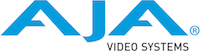 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
9 #ifndef _NTV2ENCODEHEVCVIF_H
10 #define _NTV2ENCODEHEVCVIF_H
29 #define VIDEO_RING_SIZE 60
30 #define AUDIO_RING_SIZE (3*VIDEO_RING_SIZE)
61 const uint32_t inAudioChannels = 0,
62 const bool inInfoData =
false,
63 const uint32_t inMaxFrames = 0xffffffff);
81 virtual void Quit (
void);
196 const std::string mDeviceSpecifier;
213 uint32_t mNumAudioChannels;
214 uint32_t mFileAudioChannels;
218 bool mLastFrameInput;
220 bool mLastFrameVideo;
223 uint32_t mVideoBufferSize;
224 uint32_t mPicInfoBufferSize;
225 uint32_t mEncInfoBufferSize;
226 uint32_t mAudioBufferSize;
227 uint32_t mOverlayBufferSize;
236 uint32_t * mSilentBuffer;
237 uint32_t mVideoInputFrameCount;
238 uint32_t mCodecHevcFrameCount;
239 uint32_t mAVFileFrameCount;
240 uint32_t mRawFrameCount;
241 uint32_t mInfoFrameCount;
243 uint32_t * mOverlayBuffer [2];
244 uint32_t mOverlayFrame [2];
245 uint32_t mOverlayIndex;
251 #endif // _NTV2ENCODEHEVCVIF_H
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
static void CodecHevcThreadStatic(AJAThread *pThread, void *pContext)
This is the codec hevc thread's static callback function that gets called when the thread starts....
@ NTV2_FBF_10BIT_YCBCR_420PL2
10-Bit 4:2:0 2-Plane YCbCr
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
Declares device capability functions.
virtual void StartAVFileThread(void)
Start the audio/video file writer thread.
virtual void RouteInputSignal(void)
Sets up device routing for capture.
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
This file contains some structures, constants, classes and functions that are used in some of the hev...
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
Declares the AJATimeCode class.
NTV2FrameRate
Identifies a particular video frame rate.
Declares the AJATimeCodeBurn class.
virtual ~NTV2EncodeHEVCVif()
Declares the CNTV2DeviceScanner class.
virtual void StartVideoInputThread(void)
Start the video input thread.
Enumerations for controlling NTV2 devices.
I interrogate and control an AJA video/audio capture/playout device.
@ M31_FILE_1280X720_420_8_5994p
virtual AJAStatus Run(void)
Runs me.
virtual void AVFileWorker(void)
Repeatedly removes hevc frame from the hevc ring and writes them to the hevc output file.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
NTV2InputSource
Identifies a specific video input source.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual void Quit(void)
Gracefully stops me from running.
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
Declares the enumeration constants used in the ajabase library.
virtual void CodecHevcWorker(void)
Repeatedly transfers hevc frames from the codec and adds them to the hevc ring.
virtual void SetupAutoCirculate(void)
Initializes AutoCirculate.
Enumerations for controlling NTV2 devices with m31 HEVC encoders.
static void AVFileThreadStatic(AJAThread *pThread, void *pContext)
This is the video file writer thread's static callback function that gets called when the thread star...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
virtual void StartCodecHevcThread(void)
Start the codec hevc thread.
virtual void GetStatus(AVHevcStatus *outInputStatus)
Provides status information about my input (capture) process.
NTV2EncodeHEVCVif(const std::string inDeviceSpecifier="0", const M31VideoPreset inM31Preset=M31_FILE_1280X720_420_8_5994p, const NTV2FrameBufferFormat inPixelFormat=NTV2_FBF_10BIT_YCBCR_420PL2, const uint32_t inAudioChannels=0, const bool inInfoData=(0), const uint32_t inMaxFrames=0xffffffff)
Constructs me using the given settings.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
virtual void VideoInputWorker(void)
Repeatedly captures video frames using AutoCirculate and add them to the video input ring.
virtual void TransferPictureInfo(CNTV2m31 *pM31)
Transfer picture information to the codec.
Declaration of AJACircularBuffer template class.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
This structure encapsulates the video and audio buffers used by the HEVC demo applications....
Declares the AJAThread class.
static void VideoInputThreadStatic(AJAThread *pThread, void *pContext)
This is the video input thread's static callback function that gets called when the thread starts....
Declares the AJATimeBase class.