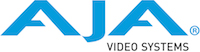 |
AJA NTV2 SDK
17.6.0.1688
NTV2 SDK 17.6.0.1688
|
Go to the documentation of this file.
8 #ifndef _BASEMACHINECONTROL_H
9 #define _BASEMACHINECONTROL_H
33 CONTROL_UNIMPLEMENTED = 0xFFFFFFFF
34 } ControUnimplemented;
41 virtual bool Open (
void) = 0;
42 virtual void Close (
void) = 0;
45 virtual inline ULWord Play (
void) {
return CONTROL_UNIMPLEMENTED;}
47 virtual inline ULWord Stop (
void) {
return CONTROL_UNIMPLEMENTED;}
49 virtual inline ULWord Rewind (
void) {
return CONTROL_UNIMPLEMENTED;}
57 virtual inline ULWord Loop (
ULWord inStartFrameNumber,
ULWord inEndFrameNumber) {(
void) inStartFrameNumber; (
void) inEndFrameNumber;
return CONTROL_UNIMPLEMENTED;}
65 #endif // _BASEMACHINECONTROL_H
Defines the import/export macros for producing DLLs or LIBs.
virtual ULWord Rewind(void)
virtual ULWord GotoFrameByHMS(UByte inHrs, UByte inMins, UByte inSecs, UByte inFrames)
A base class for deck control.
virtual ~CBaseMachineControl()
virtual ULWord Play(void)
virtual ULWord RecordAtFrame(ULWord inFrameNumber)
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
virtual ULWord FastForward(void)
virtual ULWord GotoFrameByString(SByte *pInFrameString)
Enumerations for controlling NTV2 devices.
virtual ULWord BackFrame(void)
virtual ULWord GetTimecodeString(SByte *pOutTimecodeString)
virtual ULWord AdvanceFrame(void)
virtual ULWord Stop(void)
virtual ControlType GetControlType(void) const
virtual ULWord GotoFrame(ULWord inFrameNumber)
virtual ULWord Loop(ULWord inStartFrameNumber, ULWord inEndFrameNumber)
virtual ULWord ReversePlay(void)