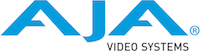 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
21 #define NTV2_BUFFER_LOCKING // Define this to pre-lock video/audio buffers in kernel
24 #define TCFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
25 #define TCWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
26 #define TCNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
27 #define TCINFO(_expr_) AJA_sINFO (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
28 #define TCDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
50 static const double gFrequencies [] = {250.0, 500.0, 1000.0, 2000.0};
52 static const double gAmplitudes [] = { 0.10, 0.15, 0.20, 0.25, 0.30, 0.35, 0.40, 0.45, 0.50, 0.55, 0.60, 0.65, 0.70, 0.75, 0.80, 0.85,
53 0.85, 0.80, 0.75, 0.70, 0.65, 0.60, 0.55, 0.50, 0.45, 0.40, 0.35, 0.30, 0.25, 0.20, 0.15, 0.10};
65 mToneFrequency (440.0),
91 while (mProducerThread.
Active())
94 while (mConsumerThread.
Active())
97 #if defined(NTV2_BUFFER_LOCKING)
99 #endif // NTV2_BUFFER_LOCKING
131 <<
" -- only supports Ch1" << (maxNumChannels > 1 ? string(
"-Ch") + string(1,
char(maxNumChannels+
'0')) :
"") << endl;
170 {cerr <<
"## ERROR: RenderTimeCodeFont failed for: " << mFormatDesc << endl;
return AJA_STATUS_UNSUPPORTED;}
174 cerr << mConfig << endl;
175 #endif // defined(_DEBUG)
187 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
192 { cerr <<
"## ERROR: '" << mDevice.
GetDisplayName() <<
"' doesn't support "
201 {cerr <<
"## ERROR: No CSC for channel " <<
DEC(mConfig.
fOutputChannel+1) <<
" to convert RGB pixel format" << endl;
234 {cerr <<
"## WARNING: HDR Anc requested, but device can't do custom anc" << endl;
257 numAudioChannels = 8;
309 if (mConfig.WithVideo())
322 if (mConfig.WithAudio())
333 mFrameDataRing.
Add (&frameData);
343 vector<NTV2TestPatternSelect> testPatIDs;
354 mTestPatRasters.clear();
355 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
360 if (mFormatDesc.IsVANC())
364 for (
size_t tpNdx(0); tpNdx < testPatIDs.size(); tpNdx++)
368 {
PLFAIL(
"Test pattern buffer " <<
DEC(tpNdx+1) <<
" of " <<
DEC(testPatIDs.size()) <<
": "
375 if (!testPatternGen.
DrawTestPattern (testPatIDs.at(tpNdx), mFormatDesc, mTestPatRasters.at(tpNdx)))
377 cerr <<
"## ERROR: DrawTestPattern " <<
DEC(tpNdx) <<
" failed: " << mFormatDesc << endl;
381 #ifdef NTV2_BUFFER_LOCKING
398 const bool canVerify (mDevice.HasCanConnectROM());
399 UWord connectFailures (0);
405 if (!mDevice.
Connect (cscInputXpt, fsVidOutXpt, canVerify))
426 if (!mDevice.
Connect (cscInputXpt, fsVidOutXpt, canVerify))
441 if (mConfig.WithAudio())
456 PLWARN(
DEC(connectFailures) <<
" 'Connect' call(s) failed");
457 return connectFailures == 0;
481 mConsumerThread.
Start();
502 ULWord goodQueue(0), badQueue(0), goodRelease(0), starves(0), noRoomWaits(0);
509 cerr <<
"## ERROR: Stream initialize failed: " << status << endl;
520 cerr <<
"## ERROR: Stream status failed: " << status << endl;
542 cerr <<
"## ERROR: Stream buffer add failed: " << status << endl;
551 cerr <<
"## ERROR: Stream initialize failed: " << status << endl;
584 cerr <<
"## ERROR: Stream initialize failed: " << status << endl;
601 cerr <<
"## ERROR: Stream release failed: " << status << endl;
605 PLNOTE(
"Thread completed: " <<
DEC(goodQueue) <<
" queued, " <<
DEC(badQueue) <<
" failed, "
606 <<
DEC(starves) <<
" starves, " <<
DEC(noRoomWaits) <<
" VBI waits");
620 mProducerThread.
Start();
636 ULWord testPatNdx(0), badTally(0);
659 testPatNdx = (testPatNdx + 1) %
ULWord(mTestPatRasters.size());
662 PLNOTE(
"Thread completed: " <<
DEC(mCurrentFrame) <<
" frame(s) produced, " <<
DEC(badTally) <<
" failed");
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
virtual ULWord StreamBufferQueue(const NTV2Channel inChannel, NTV2_POINTER inBuffer, ULWord64 bufferCookie, NTV2StreamBuffer &status)
Queue a buffer to the stream. The bufferCookie is a user defined identifier of the buffer used by the...
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
virtual bool GetAncRegionOffsetFromBottom(ULWord &outByteOffsetFromBottom, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All)
Answers with the byte offset to the start of an ancillary data region within a device frame buffer,...
@ NTV2_TestPatt_CheckField
Declares the AJAAncillaryData_HDR_HLG class.
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
#define NTV2_IS_VALID_TASK_MODE(__m__)
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
@ NTV2_TestPatt_MultiBurst
static const double gAmplitudes[]
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
#define IS_KNOWN_AJAAncDataType(_x_)
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
ULWord GetByteCount(void) const
virtual AJAStatus SetUpHostBuffers(void)
Sets up my host video & audio buffers.
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
ULWord GetQueueDepth(void)
Gets the queue depth.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
Declares the NTV2TestPatternGen class.
virtual bool DisableChannel(const NTV2Channel inChannel)
Disables the given FrameStore.
@ NTV2_TestPatt_ColorBars100
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
@ NTV2_AncRgn_Field2
Identifies the "normal" Field 2 ancillary data region.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
UWord NTV2DeviceGetNumCSCs(const NTV2DeviceID inDeviceID)
virtual ULWord StreamChannelRelease(const NTV2Channel inChannel)
Release a stream. Releases all buffers remaining in the queue.
#define NTV2_IS_2K_1080_VIDEO_FORMAT(__f__)
bool NTV2DeviceCanDoFrameStore1Display(const NTV2DeviceID inDeviceID)
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
I play out SD or HD test pattern (with timecode) to an output of an AJA device with or without audio ...
enum NTV2InputCrosspointID NTV2InputXptID
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
bool NTV2DeviceCanDoFrameBufferFormat(const NTV2DeviceID inDeviceID, const NTV2FrameBufferFormat inFBFormat)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
const char * NTV2FrameBufferFormatString(NTV2FrameBufferFormat fmt)
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
virtual void ConsumeFrames(void)
My consumer thread that repeatedly plays frames using AutoCirculate (until quit).
virtual void Quit(void)
Gracefully stops me from running.
bool NTV2DeviceCanDoVideoFormat(const NTV2DeviceID inDeviceID, const NTV2VideoFormat inVideoFormat)
AJAAncDataType fTransmitHDRType
Specifies the HDR anc data packet to transmit, if any.
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
virtual ~NTV2StreamPlayer(void)
virtual bool DMABufferUnlockAll()
Unlocks all previously-locked buffers used for DMA transfers.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
virtual void StartConsumerThread(void)
Starts my consumer thread.
Declares the AJAProcess class.
virtual AJAStatus SetUpVideo(void)
Performs all video setup.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
virtual AJAStatus SetUpAudio(void)
Performs all audio setup.
NTV2Standard
Identifies a particular video standard.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual AJAStatus SetUpTestPatternBuffers(void)
Creates my test pattern buffers.
@ NTV2_TestPatt_FlatField
NTV2Buffer fVideoBuffer
Host video buffer.
Declares the CNTV2DeviceScanner class.
virtual ULWord StreamChannelStart(const NTV2Channel inChannel, NTV2StreamChannel &status)
Start a stream. Put the stream is the active state to start processing queued buffers....
bool NTV2DeviceHasBiDirectionalSDI(const NTV2DeviceID inDeviceID)
virtual ULWord StreamChannelStatus(const NTV2Channel inChannel, NTV2StreamChannel &status)
Get the current stream status.
static size_t SetDefaultPageSize(void)
static const ULWord gNumFrequencies(sizeof(gFrequencies)/sizeof(double))
static const uint32_t gAudMaxSizeBytes(256 *1024)
The maximum number of bytes of 48KHz audio that can be transferred for a single frame....
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
UWord NTV2DeviceGetNumHDMIVideoOutputs(const NTV2DeviceID inDeviceID)
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread star...
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=false)
Connects the given widget signal input (sink) to the given widget signal output (source).
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
bool CopyFrom(const void *pInSrcBuffer, const ULWord inByteCount)
Replaces my contents from the given memory buffer, resizing me to the new byte count.
NTV2VideoFormat fVideoFormat
The video format to use.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
NTV2Channel fOutputChannel
The device channel to use.
bool NTV2DeviceCanDo3GLevelConversion(const NTV2DeviceID inDeviceID)
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
virtual ULWord StreamBufferRelease(const NTV2Channel inChannel, NTV2StreamBuffer &status)
Remove the oldest buffer released by the streaming engine from the buffer queue.
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=false, bool inRDMA=false)
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
UWord NTV2DeviceGetNumFrameStores(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumAnalogVideoOutputs(const NTV2DeviceID inDeviceID)
bool IsVideoFormatA(const NTV2VideoFormat format)
@ NTV2_TestPatt_ColorBars75
virtual bool IsDeviceReady(const bool inCheckValid=(0))
bool NTV2DeviceCanDoPlayback(const NTV2DeviceID inDeviceID)
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
std::vector< std::string > NTV2StringList
static ULWord gAncMaxSizeBytes(NTV2_ANCSIZE_MAX)
The maximum number of bytes of ancillary data that can be transferred for a single field....
@ NTV2_INPUT_CROSSPOINT_INVALID
virtual bool SetHDMIOutAudioRate(const NTV2AudioRate inNewValue)
Sets the HDMI output's audio rate.
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
The NTV2 test pattern generator.
virtual AJAStatus Run(void)
Runs me.
NTV2Buffer fAudioBuffer
Host audio buffer.
virtual bool SetSDIOutputStandard(const UWord inOutputSpigot, const NTV2Standard inValue)
Sets the SDI output spigot's video standard.
virtual ULWord StreamChannelWait(const NTV2Channel inChannel, NTV2StreamChannel &status)
Wait for any stream event. Returns for any state or buffer change.
@ NTV2_OUTPUTDESTINATION_HDMI
Declares the AJAAncillaryData_HDR_HDR10 class.
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
std::string fDeviceSpec
The AJA device to use.
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the producer thread's static callback function that gets called when the producer thread star...
bool IsRGBFormat(const NTV2FrameBufferFormat format)
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual bool SetSDIOutputDS2AudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the Audio System that will supply audio for the given SDI output's audio embedder for Data Strea...
@ NTV2_AudioChannel1_8
This selects audio channels 1 thru 8.
#define NTV2_STREAM_STATUS_SUCCESS
Used in NTV2Stream success.
virtual NTV2DeviceID GetDeviceID(void)
virtual ULWord StreamChannelInitialize(const NTV2Channel inChannel)
Initialize a stream. Put the stream queue and hardware in a known good state ready for use....
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
static const double gFrequencies[]
@ NTV2_TestPatt_MultiPattern
Configures an NTV2Player instance.
bool fDoABConversion
If true, do level-A/B conversion; otherwise don't.
virtual bool SetHDMIOutAudioSource8Channel(const NTV2Audio8ChannelSelect inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the HDMI output's 8-channel audio source.
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
NTV2PixelFormat fPixelFormat
The pixel format to use.
enum NTV2OutputCrosspointID NTV2OutputXptID
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
static NTV2TestPatternNames getTestPatternNames(void)
bool NTV2DeviceCanDoCustomAnc(const NTV2DeviceID inDeviceID)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
@ NTV2_TestPatt_LineSweep
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
Declares the AJAAncillaryData_HDR_SDR class.
virtual AJAStatus Start()
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=true)
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
#define xHEX0N(__x__, __n__)
#define AJA_FAILURE(_status_)
virtual bool RouteOutputSignal(void)
Performs all widget/signal routing for playout.
NTV2StreamPlayer(const PlayerConfig &inConfig)
Constructs me using the given configuration settings.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
bool NTV2DeviceCanDo2110(const NTV2DeviceID inDeviceID)
virtual bool SetHDMIOutAudioFormat(const NTV2AudioFormat inNewValue)
Sets the HDMI output's audio format.
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
@ NTV2_OUTPUTDESTINATION_ANALOG
static const bool BUFFER_PAGE_ALIGNED(true)
virtual void StartProducerThread(void)
Starts my producer thread.
virtual void GetStreamStatus(NTV2StreamChannel &outStatus)
Provides status information about my output (playout) process.
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID
Declares the AJATimeBase class.
virtual void ProduceFrames(void)
My producer thread that repeatedly produces video frames.