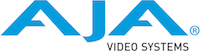 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
19 #define NTV2_AUDIOSIZE_MAX (401 * 1024)
21 #define NUM_OVERLAY_BARS 12
28 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000,
29 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000,
30 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000,
31 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000, 0x00000000 };
33 0x00000000, 0xc00000c0, 0x00000000, 0xc000c000, 0x00000000, 0xc0c00000,
34 0x00000000, 0xc0c000c0, 0x00000000, 0xc0c0c000, 0x00000000, 0xc000c0c0,
35 0x00000000, 0xc00000c0, 0x00000000, 0xc000c000, 0x00000000, 0xc0c00000,
36 0x00000000, 0xc0c000c0, 0x00000000, 0xc0c0c000, 0x00000000, 0xc000c0c0 };
47 const uint32_t inAudioChannels,
48 const bool inInfoData,
49 const uint32_t inMaxFrames)
57 mDeviceSpecifier (inDeviceSpecifier),
58 mWithAudio (inAudioChannels != 0),
68 mCodecPixelFormat (inPixelFormat),
69 mWithInfo (inInfoData),
72 mNumAudioChannels (0),
73 mFileAudioChannels (inAudioChannels),
74 mMaxFrames (inMaxFrames),
76 mLastFrameInput (
false),
77 mLastFrameHevc (
false),
78 mLastFrameVideo (
false),
82 mVideoInputFrameCount (0),
83 mCodecHevcFrameCount (0),
84 mAVFileFrameCount (0),
89 ::memset (mACInputBuffer, 0x0,
sizeof (mACInputBuffer));
90 ::memset (mVideoHevcBuffer, 0x0,
sizeof (mVideoHevcBuffer));
91 ::memset (&mOverlayBuffer, 0,
sizeof (mOverlayBuffer));
92 ::memset (&mOverlayFrame, 0,
sizeof (mOverlayFrame));
108 if (mACInputBuffer[bufferNdx].pVideoBuffer)
113 if (mACInputBuffer[bufferNdx].pInfoBuffer)
118 if (mACInputBuffer[bufferNdx].pAudioBuffer)
124 if (mVideoHevcBuffer[bufferNdx].pVideoBuffer)
129 if (mVideoHevcBuffer[bufferNdx].pInfoBuffer)
134 if (mVideoHevcBuffer[bufferNdx].pAudioBuffer)
143 delete [] mSilentBuffer;
148 delete [] mOverlayBuffer[0];
153 delete [] mOverlayBuffer[1];
162 if (mM31 && !mLastFrame && !mGlobalQuit)
169 { cerr <<
"## ERROR: ChangeEHState ready to stop failed" << endl; }
174 for (i = 0; i < timeout; i++)
176 if (mLastFrameVideo)
break;
180 { cerr <<
"## ERROR: Wait for last frame timeout" << endl; }
183 { cerr <<
"## ERROR: ChangeEHState stop failed" << endl; }
187 { cerr <<
"## ERROR: ChangeVInState stop failed" << endl; }
191 { cerr <<
"## ERROR: ChangeMainState to init failed" << endl; }
197 while (mACInputThread.
Active())
200 while (mCodecHevcThread.
Active())
203 while (mAVFileThread.
Active())
230 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return AJA_STATUS_OPEN; }
243 cerr <<
"## ERROR: M31 not found" << endl;
248 mM31 =
new CNTV2m31 (&mDevice);
260 if (!CNTV2m31::IsPresetVIF(mPreset))
264 mInputFormat = CNTV2m31::GetPresetVideoFormat(mPreset);
265 mCodecPixelFormat = CNTV2m31::GetPresetFrameBufferFormat(mPreset);
285 if(!CNTV2m31::ConvertVideoFormatToPreset(mInputFormat, mCodecPixelFormat,
true, mPreset))
290 switch (mInputFormat)
319 status = mHevcCommon->
SetupHEVC (mM31, mPreset, mEncodeChannel,
false, mWithInfo);
390 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
393 mACInputBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
397 mACInputBuffer[bufferNdx].
pAudioBuffer =
new uint32_t [mAudioBufferSize/4];
400 mACInputBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
404 mACInputCircularBuffer.
Add (& mACInputBuffer[bufferNdx]);
409 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
412 mVideoHevcBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
416 mVideoHevcBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mEncInfoBufferSize/4];
420 mVideoHevcCircularBuffer.
Add (& mVideoHevcBuffer[bufferNdx]);
424 mSilentBuffer =
new uint32_t [mAudioBufferSize/4];
425 memset(mSilentBuffer, 0, mAudioBufferSize);
428 mOverlayBuffer[0] =
new uint32_t [mOverlayBufferSize/4];
429 mOverlayBuffer[1] =
new uint32_t [mOverlayBufferSize/4];
431 uint32_t* buf0 = mOverlayBuffer[0];
432 uint32_t* buf1 = mOverlayBuffer[1];
433 uint32_t pixelsPerLine = overlayD.
linePitch*2;
438 for (uint32_t j = 0; j < linesPerBar; j++)
440 for (uint32_t k = 0; k < pixelsPerLine; k++)
473 mOverlayFrame[0] = 32;
474 mOverlayFrame[1] = 36;
475 mDevice.
DMAWriteFrame (mOverlayFrame[0], mOverlayBuffer[0], mOverlayBufferSize);
476 mDevice.
DMAWriteFrame (mOverlayFrame[1], mOverlayBuffer[1], mOverlayBufferSize);
501 for (
UWord i = 0; i < 4; i++)
617 cout << endl <<
"## WARNING: No video signal present on the input connector" << endl;
626 for (
int i = 0; i < 32; i++)
644 mACInputThread.
Start();
668 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
671 m31 =
new CNTV2m31 (&device);
713 if (pVideoData->
lastFrame && !mLastFrameInput)
715 printf (
"\nCapture last frame number %d\n", mVideoInputFrameCount );
716 mLastFrameInput =
true;
719 mVideoInputFrameCount++;
724 if ((mVideoInputFrameCount%60) == 0)
726 if (mOverlayIndex == 0)
762 mCodecHevcThread.
Start();
785 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
788 m31 =
new CNTV2m31 (&device);
799 m31->EncTransfer(mEncodeChannel,
820 if (mWithInfo && !mLastFrame)
829 mLastFrameHevc =
true;
831 mCodecHevcFrameCount++;
850 mAVFileThread.
Start();
881 if (!mLastFrameVideo)
895 printf (
"Video file last frame number %d\n", mAVFileFrameCount );
896 mLastFrameVideo =
true;
917 if (abs(mFrameData->
frameTime - encodeTime) < 50000)
925 if (mRawFrameCount == 0)
937 else if (mFrameData->
frameTime < encodeTime)
939 printf (
"Skip autocirculate raw audio/video frame - time diff %d us\n",
940 (int32_t)(mFrameData->
frameTime - encodeTime)/10);
947 printf (
"Add autocirculate raw audio/video frame - time diff %d us\n",
948 (int32_t)(mFrameData->
frameTime - encodeTime)/10);
954 printf (
"Add autocirculate raw audio/video frame - no input\n");
963 mHevcCommon->
WriteAiffData(mSilentBuffer, mNumAudioChannels, numSamples);
988 picData.
ptsValueLow = (uint32_t)(pts & 0xffffffff);
994 pM31->RawTransfer(mEncodeChannel,
void WriteAiffData(void *pBuffer, uint32_t numChannels, uint32_t numSamples)
virtual bool SetSDIInLevelBtoLevelAConversion(const NTV2ChannelSet &inSDIInputs, const bool inEnable)
Enables or disables 3G level B to 3G level A conversion at the SDI input(s).
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
@ NTV2_XptMixer4BGKeyInput
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
@ NTV2_XptMixer3FGVidInput
void WriteRawData(void *pBuffer, uint32_t bufferSize)
@ NTV2_FORMAT_4x1920x1080p_6000
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
static void CodecHevcThreadStatic(AJAThread *pThread, void *pContext)
This is the codec hevc thread's static callback function that gets called when the thread starts....
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
@ NTV2MIXERINPUTCONTROL_UNSHAPED
ULWord GetCapturedAudioByteCount(void) const
virtual bool SetQuadFrameEnable(const bool inValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Enables or disables quad-frame mode on the device.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
virtual bool Set4kSquaresEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 "2K quadrants" mode for the given FrameStore bank on the device....
@ NTV2_XptMixer2BGKeyInput
virtual bool SetEmbeddedAudioClock(const NTV2EmbeddedAudioClock inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2EmbeddedAudioClock setting for the given NTV2AudioSystem.
Declares device capability functions.
@ NTV2_XptMixer2FGKeyInput
virtual void StartAVFileThread(void)
Start the audio/video file writer thread.
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
uint32_t videoDataSize2
Size of field 2 video data (bytes)
virtual void RouteInputSignal(void)
Sets up device routing for capture.
uint32_t audioDataSize
Size of audio data (bytes)
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=false)
Applies the given routing table to the AJA device.
void WriteHevcData(void *pBuffer, uint32_t bufferSize)
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual bool SetMixerCoefficient(const UWord inWhichMixer, const ULWord inMixCoefficient)
Sets the current mix coefficient of the given mixer/keyer.
virtual bool SetMixerBGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the background input control value for the given mixer/keyer.
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
@ NTV2_XptMixer3BGKeyInput
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
bool lastFrame
Indicates last captured frame.
AJAStatus CreateRawFile(const std::string &inFileName, uint32_t maxFrames)
const FRAME_STAMP & GetFrameInfo(void) const
Returns a constant reference to my FRAME_STAMP.
Declares the AJATime class.
static const uint32_t sOverlayBar1[]
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
ULWord GetProcessedFrameCount(void) const
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
uint32_t * pVideoBuffer
Pointer to host video buffer.
virtual bool DisableChannel(const NTV2Channel inChannel)
Disables the given FrameStore.
void WriteEncData(void *pBuffer, uint32_t bufferSize)
ULWord GetBufferLevel(void) const
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
virtual bool SetOutputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the output frame index number for the given FrameStore. This identifies which frame in device SD...
@ NTV2_XptMixer3FGKeyInput
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
AJAStatus DetermineInputFormat(NTV2VideoFormat sdiFormat, bool quad, NTV2VideoFormat &videoFormat)
uint32_t infoDataSize
Size of the information data (bytes)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
@ NTV2_XptMixer4BGVidInput
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
uint32_t * pInfoBuffer
Picture information (raw) or encode information (hevc)
uint32_t infoDataSize2
Size of the field 2 information data (bytes)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
@ NTV2_XptMixer4FGVidInput
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
uint32_t videoDataSize
Size of video data (bytes)
void SetAJAFrameRate(AJA_FrameRate ajaFrameRate)
Declares the AJAProcess class.
@ NTV2_XptFrameBuffer1Input
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
NTV2EmbeddedAudioInput NTV2ChannelToEmbeddedAudioInput(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2EmbeddedAudioInput.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual ~NTV2EncodeHEVCVif()
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
@ NTV2_XptMixer1FGKeyInput
virtual void StartVideoInputThread(void)
Start the video input thread.
int64_t frameTime
Capture time stamp.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
@ NTV2_MAX_NUM_VIDEO_FORMATS
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
@ NTV2_EMBEDDED_AUDIO_CLOCK_VIDEO_INPUT
Audio clock derived from the video input.
Declares numerous NTV2 utility functions.
uint32_t * pAudioBuffer
Pointer to host audio buffer.
@ NTV2_XptMixer2FGVidInput
I interrogate and control an AJA video/audio capture/playout device.
@ NTV2MIXERMODE_FOREGROUND_ON
Passes only foreground video + key to the Mixer output.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
struct HevcPictureInfo HevcPictureInfo
ULWord GetVideoActiveSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
AJAStatus CreateEncFile(const std::string &inFileName, uint32_t maxFrames)
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
bool NTV2DeviceHasHEVCM31(const NTV2DeviceID inDeviceID)
@ NTV2_FORMAT_1080p_6000_A
struct HevcEncodedInfo HevcEncodedInfo
virtual AJAStatus Run(void)
Runs me.
This class is a collection of widget input-to-output connections that can be applied all-at-once to a...
@ NTV2_STANDARD_TASKS
1: Standard/Retail: Device is completely controlled by AJA ControlPanel, service/daemon,...
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual void AVFileWorker(void)
Repeatedly removes hevc frame from the hevc ring and writes them to the hevc output file.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
virtual bool SetMixerFGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the foreground input control value for the given mixer/keyer.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
struct HevcPictureData HevcPictureData
@ NTV2_Xpt4KDownConverterOut
virtual void Quit(void)
Gracefully stops me from running.
@ NTV2_XptFrameBuffer8RGB
@ NTV2_XptMixer1FGVidInput
@ NTV2_XptMixer4FGKeyInput
@ NTV2_XptFrameBuffer6RGB
@ NTV2_XptMixer1BGVidInput
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
@ NTV2_XptMixer3BGVidInput
bool IsRunning(void) const
@ NTV2_FORMAT_4x1920x1080p_5994
@ NTV2_MODE_CAPTURE
Capture (input) mode, which writes into device SDRAM.
static const uint32_t sOverlayBar0[]
@ NTV2MIXERINPUTCONTROL_FULLRASTER
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool SetMixerMode(const UWord inWhichMixer, const NTV2MixerKeyerMode inMode)
Sets the mode for the given mixer/keyer.
@ NTV2_XptFrameBuffer5RGB
virtual void CodecHevcWorker(void)
Repeatedly transfers hevc frames from the codec and adds them to the hevc ring.
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
@ NTV2_FORMAT_1080p_5000_A
virtual void SetupAutoCirculate(void)
Initializes AutoCirculate.
ULWord GetDroppedFrameCount(void) const
ULWord GetAudioSamplesPerFrame(const NTV2FrameRate inFrameRate, const NTV2AudioRate inAudioRate, ULWord inCadenceFrame=0, bool inIsSMPTE372Enabled=false)
Returns the number of audio samples for a given video frame rate, audio sample rate,...
int64_t FramesToMicroseconds(int64_t frames, bool round=false) const
static void AVFileThreadStatic(AJAThread *pThread, void *pContext)
This is the video file writer thread's static callback function that gets called when the thread star...
@ NTV2_XptMixer2BGVidInput
@ NTV2_FORMAT_4x1920x1080p_5000
virtual NTV2VideoFormat GetSDIInputVideoFormat(NTV2Channel inChannel, bool inIsProgressive=false)
Returns the video format of the signal that is present on the given SDI input source.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
AJAStatus CreateAiffFile(const std::string &inFileName, uint32_t numChannels, uint32_t maxFrames, uint32_t bufferSize)
@ Hevc_EhState_ReadyToStop
virtual void StartCodecHevcThread(void)
Start the codec hevc thread.
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual void GetStatus(AVHevcStatus *outInputStatus)
Provides status information about my input (capture) process.
virtual bool SetMixerVancOutputFromForeground(const UWord inWhichMixer, const bool inFromForegroundSource=true)
Sets the VANC source for the given mixer/keyer to the foreground video (or not). See the Ancillary Da...
@ NTV2_FORMAT_1080p_5994_A
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
NTV2EncodeHEVCVif(const std::string inDeviceSpecifier="0", const M31VideoPreset inM31Preset=M31_FILE_1280X720_420_8_5994p, const NTV2FrameBufferFormat inPixelFormat=NTV2_FBF_10BIT_YCBCR_420PL2, const uint32_t inAudioChannels=0, const bool inInfoData=(0), const uint32_t inMaxFrames=0xffffffff)
Constructs me using the given settings.
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=false)
Returns the video format of the signal that is present on the given input source.
AJAStatus CreateHevcFile(const std::string &inFileName, uint32_t maxFrames)
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
uint32_t videoBufferSize
Size of host video buffer (bytes)
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
virtual void VideoInputWorker(void)
Repeatedly captures video frames using AutoCirculate and add them to the video input ring.
uint32_t infoBufferSize
Size of the host information buffer (bytes)
#define NTV2_AUDIOSIZE_MAX
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
LWord64 acFrameTime
On exit, contains host OS clock at time of capture/play. On entry, contains NTV2Channel of interest,...
virtual void TransferPictureInfo(CNTV2m31 *pM31)
Transfer picture information to the codec.
uint32_t AlignDataBuffer(void *pBuffer, uint32_t bufferSize, uint32_t dataSize, uint32_t alignBytes, uint8_t fill)
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
virtual AJAStatus Start()
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=true)
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
AJA_FrameRate GetAJAFrameRate(NTV2FrameRate frameRate)
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
This structure encapsulates the video and audio buffers used by the HEVC demo applications....
#define AJA_FAILURE(_status_)
@ NTV2_XptMixer1BGKeyInput
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
virtual bool AddConnection(const NTV2InputXptID inSignalInput, const NTV2OutputXptID inSignalOutput=NTV2_XptBlack)
Adds a connection between a widget's signal input (sink) and another widget's signal output (source).
@ NTV2_XptFrameBuffer7RGB
static void VideoInputThreadStatic(AJAThread *pThread, void *pContext)
This is the video input thread's static callback function that gets called when the thread starts....
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
virtual bool GetSDIInput3GbPresent(bool &outValue, const NTV2Channel channel)
@ NTV2_FBF_ABGR
See 8-Bit ARGB, RGBA, ABGR Formats.
bool SetBuffers(ULWord *pInVideoBuffer, const ULWord inVideoByteCount, ULWord *pInAudioBuffer, const ULWord inAudioByteCount, ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
Declares the NTV2EncodeHEVCVif class.
uint32_t audioBufferSize
Size of host audio buffer (bytes)
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
AJAStatus SetupHEVC(CNTV2m31 *pM31, M31VideoPreset preset, M31Channel encodeChannel, bool multiStream, bool withInfo)
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID