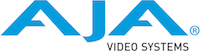 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
18 #if defined(NTV2_ANCSIZE_MAX)
19 #undef NTV2_ANCSIZE_MAX
21 #define NTV2_ANCSIZE_MAX (256 * 1024) // Super Size Me! 0x40000
26 #define AsULWordPtr(_p_) reinterpret_cast<ULWord*>(_p_)
27 #define AsCU8Ptr(_p_) reinterpret_cast<const uint8_t*>(_p_)
39 mAudioInLastAddress (0),
40 mAudioOutLastAddress (0),
73 while (mRunThread.
Active())
94 {cerr <<
"## ERROR: Device cannot both capture & play video" << endl;
return AJA_STATUS_BAD_PARAM; }
104 cerr <<
"## ERROR: Unable to acquire device because another app (pid " << appPID <<
") owns it" << endl;
130 if (mConfig.WithHanc())
138 {cerr <<
"## WARNING: Device doesn't support custom Anc, '-a -h' option ignored" << endl; mConfig.
fWithAnc =
false; mConfig.
fWithHanc =
false;}
172 {cerr <<
"## ERROR: This device cannot receive input from the specified source" << endl;
return AJA_STATUS_BAD_PARAM;}
205 bool isTransmit (
false);
216 cerr <<
"## WARNING: Non-SDI input source, no Anc capture possible" << endl;
222 cerr <<
"## ERROR: The specified input has no signal, or the device cannot handle the signal format" << endl;
235 cerr <<
"## WARNING: Non-SDI output destination, no Anc playout possible" << endl;
292 bool isBypassEnabled (
false);
375 if (!mpHostVideoBuffer || !mpHostAudioBuffer || (mConfig.WithAnc() && !mpHostF1AncBuffer) || (mConfig.WithAnc() && !mpHostF2AncBuffer))
377 cerr <<
"## ERROR: Unable to allocate host buffer(s)" << endl;
399 mDevice.
Connect (cscVideoInputXpt, inputOutputXpt);
400 mDevice.
Connect (fbInputXpt, cscOutputXpt);
403 mDevice.
Connect (fbInputXpt, inputOutputXpt);
414 mRP188Outputs.clear();
421 mDevice.
Connect (cscVidInputXpt, fbOutputXpt);
422 mDevice.
Connect (outputInputXpt, cscVidOutputXpt);
423 outputXpt = cscVidOutputXpt;
426 mDevice.
Connect (outputInputXpt, outputXpt);
440 mRP188Outputs.insert(chan);
510 uint32_t currentInFrame (0);
511 uint32_t currentOutFrame (2);
512 uint32_t currentAudioInAddress (0);
513 uint32_t audioReadOffset (0);
514 uint32_t audioInWrapAddress (0);
515 uint32_t audioOutWrapAddress (0);
516 uint32_t audioBytesCaptured (0);
517 uint32_t ancBytesCapturedF1 (0);
518 uint32_t ancBytesCapturedF2 (0);
519 bool audioIsReset (
true);
521 string timeCodeString;
532 ULWord savedF1ByteCapacity(0), savedF2ByteCapacity(0), offset(0);
542 if (mConfig.WithHanc())
592 mFramesProcessed = mFramesDropped = 0;
599 mDevice.
DMAWriteAnc (0, zeroesBuffer, zeroesBuffer);
600 mDevice.
DMAWriteAnc (1, zeroesBuffer, zeroesBuffer);
601 mDevice.
DMAWriteAnc (2, zeroesBuffer, zeroesBuffer);
602 mDevice.
DMAWriteAnc (3, zeroesBuffer, zeroesBuffer);
607 currentOutFrame ^= 1;
612 if (doAncInput && isInterlace)
622 mAudioInLastAddress = audioReadOffset;
623 audioInWrapAddress = audioOutWrapAddress + audioReadOffset;
624 mAudioOutLastAddress = 0;
627 currentOutFrame ^= 1;
632 if (doAncInput && isInterlace)
644 currentOutFrame ^= 1;
646 if (mConfig.WithAudio())
650 currentAudioInAddress &= ~0x3UL;
651 currentAudioInAddress += audioReadOffset;
653 if (audioIsReset && mAudioOutLastAddress)
657 audioIsReset =
false;
660 if (currentAudioInAddress < mAudioInLastAddress)
664 audioBytesCaptured = audioInWrapAddress - mAudioInLastAddress;
666 mDevice.
DMAReadAudio (mAudioSystem, mpHostAudioBuffer, mAudioInLastAddress, audioBytesCaptured);
670 audioReadOffset, currentAudioInAddress - audioReadOffset);
672 audioBytesCaptured += currentAudioInAddress - audioReadOffset;
676 audioBytesCaptured = currentAudioInAddress - mAudioInLastAddress;
679 mDevice.
DMAReadAudio (mAudioSystem, mpHostAudioBuffer, mAudioInLastAddress, audioBytesCaptured);
682 mAudioInLastAddress = currentAudioInAddress;
697 mpHostF1AncBuffer.
Fill(0);
699 mpHostF2AncBuffer.
Fill(0);
702 mDevice.
DMAReadAnc (currentInFrame, mpHostF1AncBuffer, mpHostF2AncBuffer);
710 mDevice.
DMAWriteAnc (currentInFrame, zeroesBuffer, zeroesBuffer);
719 CRP188 inputRP188Info (timecodeValue);
727 CRP188 analogRP188Info (timecodeValue);
736 const CRP188 frameRP188Info (mFramesProcessed, tcFormat);
746 if (mConfig.WithAudio())
749 if ((mAudioOutLastAddress + audioBytesCaptured) > audioOutWrapAddress)
752 mDevice.
DMAWriteAudio (mAudioSystem, mpHostAudioBuffer, mAudioOutLastAddress, audioOutWrapAddress - mAudioOutLastAddress);
756 0, audioBytesCaptured - (audioOutWrapAddress - mAudioOutLastAddress));
758 mAudioOutLastAddress = audioBytesCaptured - (audioOutWrapAddress - mAudioOutLastAddress);
763 mDevice.
DMAWriteAudio (mAudioSystem, mpHostAudioBuffer, mAudioOutLastAddress, audioBytesCaptured);
765 mAudioOutLastAddress += audioBytesCaptured;
785 +
ULWord(F1AncDataLoc.GetLineNumber())
788 pktData =
ULWord(pktData << 16) |
ULWord(pktData >> 16);
796 mDevice.
DMAWriteAnc (currentOutFrame, mpHostF1AncBuffer, mpHostF2AncBuffer);
806 uint32_t readBackOut;
810 if ((readBackIn == currentInFrame) || (readBackOut == currentOutFrame))
812 cerr <<
"## WARNING: Drop detected: current in " << currentInFrame <<
", readback in " << readBackIn
813 <<
", current out " << currentOutFrame <<
", readback out " << readBackOut << endl;
823 if (doAncInput && isInterlace)
829 if (doAncOutput && isInterlace)
833 if (mFramesProcessed == 1)
843 if (doAncInput && mConfig.WithHanc())
848 BURNNOTE(
"Thread completed, will exit");
858 outFramesProcessed = mFramesProcessed;
859 outFramesDropped = mFramesDropped;
866 switch (inInputSource)
889 if (regNum && mDevice.
ReadRegister(regNum, regValue) && regValue &
BIT(16))
904 default:
return false;
907 return regValue ?
true :
false;
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
bool NTV2DeviceCanDoCapture(const NTV2DeviceID inDeviceID)
virtual bool StopAudioInput(const NTV2AudioSystem inAudioSystem)
Stops the capture side of the given NTV2AudioSystem, and resets the capture position (i....
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
@ NTV2_INPUTSOURCE_SDI4
Identifies the 4th SDI video input.
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
#define NTV2_IS_SD_VIDEO_FORMAT(__f__)
Defines where the ancillary data can be found within a video stream.
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing and playing video.
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
@ NTV2_INPUTSOURCE_SDI6
Identifies the 6th SDI video input.
virtual AJAStatus SetSID(const uint8_t inSID)
Sets my Secondary Data ID (SID) - (aka the Data Block Number (DBN) for "Type 1" SMPTE-291 packets).
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
virtual bool AncInsertSetEnable(const UWord inSDIOutput, const bool inIsEnabled)
Enables or disables the given SDI output's Anc inserter frame buffer reads. (Call NTV2DeviceCanDoCust...
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
virtual bool StartAudioInput(const NTV2AudioSystem inAudioSystem, const bool inWaitForVBI=false)
Starts the capture side of the given NTV2AudioSystem, writing incoming audio samples into the Audio S...
ULWord GetFirstActiveLine(const NTV2FieldID inRasterFieldID=NTV2_FIELD0) const
virtual bool KickSDIWatchdog(void)
Restarts the countdown timer to prevent the watchdog timer from timing out.
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
virtual bool SetSDIWatchdogEnable(const bool inEnable, const UWord inIndex0)
Sets the connector pair relays to be under watchdog timer control or manual control.
virtual bool AncInsertInit(const UWord inSDIOutput, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Standard inStandard=NTV2_STANDARD_INVALID)
Initializes the given SDI output's Anc inserter for custom Anc packet insertion. (Call NTV2DeviceCanD...
virtual bool SetRP188Mode(const NTV2Channel inChannel, const NTV2_RP188Mode inMode)
Sets the current RP188 mode – NTV2_RP188_INPUT or NTV2_RP188_OUTPUT – for the given channel.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
Declares common types used in the ajabase library.
bool Is8BitFrameBufferFormat(const NTV2FrameBufferFormat fbFormat)
bool GetRP188Reg(RP188_STRUCT &outRP188) const
@ NTV2_INPUTSOURCE_SDI7
Identifies the 7th SDI video input.
#define NTV2_VIDEO_FORMAT_HAS_PROGRESSIVE_PICTURE(__f__)
static AJAStatus SetFromDeviceAncBuffers(const NTV2Buffer &inF1AncBuffer, const NTV2Buffer &inF2AncBuffer, AJAAncillaryList &outPackets, const uint32_t inFrameNum=0)
Returns all ancillary data packets found in the given F1 and F2 ancillary data buffers.
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
ULWord GetLastLine(const NTV2FieldID inRasterFieldID=NTV2_FIELD0) const
ULWord GetByteCount(void) const
#define NTV2_ASSERT(_expr_)
virtual bool StopAudioOutput(const NTV2AudioSystem inAudioSystem)
Stops the playout side of the given NTV2AudioSystem, parking the "Read Head" at the start of the play...
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool SetRP188Data(const NTV2Channel inSDIOutput, const NTV2_RP188 &inRP188Data)
Writes the raw RP188 data into the DBB/Low/Hi registers for the given SDI output. These values are la...
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
AJAAncDataLoc & SetLineNumber(const uint16_t inLineNum)
Sets my anc data line number value.
virtual bool ReadAnalogLTCInput(const UWord inLTCInput, RP188_STRUCT &outRP188Data)
Reads the current contents of the device's analog LTC input registers.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
Declares the AJAAncillaryList class.
virtual bool GetAudioWrapAddress(ULWord &outWrapAddress, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the wrap address, the threshold at which input/record or out...
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
Defines a number of handy byte-swapping macros.
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
bool fSuppressAudio
If true, suppress audio; otherwise include audio.
NTV2TCIndex fTimecodeSource
Timecode source to use.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
virtual bool DMAReadAnc(const ULWord inFrameNumber, NTV2Buffer &outAncF1Buffer, NTV2Buffer &outAncF2Buffer=NULL_POINTER, const NTV2Channel inChannel=NTV2_CHANNEL1)
Transfers the contents of the ancillary data buffer(s) from a given frame on the AJA device to the ho...
NTV2Channel NTV2OutputDestinationToChannel(const NTV2OutputDestination inOutputDest)
Converts a given NTV2OutputDestination to its equivalent NTV2Channel value.
NTV2EmbeddedAudioInput NTV2InputSourceToEmbeddedAudioInput(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2EmbeddedAudioInput value.
virtual bool AncSetFrameBufferSize(const ULWord inF1Size, const ULWord inF2Size)
Sets the capacity of the ANC buffers in device frame memory. (Call NTV2DeviceCanDoCustomAnc to determ...
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
NTV2InputXptID GetFrameBufferInputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsBInput=false)
bool NTV2DeviceCanDoDualLink(const NTV2DeviceID inDeviceID)
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
virtual bool GetInputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current input frame index number for the given FrameStore. This identifies which par...
Header file for the low latency NTV2Burn demonstration class.
@ NTV2_AncRgn_Field2
Identifies the "normal" Field 2 ancillary data region.
virtual bool SetOutputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the output frame index number for the given FrameStore. This identifies which frame in device SD...
virtual bool AncExtractSetFilterDIDs(const UWord inSDIInput, const NTV2DIDSet &inDIDs)
Replaces the DIDs to be excluded (filtered) by the given SDI input's Anc extractor....
virtual bool AncExtractGetFilterDIDs(const UWord inSDIInput, NTV2DIDSet &outDIDs)
Answers with the DIDs currently being excluded (filtered) by the SDI input's Anc extractor....
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
virtual AJAStatus SetDID(const uint8_t inDataID)
Sets my Data ID (DID).
NTV2ChannelSet::const_iterator NTV2ChannelSetConstIter
A handy const iterator into an NTV2ChannelSet.
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
bool NTV2DeviceCanDoFrameBufferFormat(const NTV2DeviceID inDeviceID, const NTV2FrameBufferFormat inFBFormat)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool GetSDITransmitEnable(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the specified SDI connector is currently acting as a transmitter (i....
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
@ NTV2_FIELD0
Identifies the first field in time for an interlaced video frame, or the first and only field in a pr...
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
I am an ordered collection of AJAAncillaryData instances which represent one or more SMPTE 291 data p...
virtual void ProcessFrames(void)
Repeatedly captures, burns, and plays frames without using AutoCirculate (until global quit flag set)...
NTV2FrameRate
Identifies a particular video frame rate.
@ NTV2_INPUTSOURCE_HDMI3
Identifies the 3rd HDMI video input.
virtual bool AncExtractSetField2WriteParams(const UWord inSDIInput, const ULWord inFrameNumber, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Framesize inFrameSize=NTV2_FRAMESIZE_INVALID)
Configures the given SDI input's Anc extractor to receive the next frame's F2 Anc data....
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
NTV2AudioSystem NTV2InputSourceToAudioSystem(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2AudioSystem value.
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
virtual bool EnableOutputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to output vertical blanking interrupts originat...
virtual bool GetAncRegionOffsetAndSize(ULWord &outByteOffset, ULWord &outByteCount, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All)
Answers with the offset and size of an ancillary data region within a device frame buffer.
virtual AJAStatus SetDataLocation(const AJAAncDataLoc &inLoc)
Sets my ancillary data "location" within the video stream.
virtual bool AncInsertSetComponents(const UWord inSDIOutput, const bool inVancY, const bool inVancC, const bool inHancY, const bool inHancC)
Enables or disables individual Anc insertion components for the given SDI output. (Call NTV2DeviceCan...
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
const uint32_t kAppSignature(((((uint32_t)( 'L'))<< 24)|(((uint32_t)( 'l'))<< 16)|(((uint32_t)( 'b'))<< 8)|(((uint32_t)( 'u'))<< 0)))
NTV2PixelFormat fPixelFormat
The pixel format to use.
virtual void StartRunThread(void)
Starts my main worker thread.
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
ULWord GetIndexForNTV2InputSource(const NTV2InputSource inValue)
virtual bool DMAWriteAudio(const NTV2AudioSystem inAudioSystem, const ULWord *pInAudioBuffer, const ULWord inOffsetBytes, const ULWord inByteCount)
Synchronously transfers audio data from the specified host buffer to the given Audio System's buffer ...
@ NTV2_INPUTSOURCE_ANALOG1
Identifies the first analog video input.
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
#define NTV2_IS_ANALOG_TIMECODE_INDEX(__x__)
NTV2OutputDestination NTV2ChannelToOutputDestination(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its ordinary equivalent NTV2OutputDestination.
static size_t DefaultPageSize(void)
static void RunThreadStatic(AJAThread *pThread, void *pContext)
This is the worker thread's static callback function that gets called when the thread runs....
virtual bool GetRP188Data(const NTV2Channel inSDIInput, NTV2_RP188 &outRP188Data)
Reads the raw RP188 data from the DBB/Low/Hi registers for the given SDI input. On newer devices with...
virtual bool SetRP188SourceFilter(const NTV2Channel inSDIInput, const UWord inFilterValue)
Sets the RP188 DBB filter for the given SDI input.
virtual bool AncExtractGetField1Size(const UWord inSDIInput, ULWord &outF1Size)
Answers with the number of bytes of field 1 ANC extracted. (Call NTV2DeviceCanDoCustomAnc to determin...
virtual bool AncExtractSetWriteParams(const UWord inSDIInput, const ULWord inFrameNumber, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Framesize inFrameSize=NTV2_FRAMESIZE_INVALID)
Configures the given SDI input's Anc extractor to receive the next frame's F1 Anc data....
std::set< UByte > NTV2DIDSet
A set of distinct NTV2DID values.
virtual bool GetOutputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current output frame number for the given FrameStore (expressed as an NTV2Channel).
virtual bool AncInsertSetField2ReadParams(const UWord inSDIOutput, const ULWord inFrameNumber, const ULWord inF2Size, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Framesize inFrameSize=NTV2_FRAMESIZE_INVALID)
Configures the Anc inserter for the next frame's F2 Anc data to embed/transmit. (Call NTV2DeviceCanDo...
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
static const bool REPLACE_OUTGOING_ANC_WITH_CUSTOM_PACKETS((0))
NTV2Standard
Identifies a particular video standard.
@ NTV2_AUDIOSYSTEM_2
This identifies the 2nd Audio System.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
@ NTV2_VANCDATA_8BITSHIFT_ENABLE
bool NTV2DeviceCanDoWidget(const NTV2DeviceID inDeviceID, const NTV2WidgetID inWidgetID)
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing and playing audio.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
virtual bool AncExtractGetField2Size(const UWord inSDIInput, ULWord &outF2Size)
Answers with the number of bytes of field 2 ANC extracted. (Call NTV2DeviceCanDoCustomAnc to determin...
virtual bool GetAudioReadOffset(ULWord &outReadOffset, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset from the start of the audio buffer to the fi...
@ NTV2_AncRgn_Field1
Identifies the "normal" Field 1 ancillary data region.
#define NTV2EndianSwap32(__val__)
@ AJAAncDataChannel_Y
The ancillary data is associated with the luminance (Y) channel of the video stream.
bool NTV2DeviceHasBiDirectionalSDI(const NTV2DeviceID inDeviceID)
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
static size_t SetDefaultPageSize(void)
virtual bool InputSignalHasTimecode(void)
Returns true if the current input signal has timecode embedded in it; otherwise returns false.
bool fWithHanc
If true, capture & play HANC data, including audio (LLBurn). Defaults to false.
@ NTV2_INPUTSOURCE_INVALID
The invalid video input.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
@ NTV2_INPUTSOURCE_SDI5
Identifies the 5th SDI video input.
virtual bool ReadAudioLastIn(ULWord &outValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
For the given Audio System, answers with the byte offset to the last byte of the latest chunk of 4-by...
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=false)
Connects the given widget signal input (sink) to the given widget signal output (source).
virtual bool SetLTCInputEnable(const bool inEnable)
Enables or disables the ability for the device to read analog LTC on the reference input connector.
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
virtual bool DMAWriteAnc(const ULWord inFrameNumber, NTV2Buffer &inAncF1Buffer, NTV2Buffer &inAncF2Buffer=NULL_POINTER, const NTV2Channel inChannel=NTV2_CHANNEL1)
Transfers the contents of the ancillary data buffer(s) from the host to a given frame on the AJA devi...
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
NTV2InputCrosspointID
Identifies a widget input that potentially can accept a signal emitted from another widget's output (...
virtual bool DMAReadFrame(const ULWord inFrameNumber, ULWord *pOutFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the AJA device to the host.
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
virtual AJAStatus Run(void)
Runs me.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
UWord NTV2DeviceGetNumFrameStores(const NTV2DeviceID inDeviceID)
virtual bool SetAudioOutputEmbedderState(const NTV2Channel inSDIOutputConnector, const bool &inEnable)
Enables or disables the audio output embedder for the given SDI output connector (specified as a chan...
virtual AJAStatus AddAncillaryData(const AJAAncillaryList &inPackets)
Appends a copy of the given list's packets to me.
virtual AJAStatus SetPayloadData(const uint8_t *pInData, const uint32_t inByteCount)
Copy data from external memory into my local payload memory.
virtual void RouteInputSignal(void)
Sets up board routing for capture.
virtual bool IsDeviceReady(const bool inCheckValid=(0))
@ NTV2_INPUTSOURCE_HDMI4
Identifies the 4th HDMI video input.
virtual bool DMAReadAudio(const NTV2AudioSystem inAudioSystem, ULWord *pOutAudioBuffer, const ULWord inOffsetBytes, const ULWord inByteCount)
Synchronously transfers audio data from a given Audio System's buffer memory on the AJA device to the...
bool NTV2DeviceCanDoPlayback(const NTV2DeviceID inDeviceID)
virtual std::ostream & Print(std::ostream &inOutStream, const bool inDetailed=true) const
Dumps a human-readable description of every packet in my list to the given output stream.
bool fWithAnc
If true, capture & play anc data (LLBurn). Defaults to false.
virtual uint32_t CountAncillaryData(void) const
Answers with the number of AJAAncillaryData objects I contain (any/all types).
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
static const bool CLEAR_DEVICE_ANC_BUFFER_AFTER_READ((0))
virtual void Quit(void)
Gracefully stops me from running.
#define NTV2_AUDIOSIZE_MAX
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual bool SetSDIWatchdogTimeout(const ULWord inValue)
Specifies the amount of time that must elapse before the watchdog timer times out.
#define NTV2_OUTPUT_DEST_IS_SDI(_dest_)
NTV2SmpteLineNumber GetSmpteLineNumber(const NTV2Standard inStandard)
For the given video standard, returns the SMPTE-designated line numbers for Field 1 and Field 2 that ...
@ NTV2_DISABLE_TASKS
0: Disabled: Device is completely configured by controlling application(s) – no driver involvement.
virtual AJAStatus SetDataCoding(const AJAAncDataCoding inCodingType)
Sets my ancillary data coding type (e.g. digital or analog/raw waveform).
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
NTV2Channel fInputChannel
The input channel to use.
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
@ NTV2_INPUTSOURCE_HDMI2
Identifies the 2nd HDMI video input.
NTV2OutputCrosspointID
Identifies a widget output, a signal source, that potentially can drive another widget's input (ident...
std::string fDeviceSpec
The AJA device to use.
void * GetHostPointer(void) const
NTV2InputSource
Identifies a specific video input source.
virtual bool AncInsertSetReadParams(const UWord inSDIOutput, const ULWord inFrameNumber, const ULWord inF1Size, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Framesize inFrameSize=NTV2_FRAMESIZE_INVALID)
Configures the Anc inserter for the next frame's F1 Anc data to embed/transmit. (Call NTV2DeviceCanDo...
NTV2InputSource fInputSource
The device input connector to use.
Used to describe Start of Active Video (SAV) location and field dominance for a given NTV2Standard....
bool NTV2DeviceHasSDIRelays(const NTV2DeviceID inDeviceID)
@ AJAAncDataStream_1
The ancillary data is associated with DS1 of the video stream (Link A).
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
@ NTV2_OUTPUTDESTINATION_INVALID
virtual bool AncExtractInit(const UWord inSDIInput, const NTV2Channel inChannel=NTV2_CHANNEL_INVALID, const NTV2Standard inStandard=NTV2_STANDARD_INVALID)
Initializes the given SDI input's Anc extractor for custom Anc packet detection and de-embedding....
@ NTV2_MODE_CAPTURE
Capture (input) mode, which writes into device SDRAM.
@ NTV2_INPUTSOURCE_SDI8
Identifies the 8th SDI video input.
bool NTV2DeviceCanDoInputSource(const NTV2DeviceID inDeviceID, const NTV2InputSource inInputSource)
virtual AJAStatus GetTransmitData(NTV2Buffer &F1Buffer, NTV2Buffer &F2Buffer, const bool inIsProgressive=true, const uint32_t inF2StartLine=0)
Encodes my AJAAncillaryData packets into the given buffers in the default SDI Anc Buffer Data Format ...
@ NTV2_INPUTSOURCE_SDI2
Identifies the 2nd SDI video input.
This struct replaces the old RP188_STRUCT.
@ NTV2_TCINDEX_LTC2
Analog LTC 2.
bool IsRGBFormat(const NTV2FrameBufferFormat format)
Captures video and audio from a signal provided to an input of an AJA device, burns timecode into the...
NTV2LLBurn(const BurnConfig &inConfig)
Constructs me using the given configuration settings.
static const bool PRINT_ANC_PACKETS_AFTER_READ((0))
static const bool CLEAR_HOST_ANC_BUFFER_BEFORE_READ((0))
ULWord NTV2DeviceGetNumVideoChannels(const NTV2DeviceID inDeviceID)
A handy class that makes it easy to "bounce" an unsigned integer value between a minimum and maximum ...
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
static bool GetWidgetForInput(const NTV2InputXptID inInputXpt, NTV2WidgetID &outWidgetID, const NTV2DeviceID inDeviceID=DEVICE_ID_NOTFOUND)
Returns the widget that "owns" the specified input crosspoint.
virtual NTV2DeviceID GetDeviceID(void)
virtual bool StartAudioOutput(const NTV2AudioSystem inAudioSystem, const bool inWaitForVBI=false)
Starts the playout side of the given NTV2AudioSystem, reading outgoing audio samples from the Audio S...
@ AJAAncDataCoding_Digital
The ancillary data is in the form of a SMPTE-291 Ancillary Packet.
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
NTV2OutputXptID GetInputSourceOutputXpt(const NTV2InputSource inInputSource, const bool inIsSDI_DS2=false, const bool inIsHDMI_RGB=false, const UWord inHDMI_Quadrant=0)
virtual void GetStatus(ULWord &outFramesProcessed, ULWord &outFramesDropped)
Provides status information about my input (capture) and output (playout) processes.
virtual bool SetInputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the input frame index number for the given FrameStore. This identifies which frame in device SDR...
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
virtual bool SetEnableVANCData(const bool inVANCenabled, const bool inTallerVANC, const NTV2Standard inStandard, const NTV2FrameGeometry inGeometry, const NTV2Channel inChannel=NTV2_CHANNEL1)
virtual void RouteOutputSignal(void)
Sets up board routing for playout.
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=false)
Returns the video format of the signal that is present on the given input source.
virtual bool IsRP188BypassEnabled(const NTV2Channel inSDIOutput, bool &outIsBypassEnabled)
Answers if the SDI output's RP-188 bypass mode is enabled or not.
virtual bool DisableRP188Bypass(const NTV2Channel inSDIOutput)
Configures the SDI output's embedder to embed SMPTE 12M timecode specified in calls to CNTV2Card::Set...
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
bool NTV2DeviceCanDoCustomAnc(const NTV2DeviceID inDeviceID)
@ AJAAncDataSpace_VANC
Ancillary data found between SAV and EAV (.
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
virtual bool WaitForInputFieldID(const NTV2FieldID inFieldID, const NTV2Channel inChannel=NTV2_CHANNEL1)
Efficiently sleeps the calling thread/process until the next input VBI for the given field and input ...
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
@ NTV2_INPUTSOURCE_SDI3
Identifies the 3rd SDI video input.
virtual AJAStatus Start()
NTV2AudioSource NTV2InputSourceToAudioSource(const NTV2InputSource inInputSource)
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=true)
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
#define AJA_FAILURE(_status_)
virtual bool AncExtractSetEnable(const UWord inSDIInput, const bool inIsEnabled)
Enables or disables the given SDI input's Anc extractor. (Call NTV2DeviceCanDoCustomAnc to determine ...
@ NTV2_TCINDEX_LTC1
Analog LTC 1.
#define NTV2_INPUT_SOURCE_IS_ANALOG(_inpSrc_)
Configures an NTV2Burn or NTV2FieldBurn instance.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
virtual bool SetAudioCaptureEnable(const NTV2AudioSystem inAudioSystem, const bool inEnable)
Enables or disables the writing of incoming audio into the given Audio System's capture buffer.
@ AJAAncDataLink_A
The ancillary data is associated with Link A of the video stream.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
virtual AJAStatus SetupHostBuffers(void)
Sets up my circular buffers.
static ULWord GetRP188DBBRegNumForInput(const NTV2InputSource inInputSource)
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
bool GetRP188Str(std::string &sRP188) const
NTV2Channel fOutputChannel
The output channel to use.
@ NTV2_FIELD1
Identifies the last (second) field in time for an interlaced video frame.
virtual bool AnalogLTCInputHasTimecode(void)
Returns true if there is a valid LTC signal on my device's primary analog LTC input port; otherwise r...
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
virtual bool AncExtractSetComponents(const UWord inSDIInput, const bool inVancY, const bool inVancC, const bool inHancY, const bool inHancC)
Enables or disables the Anc extraction components for the given SDI input. (Call NTV2DeviceCanDoCusto...