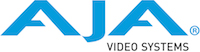 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
35 if (inSerialPortIndexNum > 0)
120 SByte timecodeString [12];
128 UByte hours = (hoursBCD>>4)*10 + (hoursBCD&0xF);
129 UByte minutes = (minutesBCD>>4)*10 + (minutesBCD&0xF);
130 UByte seconds = (secondsBCD>>4)*10 + (secondsBCD&0xF);
131 UByte frames = (framesBCD>>4)*10 + (framesBCD&0xF);
156 SByte timecodeString[12];
163 UByte hours = (hoursBCD>>4)*10 + (hoursBCD&0xF);
164 UByte minutes = (minutesBCD>>4)*10 + (minutesBCD&0xF);
165 UByte seconds = (secondsBCD>>4)*10 + (secondsBCD&0xF);
166 UByte frames = (framesBCD>>4)*10 + (framesBCD&0xF);
173 if ( seconds == 255 )
177 if ( minutes == 255 )
195 static char bcd [] = {
'0',
'1',
'2',
'3',
'4',
'5',
'6',
'7',
'8',
'9',
'0',
'0',
'0',
'0',
'0',
'0'};
207 timecodeString [0] =
bcd[hours>>4];
208 timecodeString [1] =
bcd[hours&0xF];
209 timecodeString [2] =
':';
210 timecodeString [3] =
bcd[minutes>>4];
211 timecodeString [4] =
bcd[minutes&0xF];
212 timecodeString [5] =
':';
213 timecodeString [6] =
bcd[seconds>>4];
214 timecodeString [7] =
bcd[seconds&0xF];
215 timecodeString [8] =
':';
216 timecodeString [9] =
bcd[frames>>4];
217 timecodeString [10] =
bcd[frames&0xF];
218 timecodeString [11] =
'\0';
231 UByte cueUpWithDataCommand [] = {0x6, 0x24, 0x31, 0x00, 0x00, 0x00, 0x00};
233 cueUpWithDataCommand [6] = ((hours / 10) << 4) + (hours % 10);
234 cueUpWithDataCommand [5] = ((minutes / 10) << 4) + (minutes % 10);
235 cueUpWithDataCommand [4] = ((seconds / 10) << 4) + (seconds % 10);
236 cueUpWithDataCommand [3] = ((frames / 10) << 4) + (frames % 10);
254 UByte commandLength = txBuffer [0];
259 if (commandLength == 0 || commandLength > 14)
266 for (
UWord count = 1; count <= commandLength; count++)
268 UByte value = txBuffer [count];
275 for (i = 0; i < 3; i++)
281 if ((val &
BIT_1) != 0)
289 for (i = 0; i < 3; i++)
303 for (i = 0; i < 1000; i++)
306 if ((val &
BIT_4) == 0)
349 for (
UWord count = 0; count < length; count++)
369 while (val &
BIT_4 && numRead < maxLength)
373 rxBuffer [numRead++] = data & 0xFF;
376 actualLength = numRead;
virtual bool GotACK(void)
virtual ULWord GetTimecodeString(SByte *timecodeString)
static UByte stopCommand[]
Declares device capability functions.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
static UByte playCommand[]
virtual ULWord FastForward(void)
Declares the AJATime class.
#define NTV2_SERIAL_RESPONSE_SIZE
SerialMachineResponse _serialMachineResponse
virtual ULWord ReversePlay(void)
virtual bool WaitForInterrupt(const INTERRUPT_ENUMS type, const ULWord timeout=50)
UWord NTV2DeviceGetNumSerialPorts(const NTV2DeviceID inDeviceID)
static UByte ltcTimeDataCommand[]
virtual ULWord Play(void)
static UByte fastForwardCommand[]
virtual bool EnableInterrupt(const INTERRUPT_ENUMS inEventCode)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
virtual bool ReadRxBuffer(UByte *rxBuffer, UWord &actualLength, UWord maxlength)
virtual ~CNTV2SerialControl()
virtual bool SubscribeEvent(const INTERRUPT_ENUMS inEventCode)
Causes me to be notified when the given event/interrupt is triggered for the AJA device.
Declares the CNTV2DeviceScanner class.
virtual const SerialMachineResponse & GetLastResponse(void) const
virtual ULWord Stop(void)
virtual bool WriteCommand(const UByte *txBuffer, bool response=(!(0)))
static UByte rewindCommand[]
virtual ULWord AdvanceFrame(void)
virtual bool WriteTxBuffer(const UByte *txBuffer, UWord length)
RegisterNum _controlRegisterNum
Which UART control register to use: kRegRS422Control (72) or kRegRS4222Control (246)
CNTV2SerialControl(const UWord inDeviceIndex=0, const UWord inSerialPortIndexNum=0)
static bool GetDeviceAtIndex(const ULWord inDeviceIndexNumber, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device having the given zero-bas...
RegisterNum _transmitRegisterNum
Which UART transmit data register to use: kRegRS422Transmit (70) or kRegRS4222Transmit (244)
virtual bool WaitForRxInterrupt(void)
virtual bool WaitForTxInterrupt(void)
static UByte reversePlayCommand[]
virtual NTV2DeviceID GetDeviceID(void)
virtual ULWord Rewind(void)
virtual ULWord GotoFrameByHMS(UByte inHrs, UByte inMins, UByte inSecs, UByte inFrames)
virtual bool DisableInterrupt(const INTERRUPT_ENUMS inEventCode)
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
RegisterNum _receiveRegisterNum
Which UART receive data register to use: kRegRS422Receive (71) or kRegRS4222Receive (245)
virtual bool Close(void)
Closes me, releasing host resources that may have been allocated in a previous Open call.
virtual ULWord BackFrame(void)