices.
I interrogate and control an AJA video/audio capture/playout device.
static void CodecRawThreadStatic(AJAThread *pThread, void *pContext)
This is the codec raw thread's static callback function that gets called when the thread starts....
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
virtual void StartVideoProcessThread(void)
Start the video process thread.
virtual void VideoProcessWorker(void)
Repeatedly removes video frames from the video input ring, calls a custom video process method and ad...
Declares the enumeration constants used in the ajabase library.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
Enumerations for controlling NTV2 devices with m31 HEVC encoders.
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
NTV2EncodeHEVCFile(const std::string inDeviceSpecifier="0", const NTV2Channel inChannel=NTV2_CHANNEL1, const std::string inFilePath="", const uint32_t inFrameWidth=0, const uint32_t inFrameHeight=0, const M31VideoPreset inM31Preset=M31_FILE_1920X1080_420_8_24p)
Constructs me using the given settings.
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
virtual void StartVideoInputThread(void)
Start the video input thread.
static void CodecHevcThreadStatic(AJAThread *pThread, void *pContext)
This is the codec hevc thread's static callback function that gets called when the thread starts....
virtual void Quit(void)
Gracefully stops me from running.
Declaration of AJACircularBuffer template class.
virtual void VideoInputWorker(void)
Repeatedly captures video frames using AutoCirculate and add them to the video input ring.
This structure encapsulates the video and audio buffers used by the HEVC demo applications....
Declares the AJAThread class.
virtual void StartCodecHevcThread(void)
Start the codec hevc thread.
Utility class for timecodes.
Declares the AJATimeBase class.